This problem statement contains images, subscripts and superscripts that may appear incorrectly or not appear at all in some plugins. In that case, use the standard view in the arena to see it correctly.
In a simplified version of basketball the goal is to score by getting the ball in a special scoring place. There are two teams, and each team contains the same number of players. When a player has possession of the ball, he has two choices: take a shot, or pass the ball to a teammate.
When taking a shot, the player throws the ball in a straight line to the scoring place. When passing the ball, he throws the ball in a straight line to the target teammate. In both cases, at most one of the rival players will try to intercept the shot or pass.
The probability of a pass being successful is:
Cp * (1 - (ls / 150)2) * dr / (dr + 1)
And the probability of a shot being successful (score) is:
(Cs * dr / (dr + 1))ln(ls)
Where Cp and Cs are constants defined for the problem instance, ls is the length of the shot or pass, dr is the distance between the intercepting rival and the ball trajectory and ln is the natural logarithm (logarithm in base e).
When trying to intercept a shot or a pass, only the best suitable player of the other team to do so (i.e., the one that produces the lowest dr) will try. If no player on the other team can do it, the factor dr/(dr+1) in the formula is considered to have a value of 1 (i.e., it is ignored). A player of the rival team is only allowed to try to intercept the ball if the line that passes through him and is perpendicular to the ball trajectory intersects the trajectory at some point between the two endpoints of the trajectory, inclusive.
For example, in this picture:
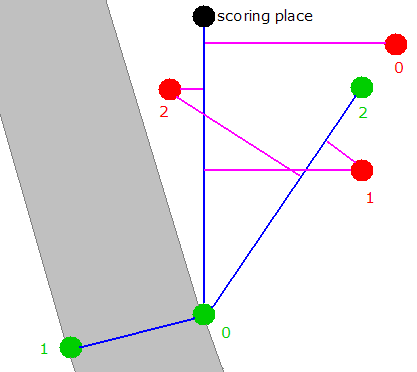
There are 3 players in each team, green players are your team and red players are rivals. Player 0 has the ball and has 3 options marked as blue lines, 2 passes and taking a shot. The shot, if taken, can be intercepted by any of the rivals, but only number 2 will try because he is clearly the nearest. The pass to player 1 is impossible to intercept for the rivals, because any player that can intercept that pass should be inside the gray area. The pass to player 2 can be intercepted by rivals 1 or 2. Rival player 0 is not on an intersecting perpendicular line, so he cannot try to intercept it. In this last case, rival 1 will try to intercept because he is nearer than rival 2.
You will be given two String[]s team and rivals with the same number of elements representing the members of each team. Each element of team and rivals will be in the format "X Y" where X and Y will be positive integers with no leading zeroes representing the x and y coordinates of that player in the field. You will also be given Cp and Cs, the constants for the probability calculations of each type of movement. When the game starts, the ball is in possession of the player on your team with index 0. The scoring place is at X=50, Y=0. Your team is only allowed to take one shot, and you are to determine and return the probability that your team will score if it follows the best strategy. A strategy consists of zero or more passes followed by a shot. If your team loses the ball at any point during the strategy, you will not score. See examples for further clarification.
|