Problem Statement |
| You have just been hired by a cartography company, and your first task is to help them with a map they are trying to make. The owner of the company thinks it should always be possible to color a map using only four colors (such that no touching states have the same color), but so far they have been unable to do so with the map of Familyland. This is quite important to him, as he can print maps using four colors of ink for only $0.00001 per square millimeter. Each additional ink, however, has a base cost of $N/100 and a usage cost of $N/100000 per square millimeter (where N is the number of the ink, so N=5 is the first ink that is more expensive).
The company will be printing 100mm x 100mm maps of Familyland, so you can see that the costs will add up quickly if many additional inks are needed. If the map can be made with only 4 inks, it will cost only $0.10 each to make, but if a 5th ink were needed, even on only 1 square millimeter of the map, the cost would rise to $0.15004 (note that you still only pay $0.00001/mm^2 for the first four inks).
The problem with this particular map is that the country of Familyland has a representative government, but the citizens would not put up with anyone but a family member being their representative. To deal with this, Familyland declared each family to be its own state. Since not all family members live next to one another, the states are not contiguous. Of course, in making the map, each state must be entirely one color.
If you want to keep your new job, you will need to come up with a coloring of the map such that it can be printed as cheaply as possible. Your score on each test case will be the cost in dollars of printing the map using the coloring you return. Your total score (the one displayed in the division summary) will be the sum over all testcases of the lowest cost anyone has achieved for the case divided by your cost for the case. In the unlikely event of a true tie (as computed using high precision numerics), the competitor with the lowest total runtime on all cases will be declared the winner.
You will be given the area of each state (in square millimeters in map scale), and an adjacency matrix where character i of string j is '1' if states i and j are adjacent and '0' if they are not. Character i of string i will always be '0'. You must return an ink color for each state such that no two adjacent states have the same color. In the event that your returned coloring is invalid (you return a different number of colors than there are states, or you color two adjacent states the same), or your program exceeds the 30 second time limit, you will receive a 0 on the case.
The test cases will be generated as follows (unless otherwise stated, all random choices are uniform and independent and ranges are inclusive):
- The number of states (S) will be randomly chosen between 10 and 500.
- The number of properties will be randomly chosen between S and 4*S.
- For the first S properties, a random x and y will be chosen between 0 and 99 (assuring that no two properties are at the same location). The pixel at the x and y coordinate on the 100x100 map is marked as belonging to the ith state.
- Each remaining property is placed on the map in the same manner, except that a random state between 0 and S-1 is assigned to the x and y coordinate on the map.
- The remaining map pixels are assigned to states by randomly picking an unassigned map pixel that borders an assigned pixel, randomly choosing one of the assigned pixels that borders it, and assigning that pixel's state to the unassigned pixel. This process continues until the map is filled.
- The adjacency matrix and the array of state areas are constructed from this map.
|
|
Definition |
| Class: | MapMaker | Method: | colorStates | Parameters: | int[], String[] | Returns: | int[] | Method signature: | int[] colorStates(int[] area, String[] adj) | (be sure your method is public) |
|
|
|
|
Notes |
- | The time limit for each test case is 30 seconds. |
- | There are 50 non-example test cases. |
- | The memory limit is 1GB, and the thread limit is 32. |
- | There will be between 10 and 500 states inclusive. (chosen uniformly) |
- | Returned colors must all be >= 1. |
- | To facilitate testing, the values of S and P were not chosen randomly for example cases 0 through 3. |
|
Examples |
0) | |
| | Returns:
"</pre>10 states, 10 properties<br>
<br>
Areas:<br>
1477, 538, 1429, 1106, 381, 685, 1605, 224, 1807, 748 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>0000011010
0000000111
0000101000
0000011000
0010001101
1001001000
1011110011
0100100001
1100001001
0100101110
</pre>" |
Here is an image of the generated map that was used to construct the adjacency matrix and calculate the areas:
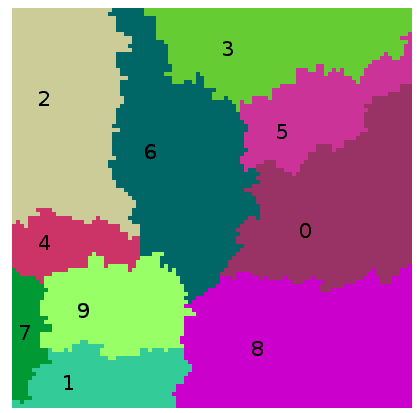 |
|
|
1) | |
| | Returns:
"</pre>10 states, 20 properties<br>
<br>
Areas:<br>
1403, 777, 954, 2302, 918, 2217, 495, 282, 91, 561 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>0111111001
1011110001
1101100000
1110110100
1111010010
1101101111
1000010101
0001011000
0000110000
1100011000
</pre>" |
Here is an image of the generated map that was used to construct the adjacency matrix and calculate the areas:
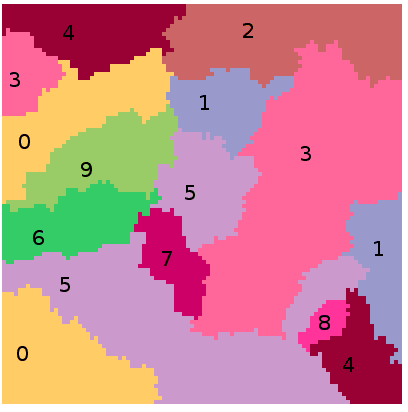 |
|
|
2) | |
| | Returns:
"</pre>10 states, 40 properties<br>
<br>
Areas:<br>
845, 1484, 725, 755, 620, 369, 1084, 619, 1238, 2261 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>0111100111
1001111111
1001000111
1110011111
1100011011
0101101001
0101110111
1111001011
1111101101
1111111110
</pre>" |
Here is an image of the generated map that was used to construct the adjacency matrix and calculate the areas:
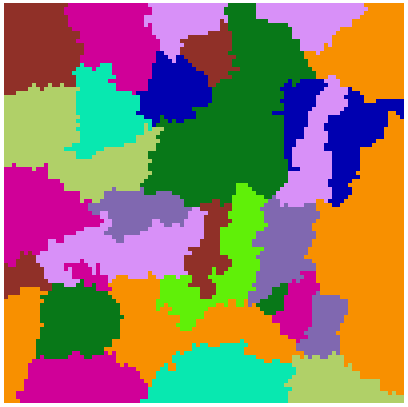 |
|
|
3) | |
| | Returns:
"</pre>500 states, 2000 properties<br>
<br>
Areas:<br>
3, 29, 51, 18, 48, 14, 20, 21, 37, 14, 15, 24, 34, 17, 16,<br>
9, 11, 17, 27, 27, 1, 27, 39, 31, 4, 20, 9, 24, 12, 11,<br>
31, 9, 43, 5, 34, 11, 38, 25, 30, 11, 20, 17, 32, 35, 25,<br>
13, 3, 18, 17, 26, 38, 7, 46, 17, 13, 4, 11, 17, 6, 1,<br>
11, 28, 23, 13, 31, 23, 31, 11, 11, 39, 17, 21, 43, 12, 44,<br>
23, 12, 31, 10, 21, 31, 17, 35, 9, 8, 29, 4, 22, 28, 9,<br>
25, 25, 38, 20, 20, 4, 35, 9, 20, 16, 17, 14, 24, 10, 8,<br>
22, 7, 20, 23, 19, 13, 19, 28, 15, 16, 15, 19, 11, 32, 8,<br>
11, 10, 32, 40, 27, 6, 22, 31, 16, 14, 17, 14, 17, 18, 19,<br>
12, 21, 11, 13, 10, 14, 38, 24, 20, 27, 21, 24, 55, 20, 13,<br>
18, 24, 35, 35, 27, 8, 24, 10, 17, 22, 29, 13, 10, 10, 10,<br>
43, 12, 18, 11, 14, 10, 9, 24, 29, 14, 20, 31, 30, 3, 15,<br>
15, 19, 5, 20, 4, 47, 15, 26, 30, 23, 30, 18, 44, 20, 13,<br>
11, 26, 23, 21, 10, 23, 16, 14, 13, 24, 17, 9, 27, 20, 46,<br>
20, 10, 15, 14, 14, 14, 7, 40, 21, 39, 58, 8, 22, 11, 28,<br>
11, 32, 34, 10, 30, 6, 25, 19, 33, 3, 19, 32, 23, 35, 5,<br>
19, 14, 11, 24, 26, 36, 19, 18, 20, 17, 24, 23, 19, 9, 34,<br>
12, 29, 5, 18, 41, 38, 17, 34, 29, 19, 22, 25, 7, 47, 19,<br>
6, 10, 5, 10, 17, 18, 24, 4, 15, 24, 19, 3, 19, 4, 15,<br>
11, 13, 13, 15, 28, 16, 8, 20, 12, 13, 34, 8, 6, 22, 16,<br>
35, 9, 26, 30, 16, 39, 19, 9, 42, 36, 16, 40, 15, 8, 27,<br>
6, 21, 18, 20, 20, 14, 24, 15, 17, 25, 9, 11, 13, 23, 17,<br>
45, 4, 8, 12, 62, 10, 15, 44, 9, 33, 25, 38, 22, 21, 20,<br>
30, 12, 21, 6, 34, 10, 19, 43, 14, 16, 33, 17, 5, 18, 5,<br>
27, 16, 34, 38, 21, 11, 6, 7, 40, 5, 11, 30, 25, 21, 30,<br>
29, 32, 27, 4, 22, 9, 10, 7, 1, 12, 24, 28, 35, 13, 30,<br>
19, 5, 28, 22, 16, 17, 18, 27, 9, 21, 22, 2, 13, 5, 47,<br>
16, 17, 23, 29, 17, 23, 8, 2, 13, 29, 18, 13, 11, 33, 8,<br>
11, 24, 23, 19, 13, 13, 19, 33, 40, 17, 32, 19, 18, 29, 7,<br>
21, 30, 14, 12, 12, 21, 17, 14, 8, 14, 15, 33, 13, 12, 45,<br>
19, 12, 14, 8, 14, 8, 23, 54, 13, 22, 26, 46, 8, 23, 9,<br>
17, 12, 15, 23, 16, 28, 33, 18, 14, 30, 44, 2, 30, 27, 14,<br>
12, 33, 13, 41, 8, 10, 21, 9, 15, 38, 8, 22, 13, 4, 14,<br>
36, 12, 33, 30, 7 " | |
|
4) | |
| | Returns:
"</pre>358 states, 1000 properties<br>
<br>
Areas:<br>
15, 50, 15, 15, 57, 52, 19, 28, 10, 31, 15, 26, 47, 45, 40,<br>
15, 9, 15, 33, 95, 19, 60, 24, 12, 32, 9, 49, 33, 31, 25,<br>
5, 1, 51, 54, 35, 46, 38, 30, 19, 78, 113, 29, 31, 25, 42,<br>
11, 12, 21, 16, 14, 48, 33, 15, 12, 16, 11, 25, 18, 4, 36,<br>
30, 127, 31, 43, 32, 28, 38, 31, 11, 48, 17, 14, 60, 37, 14,<br>
40, 13, 31, 48, 19, 13, 12, 13, 23, 35, 21, 17, 16, 15, 19,<br>
14, 33, 20, 29, 40, 64, 55, 4, 17, 21, 28, 29, 11, 77, 63,<br>
15, 5, 13, 1, 50, 24, 52, 42, 21, 16, 31, 23, 25, 28, 17,<br>
26, 38, 4, 23, 12, 27, 30, 29, 28, 7, 2, 21, 28, 26, 15,<br>
15, 77, 8, 5, 67, 18, 43, 25, 35, 10, 37, 16, 52, 16, 34,<br>
9, 14, 33, 41, 6, 32, 37, 44, 14, 14, 73, 20, 55, 27, 19,<br>
45, 45, 43, 28, 18, 23, 13, 13, 30, 46, 11, 11, 3, 5, 18,<br>
25, 79, 21, 8, 14, 48, 19, 36, 18, 14, 4, 25, 10, 39, 7,<br>
32, 33, 15, 16, 15, 11, 26, 9, 14, 21, 46, 9, 30, 37, 19,<br>
15, 19, 15, 26, 48, 18, 33, 37, 32, 12, 7, 53, 20, 20, 7,<br>
41, 18, 23, 30, 25, 14, 31, 14, 17, 13, 25, 21, 62, 40, 25,<br>
8, 38, 22, 13, 59, 16, 26, 49, 3, 13, 17, 16, 23, 22, 34,<br>
24, 10, 19, 50, 35, 29, 5, 6, 59, 9, 52, 13, 7, 41, 35,<br>
12, 23, 77, 34, 20, 4, 31, 29, 25, 26, 13, 54, 66, 36, 29,<br>
47, 26, 56, 25, 51, 30, 29, 1, 56, 37, 34, 28, 24, 21, 20,<br>
12, 40, 13, 8, 3, 10, 3, 36, 18, 29, 47, 26, 29, 52, 38,<br>
10, 13, 4, 46, 25, 41, 22, 8, 16, 30, 35, 50, 10, 17, 37,<br>
25, 14, 41, 23, 21, 36, 44, 63, 54, 9, 28, 29, 52, 6, 28,<br>
25, 17, 36, 30, 57, 21, 107, 31, 20, 47, 30, 9, 48 " | |
|
5) | |
| | Returns:
"</pre>232 states, 784 properties<br>
<br>
Areas:<br>
90, 40, 9, 97, 42, 94, 25, 16, 21, 43, 73, 44, 25, 77, 67,<br>
60, 79, 43, 50, 9, 18, 24, 7, 60, 69, 7, 106, 63, 39, 46,<br>
50, 82, 42, 33, 15, 53, 63, 98, 78, 59, 51, 14, 56, 110, 59,<br>
37, 33, 53, 93, 48, 18, 25, 61, 11, 143, 42, 33, 24, 63, 5,<br>
67, 3, 99, 107, 16, 33, 71, 11, 6, 32, 24, 37, 60, 9, 71,<br>
51, 28, 34, 2, 79, 44, 36, 51, 3, 42, 44, 102, 22, 127, 14,<br>
26, 29, 15, 79, 15, 62, 20, 17, 39, 28, 21, 31, 54, 49, 7,<br>
17, 41, 14, 22, 28, 39, 31, 41, 33, 62, 88, 30, 55, 80, 8,<br>
59, 45, 22, 52, 49, 22, 25, 31, 51, 17, 61, 10, 73, 15, 38,<br>
33, 62, 55, 21, 103, 1, 20, 20, 52, 66, 62, 36, 14, 91, 20,<br>
129, 74, 22, 50, 72, 21, 31, 22, 30, 15, 47, 31, 19, 71, 63,<br>
41, 50, 19, 52, 44, 13, 46, 32, 57, 60, 14, 70, 23, 33, 15,<br>
48, 26, 8, 47, 23, 74, 61, 102, 15, 31, 6, 13, 10, 8, 71,<br>
95, 34, 45, 47, 75, 64, 5, 33, 35, 20, 24, 9, 28, 68, 50,<br>
37, 14, 37, 33, 51, 71, 11, 86, 68, 14, 56, 51, 13, 26, 38,<br>
65, 24, 57, 73, 61, 44, 2 " | |
|
6) | |
| | Returns:
"</pre>420 states, 1393 properties<br>
<br>
Areas:<br>
23, 9, 33, 32, 43, 24, 12, 18, 22, 31, 17, 14, 11, 48, 24,<br>
26, 10, 22, 33, 17, 6, 25, 28, 30, 24, 26, 63, 71, 43, 17,<br>
50, 15, 22, 46, 6, 15, 16, 34, 13, 20, 12, 3, 22, 15, 47,<br>
39, 29, 42, 30, 40, 19, 42, 19, 30, 36, 27, 2, 29, 44, 22,<br>
13, 8, 24, 30, 37, 19, 52, 14, 15, 33, 7, 22, 25, 30, 35,<br>
13, 3, 30, 33, 32, 11, 31, 25, 38, 45, 10, 12, 45, 25, 14,<br>
41, 17, 16, 28, 16, 64, 27, 30, 17, 8, 19, 9, 1, 61, 14,<br>
4, 8, 15, 24, 6, 38, 26, 14, 4, 16, 22, 15, 20, 16, 2,<br>
14, 12, 1, 6, 8, 8, 25, 37, 8, 18, 52, 8, 21, 40, 31,<br>
4, 16, 16, 17, 17, 29, 18, 9, 18, 7, 18, 33, 69, 20, 38,<br>
15, 8, 47, 22, 36, 18, 30, 4, 16, 18, 19, 21, 19, 25, 22,<br>
29, 7, 26, 3, 18, 31, 27, 29, 32, 45, 45, 21, 37, 10, 37,<br>
17, 10, 25, 25, 15, 7, 9, 23, 11, 45, 8, 18, 9, 8, 46,<br>
21, 20, 47, 30, 10, 20, 8, 7, 12, 18, 26, 25, 21, 18, 25,<br>
27, 35, 24, 15, 12, 32, 4, 14, 19, 11, 31, 12, 28, 22, 22,<br>
36, 20, 64, 14, 10, 14, 23, 5, 31, 10, 16, 28, 19, 35, 29,<br>
4, 47, 29, 1, 18, 13, 18, 34, 45, 6, 56, 41, 30, 14, 47,<br>
23, 13, 12, 16, 20, 25, 29, 12, 69, 18, 11, 5, 41, 9, 32,<br>
7, 37, 34, 6, 16, 7, 15, 44, 15, 7, 54, 20, 22, 33, 33,<br>
22, 27, 25, 13, 22, 6, 33, 17, 12, 31, 33, 26, 13, 13, 13,<br>
24, 7, 36, 19, 7, 63, 43, 32, 1, 13, 25, 19, 16, 33, 53,<br>
27, 39, 9, 12, 45, 13, 23, 21, 10, 48, 4, 51, 19, 36, 19,<br>
34, 9, 18, 30, 14, 36, 51, 10, 15, 35, 22, 19, 29, 38, 25,<br>
22, 23, 13, 66, 20, 30, 8, 14, 9, 47, 36, 11, 16, 5, 22,<br>
19, 25, 12, 55, 26, 74, 53, 36, 26, 17, 19, 46, 47, 3, 4,<br>
39, 9, 24, 25, 12, 21, 30, 29, 56, 18, 20, 38, 5, 25, 45,<br>
39, 24, 26, 63, 33, 34, 36, 3, 19, 27, 2, 23, 18, 43, 33,<br>
24, 13, 5, 18, 56, 24, 30, 32, 8, 27, 16, 15, 10, 24, 31 " | |
|
7) | |
| | Returns:
"</pre>92 states, 286 properties<br>
<br>
Areas:<br>
100, 165, 136, 65, 94, 95, 35, 48, 2, 104, 108, 128, 160, 58, 52,<br>
64, 231, 107, 52, 166, 114, 69, 93, 59, 123, 211, 91, 215, 73, 200,<br>
438, 193, 136, 31, 58, 64, 77, 76, 86, 98, 42, 47, 132, 152, 66,<br>
92, 101, 40, 118, 64, 53, 76, 142, 118, 89, 113, 94, 89, 136, 39,<br>
224, 83, 162, 67, 182, 65, 129, 155, 175, 127, 59, 107, 42, 68, 72,<br>
293, 44, 14, 151, 83, 200, 35, 14, 101, 242, 77, 75, 142, 70, 120,<br>
83, 261 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>00000000000000000010001000000000100000100000000000001100000000110000001000000000000000000010
00000000000000001100011000000111000001100000100011000000001000101000001000010000000110000000
00010001000000000000000010000010100000000010000000000001010010010010000100010000000000001001
00100000000000001000000010000010000000000000000000010100000000001000000000010000000000000001
00000000001001000100101000000010000000000000000000000000000000101000000000000000010000001001
00000000000000000000000000110000000000000010000100001010000000000000100000000010000000000110
00000000000100000001000000000000000000000000001000000000000000010000000000010000000010000001
00100000000000001000001010100001000100000000000000000001000000000000000100010000000000000001
00000000000000000000000000000000000000000000000000000000000000000000000000000010000000010000
00000000000000001011100101000001011000000010001000010100000100000000000000100010000000010100
00001000000001101000010010000000000000000001010001001100100110000100000100000010010000010101
00000010000011010001000000001010000000000101001000000000101010001010000000000000000000000001
00000000000101100001000001010011000000000000000010000001100000000001000100000000100001100000
00001000001110000000000000000010000000000000010010001000000000100000000000000000000000000001
00000000001010000000000000010010000000000001000000000001100000000000000100000000000000000000
00000000000100000000000000000100010000000000000000000000000001000010000000010100000100000001
01010001011000000001001010000010110100000000000011010111000000100000111000010000101010100111
01001000000000000010000000000010000000001000000001000000001011001000000000010000000010000010
10000000010000000100000000010001000000001000000000000000100000000100001000000010000000000010
00000010010110001000000000010100100000000001000000000000011010010000000000010010000010010010
00001000010000000000001001010010000000010010000000000000001111000000000000000010000000000000
01000000001000000000000100000100000000100000001000000100000100000100000000000000000000010001
11001001000000001000100001000011100001000000000000001011001000100001010000000000000000000001
00000000010000000000010001000000001100100010001000010000000000000000000000000000000000000000
00110001001000001000000000100011100000000010010001000000000010000000110100000001000000000001
00000000010010000000101100010000100100000010111000011010001010100001000100000000101010010000
00000101000000000000000010010001000100000000000101100000000010000000000011010000000000100000
00000100000010100011100001100011000000100011000000000010101001000001000000000010000010110000
00000000000100000000000000000000000000000000000000001000000010100010000000000000100001100101
01000000000000010001010000000000000000000000000010000010000010001010100100000001100000100001
01111000000111101100101010010001000000111111000110011111110010001001101110100011100110001000
01000001010010000010001010110010100001000011100010001101101000000000001011010010000100100011
10100000000000001001001011000001000000100000000000000000000000110000001001010010000010000011
00000000010000011000000000000000000000000000000010000000000000000000000000010010000001000000
00000000010000000000000100000000000100000010001000001000000110000000000000000000000010000100
00000001000000001000000101100000001000000000000001000000000000000000000000000000100000000100
00000000000000000000000000000000000000010000000010100110000000000100000000000011000000000000
01000000000000000000001000000001000000000000010000000001000000000010111000001001000000000001
11000000000000000000010100010010100000000010100000000110001000000011011100000000000000110000
00000000000000000000100000000010000010000010000110100000000000000000000001010000000000000001
00000000000000000110000000000010000000000010000000000000000000000100000000000000000000000000
00000000000100000000000000000010000000000011000000000000000001001000000000000000000010000000
00100100010000000000100111010011001000111101000100000100000000000110001100000011000010001010
00000000001100100001000000010011000000000110010000000000100000001001000000010010000100000000
01000000000000000000000001000001000000100000000000000000001000001000010000000000000100010000
00000000001001000000000011000000000001000001000000000000100000000001100000001000100110000101
00000010010100000000010101000000001000000000000000000000001010010000000000000000001010100001
00000100000000000000000000100010000000010010000000000000000000000000000010000000000000001010
01000000000011001000000000000111010010010000000000001001100000101000101000000000000001000101
01000000001000001100000010100000000100000000000000000000000000000000000000000000000000000001
00000000000000000000000000100000000010010000000000000000000010001100000001000000000000000000
00010000010000001000000101000010000000000000000000001100010000000100000000000000000010010000
10000100001001000000001001001011001000000000000010010010000010100100010000010011000011000110
10010000011000001000010000000011000010100010000000010000100000001100101101100001000000010000
00000100000000001000001001010110000010100000000000001000000000000000100000010011000001100000
00100001000010101000001000000011000001000000000010000000000000000000000000010000100000000001
00000000001110100010000000010011000000000001010010000100000000100100101100000010000011100101
00100000000000000001000000000010000000000000000000010000000000000000000000010000000010000000
01000000000100000101101001010001000000100000101000000000000010000001000000000000000000000000
00000000011000000000110000000000001000000000000000000000000010000100000000000000000000010000
00100000001100000101100011101110001000000000001000101000001100011110000100000000110000110001
00000000000000010100100000010000000000000100000000000000000000001001000000000000000110001000
11001000000001001000001001001000100000000000000010001000100000000001000010010010100010001000
10100010000000000001000000000000100000000000001000000000000010000000000000000000000000000000
01011000000100000100000000000110000000000101100010100100000011000100010000000000110100000010
00000000001000000010010000000000000010001010000000111100100110001000010000000000000000010011
00100000000100010000000000001100000001100010000000000000000010000000100100000100100000000001
00000000000010000000001001010010000000100001010000000000001001100000001000010000100000001000
00000100000000001000000010000110000001000000010010000110100000000010010000000000000000000100
00000000000000001000001010000000000001100000100000001000000000001100101000000001000000010010
11000000000000001010000000000011100001100010000010000100100000000001010100001000000011000010
00100001001010100000000011000110000000100010000000000100100010000010001000000000000011100101
00000000000000000000000000100011000000000000000100000000000000100000000000000000000010001000
00000000000000000000000000100001100000010000000000100100000000000000000000110010000000000000
00000000010000000000000000000010000000000000000000000100000000000000000001000010000100010000
01110011000000011101000000100001110000010001000000001011010000100001000001000000000011000101
00000000000000000000000000000000000001000000010000000000000000000000001000000001000000000000
00000000000000010000000000000000000000000000000000000000000000000010000000000000100100000000
00000100111000000011100000010011110010000011000000001010100000100000000001100000000001010101
00000000000000000000000010000110000011000010000000001110000000000000010000001000100000000000
00000000000010001000000001001110000100000000010000000001000010101011000000000101000101000100
00001000001000000000000000000000000000000000000000000000000010001000000000000000000000000000
00000000000000001000000001000000000000000000001000000000000000000000000000000000000000100000
01000000000000010000000000000011000000000001110000000000000001001000000000100100100000010000
01000010000000001101000001010010101000000110011000011000110001100000001110010000000000001010
00000000000010000000000000001000010000000000000010001010100000000000001100010010100000100100
00000000000010001000000000111101000000100000001000000010100010000000000100000000001001000101
00000000111000000001010001010000000000100000100000010100000110000100010000100010000100000000
00101000000000000000000000000010000000000010000100000000000001100001000010000000000010000010
00000100011000001000000000001000001100000000010010001000100000000000100100010010100001100001
10000100000000001111000000000001100000000010000100001000000000001100011000000000000010001000
00111011001101011000011010001101100001010000011011000001100010000110000100010010000000100100
</pre>" | |
|
8) | |
| | Returns:
"</pre>207 states, 439 properties<br>
<br>
Areas:<br>
28, 71, 9, 73, 52, 145, 42, 42, 9, 51, 38, 8, 21, 44, 51,<br>
75, 77, 21, 64, 107, 51, 121, 53, 25, 42, 94, 38, 2, 11, 22,<br>
33, 44, 1, 22, 31, 60, 26, 79, 19, 51, 27, 60, 106, 98, 5,<br>
27, 33, 45, 95, 95, 58, 50, 29, 19, 16, 8, 48, 42, 32, 52,<br>
50, 41, 64, 57, 69, 46, 80, 59, 51, 26, 13, 128, 30, 7, 106,<br>
29, 20, 11, 32, 7, 81, 15, 20, 119, 31, 35, 199, 9, 16, 81,<br>
65, 16, 54, 42, 30, 82, 65, 95, 84, 38, 35, 52, 22, 24, 35,<br>
97, 44, 8, 76, 84, 23, 27, 33, 52, 56, 102, 58, 58, 41, 53,<br>
56, 24, 39, 29, 87, 51, 38, 25, 4, 64, 107, 50, 1, 54, 26,<br>
23, 17, 66, 69, 6, 27, 54, 25, 48, 24, 80, 36, 27, 74, 47,<br>
21, 26, 118, 70, 55, 76, 50, 62, 49, 56, 29, 160, 24, 50, 54,<br>
29, 32, 108, 64, 21, 49, 39, 137, 11, 28, 25, 74, 3, 44, 41,<br>
33, 68, 37, 83, 15, 69, 82, 108, 27, 5, 74, 55, 16, 14, 29,<br>
52, 33, 14, 74, 29, 26, 66, 9, 66, 70, 81, 1 " | |
|
9) | |
| | Returns:
"</pre>67 states, 202 properties<br>
<br>
Areas:<br>
144, 231, 413, 64, 167, 193, 39, 160, 244, 333, 55, 67, 315, 108, 134,<br>
305, 163, 67, 5, 148, 105, 54, 196, 77, 234, 84, 78, 227, 280, 255,<br>
103, 101, 346, 120, 109, 146, 50, 153, 111, 35, 30, 209, 112, 174, 262,<br>
84, 174, 137, 128, 163, 85, 79, 88, 17, 39, 174, 247, 167, 270, 187,<br>
108, 69, 159, 234, 281, 87, 17 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>0001000010010000000000001100001100011101100100100000000010010101100
0000000100001100100010001011000011000000000011000000101010100011000
0001010111001100000101010001100010000110011010101101001001000001010
1010000001000000000000001000000000000000011010000010100010100000000
0000000011000000000010001001111011000000100000001010000110010000000
0010000100000000100000000000100000000000001010001110000001000010110
0000000010001000000110000000100010000000101000000001000001000000000
0110010010001000100000100000000010000000000000100000001001100000010
1010101101000001000010001000111001010100010011011000000110000100101
0011100010010011000001001000010010011101010000101010000101100001100
0000000000000010100000100000010000000000000000000000000000010000000
1000000001000001100000000100000000000110000000000000000000000001000
0110001100000101010111101001100011010000110011001000000110111000000
0110000000001001000000110010000000000000010010000000000000000000000
0000000001100000000000000000010010000000000100100100000000110011000
0000000011011100010011110001100010000101001010000000100110000001000
0100010100110000000000100000010010000000000000000000001100001001100
0000000000001001000100000000100010010000100000000000100000000000000
0000000000000000000000000010000000100000000000000000000000000000000
0010001000001000010001000000000010000000001000101111000001010000000
0100101010001001000000001000100000000000100000000000100000000011100
0010000001001001000100000000000000011000000000100001000000000000010
0000000100101101100000000000000010100100010000010000000110111101000
0010000000000101000000000000000100000100001000000101000001000010000
1101100011001000000010000101100001000000000011000000000001100000100
1000000000010000000000001000001000000010000000000000000000100000000
0100000000000100001000000001100000100010010001000100000000001011000
0110100000001001000000001010001011000010010010000000000100000001000
0010111010001001010010001010000001000010010011000000100000110000101
0000100011100010100000000000000100100000010000000000000000100010100
1000100010000000000000000101000000000000001100000010000010100000000
1000000000000000000000010000010000000000100000000010000001000010100
0110101101001011110100100001000000010000001000111011000001111010110
0100100010001000000000001001100000000010000010110000000011000000000
0000000000000000001000100010010000000100010000000000000000001010000
1000000011001000010001000000000010001100100010110000100100000001001
1000000001000000000001000000000000010101000000000000000000000000000
1010000011010001000000110000000000111000000001010100000000011000011
0010000000010000000000000111100001000000000000000000000000100001000
1000000001000001000000000000000000001000000000000000000000000100000
1000101000001000010010000000000100010000000000000010100000010000000
0011000011001100000000100011110000100000000011010000001110110000000
0011011000000001000100010000001010000000000000000010000011100100000
1000000000000010000000000000001000000000000000000000000110000111010
0111010010001101000000001001100001010000010001000000100101100001000
0100000010001000000000001010100000000100010010000000000100100000001
1010000101000010000101000000000011010000000000011100000001100001000
0000000010000000000000100000000011010100010000101000000110011100010
0010110011001000000100000000000010000000000000110110000010000000000
0010010000000010000100010010000000000100000000101000000000001010000
0001110001000000000100000000001110000000101000001000000001100000000
0010001000000000000101010000000010000000000000000000010001000000100
0101000000000001010010000000100000010000100010000000000010100000100
0000000000000000000000000000000000000000000000000001000000000010000
0110000100000000100000000000000000000000010000000000000100000000000
0000100011001001100000100001000000010000010111010000001010010001000
1101100010001001000000100000001001000000011100011000100100100100000
0010011101000000000100011000000111000000001010100011000000100000010
0101000101001010000000101100111010000010011011100010100011000000100
1000100000101010000100100000100010000100110000010000000100001001100
0000000000001000100000100010000010100100000000010100000000010000000
1000000010000000000000100000000000000001001100010000000010000001000
0100010000000010000010010010010110100000000100000100010000000000010
1110000001010011100010100011000000010010000110100000000100010100000
1000010011000000100010001000110110000000000000000001100000110000000
0010010100000000000001000000000010000100000100010000000001000010000
0000000010000000000000000000100000010100000001000000000000000000000
</pre>" | |
|