Problem Statement |
| HTML is the markup language used on the World Wide Web. In HTML, the simplest type of table is formed as follows:
The table starts with "<table>" and ends with "</table>". These are the outermost tags, and within them are a number of rows. Each row starts with "<tr>" and ends with "</tr>". Within each row, there are a number of cells. Each cell starts with "<td>" and ends with "</td>". Between the "td" tags are the contents of the cell, which may be 0 or more characters. The rows are displayed in the same order they appear, and the cells within a row are also displayed in the same order they appear. For the purposes of this problem, every row will have the same number of cells. For example, the following HTML will render the table below:
<table>
<tr><td>A</td><td>B</td></tr>
<tr><td></td><td>C</td></tr>
</table>

Your task is to make a calendar for a particular month in HTML. The month has days days in it, and starts on day start, where 0 is Sunday, 1 is Monday, and so on. You should make a table for this month, with a single row for each week, and a single column for each day. In addition, you should have a header row, with 7 cells, containing the letters (in order) 'S', 'M', 'T', 'W', 'T', 'F', 'S'. You should then fill in the calendar with the days of the month, starting from the appropriate day of the first week (in the second row of the table) with a "1". Note that some of the cells at the beginning and end of the calendar may be empty, "<td></td>", but must still be included in the return. Be careful not to include any completely empty rows in your return though. For example, if days = 30 and start = 3, you would want to generate the following table:
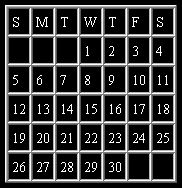
You should return a String[], the first element of which is "<table>", and the last element of which is "</table>". Each element in between should represent a single row in the table, starting with "<tr>", and ending with "</tr>"
|
|
Definition |
| Class: | CalendarHTML | Method: | makeTable | Parameters: | int, int | Returns: | String[] | Method signature: | String[] makeTable(int days, int start) | (be sure your method is public) |
|
|
|
|
Notes |
- | All of the tags in the return should be lowercase, while the contents of the cells in the first row ('S', 'M', 'T', 'W', 'T', 'F', 'S') should be uppercase. |
- | There should be no spaces or leading zeros anywhere in the return. |
|
Constraints |
- | days will be between 28 and 31, inclusive. |
- | start will be between 0 and 6, inclusive. |
|
Examples |
0) | |
| | Returns:
{ "<table>",
"<tr><td>S</td><td>M</td><td>T</td><td>W</td><td>T</td><td>F</td><td>S</td></tr>",
"<tr><td></td><td></td><td></td><td>1</td><td>2</td><td>3</td><td>4</td></tr>",
"<tr><td>5</td><td>6</td><td>7</td><td>8</td><td>9</td><td>10</td><td>11</td></tr>",
"<tr><td>12</td><td>13</td><td>14</td><td>15</td><td>16</td><td>17</td><td>18</td></tr>",
"<tr><td>19</td><td>20</td><td>21</td><td>22</td><td>23</td><td>24</td><td>25</td></tr>",
"<tr><td>26</td><td>27</td><td>28</td><td>29</td><td>30</td><td></td><td></td></tr>",
"</table>" } | |
|
1) | |
| | Returns:
{ "<table>",
"<tr><td>S</td><td>M</td><td>T</td><td>W</td><td>T</td><td>F</td><td>S</td></tr>",
"<tr><td>1</td><td>2</td><td>3</td><td>4</td><td>5</td><td>6</td><td>7</td></tr>",
"<tr><td>8</td><td>9</td><td>10</td><td>11</td><td>12</td><td>13</td><td>14</td></tr>",
"<tr><td>15</td><td>16</td><td>17</td><td>18</td><td>19</td><td>20</td><td>21</td></tr>",
"<tr><td>22</td><td>23</td><td>24</td><td>25</td><td>26</td><td>27</td><td>28</td></tr>",
"</table>" } | |
|
2) | |
| | Returns:
{ "<table>",
"<tr><td>S</td><td>M</td><td>T</td><td>W</td><td>T</td><td>F</td><td>S</td></tr>",
"<tr><td></td><td></td><td></td><td></td><td></td><td></td><td>1</td></tr>",
"<tr><td>2</td><td>3</td><td>4</td><td>5</td><td>6</td><td>7</td><td>8</td></tr>",
"<tr><td>9</td><td>10</td><td>11</td><td>12</td><td>13</td><td>14</td><td>15</td></tr>",
"<tr><td>16</td><td>17</td><td>18</td><td>19</td><td>20</td><td>21</td><td>22</td></tr>",
"<tr><td>23</td><td>24</td><td>25</td><td>26</td><td>27</td><td>28</td><td>29</td></tr>",
"<tr><td>30</td><td>31</td><td></td><td></td><td></td><td></td><td></td></tr>",
"</table>" } | |
|