"17 &&&%&&&&%%&&%&&&&&%&&$&%%%&&%&&&&%&&%&&&&&&&%$&%&%%&%&&%&&&%&%%&&%&%%&&&%&&&%&&&&&&&&%&&&&%&&&&&&%&&%&%&&&&%&%&%&&%&&&&%&&&&&&&&&&&&$&%%&&&%&%&%&%&&%&&%&&%%&&%%&%&%&&&&%%&%%&&&%&&&%%%&&&&&&&&%&&&&'&&&%&&&%&&&&&%&&&&%&&%&&%&&%%%&&&%%&&&%&&%%%&&&&&&&&&%&&%%&%&&&&%&&&&%%&&$&&&$%&%'&%%%&&%&&&&&&&%%&%%&$&&&&&&%&&&&&%&&&&&&%&%%&&&&%&%$%&&&&&%%&&&%&&&%&&%%&%%&&%%%&&&&&&%%%&&%%&%%&%&%%&%$&&%&&&%%&&%&%&&&%&%%%&&&%%&%%&%&%%$&&%%&$&&&%&&&&%&%&%$&%&&&&%&&%&%&&&&%&&&%&%%&&&&$%&&&%%%&&%%&&&&%&&%&&%%&%%%&&%%&%&&&&%&&&&&%&%%&&&&&&%&%&%%%&&&&&%%%&&&%&%&%&&&&&&&&&&&&%&'%&&&%&&%&%&&&&&&%&%&&&&&%&&%&&&%&&&%&%%&&&&&&%&&&%%%'%&&%&%%&&%%&&&&&&&&&%&&&&&&%&&&&&&%&%%%&&&&&%&$&%&&%&&&&$%&&%&&&&&%%&&%&&&&&&&%%&&&%&&&%&%%&%%&&&&&%%&&%&&%&&&&&&&%&%%%&&%&&&&%&&&&&&%&&&&&&&%&&%&%&&&%&%&%&%%%&%&&&&&&&%&&&&&&%%&%&&&&&&%%%&%&%&%&&%%&&&%&%&%%%&%&&&&&%&%&&&&%&&%&&&&&%%&&&%&%%&&&&%&%&&&&&%$&&&&%&%&&%$%%&&%%&%&%&&%&%%%%&&&&&&%&&&&%&%&&&%%%&%%&&&&&&%%%&%&&%&&&%&&%&&%%$&%&%%%%%&&&&&&%&&&&&&&&&$&%&&%%%&%&&&$&&&&%%%&&%&&&%&&&&&&&%&%&&&&&$%&%&&&%&&&%&%%%&&&&&%%&&&%&&&&&&&&%&&%%&%%%%&&%%&%&&&%&&%&&&&&&&%&%&%&&$%&&&&&%&&&&%&&&&%&&%&%%&&&&&'&$&$%&&&&&&%%&&&&%&&&&&&&&&&%&&%&%&%%%&&&%&&%&%&&%&&%%%&%%&&%%&%%&%&&%&&&&&&%&%&&%&&&%&%&'&&&&%&&&%&%&%&&%&&&&%$&&&&&&&%&&%&%%&&$&%&%&&&&&&%&&&%&&%%&%&%&&&%%&%%&&&%&&&&&&&&&&&&&&&&%&&&&&&&%%&&&%&%&&%&%$%$&&%&&%%&&&%%&%&&%%&%%&&&&&%%&&%%&%&&%%%&%%&&%&&&&%%&%&%&%&%&&&%%&&&&%&&%&&&&&&%&&%&&&%&&&&&&%&&&&&&&%&&$&&%&&&%$%&%&&&&%&&&&&&%&%%&&&&&&&%&%%&%&%&&%&%%&%&&&&%&&&%%&%&%&&&%%%%&&&&&&&%%%%%&&%&&%&&&&%&%&&%&%&&&&&&&&&%%&%&%&&%&&%&&&&%&&&&&%&&&%%%%&&%%%&&%&%&&&&&%&&%&&&&&%&&&&&&&&&%%&&&&$%&&&&&&&%%&&%&&&%&&&%%&&&&&&%&%%%%&&%%&&&&&%&&&&&$&&%%&&&&&&&%%%%%&&&&%&%&%&&%&&%%&%&&&&&&&&&%&%&%&&%&&&%%&&&&%&%&&&%&&%&&&$&&&%&%%&&%&%%%&%%&&&&&%&%&%%&&&&&%&%%&&&%&&%&%%&&&%%%&&&&&&%%&&&&&%&&&&&%&&%&&%$&
Problem Statement | | When the Christmas dinner is over, it's time to sing carols. Unfortunately, not all the family members know the lyrics to the same carols. Everybody knows at least one, though.
You are given a String[] lyrics. The j-th character of the i-th element of lyrics is 'Y' if the i-th person knows the j-th carol, and 'N' if he doesn't. Return the minimal number of carols that must be sung to allow everyone to sing at least once.
| | Definition | | Class: | CarolsSinging | Method: | choose | Parameters: | String[] | Returns: | int | Method signature: | int choose(String[] lyrics) | (be sure your method is public) |
| | | | Constraints | - | lyrics will contain between 1 and 30 elements, inclusive. | - | Each element of lyrics will contain between 1 and 10 characters, inclusive. | - | Each element of lyrics will contain the same number of characters. | - | Each element of lyrics will contain only 'Y' and 'N' characters. | - | Each element of lyrics will contain at least one 'Y' character. | | Examples | 0) | | | | Returns: 2 | Both carols need to be sung. |
|
| 1) | | | | Returns: 1 | Everybody knows the first carol, so singing just that one is enough. |
|
| 2) | | | {"YNN","YNY","YNY","NYY","NYY","NYN"} |
| Returns: 2 | Singing the best known carol is not always the optimal strategy. Here, the optimal way is to pick the first two carols even though four people know the third one. |
|
| 3) | | | {"YNNYYY","YYNYYY","YNNYYN","NYYNNN","YYYNNN","YYYNNY","NYYYYY","NYNYYY","NNNNYY",
"YYYYYY","YNNNNN","YYYYNY","YYNNNN","NNYYYN","NNNNYY","YYYNNN","NYNNYN","YNNYYN",
"YYNNNY","NYYNNY","NNYYYN","YNYYYN","NNNYNY","YYYYNN","YYNYNN","NYYNYY","YYNYYN"} |
| Returns: 4 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | The common method to print the standings for a volleyball tournament is to print the number of won matches, the number of lost matches, the number of won sets, and the number of lost sets for each team.
Each match consists of three to five sets, where each set is won by one of the teams. The first team to win three sets is declared the winner of the match.
You are given one row of the standings table. That is, you are given an int wonMatches, denoting the number of matches won by a team, an int lostMatches, denoting the number of matches lost by that team, an int wonSets, denoting the total number of sets won by that team, and an int lostSets, denoting the total number of sets lost by that team. These numbers represent a possible tournament outcome for a team, and you are to reconstruct the results of all matches for that team, and return them as a comma-separated list, sorted lexicographically. Each match in the list must be formatted as the number of sets won by the team, followed by a dash ('-'), followed by the number of sets lost by the team. The possible outcomes for a match are "0-3", "1-3", "2-3", "3-0", "3-1", and "3-2" (all quotes for clarity only). If multiple different result sets are possible (sets that are reorderings of each other are not considered different), return "AMBIGUITY" (quotes for clarity) instead. | | Definition | | Class: | VolleyballTournament | Method: | reconstructResults | Parameters: | int, int, int, int | Returns: | String | Method signature: | String reconstructResults(int wonMatches, int lostMatches, int wonSets, int lostSets) | (be sure your method is public) |
| | | | Notes | - | The returned string must not contain spaces. | | Constraints | - | wonMatches and lostMatches will each be between 0 and 100, inclusive. | - | At least one of wonMatches and lostMatches will be greater than 0. | - | wonSets and lostSets will each be between 0 and 500, inclusive. | - | The numbers given will correspond to at least one possible result set. | | Examples | 0) | | | | Returns: "0-3,0-3,0-3,3-0,3-0,3-0" | |
| 1) | | | | 2) | | | | Returns: "AMBIGUITY" | It could have been "3-0,3-1,3-2" or "3-1,3-1,3-1". |
|
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You are given an integer N, and you are allowed to perform the following operation: take two nonzero digits of N, decrease each of them by one and swap the resulting digits. For example, if N is 166, you can reach the following numbers in one operation: 506 (swap '1' and the first '6'), 155 (swap the '6's) and 560 (swap '1' and the last '6'). You are allowed to perform the operation zero or more times consecutively. Return the largest number you can reach.
| | Definition | | Class: | RemissiveSwaps | Method: | findMaximum | Parameters: | int | Returns: | int | Method signature: | int findMaximum(int N) | (be sure your method is public) |
| | | | Constraints | - | N will be between 1 and 1,000,000, inclusive. | | Examples | 0) | | | | Returns: 560 | The example from the problem statement. |
|
| 1) | | | | Returns: 8832 | The following sequence of operations is optimal: 3499 -> 8492 -> 8832. |
|
| 2) | | | | Returns: 88220 | The following sequence of operations is optimal: 34199 -> 84129 -> 88123 -> 88220. |
|
| 3) | | | | Returns: 809070 | No operations can increase the number. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You are to populate a 5x5 square with each of the numbers between 1 and 25, inclusive. The numbers in each row must be in increasing order from left to right. Some of the cells are already filled in, but no more than one number per row. You must fill in the remaining cells.
You will be given a String[] square. Each row is given as a space-separated list of cells. Each cell is either a number written with two digits (numbers less than 10 have an additional leading zero) or two '?' characters which represent an empty cell. Your method should return a String[] in the same format, but with all the empty cells filled in. If there are no arrangements possible return an empty String[]. If there are several arrangements possible return the one with the smallest first number in the first row. If there are still several arrangements possible return the one with the smallest second number in the first row, and so on.
| | Definition | | Class: | NewMagicSquare | Method: | completeTheSquare | Parameters: | String[] | Returns: | String[] | Method signature: | String[] completeTheSquare(String[] square) | (be sure your method is public) |
| | | | Constraints | - | square will contain exactly 5 elements. | - | Each element of square will contain exactly 14 characters. | - | Each element of square will be a space-separated list of cells. | - | Each cell will be either a number written with two digits or two '?' characters. | - | Each element of square will contain at most one filled cell. | - | All numbers in square will be between 1 and 25, inclusive. | - | All numbers in square will be distinct. | | Examples | 0) | | | {"?? ?? ?? ?? ??",
"?? ?? ?? ?? ??",
"?? ?? ?? ?? ??",
"?? ?? ?? ?? ??",
"?? ?? ?? ?? ??"} |
| Returns:
{"01 02 03 04 05",
"06 07 08 09 10",
"11 12 13 14 15",
"16 17 18 19 20",
"21 22 23 24 25" } | You are not limited by prefilled cells. The answer is the lexicographically smallest square. |
|
| 1) | | | {"?? ?? 20 ?? ??",
"?? ?? ?? ?? ??",
"?? ?? ?? 05 ??",
"?? ?? ?? ?? ??",
"?? ?? ?? ?? ??"} |
| Returns:
{"01 06 20 21 22",
"07 08 09 10 11",
"02 03 04 05 12",
"13 14 15 16 17",
"18 19 23 24 25" } | |
| 2) | | | {"?? ?? ?? ?? ??",
"?? ?? ?? ?? 24",
"?? ?? ?? ?? ??",
"?? ?? ?? ?? ??",
"21 ?? ?? ?? ??"} |
| Returns: { } | You should place four numbers greater than 21 into the 5th row. There are four such numbers - 22, 23, 24 and 25, but 24 is already used in the second row. |
|
| 3) | | | {"?? ?? 15 ?? ??",
"02 ?? ?? ?? ??",
"?? ?? ?? 07 ??",
"?? ?? 16 ?? ??",
"?? ?? ?? ?? 21"} |
| Returns:
{"01 03 15 17 18",
"02 08 09 10 22",
"04 05 06 07 23",
"11 12 16 24 25",
"13 14 19 20 21" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
There is a direct bidirectional road between every pair of distinct towns in the country. Terrorists want to blow up enough of these roads that there are at least two towns that are no longer connected to each other by any direct or indirect paths.
You will be given a String[] roads. The j-th character of the i-th element of roads is a digit representing the cost of blowing up the road from the i-th town to the j-th town. Return the minimal total cost required for the terrorists to achieve their goal.
| | Definition | | Class: | Terrorists | Method: | requiredCost | Parameters: | String[] | Returns: | int | Method signature: | int requiredCost(String[] roads) | (be sure your method is public) |
| | | | Constraints | - | roads will contain between 2 and 50 elements, inclusive. | - | Each element of roads will contain the same number of characters as the number of elements in roads. | - | Each element of roads will consist of digits ('0' - '9') only. | - | The i-th character of the j-th element of roads will be equal to the j-th character of the i-th element for all pairs of i and j. | - | The i-th character of the i-th element of roads will be '0' for all i. | | Examples | 0) | | | {"0911",
"9011",
"1109",
"1190"} |
| Returns: 4 | The best decision is to isolate towns 0 and 1 from towns 2 and 3. So, the terrorists should blow up roads (0,2), (0,3), (1,2) and (1,3).
|
|
| 1) | | | {"0399",
"3033",
"9309",
"9390"} |
| Returns: 9 | The cheapest plan is to isolate town 1. So, the roads to blow up are (1,0), (1,2) and (1,3). |
|
| 2) | | | {"030900",
"304120",
"040174",
"911021",
"027207",
"004170"} |
| Returns: 8 | |
| 3) | | | {"044967263",
"409134231",
"490642938",
"916036261",
"634306024",
"742660550",
"229205069",
"633625604",
"318140940"} |
| Returns: 27 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | The Kingdom of Byteland consists of several cities connected by bidirectional roads. There are castles standing in some of the cities. Byteasar, the King of Byteland is unhappy with his kingdom's defensive power, so he decided to build k additional castles (he can only afford that many).
Whenever one of the cities is attacked by the enemy, the army from the nearest castle must come with help. The enemy is smart, so it will always find and attack the city that is the farthest away from its nearest castle. Therefore, the distance from the attacked city to its nearest castle must be as small as possible. Your job is to calculate and return this distance, assuming that you can decide where to build the k additional castles.
The cities are built in a specific manner. Each city has a main gate and several other gates. Every gate is connected to another gate by a road. It is possible that two gates of the same city are connected (see example 2). For defensive reasons, every road has exactly one main gate on one of its ends. Please note that every road can be traversed in both directions.
The road going out of the main gate of the i-th city leads to the road[i]'th city and is distance[i] miles long. The int[] castles contains the 0-based indices of the cities in which a castle is already built.
| | Definition | | Class: | ByteLand | Method: | buildCastles | Parameters: | int[], int[], int[], int | Returns: | int | Method signature: | int buildCastles(int[] road, int[] distance, int[] castle, int k) | (be sure your method is public) |
| | | | Constraints | - | road will contain between 2 and 50 elements, inclusive (let's call this number n). | - | road and distance will contain the same number of elements. | - | Each element of road will be between 0 and n-1, inclusive. | - | Each element of distance will be between 1 and 10^6, inclusive. | - | k will be between 1 and n, inclusive. | - | castle will contain between 0 and n-k elements, inclusive. | - | Each element of castle will be between 0 and n-1, inclusive. | - | The elements of castle will be distinct. | - | It will be possible to build the castles in such a way that you can always reach a castle from each city. | | Examples | 0) | | | {1,2,3,4,0} | {1,1,1,1,1} | {} | 1 |
| Returns: 2 | Building the castle in any of the cities results in the maximum distance from a city to a castle being 2. |
|
| 1) | | | | 2) | | | | 3) | | | {0,2,0,0,2,2,8,3,8,7} | {10,9,1,8,1,3,7,2,8,1} | {3,4,6} | 3 |
| Returns: 3 | |
| 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Your new graphical programming language Honu contains the primitives 'L' (turn left),
'R' (turn right), 'U' (turn up), 'D' (turn down), and 'F' (move forward), to describe the
actions of a trail-leaving turtle in the three-dimensional sea.
Only the 'F' command actually moves
the turtle, and it always moves it one unit forward. All other commands simply
change the orientation of the turtle. The turn commands all are with respect to
the turtle's current orientation. That is, 'R' means the turtle turns towards its
current right side; 'U' means the turtle turns towards where its shell is facing.
In addition, commands can be repeated if they are immediately followed by a
single digit from 1 to 9. So "F3" moves forward 3 units. There will not be
adjacent digits in the command string. Digits may only follow the characters
'U', 'D', 'R', 'L', 'F', or ')'. The repeat count only applies to the
immediately previous command, so "FF3" only advances four units.
Commands can also be grouped by parentheses. So "(FRFL)5" traces a zig-zag
line, repeating "FRFL" five times.
Parentheses can be nested, so "((F2)2)2" moves forward 8 units.
Given a sequence of these commands, your method must return the
Euclidean distance from where the
turtle began to where it ended up.
| | Definition | | Class: | TurtleGraphics | Method: | distance | Parameters: | String | Returns: | double | Method signature: | double distance(String command) | (be sure your method is public) |
| | | | Notes | - | Your return value must have an absolute or relative error less than 1e-9. | | Constraints | - | command will contain between 0 and 50 characters, inclusive. | - | command will consist only of the characters 'R', 'L', 'U', 'D', and 'F', '(', ')', and the digits '1'-'9'. | - | command will be properly formatted according to the description above. | | Examples | 0) | | | | Returns: 1.4142135623730951 | Going forward, turning right, and then going forward again leaves the turtle at a distance of the square root of two. |
|
| 1) | | | | Returns: 0.0 | The turtle traces a vertical square and ends up where he started. |
|
| 2) | | | | Returns: 1.7320508075688772 | |
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | String matching is extraordinarily useful. In our application, we define the
match quality between 2 strings of lowercase letters to be the number
of positions that match
exactly. For example, the match quality between
"abcdedd" and "bcddd" is 1, since they only match in the 4th character.
We have a string sequence, data, and are interested in finding string sequences
of the same length that fit the data well. We say that one sequence fits the data
better than another sequence if it has a higher quality match with the data at
the first position where the match qualities differ.
String sequences are ordered lexicographically based on the normal alphabetical
comparison between individual strings. That means that if position i is the first
position where 2 sequences differ, the sequence whose ith element would come later
in a dictionary is later in the ordering of sequences.
Given data and candidate return the next later permutation of candidate
that fits data better.
If no lexicographically later permutation of candidate gives a better fit,
return candidate.
| | Definition | | Class: | NextBetter | Method: | nextPerm | Parameters: | String[], String[] | Returns: | String[] | Method signature: | String[] nextPerm(String[] data, String[] candidate) | (be sure your method is public) |
| | | | Constraints | - | data will contain between 1 and 50 elements, inclusive. | - | candidate will contain the same number of elements as data. | - | Each element of data and candidate will contain between 1 and 10 characters, inclusive. | - | Each element of data and candidate will contain only lowercase letters ('a'-'z'). | | Examples | 0) | | | | Returns: {"xx", "ade" } |
The only permutation of "xx","ade" is earlier (since "ade" precedes "xx"). Therefore, there is no next permutation, and candidate is returned.
|
|
| 1) | | | | Returns: {"yde", "xx" } |
"yde","xx" is the next permutation of "xx","yde" and it fits data better
since it has
a match quality of 1 in the first position ("yde" vs "ad") while candidate
has a match quality of 0 in the first position of data. |
|
| 2) | | | {"ade","cde","acd"} | {"ab","ae","c"} |
| Returns: {"ab", "c", "ae" } |
All 5 permutations of candidate come later than candidate. "ab","c","ae" fits
data better than candidate, and so does "ae","c","ab". None of the
other permutations fits data better than candidate. So the return is
the earlier of these 2. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | There are a number of nodes placed at lattice points on a grid. You need to design a network that connects them. You may install new nodes on lattice points, and you may run network cable between any two nodes.
You have two goals in the design of your network. First, you want to minimize the cost of the network you build. That means not installing too many new nodes or running too much cable. Second, you want to make the network fault tolerant. Each node that you install as well as the original nodes, and each unit of cable that you run has some probability of failing.
The inputs x and y will specify the locations of the nodes that must be connected. The input cost will specify how much it will cost if there is a failure that prevents some of the original nodes from communicating. The jth integer in element i of cost will specify the cost incurred if node/cable failures prevents nodes i and j from communicating. The input p represents the probability that a node will fail. The input q respresents the probability that one unit of cable will fail (thus, the probability that a length L cable will fail is 1-(1-q)L). Cable costs 1 per unit length, while nodeCost gives the cost of adding a new node.
Your network design will be judged based on it's expected cost: the sum of the cost of the nodes and cable installed, plus the expected cost resulting from network failures. You should return a String[] where each element specifies either a new node, or a connection between two nodes. If you are installing a new node, the element of the String should specify its coordinates formatted as "x y". If you are installing cable, the element should be formatted as "x1 y1 x2 y2", indicating cable from (x1,y1) to (x2,y2). You may install new nodes in at most 30 unique new locations, and your returned String[] may have at most 1000 elements. If your return is invalid in any way (bad formatting, cables not ending at nodes, etc.), you will be judged as if you returned {} -- you will pay the cost of all communications failing. If you cause a runtime error or exceed the time limit (of 20 seconds) you will also be judged in this way.
The inputs will be randomly generated. All random numbers will be generated uniformly and independently. All ranges are inclusive. First, the number of nodes, N, will be chosen between 5 and 20. Each node will be placed on a unique random lattice point with x and y coordinates between 0 and 99. p and q will be between 0.0 and 0.01. The cost between each pair of nodes will be an integer between 0 and 100,000. nodeCost will be between 10 and 100.
To compute the probability that two nodes can communicate, a monte carlo method will be employed. The random failure process will be simulated 1,000,000 times and the expected cost of the failures will be approximated by the average of these trials.
Your score for each test case will simply be your expected cost as computed by the monte carlo method. Your overall score will simply be 100000 minus the average of your scores on the individual cases. If your score is less than 0, it will be changed to 0. | | Definition | | Class: | GraphBuilder | Method: | build | Parameters: | int[], int[], double, double, int, String[] | Returns: | String[] | Method signature: | String[] build(int[] x, int[] y, double p, double q, int nodeCost, String[] costs) | (be sure your method is public) |
| | | | Notes | - | You may run multiple cables between the same two nodes. | - | You may install multiple nodes at the same lattice point. If you do, they will be connected to each other by default (and of course this connection cannot fail, having length 0). Furthermore, as long as at least one of the multiple nodes at a point does not fail, all cables terminating at that point will be able to communicate through that point. In other words, installing two nodes at a point is equivalent to installing one node at that point that has a probability of failing of p2. | - | The original nodes act just like the ones you may add, with the same failure rate as other nodes. | - | If one of the original nodes fails, then clearly it will not be able to communicate with any of the other nodes. This is unavoidable, and thus this cost is not counted against you. | - | To keep the simulation times down, java.util.Random will be used for random number generation. | - | costs will be symmetric and its diagonal entries will be 0. | - | The cost of a cable between two points will be the Euclidean distance between those points. | - | Cables may cross, and may pass over nodes without being incident to them. For example, a node at (1,1) does not have any effect on a cable from (0,0) to (2,2). | - | The memory limit is 64MB. | - | Your return should be single-space delimited without leading or trailing spaces. | - | The 30 new unique node location limit means that you may create as many nodes as you want in up to 30 new locations. The locations of the nodes given to you do not count against this limit. | | Examples | 0) | | | | Returns:
"x = {6, 48, 17, 92, 32, 53}
y = {78, 69, 63, 62, 10, 37}
p = 0.004100808114922016
q = 0.0020771484130971706
nodeCost = 17
costs =
0 95194 25379 48473 75678 39722
95194 0 49336 1729 76360 96659
25379 49336 0 16847 19035 30327
48473 1729 16847 0 54422 94630
75678 76360 19035 54422 0 34098
39722 96659 30327 94630 34098 0 " | One simple strategy would be to place a single node at (50,50) and then connect each of the original nodes to it. However, this is very prone to failure. One way to reduce the risk of failure is to have redundant nodes and edges. If we add two nodes at (50,50), and add 4 cables from every computer to (50,50), we will reduce the risk considerably. Now, there will be a functioning node at (50,50) with probability 1 - p2, and each node will have a functioning cable to (50,50) with pretty high probability.
The cost of the nodes and cables with this strategy is 864.5 and the expected cost from failures is about 74, for a total cost of roughly 939. |
|
| 1) | | | | Returns:
"x = {19, 68, 34, 67, 47, 35, 30, 58, 66}
y = {47, 94, 14, 99, 99, 53, 24, 19, 98}
p = 0.009014476240300544
q = 0.004968225934308908
nodeCost = 31
costs =
0 91362 16908 94139 90780 74980 58776 68432 12961
91362 0 42371 69340 73967 41612 75504 1122 79824
16908 42371 0 26023 8107 78814 9306 60845 44772
94139 69340 26023 0 62344 4468 34904 40340 52441
90780 73967 8107 62344 0 2675 7898 86637 70758
74980 41612 78814 4468 2675 0 56628 65273 94046
58776 75504 9306 34904 7898 56628 0 54764 11262
68432 1122 60845 40340 86637 65273 54764 0 91519
12961 79824 44772 52441 70758 94046 11262 91519 0 " | |
| 2) | | | | Returns:
"x = {29, 69, 42, 46, 95, 72, 93, 1, 9}
y = {33, 89, 44, 95, 75, 5, 68, 88, 34}
p = 0.00811194812081668
q = 0.00534613102433974
nodeCost = 19
costs =
0 91710 26818 47023 62803 201 26321 43902 69137
91710 0 27276 25856 1954 77473 63667 90034 93532
26818 27276 0 75046 33704 90756 13079 32444 61138
47023 25856 75046 0 82148 12866 98485 86568 83739
62803 1954 33704 82148 0 44777 75993 38130 11574
201 77473 90756 12866 44777 0 95959 65328 2423
26321 63667 13079 98485 75993 95959 0 24480 29330
43902 90034 32444 86568 38130 65328 24480 0 40488
69137 93532 61138 83739 11574 2423 29330 40488 0 " | |
| 3) | | | | Returns:
"x = {64, 51, 58, 42, 88}
y = {75, 95, 45, 65, 96}
p = 0.0040731303630733165
q = 0.0013070565365267284
nodeCost = 55
costs =
0 13927 7998 42420 57221
13927 0 3176 92108 94332
7998 3176 0 70383 16346
42420 92108 70383 0 52311
57221 94332 16346 52311 0 " | |
| 4) | | | | Returns:
"x = {44, 8, 96, 17, 12, 22, 43, 40, 36, 62, 99, 19, 97, 87, 87, 8}
y = {32, 42, 90, 43, 36, 2, 71, 10, 6, 43, 13, 16, 69, 77, 1, 44}
p = 0.0016942263381844326
q = 0.003814951307056981
nodeCost = 91
costs =
0 77669 65398 43088 71887 12439 81519 21556 19523 58242 63192 20445 19681 52511 82820 7224
77669 0 85952 13838 29342 78256 31831 45959 8151 67026 20196 76116 83809 54635 4011 43020
65398 85952 0 35761 17702 82358 25360 17420 5792 41513 83982 61359 66083 26928 86775 26943
43088 13838 35761 0 35729 36433 27150 45651 57224 52420 86476 56070 57659 74556 72981 15669
71887 29342 17702 35729 0 91173 82855 18454 8250 2430 20641 6656 52647 61889 2068 82851
12439 78256 82358 36433 91173 0 2324 66826 30278 73358 245 73734 9016 25617 29616 23372
81519 31831 25360 27150 82855 2324 0 17920 13750 55476 75191 79110 26125 25264 76076 13602
21556 45959 17420 45651 18454 66826 17920 0 31454 48183 29439 38870 85360 31125 40906 68780
19523 8151 5792 57224 8250 30278 13750 31454 0 98482 56835 58689 34467 70294 16824 18871
58242 67026 41513 52420 2430 73358 55476 48183 98482 0 71174 3710 95894 16615 75165 20514
63192 20196 83982 86476 20641 245 75191 29439 56835 71174 0 48763 43067 8333 48449 32499
20445 76116 61359 56070 6656 73734 79110 38870 58689 3710 48763 0 81429 10294 9957 20285
19681 83809 66083 57659 52647 9016 26125 85360 34467 95894 43067 81429 0 53857 29478 31645
52511 54635 26928 74556 61889 25617 25264 31125 70294 16615 8333 10294 53857 0 2518 1973
82820 4011 86775 72981 2068 29616 76076 40906 16824 75165 48449 9957 29478 2518 0 16617
7224 43020 26943 15669 82851 23372 13602 68780 18871 20514 32499 20285 31645 1973 16617 0 " | |
| 5) | | | | Returns:
"x = {3, 15, 22, 49, 39, 60, 82, 66, 50}
y = {48, 63, 64, 87, 11, 63, 3, 0, 11}
p = 0.008264446916240231
q = 0.007788336306338746
nodeCost = 92
costs =
0 77967 51877 27131 80229 31822 74540 46540 12912
77967 0 66155 16155 8034 35808 22795 60135 11773
51877 66155 0 56078 90246 77516 35786 64769 85614
27131 16155 56078 0 49291 93171 69545 4903 86022
80229 8034 90246 49291 0 40058 43650 40456 82616
31822 35808 77516 93171 40058 0 16614 25592 33985
74540 22795 35786 69545 43650 16614 0 60560 18621
46540 60135 64769 4903 40456 25592 60560 0 51704
12912 11773 85614 86022 82616 33985 18621 51704 0 " | |
| 6) | | | | Returns:
"x = {7, 57, 11, 80, 55, 92}
y = {72, 41, 31, 74, 47, 27}
p = 0.006583582796657616
q = 0.006224951045415638
nodeCost = 96
costs =
0 99469 40545 36911 89709 39339
99469 0 70083 25060 82569 91026
40545 70083 0 48313 90775 28948
36911 25060 48313 0 19095 8917
89709 82569 90775 19095 0 10560
39339 91026 28948 8917 10560 0 " | |
| 7) | | | | Returns:
"x = {75, 66, 2, 91, 30, 67, 66, 7, 43, 74, 72, 33, 2, 57}
y = {27, 28, 93, 98, 22, 44, 80, 85, 97, 69, 46, 26, 91, 57}
p = 0.005119199353456559
q = 0.00233858613264591
nodeCost = 79
costs =
0 73318 23837 13038 48840 44716 51384 45139 605 78222 2383 25761 58234 93207
73318 0 24708 93061 78532 63220 28310 17893 12286 42328 78832 86212 76052 71079
23837 24708 0 80561 98642 19790 21705 93011 9697 17833 46922 22582 508 82797
13038 93061 80561 0 9509 84335 91762 51204 84954 53870 4306 34086 35528 12956
48840 78532 98642 9509 0 15124 90317 14283 64762 90430 80507 90203 95766 53457
44716 63220 19790 84335 15124 0 41504 54854 2471 12175 14678 78092 9080 44902
51384 28310 21705 91762 90317 41504 0 14884 28467 52053 81362 810 37463 38426
45139 17893 93011 51204 14283 54854 14884 0 61643 19029 69225 11994 53928 80220
605 12286 9697 84954 64762 2471 28467 61643 0 8673 69183 37395 16015 41185
78222 42328 17833 53870 90430 12175 52053 19029 8673 0 17901 20715 67703 53976
2383 78832 46922 4306 80507 14678 81362 69225 69183 17901 0 6495 56554 24308
25761 86212 22582 34086 90203 78092 810 11994 37395 20715 6495 0 41860 64687
58234 76052 508 35528 95766 9080 37463 53928 16015 67703 56554 41860 0 33033
93207 71079 82797 12956 53457 44902 38426 80220 41185 53976 24308 64687 33033 0 " | |
| 8) | | | | Returns:
"x = {59, 23, 32, 5, 38, 48, 13, 75, 53, 23, 86, 84, 1, 18, 98, 70, 87, 88, 19}
y = {72, 20, 23, 61, 0, 8, 85, 69, 72, 39, 84, 30, 10, 4, 75, 92, 12, 14, 47}
p = 0.00724697587422273
q = 3.594426926882632E-4
nodeCost = 33
costs =
0 76020 48775 52436 62850 46095 47662 68187 91453 82801 8970 12962 30917 10106 37961 20056 62292 21270 95897
76020 0 86067 21391 5614 84665 64134 24348 34702 95666 50482 30637 77441 89051 90787 54627 41232 67377 31079
48775 86067 0 41241 74226 98781 79751 82602 15650 35707 25502 21453 74002 96942 43165 21290 24956 86115 63628
52436 21391 41241 0 88338 52168 75847 53303 85202 56379 32395 23044 44457 69283 95190 82928 10532 91660 68295
62850 5614 74226 88338 0 84058 9930 48040 96374 90608 60713 22399 37078 75985 11315 79268 30410 95581 98603
46095 84665 98781 52168 84058 0 60896 15736 15828 87583 41779 92443 18468 85657 23163 48104 13422 25329 90558
47662 64134 79751 75847 9930 60896 0 10727 9094 74371 22272 82033 3294 89505 91943 16788 41995 21340 11509
68187 24348 82602 53303 48040 15736 10727 0 8592 456 94418 21096 5065 62864 10863 47783 16906 98426 28435
91453 34702 15650 85202 96374 15828 9094 8592 0 63107 82280 31573 27340 31877 19045 53187 53799 60741 957
82801 95666 35707 56379 90608 87583 74371 456 63107 0 3885 91855 65689 75931 36036 28946 76106 59341 97765
8970 50482 25502 32395 60713 41779 22272 94418 82280 3885 0 23210 58146 74625 62642 25771 61349 95272 28346
12962 30637 21453 23044 22399 92443 82033 21096 31573 91855 23210 0 53240 53844 80656 82869 55348 10227 14790
30917 77441 74002 44457 37078 18468 3294 5065 27340 65689 58146 53240 0 37344 7988 3042 16894 46929 95645
10106 89051 96942 69283 75985 85657 89505 62864 31877 75931 74625 53844 37344 0 82015 54558 60990 47323 72894
37961 90787 43165 95190 11315 23163 91943 10863 19045 36036 62642 80656 7988 82015 0 81404 85568 85386 14113
20056 54627 21290 82928 79268 48104 16788 47783 53187 28946 25771 82869 3042 54558 81404 0 24853 90567 4630
62292 41232 24956 10532 30410 13422 41995 16906 53799 76106 61349 55348 16894 60990 85568 24853 0 18497 22310
21270 67377 86115 91660 95581 25329 21340 98426 60741 59341 95272 10227 46929 47323 85386 90567 18497 0 42999
95897 31079 63628 68295 98603 90558 11509 28435 957 97765 28346 14790 95645 72894 14113 4630 22310 42999 0 " | |
| 9) | | | | Returns:
"x = {98, 13, 32, 71, 81, 57, 33, 71, 2, 62, 33, 36, 60, 25, 0, 39, 74, 63}
y = {46, 50, 98, 99, 10, 53, 52, 56, 69, 97, 66, 31, 67, 71, 18, 56, 70, 74}
p = 0.008420372767857684
q = 0.008580875521429242
nodeCost = 91
costs =
0 1938 17938 89667 34256 82565 76861 83631 61892 65080 62667 48473 38836 79925 72355 90465 52833 54901
1938 0 22624 34739 91459 44177 81269 65812 39621 80560 52651 58100 86157 71183 54465 23620 17618 81501
17938 22624 0 27863 83372 21630 37387 780 97936 86937 22607 10729 40242 63071 11432 15028 31509 87055
89667 34739 27863 0 17581 31996 78747 44222 75622 36459 50317 85008 34504 54641 88035 96912 20273 60262
34256 91459 83372 17581 0 60058 40919 80829 32569 81029 72780 13806 92055 84812 3872 25057 45768 14713
82565 44177 21630 31996 60058 0 15596 68456 97168 95864 23942 88530 39566 46800 96425 63799 78716 70750
76861 81269 37387 78747 40919 15596 0 37241 75619 98363 34941 78223 51753 13622 76679 20763 71354 73555
83631 65812 780 44222 80829 68456 37241 0 61949 92386 83844 30854 73186 93073 9520 12627 33074 83611
61892 39621 97936 75622 32569 97168 75619 61949 0 63787 16190 70021 61037 38069 47231 36845 75340 59116
65080 80560 86937 36459 81029 95864 98363 92386 63787 0 84618 4772 94828 77198 34802 77159 76000 86054
62667 52651 22607 50317 72780 23942 34941 83844 16190 84618 0 60963 96085 29839 34144 70568 68331 50930
48473 58100 10729 85008 13806 88530 78223 30854 70021 4772 60963 0 47190 96335 36845 3227 7848 79810
38836 86157 40242 34504 92055 39566 51753 73186 61037 94828 96085 47190 0 33086 88326 94790 59320 56625
79925 71183 63071 54641 84812 46800 13622 93073 38069 77198 29839 96335 33086 0 75347 34727 73380 20289
72355 54465 11432 88035 3872 96425 76679 9520 47231 34802 34144 36845 88326 75347 0 33177 47237 83013
90465 23620 15028 96912 25057 63799 20763 12627 36845 77159 70568 3227 94790 34727 33177 0 69708 31363
52833 17618 31509 20273 45768 78716 71354 33074 75340 76000 68331 7848 59320 73380 47237 69708 0 73260
54901 81501 87055 60262 14713 70750 73555 83611 59116 86054 50930 79810 56625 20289 83013 31363 73260 0 " | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Consider a necklace composed of marbles of various colors arranged in a circle. The colors are represented by uppercase letters. We can describe a necklace with a string of characters as follows: start with any marble and go through all the marbles in either a clockwise or counter-clockwise direction, until the starting marble is reached again, meanwhile appending to the string the colors of the marbles in the order they are visited. Obviously, there could be many different strings describing the same necklace. For example, the necklace described by the string "CDAB" can also be described by seven other strings (see example 0).
You are given a String necklace containing the description of a necklace. Return the description for that necklace that comes earliest alphabetically.
| | Definition | | Class: | MarbleNecklace | Method: | normalize | Parameters: | String | Returns: | String | Method signature: | String normalize(String necklace) | (be sure your method is public) |
| | | | Constraints | - | necklace will contain between 1 and 50 characters, inclusive. | - | Each character of necklace will be an uppercase letter ('A'-'Z'). | | Examples | 0) | | | | Returns: "ABCD" | This necklace can be described by the eight strings "CDAB", "DABC", "ABCD", "BCDA", "CBAD", "DCBA", "ADCB", "BADC". "ABCD" comes first lexicographically. |
|
| 1) | | | | 2) | | | | 3) | | | "SONBZELGFEQMSULZCNPJODOWPEWLHJFOEW" |
| Returns: "BNOSWEOFJHLWEPWODOJPNCZLUSMQEFGLEZ" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You ran into some trouble when playing your favorite video game. There are N towns on the map, numbered from 0 to N-1. For each pair of distinct towns, there is exactly one way to get from one to the other by following one or more roads. Your enemy has just occupied some of the towns, and your first attempt at blocking him is to remove some of the roads in order to block off communication between every pair of distinct occupied towns. You would like to do this with as little effort as possible.
You will be given a String[] roads describing the roads between towns. Each element of roads is in the form "a b e" (quotes for clarity only), meaning that there is a bidirectional road between towns a and b, and the effort required to destroy the road is e. You will also be given a int[] occupiedTowns containing the towns occupied by your enemy. Return the minimum total effort required to destroy enough roads so that no two distinct occupied towns have a path (direct or indirect) between them.
| | Definition | | Class: | BlockEnemy | Method: | minEffort | Parameters: | int, String[], int[] | Returns: | int | Method signature: | int minEffort(int N, String[] roads, int[] occupiedTowns) | (be sure your method is public) |
| | | | Constraints | - | N will be between 2 and 50, inclusive. | - | roads will contain between 1 and 50 elements, inclusive. | - | Each element of roads will contain between 5 and 50 characters, inclusive. | - | Each element of roads will be in the form "a b e" (quotes for clarity) where a and b are distinct integers between 0 and N-1, inclusive, and e is an integer between 1 and 1,000,000, inclusive. | - | The integers in roads will not contain extra leading zeroes. | - | There will be exactly one way to get from one town to any other, by following one or more roads. | - | occupiedTowns will contain between 1 and 50 elements, inclusive. | - | Each element of occupiedTowns will be between 0 and N-1, inclusive. | - | The elements of occupiedTowns will be distinct. | | Examples | 0) | | | 5 | {"1 0 1", "1 2 2", "0 3 3", "4 0 4"} | {3, 2, 4} |
| Returns: 4 | To make the communication between town 2 and the other occupied towns impossible, we must destroy one of the first two roads. We choose the first one as it requires less effort. Similarly, we choose between the last two roads in order to disconnect towns 3 and 4. The total cost is therefore 4. |
|
| 1) | | | 5 | {"1 0 1", "1 2 2", "0 3 3", "4 0 4"} | {3} |
| Returns: 0 | This is the same map as above. Since there is only one occupied town, we don't need to destroy any road here. |
|
| 2) | | | 12 | {"0 1 3", "2 0 5", "1 3 1", "1 4 8", "1 5 4", "2 6 2",
"4 7 11", "4 8 10", "6 9 7", "6 10 9", "6 11 6"} | {1, 2, 6, 8} |
| Returns: 13 | Towns 1 and 2 are on the path from 6 to 8. We have to destroy the sixth road to disconnect town 6 from 2. We must destroy one of the first two roads to disconnect towns 1 and 2 and we pick the first one. Also, we should destroy one of the roads on the path from 1 to 8 and we choose the fourth road. The total cost is 2 + 3 + 8 = 13. |
|
| 3) | | | 12 | {"0 1 3", "2 0 5", "1 3 1", "1 4 8", "1 5 4", "2 6 2",
"4 7 11", "4 8 10", "6 9 7", "6 10 9", "6 11 6"} | {0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11} |
| Returns: 66 | We have to destroy all roads this time. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Postfix notation (or reverse Polish notation) is a method for writing down arithmetic expressions without brackets, but still maintaining the order of operations. The postfix notation for an expression consisting of a single variable or number consists only of that variable or number. The postfix notation for an expression "A#B" (here A and B are other expressions and '#' is the operator evaluated last in our expression) is obtained by concatenating the postfix notation for A, the postfix notation for B, and '#'.
For example, the postfix notation for "((a+f)*b)*(c*d)" is "af+b*cd**". Another way of looking at the postfix notation is that it is a program for a stack calculator. Every variable or number means "push that variable or number on the stack". Every operator means "pop the two top items from the stack, perform the operation on them, and push the result back on the stack". For example, when evaluating "af+b*cd**", the stack changes as follows: {a}, {a,f}, {a+f}, {a+f,b}, {(a+f)*b}, {(a+f)*b,c}, {(a+f)*b,c,d}, {(a+f)*b,c*d}, {((a+f)*b)*(c*d)}.
You are given an expression in postfix notation, containing only variables and operators. All the operators are binary (take two operands), associative, and commutative. That means for any operator #, expressions A, B, and C, the following properties hold:
- Associativity: A#(B#C)=(A#B)#C.
- Commutativity: A#B=B#A.
That allows you to rearrange operands corresponding to the same operator. For example, using both rules one could rearrange the expression shown above:
- As expression: "((a+f)*b)*(c*d)" can be rearranged to "d*((a+f)*(b*c))".
- In postfix notation: "af+b*cd**" can be rearranged to "daf+bc***".
Your task is to find a postfix expression that can be obtained by rearranging the initial expression using only the associativity and commutativity rules, and having the least RLE-size. The RLE-size of a string is the number of blocks of consecutive equal characters in it (i.e., the RLE-size of "xx+yy+zz+**" is 7).
The input for this problem is given as a String[] expression. Concatenate all elements of that String[] to get the initial expression in postfix notation. Each variable is denoted by a lowercase letter ('a'-'z'), and the operators are denoted by characters '+', '*', '#', '!', '@', '$', '%' and '^'. You are to return the smallest possible RLE-size of a postfix expression that can be obtained from the initial expression by applying some (possibly none) of the above rules. | | Definition | | Class: | PostfixRLE | Method: | getCompressedSize | Parameters: | String[] | Returns: | int | Method signature: | int getCompressedSize(String[] expression) | (be sure your method is public) |
| | | | Notes | - | All the quotes in the problem statement are for clarity only. | | Constraints | - | expression will contain between 1 and 50 elements, inclusive. | - | Each element of expression will contain between 1 and 50 characters, inclusive. | - | Each character in each element of expression will be a lowercase letter ('a'-'z'), '+', '*', '#', '!', '@', '$', '%' or '^'. | - | expression will be a valid arithmetic expression in postfix notation. | | Examples | 0) | | | | Returns: 7 | "daf+bc***" is one of the possible best rearrangements. |
|
| 1) | | | | Returns: 3 | Yields "xxxyyy*****" or "yyyxxx*****". |
|
| 2) | | | | Returns: 13 | No point in changing anything here. |
|
| 3) | | | | 4) | | | {"abc++",
"abc++",
"abc++",
"a",
"b",
"c",
"*",
"*",
"*",
"*",
"*"} |
| Returns: 13 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Before the final round of the TCCC, audience members head to the buffet for lunch.
The buffet line contains a number of food items, in a fixed order.
Those indulging line up at the buffet, each intending to sample a subset of the items available.
It takes each person exactly 10 seconds to help themselves to a food item.
Every 10 seconds, each person moves up as far as they can (without passing by any food they want, of course!). This movement takes no time.
The first person in line moves immediately to each item he wants, in order, spending 10 seconds at each item.
Depending on which items the rest of the people want, they may be able to move up to each item immediately, but also may have to spend some time waiting for the person they are following.
Each person is always either waiting in line before reaching the first food item, standing in front of exactly one food item, or has passed the last food item and is looking for a place to sit.
Two people may not stand in front of the same food item at the same time.
Also, people always remain in the order in which they lined up, and they never move backward in line.
No one ever passes another person in line -- even after they have taken all the food they want, they still exit the buffet in line.
People can only take a food item that they are standing directly in front of.
Anyone who cannot reach the next food item they want (because they are waiting for the person in front of them) will advance as far as possible in line, to avoid blocking the people behind them unnecessarily.
Anxious to get back to the TCCC event, the people wish to get the group through the buffet line as quickly as possible.
Given the list of food items in the buffet and the items that each person wants, return the optimal order in which the people should line up at the buffet so that everyone can get all the food they desire in the least total amount of time.
The food items available at the buffet will be given as a String food.
Each item in food will contain only letters ('a'-'z', 'A'-'Z'), and there will be exactly one space between each pair of adjacent items.
Each item in food will be unique.
The order of items in food is the order they appear in the buffet line, with the first item in food being the first item available.
The items each person wants are given in a String[] cravings.
There will be one element in cravings for each person.
Each element in cravings will be a list of food items found in food, with exactly one space between each food items.
Within each element of cravings, each food item will be unique.
Return a int[] containing the optimal order, where each element is the 0-based index of a person in cravings, and elements are ordered from first to last in line. If there are multiple solutions, return the one that comes first lexicographically. A int[] a1 comes before a int[] a2 lexicographically if, at the first element at which they differ, a1 contains the smaller value.
| | Definition | | Class: | BuffetLine | Method: | order | Parameters: | String, String[] | Returns: | int[] | Method signature: | int[] order(String food, String[] cravings) | (be sure your method is public) |
| | | | Constraints | - | food will contain between 1 and 50 characters, inclusive. | - | food will be formatted as described in the problem statement and contain between 1 and 8 items, inclusive. | - | food will not contain any duplicate items. | - | cravings will contain between 1 and 12 elements, inclusive. | - | Each element of cravings will contain between 0 and 50 characters, inclusive. | - | Each element of cravings will be formatted as described in the problem statement. | - | Each word in each element of cravings will be an item from food. | - | Each element of cravings will not contain any duplicate items. | | Examples | 0) | | | "applesauce beans carrots dates eggplant" | { "beans",
"applesauce",
"dates" } |
| Returns: {2, 0, 1 } | All three people can get through the line in 10 seconds if the person who wants dates goes first, followed by the person who wants beans, and then the person who wants applesauce. Any other order would require 20 or 30 seconds. |
|
| 1) | | | "applesauce beans carrots dates eggplant" | { "beans",
"applesauce",
"beans",
"dates" } |
| Returns: {0, 1, 3, 2 } | In this example there are two people who want beans. Since only one person can take any given food item at once, it will take at least 20 seconds for all 4 people to get through the line. There are several orders that get them through in 20 seconds, and { 0, 1, 3, 2 } is selected according to the tiebreaking rules. |
|
| 2) | | | | 3) | | | "A B C D E F" | { "A C E D",
"A B D E F",
"B C A D",
"B C F D E",
"B D C F" } |
| Returns: {3, 1, 0, 4, 2 } | |
| 4) | | | "A B C" | { "A", "A", "A", "A",
"B", "B", "B", "B",
"C", "C", "C", "C" } |
| Returns: {8, 4, 0, 9, 5, 1, 10, 6, 2, 11, 7, 3 } | |
| 5) | | | "A G E F D B H C" | { "D C A H",
"H B F G A",
"B F G D",
"F C E B A G",
"D H B C",
"B A D F G",
"E B C F D",
"A D H E B G",
"A H B G E C",
"F D A B C",
"B D A G C",
"A G E C F" }
|
| Returns: {0, 4, 1, 10, 9, 6, 2, 3, 11, 8, 7, 5 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Almost every different government in the world has a different method of assigning license plates to vehicles. In any of these systems, it is possible to take all possible plates, arrange them in lexicographical order, and number them, where the first plate is 0, the second plate is 1, etc. Your local government has decided to do this with the license plates that it issues, and right before you leave to eat lunch, you have been assigned this daunting project. So that you can actually have a warm meal today, you have decided to write a program to do this for you.
You will be given a String format, containing the format of the local license plates. Each character of format will be either an uppercase letter ('A'-'Z'), a number ('0'-'9'), a space (' '), a dash ('-'), or the lowercase letters 'u', 'n', or 'a'. If the character is a 'u', it means that any uppercase letter can be used in that place. If the character is an 'n', it means that any number can be used in that place. If the character is an 'a', it means that any uppercase letter or number can be used in that place. If the character is not a lowercase letter, then the character in that spot must be the one used in format. For instance, if the format was "AB-una", then "AB-C52" and "AB-D3G" would be legal license plates, but "AC-D3G", "AB-111", and "AB-DEF" would not.
Given the format, you are to return the n-th earliest (0-indexed) license plate that can be formed in this system. If the n-th license plate cannot be formed using the given format, return an empty String. Note that numbers occur earlier lexicographically than letters (e.g., '4' comes before 'B').
| | Definition | | Class: | LicensePlate | Method: | getPlate | Parameters: | String, int | Returns: | String | Method signature: | String getPlate(String format, int n) | (be sure your method is public) |
| | | | Constraints | - | format will contain between 1 and 50 characters, inclusive. | - | Each character of format will be an uppercase letter ('A'-'Z'), a number ('0'-'9'), a space (' '), a hyphen ('-'), or the lowercase letters 'a', 'n', or 'u'. | - | n will be between 0 and 2^31-1, inclusive. | | Examples | 0) | | | | Returns: "ABC" | The license plates in this format are "ABA", "ABB", "ABC", "ABD", etc. We return the 2nd (0-based) element in this list, which is "ABC". |
|
| 1) | | | | 2) | | | | Returns: "ABC" | Remember that numbers come first in lexicographical order. |
|
| 3) | | | | Returns: "" | There are only 1 possible license plate in this format ("ABC"), so we must return a blank plate. |
|
| 4) | | | | 5) | | | "ABa DEuF-GHuJ KL-aMNaa5390u" | 1345876 |
| Returns: "AB0 DEAF-GHBJ KL-3MNXW5390M" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
The maximum matching of a graph is the largest possible set of edges in which no two edges share a common vertex.
Bipartite graphs are graphs whose nodes can be divided into two sets A and B such that each edge in the graph has one endpoint in A and one endpoint in B. In these graphs, the maximum matching problem can be perfectly solved in polynomial time using maximum flow algorithms.
In this problem you will be given a graph that is almost bipartite and you will have to calculate the maximum matching of that graph. An almost bipartite graph is a graph whose nodes can be divided into two sets A = {a0,...,anA-1} and B = {b0,...,bnB-1} such that all edges have one endpoint in A and one endpoint in B with the exception of the edges from ai to ai+1 that exist for every i between 0 and nA-2, inclusive, and the edges from bi to bi+1 that allways exist for every i between 0 and nB-2, inclusive.
You will be given nA and nB as two ints. The set of edges that have one endpoint in A and one endpoint in B is given as two int[]s edgesA and edgesB. The ith edge has one endpoint aedgesA[ i ] and one endpoint bedgesB[ i ]. Since they are always part of the graph, edges with two endpoints in the same set are implicit. Return the size of the maximum set of edges in this graph that do not share a vertex.
| | Definition | | Class: | AlmostBipartiteMatching | Method: | maxMatching | Parameters: | int, int, int[], int[] | Returns: | int | Method signature: | int maxMatching(int nA, int nB, int[] edgesA, int[] edgesB) | (be sure your method is public) |
| | | | Constraints | - | nA and nB will each be between 1 and 1000, inclusive. | - | edgesA will contain between 0 and 50 elements, inclusive. | - | edgesA and edgesB will contain the same number of elements. | - | Each element of edgesA will be between 0 and nA-1, inclusive. | - | Each element of edgesB will be between 0 and nB-1, inclusive. | | Examples | 0) | | | | Returns: 3 | A perfect matching can be achieved by using all the edges with one endpoint in each set. There are other ways to get the same result. |
|
| 1) | | | | 2) | | | | 3) | | | 13 | 16 | {5,2,6,2,7,2,7,6,2,6,1,3,0,10,12,11,10} | {7,3,10,12,0,0,3,4,7,2,5,2,14,15,1,1,1} |
| Returns: 14 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
This problem statement contains superscripts that may not display correctly in some plugins. If that's the case, use the arena to see them.
You have recently bought a game of extended dominoes. Each domino is a rectangle partitioned into two squares, and each square contains a number between 0 and 9, inclusive. There are exactly 45 pieces - one for each pair of distinct numbers. Pieces may be reversed, so while there is only one piece containing the numbers 1 and 2, it can be used in either of the following ways:
+---+---+ +---+---+
| 1 | 2 | | 2 | 1 |
+---+---+ +---+---+
Unfortunately, some of the pieces have disappeared, but there is still a possible game left: How many ways are there to construct a cycle collection with all the remaining pieces?
A cycle collection is a set of 1 or more cycles that do not share pieces. A cycle is constructed by ordering and orienting the pieces in such a way that the left number of the piece in position i is equal to the right number of the piece in position i-1, and the left number of the piece in the first position is equal to the right number of the piece in the last position (positions are numbered from left to right).
+---+---++---+---++---+---++---+---++---+---++---+---+
| 1 | 2 || 2 | 5 || 5 | 4 || 4 | 2 || 2 | 8 || 8 | 1 |
+---+---++---+---++---+---++---+---++---+---++---+---+
+---+---++---+---++---+---++---+---++---+---++---+---+
| 1 | 2 || 2 | 4 || 4 | 5 || 5 | 2 || 2 | 8 || 8 | 1 |
+---+---++---+---++---+---++---+---++---+---++---+---+
+---+---++---+---++---+---+ +---+---++---+---++---+---+
| 1 | 2 || 2 | 8 || 8 | 1 | | 4 | 5 || 5 | 2 || 2 | 4 |
+---+---++---+---++---+---+ +---+---++---+---++---+---+
The figure above shows three possible cycle collections that use the same set of pieces. We say that each piece is connected to the two pieces that surround it, and the first and last pieces are also connected. Two cycles are considered the same if each piece is connected to the same two other pieces in both cycles. Two cycle collections are the same if they contain the same set of cycles.
You will be given the existing pieces as a String[] pieces, where each element contains exactly two digits representing the two numbers on a single domino. Return the number of distinct cycle collections that can be formed with these pieces.
| | Definition | | Class: | ExtendedDominoes | Method: | countCycles | Parameters: | String[] | Returns: | long | Method signature: | long countCycles(String[] pieces) | (be sure your method is public) |
| | | | Notes | - | Remember that each cycle collection must use all the pieces, and no two cycles in a collection can share pieces. | | Constraints | - | pieces will contain between 1 and 45 elements, inclusive. | - | Each element of pieces will contain exactly 2 characters. | - | Each character of each element of pieces will be a digit ('0'-'9'). | - | In each element of pieces the value of its first character will be less than the value of its second character. | - | No two elements of pieces will be equal. | - | The number of cycles for pieces will be less than 263. | | Examples | 0) | | | {"12","25","45","24","28","18"} |
| Returns: 3 | The example in the problem statement. |
|
| 1) | | | {"01","12","23","34","45"} |
| Returns: 0 | There is no way to form a cycle here. |
|
| 2) | | | {"09","12","24","14","57","79","05"} |
| Returns: 1 | The only possibility is:
+---+---++---+---++---+---++---+---+ +---+---++---+---++---+---+
| 0 | 9 || 9 | 7 || 7 | 5 || 5 | 0 | | 1 | 2 || 2 | 4 || 4 | 1 |
+---+---++---+---++---+---++---+---+ +---+---++---+---++---+---+
|
|
| 3) | | | {"34","35","36","37","45","46","47","56","57","67"} |
| Returns: 243 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Your friend likes riding his bike on the hills outside your town. He asked you to help him count the number of routes he can take. There are several locations on the hills, some of them directly connected by paths (you can take each path only in one given direction). He would like to start at one of the locations given in startPoints and end at location endPoint. Return the number of distinct routes that he can use to do that. If the number is larger than n or infinite, return -1.
The definition of a route is as follows:
- A legal route is a sequence of locations starting with one from startPoints and ending with endPoint. Each location on the route other than the last one has a path leading to the next consecutive location.
- Two routes are distinct if they each have a different sequence of locations.
- A route can have any length.
- Any location (including endPoint) may be present multiple times on the route.
You are given all locations and connections between them in the String[] paths. The j-th character of the i-th element of paths is '1' (one) if there is a direct one-way path from location i to location j, and '0' (zero) otherwise.
| | Definition | | Class: | BikeRiding | Method: | countRoutes | Parameters: | String[], int[], int, int | Returns: | int | Method signature: | int countRoutes(String[] paths, int[] startPoints, int endPoint, int n) | (be sure your method is public) |
| | | | Constraints | - | paths will contain between 2 and 50 elements, inclusive (let k be this number). | - | Each element of paths will contain exactly k characters. | - | Each element of paths will contain only '0' (zero) and '1' (one) characters. | - | endPoint and each element of startPoints will be between 0 and k-1. | - | Each element in startPoints will be distinct. | - | None of the elements in startPoints will be equal to endPoint | - | n will be between 2 and 10^9, inclusive. | | Examples | 0) | | | {"011" ,"001", "000"} | {0,1} | 2 | 5 |
| Returns: 3 | There is one possible route from location 1, and two from location 0. |
|
| 1) | | | {"01000",
"00100",
"00010",
"01001",
"00000"} | {0} | 4 | 10 |
| Returns: -1 | The number of routes is infinite. |
|
| 2) | | | {"000111000000000","000111000000000","000111000000000",
"000000111000000","000000111000000","000000111000000",
"000000000111000","000000000111000","000000000111000",
"000000000000111","000000000000111","000000000000111",
"000000000000001","000000000000001","000000000000000"} | {0,1,2} | 14 | 1000 |
| Returns: 243 | |
| 3) | | | {"010", "100", "001"} | {0} | 2 | 10 |
| Returns: 0 | There is no possible route. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | There is a matrix with 9 rows and 9 columns. Each cell of the matrix is either black or white. With a single repaint operation, you can repaint all the cells in a single row or column black if the row or column already contains at least 5 black cells. Your goal is to make all the cells in the matrix black using a minimal number of repaint operations.
You will be given a String[] matrix, where the jth character of the ith element represents the cell at row i, column j. Black cells will be written as '1' (one), and white cells will be written as '0' (zero). Return the minimal number of repaint operations required to make all the cells black, or -1 if this is impossible.
| | Definition | | Class: | MatrixPainting | Method: | countRepaints | Parameters: | String[] | Returns: | int | Method signature: | int countRepaints(String[] matrix) | (be sure your method is public) |
| | | | Constraints | - | matrix will contain exactly 9 elements. | - | Each element of matrix will contain exactly 9 characters. | - | Each element of matrix will consist of '0' and '1' characters only. | | Examples | 0) | | | {"001111111",
"011111111",
"011111111",
"011111111",
"011111111",
"101111111",
"101111111",
"101111111",
"101111111"}
|
| Returns: 3 | First, you should repaint the first row. After that, you can repaint the first and the second column. |
|
| 1) | | | {"011111111",
"101111111",
"110111111",
"111011111",
"111101111",
"111110111",
"111111011",
"111111101",
"111111110"}
|
| Returns: 9 | Each white cell must be repainted separately. |
|
| 2) | | | {"000000001",
"000000011",
"000000111",
"000001111",
"000011111",
"000011110",
"000011100",
"000011000",
"000010000"}
|
| Returns: 14 | After repainting the 5 rightmost columns, you will be able to repaint the rows. |
|
| 3) | | | {"000000001",
"000000011",
"000000111",
"000001111",
"000011111",
"000011110",
"000011100",
"000011000",
"000000000"}
|
| Returns: -1 | |
| 4) | | | {"011111111",
"010001001",
"111111101",
"011111111",
"101010100",
"011111111",
"111111101",
"111011101",
"011111111"}
|
| Returns: 5 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You are given a directed graph. The graph may contain loops, and multiple edges may exist between pairs of vertices. You can merge a pair of vertices into a single vertex. When a pair of vertices is merged, outgoing and incoming edges from both vertices become outgoing and incoming edges, respectively, of the resulting vertex.
For example, consider a graph with four vertices {a, b, c, d} and three edges {a->b, c->b, c->d}. If we merge vertices b and c, the resulting graph will have three vertices {a, bc, d} and three edges {a->bc, bc->bc, bc->d}.
A graph is a cycle if its vertices can be ordered v0, v1, ..., vn-1 (where n is the total number of vertices) such that the following two properties are satisfied:
- Every edge in the graph goes from vi to v(i+1)%n.
- For all i between 0 and n-1, inclusive, there exists at least one edge that goes from vi to v(i+1)%n.
You are given a String[] adj. The ith element of adj is a space delimited list of integers representing all the outgoing edges from vertex i. Each integer represents the target vertex of an outgoing edge. Return the minimal number of merge operations necessary to convert the given graph into a cycle. If a solution doesn't exist, return the number of vertices in the given graph instead.
| | Definition | | Class: | MergingGraph | Method: | distanceToCycle | Parameters: | String[] | Returns: | int | Method signature: | int distanceToCycle(String[] adj) | (be sure your method is public) |
| | | | Constraints | - | adj will contain between 1 and 50 elements, inclusive. | - | Each element of adj will contain between 0 and 50 characters, inclusive. | - | Each element of adj will be a single space separated list of integers. | - | Each number in each element of adj will be between 0 and (n - 1) inclusive, where n is the number of elements in adj. | - | Each number in each element of adj will contain no leading zeroes. | | Examples | 0) | | | | Returns: 0 | This graph is already a cycle. |
|
| 1) | | | | Returns: 2 | The resulting cycle is a loop. |
|
| 2) | | | | Returns: 2 | The resulting cycle has 2 vertices. |
|
| 3) | | | {"2", "5 4", "", "0", "0", "0 0"} |
| Returns: 3 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are given a int[] data, and you must order the elements of data such that no two consecutive elements have consecutive values. In other words, result[i] + 1 != result[i + 1] for all i, where 0 <= i < n-1, and n is the number of elements in data. If more than one ordering exists, return the one that comes first lexicographically. | | Definition | | Class: | WeirdSort | Method: | sortArray | Parameters: | int[] | Returns: | int[] | Method signature: | int[] sortArray(int[] data) | (be sure your method is public) |
| | | | Constraints | - | data will contain between 1 and 50 elements, inclusive. | - | Each element of data will be between 0 and 1000, inclusive. | | Examples | 0) | | | | Returns: {2, 1 } | Only one possible ordering exists. |
|
| 1) | | | | Returns: {1, 3, 2 } | There are only three possible orderings: {1, 3, 2}, {2, 1, 3} and {3, 2, 1}. The first one is lexicographically smallest. |
|
| 2) | | | {1, 1, 1, 1, 2, 2, 2, 2, 2} |
| Returns: {2, 2, 2, 2, 2, 1, 1, 1, 1 } | All 2's should be before 1's. |
|
| 3) | | | | Returns: {1, 3, 2, 4, 6, 5 } | |
| 4) | | | | Returns: {1, 1, 3, 3, 2, 2 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You have just been hired by a cartography company, and your first task is to help them with a map they are trying to make. The owner of the company thinks it should always be possible to color a map using only four colors (such that no touching states have the same color), but so far they have been unable to do so with the map of Familyland. This is quite important to him, as he can print maps using four colors of ink for only $0.00001 per square millimeter. Each additional ink, however, has a base cost of $N/100 and a usage cost of $N/100000 per square millimeter (where N is the number of the ink, so N=5 is the first ink that is more expensive).
The company will be printing 100mm x 100mm maps of Familyland, so you can see that the costs will add up quickly if many additional inks are needed. If the map can be made with only 4 inks, it will cost only $0.10 each to make, but if a 5th ink were needed, even on only 1 square millimeter of the map, the cost would rise to $0.15004 (note that you still only pay $0.00001/mm^2 for the first four inks).
The problem with this particular map is that the country of Familyland has a representative government, but the citizens would not put up with anyone but a family member being their representative. To deal with this, Familyland declared each family to be its own state. Since not all family members live next to one another, the states are not contiguous. Of course, in making the map, each state must be entirely one color.
If you want to keep your new job, you will need to come up with a coloring of the map such that it can be printed as cheaply as possible. Your score on each test case will be the cost in dollars of printing the map using the coloring you return. Your total score (the one displayed in the division summary) will be the sum over all testcases of the lowest cost anyone has achieved for the case divided by your cost for the case. In the unlikely event of a true tie (as computed using high precision numerics), the competitor with the lowest total runtime on all cases will be declared the winner.
You will be given the area of each state (in square millimeters in map scale), and an adjacency matrix where character i of string j is '1' if states i and j are adjacent and '0' if they are not. Character i of string i will always be '0'. You must return an ink color for each state such that no two adjacent states have the same color. In the event that your returned coloring is invalid (you return a different number of colors than there are states, or you color two adjacent states the same), or your program exceeds the 30 second time limit, you will receive a 0 on the case.
The test cases will be generated as follows (unless otherwise stated, all random choices are uniform and independent and ranges are inclusive):
- The number of states (S) will be randomly chosen between 10 and 500.
- The number of properties will be randomly chosen between S and 4*S.
- For the first S properties, a random x and y will be chosen between 0 and 99 (assuring that no two properties are at the same location). The pixel at the x and y coordinate on the 100x100 map is marked as belonging to the ith state.
- Each remaining property is placed on the map in the same manner, except that a random state between 0 and S-1 is assigned to the x and y coordinate on the map.
- The remaining map pixels are assigned to states by randomly picking an unassigned map pixel that borders an assigned pixel, randomly choosing one of the assigned pixels that borders it, and assigning that pixel's state to the unassigned pixel. This process continues until the map is filled.
- The adjacency matrix and the array of state areas are constructed from this map.
| | Definition | | Class: | MapMaker | Method: | colorStates | Parameters: | int[], String[] | Returns: | int[] | Method signature: | int[] colorStates(int[] area, String[] adj) | (be sure your method is public) |
| | | | Notes | - | The time limit for each test case is 30 seconds. | - | There are 50 non-example test cases. | - | The memory limit is 1GB, and the thread limit is 32. | - | There will be between 10 and 500 states inclusive. (chosen uniformly) | - | Returned colors must all be >= 1. | - | To facilitate testing, the values of S and P were not chosen randomly for example cases 0 through 3. | | Examples | 0) | | | | Returns:
"</pre>10 states, 10 properties<br>
<br>
Areas:<br>
1477, 538, 1429, 1106, 381, 685, 1605, 224, 1807, 748 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>0000011010
0000000111
0000101000
0000011000
0010001101
1001001000
1011110011
0100100001
1100001001
0100101110
</pre>" |
Here is an image of the generated map that was used to construct the adjacency matrix and calculate the areas:
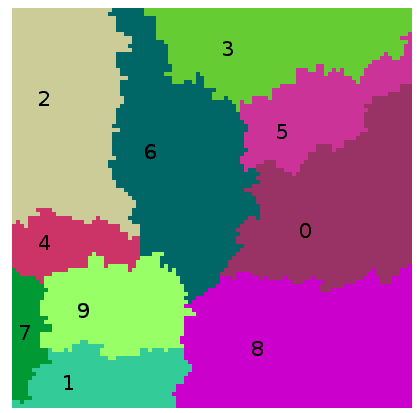 |
|
| 1) | | | | Returns:
"</pre>10 states, 20 properties<br>
<br>
Areas:<br>
1403, 777, 954, 2302, 918, 2217, 495, 282, 91, 561 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>0111111001
1011110001
1101100000
1110110100
1111010010
1101101111
1000010101
0001011000
0000110000
1100011000
</pre>" |
Here is an image of the generated map that was used to construct the adjacency matrix and calculate the areas:
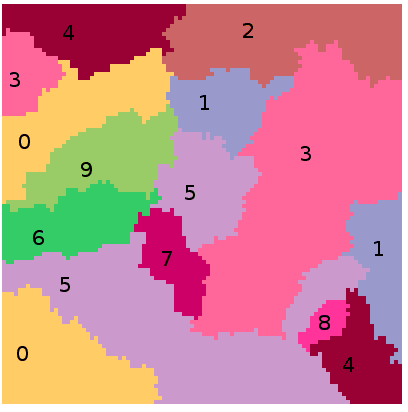 |
|
| 2) | | | | Returns:
"</pre>10 states, 40 properties<br>
<br>
Areas:<br>
845, 1484, 725, 755, 620, 369, 1084, 619, 1238, 2261 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>0111100111
1001111111
1001000111
1110011111
1100011011
0101101001
0101110111
1111001011
1111101101
1111111110
</pre>" |
Here is an image of the generated map that was used to construct the adjacency matrix and calculate the areas:
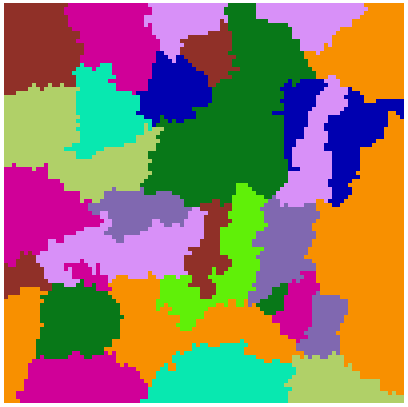 |
|
| 3) | | | | Returns:
"</pre>500 states, 2000 properties<br>
<br>
Areas:<br>
3, 29, 51, 18, 48, 14, 20, 21, 37, 14, 15, 24, 34, 17, 16,<br>
9, 11, 17, 27, 27, 1, 27, 39, 31, 4, 20, 9, 24, 12, 11,<br>
31, 9, 43, 5, 34, 11, 38, 25, 30, 11, 20, 17, 32, 35, 25,<br>
13, 3, 18, 17, 26, 38, 7, 46, 17, 13, 4, 11, 17, 6, 1,<br>
11, 28, 23, 13, 31, 23, 31, 11, 11, 39, 17, 21, 43, 12, 44,<br>
23, 12, 31, 10, 21, 31, 17, 35, 9, 8, 29, 4, 22, 28, 9,<br>
25, 25, 38, 20, 20, 4, 35, 9, 20, 16, 17, 14, 24, 10, 8,<br>
22, 7, 20, 23, 19, 13, 19, 28, 15, 16, 15, 19, 11, 32, 8,<br>
11, 10, 32, 40, 27, 6, 22, 31, 16, 14, 17, 14, 17, 18, 19,<br>
12, 21, 11, 13, 10, 14, 38, 24, 20, 27, 21, 24, 55, 20, 13,<br>
18, 24, 35, 35, 27, 8, 24, 10, 17, 22, 29, 13, 10, 10, 10,<br>
43, 12, 18, 11, 14, 10, 9, 24, 29, 14, 20, 31, 30, 3, 15,<br>
15, 19, 5, 20, 4, 47, 15, 26, 30, 23, 30, 18, 44, 20, 13,<br>
11, 26, 23, 21, 10, 23, 16, 14, 13, 24, 17, 9, 27, 20, 46,<br>
20, 10, 15, 14, 14, 14, 7, 40, 21, 39, 58, 8, 22, 11, 28,<br>
11, 32, 34, 10, 30, 6, 25, 19, 33, 3, 19, 32, 23, 35, 5,<br>
19, 14, 11, 24, 26, 36, 19, 18, 20, 17, 24, 23, 19, 9, 34,<br>
12, 29, 5, 18, 41, 38, 17, 34, 29, 19, 22, 25, 7, 47, 19,<br>
6, 10, 5, 10, 17, 18, 24, 4, 15, 24, 19, 3, 19, 4, 15,<br>
11, 13, 13, 15, 28, 16, 8, 20, 12, 13, 34, 8, 6, 22, 16,<br>
35, 9, 26, 30, 16, 39, 19, 9, 42, 36, 16, 40, 15, 8, 27,<br>
6, 21, 18, 20, 20, 14, 24, 15, 17, 25, 9, 11, 13, 23, 17,<br>
45, 4, 8, 12, 62, 10, 15, 44, 9, 33, 25, 38, 22, 21, 20,<br>
30, 12, 21, 6, 34, 10, 19, 43, 14, 16, 33, 17, 5, 18, 5,<br>
27, 16, 34, 38, 21, 11, 6, 7, 40, 5, 11, 30, 25, 21, 30,<br>
29, 32, 27, 4, 22, 9, 10, 7, 1, 12, 24, 28, 35, 13, 30,<br>
19, 5, 28, 22, 16, 17, 18, 27, 9, 21, 22, 2, 13, 5, 47,<br>
16, 17, 23, 29, 17, 23, 8, 2, 13, 29, 18, 13, 11, 33, 8,<br>
11, 24, 23, 19, 13, 13, 19, 33, 40, 17, 32, 19, 18, 29, 7,<br>
21, 30, 14, 12, 12, 21, 17, 14, 8, 14, 15, 33, 13, 12, 45,<br>
19, 12, 14, 8, 14, 8, 23, 54, 13, 22, 26, 46, 8, 23, 9,<br>
17, 12, 15, 23, 16, 28, 33, 18, 14, 30, 44, 2, 30, 27, 14,<br>
12, 33, 13, 41, 8, 10, 21, 9, 15, 38, 8, 22, 13, 4, 14,<br>
36, 12, 33, 30, 7 " | |
| 4) | | | | Returns:
"</pre>358 states, 1000 properties<br>
<br>
Areas:<br>
15, 50, 15, 15, 57, 52, 19, 28, 10, 31, 15, 26, 47, 45, 40,<br>
15, 9, 15, 33, 95, 19, 60, 24, 12, 32, 9, 49, 33, 31, 25,<br>
5, 1, 51, 54, 35, 46, 38, 30, 19, 78, 113, 29, 31, 25, 42,<br>
11, 12, 21, 16, 14, 48, 33, 15, 12, 16, 11, 25, 18, 4, 36,<br>
30, 127, 31, 43, 32, 28, 38, 31, 11, 48, 17, 14, 60, 37, 14,<br>
40, 13, 31, 48, 19, 13, 12, 13, 23, 35, 21, 17, 16, 15, 19,<br>
14, 33, 20, 29, 40, 64, 55, 4, 17, 21, 28, 29, 11, 77, 63,<br>
15, 5, 13, 1, 50, 24, 52, 42, 21, 16, 31, 23, 25, 28, 17,<br>
26, 38, 4, 23, 12, 27, 30, 29, 28, 7, 2, 21, 28, 26, 15,<br>
15, 77, 8, 5, 67, 18, 43, 25, 35, 10, 37, 16, 52, 16, 34,<br>
9, 14, 33, 41, 6, 32, 37, 44, 14, 14, 73, 20, 55, 27, 19,<br>
45, 45, 43, 28, 18, 23, 13, 13, 30, 46, 11, 11, 3, 5, 18,<br>
25, 79, 21, 8, 14, 48, 19, 36, 18, 14, 4, 25, 10, 39, 7,<br>
32, 33, 15, 16, 15, 11, 26, 9, 14, 21, 46, 9, 30, 37, 19,<br>
15, 19, 15, 26, 48, 18, 33, 37, 32, 12, 7, 53, 20, 20, 7,<br>
41, 18, 23, 30, 25, 14, 31, 14, 17, 13, 25, 21, 62, 40, 25,<br>
8, 38, 22, 13, 59, 16, 26, 49, 3, 13, 17, 16, 23, 22, 34,<br>
24, 10, 19, 50, 35, 29, 5, 6, 59, 9, 52, 13, 7, 41, 35,<br>
12, 23, 77, 34, 20, 4, 31, 29, 25, 26, 13, 54, 66, 36, 29,<br>
47, 26, 56, 25, 51, 30, 29, 1, 56, 37, 34, 28, 24, 21, 20,<br>
12, 40, 13, 8, 3, 10, 3, 36, 18, 29, 47, 26, 29, 52, 38,<br>
10, 13, 4, 46, 25, 41, 22, 8, 16, 30, 35, 50, 10, 17, 37,<br>
25, 14, 41, 23, 21, 36, 44, 63, 54, 9, 28, 29, 52, 6, 28,<br>
25, 17, 36, 30, 57, 21, 107, 31, 20, 47, 30, 9, 48 " | |
| 5) | | | | Returns:
"</pre>232 states, 784 properties<br>
<br>
Areas:<br>
90, 40, 9, 97, 42, 94, 25, 16, 21, 43, 73, 44, 25, 77, 67,<br>
60, 79, 43, 50, 9, 18, 24, 7, 60, 69, 7, 106, 63, 39, 46,<br>
50, 82, 42, 33, 15, 53, 63, 98, 78, 59, 51, 14, 56, 110, 59,<br>
37, 33, 53, 93, 48, 18, 25, 61, 11, 143, 42, 33, 24, 63, 5,<br>
67, 3, 99, 107, 16, 33, 71, 11, 6, 32, 24, 37, 60, 9, 71,<br>
51, 28, 34, 2, 79, 44, 36, 51, 3, 42, 44, 102, 22, 127, 14,<br>
26, 29, 15, 79, 15, 62, 20, 17, 39, 28, 21, 31, 54, 49, 7,<br>
17, 41, 14, 22, 28, 39, 31, 41, 33, 62, 88, 30, 55, 80, 8,<br>
59, 45, 22, 52, 49, 22, 25, 31, 51, 17, 61, 10, 73, 15, 38,<br>
33, 62, 55, 21, 103, 1, 20, 20, 52, 66, 62, 36, 14, 91, 20,<br>
129, 74, 22, 50, 72, 21, 31, 22, 30, 15, 47, 31, 19, 71, 63,<br>
41, 50, 19, 52, 44, 13, 46, 32, 57, 60, 14, 70, 23, 33, 15,<br>
48, 26, 8, 47, 23, 74, 61, 102, 15, 31, 6, 13, 10, 8, 71,<br>
95, 34, 45, 47, 75, 64, 5, 33, 35, 20, 24, 9, 28, 68, 50,<br>
37, 14, 37, 33, 51, 71, 11, 86, 68, 14, 56, 51, 13, 26, 38,<br>
65, 24, 57, 73, 61, 44, 2 " | |
| 6) | | | | Returns:
"</pre>420 states, 1393 properties<br>
<br>
Areas:<br>
23, 9, 33, 32, 43, 24, 12, 18, 22, 31, 17, 14, 11, 48, 24,<br>
26, 10, 22, 33, 17, 6, 25, 28, 30, 24, 26, 63, 71, 43, 17,<br>
50, 15, 22, 46, 6, 15, 16, 34, 13, 20, 12, 3, 22, 15, 47,<br>
39, 29, 42, 30, 40, 19, 42, 19, 30, 36, 27, 2, 29, 44, 22,<br>
13, 8, 24, 30, 37, 19, 52, 14, 15, 33, 7, 22, 25, 30, 35,<br>
13, 3, 30, 33, 32, 11, 31, 25, 38, 45, 10, 12, 45, 25, 14,<br>
41, 17, 16, 28, 16, 64, 27, 30, 17, 8, 19, 9, 1, 61, 14,<br>
4, 8, 15, 24, 6, 38, 26, 14, 4, 16, 22, 15, 20, 16, 2,<br>
14, 12, 1, 6, 8, 8, 25, 37, 8, 18, 52, 8, 21, 40, 31,<br>
4, 16, 16, 17, 17, 29, 18, 9, 18, 7, 18, 33, 69, 20, 38,<br>
15, 8, 47, 22, 36, 18, 30, 4, 16, 18, 19, 21, 19, 25, 22,<br>
29, 7, 26, 3, 18, 31, 27, 29, 32, 45, 45, 21, 37, 10, 37,<br>
17, 10, 25, 25, 15, 7, 9, 23, 11, 45, 8, 18, 9, 8, 46,<br>
21, 20, 47, 30, 10, 20, 8, 7, 12, 18, 26, 25, 21, 18, 25,<br>
27, 35, 24, 15, 12, 32, 4, 14, 19, 11, 31, 12, 28, 22, 22,<br>
36, 20, 64, 14, 10, 14, 23, 5, 31, 10, 16, 28, 19, 35, 29,<br>
4, 47, 29, 1, 18, 13, 18, 34, 45, 6, 56, 41, 30, 14, 47,<br>
23, 13, 12, 16, 20, 25, 29, 12, 69, 18, 11, 5, 41, 9, 32,<br>
7, 37, 34, 6, 16, 7, 15, 44, 15, 7, 54, 20, 22, 33, 33,<br>
22, 27, 25, 13, 22, 6, 33, 17, 12, 31, 33, 26, 13, 13, 13,<br>
24, 7, 36, 19, 7, 63, 43, 32, 1, 13, 25, 19, 16, 33, 53,<br>
27, 39, 9, 12, 45, 13, 23, 21, 10, 48, 4, 51, 19, 36, 19,<br>
34, 9, 18, 30, 14, 36, 51, 10, 15, 35, 22, 19, 29, 38, 25,<br>
22, 23, 13, 66, 20, 30, 8, 14, 9, 47, 36, 11, 16, 5, 22,<br>
19, 25, 12, 55, 26, 74, 53, 36, 26, 17, 19, 46, 47, 3, 4,<br>
39, 9, 24, 25, 12, 21, 30, 29, 56, 18, 20, 38, 5, 25, 45,<br>
39, 24, 26, 63, 33, 34, 36, 3, 19, 27, 2, 23, 18, 43, 33,<br>
24, 13, 5, 18, 56, 24, 30, 32, 8, 27, 16, 15, 10, 24, 31 " | |
| 7) | | | | Returns:
"</pre>92 states, 286 properties<br>
<br>
Areas:<br>
100, 165, 136, 65, 94, 95, 35, 48, 2, 104, 108, 128, 160, 58, 52,<br>
64, 231, 107, 52, 166, 114, 69, 93, 59, 123, 211, 91, 215, 73, 200,<br>
438, 193, 136, 31, 58, 64, 77, 76, 86, 98, 42, 47, 132, 152, 66,<br>
92, 101, 40, 118, 64, 53, 76, 142, 118, 89, 113, 94, 89, 136, 39,<br>
224, 83, 162, 67, 182, 65, 129, 155, 175, 127, 59, 107, 42, 68, 72,<br>
293, 44, 14, 151, 83, 200, 35, 14, 101, 242, 77, 75, 142, 70, 120,<br>
83, 261 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>00000000000000000010001000000000100000100000000000001100000000110000001000000000000000000010
00000000000000001100011000000111000001100000100011000000001000101000001000010000000110000000
00010001000000000000000010000010100000000010000000000001010010010010000100010000000000001001
00100000000000001000000010000010000000000000000000010100000000001000000000010000000000000001
00000000001001000100101000000010000000000000000000000000000000101000000000000000010000001001
00000000000000000000000000110000000000000010000100001010000000000000100000000010000000000110
00000000000100000001000000000000000000000000001000000000000000010000000000010000000010000001
00100000000000001000001010100001000100000000000000000001000000000000000100010000000000000001
00000000000000000000000000000000000000000000000000000000000000000000000000000010000000010000
00000000000000001011100101000001011000000010001000010100000100000000000000100010000000010100
00001000000001101000010010000000000000000001010001001100100110000100000100000010010000010101
00000010000011010001000000001010000000000101001000000000101010001010000000000000000000000001
00000000000101100001000001010011000000000000000010000001100000000001000100000000100001100000
00001000001110000000000000000010000000000000010010001000000000100000000000000000000000000001
00000000001010000000000000010010000000000001000000000001100000000000000100000000000000000000
00000000000100000000000000000100010000000000000000000000000001000010000000010100000100000001
01010001011000000001001010000010110100000000000011010111000000100000111000010000101010100111
01001000000000000010000000000010000000001000000001000000001011001000000000010000000010000010
10000000010000000100000000010001000000001000000000000000100000000100001000000010000000000010
00000010010110001000000000010100100000000001000000000000011010010000000000010010000010010010
00001000010000000000001001010010000000010010000000000000001111000000000000000010000000000000
01000000001000000000000100000100000000100000001000000100000100000100000000000000000000010001
11001001000000001000100001000011100001000000000000001011001000100001010000000000000000000001
00000000010000000000010001000000001100100010001000010000000000000000000000000000000000000000
00110001001000001000000000100011100000000010010001000000000010000000110100000001000000000001
00000000010010000000101100010000100100000010111000011010001010100001000100000000101010010000
00000101000000000000000010010001000100000000000101100000000010000000000011010000000000100000
00000100000010100011100001100011000000100011000000000010101001000001000000000010000010110000
00000000000100000000000000000000000000000000000000001000000010100010000000000000100001100101
01000000000000010001010000000000000000000000000010000010000010001010100100000001100000100001
01111000000111101100101010010001000000111111000110011111110010001001101110100011100110001000
01000001010010000010001010110010100001000011100010001101101000000000001011010010000100100011
10100000000000001001001011000001000000100000000000000000000000110000001001010010000010000011
00000000010000011000000000000000000000000000000010000000000000000000000000010010000001000000
00000000010000000000000100000000000100000010001000001000000110000000000000000000000010000100
00000001000000001000000101100000001000000000000001000000000000000000000000000000100000000100
00000000000000000000000000000000000000010000000010100110000000000100000000000011000000000000
01000000000000000000001000000001000000000000010000000001000000000010111000001001000000000001
11000000000000000000010100010010100000000010100000000110001000000011011100000000000000110000
00000000000000000000100000000010000010000010000110100000000000000000000001010000000000000001
00000000000000000110000000000010000000000010000000000000000000000100000000000000000000000000
00000000000100000000000000000010000000000011000000000000000001001000000000000000000010000000
00100100010000000000100111010011001000111101000100000100000000000110001100000011000010001010
00000000001100100001000000010011000000000110010000000000100000001001000000010010000100000000
01000000000000000000000001000001000000100000000000000000001000001000010000000000000100010000
00000000001001000000000011000000000001000001000000000000100000000001100000001000100110000101
00000010010100000000010101000000001000000000000000000000001010010000000000000000001010100001
00000100000000000000000000100010000000010010000000000000000000000000000010000000000000001010
01000000000011001000000000000111010010010000000000001001100000101000101000000000000001000101
01000000001000001100000010100000000100000000000000000000000000000000000000000000000000000001
00000000000000000000000000100000000010010000000000000000000010001100000001000000000000000000
00010000010000001000000101000010000000000000000000001100010000000100000000000000000010010000
10000100001001000000001001001011001000000000000010010010000010100100010000010011000011000110
10010000011000001000010000000011000010100010000000010000100000001100101101100001000000010000
00000100000000001000001001010110000010100000000000001000000000000000100000010011000001100000
00100001000010101000001000000011000001000000000010000000000000000000000000010000100000000001
00000000001110100010000000010011000000000001010010000100000000100100101100000010000011100101
00100000000000000001000000000010000000000000000000010000000000000000000000010000000010000000
01000000000100000101101001010001000000100000101000000000000010000001000000000000000000000000
00000000011000000000110000000000001000000000000000000000000010000100000000000000000000010000
00100000001100000101100011101110001000000000001000101000001100011110000100000000110000110001
00000000000000010100100000010000000000000100000000000000000000001001000000000000000110001000
11001000000001001000001001001000100000000000000010001000100000000001000010010010100010001000
10100010000000000001000000000000100000000000001000000000000010000000000000000000000000000000
01011000000100000100000000000110000000000101100010100100000011000100010000000000110100000010
00000000001000000010010000000000000010001010000000111100100110001000010000000000000000010011
00100000000100010000000000001100000001100010000000000000000010000000100100000100100000000001
00000000000010000000001001010010000000100001010000000000001001100000001000010000100000001000
00000100000000001000000010000110000001000000010010000110100000000010010000000000000000000100
00000000000000001000001010000000000001100000100000001000000000001100101000000001000000010010
11000000000000001010000000000011100001100010000010000100100000000001010100001000000011000010
00100001001010100000000011000110000000100010000000000100100010000010001000000000000011100101
00000000000000000000000000100011000000000000000100000000000000100000000000000000000010001000
00000000000000000000000000100001100000010000000000100100000000000000000000110010000000000000
00000000010000000000000000000010000000000000000000000100000000000000000001000010000100010000
01110011000000011101000000100001110000010001000000001011010000100001000001000000000011000101
00000000000000000000000000000000000001000000010000000000000000000000001000000001000000000000
00000000000000010000000000000000000000000000000000000000000000000010000000000000100100000000
00000100111000000011100000010011110010000011000000001010100000100000000001100000000001010101
00000000000000000000000010000110000011000010000000001110000000000000010000001000100000000000
00000000000010001000000001001110000100000000010000000001000010101011000000000101000101000100
00001000001000000000000000000000000000000000000000000000000010001000000000000000000000000000
00000000000000001000000001000000000000000000001000000000000000000000000000000000000000100000
01000000000000010000000000000011000000000001110000000000000001001000000000100100100000010000
01000010000000001101000001010010101000000110011000011000110001100000001110010000000000001010
00000000000010000000000000001000010000000000000010001010100000000000001100010010100000100100
00000000000010001000000000111101000000100000001000000010100010000000000100000000001001000101
00000000111000000001010001010000000000100000100000010100000110000100010000100010000100000000
00101000000000000000000000000010000000000010000100000000000001100001000010000000000010000010
00000100011000001000000000001000001100000000010010001000100000000000100100010010100001100001
10000100000000001111000000000001100000000010000100001000000000001100011000000000000010001000
00111011001101011000011010001101100001010000011011000001100010000110000100010010000000100100
</pre>" | |
| 8) | | | | Returns:
"</pre>207 states, 439 properties<br>
<br>
Areas:<br>
28, 71, 9, 73, 52, 145, 42, 42, 9, 51, 38, 8, 21, 44, 51,<br>
75, 77, 21, 64, 107, 51, 121, 53, 25, 42, 94, 38, 2, 11, 22,<br>
33, 44, 1, 22, 31, 60, 26, 79, 19, 51, 27, 60, 106, 98, 5,<br>
27, 33, 45, 95, 95, 58, 50, 29, 19, 16, 8, 48, 42, 32, 52,<br>
50, 41, 64, 57, 69, 46, 80, 59, 51, 26, 13, 128, 30, 7, 106,<br>
29, 20, 11, 32, 7, 81, 15, 20, 119, 31, 35, 199, 9, 16, 81,<br>
65, 16, 54, 42, 30, 82, 65, 95, 84, 38, 35, 52, 22, 24, 35,<br>
97, 44, 8, 76, 84, 23, 27, 33, 52, 56, 102, 58, 58, 41, 53,<br>
56, 24, 39, 29, 87, 51, 38, 25, 4, 64, 107, 50, 1, 54, 26,<br>
23, 17, 66, 69, 6, 27, 54, 25, 48, 24, 80, 36, 27, 74, 47,<br>
21, 26, 118, 70, 55, 76, 50, 62, 49, 56, 29, 160, 24, 50, 54,<br>
29, 32, 108, 64, 21, 49, 39, 137, 11, 28, 25, 74, 3, 44, 41,<br>
33, 68, 37, 83, 15, 69, 82, 108, 27, 5, 74, 55, 16, 14, 29,<br>
52, 33, 14, 74, 29, 26, 66, 9, 66, 70, 81, 1 " | |
| 9) | | | | Returns:
"</pre>67 states, 202 properties<br>
<br>
Areas:<br>
144, 231, 413, 64, 167, 193, 39, 160, 244, 333, 55, 67, 315, 108, 134,<br>
305, 163, 67, 5, 148, 105, 54, 196, 77, 234, 84, 78, 227, 280, 255,<br>
103, 101, 346, 120, 109, 146, 50, 153, 111, 35, 30, 209, 112, 174, 262,<br>
84, 174, 137, 128, 163, 85, 79, 88, 17, 39, 174, 247, 167, 270, 187,<br>
108, 69, 159, 234, 281, 87, 17 <br><br>
Adjacency Matrix:<br>
<pre style='font-size:8px;'>0001000010010000000000001100001100011101100100100000000010010101100
0000000100001100100010001011000011000000000011000000101010100011000
0001010111001100000101010001100010000110011010101101001001000001010
1010000001000000000000001000000000000000011010000010100010100000000
0000000011000000000010001001111011000000100000001010000110010000000
0010000100000000100000000000100000000000001010001110000001000010110
0000000010001000000110000000100010000000101000000001000001000000000
0110010010001000100000100000000010000000000000100000001001100000010
1010101101000001000010001000111001010100010011011000000110000100101
0011100010010011000001001000010010011101010000101010000101100001100
0000000000000010100000100000010000000000000000000000000000010000000
1000000001000001100000000100000000000110000000000000000000000001000
0110001100000101010111101001100011010000110011001000000110111000000
0110000000001001000000110010000000000000010010000000000000000000000
0000000001100000000000000000010010000000000100100100000000110011000
0000000011011100010011110001100010000101001010000000100110000001000
0100010100110000000000100000010010000000000000000000001100001001100
0000000000001001000100000000100010010000100000000000100000000000000
0000000000000000000000000010000000100000000000000000000000000000000
0010001000001000010001000000000010000000001000101111000001010000000
0100101010001001000000001000100000000000100000000000100000000011100
0010000001001001000100000000000000011000000000100001000000000000010
0000000100101101100000000000000010100100010000010000000110111101000
0010000000000101000000000000000100000100001000000101000001000010000
1101100011001000000010000101100001000000000011000000000001100000100
1000000000010000000000001000001000000010000000000000000000100000000
0100000000000100001000000001100000100010010001000100000000001011000
0110100000001001000000001010001011000010010010000000000100000001000
0010111010001001010010001010000001000010010011000000100000110000101
0000100011100010100000000000000100100000010000000000000000100010100
1000100010000000000000000101000000000000001100000010000010100000000
1000000000000000000000010000010000000000100000000010000001000010100
0110101101001011110100100001000000010000001000111011000001111010110
0100100010001000000000001001100000000010000010110000000011000000000
0000000000000000001000100010010000000100010000000000000000001010000
1000000011001000010001000000000010001100100010110000100100000001001
1000000001000000000001000000000000010101000000000000000000000000000
1010000011010001000000110000000000111000000001010100000000011000011
0010000000010000000000000111100001000000000000000000000000100001000
1000000001000001000000000000000000001000000000000000000000000100000
1000101000001000010010000000000100010000000000000010100000010000000
0011000011001100000000100011110000100000000011010000001110110000000
0011011000000001000100010000001010000000000000000010000011100100000
1000000000000010000000000000001000000000000000000000000110000111010
0111010010001101000000001001100001010000010001000000100101100001000
0100000010001000000000001010100000000100010010000000000100100000001
1010000101000010000101000000000011010000000000011100000001100001000
0000000010000000000000100000000011010100010000101000000110011100010
0010110011001000000100000000000010000000000000110110000010000000000
0010010000000010000100010010000000000100000000101000000000001010000
0001110001000000000100000000001110000000101000001000000001100000000
0010001000000000000101010000000010000000000000000000010001000000100
0101000000000001010010000000100000010000100010000000000010100000100
0000000000000000000000000000000000000000000000000001000000000010000
0110000100000000100000000000000000000000010000000000000100000000000
0000100011001001100000100001000000010000010111010000001010010001000
1101100010001001000000100000001001000000011100011000100100100100000
0010011101000000000100011000000111000000001010100011000000100000010
0101000101001010000000101100111010000010011011100010100011000000100
1000100000101010000100100000100010000100110000010000000100001001100
0000000000001000100000100010000010100100000000010100000000010000000
1000000010000000000000100000000000000001001100010000000010000001000
0100010000000010000010010010010110100000000100000100010000000000010
1110000001010011100010100011000000010010000110100000000100010100000
1000010011000000100010001000110110000000000000000001100000110000000
0010010100000000000001000000000010000100000100010000000001000010000
0000000010000000000000000000100000010100000001000000000000000000000
</pre>" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are given an 8 x 8 chessboard, and you must place the minimum possible number of queens onto the board such that all cells are under attack from at least one queen, and no queens attack each other. Some of the cells on the board are destroyed. A queen cannot be placed on a destroyed cell, and destroyed cells don't need to be under attack. A queen attacks a cell if it is in the same row, same column, or same diagonal as that cell (even if there are destroyed cells between them). Rows are labelled from 1 to 8 and columns from 'A' to 'H'.
You are given a String[] board, where the jth character of the ith element represents the cell at row i, column j. A '.' denotes an empty cell, and a '#' denotes a destroyed cell. A solution is represented by a string which concatenates the positions of the queens. Each queen will be represented by two characters rc, where r is the row label and c is the column label. For example, if the solution contains one queen at row 1, column H and another at row 5, column C, it can be represented as "1H5C" or "5C1H". If there are several solutions which use the minimal number of queens, return the one that comes first lexicographically. | | Definition | | Class: | QueenCovering | Method: | getPlacement | Parameters: | String[] | Returns: | String | Method signature: | String getPlacement(String[] board) | (be sure your method is public) |
| | | | Constraints | - | board will have exactly 8 elements. | - | Each element of board will contain exactly 8 characters. | - | Each character of board will be either '.' or '#'. | | Examples | 0) | | | {
"........",
"..######",
".#.#####",
".##.####",
".###.###",
".####.##",
".#####.#",
"........"} |
| Returns: "1A8B" | We place one queen on the first row and the first column, and another queen on the last row and on the second column. |
|
| 1) | | | {
"#......#",
".#......",
"..#...#.",
"........",
"..#.....",
"..#..#..",
"#.......",
"#...###."} |
| Returns: "1B2D3A4C5E" | |
| 2) | | | {
"........",
"........",
"........",
"........",
"........",
"........",
"........",
"........"} |
| Returns: "1A2C3E4B5D" | |
| 3) | | | {
"..##.##.",
".###..##",
"##..###.",
"#..#...#",
".#.##.#.",
"#...##..",
"#..#####",
"..#..#.#"} |
| Returns: "1A4C5H6B" | |
| 4) | | | {
"########",
"########",
"########",
"########",
"########",
"########",
"########",
"########"
}
|
| Returns: "" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are given a list of people, and you must divide them into as many groups as possible. Each person must belong to exactly one group, and each group must contain one or more people. Friends must not be split apart, so if two people are friends, they must be in the same group.
You will be given a String[] names, each element of which is the name of a single person. You will also be given a String[] friends, each element of which is formatted as "<name1> <name2>" (quotes for clarity only), meaning that <name1> and <name2> are friends. Each <name1> and <name2> will exist in names. Return the maximum number of groups that can be formed. | | Definition | | Class: | FriendsTrouble | Method: | divide | Parameters: | String[], String[] | Returns: | int | Method signature: | int divide(String[] names, String[] friends) | (be sure your method is public) |
| | | | Constraints | - | names will contain between 1 and 50 elements, inclusive. | - | Each element of names will contain between 1 and 20 uppercase letters ('A'-'Z'), inclusive. | - | All elements in names will be distinct. | - | friends will contain between 0 and 50 elements, inclusive. | - | Each element of friends will be formatted as "<name1> <name2>" (quotes for clarity only), where both <name1> and <name2> are elements of names. | | Examples | 0) | | | {"BOB", "HARRY", "ALICE", "SALLY"} | {"BOB ALICE", "HARRY SALLY"} |
| Returns: 2 | BOB and ALICE form one group, and HARRY and SALLY form a second group. The only other valid division is putting them all in a single group, but we want to maximize the number of groups |
|
| 1) | | | {"BOB", "HARRY", "ALICE", "SALLY"} | {"BOB HARRY", "HARRY ALICE", "ALICE SALLY" } |
| Returns: 1 | There is no way to form more than one group without separating a pair of friends. |
|
| 2) | | | {"CHUCK"} | {"CHUCK CHUCK","CHUCK CHUCK","CHUCK CHUCK"} |
| Returns: 1 | CHUCK is his own only friend. Note that friends may have duplicate entries. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Linez is a single-player board game played on a 9x9 grid. On each turn, the player must move one of the numbers on the board to an empty cell where a clear path (consisting of horizontally connected or vertically connected empty cells) exists between the new position and the old. If five or more same numbers are aligned in a row, column, or diagonal, they will be removed from the board. The player will gain 10+k*(k-5)/2 points (k being the number of removals) and can make another move. Otherwise, three more numbers will be placed onto the board at randomly chosen positions before the player can move again. The objective is to score as many points as possible before the whole board fills up. (An online version of the Linez game is available at http://linez.varten.net/.)
Your task is to write a class to play the Linez game. The method nextMove will be given the board configuration and the next three numbers. board will contain 9 Strings representing the rows of the board from top to bottom. Each element will contain 9 characters. Each character will be '1' to '7' representing the number on that cell, or '.' if that position is unoccupied. nextThree will contain 3 characters. Each element of nextThree will be '1' to '7' representing the number to be added to the board next turn. The return value of nextMove must contain 4 characters, the first two representing the original position of the number to be moved, the next two representing the target position. For example, to move the number "4" from "I8" to "F6" as shown below, return "I8F6".

You will have a total of 20 seconds for each test case. The memory limit is 64MB. Your final score will be the mean score on all the test cases. | | Definition | | Class: | Linez | Method: | nextMove | Parameters: | String[], String | Returns: | String | Method signature: | String nextMove(String[] board, String nextThree) | (be sure your method is public) |
| | | | Notes | - | Each number of nextThree will be chosen uniformly between 1 and 7, inclusive. | - | Each empty cell has an equal probability to be chosen when the next number is added to the board. | - | If your solution crashes, runs out of time, or tries to make an invalid move it will receive the points it has made so far for that test case. | | Examples | 0) | | | | Returns:
"board:<br>{<br> ".........",<br> ".....4...",<br> ".........",<br> "......5..",<br> ".........",<br> ".........",<br> "......3..",<br> ".........",<br> "........."<br>}<br>nextThree:<br> "545"<br>" | |
| 1) | | | | Returns:
"board:<br>{<br> ".........",<br> ".........",<br> ".......5.",<br> "3........",<br> ".........",<br> ".........",<br> ".........",<br> "....7....",<br> "........."<br>}<br>nextThree:<br> "773"<br>" | |
| 2) | | | | Returns:
"board:<br>{<br> "..6.....4",<br> ".........",<br> ".........",<br> ".........",<br> ".........",<br> "...1.....",<br> ".........",<br> ".........",<br> "........."<br>}<br>nextThree:<br> "154"<br>" | |
| 3) | | | | Returns:
"board:<br>{<br> ".........",<br> ".........",<br> ".........",<br> ".........",<br> "7........",<br> ".........",<br> ".........",<br> ".........",<br> "..6....2."<br>}<br>nextThree:<br> "563"<br>" | |
| 4) | | | | Returns:
"board:<br>{<br> ".6.......",<br> ".....6...",<br> ".4.......",<br> ".........",<br> ".........",<br> ".........",<br> ".........",<br> ".........",<br> "........."<br>}<br>nextThree:<br> "745"<br>" | |
| 5) | | | | Returns:
"board:<br>{<br> ".........",<br> ".........",<br> ".6.4.....",<br> "........6",<br> ".........",<br> ".........",<br> ".........",<br> ".........",<br> "........."<br>}<br>nextThree:<br> "151"<br>" | |
| 6) | | | | Returns:
"board:<br>{<br> ".........",<br> ".........",<br> "......3..",<br> "..3......",<br> ".........",<br> ".........",<br> "..2......",<br> ".........",<br> "........."<br>}<br>nextThree:<br> "455"<br>" | |
| 7) | | | | Returns:
"board:<br>{<br> ".........",<br> ".........",<br> ".....1...",<br> ".........",<br> ".6.......",<br> "......5..",<br> ".........",<br> ".........",<br> "........."<br>}<br>nextThree:<br> "527"<br>" | |
| 8) | | | | Returns:
"board:<br>{<br> ".2.......",<br> ".........",<br> ".........",<br> ".........",<br> "...6.....",<br> ".........",<br> ".......1.",<br> ".........",<br> "........."<br>}<br>nextThree:<br> "564"<br>" | |
| 9) | | | | Returns:
"board:<br>{<br> "........2",<br> ".........",<br> ".........",<br> ".........",<br> ".....2...",<br> ".........",<br> ".........",<br> "....5....",<br> "........."<br>}<br>nextThree:<br> "624"<br>" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Dominoes is a game played with rectangular tiles. Each tile (domino) is split in half vertically and contains two numbers between 0 and 6, inclusive. One tile A can be played after tile B if the left number of A is the same as the right number of B. Several consecutively played tiles are called a line. For example, the picture below shows a line containing four tiles.
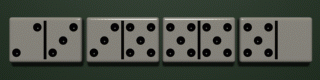
You will be given a String[] tiles. Each element of tiles will be in the format "X:Y" where X and Y are digits between 0 and 6, inclusive. You should arrange these tiles into as few lines as possible. You can turn over any tile, i.e., the tile "2:5" can also be used as "5:2". Each tile should be used exactly once. Your method should return the constructed lines as a String[]. Each element should represent a single line, formatted as a dash-separated list of tiles in the order and orientation they occur in the line. If there is more than one solution possible, return the solution whose first element comes first lexicographically.
If there are still multiple solutions, return the one among them whose second element comes first lexicographically, and so on.
| | Definition | | Class: | DominoesLines | Method: | constructLines | Parameters: | String[] | Returns: | String[] | Method signature: | String[] constructLines(String[] tiles) | (be sure your method is public) |
| | | | Constraints | - | tiles will contain between 1 and 50 elements, inclusive. | - | Each element of tiles will be in the format "X:Y" where X and Y are digits between 0 and 6, inclusive. | | Examples | 0) | | | {"1:0", "1:1", "1:2", "1:3", "1:4"} |
| Returns: {"0:1-1:1-1:2", "3:1-1:4" } | |
| 1) | | | {"4:0", "4:1", "4:2", "4:3", "4:4"} |
| Returns: {"0:4-4:1", "2:4-4:4-4:3" } | |
| 2) | | | {"0:0", "1:1", "2:2", "3:3", "6:6", "6:0"} |
| Returns: {"0:0-0:6-6:6", "1:1", "2:2", "3:3" } | |
| 3) | | | {"0:4", "6:6", "1:2", "1:1", "3:1", "4:4", "0:1", "1:5", "5:0"} |
| Returns: {"0:1-1:1-1:2", "3:1-1:5-5:0-0:4-4:4", "6:6" } | |
| 4) | | | {"3:1", "0:2", "6:1", "6:3", "4:6", "6:4",
"2:3", "4:0", "5:2", "2:2", "6:6"} |
| Returns: {"2:0-0:4", "3:1-1:6-6:4-4:6-6:6-6:3-3:2-2:2-2:5" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
PenPuzzle is a variation on the famous Rubik's cube. Imagine a polygonal cylinder with k sides, where each side is divided into m slots (for a total of k*m slots). All slots are of the same height, so the slots form several levels (the lowermost slots on each side form one level, the slots directly above those form another level, and so on). Each slot contains a single colored plate. There are k different colors, and m plates of each color. The goal of the game is to arrange the plates such that each side contains only plates of the same color. The following picture shows a 6-sided puzzle with 6 levels on each side:
You can use the following two actions to solve the puzzle. First, any level can be rotated around the axis of the cylinder in any direction. A single rotation will move each plate on a level to the slot on the next side at the same level. For example, if we take the puzzle from the picture and rotate level 2 (the second from the bottom) in a clockwise direction, the blue plate will move to the green plate's original position, and the yellow plate will take the blue plate's place. Each such rotation takes 1 second. A full turn around the example puzzle would therefore take 6 seconds.
The second allowed action is shifting plates within a single side. If there's an empty slot directly adjacent to a plate on the same side, the plate can be moved into that slot. For example, the bottommost slot on the middle side of the example puzzle (between the red and green plates) is empty, so the blue plate from the second level can be moved to the first level (leaving its original slot on the second level empty). This movement also takes 1 second. Please note that neither the red nor green plate on the bottommost level can be moved to the empty slot because the puzzle is only constructed to allow plates to be shifted within the same side. If we were to rotate the first level counterclockwise, the empty slot would replace the green plate, the red plate would replace the empty slot, etc.
You will be given a String[] puzzle representing the initial state of the puzzle. Each element of puzzle represents one level of the puzzle, with the first k uppercase letters representing the k different colors.
The i-th character of each element of puzzle corresponds to the i-th side.
You must remove any one plate from the puzzle to create an empty slot and make shifting plates possible. Find the plate which will allow you to solve the puzzle in the minimal time and return this time.
| | Definition | | Class: | PenPuzzle | Method: | solve | Parameters: | String[] | Returns: | int | Method signature: | int solve(String[] puzzle) | (be sure your method is public) |
| | | | Constraints | - | puzzle will contain between 2 and 3 elements, inclusive. | - | All elements of puzzle will contain the same number of characters. | - | Each element of puzzle will contain between 3 and 4 characters, inclusive. | - | Each element of puzzle will contain only the first k uppercase letters, where k is the length of any element of puzzle. All elements of puzzle will contain the same total number of appearances of each of the first k uppercase letters. | | Examples | 0) | | | | Returns: 0 | This puzzle is already solved. You can remove any plate and it still will be solved. |
|
| 1) | | | | Returns: 1 | One rotation solves this puzzle. |
|
| 2) | | | | Returns: 2 | The initial state of the puzzle is
ABA
BCC
Remove the second 'A' plate to get to the following configuration ('_' stands for an empty slot):
AB_
BCC
Shift the second 'C' to the upper level:
ABC
BC_
And rotate the upper row to solve the puzzle:
BCA
BC_
|
|
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Consider the figures made up of line segments below. There are 5 connected figures,
but only 3 distinct figures if one considers their topological features (the first
and second are equivalent, as are the third and fourth). Two figures are considered
to be topological equivalent if one of the figures can be deformed by stretching,
bending, etc. into the other (but not necessarily in 2D space).
A more formal definition can be expressed in terms of graph theory.
A figure can be considered to be an embedding of a multigraph (it may have loops and multiple edges)
in the plane, where each vertex has been assigned to a distinct point, and each edge to some polygonal
path without self-intersections. We consider two figures to be equivalent if their embeddings could
have both originated from the same multigraph.
In this problem, we will assume that each edge is disjointly embedded. That is,
two embedded edges can only meet at a common endpoint.
Create a class TopologicalEquivalence containing the method countDistinct which takes a set of non-intersecting and non-overlapping
line segments and counts the number of distinct connected figures (according to the definition of topological equivalence above).
The line segments will be given in a String[] lineSegs, where each element may contain several line segments.
A line segment will be given in the form (quotes for clarity) "x1,y1-x2,y2" and
within each element they will be single space separated. Two line segments are connected if they share a common endpoint. A figure
is a minimal non-empty subset of the line segments such that no line segment in the figure is connected to a line segment not in the figure.
| | Definition | | Class: | TopologicalEquivalence | Method: | countDistinct | Parameters: | String[] | Returns: | int | Method signature: | int countDistinct(String[] lineSegs) | (be sure your method is public) |
| | | | Constraints | - | The line segments making up a figure will have at most 8 distinct end points. | - | lineSegs will contain between 1 and 50 elements, inclusive. | - | Each element in lineSegs will contain between 7 and 50 characters, inclusive. | - | Each element in lineSegs will be a space-separated list of line segments specified in the format above. There will be no leading, trailing, or consecutive spaces. | - | The coordinates for each line segment will be integers between 0 and 1000, inclusive, with no extra leading zeros. | - | No two line segments will partially overlap or intersect, except at the endpoints. | - | All line segments will have a positive length. | | Examples | 0) | | | {"0,7-5,7 5,7-5,2 5,2-0,2 0,2-0,7 5,2-7,0",
"8,7-15,7 15,7-10,2 10,2-8,7 10,2-10,5 10,5-12,5",
"16,6-19,2 16,6-22,4 22,4-21,2 21,2-19,4",
"24,7-24,2",
"25,5-27,3 27,7-29,5 25,2-27,3 29,2-27,3 25,5-27,7","27,3-29,5"}
|
| Returns: 3 | This corresponds to the figures in the pictures. Here, each element correspond to one of the figures (although this is not generally the case, see further examples). |
|
| 1) | | | {"16,0-17,1 17,1-17,3 17,3-16,4 16,4-14,4 14,4-14,0",
"9,1-9,3 9,3-10,4 10,4-12,4 12,4-13,3 14,0-16,0",
"0,4-1,2 1,2-2,0 2,0-3,2 1,2-3,2 3,2-4,4 5,0-7,0",
"25,0-27,0 24,1-24,3 24,3-25,4 25,4-27,4 27,4-27,2",
"7,0-8,1 8,1-7,2 7,2-8,3 8,3-7,4 7,4-5,4 5,4-5,2",
"18,0-20,0 18,0-18,2 18,2-18,3 18,2-18,4 18,4-20,4",
"21,0-23,0 21,0-21,2 21,2-22,2 21,2-21,4 24,1-25,0",
"5,2-5,0 5,2-7,2 9,1-10,0 10,0-12,0 12,0-13,1",
"27,2-25,2 28,0-28,2 28,2-28,4 30,0-30,2 30,2-30,4",
"28,2-30,2 31,0-31,4"}
|
| Returns: 6 | The 9 figures in this input correspond to the letters A, B, C, D, E, F, G, H and I. Topologically, the three letters C, G and I are the same, as well as the two letters E and F. The remaining 4 letters have distinct topological features. |
|
| 2) | | | {"0,2-2,2 10,2-12,0 2,2-4,0 12,0-14,2",
"4,0-4,4 14,2-10,2 4,4-2,2 10,2-12,4",
"4,4-6,2 12,4-14,2 6,2-8,2 12,4-12,6",
"14,8-12,6 6,2-4,0 12,6-10,8"}
|
| Returns: 2 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
There is a group of friends that is not as close as it should be. Not everybody inside the group
likes everybody else. One of the friends has some important news that needs to be spread to
every member of the group. He has information on who would be willing to call each other and
has to develop a strategy to make sure all members of the group receive the news as soon as possible.
Each person can only call one friend each minute, and during that minute, he will transmit the news
along with the calling strategy that the receiver should follow. Any person that has already been
called is allowed to call other friends, but nobody can be on the phone (passing on or receiving
the news) with more than one other person during the same minute.
You will be given a String[] conn, where the jth character of the
ith element is 'Y' if the ith friend is willing to call the jth
friend, or 'N' otherwise. The 0th person (0-based) is the one that initially learns
about the news. Return the least number of minutes needed for the entire group to be aware of
the news. If it is impossible for this to happen, return -1.
| | Definition | | Class: | FastGossip | Method: | minTime | Parameters: | String[] | Returns: | int | Method signature: | int minTime(String[] conn) | (be sure your method is public) |
| | | | Notes | - | Note that the fact that one friend is willing to call another does not necessarily imply that the other is willing to call the first. See examples for further clarification. | | Constraints | - | conn will contain between 1 and 14 elements, inclusive. | - | Each element of conn will contain exactly N characters, where N is the number of elements in conn. | - | Each character of each element of conn will be either 'Y' or 'N'. | - | The ith character of the ith element of conn will be 'N'. | | Examples | 0) | | | {"NYNN","NNYN","NNNY","NNNN"} |
| Returns: 3 | Individual 0 must call individual 1. Individual 1 must call individual 2. Individual 2 must call individual 3. Since all these actions must happen sequentially, the total time to reach the last individual is 3 minutes. |
|
| 1) | | | {"NYYY",
"YNYY",
"YYNY",
"YYYN"} |
| Returns: 2 | Everybody is a close friend. Individual 0 can call any one of the other individuals in the first minute, and during the second minute, both of them can call one of the remaining two. |
|
| 2) | | | | Returns: -1 | Individual 0 can't call anybody, and therefore, it is impossible to spread the news to individual 1. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are playing a game with a friend involving two robots on a rectangular board. Each cell of the board is either empty, an obstacle, a starting point for a robot, or the destination. You win the game only if your robot is the first to reach the destination cell. Note that if both robots reach the destination cell at the same time or if neither reaches the destination at all, you lose.
Both robots listen to the following movement commands:
- 'S' -- move south (the current row increases by one)
- 'N' -- move north (the current row decreases by one)
- 'E' -- move east (the current column increases by one)
- 'W' -- move west (the current column decreases by one)
During one time unit, the same movement command is given to both robots. A robot may choose to follow the command given or ignore it. Note that if the command makes the robot leave the board, or enter a cell occupied by an obstacle, it is automatically ignored and that both robots can be in the same cell at the same time. The robots will always make an optimal decision so that they reach the destination cell as soon as possible.
You will be given a String[] board. Each character in board will represent a single cell on the game board and will be either '.' denoting an empty space, '#' denoting an obstacle, 'Y' denoting the starting point for your robot, 'F' denoting the starting point for your friend's robot, or 'X' denoting the destination cell. You will also be given a String[] commands. You should concatenate all elements of commands and make a long String of commands. The ith character of this string will represent the command given during the ith time unit. Starting the game at time 0 (in other words, from the first character of the string of commands) may not guarantee that your robot wins the race. Return the earliest starting time that will guarantee your robot will win. Starting the race at time T means that only the commands starting at index T in the input string are given, the ones with lower indexes will be ignored by both robots. If there is no starting time that can make your robot win, return -1.
| | Definition | | Class: | RoboRace | Method: | startTime | Parameters: | String[], String[] | Returns: | int | Method signature: | int startTime(String[] board, String[] commands) | (be sure your method is public) |
| | | | Constraints | - | board will contain between 1 and 50 elements, inclusive. | - | Each element of board will contain between 1 and 50 characters, inclusive. | - | All elements of board will contain the same number of characters. | - | Each character of each element of board will be either '.', '#', 'Y', 'F' or 'X'. | - | The characters 'Y', 'F' and 'X' will each appear exactly once in board. | - | commands will contain between 1 and 50 elements, inclusive. | - | Each element of commands will contain between 1 and 50 characters, inclusive. | - | Each character of each element of commands will be either 'S', 'N', 'E' or 'W'. | | Examples | 0) | | | | Returns: 0 | Your robot needs to move east to reach the destination, while your friend's robot needs to move south. You have three options: start the race at times 0, 1, or 2. If you start the race at time 2, your friend will win. If the race starts at time 0, both robots will have no choice but to ignore the first command, and your robot will win by following the second command. From the two options that guarantee your robot wins, choose the first one, at time 0. |
|
| 1) | | | {"########",
"#......#",
"#.Y....#",
"#.F.#..#",
"#...X..#",
"#...#..#",
"#......#",
"########"} | {"SSEEESSW"} |
| Returns: 2 | Start the race at time 2 and your robot will follow the last 6 commands and reach the destination. Your friend's robot needs one of the first two 'S' commands, so you can not start the race sooner than time 2. |
|
| 2) | | | {"########",
"#......#",
"#.Y....#",
"#.F.#..#",
"#...X..#",
"#...#..#",
"#......#",
"########"} | {"ESSEESSW"} |
| Returns: -1 | The only way for your robot to reach the destination is to start the race at time 0. However, the other robot will reach the destination sooner in this case. |
|
| 3) | | | {"##.#.#.",
"..##...",
"..#...X",
"Y...##.",
"#...#.#",
"..#..F."} | {"SSSNWSSSEWNSENENENNNNENWNEWESE"} |
| Returns: 5 | If the race starts at time 5, the destination is reached at time 26. The commands followed by your robot are EEENEEE (the others are ignored). Note that starting the race at time 4 would result in both robots reaching the destination cell at the same time. |
|
| 4) | | | {"#..#.........#...X##....",
"#........#..........##.#",
".#.#........#.....#.#...",
"..###...#..##.##...#....",
"..#.#.....#....#.#.####.",
"#...##.##.##..#.....##..",
".##...#.#....#.......#.#",
"....##.#..#....#....#...",
"....###.##.....###...#..",
"#.#.......#.#......#..#.",
".##....##.#.##.......#.#",
"......###...####......#.",
"..#.##.#..#.#...#...#...",
".....#.#..........#...#.",
"##.#....##F#.....#.##.#.",
".##....#.......##.##.##.",
"..#...#..##....#..#...Y.",
"#...........#...###..###",
".....#...#..#.........#.",
".#...##..#.#...#..#.##..",
"#..#...######....###.#..",
"#.#.....#.......#.##....",
"#..#....###....#.#..#...",
"..#...#.##.##.#.##.##..#",
"#....##.##..........#..#"} | {"NWWSEWSSNWESSWES",
"ESEEENSNWNNWSNSNWWNWWNNNWE",
"NSNENENNSEENWWNSNNNNWWSSN",
"EENEWNWESESEEESNNNSEENNEWNNESNEESSEESEEENENNNWSSW",
"NWNNWSNWSWSSSSEEWSSWSESWWNNWWENSNNWWSSWWNNE",
"NWEWNEWSNEN",
"NNNEWNSWSNWESWNNNSWWNNNWWWNNEWNEEWSSWNSSWWNNWESEWS",
"WSSSEESSEEEEENNSWEWWWENSENWNSEENES",
"NNSNESESWNESNENSEESESWSENNESESNESNESEEW",
"ESNENEENWSNS"} |
| Returns: 18 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | There are several unmarried men and women living in a society where marriage is defined as either one husband with one or more wives, or one wife with one or more husbands. You are given a String[] preferences. The j-th character of the i-th element of preferences is '1' (one) if the i-th man and the j-th woman are willing to be part of the same marriage, and '0' (zero) otherwise.
Your task is to group these people into the minimum possible number of marriages. Each person must be a member of exactly one marriage, and each marriage must contain only willing members. Return the number of marriages, or -1 if this is not possible. | | Definition | | Class: | MarriageProblemRevised | Method: | neededMarriages | Parameters: | String[] | Returns: | int | Method signature: | int neededMarriages(String[] preferences) | (be sure your method is public) |
| | | | Constraints | - | preferences will contain between 1 and 12 elements, inclusive. | - | The length of each element of preferences will be betwen 1 and 12, inclusive. | - | All elements of preferences will be of the same length. | - | Each element of preferences will contain only '0' (zeroes) and '1' (ones). | | Examples | 0) | | | | Returns: 1 | Here, we have one man and three women, and everybody is willing to be in the same marriage. Therefore, we only need one marriage. |
|
| 1) | | | | 2) | | | | Returns: -1 | Nobody is willing to be in the same marriage as anybody else, so there cannot be any marriages in this case. |
|
| 3) | | | {"0001", "0001", "0001", "1111"} |
| Returns: 2 | |
| 4) | | | {"11101011","00011110","11100100","01010000","01000010","10100011","01110110","10111111"} |
| Returns: 3 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | For a given integer k, we call a string S k-unrepeatable if there is no substring that appears k consecutive times in S.
For example, the string CCABAABAABAC is 4-unrepeatable. But it's not 3-unrepeatable, because the string ABA appears in it 3 times in a row. CCABAABACABA and ABABAABA are examples of 3-unrepeatable strings.
You are given three integers, k, n and allowed. Return the lexicographically smallest k-unrepeatable word of length n that uses only the first allowed uppercase characters of the English alphabet. If no such word exists, return the empty string.
| | Definition | | Class: | UnrepeatableWords | Method: | getWord | Parameters: | int, int, int | Returns: | String | Method signature: | String getWord(int k, int n, int allowed) | (be sure your method is public) |
| | | | Constraints | - | k will be between 2 and 10, inclusive. | - | n will be between 1 and 50, inclusive. | - | allowed will be between 1 and 26, inclusive. | | Examples | 0) | | | | Returns: "AABAA" | All lexicographically smaller strings of length 5 contain three consecutive occurrences of the letter A, so they aren't 3-unrepeatable. |
|
| 1) | | | | Returns: "" | The only possible string is AAAAA, which is not 3-unrepeatable. |
|
| 2) | | | | 3) | | | | Returns: "AABAABABAABAABBAABAABABAABAABBAABAABABAABABBAABAAB" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You have a large square box and some smaller triangular boxes (all two-dimensional). The triangular boxes all lie inside the square box. Triangular boxes may lie inside other triangular boxes, but they must not overlap. You randomly throw an object (represented as a point) into the square box, and you want to know if it lands inside exactly inBox triangular boxes.
You are given a String[] boxes, each element of which is formatted as "X1.Y1 X2.Y2 X3.Y3" (quotes for clarity only), representing the three corners of a triangular box. The large square box has sides parallel to the axes, and has corners at (0, 0) and (100, 100). Return the probability (between 0 and 1) that a random point within the square is contained in exactly inBox triangular boxes. | | Definition | | Class: | SetOfBoxes | Method: | countThrow | Parameters: | String[], int | Returns: | double | Method signature: | double countThrow(String[] boxes, int inBox) | (be sure your method is public) |
| | | | Notes | - | Your return value must have an absolute or relative error less than 1e-9. | | Constraints | - | boxes will contain between 0 and 50 elements, inclusive. | - | Each element of boxes will be formatted as "X1.Y1 X2.Y2 X3.Y3" (quotes for clarity only). | - | X1, Y1, X2, Y2, X3, Y3 will be integers with no leading zeros, each between 0 and 100, inclusive. | - | Triangles represented by different elements of boxes will have no common points. | - | inBox will be between 0 and 50, inclusive. | | Examples | 0) | | | | 1) | | | {"0.0 20.0 0.10", "1.1 6.1 1.5"} | 1 |
| Returns: 0.0090 | Here it is the area of the first triangle minus the area of the second one. |
|
| 2) | | | {"0.0 10.0 0.20", "0.100 0.90 20.100", "50.50 60.60 50.70", "51.55 55.60 51.65"} | 1 |
| Returns: 0.028 | |
| 3) | | | {"0.0 10.0 0.20", "0.100 0.90 20.100", "50.50 60.60 50.70", "51.55 55.60 51.65"} | 0 |
| Returns: 0.97 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | When programming, I don't like to take my fingers off the keyboard, and hence, I don't use the mouse. So, when navigating my source, I like to do so in such a way as to minimize keystrokes. My text editor supports the following keystrokes: left, right, up, down, home, end, top, bottom, word left and word right. The word left and word right keystrokes position the cursor at a word beginning; a word beginning is the first letter of the word. None of the keystrokes cause the cursor to wrap. When moving the cursor vertically, my text editor does not change the cursor's horizontal position. So, if the cursor is at column 50 and the up arrow is pressed, moving the cursor to a row with only 10 characters, only the row changes. My text editor does not allow the cursor to be positioned left of the first column, above the first row or below the last row.
The keys left, right, up, down, home, end, top, bottom, word left and word
right behave as described below:
1. left, right, up and down - move the cursor one position in the indicated direction (see notes).
2. home - causes the cursor to move to column 0 of the current row.
3. end - causes the cursor to move to the last character of source in the current row.
4. top - causes the cursor to move to the top row (row 0) retaining its column position.
5. bottom - causes the cursor to move to the bottom row retaining its column position.
6. word left - causes the cursor to jump to the first word beginning that is strictly left of the cursor position, if one exists. Otherwise does nothing.
7. word right - causes the cursor to jump to the first word beginning that is strictly right of the cursor position, if one exists. Otherwise does nothing.
You will be given a String[] source representing the source code, a int[] start representing the starting position of the cursor and a int[] finish representing the ending position of the cursor. The first int in both start and finish specifies the 0-indexed row and the second int specifies the 0-indexed column. You are to calculate and return the minimum number of keystrokes required to get from start to finish. | | Definition | | Class: | TextEditorNavigation | Method: | keystrokes | Parameters: | String[], int[], int[] | Returns: | int | Method signature: | int keystrokes(String[] source, int[] start, int[] finish) | (be sure your method is public) |
| | | | Notes | - | For the purposes of this problem, we use the convention that the cursor covers each character. Some editors (normally in "insert mode") have the cursor preceding each character. | - | A keypress may not have any effect. For example, pressing up in the top row does nothing, pressing left in the first column does nothing and pressing down in the bottom row does nothing. Pressing right always has an effect. | | Constraints | - | source will contain between 1 and 50 elements, inclusive. | - | Each element of source will contain between 1 and 50 characters, inclusive. | - | Each character must be a letter ('a'-'z', 'A'-'Z') or ' '. | - | start and finish will each contain exactly 2 elements. | - | start and finish will each represent character positions that exist in source. | | Examples | 0) | | | {"AAAAA AAA AAAAAAAAAAAAA AAAA",
"AA AAAAAAAAA AAAA AAAA",
"BBBBBBBBBBBBBBBBBBBBBBBBBBB",
"BBBBBBB BBBBBBBBBB BBBBBBB",
"CCC CCCC CCCCCC CCCC",
"DDDDDDDDDDDDDDDDDDD"} | {5, 7} | {2, 2} |
| Returns: 6 | This can be achieved by the following keystrokes in the given order: home, top, down, down, right, right. |
|
| 1) | | | {"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"BB BB BB BB BB BB BB BB BB BB BB BB BB BB BB BB BB",
"CCC CCC CCC CCC CCC CCC CCC CCC CCC CCC CCC CCC CC",
"DDDD DDDD DDDD DDDD DDDD DDDD DDDD DDDD DDDD DDDD ",
"EEEEE EEEEE EEEEE EEEEE EEEEE EEEEE EEEEE EEEEE EE",
"FFFF FFFF FFFF FFFF FFFF FFFF FFFF FFFF FFFF FFFF ",
"GGG GGG GGG GGG GGG GGG GGG GGG GGG GGG GGG GGG GG",
"HHHHHHHHHHH HHHHHHHHHH HHHHHHHHHH HHHHHHHHHH HHHHH",
"IIIIIIIIIIIIIII IIIIIIIIIIIIIII IIIIIIIIIIIIIII ",
"JJJJJJJJ JJJJJJJJ JJJJJJJJ JJJJJJJJ JJJJJJJJ JJJJJ",
"KKKKKKKKKKKKKKKKKKKKKKKKKK KKKKKKKKKKKKKKKKKKKKKKK",
"LLLLLLLLLL LLLLLLLLLLLLLLLLLLLLLLLLLLLLLLLLLLLLLLL",
"MMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMMM",
"N N N N N N N N N N N N N N N N N N N N N N N N N ",
"OOOOO OOOO OOO OO O O OO OOO OOOO OOOOO OOOOOO OOO",
"PPPPPPP PPPPPP PPPPP PPPP PPP PP P P PP PPP PPPP P",
"QQQQQQ QQQQQ QQQQ QQQ QQ Q Q QQ QQQ QQQQ QQQQQ QQQ",
"ZZZZ ZZ ZZZ ZZ ZZZZ ZZ ZZZ ZZ ZZZZ ZZ ZZZ ZZ ZZZZ ",
"SSS S SSS S SSS S SSS S SSS S SSS S SSS S SSS S SS",
"TT TT TT TT TT TTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTTT"} | {12, 20} | {4, 36} |
| Returns: 8 | |
| 2) | | | {"A A A A AAAAAAA A A A A A A A A A A",
"B BBBBB B B B B BBBBB B B B B B B B B"} | {1, 0} | {1, 22} |
| Returns: 6 | |
| 3) | | | {"AAAAAAAAAAAAAA A A A A A A A A A A"} | {0, 2} | {0, 15} |
| Returns: 1 | |
| 4) | | | {"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"N N N N N N N N N N N N N N N N N N N N N N N N N ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
"A A A A A A A A A A A A A A A A A A A A A A A A A ",
" O O O O O O O O O O O O OO O O O O O O O O O O O ",
" P P P P P P P P P P P P P PP P P P P P P P P P P ",
" Q Q Q Q Q Q Q Q Q Q Q Q Q Q QQ Q Q Q Q Q Q Q Q Q ",
" R R R R R R R R R R R R R R R RR R R R R R R R R ",
" S S S S S S S S S S S S S S S S SS S S S S S S S ",
" T T T T T T T T T T T T T T T T T TT T T T T T T ",
" U U U U U U U U U U U U U U U U U U UU U U U U U ",
" V V V V V V V V V V V V V V V V V V V VV V V V V ",
" W W W W W W W W W W W W W W W W W W W W WW W W W ",
" X X X X X X X X X X X X X X X X X X X X X XX X X ",
" Y Y Y Y Y Y Y Y Y Y Y Y Y Y Y Y Y Y Y Y Y Y YY Y ",
" Z Z Z Z Z Z Z Z Z Z Z Z Z Z Z Z Z Z Z Z Z Z Z ZZZ"} | {49, 49} | {38, 26} |
| Returns: 23 | |
| 5) | | | {"AAA", "BB", "CCC"} | {1, 1} | {1, 1} |
| Returns: 0 | |
| 6) | | | {"AAAAA AAA AAAAAAAAAAAAA AAAA",
"AA AAAAAAAAA AAAA AAAA",
"BBBBBBBBBBBBBBBBBBBBBBBBBBB",
"BBBBBBB BBBBBBBBBB BBBBBBB",
"CCC CCCC CCCCCC CCCC",
"DDDDDDDDDDDDDDDDDDD"} | {2, 17} | {1, 2} |
| Returns: 4 | |
| 7) | | | {"A PC to do CAD huh Sounds reasonable",
"Aurthor go out and buy us five new PCs",
"Dont you want to think about this for a minute",
"No every second counts and we want to be ahead of",
"the competition",
" OK Greate idea Please place lOOk worth of",
"unmarked bills in my suitcase and Ill be on my way"} | {0, 11} | {1, 15} |
| Returns: 2 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A sentence is composed of words, spaces and punctuation. For this problem, words are maximal contiguous strings of letters. Given a sentence, sort the words in the sentence while preserving the spaces and punctuation. Sorting is case sensitive, so uppercase letters precede lowercase letters. Return the newly constructed sentence.
Example:
"The big, brown dog ran down the street!"
Returns:
"The big, brown dog down ran street the!"
Observe the space and punctuation preservation between the original sentence and the resulting sentence.
| | Definition | | Class: | SuperSort | Method: | wordSort | Parameters: | String | Returns: | String | Method signature: | String wordSort(String sentence) | (be sure your method is public) |
| | | | Notes | - | Watch out for multiple consecutive spaces (they should be preserved). | | Constraints | - | sentence will contain between 1 and 50 characters, inclusive. | - | Each character in sentence will be either a letter ('a'-'z', 'A'-'Z'), ' ', '.', ',', '!' or '?'. | | Examples | 0) | | | "The big, brown dog ran down the street!" |
| Returns: "The big, brown dog down ran street the!" | |
| 1) | | | "This is the first sentence of the paragraph." |
| Returns: "This first is of paragraph sentence the the." | |
| 2) | | | "t.d,a!f?g.b,q!i?p.h,s!u?m.l,e!v?y.c,j!w?k.n,x!o?r." |
| Returns: "a.b,c!d?e.f,g!h?i.j,k!l?m.n,o!p?q.r,s!t?u.v,w!x?y." | |
| 3) | | | | 4) | | | | 5) | | | | 6) | | | | 7) | | | | Returns: "A B C D E a b c d e" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
This problem statement contains images, subscripts and superscripts that may appear incorrectly or not appear at all in some plugins. In that case, use the standard view in the arena to see it correctly.
In a simplified version of basketball the goal is to score by getting the ball in a special scoring place. There are two teams, and each team contains the same number of players. When a player has possession of the ball, he has two choices: take a shot, or pass the ball to a teammate.
When taking a shot, the player throws the ball in a straight line to the scoring place. When passing the ball, he throws the ball in a straight line to the target teammate. In both cases, at most one of the rival players will try to intercept the shot or pass.
The probability of a pass being successful is:
Cp * (1 - (ls / 150)2) * dr / (dr + 1)
And the probability of a shot being successful (score) is:
(Cs * dr / (dr + 1))ln(ls)
Where Cp and Cs are constants defined for the problem instance, ls is the length of the shot or pass, dr is the distance between the intercepting rival and the ball trajectory and ln is the natural logarithm (logarithm in base e).
When trying to intercept a shot or a pass, only the best suitable player of the other team to do so (i.e., the one that produces the lowest dr) will try. If no player on the other team can do it, the factor dr/(dr+1) in the formula is considered to have a value of 1 (i.e., it is ignored). A player of the rival team is only allowed to try to intercept the ball if the line that passes through him and is perpendicular to the ball trajectory intersects the trajectory at some point between the two endpoints of the trajectory, inclusive.
For example, in this picture:
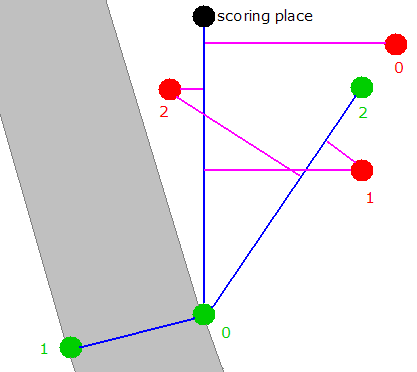
There are 3 players in each team, green players are your team and red players are rivals. Player 0 has the ball and has 3 options marked as blue lines, 2 passes and taking a shot. The shot, if taken, can be intercepted by any of the rivals, but only number 2 will try because he is clearly the nearest. The pass to player 1 is impossible to intercept for the rivals, because any player that can intercept that pass should be inside the gray area. The pass to player 2 can be intercepted by rivals 1 or 2. Rival player 0 is not on an intersecting perpendicular line, so he cannot try to intercept it. In this last case, rival 1 will try to intercept because he is nearer than rival 2.
You will be given two String[]s team and rivals with the same number of elements representing the members of each team. Each element of team and rivals will be in the format "X Y" where X and Y will be positive integers with no leading zeroes representing the x and y coordinates of that player in the field. You will also be given Cp and Cs, the constants for the probability calculations of each type of movement. When the game starts, the ball is in possession of the player on your team with index 0. The scoring place is at X=50, Y=0. Your team is only allowed to take one shot, and you are to determine and return the probability that your team will score if it follows the best strategy. A strategy consists of zero or more passes followed by a shot. If your team loses the ball at any point during the strategy, you will not score. See examples for further clarification.
| | Definition | | Class: | BasketballStrategy | Method: | scoreProbability | Parameters: | String[], String[], double, double | Returns: | double | Method signature: | double scoreProbability(String[] team, String[] rivals, double Cp, double Cs) | (be sure your method is public) |
| | | | Notes | - | The returned value must be accurate to within a relative or absolute value of 1E-9. | - | Pictures are just approximations. The players are considered to be perfect points with 0 surface and 0 length and trajectories and other lines are perfect lines with 0 surface. | - | The same rival may try to intercept many passes along the game (see example 3 for further clarification). | | Constraints | - | team and rivals will each contain exactly N elements, where N is between 1 and 50, inclusive. | - | Each element of team and rivals will be two integers between 1 and 99, inclusive, with no leading zeroes, separated with exactly one space character, with no leading or trailing spaces. | - | All elements of team and rivals together will be distinct. | - | Cp and Cs will each be greater than 0 and less than or equal to 1. | | Examples | 0) | | | {"50 50","35 60","70 15"} | {"75 5","72 25","45 17"} | 1 | 1 |
| Returns: 0.6100612919616956 | This is the example from the problem statement. The best strategy is to pass the ball to player 2 and make him shoot. |
|
| 1) | | | | Returns: 0.3825461314703953 | There's no teammate to pass the ball to, so you must take the shot directly. Since the only rival player is not in a position to intercept, the probability of making the shot is 0.5ln(4). |
|
| 2) | | | | Returns: 0.0 | You can't pass the ball and your rival can perfectly block you; therefore it's impossible to score. |
|
| 3) | | | {"50 50","40 50","40 40","40 30","50 20"} | {"50 41","44 29","48 27","45 41","48 64"} | 0.999999 | 0.8 |
| Returns: 0.25546407305110735 | This picture illustrates the locations. The best strategy is marked in blue and the pink lines show possible interception lines from a nearest rival. Note that passes 2-3 and the shot taken by player 4 cannot be intercepted.
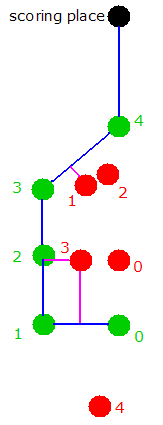
Here player 0 cannot take a shot because he will always be blocked. You should try to get the ball to player 4 so he can take the shot instead. Note that in this case it is better to make more passes and get to player 4 for the shot because the difficulty of longer passes and shots makes other strategies less likely to succeed. |
|
| 4) | | | {"50 50","50 25"} | {"40 40","60 20"} | 1 | 0.7 |
| Returns: 0.20631213370921644 | Note that getting closer can be better even if you are good at taking shots. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are organizing a local Rock-Paper-Scissors Tournament, where the best N players in your town will compete for fame and prizes. You have already ranked the participants according to their performance in the qualifying round, so each one of them will have a seed for winning the tournament in the range from 1 to N.
The tournament will be in a knockout format and will consist of multiple rounds. During each round the competitors still left in the competition are randomly paired up with each other. The winners from each game advance to the next round. The great champion is the winner of the final game between the last two remaining players.
The tournament starts soon, and you are preoccupied with some pre-statistics. You estimate that a player with any seed S can win against players with greater seeds, and against ones with lower seeds, if the difference between their seeds is not greater than sqrt(C * S), where C is a constant. Assuming that the outcome of all the played games during the tournament agrees with your estimation, a player can win the tournament if there exists an assignment of games in each of the rounds such that the player can win each round.
You will be given two ints, rounds, denoting the number of rounds, and the constant C. Return the greatest seed of a player that could win the tournament. Note that N, the number of competitors will be N = 2rounds.
| | Definition | | Class: | RPSTournament | Method: | greatestSeed | Parameters: | int, int | Returns: | int | Method signature: | int greatestSeed(int rounds, int C) | (be sure your method is public) |
| | | | Constraints | - | rounds will be between 1 and 16, inclusive. | - | C will be between 0 and 1500, inclusive. | | Examples | 0) | | | | Returns: 1 | There is no room for surprises here. Since nobody can win against a higher-rated opponent, the lowest seed will win the tournament. |
|
| 1) | | | | Returns: 6 | Player 2 can win against player 1, so both of them can win the final if they are to meet in that phase of the competition. Since players 3 and 4 can beat player 2, they can win the tournament as long as player 2 wins against player 1 somewhere before the final. Finally, players 5 and 6 can beat player 4 in the final, as long as player 4 wins against player 2 in the semifinals and player 2 eliminates player 1 in the first round. It is impossible for players 7 and 8 to win the tournament. We return the greatest seed of those who can win, namely 6. |
|
| 2) | | | | 3) | | | | 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are the manager of a company, and you want all of your employees to be notified of an important news item as quickly as possible. Your company is organized in a tree-like structure: each employee has exactly one direct supervisor, no employee is his own direct or indirect supervisor, and every employee is your direct or indirect subordinate. You will make a phone call to each of your direct subordinates, one at a time. After hearing the news, each subordinate must notify each of his direct subordinates, one at a time. The process continues this way until everyone has heard the news. Each person may only call direct subordinates, and each phone call takes exactly one minute. Note that there may be multiple phone calls taking place simultaneously. Return the minimum amount of time, in minutes, required for this process to be completed.
Employees will be numbered starting from 1, while you will be numbered 0. Furthermore, every supervisor is numbered lower than his or her direct subordinates. You are given a int[] supervisors, the ith element of which is the direct supervisor of employee i. The first element of supervisors will be -1, since the manager has no supervisors.
| | Definition | | Class: | SpreadingNews | Method: | minTime | Parameters: | int[] | Returns: | int | Method signature: | int minTime(int[] supervisors) | (be sure your method is public) |
| | | | Constraints | - | supervisors will contain between 1 and 50 elements, inclusive. | - | Element i of supervisors will be between 0 and i-1, inclusive, except for the first element which will be -1. | | Examples | 0) | | | | Returns: 2 | Call subordinate 1 at time 0 and then subordinate 2 at time 1. By time 2, both subordinates will have heard the news. |
|
| 1) | | | | Returns: 3 | This time call employee 2 first, and then employee 1 at time 1. After hearing the news, employee 2 will call employee 3 at time 1 and employee 4 at time 2. It takes 3 minutes for everybody to hear the news. |
|
| 2) | | | | Returns: 4 | Everyone in the company has only one subordinate, resulting in a chain of phone calls. |
|
| 3) | | | {-1, 0, 0, 1, 1, 1, 2, 2, 3, 3, 4, 4, 5, 5, 6, 7, 7, 8, 12, 13, 14, 16, 16, 16} |
| Returns: 7 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You are programming the process scheduler for an operating system that controls many processors. The parallelism achieved by this new system is total, meaning that any number of processes can run at the same time without disturbing each other. Of course, certain processes may need some other processes to end before they start.
You will be given the execution time of each process as a int[], where the ith element is the number of milliseconds the ith process takes to complete execution. The information about precedences will be given as a String[] prec, where the jth character of the ith element is 'Y' if process j needs process i to be finished before executing itself and 'N' otherwise. Return the minimum number of milliseconds needed for all processes to be finished. If it's impossible for all the processes to finish, return -1.
| | Definition | | Class: | ParallelProgramming | Method: | minTime | Parameters: | int[], String[] | Returns: | int | Method signature: | int minTime(int[] time, String[] prec) | (be sure your method is public) |
| | | | Constraints | - | time will contain between 1 and 50 elements, inclusive. | - | time and prec will contain the same number of elements. | - | Each element of time will be between 1 and 1000, inclusive. | - | Each element of prec will contain exactly N characters, where N is the number of elements in prec. | - | Each element of prec will contain only 'N' and 'Y'. | - | The ith character of the ith element of prec will be 'N'. | | Examples | 0) | | | {150,200,250} | {"NNN",
"NNN",
"NNN"} |
| Returns: 250 | In this case, all processes can run in parallel, so after 250 milliseconds, they will all finish. |
|
| 1) | | | {150,200,250} | {"NNN",
"YNN",
"NNN"} |
| Returns: 350 | In this case, process 0 has to wait for process 1 to end, so the finishing time of process 0 cannot be sooner than 350. Process 2 can execute at any time during those 350 milliseconds because it has no precedence relationships. |
|
| 2) | | | {150,200,250} | {"NYN",
"NNY",
"NNN"} |
| Returns: 600 | In this case there is no parallelism, so the three processes need to be executed in the sequence 0-1-2. |
|
| 3) | | | {150,200,250} | {"NYN",
"NNY",
"YNN"} |
| Returns: -1 | No process can begin execution here because all of them are waiting for another process to finish. Therefore, it is impossible for all of the processes to finish. |
|
| 4) | | | {345,335,325,350,321,620} | {"NNNNNN",
"NNYYYY",
"YNNNNN",
"NNYNYN",
"NNNNNN",
"NNNNNN"} |
| Returns: 1355 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You want to arrange a group of students so that their heights are in non-descending order, and boys and girls alternate with each other.
You will be given a String[] students. Each element of students will be in the format "name height sex" (quotes for clarity only), where height is an integer in centimeters and sex is either "boy" or "girl" (quotes for clarity only). Return a String containing a dash-separated list of the students' names in the desired order. If multiple return values are possible, return the one that comes first lexicographically. If there is no possible ordering, return "" (empty String). Note that dashes ('-') come earlier lexicographically than letters.
| | Definition | | Class: | StudentsOrdering | Method: | findOrder | Parameters: | String[] | Returns: | String | Method signature: | String findOrder(String[] students) | (be sure your method is public) |
| | | | Constraints | - | students will contain between 1 and 50 elements, inclusive. | - | Each element of students will be contain between 1 and 50 characters, inclusive. | - | Each element of students will be formatted as described in the problem statement. | - | The name of each student will consist of letters ('A'-'Z', 'a'-'z') only. | - | The height of each student will be between 100 and 250, inclusive. | - | The height of each student will contain no leading zeroes. | | Examples | 0) | | | {"Alex 180 boy",
"Josh 181 boy",
"Mary 158 girl",
"An 158 girl",
"Tanya 180 girl",
"Ted 158 boy"} |
| Returns: "An-Ted-Mary-Alex-Tanya-Josh" | |
| 1) | | | {"Alex 180 boy",
"Josh 158 boy",
"Mary 180 girl",
"An 158 girl",
"Mary 180 girl",
"Ted 158 boy"} |
| Returns: "Josh-An-Ted-Mary-Alex-Mary" | Student names can repeat. |
|
| 2) | | | {"Alex 180 boy",
"Josh 170 boy",
"An 158 girl",
"Mary 180 girl",
"Ted 175 boy"} |
| Returns: "" | There is no girl to place between Josh and Ted. |
|
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Year 2100. Humanity is starting to inhabit Antarctica. So far there are N towns, some of them connected with narrow one-way roads.
The president of Antarctica has decided to establish police in his country. The cost of building a police station in each town is given. However, there may not be a need to build a police station in every town. The only requirement is that every town must be reachable from some police station using roads.
As the next elections are approaching, the president doesn't want to expose large police expenses. Thus he wants the average cost of a built station to be as low as possible.
You are given a int[] costs, the i-th element of which represents the cost of building a police station in town i, and a String[] roads representing the layout of the one-way roads. The j-th character of the i-th element of roads is 'Y' if there is a road from town i to town j, or 'N' if there is not. Make the average cost of a built station to be as low as possible and return this cost. | | Definition | | Class: | AntarcticaPolice | Method: | minAverageCost | Parameters: | int[], String[] | Returns: | double | Method signature: | double minAverageCost(int[] costs, String[] roads) | (be sure your method is public) |
| | | | Notes | - | The returned value must be accurate to within a relative or absolute error of 1E-9. | | Constraints | - | costs will contain between 1 and 50 elements, inclusive. | - | Each element of costs will be between 1 and 1000, inclusive. | - | roads will contain exactly N elements, where N is the number of elements in costs. | - | Each element of roads will contain exactly N characters. | - | Each character of each element of roads will be 'Y' or 'N'. | - | The ith character of the ith element of roads will be 'N'. | | Examples | 0) | | | {1,2,3,4} | {"NYNN","NNYN","NNNY","YNNN"} |
| Returns: 1.0 | It is possible to get everywhere from anywhere (the towns form a big circle), so we need only the cheapest station. |
|
| 1) | | | {1,2,3,4} | {"NYNN","NNYN","NNNY","NYNN"} |
| Returns: 1.0 | Once again, the cheapest station is enough. |
|
| 2) | | | {5,6,7,8} | {"NYNN","YNNN","NNNY", "NNYN"} |
| Returns: 6.0 | We have two separate parts, so we build one cheapest station in each part : (5+7)/2=6. |
|
| 3) | | | | Returns: 7.5 | We have three choices here: build a police station in town 0, in town 1, or in both towns. The second choice doesn't satisfy the requirements since it's impossible to get from town 1 to town 0. Of the two remaining choices, building both is better because it minimizes the average cost. |
|
| 4) | | | {34,22,25,43,12} | {"NYNNY","YNNYN","NNNYY","NNNNN","NNNNN"} |
| Returns: 19.666666666666668 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | This problem contains an image that can be viewed in the applet.
A player has four draughts which are placed in the bottom right corner of a 6 x 6 chessboard. The draughts are arranged in a square with 2 rows and 2 columns (see picture below). Some cells on the board may contain red flags, and some may contain stones.
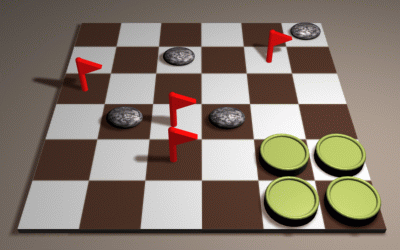
The player's goal is to move all the draughts to the top left corner and arrange them in a square using a minimal number of moves. The draughts are indistinguishable, so their order in the final position doesn't matter. The target cells are guaranteed to be free. There are two kinds of moves that can be made by a draught. The first is to move to any vertically or horizontally adjacent free cell. The second is to jump over a single vertically or horizontally adjacent draught or stone into a free cell. The player can never move a draught into a cell that contains a flag, stone or other draught and he can never jump over a flag or an empty space.
You will be given a String[] board where each element represents a single row of the chessboard. The rows are given from top to bottom, and each row is given from left to right. '.' represents a free cell, 'r' represents a cell with a red flag, and 's' represents a cell with a stone. Return the minimal number of moves necessary to reach the goal, or -1 if it is impossible.
| | Definition | | Class: | CornersGame | Method: | countMoves | Parameters: | String[] | Returns: | int | Method signature: | int countMoves(String[] board) | (be sure your method is public) |
| | | | Constraints | - | board will contain exactly 6 elements. | - | Each element of board will contain exactly 6 characters. | - | Each element of board will contain only the characters 'r', 's', and '.'. | - | board will contain '.' characters at the initial and desired final positions of the draughts. | | Examples | 0) | | | {"......",
"......",
"......",
"......",
"......",
"......"} |
| Returns: 16 | |
| 1) | | | {".....s",
"..s.r.",
"r.....",
".srs..",
"..r...",
"......"} |
| Returns: 19 | The board shown on the picture above. |
|
| 2) | | | {"......",
"......",
"....ss",
"....ss",
"...r..",
"...r.."} |
| Returns: -1 | We can not make any move. |
|
| 3) | | | {"...s.r",
"..r.s.",
"rr.s..",
"..s.rr",
"s.rr..",
".s.s.."} |
| Returns: 54 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are given a int[] data. All elements in data are distinct. Only consecutive pairs of elements in data can be swapped. You are allowed to perform at most nSwaps swaps. Return the lexicographically maximal possible result.
| | Definition | | Class: | PartSorting | Method: | process | Parameters: | int[], int | Returns: | int[] | Method signature: | int[] process(int[] data, int nSwaps) | (be sure your method is public) |
| | | | Notes | - | Given two int[]s a and b of equal length, a is lexicographically greater than b if an index i (0 <= i < n) exists such that a[0] = b[0], a[1] = b[1], ..., a[i - 1] = b[i - 1] and a[i] > b[i], where n is the size of a. | | Constraints | - | data will contain between 1 and 50 elements, inclusive. | - | Each element in data will be between 1 and 1000000, inclusive. | - | All elements in data will be distinct. | - | nSwaps will be between 0 and 1000000, inclusive. | | Examples | 0) | | | {10, 20, 30, 40, 50, 60, 70} | 1 |
| Returns: {20, 10, 30, 40, 50, 60, 70 } | Swap the first two elements. |
|
| 1) | | | | Returns: {5, 3, 2, 1, 4 } | First, swap the third and fourth elements, and then, swap the first and second elements.
|
|
| 2) | | | {19, 20, 17, 18, 15, 16, 13, 14, 11, 12} | 5 |
| Returns: {20, 19, 18, 17, 16, 15, 14, 13, 12, 11 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are given a directed graph g, and you must determine the number of distinct cycles in g that have length less than k. Since this number can be really big, return the answer modulo m. A cycle is a non-empty sequence of nodes (not necessarily distinct) in which there is an edge from each node to the next node, and an edge from the last node in the sequence to the first node. Two cycles are distinct if their sequences are not identical. See example 0 for further clarification.
g will be given as a String[] where the jth character of the ith element indicates whether there is an edge from node i to node j ('Y' means there is one, and 'N' means there is not). | | Definition | | Class: | TourCounting | Method: | countTours | Parameters: | String[], int, int | Returns: | int | Method signature: | int countTours(String[] g, int k, int m) | (be sure your method is public) |
| | | | Notes | - | The answer modulo m means that you must return the remainder of dividing the result by m. | | Constraints | - | g will have between 1 and 35 elements, inclusive. | - | Each element of g will have exactly N characters, where N is the number of elements in g. | - | Each character of each element of g will be 'Y' or 'N'. | - | The ith character of the ith element of g will be 'N'. | - | k will be between 1 and 1000000 (106), inclusive. | - | m will be between 1 and 1000000000 (109), inclusive. | | Examples | 0) | | | {"NYNY",
"NNYN",
"YNNN",
"YNNN"} | 6 | 100 |
| Returns: 12 | The possible cycles with length less than 6 are:
(0,3) ; (3,0) ; (0,1,2) ; (1,2,0) ; (2,0,1)
(0,3,0,3) ; (3,0,3,0) ; (0,1,2,0,3) ; (0,3,0,1,2)
(1,2,0,3,0) ; (2,0,3,0,1) ; (3,0,1,2,0)
Note that (0,3), (3,0) and (0,3,0,3) are all considered different. |
|
| 1) | | | {"NYNNNNN",
"NNYNNNN",
"NNNYNNN",
"NNNNYNN",
"NNNNNYN",
"NNNNNNY",
"YNNNNNN"} | 40 | 13 |
| Returns: 9 | All cycles have lengths that are multiples of 7. For each starting node and each multiple of 7 there exists one cycle. There are 5 non-zero multiples of 7 that are less than 40 (7,14,21,28,35) and 7 possible starting nodes. Therefore, the total number of cycles is 5x7=35. 35 modulo 13 is 9. |
|
| 2) | | | {"NYNY",
"NNNN",
"YYNY",
"NYNN"} | 1000000 | 1000000000 |
| Returns: 0 | The graph does not have cycles. |
|
| 3) | | | | Returns: 0 | Any number modulo 1 is zero. |
|
| 4) | | | {"NYYNYYN",
"YNYNYNY",
"NYNYNYN",
"YYYNYNY",
"NNYYNNY",
"NYYYNNY",
"YYYYYYN"} | 30 | 100 |
| Returns: 72 | |
| 5) | | | {"NYYY",
"YNYY",
"YYNY",
"YNYN"} | 1000 | 10000 |
| Returns: 3564 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Given a simple undirected graph G, your task is to partition it into C disjoint sets of nodes. Your goal is to do this in such a way that as few edges are cut as possible, while each of the sets is relatively large. More specifically, you want to minimize the ratio: (edges cut)/(size of smallest set).
In order to receive any points on a test case, your ratio must be within 0.01% of the smallest ratio of any competitor. Assuming that you achieve this ratio, your score will be based on the time it takes you to find the answer. Your score for a test case will be inversely proportional to the time it takes plus 100 milliseconds, while your total score will simply be the sum of the scores for the test cases. Thus, if you have the smallest ratio on two cases with times 100 and 200 milliseconds, your score will be proportional to 1/(100+100)+1/(200+100).
The graphs will be generated as follows:
- C, the number of clusters (and input to you) will be chosen uniformly between 2 and 10, inclusive.
- N, the number of nodes in the graph will be chosen uniformly between 100 and 5,000, inclusive.
- p, the probability of an edge existing between nodes in different clusters, will be chosen uniformly between 0.05 and 0.25, inclusive.
- q, the probability of an edge between nodes in the same cluster, will be chosen uniformly between p+2*sqrt(p*C/N) and p+8*sqrt(p*C/N) (with the upper bound capped at 1).
- A graph is generated with N nodes, each node is in one of the C clusters with equal probability. Every undirected edge is then added with probability p or q depending on whether the edge is between different clusters or within one cluster.
For example, consider a graph with two clusters and 100 nodes, where nodes 0-49 are in cluster 0, while nodes 50-99 are in cluster 1. Imagine that p were chosen as 0.1, and q was chosen as 0.2. Then, for every pair of integers (i,j), if i and j were both in the same cluster (both 0-49 or both 50-99), there would be an edge between i and j with probability 0.2. If i and j were in different clusters, then the probability of an edge between i and j would be 0.1. Thus, in this case we would expect each node to have 49*0.2 edges to nodes in the same cluster, and 50*0.1 edges to nodes in the other cluster. If our algorithm found this parition, we would expect it to cut roughly 50*0.1*50=250 edges, and thus have a ratio of 250/50 = 5.
The graph will be given to you as two int[]'s: u and v. Corresponding elements of u and v represent a single undirected edge. For instance, there is an edge between u[0] and v[0]. The number of nodes are given as an input nodes. Your return should be a int[] with nodes elements. Element i of the return should represent the partition (out of C total) that node i is in. Everything is indexed from 0, including the return. | | Definition | | Class: | PartitionGraph | Method: | partition | Parameters: | int[], int[], int, int | Returns: | int[] | Method signature: | int[] partition(int[] u, int[] v, int nodes, int C) | (be sure your method is public) |
| | | | Notes | - | The graph is undirected, so edges will only be listed once in the input. | - | The time limit is 60 seconds. | - | The memory limit is 1024 megabytes. | - | A partition of nodes into C disjoint sets means that you should assign an integer between 0 and C-1 to every node. | - | An edge is cut if its two end points are assigned different numbers (as described in the previous note). | - | The scores you see for individual test cases encode the number of edges you cut and the size of your minimum partition. If the score is written down as an integer, the last 5 digits are your time, the 4 digits before them gives the size of your smallest partition, while the rest of the digits give the number of edges cut. For example, the score 1096 0012 00004 (spaces for clarity) indicates that your time was 4 milliseconds, your smallest cluster was 12 nodes, and you cut 1096 edges. This information will also appear in the "fatal errors" section for examples, though it is clearly not actually a fatal error. | - | There are 20 non-example test cases. After the competition is over, all solutions will be re-run on a larger test set. | - | The total scores will be normalized such that the highest score is exactly 10,000. | - | The zip files for the examples contain one edge per line. | | Constraints | - | The graph will be generated as described above. | | Examples | 0) | | | | Returns:
"This graph has 100 nodes, and 9 clusters. p = 0.1298495467416172, q = 0.562381664187069. The input C=9 " | |
| 1) | | | | Returns:
"This graph has 100 nodes, and 2 clusters. p = 0.05512270939535211, q = 0.23170504638351752. The input C=2 " | |
| 2) | | | | Returns:
"This graph has 100 nodes, and 6 clusters. p = 0.07393663470458767, q = 0.42640730225653994. The input C=6 " | |
| 3) | | | | Returns:
"This graph has 2103 nodes, and 4 clusters. p = 0.23752417679934573, q = 0.3425110757342353. The input C=4 " | |
| 4) | | | | Returns:
"This graph has 4909 nodes, and 10 clusters. p = 0.11841855221632161, q = 0.20495237307524405. The input C=10 " | |
| 5) | | | | Returns:
"This graph has 3064 nodes, and 2 clusters. p = 0.20617708771524107, q = 0.28020071025728954. The input C=2 " | |
| 6) | | | | Returns:
"This graph has 390 nodes, and 10 clusters. p = 0.06041993112425246, q = 0.2733661251252104. The input C=10 " | |
| 7) | | | | Returns:
"This graph has 2789 nodes, and 4 clusters. p = 0.16688716607823517, q = 0.2312691747743178. The input C=4 " | |
| 8) | | | | Returns:
"This graph has 2953 nodes, and 2 clusters. p = 0.1474143432742407, q = 0.18596635921872035. The input C=2 " | |
| 9) | | | | Returns:
"This graph has 4324 nodes, and 9 clusters. p = 0.20187512571848237, q = 0.2749548626937394. The input C=9 " | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A random walk in a directed graph starts at some node in the graph and then follows one of that node's outgoing edges uniformly at random. The process is then repeated from the next node and so on. If a graph consists of a single strongly connected component, then a random walk will eventually visit every node in the graph. In this problem, your program will make a random walk of a graph. However, you will only be able to observe the in and out degrees of the vertices that you visit. Your task is to make as short a random walk as possible such that you have visited every node in the graph.
You should write two methods: init and walk. The init method will be called first with a single integer argument: the number of vertices in the graph. Subsequent calls will be made to the walk method and will give a sequences of in and out degree observations. Both of these methods should return an integer between 0 and one million, inclusive, indicating the number of steps your program wants to move at once. So, if you want to take 10 steps in the first iteration, your init method should return 10. Then, you will be given 10 degree observations in the next call to the walk method.
You are allowed to observe at most 10 sequences of degrees (walk will be called at most 10 times). Each time it will be given a int[] with 2 elements per observations. Element 2*i and 2*i+1 will given the in and out degrees of the ith observation, respectively. If you ever return 0, it will be assumed that you are done and want to make no further observations.
Scoring will be based on two factors: the number of nodes that you visit before quiting, and the total number of observations you make. In the best case, you will stop as soon as you hit the last unvisited node, in which case you will get 100 points. If you don't visit all the nodes, you will lose half your points for each unvisited nodes, so your score will be 100*0.5unvisited. If you visit all the nodes and make some additional observations, your score will be 100-(percent extra observations)/3 (with a minimum of 0). For example, if you visited all but 2 nodes, you would get 25 points. If you visisted the last node on observation 100, but you made a total of 130 observations, you would receive 90 points (having made 30% extra observations). Your total score will simply be the sum of your individual scores.
The graphs will be generated via a very simple model. First, the number of nodes will be selected between 10 and 100, inclusive, with size K chosen with probability proportional to 1/K. Then, a probability, p is chosen between 0 and 10/K. For each potential edge (u,v) (where u does not equal v), that edge is added with probability p. If the generated graph has a single strongly connected component, it is kept, otherwise, p and all the edges are regenerated (the size is kept), and the process is repeated until a graph with a single strong component is generated. | | Definition | | Class: | RandomWalking | Method: | init | Parameters: | int | Returns: | int | Method signature: | int init(int nodes) | | | Method: | walk | Parameters: | int[] | Returns: | int | Method signature: | int walk(int[] seq) | (be sure your methods are public) |
| | | | Notes | - | You will have a total of 20 seconds execution time (not 20 seconds per sequence). | - | The memory limit is 64 MB. | - | There are 100 test cases. | - | Graphs are given as adjacency lists. For instance, in example 0, there are edges from node 0 to nodes 1, 2 and 9. | - | The return from the 10th call to walk is ignored (treated as if it is 0). | - | The calls to walk form a single continuous random walk. In other words, concatenating all of the inputs to the walk methods gives a continuous walk. | | Examples | 0) | | | | Returns:
"0: 1 2 9
1: 0 4 5 6 7
2: 3 8 9
3: 2 5 9
4: 0 2 3 6 7 8
5: 8
6: 3 4 5 7 9
7: 1 4 6 8 9
8: 1 3 7
9: 0 1 2 5 7
" | |
| 1) | | | | Returns:
"0: 4 5 7 8 9
1: 0 3 4 5 6 7 8 9
2: 0 1 3 4 5 6 7 9
3: 0 1 2 4 5 6 7 8 9
4: 1 3 5 6 8 9
5: 0 1 2 3 4 6 7 8 9
6: 1 2 3 4 7 8 9
7: 0 1 2 3 5 6 8 9
8: 1 2 3 4 5 7 9
9: 0 2 3 4 6 8
" | |
| 2) | | | | Returns:
"0: 19 28 41 43 45 46 48
1: 4 11 15 17 19 25 26 34 39 40 44 47
2: 3 9 12 16 31 34 40 43 46
3: 2 5 13 16 25 28 30 32 35 38 40 44
4: 6 14 18 22 31 35 47
5: 2 4 14 16 20 26 27 37
6: 0 5 12 14 16 21 25 27 33 36 37 44 47
7: 0 31 32 36 37 43 44
8: 0 10 17 23 27 29 31 32 33 35 37 40 45 47
9: 2 3 10 11 14 15 16 32 38 48
10: 6 8 12 23 37 44 48
11: 8 9 10 17 19 20 34 48
12: 1 2 13 20 22 30 31 33 39 41 45
13: 1 6 9 19 33 36 41 45
14: 6 20 22 25 31 37 41 43 49
15: 3 8 9 21 24 33 39
16: 6 12 22 23 24 26 27 29 36 38 39 44
17: 5 9 13 21 23 24 27 34 39 42 43 45 49
18: 9 10 14 20 22 24 25 26 29 31 32 35 44
19: 12 16 20 22 23 25 28 34 35 46
20: 0 1 2 3 7 11 18 21 25 27 44 45 49
21: 3 4 7 9 10 14 23 28 33 37 42 48 49
22: 1 7 12 19 21 26 30 40 43 44
23: 0 3 4 5 8 9 11 14 27 30 38 41 43 45 49
24: 5 6 8 11 15 16 23 30 32 34 35 36 37 42
25: 0 8 16 18 19 31 33 34 46
26: 0 11 13 27 33 35 40 45 49
27: 7 11 21 23 38 39 40
28: 0 7 8 9 24 27 30 31 33 41 44
29: 6 7 9 12 18 19 26 30 32 34 38 41 47
30: 1 4 7 14 18 22 23 26 29 31 32 44 45 47
31: 0 4 11 14 18 22 29 30 37 45
32: 9 13 17 20 21 24 26 28 29 30 35 42
33: 6 8 9 14 16 21 22 24 25 28 30 37 40 42 45 47
34: 7 21 29 36 43
35: 11 15 18 41 43 46
36: 9 25 28 48
37: 0 7 10 18 28 38 42
38: 3 4 12 13 15 17 18 23 27 35
39: 1 19 35 37 47
40: 9 16 18 29 38 42
41: 2 3 15 16 17 22 25 32 36 37 40 42 45
42: 2 9 15 16 23 28 30 34
43: 0 8 22 23 27 29 33 37 45 46
44: 2 18 30 42 47 48
45: 0 8 12 26 32 34 40
46: 7 9 18 23 24 25 30 33 36 37 40
47: 11 12 13 14 19 29 36 44 48
48: 15 23 25 33 38
49: 12 18 22 25 26 42 43
" | |
| 3) | | | | Returns:
"0: 1 6 7 10 11 14 17 18 19 20 24 25
1: 0 2 3 4 6 7 8 17 23 25
2: 0 3 4 5 6 11 16 19 21 24
3: 0 2 10 13 14 15 16 18 20 23
4: 0 3 10 13 14 15 18 20 21 22 23 25
5: 6 8 11 12 14 15 16 17
6: 0 3 5 8 9 10 11 12 16 19 20 22 23
7: 1 17 18 20 23
8: 1 4 5 7 12 13 15 17 20 22
9: 1 4 5 6 8 11 12 17 19 21 22
10: 1 5 7 9 11 14 16 18 19
11: 0 4 6 9 10 18 21 24
12: 2 3 5 7 8 9 10 11 17 19 20
13: 1 7 8 9 12 22 24
14: 1 4 7 8 9 16 17 21
15: 1 7 10 12 16 18 19 22
16: 1 13 14 15 21 22
17: 0 2 4 14 15 18
18: 0 3 4 12 16 17 19 22 23 24
19: 0 1 4 7 8 9 10 11 12 20 21 22 24
20: 2 3 4 5 6 7 9 10 12 13 16 17 19 22 24 25
21: 0 1 6 7 8 9 14 20 25
22: 3 4 5 6 7 10 11 17
23: 2 4 5 6 8 12 16 18 20 24 25
24: 0 9 11 13 14 17 18 25
25: 0 1 6 7 9 15 21 23 24
" | |
| 4) | | | | Returns:
"0: 1 2 3 4 5 6 7 8 9 10
1: 0 2 4 5 8 10
2: 0 3 4 5 6 7 8 10
3: 0 1 2 4 5 6 7 8 10
4: 0 1 2 3 6 7 8 10
5: 0 1 2 3 6 8 9 10
6: 0 1 2 3 4 7 8 9 10
7: 0 1 2 3 4 8 9 10
8: 1 3 4 6 7 9 10
9: 0 1 2 3 4 5 7 8 10
10: 0 1 2 3 5 8 9
" | |
| 5) | | | | Returns:
"0: 5 22 25 28 63
1: 13 24 26 43 58 61
2: 5 9 14 17 20 23 26 40 50
3: 9 27 35 37 38 49 54 57
4: 29 44 45 62
5: 9 44 48 62 63
6: 9 18 31 43 46 61 65
7: 0 12 19 25 34 39
8: 9 17 21 44 53 62 63
9: 0 3 5 17 27 44 53
10: 7 16 24 37 38 56 67
11: 3 4 13 15 32 50 64
12: 1 7 14 20 28 29 30 32 52 59 60 65
13: 2 4 34 44 51 57
14: 8 23 28 36 53 54 55 62 63 65
15: 1 6 27 30 48 55 57
16: 2 10 26 47 53 61 66
17: 5 8 11 24 30 41 42 50 59
18: 0 6 11 33 35 44 52 59 68
19: 6 41 49 55
20: 18 25 36 39 48 58
21: 1 4 19 27 30 35 43 47 48
22: 11 15 32 41 58 62 66
23: 5 17 18 20 21 34 35 43
24: 0 5 37 50 51 61 62 63
25: 7 15 21 36
26: 8 10 20 36 41 43 56
27: 3 12 14 34 38 51 55 59 63 64 66
28: 3 15 26 49 56
29: 2 9 22 35 41 44 46 49 59 63
30: 28 32 40 41
31: 5 9 27 49 52 55 56
32: 2 5 33 41 55 57 59 63
33: 26 38 51 63 66
34: 14 41 42
35: 7 10 28 31 57 67
36: 2 13 19 50
37: 6 13 14 41 47 49 54 60 63
38: 6 22 39
39: 9 13 16 22 48 58
40: 8 25 28 47 59
41: 0 8 18 25 38 46 53 60 61 62
42: 13 18 33 39 56 60 65
43: 2 5 13 14 27 34 57
44: 8 11 17 25 27 37
45: 16 36 38 48 50 56 59 64 65
46: 1 3 8 14 16 19 24 50 64
47: 14 25 32 40 60 67
48: 3 10 18 32 35 50 56 61
49: 8 20 25 29 43 54
50: 12 13 14 16 31 33 34 47 49 57 58 61
51: 24 36 46 61 62
52: 33 35 38 41 44 48 64
53: 5 12 13 24 28 40
54: 3 22 34 42 45 65
55: 1 9 10 15 16 31 36 38 61 67
56: 4 6 9 32 38 54 59 67
57: 5 13 16 23 24 25 29 35 36 41 46 54 65
58: 13 18 23 26 33 34 57 60 61
59: 0 4 14 19 35 37 42 47 54 65
60: 8 18 21 24 53 66
61: 0 3 11 13 19 22 51 62
62: 4 8 20 28 48 49 50 54
63: 2 3 28 35 39 51 52 53 64 68
64: 13 21 36 46 55
65: 3 6 14 29 36 43 50 55
66: 19 20 25 54 55 56 59
67: 3 5 7 17 19 22 48 54 59
68: 2 8 20 23 31 48
" | |
| 6) | | | | Returns:
"0: 1 19 24 32
1: 7 13 20 27
2: 3 5 29
3: 6 16 28 29 30
4: 6
5: 11 14 16 17 22 31
6: 1 2 8 31
7: 29 30
8: 4 10 12 19
9: 15 22
10: 0 12 13 18 29
11: 2 7 17 20
12: 11 13 20 27 29
13: 3 5 6 11 23
14: 21 25 27 28
15: 11 24 25 31
16: 1 29
17: 5 9 28
18: 1 6 29
19: 5 6 24 26
20: 0 5 12 17 30 31
21: 14 20 30
22: 1 15 19 20 26 30
23: 6 8 13 26 28
24: 0 13 14 16 20
25: 3 23 31
26: 14 20 23 24
27: 3 15 20 23 30
28: 1 10 12 22 29 31
29: 9 18 28 31 32
30: 27
31: 5 16 24 30
32: 26 28 30 31
" | |
| 7) | | | | Returns:
"0: 3 4 7 10 11 13 15
1: 2 3 5 8 9 13 17
2: 4 5 8 9 17
3: 6 9 12
4: 3 5 6 10 11 13 15 17
5: 0 2 3 6 7 11 13 16
6: 1 2 3 4 8 10 11 13 14 15 17
7: 0 1 2 3 9 12 13 15 16
8: 0 5 7 14
9: 0 3 5 6 7 8 10 13 17
10: 0 1 2 3 5 6 8 14 15 16
11: 0 1 4 12 16 17
12: 1 2 3 5 6 9 11
13: 3 5 7 8 14 15 16 17
14: 2 3 4 7 11 12 13 16
15: 1 2 4 6 7 8 10 12 13
16: 0 5 6 8 10 12 13 14
17: 2 5 7 8 13
" | |
| 8) | | | | Returns:
"0: 1 2 5 6 7 9 10 11
1: 4 6 10
2: 5 6 8 10
3: 4 5 7 9
4: 5 7 8 9 11
5: 0 1 3 7 9 10
6: 2 3 4 5 8 11
7: 1 4 6 9 10
8: 4 6 9 11
9: 2 8 11
10: 0 1 2 3 6 8
11: 6
" | |
| 9) | | | | Returns:
"0: 1 7 12 13 18 19 38 45
1: 5 6 12 14 21 26 31 35 38 43 44
2: 4 5 6 16 17 27 33 36 43
3: 7 8 9 16 23 28 29 30 46
4: 3 7 17 23 28 31 32 35 36 37 38 39 46
5: 1 4 13 14 15 17 18 31 33 41
6: 2 7 14 15 18 29 30 34 38 42 44
7: 2 10 11 12 16 18 19 35 45
8: 16 18 19 28 38 47
9: 3 18 21 37 38 39 41 46
10: 0 3 5 7 9 19 23 27 29 33 34 38
11: 5 20 22 36 38 39 41
12: 4 10 16 17 26 33 37
13: 1 3 6 7 11 21 22 25 26 30 31 32 34 36 37 44
14: 1 5 7 8 12 16 18 30 31 32 40 41 42
15: 2 10 14 20 25 35 40
16: 8 12 14 15 20 21 25 26 35 36
17: 2 12 14 15 20 26 28 29 32
18: 5 8 9 13 14 17 19 23 26 27 38
19: 7 16 17 28 29 35 36 39 40 42 45
20: 1 6 9 12 13 27 29 33 39 47
21: 15 23 26 33 41 44
22: 10 12 21 32 35 36 39 41 42 45
23: 0 4 7 10 24 28 32 36 38 41 47
24: 6 8 10 12 14 16 18 21 29 32 33 39 41
25: 0 9 16 21 24 27 36 37 38
26: 2 10 14 16 17 22 33 34 37 43 45
27: 4 10 11 18 23 28 34 35 36 39 41 43
28: 8 9 13 15 22 24 25 26 30 35 40
29: 5 9 24 26 31 33 46
30: 7 9 13 17 21 41
31: 4 9 14 15 23 24 40
32: 5 9 10 11 16 17 18 24 37 40 41 43
33: 2 6 7 16 21 25 39 42 44 46
34: 1 2 5 8 10 11 14 20 28 32 35 40 46
35: 1 10 13 15 16 24 40 41
36: 3 8 10 14 15 17 26 30 40 41 45 46
37: 1 4 17 22 23 25 26 28 29 39 43
38: 2 5 23 24 27 28 31 45
39: 1 3 7 16 17 34 41 46
40: 1 14 20 24 29 33 38 44
41: 2 6 12 23 31 34 40 42 47
42: 1 2 12 15 29 30 38 39 47
43: 9 12 31 38
44: 3 5 7 10 20 35 36 37 40 46
45: 1 4 6 17 21 23 25 30 31 34 40 42 46
46: 4 5 7 11 13 23 29 31 34 41
47: 4 8 9 14 17 21 32 36 38 44 45
" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A spell is defined by its incantation, the word spoken when the spell is being cast. If you know the incantation of a spell, you can create counterspells in the following manner. First, you can optionally delete any number of characters from the original incantation. Note that empty incantations are not valid so you cannot delete all characters. Then, you can replace any number of the remaining characters according to a set of replacement rules. The replacement rules tell you which letters can be replaced, and the letters with which they can be replaced. For example, if the original incantation is "AA" and the allowed replacement is 'A' to 'Z', the following counterspells are possible: "AA", "A", "Z", "ZZ", "AZ", "ZA".
The best counterspell is the one with the greatest power. The power of a spell is the product of all its character values modulo 77077, where the value for a character is its position in the alphabet (character 'A' has value 1, character 'B' value 2, character 'C' value 3, and so on). The best possible counterspell for "AA" in the example above is "ZZ", which has a power of 676.
Please note that if the allowed replacements are 'A' to 'B' and 'B' to 'C', the counterspell for "AB" is "BC" not "CC". You cannot change a character that has already been changed, even if it would lead to a more powerful spell.
You will be given a String spell, the incantation of the spell you are going to counter, and a String rules, the allowed replacements for letters. The first character in rules is the allowed replacement for the letter 'A', the second for 'B' and so on. The character '-' is used to denote a letter that cannot be replaced. Your program must return a String, the most powerful counterspell available. If multiple return values are possible, return the shortest among them. If a tie still exists return the lexicographically earliest. | | Definition | | Class: | Wizarding | Method: | counterspell | Parameters: | String, String | Returns: | String | Method signature: | String counterspell(String spell, String rules) | (be sure your method is public) |
| | | | Constraints | - | spell will contain between 1 and 13 characters, inclusive. | - | spell will contain only uppercase letters ('A'-'Z'). | - | rules will contain exactly 26 characters. | - | rules will contain only uppercase letters ('A'-'Z') and dashes ('-'). | | Examples | 0) | | | "AA" | "Z-------------------------" |
| Returns: "ZZ" | The example from the problem statement. |
|
| 1) | | | "AB" | "ZS------------------------" |
| Returns: "ZS" | The possible counterspells are "AB", "A", "B", "Z", "S", "ZB", "AS" and "ZS".
"ZS" is the most powerful. |
|
| 2) | | | "ZZZZ" | "-------------------------Z" |
| Returns: "ZZZZ" | |
| 3) | | | "ABCDE" | "ZYXXYXZZXYXXZZXZYYXZZZX---" |
| Returns: "ZXXE" | |
| 4) | | | "ABCDEABCDEABC" | "ACBDESKADSLOEDDDASDBADEDAE" |
| Returns: "CCDECCECC" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | In a jail, some pairs of prisoners are chained together to make it more difficult for them to escape. A group of rebels has decided to escape, and one important part of their plan involves pressing two distant buttons simultaneously. They must determine the two prisoners best suited for this task.
Given the configuration of chains between prisoners, return the maximum distance that can be achieved between two prisoners. If there is no limit to that distance, return -1. You will be given a String[] chain, where the ith character of the jth element represents the length of the chain between prisoners i and j:
'0'-'9': Distances 0 to 9 feet, in order.
'a'-'z': Distances 10 to 35 feet, in order.
'A'-'Z': Distances 36 to 61 feet, in order.
' ': Space means there is no chain between that pair of prisoners. | | Definition | | Class: | EscapingJail | Method: | getMaxDistance | Parameters: | String[] | Returns: | int | Method signature: | int getMaxDistance(String[] chain) | (be sure your method is public) |
| | | | Constraints | - | chain will have between 2 and 50 elements, inclusive. | - | Each element of chain will have exactly N characters, where N is the number of elements of chain. | - | Character i of element j of chain and character j of element i of chain will be equal. | - | Character i of element i of chain will be '0'. | - | Each character of each element of chain will be a digit ('0'-'9'), an uppercase letter ('A'-'Z'), a lowercase letter ('a'-'z') or a space ' '. | | Examples | 0) | | | {"0568",
"5094",
"6903",
"8430"} |
| Returns: 8 | Prisoners 1 and 2 are chained with length 9. However, they are both also chained to prisoner 3 with chains of length 3 and 4 respectively, so they cannot stand more than 7 feet apart. Prisoners 0 and 3, on the other hand, can stand 8 feet apart without trouble. |
|
| 1) | | | | Returns: -1 | Both prisoners are completely unchained, so there is no limit to the distance they can achieve. |
|
| 2) | | | {"0AxHH190",
"A00f3AAA",
"x00 ",
"Hf 0 x ",
"H3 0 ",
"1A 0 ",
"9A x 0Z",
"0A Z0"} |
| Returns: 43 | |
| 3) | | | | Returns: 0 | They are not going too far. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are given a String[] words. Return the shortest String that contains all the words as substrings. If there are several possible answers, return the one that comes first lexicographically.
| | Definition | | Class: | JoinedString | Method: | joinWords | Parameters: | String[] | Returns: | String | Method signature: | String joinWords(String[] words) | (be sure your method is public) |
| | | | Constraints | - | words will contain between 1 and 12 elements, inclusive. | - | Each element of words will contain between 1 and 50 characters, inclusive. | - | Each element of words will consist of only uppercase letters ('A'-'Z'). | | Examples | 0) | | | | Returns: "ABAB" | There are two strings of length 4 that contain both given words: "ABAB" and "BABA". "ABAB" comes earlier lexicographically. |
|
| 1) | | | {"ABABA", "AKAKA", "AKABAS", "ABAKA"} |
| Returns: "ABABAKAKABAS" | |
| 2) | | | {"AAA","BBB", "CCC", "ABC", "BCA", "CAB"} |
| Returns: "AAABBBCABCCC" | |
| 3) | | | {"OFG", "SDOFGJTILM", "KBWNF", "YAAPO", "AWX", "VSEAWX", "DOFGJTIL", "YAA"} |
| Returns: "KBWNFSDOFGJTILMVSEAWXYAAPO" | |
| 4) | | | {"NVCSKFLNVS", "HUFSPMRI", "FLNV", "KMQD", "RPJK", "NVSQORP", "UFSPMR", "AIHUFSPMRI"} |
| Returns: "AIHUFSPMRINVCSKFLNVSQORPJKMQD" | |
| 5) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Given a collection of facts, we would like to get rid of as much redundancy as possible.
The facts involved in this problem are members of a transitive relation between
uppercase letters. So each fact is a pair of uppercase letters such as AB
meaning that A is related to B. A letter may or may not be related to itself,
but transitivity holds: if A is related to B and B is related to C then we
can infer that A is related to C.
Create a class FactCount that contains a method minFacts that is given a
String[] known and that returns the size of the smallest set of facts that
will allow us to infer everything (and only those things) that can be inferred from the facts
contained in known.
Each element of known will contain 1 or more facts separated by a single space. The smallest set of facts may contain facts that can be inferred from
known but that are not contained in it.
| | Definition | | Class: | FactCount | Method: | minFacts | Parameters: | String[] | Returns: | int | Method signature: | int minFacts(String[] known) | (be sure your method is public) |
| | | | Constraints | - | known will contain between 1 and 50 elements, inclusive. | - | Each element of known will contain between 2 and 50 characters, inclusive. | - | Each element of known will consist of pairs of uppercase letters ('A'-'Z'), separated by a space (' '). | | Examples | 0) | | | | Returns: 2 |
AA and AB capture all the content of the 4 facts in known.
|
|
| 1) | | | | Returns: 4 |
AB, CA, BC, and AD allow us to infer both AA (AB, BC, CA gives AA by transitivity)
and AC (AB, BC gives AC by transitivity), and there is no smaller subset that
allows us to infer all the known facts. |
|
| 2) | | | | Returns: 3 |
The set {AC,BA,CB} allows us to infer exactly the same facts. Note that AC is
not a fact contained in known, but these 3 facts allow us to infer exactly the
same things that we can infer from the 4 facts contained in known.
|
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | There is an integer K. You are allowed to add to K any of its divisors not equal to 1 and K. The same operation can be applied to the resulting number and so on. Notice that starting from the number 4, we can reach any composite number by applying several such operations. For example, the number 24 can be reached starting from 4 using 5 operations: 4->6->8->12->18->24.
You will solve a more general problem. Given ints N and M, return the minimal number of the described operations necessary to transform N into M. Return -1 if M can't be obtained from N.
| | Definition | | Class: | DivisorInc | Method: | countOperations | Parameters: | int, int | Returns: | int | Method signature: | int countOperations(int N, int M) | (be sure your method is public) |
| | | | Constraints | - | N will be between 4 and 100000, inclusive. | - | M will be between N and 100000, inclusive. | | Examples | 0) | | | | Returns: 5 | The example from the problem statement. |
|
| 1) | | | | Returns: 14 | The shortest order of operations is:
4->6->8->12->18->27->36->54->81->108->162->243->324->432->576. |
|
| 2) | | | | Returns: 10 | The shortest order of operations is:
8748->13122->19683->26244->39366->59049->78732->83106->83448->83460->83462. |
|
| 3) | | | | 4) | | | | Returns: -1 | The number 99991 can't be obtained because it is prime. |
|
| 5) | | | | Returns: 0 | We don't need any operations. N and M are already equal. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Consider the following code, which traverses a tree and prints a sequence of characters:
bypass(Node v) {
for i=0..size(v.children)-1 {
write('1');
bypass(v.children[i]);
write('0');
}
}
Starting from the root this code will generate a string of 0's and 1's that fully describes a tree.
For example, "1101100110011000" will represent the tree in the following picture (nodes are marked in the order of traversal).
A tree is called symmetrical if, after reversing the order of children for all nodes, the string representation of the tree is not changed. For example, the tree in the picture is not symmetrical because the string representation changes to "1110011001100100" after the order of children is reversed for all nodes.
You will be given a String tree. You are to make it symmetrical by removing some nodes and changing the order of children for some nodes. You can remove a node only if all its children are removed too. You should return tree after making it symmetrical. If there are multiple ways to make tree symmetrical, you should minimize the number of removed nodes. If two or more solutions still remain, return the one that comes first lexicographically.
| | Definition | | Class: | SymmetricalTree | Method: | makeSymmetrical | Parameters: | String | Returns: | String | Method signature: | String makeSymmetrical(String tree) | (be sure your method is public) |
| | | | Constraints | - | tree will contain between 1 and 40 characters, inclusive. | - | tree will only contain the digits '0' and '1'. | - | tree will represent a valid tree. | | Examples | 0) | | | | Returns: "11011001100100" | This is the example from the problem statement. We should remove the 9th node (see picture). |
|
| 1) | | | | Returns: "10110010" | We can make the tree symmetrical by using only rearrangements. |
|
| 2) | | | | 3) | | | | Returns: "11011010100100" | |
| 4) | | | "11010010110110010101100010110100" |
| Returns: "10110100110110010110010011010010" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | In this problem, you will write a program that controls the actions of a progress indicator. The indicator is a single bar character with one of 4 states: '|', '-', '\', and '/'. The program consists of a sequence of instructions, where each instruction is one of 7 possible actions:
- 'L': Spin left. If the bar is in state '|', it goes to '\'. State '\' goes to '-', '-' goes to '/', and '/' goes to '|'.
- 'R': Spin right. This is the exact opposite of 'L': '\' goes to '|', '|' goes to '/', '/' goes to '-', and '-' goes to '\'.
- 'F': Flip. The bar is rotated 90 degrees: '\' becomes '/', '/' becomes '\', '-' becomes '|', and '|' becomes '-'.
- 'U': The bar moves up.
- 'D': The bar moves down.
- '<': The bar moves left.
- '>': The bar moves right.
Each instruction requires one second to complete. The computer's screen refresh is broken, so whenever the bar moves to a new location, its state at the previous location remains visible and the new location is overwritten with the bar's current state (see examples for clarification). To take advantage of this unexpected "feature", you've decided to come up with a game.
Initially, the bar is at (0, 0), which is the upper-left corner of the screen, and it is in state startState. The rest of the screen is empty. You are given a String[] with a desiredScreen that is your target. You must construct a program that will end with the screen looking exactly like desiredScreen.
For example, if your desiredScreen is
{"///",
"///",
"---"}
and your startState is '-', you can achieve that with "L>>D<<DR>>". In this case, it took 10 seconds to get the screen to desiredScreen.
For the given desiredScreen and startState, return the minimum time needed to achieve this goal, or -1 if it is impossible to do so. | | Definition | | Class: | IndicatorMotionDrawing | Method: | getMinSteps | Parameters: | String[], char | Returns: | int | Method signature: | int getMinSteps(String[] desiredState, char startState) | (be sure your method is public) |
| | | | Notes | - | The bar cannot be moved outside the boundary of desiredScreen. | - | Note that desiredScreen may contain spaces. The bar can never pass through a cell which has a space in desiredScreen because it would leave a character that would never be erased. | - | In the examples the character '\' appears as '\\' because of the C++/Java syntax for escaping characters. | | Constraints | - | startState will be one of '/', '\', '|', or '-'. | - | desiredScreen will have between 1 and 3 elements, inclusive. | - | Each element of desiredScreen will contain exactly N characters, where N is an integer between 1 and 4, inclusive. | - | Each character of each element of desiredScreen will be one of ' ', '/', '\', '|', or '-'. | | Examples | 0) | | | | Returns: 10 | The example from the problem statement. |
|
| 1) | | | | Returns: -1 | Since the bar is initially at (0,0), it's impossible to achieve a desiredScreen that has a space at that location. |
|
| 2) | | | | Returns: 9 | Don't step on spaces!
Here, you are forced to step twice at least in (0,0). One way to achieve the best time is "R><LDD>R>".
This will lead to this sequence of screens, with dots representing spaces and and the location of the bar written below each screen as (row,column):
/.. -.. --. --. /-. /-. /-. /-. /-. /-.
... -> ... -> ... -> ... -> ... -> /.. -> /.. -> /.. -> /.. -> /..
... ... ... ... ... ... /.. //. /-. /--
(0,0) (0,0) (0,1) (0,0) (0,0) (1,0) (2,0) (2,1) (2,1) (2,2)
|
|
| 3) | | | {"/-|/",
"/ |/",
"-/\\/"} | '\\' |
| Returns: 18 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You have m workers numbered 1 through m, and you have n tasks that must be completed. You know which tasks can be performed by each of the workers. If worker i completes t different tasks, he must be paid cost[i]*t2. You would like to minimize the total cost of completing all the tasks.
You will be given a String[] can describing the capabilities of your workers. The i-th element describes worker i and contains n characters. The j-th character is 'Y' if the worker can perform the j-th task, and 'N' if he can't. You will also be given a int[] cost. The i-th element of cost is the cost of worker i (as used in the formula above). All indices are 1-based. Return the minimal total cost required to complete all the tasks, or -1 if all the tasks cannot be completed.
| | Definition | | Class: | JobPlanner | Method: | minimalCost | Parameters: | String[], int[] | Returns: | int | Method signature: | int minimalCost(String[] can, int[] cost) | (be sure your method is public) |
| | | | Constraints | - | can will contain between 1 and 50 elements, inclusive. | - | All elements of can will contain the same number of characters. | - | Each element of can will contain between 1 and 50 characters, inclusive. | - | Each element of can will contain only 'Y' and 'N' characters. | - | cost will contain the same number of elements as can. | - | Each element of cost will be between 1 and 500,000, inclusive. | | Examples | 0) | | | | Returns: 3 | In this case each worker can perform any task. It is better to give one task to the first worker, and another task to the second worker. The total cost is 1 * 12 + 2 * 12 = 3. |
|
| 1) | | | | Returns: 4 | In this case it is better to give both tasks to the first worker since the second one is too expensive. The total cost is 1 * 22 + 5 * 02 = 4. |
|
| 2) | | | | Returns: 6 | In this case the first worker cannot perform the second task, so we need to use the second worker. The total cost is 1 * 12 + 5 * 12 = 6. |
|
| 3) | | | | Returns: -1 | In this case the second task cannot be completed at all. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You have n distinct integers between 1 and n, inclusive. A permutation of these integers is called an (m,k)-ladder permutation if its longest increasing subsequence has length m and its longest decreasing subsequence has length k. A subsequence is a sequence created by removing zero or more elements from an original sequence. The relative order of the remaining elements must be preserved. For example, {1, 3} is a subsequence of {1, 2, 3}, but {3, 2} is not. An increasing sequence is one in which each element is greater than the previous element, and a decreasing sequence is one in which each element is less than the previous element.
You are given ints n, m and k. Return a int[] containing the (m,k)-ladder permutation of size n. If there are multiple possibilities, return the one that comes first lexicographically. If there is no such permutation, return an empty int[]. Sequence A comes before sequence B lexicographically if A contains a lower value at the first position where they differ.
| | Definition | | Class: | LadderPermutation | Method: | createLadder | Parameters: | int, int, int | Returns: | int[] | Method signature: | int[] createLadder(int n, int m, int k) | (be sure your method is public) |
| | | | Constraints | - | n will be between 1 and 50, inclusive. | - | m will be between 1 and n, inclusive. | - | k will be between 1 and n, inclusive. | | Examples | 0) | | | | Returns: {2, 1, 4, 3 } | In this case, all longest increasing subsequences have length 2 (for example, {1, 3}), and all longest decreasing subsequences have length 2 (for example, {2, 1}).
|
|
| 1) | | | | 2) | | | | Returns: { } | In this case, the two numbers will always form an increasing or decreasing sequence of length 2. There is no permutation where the longest increasing/decreasing subsequence only has length 1.
|
|
| 3) | | | | Returns: {2, 1, 4, 3, 6, 5 } | |
| 4) | | | | Returns: {3, 2, 1, 6, 5, 4 } | |
| 5) | | | | Returns: {1, 2, 3, 7, 6, 5, 4 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | The first thing you have to do is to concatenate all elements of actions into one long string, and that will be your input string.
In this problem, you will write a program that controls the actions of a progress indicator. The indicator is a single bar character in the middle of the screen with one of 4 states: '|', '/', '-', and '\'. From now on, we will refer to '\' as 'N', for programming convenience. The program is a sequence of instructions in the form:
<instr> <secs>
where <instr> represents one of 4 possible actions, and <secs> is the action's duration in seconds. The action is performed once each second. The 4 possible actions are:
- 'L': Spin left. If the bar is in state '|', it goes to 'N'. State 'N' goes to '-', '-' goes to '/', and '/' goes to '|'.
- 'R': Spin right. This is the exact opposite of 'L': 'N' goes to '|', '|' goes to '/', '/' goes to '-', and '-' goes to 'N'.
- 'S': Stay. The bar remains in its current state.
- 'F': Flip. The bar is rotated 90 degrees: '|' becomes '-', '-' becomes '|', '/' becomes 'N', and 'N' becomes '/'.
So, the sequence "F03L02" and the starting state of '-' leads to the following sequence: "-|-|N-".
Given a String[] actions representing a sequence of actions, return the shortest program that leads to that sequence. The first character of the sequence is the starting state, so your program should run for K-1 seconds where K is the length of the given sequence. If there are multiple shortest programs that produce the given sequence, return the lexicographically first among them. If the return string has more than 100 characters, return only the first 97 followed by "..." (see last example for clarification). | | Definition | | Class: | IndicatorMotionReverse | Method: | getMinProgram | Parameters: | String[] | Returns: | String | Method signature: | String getMinProgram(String[] actions) | (be sure your method is public) |
| | | | Notes | - | You can't make an action last more than 99 seconds with a single instruction, but you can use the same instruction multiple times consecutively (see example 2 for further clarification). | - | If two programs have the same size, the one that comes earlier lexicographically is the one with the lower ASCII value in the first position at which they differ. | | Constraints | - | actions will have between 1 and 50 elements, inclusive. | - | Each element in actions will have between 1 and 50 characters, inclusive. | - | Each character of each element of actions will be one of '|', 'N', '-' or '/'. | | Examples | 0) | | | | Returns: "F03R02L02R01S05R01L01" | The actions needed after the first '-', which is the starting state, are:
-|-|/-/|//////-/
.FFFRRLLRSSSSSRL
which can be optimally represented using the syntax above. |
|
| 1) | | | | Returns: "" | Sometimes you need an empty program. |
|
| 2) | | | {"||||||||||||||||||||||||||||||||||||||||||||||||||",
"||||||||||||||||||||||||||||||||||||||||||||||||||",
"||||||||||||||||||||||||||||||||||||||||||||||||||"} |
| Returns: "S50S99" | Here you need 149 stays and it is necessary to break them into 2 'S' instructions. The lexicographically first way to do this is S50S99. |
|
| 3) | | | {"N",
"-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N",
"-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N",
"-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N",
"-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N",
"-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N",
"-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N",
"-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N",
"-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N-N"} |
| Returns:
"L01R01L01R01L01R01L01R01L01R01L01R01L01R01L01R01L01R01L01R01L01R01L01R01L01R01L01R01L01R01L01R01L..." | In this case the output program has 400 instructions, which is 3x400=1200 characters. Remember to return only the first 97 followed by "...". |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are given a network where each pair of nodes is connected by at most 1 path. Your boss has told you to place traffic monitors on certain nodes to better supervise the network. A single monitor can watch all links directly connected to the node it is attached to. Assuming every link must be watched, return the fewest number of monitors that must be installed.
Nodes i and j share a symmetric link in your network if character j of element i of links is 'Y' ('N' otherwise). A path is a sequence of distinct nodes such that neighbors in the sequence share a link. | | Definition | | Class: | TrafficMonitor | Method: | getMin | Parameters: | String[] | Returns: | int | Method signature: | int getMin(String[] links) | (be sure your method is public) |
| | | | Constraints | - | links will contain between 1 and 50 elements, inclusive. | - | Each element of links will contain exactly N characters, where N is the number of elements in links. | - | Each character of links will be 'Y' or 'N'. | - | Character i of element i of links will be 'N'. | - | Character i of element j of links will equal character j of element i. | - | There will be at most one path between any two nodes. | | Examples | 0) | | | | Returns: 0 | There are no edges to monitor. |
|
| 1) | | | {
"NYNN",
"YNYN",
"NYNY",
"NNYN"
} |
| Returns: 2 | |
| 2) | | | {
"NNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNYNNNNNNN",
"NNNNNNNNNNNNNYNNYNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNN",
"NNNNNNNNNNNNNNYNNNNNYNNNNNNNYNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNN",
"YNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNN",
"NYNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNYNNNNN",
"NNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNYNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNYNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNYNNYNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNYNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNYN",
"NNNYNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNYNNN",
"NNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNN",
"NNNNNNNNYYNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNYNNNNNNNNNNNNNNNNNNNNNNYNNNNYNNNNNYNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNYN",
"NNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNY",
"NNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNYNN",
"NNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNYNNNN",
"NNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNYNNN",
"NNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNYYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNYNNNNNN",
"NNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNYNNNNNNNNYYNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNY",
"NNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNYNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNN",
"NNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNYNNNNNNNNNN",
"NNNNNNNNNNNNNNNNYNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNYNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNYNNNNNYNNNNNNNNNNNNNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNNNNNNNNYNNNNNNNNNNNNNNNYNNNNNNNNNN"
} |
| Returns: 22 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Matrices are a common object in mathematics. A NxM matrix is basically a table with N rows of M values each. Given two matrices, one of size AxB and another of size CxD, the following multiplication rules apply:
- You can only multiply them if B is equal to C.
- The resultant matrix is of size AxD.
The number of elements in an AxB matrix is A multiplied by B. For example, a 3x7 matrix has 21 elements.
Given a list of matrices, determine if there's an ordering that allows you to multiply all of them. If multiple such orderings exist, choose the one where the result has the most elements. Return the number of elements in the result, or -1 if there is no valid ordering (see examples 0-3 for further clarification). The list of matrices is given as two int[]s, N and M, where the ith elements of N and M represent the number of rows and columns respectively of the ith matrix. | | Definition | | Class: | OrderDoesMatter | Method: | getOrder | Parameters: | int[], int[] | Returns: | int | Method signature: | int getOrder(int[] N, int[] M) | (be sure your method is public) |
| | | | Notes | - | The association order is not important because we are only interested in the dimensions of the matrices. | | Constraints | - | M will have between 1 and 50 elements, inclusive. | - | N and M will have the same number of elements. | - | Each element of N and M will be between 1 and 1000, inclusive. | | Examples | 0) | | | | Returns: 49 | Here we can legally multiply all the matrices in three different ways:
(3x3)*(3x7)*(7x3) = (3x3) (elements = 9)
(3x7)*(7x3)*(3x3) = (3x3) (elements = 9)
(7x3)*(3x3)*(3x7) = (7x7) (elements = 49)
The maximum number of elements is then 49. |
|
| 1) | | | | Returns: 3 | There's only one legal way to multiply the matrices (3x5)*(5x5)*(5x1)=(3x1) so the answer is 3*1=3. |
|
| 2) | | | | Returns: -1 | There is no legal way to multiply the matrices. |
|
| 3) | | | | Returns: -1 | Again, no legal way to multiply them all. |
|
| 4) | | | {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1} | {1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1} |
| Returns: 1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
We want to build a new phone network between numPoints points. A number of possible cables is available to construct the network. Each of the cables connects two of the points and has an associated quality and cost. We want to select a number of these cables such that:
1) All the points are connected to each other, either directly or via other points.
and
2) The quality/cost ratio (i.e., the sum of the qualities divided by the sum of the costs) is as high as possible.
What is the best achievable ratio? If it is impossible to connect all the points, return -1.
The available cables are described by a String[] cables. Each element of cables consists of four integers separated by single spaces. The first two integers describe the two points connected by the cable. The third integer describes the quality of the cable and the fourth integer describes its cost.
| | Definition | | Class: | PhoneNetwork | Method: | bestQuality | Parameters: | int, String[] | Returns: | double | Method signature: | double bestQuality(int numPoints, String[] cables) | (be sure your method is public) |
| | | | Notes | - | Your return must have relative or absolute error less than 1e-9. | | Constraints | - | numPoints is between 2 and 50, inclusive. | - | cables has between 0 and 50 elements, inclusive. | - | Each element of cables has length between 0 and 50, inclusive. | - | Each element of cables consists of four integers with no leading zeroes, separated by single spaces. | - | The first two integers of each element of cables are between 1 and numPoints, inclusive. | - | The first two integers of each element of cables are not equal. | - | The third and fourth integers of each element of cables are between 1 and 100,000, inclusive. | | Examples | 0) | | | 4 | {"1 2 6 5","2 3 3 4","3 4 5 2","4 1 3 3"} |
| Returns: 1.4 | By taking cables 1, 2 and 4, we obtain a quality/cost ratio of (6+5+3)/(5+2+3) = 1.4. |
|
| 1) | | | 5 | {"1 2 6 5","2 3 3 4","3 4 5 2","4 1 3 3"} |
| Returns: -1.0 | It's impossible to connect point 5 to the others. |
|
| 2) | | | 4 | {"1 2 1 10","2 3 3 3","2 4 3 2","3 4 3 1","3 4 2 1"} |
| Returns: 0.7058823529411765 | The cable needed to connect point 1 to the rest is so bad that it pays off to use all the other cables, including the two different cables connecting points 3 and 4.
|
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | My whole family lives in Manhattan, and consists of n people numbered 0 through (n - 1). The roads in Manhattan are a bit peculiar: they all go from North to South or from West to East, and together they form a complete grid of roads. Therefore, the distance between two intersections (x,y) and (x',y') equals abs(x-x')+abs(y-y'). Knowing the intersections where my family members live, I'd like to find the two different persons with the furthest distance between them.
You are to help me by returning a int[] containing the 0-based indices of the people who form the furthest pair. If there are several furthest pairs, return the one among them with the smallest first index. If there are still multiple pairs left, return the one among them with the smallest second index.
The intersection where the ith family member lives is defined by the following sequence:
xi = (a * yi-1 + b) % m, for i > 0.
yi = (a * xi + b) % m, for i >= 0.
with x0=0.
| | Definition | | Class: | Manhattan | Method: | furthestPair | Parameters: | int, int, int, int | Returns: | int[] | Method signature: | int[] furthestPair(int n, int a, int b, int m) | (be sure your method is public) |
| | | | Constraints | - | n will be between 2 and 250,000, inclusive. | - | a, b and m will each be between 1 and 1,000,000,000, inclusive. | | Examples | 0) | | | | Returns: {2, 6 } | The intersections where my family members live are (0,13), (12,5), (2,4), (18,1), (20,15), (3,11), (21,22), (6,9), (7,16) and (10,14). The 2nd and 6th intersections (zero-indexed) are furthest apart. The distance is abs(2-21)+abs(4-22)=37. |
|
| 1) | | | | Returns: {0, 1 } | All of them live at (0,0), so all pairs have distance 0. The lexicographically first pair is {0, 1}. Please note that we are looking for two different persons, so pair {0, 0} is invalid. |
|
| 2) | | | | Returns: {0, 54 } | Intersections 0 (0,1023) and 54 (96346,97580) are furthest apart.
|
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
A palindrome is a phrase that reads the same forward and backward (ignoring spaces). Given the first half of a palindrome (as described below), you must return a complete palindrome that contains only words from a given set of legal words. The returned palindrome must be a phrase where words are separated by single spaces.
You will be given the first half of the palindrome as a String text containing only letters and no spaces. There are two complete palindromes that can be created from this first half. For example, given "ABC", you could produce either "ABCCBA" or "ABCBA" as the complete palindrome. You must then insert spaces into the complete palindrome such that all the words in the phrase exist in the String[] words
For example, given the list of words { "A", "CANAL", "MAN", "PANAMA", "PLAN" }, and the text "AMANAPLANAC", your method would return the String "A MAN A PLAN A CANAL PANAMA".
If no palindrome can be made, your method should return "".
If more than one palindrome can be made, return the one that comes first lexicographically (please note that ' ' comes before all letters).
| | Definition | | Class: | Palindromist | Method: | palindrome | Parameters: | String, String[] | Returns: | String | Method signature: | String palindrome(String text, String[] words) | (be sure your method is public) |
| | | | Constraints | - | text will contain between 1 and 50 characters, inclusive. | - | text will contain only uppercase letters ('A'-'Z'). | - | words will contain between 1 and 50 elements, inclusive. | - | Each element of words will contain between 1 and 50 characters, inclusive. | - | Each element of words will contain only uppercase letters ('A'-'Z'). | | Examples | 0) | | | "AMANAPLANAC" | { "A", "CANAL", "MAN", "PANAMA", "PLAN" } |
| Returns: "A MAN A PLAN A CANAL PANAMA" | |
| 1) | | | "AAAAA" | { "AA", "A", "AAA" } |
| Returns: "A A A A A A A A A" | |
| 2) | | | "CBA" | { "CBABC", "CBAABC" } |
| Returns: "CBAABC" | |
| 3) | | | "RACEFAST" | { "AR", "CAR", "FAST", "RACE", "SAFE", "CEFA", "ACE", "STTS", "AFEC" } |
| Returns: "RACE FAST SAFE CAR" | |
| 4) | | | "AABAABA" | { "AA", "AAB", "BAA", "BAB" } |
| Returns: "AA BAA BAA BAA BAA" | |
| 5) | | | "STRAWNOTOOSTUPIDAF" | { "WARTS", "I", "TOO", "A", "FAD", "STUPID", "STRAW", "PUT", "NO", "ON", "SOOT" } |
| Returns: "STRAW NO TOO STUPID A FAD I PUT SOOT ON WARTS" | |
| 6) | | | | 7) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A tree is a connected graph with no cycles.
Starting from a connected tree with positive integral edge lengths, we take a subset of the nodes of that tree. Next, we compute the lengths of the shortest paths between all pairs of nodes in the subset (in the original tree). This gives us a symmetric N*N distance matrix where N is the number of nodes in the subset.
Your task is to reverse this process. Given a N*N distance matrix, you are to reconstruct a tree, if possible. Since there may be many reconstructions, you should return the minimum number of nodes in any reconstruction that agrees with the distance matrix (this will be at least N). If no reconstruction is possible, return -1.
The distance between nodes i and j (indexed from 0) can be found by treating g1[i][j] and g2[i][j] as hexadecimal digits. Putting the two digits together gives the distance (g1 has the more significant digits). | | Definition | | Class: | TreeReconstruct | Method: | reconstruct | Parameters: | String[], String[] | Returns: | int | Method signature: | int reconstruct(String[] g1, String[] g2) | (be sure your method is public) |
| | | | Constraints | - | g1 and g2 will each contain exactly N elements, where N is between 2 and 50, inclusive. | - | Each element of g1 and g2 will contain exactly N hex digits ('0'-'9' and 'A'-'F'). | - | The distance between each pair of distinct nodes will be positive. | - | The diagonal entries of g1 and g2 will be '0'. | - | g1 and g2 will each be symmetric. | | Examples | 0) | | | {"0000",
"0000",
"0000",
"0000"}
| {"0444",
"4044",
"4404",
"4440"} |
| Returns: 5 | The original tree could have been a star: one central node, with 4 nodes connected to it, each by an edge of length 2. The subset of selected nodes are the 4 non-central nodes. |
|
| 1) | | | {"0000",
"0000",
"0000",
"0000"}
| {"0233",
"2033",
"3302",
"3320"} |
| Returns: 6 | |
| 2) | | | {"00001",
"00001",
"00011",
"00100",
"11100"}
| {"066C6",
"60CA4",
"6C02C",
"CA20A",
"64CA0"}
|
| Returns: 6 | |
| 3) | | | {"00000",
"00000",
"00001",
"00000",
"00100"} | {"06839",
"60E7B",
"8E0B1",
"37B0A",
"9B1A0"} |
| Returns: 7 | |
| 4) | | | {"023825",
"202704",
"320633",
"876084",
"203805",
"543450"} | {"0198AA",
"10ED9F",
"9E0D7F",
"8DD06E",
"A97608",
"AFFE80"} |
| Returns: 9 | |
| 5) | | | {"0000",
"0000",
"0000",
"0000"} | {"0121",
"1012",
"2101",
"1210"} |
| Returns: -1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are analyzing a communications network with at most 18 nodes.
Character j in element i (both 0-based) of mat denotes whether nodes i and j can communicate ('Y' for yes, 'N' for no). Assuming a node cannot communicate with two nodes at once, return the maximum number of nodes that can communicate simultaneously. | | Definition | | Class: | SeparateConnections | Method: | howMany | Parameters: | String[] | Returns: | int | Method signature: | int howMany(String[] mat) | (be sure your method is public) |
| | | | Notes | - | If node S is communicating with node T then node T is communicating with node S. | | Constraints | - | mat will contain between 1 and 18 elements inclusive. | - | Each element of mat will contain exactly N characters, where N is the number of elements in mat. | - | Each character in mat will be 'Y' or 'N'. | - | Character i of element i of mat will be 'N'. | - | Character i of element j will be the same as character j of element i. | | Examples | 0) | | | {
"NYYYY",
"YNNNN",
"YNNNN",
"YNNNN",
"YNNNN"
} |
| Returns: 2 | All communications must occur with node 0. Since node 0 can only communicate with 1 node at a time, the returned value is 2. |
|
| 1) | | | {
"NYYYY",
"YNNNN",
"YNNNY",
"YNNNY",
"YNYYN"
} |
| Returns: 4 | In this setup, we can let node 0 communicate with node 1, and node 3 communicate with node 4. |
|
| 2) | | | {
"NNYYYYYYYYYYYYYYYY",
"NNYYYYYYYYYYYYYYYY",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN",
"YYNNNNNNNNNNNNNNNN"
} |
| Returns: 4 | |
| 3) | | | {
"NNNNNNNNNYNNNNNYN",
"NNNNNNNNNNNNNNNNN",
"NNNNNNNYNNNNNNNNN",
"NNNNNYNNNNNYNNYYY",
"NNNNNNNNNNNNNNNYN",
"NNNYNNNNNNNNNNYNN",
"NNNNNNNNNYNNNNNNN",
"NNYNNNNNNNNNNNNNN",
"NNNNNNNNNYNNNNNNN",
"YNNNNNYNYNNNNNNNY",
"NNNNNNNNNNNNNNNNN",
"NNNYNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNN",
"NNNNNNNNNNNNNNNNN",
"NNNYNYNNNNNNNNNNN",
"YNNYYNNNNNNNNNNNN",
"NNNYNNNNNYNNNNNNN"
} |
| Returns: 10 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are in a house where the light in each room is controlled by a switch that might be located in a different room. Initially, the light in the first room is on, and the lights in all the other rooms are off.
You are currently in the first room and your goal is to end up in the last room, with all the lights in the house off except the light in the last room. You can move directly from any room to any other room, and you can turn any of the switches that are located in your current room. However, you may never enter a dark room or turn off the light in your current room.
You are given a int[] switches describing the locations of the light switches. The light switch for room i is located in room switches[i]. Rooms have 0-based indices. Return the minimal number of moves required to complete your task, or -1 if it is impossible. Only moving from one room to another counts as a move (turning a switch is not counted).
| | Definition | | Class: | CrazySwitches | Method: | minimumActions | Parameters: | int[] | Returns: | int | Method signature: | int minimumActions(int[] switches) | (be sure your method is public) |
| | | | Constraints | - | switches will contain between 2 and 16 elements, inclusive. | - | Each element in switches will be between 0 and the number of elements in switches - 1, inclusive. | | Examples | 0) | | | | Returns: 1 | You can switch on the light in the last room, move into the last room and switch off the light in the first room. |
|
| 1) | | | | 2) | | | | Returns: 3 | You can switch on the light in the last room, move into the last room, switch on the light in the second room, move into the second room, switch off the light in the first room, move into the last room and switch off the light in the second room. |
|
| 3) | | | | 4) | | | {7, 11, 1, 12, 6, 3, 0, 2, 6, 0, 0, 5, 9} |
| Returns: 15 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
There are several knights located on a NxN chessboard. Chessboard rows are numbered from 1 to N, inclusive. Chessboard columns are numbered with single characters from 'A' to 'A'+N-1, inclusive. For example, a standard 8x8 chessboard has rows 1...8 and columns A...H.
A knight is a chess piece that attacks the following squares (marked with X symbols):
If one of those squares is occupied by some other knight, those two knights are attacking each other.
An arrangement of knights is called friendly if no knight attacks another knight. Your task is to determine the minimum number of knights that can be removed to make a friendly arrangement. You will be given an int N denoting the size of the chessboard and a String[] pos containing the knight positions. Each element of pos will contain a space separated list of <COL><ROW> knight positions on the board where <COL> is the column and <ROW> is the row. Each <COL> is a letter between 'A' and 'A'+N-1, inclusive, and each <ROW> is an integer between 1 and N, inclusive, with no leading zeros. There will be no leading or trailing spaces.
| | Definition | | Class: | Knights | Method: | makeFriendly | Parameters: | int, String[] | Returns: | int | Method signature: | int makeFriendly(int N, String[] pos) | (be sure your method is public) |
| | | | Constraints | - | N will be between 1 and 26, inclusive. | - | pos will contain between 1 and 50 elements, inclusive. | - | pos will be formatted as described in the statement. | - | Each element of pos will contain between 2 and 50 characters, inclusive. | - | No two knights will occupy the same position. | | Examples | 0) | | | 5 | {"A2 A4", "B1 B5", "D1 D5 E2 E4 C3"} |
| Returns: 1 | 8 knights on the chessboard are attacked by knight C3, so it should be removed. |
|
| 1) | | | | Returns: 0 | This is a 2x2 chessboard, so no knight is under attack. |
|
| 2) | | | 6 | {"A1 A5 B3 C1 C5 D2 D4 E6 F5"} |
| Returns: 3 | One possible way is to remove B3, C5 and D4. |
|
| 3) | | | 8 | {"A2 A4 A5 A6 B2 B5 B6 B7 B8",
"C3 C8 D1 D2 D3 D4 D5 D6 D8",
"E1 E3 E8 F1 F2 F8 G3 G5 H4 H7 H8"} |
| Returns: 12 | |
| 4) | | | 9 | {"A3 A4 A5 A7 A8 B6 B8 C3 C6",
"C7 C9 D4 D5 D8 D9 E1 E3 E7",
"F2 G2 G6 G7 H2 H9 I2 I4 I5",
"I6 I7 I8 I9"} |
| Returns: 10 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Your country has several army units. There are several other countries, each of which is considered to be either an enemy or neutral, and each of which has several army units of its own.
A war consists of a series of battles. Once you initiate war with a neighboring country, war will proceed until either country has no remaining army units. After defeating another country in war, you own their territory. That is, their territory is added to yours forming one new larger territory. You can only initiate war with a country that borders some part of the territory you already own.
In any single round of battle, exactly one of the two countries involved will lose an army unit. If country A attacks country B, where A and B have a and b army units, respectively, then the probability that B will lose an army unit during the battle is a2/(a2+a*b+b2), otherwise A will lose an army unit. Note that a and b correspond to the total number of units in countries A and B, respectively.
Your goal is to defeat all of the enemy nations. While it is not required that you defeat any of the neutral nations, doing so may be necessary in order to reach other enemy countries (see examples 2 and 3).
You are given a String[] armies, describing each country. Each element of armies is formatted as "type units borders" (quotes added for clarity). type is one of 'Y', 'E' or 'N', indicating if the country is yours, an enemy, or neutral. units is an integer indicating how many army units are held by that country. borders is a space delimited list of zero or more integers, indicating the zero-based indices of adjacent countries.
You are to return a double indicating the probability that you can defeat all of your enemy nations without all of your army units being lost, assuming that you choose your order of attack optimally. | | Definition | | Class: | CountryWar | Method: | defeatAll | Parameters: | String[] | Returns: | double | Method signature: | double defeatAll(String[] armies) | (be sure your method is public) |
| | | | Notes | - | The graph described by the bordering countries does not necessarily represent a planar graph. | - | The graph described by the countries is not necessarily a connected graph (see example 5). | - | Return value must be within 1e-9 absolute or relative error of the actual result. | | Constraints | - | armies will contain between 1 and 15 elements, inclusive. | - | Each element of armies will be formatted as "type units borders" (quotes added for clarity). | - | Each type will be 'Y', 'E' or 'N'. | - | Exactly one element of armies will be of type 'Y'. | - | Each units will be an integer between 1 and 20, inclusive, with no leading zeros. | - | Each number represented in borders will be an integer with no leading zeros, between 0 and n-1, inclusive, where n is the number of elements in armies. | - | Within a single element of armies, the numbers represented in borders will contain no duplicates. | - | The borders described will be symmetric (that is, if a borders b, then b borders a). | | Examples | 0) | | | | Returns: 0.3333333333333333 | Here, you and your enemy each have exactly one army. Using the formula, 12 / (12 + 1*1 + 12) = 1/3. |
|
| 1) | | | | Returns: 0.7142857142857142 | Here, superior strength is an advantage. In the first round of battle, there is a 4/7 chance of our enemy losing his only army. In the 3/7 chance that we get to a second round of battle, there is a 1/3 chance of winning. Thus, our total chances of winning are 4/7 + 3/7 * 1/3 = 5/7. |
|
| 2) | | | {"Y 1 1",
"E 1 0 2",
"N 1 1"} |
| Returns: 0.3333333333333333 | Our first battle is against our enemy, which we have a 1/3 chance of winning. Since we are not required to defeat neutral countries, we do not need to continue after that. |
|
| 3) | | | {"Y 1 1",
"N 1 0 2",
"E 1 1"} |
| Returns: 0.1111111111111111 | Here, we again have three countries lined up in a row, but this time, we are forced to first attack the neutral country, so that we can get to the enemy. We have a 1/3 chance of winning each war, thus a 1/9 chance of successfully completing both. |
|
| 4) | | | {"Y 2 1 2",
"E 2 0 2",
"E 1 0 1"} |
| Returns: 0.16250944822373392 | There are two enemy nations to attack, so our task is to determine which order of attack is optimal. |
|
| 5) | | | | Returns: 0.0 | There is no path connecting you with your enemy, therefore you cannot possibly attack (or defeat) them. |
|
| 6) | | | | Returns: 1.0 | There is only you, and nobody to defeat, so you're guaranteed to be successful. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | When scheduling tasks to be executed by a person, it is sometimes the case that when one task is completed, a later task suddenly becomes more important. In these situations, it is said that the later task is a 'Supertask' of the completed one.
In addition, when a task reaches a certain level of importance, it must be executed immediately. Each task is initially given a priority of 0. Whenever a task is completed, the priority of its Supertask increases by 1. If at some moment one of the tasks has a priority of 2, and has not been previously executed, it must be executed immediately. If no remaining task has a priority of 2, a single unexecuted task of your choice must be executed (you can not wait and must execute one of them).
You have been given N tasks (referenced by numbers 0 to N-1) that must be executed. All of these tasks (with the exception of the last) have a single Supertask, and you are guaranteed that the reference number of a task will always be lower than the reference number of its Supertask. The last task has no supertask, instead the number -1 is used. You also wish to execute the last task (with reference number N-1) as late as possible. Every task takes one minute to complete execution. Given the supertasks for each task, return the latest time (in minutes) that the last task can be started, if you start executing tasks at time 0.
You will be given the supertasks as a int[] supertasks, where element i of supertasks contains the reference number of the supertask for task i. | | Definition | | Class: | PendingTasks | Method: | latestProcess | Parameters: | int[] | Returns: | int | Method signature: | int latestProcess(int[] supertasks) | (be sure your method is public) |
| | | | Notes | - | If a task is executed after its Supertask, then its execution has no bearing on the priority of the Supertask. | - | Tasks can only be executed once | | Constraints | - | supertasks will contain between 1 and 50 elements, inclusive | - | Element i of supertasks will be between i + 1 and (number of elements in supertasks - 1), inclusive, except the last element of supertasks, which will be -1. | | Examples | 0) | | | | Returns: 2 | The final task is a supertask of all other tasks. Whenever you finish any two of first four tasks, the priority of the last will have risen from 0 to 2, so it must execute immediately. This happens after two tasks, so the latest it can start is after two minutes. |
|
| 1) | | | | Returns: 4 | You can do the tasks in order, putting the final task last, starting it at the fourth minute. |
|
| 2) | | | | Returns: 0 | The final task is also the only task, so it must be executed immediately. |
|
| 3) | | | | Returns: 7 | We have the following tree:
8
/ \
6 7
/ | \ / | \
0 1 2 3 4 5
We can not complete all of tasks 0 to 7 before task 8 because:
a) one of tasks 0, 1, 2 must be completed after task 6.
b) one of tasks 3, 4, 5 must be completed after task 7.
c) task 8 must be started as soon as both 6 and 7 are finished - so one of 0 to 5 will not be completed at that moment.
One of the possible orderings which starts the final task at the 7th minute is the following: 0, 1, 6, 2, 3, 4, 7, 8. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | We can think of a computer network as a undirected graph where nodes
represent routers and edges represent connections between the routers.
In this network, there are a number of packets, each of which has a
source and a target. Over a number of discrete time steps, each
packet must travel from its source to its target. During one time
step, a packet at router u may move to router v, if
there is a link between u and v. However, only one
packet may use a link between u and v during a
particular time step (if one packet uses a link to go from u
to v, no other packet can use that link to go from v
to u during the same time step). The problem is to figure
out the best way to route the packets through the network so that they
get to their destinations as soon as possible.
You will be given an int, 2<=N<=100,
representing the number of nodes in the graph. A
String[], g will specify the edges (up to 4950
of them), where each element is of the form "u v",
where u and v are distinct integers between 0 and
N-1, inclusive, indicating an edge between node u and node
v. There will be at most one edge between a pair of nodes. A
String[], packets, will specify the packets to be
sent in the network, where
10<=|packets|<=2,000. Each element
will be formatted as "s t", indicating a packet
with source s and target t, where s does not equal t. These graphs will be
randomly generated as described at the end of the problem
statement. Furthermore, the graphs will be connected.
Your task is to come up with a routing plan for the packets through the
network. You should return a String[], each element of
which represents the locations of the packets after each time step. Each
element of the String[] should be a space delimited list
of the packets' locations at a time step corresponding to the index of
the element (the first element of the return corresponds to the
locations of the packets after the first time step). The list should
give the locations in the same order that the packets are given to
you.
For example, consider the simple network with 2 nodes and 2
packets:
N=2
g={"0 1"}
packets={"0 1","1 0"}
In this case, there are two packets trying to go in opposite
directions along the one link. One possible return would be:
{"0 0","1 0"}
This indicates that in the first time step, the second packet moves
along the link from node 1 to node 0. Since that link is in use, the
first packet cannot also use it, so during the second time step, the
first packet moves from node 0 to node 1, and at this point all
packets have reached their destinations. Another valid return would
be {"0 1","1 1","1 0"}. In this return, none of the packets moved
during the first time step. While valid, this is clearly
suboptimal.
Your program will be scored based on the quality of your method's return, and
the time it takes your method to execute. If your method's return is
invalid in any way or if not all the packets reach their destinations,
you will get a 0. Otherwise, your score for a test case will be given
by:
quality2 - 10 * execution time (quality is defined below, time is in seconds)
If the quality or the above formula turns out to be negative, the score is set to 0. Your overall score will be the sum over all test cases
of SCORE/OPT, where OPT is the highest score anyone has achieved on a
test case (0/0 = 1 here). Thus, if you score 10 on one test case, and someone else
scores 20 on the same test case, you will get 10/20 = 0.5 towards your
final score for that test case.
The quality term used above will be the percent improvement
of your solution over a naive solution that routes packets over a
random shortest path. In the naive solution, if a packet is being routed
to node v, and is currently at node u, then a node
is chosen at random out of all nodes that are on some shortest path
from u to v and are also connected to u. The packet will eventually be sent
from u to that node, with conflicts along the link resolved
by letting a random packet use the link.
Finally, there are dozens of realistic and interesting classes of
graphs that could be randomly generated and used here. One class that has drawn a
great deal of interest in recent years is ad-hoc networks. To simulate these
graphs, we will first select a random value for N, the number
of nodes. Each node will then be assigned a random x and y value in a
cartesian plane, at a distance of no more than 50 from the origin.
Then, we will select random values for the lower and upper bounds on
each node's range (5<=lower<upper<30). Each node will be
assigned a range uniformly between these bounds. Two nodes will be
able to communicate if their distance from each other is less than both their ranges. If
the network generated is not connected, it will be scrapped and a new attempt will be made.
Otherwise, a random number of packets will be generated, the sources
and sinks of which are random. Here is pseudocode to generate a graph. The notation [x,y] indicates a random value between x and y, inclusive (the value is floating point unless an integer is clearly required). Roughly 30% of generated graphs are connected.
N = [2,100]
lower = [5,30]
upper = [5,30]
if(upper < lower)swap(lower,upper)
for(i = 0 to N-1){
do{
x[i] = [-50,50]
y[i] = [-50,50]
}while(x[i]*x[i]+y[i]*y[i] > 50*50);
r[i] = [lower,upper]
}
for(i = 0 to N - 1){
for(j = 0 to i-1){
if(dist(i,j) < range[i] && dist(i,j) < range[j]){
connected(i,j) = connected(j,i) = true
}
}
}
M = [10,2000]
for(i = 0 to M-1){
do{
packets(i,0) = [0,N-1]
packets(i,1) = [0,N-1]
}while(packets(i,0) == packets(i,1));
}
| | Definition | | Class: | RoutePackets | Method: | route | Parameters: | int, String[], String[] | Returns: | String[] | Method signature: | String[] route(int N, String[] graph, String[] packets) | (be sure your method is public) |
| | | | Notes | - | The time limit is 20 seconds. | - | The memory limit is 1 gigabyte. | - | The thread limit is 32. | - | There may be multiple packets corresponding to a single pair of nodes. | - | To reduce variance, the naive algorithm explained above is run 5 times, and the median number of steps is used for comparison. | | Examples | 0) | | | | Returns:
"The graph of 9 nodes in adjacency matrix format:<pre>000100100
001001010
010110000
101010100
001100001
010000010
100100000
010001000
000010000
</pre>The packets to be sent:<pre>8 5
6 7
4 1
0 6
7 4
7 1
3 4
4 7
5 1
7 1
0 7
5 0
0 8
8 0
1 8
7 8
3 7
7 6
0 4
6 1
</pre>" | The naive solution for this test cases takes 13 steps. Hence, if your solution took only 12 steps, it would receive a quality score of (100*(13-12)/13)2 = 59.2 |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are in a chat room that supports nickname tab-completion. Suppose
the input buffer now contains the string s and you decide to use the
completion facility. The first time you press tab you will be shown
the lexicographically first element of names that has s as a
prefix. Pressing tab again will give the lexicographically second,
and so forth. Once the possible options are exhausted the tab key
will do nothing. Having found a completion that suits you, you may
either press enter to complete the word you are typing, or continue
typing characters into the input buffer. If you decide to type
characters, they will be appended to the current completion. Your goal
is to type the word w, followed by the enter key, using as few
keystrokes as possible. Each character and each tab key count as single
keystrokes. By interchanging character typing and tab completion
sequences as many times as you like, return the fewest number of
keystrokes required.
| | Definition | | Class: | CheapestTabComplete | Method: | getFewest | Parameters: | String[], String | Returns: | int | Method signature: | int getFewest(String[] names, String w) | (be sure your method is public) |
| | | | Notes | - | If the buffer is empty, then every element of names is a possible completion for the buffer. | - | The only allowed keystrokes are letters, tabs, and enter. | | Constraints | - | names will contain between 0 and 50 elements, inclusive. | - | Each element of names will contain between 1 and 50 lowercase letters ('a'-'z'), inclusive. | - | w will contain between 1 and 50 lowercase letters ('a'-'z'), inclusive. | | Examples | 0) | | | | Returns: 7 | Since tab is useless, we type in the 6 letters of myname and then press enter. |
|
| 1) | | | | Returns: 3 | Here we press tab twice, and then enter. |
|
| 2) | | | {"abc","ab","abcd","frankies","frank","a","a"} | "frankie" |
| Returns: 5 | Here we type 'f', then tab, then 'i', 'e', and finally enter. |
|
| 3) | | | {"a","a","f","f","fr","fr","fra","fra","fran","fran","frank","frank"} | "frankie" |
| Returns: 8 | Due to the weird set of names, tab is of no use. |
|
| 4) | | | {"a","a","bcde","bcde","bcde","bcdefghij"} | "bcdefghijk" |
| Returns: 6 | |
| 5) | | | {"aaaa","aaaa","aaaa","aaaa","aaaa"} | "aaaaaaaaaaaaaaaaaaaaaaa" |
| Returns: 21 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Each character in maze will be '.' or 'X' denoting a free
square or an obstacle, respectively. Starting on some free square, and moving
exclusively through free squares, your goal is to leave the confines
of the maze through its left edge. Each move you make is either 'U', 'D', 'L', or
'R' denoting up, down, left, and right respectively. Moving upward
decreases which element of maze you are in, while moving
leftward decreases which character you are at in a particular
element. Nothing happens (your position does not change) if you move into an
obstacle, or try to leave the maze other than leftward. Similarly, nothing happens if you issue a move
and you have already left the maze (you already won).
Your friend has decided to challenge you by trying to solve the
maze with the monitor off. This means you do not know which
position you have started in or if you have won.
Return the shortest sequence of moves that will solve
the maze regardless of your initial position. If there are multiple shortest solutions, return the one that occurs first lexicographically.
If there is no solution, return an empty string. | | Definition | | Class: | BlindMazeSolve | Method: | getSolution | Parameters: | String[] | Returns: | String | Method signature: | String getSolution(String[] maze) | (be sure your method is public) |
| | | | Constraints | - | maze contains between 1 and 5 elements, inclusive. | - | Each element of maze will contain between 1 and 4 characters, inclusive. | - | Each element of maze will contain the same number of characters. | - | Each character in maze will be '.' or 'X'. | - | maze will contain at least one '.'. | | Examples | 0) | | | | Returns: "L" | Left will win immediately, so we return "L". |
|
| 1) | | | | Returns: "LL" | Regardless of your initial position, moving left twice will result in winning. |
|
| 2) | | | | 3) | | | {
"X...",
"XXX.",
"X...",
"X.XX",
"..XX"
} |
| Returns: "RRDDLLDDLL" | |
| 4) | | | | Returns: "" | If you happen to be in the rightmost position, you cannot win. |
|
| 5) | | | {
"XXX.",
"..X.",
"X...",
"XX..",
"X..."
} |
| Returns: "DDDRULULULL" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
There are a number of strange particles flying in space and interacting with each other. When two particles collide, three outcomes are possible:
- 1.) Nothing happens
- 2.) The first particle disappears
- 3.) The second particle disappears
You will be given a String[] interacts, where the jth character of the ith element indicates what happens when the ith and jth particles collide. It will be '+' if the ith particle disappears, '-' if the jth particle disappears, and '0' (zero) if nothing happens.
The particles will randomly collide and interact with each other for some period of time. You don't know the order or the number of interactions that will occur. After all the interactions are over, there will be a number of particles that have not disappeared. Return the lowest possible value for this number.
| | Definition | | Class: | StrangeParticles | Method: | remain | Parameters: | String[] | Returns: | int | Method signature: | int remain(String[] interacts) | (be sure your method is public) |
| | | | Constraints | - | interacts will contain between 1 and 50 elements, inclusive. | - | Each element of interacts will contain the same number of characters as there are elements in interacts. | - | Each character in interacts will be '+', '-' or '0'. | - | Character i in element i of interacts will be '0'. | - | Character i in element j of interacts will be opposite to character j in element i ('-' is opposite to '+' and '+' is opposite to '-', '0' is opposite to itself) | | Examples | 0) | | | | Returns: 1 | Three particles form a cycle. Only one particle can remain. |
|
| 1) | | | | Returns: 3 | No particle can disappear at all. |
|
| 2) | | | {"0++++++++++++++",
"-0+++++++++++++",
"--0++++++++++++",
"---0+++++++++++",
"----0++++++++++",
"-----0+++++++++",
"------0++++++++",
"-------0+++++++",
"--------0++++++",
"---------0+++++",
"----------0++++",
"-----------0+++",
"------------0++",
"-------------0+",
"--------------0"} |
| Returns: 1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
The inhabitants of a certain magical country are known to be clever wizards. Their King decided to test how clever they really were.
He took 3 hats (2 white and 1 black) and put 2 smart wizards around a table. He put a white hat on one of the wizards and a black hat on the other. Each wizard could see what hat was on his neighbor, but could not see what hat was on his own head. The wizards knew that there were 2 white hats and 1 black hat. The King asked: "Do you know what hat is on your head?", and the wizard with the white hat answered: "Yes". It's clear how he knew: he had seen the black hat on his neighbor, and knowing that there was only 1 black hat, concluded that his own hat was white.
The King then decided to complicate their task a bit, and he put white hats on both the wizards. He asked: "Do you know what hat is on your head?", and both wizards answered together: "No". He asked again: "Do you know what hat is on your head?", and both wizards then answered: "Yes, we know. We have white hats on our heads!". How did they find out? When the question was first asked, the first wizard had seen the white hat on his neighbor, but didn't know the color of his own hat, so he answered "No". After hearing his neighbor's answer to the same question, he realized that his neighbor was in the same situation. Therefore, both wizards knew that they were wearing white hats when the question was asked a second time.
Let's consider a more general scenario. You are given an int wizards representing the number of wizards participating in the test, and a int[] hats representing all the hats possessed by the King. Each element of hats represents a different color, and the ith element of hats is the number of hats of color i. You are also given a int[] hatsOnWizards, the ith element of which represents the number of hats of color i that are placed on wizards' heads (each wizard will receive exactly one hat). The wizards all know what hats are initially possessed by the King. Each wizard can see the hats worn by all the other wizards, but cannot see the hat on his own head. Each time the King asks his question, the wizards all answer simultaneously, and each wizard can hear the answers given by all the other wizards. Return the number of times the King must ask his question before at least one wizard will know the color of his own hat. The King will always ask the question at least once. If no wizard will ever know the color of his own hat, return -1.
| | Definition | | Class: | Wizards | Method: | questions | Parameters: | int, int[], int[] | Returns: | int | Method signature: | int questions(int wizards, int[] hats, int[] hatsOnWizards) | (be sure your method is public) |
| | | | Constraints | - | wizards will be between 1 and 75, inclusive. | - | hats will contain between 2 and 5 elements, inclusive. | - | Each element of hats will be between 1 and 15, inclusive. | - | hatsOnWizards and hats will contain the same number of elements. | - | Each element of hatsOnWizards will be between 0 and the corresponding element in hats, inclusive. | - | All elements of hatsOnWizards will sum up to wizards. | | Examples | 0) | | | | Returns: 1 | The first example from the problem statement. |
|
| 1) | | | | Returns: 2 | The second example from the problem statement. |
|
| 2) | | | | Returns: -1 | No wizard will ever know the color of his own hat. |
|
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | We are keeping a family tree. We update the data by adding information as it
becomes available. When information arrives that is not
consistent with the previous data, we need to recognize that fact immediately.
We will enforce only the most obvious rules:-
A person is either male or female.
-
A child's parent cannot be the child itself or a descendant of the child
-
A child has two parents, one male and the other female.
(A person is a descendant only of his parents, grandparents, greatgrandparents,
etc.)
Each piece of data gives either the names of a child and parent or the name of
a person and that person's gender. All occurrences of a name represent the same person.
Create a class FamilyTree that contains a method firstBad that is given a
String[] data. The method returns the (0-based) index of the first element of data that is inconsistent
with the previous elements of data, or returns -1 if all the data is consistent.
Each element of data will be formatted in one of these two forms:
"childname parentname"
"name gender"
where the two parts are separated by a single space character, each name is all
uppercase letters 'A'-'Z' and gender is a single lowercase letter, either 'm' or 'f'.
| | Definition | | Class: | FamilyTree | Method: | firstBad | Parameters: | String[] | Returns: | int | Method signature: | int firstBad(String[] data) | (be sure your method is public) |
| | | | Constraints | - | data will contain between 1 and 50 elements, inclusive. | - | Each element of data will be formatted as above. | - | Each element of data will contain between 3 and 50 characters, inclusive. | | Examples | 0) | | | {"BOB JOHN","BOB JOHN","BOB MARY","BOB m","AL f"} |
| Returns: -1 |
Repeated data elements just give us more confidence. This data is all
consistent. BOB's 2 parents are JOHN and MARY (it is not
yet known which is the father and which is the mother), and BOB is male. We
also know that AL is female.
|
|
| 1) | | | {"BOB JOHN","BOB MARY","MARY JOHN","JOHN f","MARY f","AL f"} |
| Returns: 4 |
The first 4 elements are considered consistent. They describe that BOB's 2
parents are JOHN and MARY and that (unconventionally) MARY is JOHN's child.
JOHN is female. But "MARY f" is inconsistent with this since that makes
both of BOB's parents female. |
|
| 2) | | | {"BOB JOHN", "CARLA BOB", "JOHN CARLA"} |
| Returns: 2 |
After the first 2 elements we know that CARLA is a descendant of JOHN, so
"JOHN CARLA" cannot be added. |
|
| 3) | | | {"BOB RICK", "AL RICK", "AL PAULA", "PAULA LINUS", "LINUS BOB","BOB PAULA"} |
| Returns: 5 |
The first 5 elements are consistent. BOB's descendant AL was a child of
RICK and PAULA, and RICK is BOB's parent. His other parent could not be
PAULA since PAULA is his descendant so the final element is the first one
that is inconsistent.
|
|
| 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | My company has given me a list containing the name
of each employee along with the name of his supervisor (who is also an employee) if
he has one.
The company wants to know whether the list is consistent and, if so, how many
of the employees are supervisors (supervise at least one employee).
"Consistent" means that there is no supervision cycle in which A supervises himself or A supervises B who
supervises C ... who supervises A. It is permissible to have multiple employees who
have no supervisor.
But ... we have Bob trouble. All the employees have distinct names, except that there
may be multiple distinct employees whose names are "BOB". So there may be multiple
ways to put together the supervision hierarchy. We want to construct the
hierarchy so as to minimize the number of supervisors.
Create a class BobTrouble that contains a method minSupers that is given a String[]
name and a String[] bossName giving the names of all the employees and their bosses.
It returns the minimum number of supervisors that can appear in the supervision
hierarchy. If no supervision hierarchy is consistent, it returns -1.
Each element of name refers to a distinct employee, and the supervisor of the
i-th element is given by the i-th element of bossName ("*" indicates
that the employee has no supervisor). Every employee is listed in name.
| | Definition | | Class: | BobTrouble | Method: | minSupers | Parameters: | String[], String[] | Returns: | int | Method signature: | int minSupers(String[] name, String[] bossName) | (be sure your method is public) |
| | | | Constraints | - | name contains between 1 and 50 elements, inclusive. | - | Each element of name contains between 1 and 10 uppercase letters ('A'-'Z'), inclusive. | - | The elements of name are distinct, except that "BOB" may appear more than once. | - | bossName contains the same number of elements as name. | - | Each element of bossName is "*" or matches at least one element of name. | | Examples | 0) | | | {"BOB","BOB","BOB"} | {"BOB","*","BOB"} |
| Returns: 1 |
There are 3 possible supervisory hierarchies: 1) the middle BOB supervises
the first BOB who supervises the last BOB, 2) the middle BOB supervises the
last BOB who supervises the first BOB, and 3) the middle BOB supervises the
first BOB and also supervises the last BOB. This last choice gives the
fewest supervisors.
|
|
| 1) | | | {"JOHN","AL","DON","BOB"} | {"*","*","*","*"} |
| Returns: 0 |
All the employees are unsupervised, so there are no supervisors. |
|
| 2) | | | {"BOB","BOB","BOB"} | {"*","*","BOB"} |
| Returns: 1 |
There are 2 possible hierarchies (the third BOB can be supervised by either
of the other BOBs). Either way, exactly one of the BOBs is a supervisor. |
|
| 3) | | | {"BOB", "BOB", "JACK"} | {"BOB", "BOB", "*"} |
| Returns: -1 | The first BOB must be supervised by the second BOB (it is illegal to supervise yourself) and the second BOB
must be supervised by the first BOB. But this is a supervision cycle,
so there is no legal hierarchy satisfying this data.
|
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | As part of a larger scale project, you need to write a component which generates consecutive positive integers. Only certain digits may appear in the input and in the integers generated, and leading zeros aren't allowed.
You are given a int[] allowed containing the list of allowed digits, and a String current representing the current integer. Return a String representing the first integer larger than current composed only of digits in allowed.
If current represents an invalid integer according to the first paragraph, return "INVALID INPUT" (quotes for clarity). | | Definition | | Class: | IntegerGenerator | Method: | nextInteger | Parameters: | int[], String | Returns: | String | Method signature: | String nextInteger(int[] allowed, String current) | (be sure your method is public) |
| | | | Constraints | - | allowed will contain between 0 and 10 elements, inclusive. | - | Each element in allowed will be between 0 and 9, inclusive. | - | allowed will contain no duplicates. | - | current will contain between 1 and 10 digits ('0'-'9'), inclusive. | | Examples | 0) | | | { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 } | "16" |
| Returns: "17" | With all digits available, the next number is 17.
|
|
| 1) | | | { 0, 1, 2, 3, 4, 5, 6, 8, 9 } | "16" |
| Returns: "18" | The digit 7 is no longer allowed, so the next smallest valid integer is 18. |
|
| 2) | | | | Returns: "INVALID INPUT" | The current number may not contain disallowed digits. |
|
| 3) | | | | Returns: "INVALID INPUT" | Leading zeros aren't allowed either. |
|
| 4) | | | { 9, 8, 7, 6, 5, 4, 3, 2, 1, 0 } | "999" |
| Returns: "1000" | |
| 5) | | | | Returns: "INVALID INPUT" | The generator only works with positive integers. |
|
| 6) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | It's the year 2051. You have some free time in June this year and want to visit an interesting galaxy. You will need some machines during your trip and you are wondering how many of them you can take.
There are a number of machines in a store. Each machine may depend on other machines to work properly, and you want all the machines you take to work. You noticed that although machine A may not depend directly on machine B, you may be unable to take A without B because of some indirect dependency. For example, if machine A is dependent on machine C and machine C is dependent on B, you cannot take machine A without machine B.
You discovered an interesting rule while selecting your machines. If a machine A depends on machines B and C, then B is dependent on C, C is dependent on B, or both B and C depend on each other. Although the rule you found is true for direct dependencies, you were able to prove that it also holds for indirect dependencies.
You want to buy K different machines such that all of them will work properly. You are given a String[] dependencies describing the dependencies between the machines. Element i of dependencies is a space delimited list of machines that machine i is directly dependent on. Return a int[] with all possible positive values for K in ascending order.
Example: {"1 2","0 2","3",""}
- Machine 0 needs machines 1 and 2 to work
- Machine 1 needs machines 0 and 2 to work
- Machine 2 needs machine 3 to work
- Machine 3 does not need any other machine to work
The allowed K values are 1 (you take only machine 3), 2 (you take machines 2 and 3) and 4 (you take all the machines). So you should return {1,2,4}. | | Definition | | Class: | GalaxyExpedition | Method: | possibleValues | Parameters: | String[] | Returns: | int[] | Method signature: | int[] possibleValues(String[] dependencies) | (be sure your method is public) |
| | | | Notes | - | First machine has index 0, second has index 1 and so on. | | Constraints | - | dependencies contains between 1 and 50 elements, inclusive. | - | Each element of dependencies contains between 0 and 50 characters, inclusive. | - | Each element of dependencies is a space delimited list of integers. | - | Each integer in dependencies is between 0 and the number of elements in dependencies - 1, inclusive, and contains no extra leading zeros. | - | Each element of dependencies contains no duplicate values. | - | Element i of dependencies does not contain the value i. | - | If a machine A depends on machines B and C, then B is dependent on C, C is dependent on B, or both B and C depend on each other. | | Examples | 0) | | | | Returns: {1, 2, 4 } | The example from the problem statement. |
|
| 1) | | | | Returns: {3 } | We have to take all three machines. |
|
| 2) | | | | Returns: {2, 4 } | We can take a pair {0,1} or {2,3}, and as always we can take all the machines. |
|
| 3) | | | {"","2 0","0 1","0 4","3 0"} |
| Returns: {1, 3, 5 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | It's the year 2059. You have some free time in May this year and want to visit an interesting galaxy. You will need some machines during your trip and you are wondering how many of them can you take.
There are a number of machines in a store. Each machine may need some other machines to work properly, and you want all the machines you take to work. Luckily for you, in the year 2053, a special rule was introduced to make customers' lives easier. The rule says that if a machine A depends on another machine B, then machine B also depends on machine A.
You want to buy K different machines such that all of them will work properly. You are given a String[] dependencies describing the dependencies between the machines. Element i of dependencies is a space delimited list of machines that machine i is dependent on. Return a int[] with all possible positive values for K in ascending order.
Example: {"1 2", "0", "0", ""}
- Machine 0 needs machines 1 and 2 to work
- Machine 1 needs machine 0 to work
- Machine 2 needs machine 0 to work
- Machine 3 does not need any other machine to work
The allowed K values are 1 (you take only machine 3), 3 (you take machines 0,1,2) and 4 (you take all the machines). So you should return {1,3,4}.
| | Definition | | Class: | GalaxyTrip | Method: | possibleValues | Parameters: | String[] | Returns: | int[] | Method signature: | int[] possibleValues(String[] dependencies) | (be sure your method is public) |
| | | | Notes | - | First machine has index 0, second has index 1 and so on. | | Constraints | - | dependencies contains between 1 and 30 elements, inclusive. | - | Each element of dependencies contains between 0 and 50 characters, inclusive. | - | Each element of dependencies is a space delimited list of integers. | - | Each integer in dependencies is between 0 and the number of elements in dependencies - 1, inclusive, and contains no extra leading zeros. | - | Each element of dependencies contains no duplicate values. | - | If element i of dependencies contains the value j, then element j of dependencies contains the value i. | - | Element i of dependencies does not contain the value i. | | Examples | 0) | | | | Returns: {1, 3, 4 } | The example from the problem statement. |
|
| 1) | | | | Returns: {3 } | We have to take all three machines. |
|
| 2) | | | | Returns: {1, 2, 3, 4 } | We can take any number of these machines since there are no dependencies. |
|
| 3) | | | {"4 2", "3", "0 4", "1", "0 2", "6", "5"} |
| Returns: {2, 3, 4, 5, 7 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | We have a dictionary of words dict, and we are writing words down in a single line using the following rules. The first written word can be any word from dict. Each subsequent written word must start with the same letter as the last letter of the previous written word, and that shared letter is only written once (see examples). Only words from dict can be used, but each word can be used an unlimited number of times.
Using the rules above, construct the lexicographically earliest line with length greater than or equal to len characters. Return an empty String ("") if such a line cannot be constructed. | | Definition | | Class: | StickedWords | Method: | constructLine | Parameters: | String[], int | Returns: | String | Method signature: | String constructLine(String[] dict, int len) | (be sure your method is public) |
| | | | Constraints | - | dict will contain between 1 and 50 elements, inclusive. | - | Each element of dict will contain between 2 and 50 characters, inclusive. | - | Each element of dict will contain only lowercase letters ('a'-'z'). | - | len will be between 1 and 2500, inclusive. | | Examples | 0) | | | {"salad", "sandwich", "hamburger", "rings"} | 35 |
| Returns: "hamburgeringsandwichamburgeringsalad" | The first word in the line is "hamburger". The next word must start with the letter 'r', so we write "rings". Notice that we only write the shared 'r' once, so at this point, the line is "hamburgerings". The rest of the words, in order, are: "sandwich", "hamburger", "rings", and "salad". The resulting line is 36 characters long, and is the lexicographically earliest possible line containing at least 35 characters. |
|
| 1) | | | {"salad", "hamburger", "rings"} | 35 |
| Returns: "" | |
| 2) | | | | 3) | | | {"aarb", "bcb", "bbd", "dzz"} | 15 |
| Returns: "aarbcbcbcbcbbdzz" | |
| 4) | | | {"abd", "dgga", "abdg", "gga", "gg", "gaader"} | 22 |
| Returns: "abdggabdggabdggabdgaader" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are given a sequence of digits. You must insert either a '+' (addition) operator or a '*' (multiplication) operator between each pair of adjacent digits in such a way that minimizes the value of the resulting expression. The expression should be evaluated using the standard order of operations (multiplication has a higher precedence than addition).
You will be given a String sequence. Perform the procedure described above on the sequence and return the resulting expression. If there are several possible answers, return the one that comes first lexicographically. Note that '*' comes before '+' lexicographically.
| | Definition | | Class: | OperationsArrangement | Method: | arrange | Parameters: | String | Returns: | String | Method signature: | String arrange(String sequence) | (be sure your method is public) |
| | | | Constraints | - | sequence will contain between 2 and 50 characters, inclusive. | - | Each character in sequence will be a digit ('0'-'9'). | | Examples | 0) | | | | Returns: "2*2+2" | The minimal result can be obtained in three ways: "2*2+2", "2+2*2" and "2+2+2". The first one comes first lexicographically. |
|
| 1) | | | | 2) | | | | 3) | | | | Returns: "3+9*1*1*1+8+5+7*1" | |
| 4) | | | | Returns: "1*1*1*2*2*1+9*1*1+2*1*2" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | We are given a int[] sequence containing a sequence of numbers. In a single operation, we can either increment or decrement the value of a single element by 1. Determine the minimum number of operations we must perform before the sequence contains at least K distinct elements. | | Definition | | Class: | Distincter | Method: | disperse | Parameters: | int[], int | Returns: | int | Method signature: | int disperse(int[] sequence, int K) | (be sure your method is public) |
| | | | Notes | - | Note that we can create negative elements during the process. | | Constraints | - | sequence will contain between 1 and 50 elements, inclusive. | - | Each element in sequence will be between 1 and 1000, inclusive. | - | K will be between 1 and the number of elements in sequence, inclusive. | | Examples | 0) | | | | Returns: 0 | The sequence already has two distinct elements. |
|
| 1) | | | | Returns: 6 | Some elements can become negative. |
|
| 2) | | | | 3) | | | | 4) | | | {1, 2, 3, 4, 4, 5, 7, 7, 8} | 9 |
| Returns: 4 | The optimal way to make 9 distinct elements is to increase one of the 4s two times and increase one of the 7s two times. |
|
| 5) | | | {576, 571, 571, 572, 575, 572, 571, 568, 573, 572, 569, 572} | 11 |
| Returns: 12 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are developing a database platform that supports, among other things, user defined functions. User-defined functions may call internal functions, other user-defined functions, or both. A user-defined function, FuncA, is said to be dependent on another function, FuncB, if it calls FuncB.
You have been tasked with writing a module that will "script" (list out the code for) all of the functions in a given database. However, because of dependency issues, they must be scripted in a specific order that meets the following criteria:
- A function cannot be scripted until all of its dependent functions have already been scripted.
- Whenever more than one function could be scripted (subject to the first requirement), the one whose name occurs first lexicographically will be scripted first.
You are given a String[] funcs, each element of which is the name of a function in the database. You are also given a String[] depends, a list describing the dependency of each function. Each element of depends is a space-delimited list of integers. Each element of depends corresponds to the element of funcs with the same index. Each number represented in each element of depends refers to the 0-based index of another function.
For instance, suppose a database has two functions, A and B, each of which is dependent on a third function, C. The input might then be given by:
{"B", "C", "A"}
{"1", "", "1"}
Now, since functions A and B both depend upon C, we have to script C first. After
that, either A or B could be scripted, since C has already been scripted. We use the second
rule to determine that we should script A first. Our return is thus:
{"C", "A", "B"}
You are to return a String[] containing the names of the functions in the order in which they should be scripted. | | Definition | | Class: | FunctionDependency | Method: | scriptingOrder | Parameters: | String[], String[] | Returns: | String[] | Method signature: | String[] scriptingOrder(String[] funcs, String[] depends) | (be sure your method is public) |
| | | | Constraints | - | funcs will contain between 1 and 50 elements, inclusive. | - | depends will contain the same number of elements as funcs. | - | Each element of funcs will contain between 1 and 50 upper case ('A'-'Z') character, inclusive. | - | Each element of depends will be a space-delimited list of integers, with no leading zeroes. | - | Each integer represented in depends will be between 0 and n-1, inclusive, where n is the number of elements in funcs. | - | There will be no circular dependencies. | | Examples | 0) | | | {"B", "C", "A"} | {"1", "", "1"} |
| Returns: {"C", "A", "B" } | The example from the problem statement. |
|
| 1) | | | {"B", "C", "A"} | {"1", "", "0"} |
| Returns: {"C", "B", "A" } | |
| 2) | | | {"K", "A", "B", "C", "D", "E", "F", "G", "H", "I"} | {"", "", "1 1", "2", "3", "4", "5", "6", "7", "8"} |
| Returns: {"A", "B", "C", "D", "E", "F", "G", "H", "I", "K" } | Careful! It is permissible for the same dependency to be listed twice. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are to write a procedure for a computer game that will move groups of units.
You are given a String[] start containing the starting positions of all the units. Each element is formatted as "X Y V", where (X, Y) are integer coordinates, and V is an integer velocity in meters per second. You are also given a String[] finish containing the ending positions for all the units. Each element is formatted as "X Y", where (X, Y) are integer coordinates, and each element is distinct from the other elements. The ith element of start represents a single unit which must be moved from the coordinates in start[i] to the coordinates in some element of finish at the velocity given in start[i]. Each unit must be moved to a different location (that means, each two different units from start must be moved to different ending positions). Return a double representing the minimal time (in seconds) in which all the units will reach their destinations. | | Definition | | Class: | UnitsMoving | Method: | bestTime | Parameters: | String[], String[] | Returns: | double | Method signature: | double bestTime(String[] start, String[] finish) | (be sure your method is public) |
| | | | Notes | - | The returned value must be accurate to 1e-9 relative or absolute. | | Constraints | - | start will contain between 1 and 50 elements, inclusive. | - | finish will contain the same number of elements as start. | - | Each element of start will be formatted as "X Y V", where X, Y and V are integers without leading zeroes. | - | Each element of finish will be formatted as "X Y", where X and Y are integers without leading zeroes. | - | All X and Y will be between 0 and 1000, inclusive. | - | All V will be between 1 and 10, inclusive. | - | All elements of finish will be different. | | Examples | 0) | | | {"0 0 1", "0 1 1"} | {"1 1", "1 0"} |
| Returns: 1.0 | The first unit moves to the second location for 1 second, and the second unit moves to the first location also for 1 second. |
|
| 1) | | | {"0 0 1", "0 1 1"} | {"1 1", "2 1"} |
| Returns: 2.0 | In this case, it is better to move the fist unit to the first location and the second unit - to the second one. |
|
| 2) | | | {"0 0 1", "5 0 1"} | {"5 12", "10 12"} |
| Returns: 13.0 | |
| 3) | | | {"0 0 2", "5 0 1"} | {"5 12", "10 12"} |
| Returns: 12.0 | |
| 4) | | | {"308 994 10", "157 22 9", "282 975 5", "993 17 8", "925 771 2", "843 110 6",
"860 629 8", "947 143 6", "921 348 7", "520 607 6", "735 306 3",
"253 861 7", "562 56 9", "243 168 2", "521 971 1", "745 537 7"} | {"431 911", "109 951", "177 721", "295 831", "937 256", "608 180", "863 994", "148 406",
"275 531", "635 297", "681 404", "909 151", "569 730", "332 391", "94 97", "376 142"} |
| Returns: 115.72920979597156 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A new road has been opened. It is L meters long, and it is divided
into one-meter sections called positions. There are traffic lights at various
positions on the road. If there's a traffic light at position i, it means that a
car at position i must wait until the light is green before
it can proceed to position i+1. Traffic lights are either red or green,
and they change every five seconds.
There are some cars waiting to enter into the road. Each car is one
meter long, and there can never be more than one car at a single position of the road.
Once a car enters the road it cannot overtake other cars. The cars' speeds are given
in the int[] speeds, where the i-th element is the speed of the i-th car.
Each traffic light starts out red, but the duration of that first red light varies between
one and five seconds, inclusive. Then, the five second cycle starts. The initial red light
duration may be different for each traffic light.
Suppose that the road goes from left to right. The cars move as follows:
- Step 1: Each car already in the road (from right to left) tries to move rightward as many positions as its speed indicates, but it can be blocked by another car or a red traffic light (here rightward means increasing its position).
Then, if position 0 of the road is free, the car with the lowest index in speeds that has not yet entered the road enters at position 0, without moving.
This step takes one second.
- Step 2: The traffic lights are updated, if necessary. This step takes no time.
- Step 3: If all the cars have left the road, stop, otherwise go to Step 1.
You are given L, the road length, speeds, the speed of each car, and lights, the position of each traffic light.
Determine the duration of the first red light for each traffic light that
minimizes the total time it takes for all the cars to leave the road (that is, the number of seconds elapsed until the last car leaves the road). Return a int[], where the i-th element is
the duration of the first red light of the i-th traffic light. If two solutions give the same time, return the one that
comes first lexicographically. | | Definition | | Class: | GreenWave | Method: | getFirstRed | Parameters: | int, int[], int[] | Returns: | int[] | Method signature: | int[] getFirstRed(int L, int[] speeds, int[] lights) | (be sure your method is public) |
| | | | Notes | - | When a car leaves the road (by moving past the rightmost position on the road), it can no longer block the other cars. | - | Solution A comes earlier than B lexicographically if A has a lower value than B in the first position at which they differ. | | Constraints | - | L will be between 1 and 500, inclusive. | - | speeds will contain between 0 and 10 elements, inclusive. | - | Each element of speeds will be between 1 and 30, inclusive. | - | lights will contain between 0 and 5 elements, inclusive. | - | Each element of lights will be between 0 and L-2, inclusive. | - | All the elements of lights will be distinct. | | Examples | 0) | | | | Returns: {1 } | Second 1: There are initially no cars in the road. Since position 0 is unoccupied, the car is able to enter the road.
Second 2: The traffic light has just turned green. The car can move right to position 1.
Second 10: The car moves from position 8 to position 9 (the last position on the road).
Second 11: The car leaves the road.
|
|
| 1) | | | | Returns: {1, 2, 3, 4, 5 } | |
| 2) | | | | Returns: {4 } | When the duration of the first red light is 4 seconds, neither car will ever be blocked by a red light. |
|
| 3) | | | | Returns: {3 } | Although the second car has to wait for the traffic light for one second, it is fast enough to catch up with the first one. |
|
| 4) | | | | Returns: {1 } | Remember that once a car leaves the road, it no longer blocks the other cars. |
|
| 5) | | | 340 | {16, 15, 29, 7, 9, 15, 2} | {125, 156, 274, 309, 211} |
| Returns: {5, 1, 1, 1, 1 } | |
| 6) | | | 494 | {11, 7, 11, 6} | {438, 251} |
| Returns: {3, 1 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Note: examples in this problem statement contain images which may not display properly if viewed outside the applet.
John lives in a large city and commutes to work every day. Afraid of getting hit by cars, he tends to plot his routes so that he crosses as few roads as possible, leaving home earlier if necessary. John crosses a road whenever his path intersects the road. He'll never walk along the road (on it), since that would be dangerous.
When modeled in a plane, the city is width units wide and length units long. John may never leave the city at any time. More precisely, his x-coordinate must be between 0 and width (inclusive) and his y-coordinate between 0 and length (inclusive) at all times.
You will be given the description of roads (horizontal and vertical line segments) in the city. Horizontal roads will be formatted as "y x1 x2" (quotes for clarity), vertical roads as "x y1 y2". You will also be given the coordinates of John's home and workplace, each formatted as "x y".
Return the minimum number of roads John needs to cross in order to get from home to work.
| | Definition | | Class: | SafeJourney | Method: | fewestRoadCrossings | Parameters: | int, int, String[], String[], String, String | Returns: | int | Method signature: | int fewestRoadCrossings(int width, int length, String[] horizontal, String[] vertical, String home, String work) | (be sure your method is public) |
| | | | Notes | - | Crossing an intersection of two roads (one horizontal and one vertical) diagonally counts as crossing two roads. | | Constraints | - | width and length will be between 1 and 2,000,000,000, inclusive. | - | horizontal and vertical will each contain between 0 and 50 elements, inclusive. | - | Each element of horizontal and vertical will be at most 50 characters long and will contain a comma-separated list of roads with at least one road. | - | Each road in horizontal will be formatted as "y x1 x2", where x1 is less than x2. | - | Each road in vertical will be formatted as "x y1 y2", where y1 is less than y2. | - | home and work will both be formatted as "x y". | - | All x-coordinates will be between 0 and width, inclusive, and all y-coordinates will be between 0 and length, inclusive. | - | All numbers will be integers with no leading zeros. | - | No two roads in horizontal will overlap and no two roads in vertical will overlap, although they may have common endpoints. | - | Neither home nor work will lie on a road, on either of a road's endpoints, or on the boundary of the city. | | Examples | 0) | | | 6 | 4 | { "2 0 6" } | { "2 2 4", "4 0 2" } | "1 3" | "5 1" |
| Returns: 2 | John can't get to work without crossing at least two roads.
|
|
| 1) | | | 6 | 4 | { "2 0 6" } | { "2 0 2", "4 2 4" } | "1 3" | "5 1" |
| Returns: 1 | The vertical roads have moved and John now needs to cross only one road.
|
|
| 2) | | | 4 | 5 | { "4 1 3,1 1 3" } | { "2 1 4" } | "1 2" | "3 3" |
| Returns: 0 | |
| 3) | | | 7 | 8 | { "2 0 7", "4 0 7", "5 2 4,5 5 6", "6 0 4,6 5 7", "7 1 3" }
| { "2 2 5", "3 0 6", "4 0 7", "5 0 5" } | "1 3" | "6 1" |
| Returns: 4 | |
| 4) | | | 5 | 5 | { } | { "3 0 2", "3 2 5" } | "2 4" | "4 1" |
| Returns: 1 | Roads will not overlap, but may have common endpoints. There is no way of getting from home to work without crossing at least one of the roads. |
|
| 5) | | | 100 | 100 | { "10 0 100,20 0 100,30 0 100,40 0 100,50 0 100",
"60 0 100,70 0 100,80 0 100,90 0 100" } | { "10 0 100,20 0 100,30 0 100,40 0 100,50 0 100",
"60 0 100,70 0 100,80 0 100,90 0 100" } | "15 6" | "93 95" |
| Returns: 17 | |
| 6) | | | 13 | 9 | { "7 0 5,6 0 5,4 4 8,4 9 13,3 4 13,2 4 8,3 1 3" } | { "1 1 3,3 0 3,4 2 7,5 2 7,7 5 9,8 5 9,8 0 1,9 1 3", "10 1 3" } | "2 8" | "2 1" |
| Returns: 1 | |
| 7) | | | 15 | 8 | { "1 9 14", "4 0 2", "6 2 3", "6 9 12", "7 0 2", "7 7 14" } | { "3 0 7", "4 1 8", "7 0 7", "9 1 6", "12 4 6", "14 1 6" } | "10 4" | "1 2" |
| Returns: 0 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You live in a town which is divided into sectors, numbered 0 through N-1. In addition, some sectors are connected by roads. You must pay a toll to move between sectors. The government of your town is rather greedy, and it has decided to increase the toll along one of these roads. In particular, they are going to increase the toll along a road by tollHike dollars, such that the average cost of travelling from sector 0 to sector N-1 is maximized. This average cost is determined as the average cost over all distinct valid paths from sector 0 to sector N-1. Two paths from sector 0 to sector N-1 are distinct if they either visit a different number of sectors or visit sectors in a different order. A path is valid if it does not take you through any sector more than once while travelling from sector 0 to N-1.
Create a class GreedyGovernment which contains a method maxAverageCost. You will be given a String[] tolls and an int tollHike as arguments. The j'th character in the i'th element of tolls indicates the toll to travel between sectors i and j. If the j'th character in the i'th element of tolls is an 'X', then it is not possible to travel from sector i to sector j (although you may still be able to travel from sector j to sector i). If there is no way to travel from sector 0 to sector N-1, your method should return 0. Otherwise, the method should return a double corresponding to the maximum average cost that the government can expect. | | Definition | | Class: | GreedyGovernment | Method: | maxAverageCost | Parameters: | String[], int | Returns: | double | Method signature: | double maxAverageCost(String[] tolls, int tollHike) | (be sure your method is public) |
| | | | Notes | - | Your return value must have an absolute or relative error less than 1e-9. | | Constraints | - | tolls will contain between 2 and 10 elements, inclusive. | - | Each element of tolls will contain the same number of characters as the number of elements in tolls. | - | Each element of tolls will contain only the characters '1'-'9', inclusive, or the character 'X'. | - | The i'th character of the i'th element of tolls will be 'X' for all i. | - | tollHike will be between 1 and 100, inclusive. | | Examples | 0) | | | {"X324", "XXX2", "12X5", "991X"} | 9 |
| Returns: 10.0 | Note that there are 4 ways to travel from sector 0 to sector 3:
sector 0 --> sector 3
sector 0 --> sector 1 --> sector 3
sector 0 --> sector 2 --> sector 3
sector 0 --> sector 2 --> sector 1 --> sector 3
Any other path from sector 0 to sector 3 (for example, sector 0 --> sector 2 --> sector 0 --> sector 3) visits a sector more than once, which is not allowed in your town. |
|
| 1) | | | {"X324", "5X22", "12X5", "991X"} | 57 |
| Returns: 29.2 | |
| 2) | | | | 3) | | | {"X32X", "XXXX", "XXXX", "XXXX"} | 99 |
| Returns: 0.0 | There is no way to travel from sector 0 to sector 3. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A Dyck word is a string consisting of n X's and n Y's (for some positive integer n) such that no initial segment of the string has more Y's than X's.
If we have a Dyck word we can swap any two consecutive substrings when both of them are Dyck words. It is easy to show that the result of this swap operation is a Dyck word too.
For example, in "XXYXXYYY" (quotes for clarity) we can swap the substrings "XY" and "XXYY" to get "XXXYYXYY".
If a Dyck word A can be obtained from a Dyck word B using some number of the described swap operations they are called equivalent.
You will be given a String dyckword. Return the lexicographically smallest (i.e., the one that occurs first in alphabetical order) Dyck word that is equivalent with the given dyckword. Return "" (the empty string) if the given string is not a Dyck word.
| | Definition | | Class: | DyckwordUniformer | Method: | uniform | Parameters: | String | Returns: | String | Method signature: | String uniform(String dyckword) | (be sure your method is public) |
| | | | Constraints | - | dyckword will contain between 2 and 50 characters, inclusive. | - | Each character in dyckword will be either 'X' or 'Y'. | | Examples | 0) | | | | Returns: "XXXYYXYY" | The example from the problem statement. |
|
| 1) | | | | Returns: "XXXYYYXXYYXYXY" | The result can be obtained by four swaps "XYXYXXXYYYXXYY" -> "XYXXXYYYXYXXYY" -> "XXXYYYXYXYXXYY" -> "XXXYYYXYXXYYXY" -> "XXXYYYXXYYXYXY". |
|
| 2) | | | | Returns: "XXXXYYYXYYXXXYYY" | The result can be obtained by two swaps "XXXYYYXXYXXXYYYY" -> "XXXYYYXXXXYYYXYY" -> "XXXXYYYXYYXXXYYY". |
|
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A palindrome is a string that is spelled the same forward and backward. We want to rearrange letters of the given string baseString so that it becomes a palindrome.
You will be given a String baseString. Return the palindrome that can be made from baseString. When more than one palindrome can be made, return the lexicographically earliest (i.e., the one that occurs first in alphabetical order). Return "" (the empty string) if no palindromes can be made from baseString.
| | Definition | | Class: | PalindromeMaker | Method: | make | Parameters: | String | Returns: | String | Method signature: | String make(String baseString) | (be sure your method is public) |
| | | | Constraints | - | baseString will contain between 1 and 50 characters, inclusive. | - | Each character in baseString will be an uppercase letter ('A'-'Z'). | | Examples | 0) | | | | 1) | | | | 2) | | | | 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | The organization of an array A is computed as follows: Create an array B containing the exact same elements as A, but sorted in non-descending order. Count the number of distinct values for i such that A[i] is equal to B[i]. This value is the organization of the array A. For example, the organization of { 2, 1, 1, 3 } is 2 because the second and fourth elements are not changed after sorting.
Two elements may be swapped only if the organization of the array would increase as a result of the swap.
You will be given a int[] arrayData. Return the maximal number of the swap operations that can be performed.
| | Definition | | Class: | SwapSorter | Method: | maximizeSwaps | Parameters: | int[] | Returns: | int | Method signature: | int maximizeSwaps(int[] arrayData) | (be sure your method is public) |
| | | | Constraints | - | arrayData will have between 1 and 50 elements, inclusive. | - | Each element of arrayData will be between 1 and 1000, inclusive. | | Examples | 0) | | | | Returns: 1 | The only possible swap is {2, 1, 1, 3} -> {1, 1, 2, 3} |
|
| 1) | | | | Returns: 3 | {7, 5, 3, 4} -> {3, 5, 7, 4} -> {3, 4, 7, 5} -> {3, 4, 5, 7} |
|
| 2) | | | | Returns: 2 | {2, 1, 4, 3} -> {1, 2, 4, 3} -> {1, 2, 3, 4} |
|
| 3) | | | {1, 7, 8, 12, 17, 19, 21, 23, 24, 25, 26, 27, 35} |
| Returns: 0 | The array is already sorted. |
|
| 4) | | | | 5) | | | {2, 3, 4, 1, 7, 7, 5, 5, 8, 7, 10, 10, 10, 9, 9, 9} |
| Returns: 11 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | We all know that a planar graph is one that you can draw without having any edges crossing. Perhaps less well known is that a planar graph can always be drawn with vertices on lattice points and with edges as straight lines between connected vertices.
You will be given a String[], graph, representing a planar undirected graph, where the jth character of element i of graph indicates whether vertices i and j are connected ('T' for true, 'F' for false). Your task is to find the area of the smallest rectangular box that the vertices can be placed in so that they are located on lattice points with non-overlapping straight edges between connected vertices. | | Definition | | Class: | DrawPlanar | Method: | minArea | Parameters: | String[] | Returns: | int | Method signature: | int minArea(String[] graph) | (be sure your method is public) |
| | | | Notes | - | Lattice points are ones with integer coordinates. | | Constraints | - | graph will contain between 1 and 7 elements, inclusive. | - | Each element of graph will contain the same number of characters as there are elements in graph. | - | Each character in graph will be 'T' or 'F'. | - | Character i in element i of graph will be 'F'. | - | Character i in element j of graph will be equal to character j in element i. | - | The graph will be planar. | | Examples | 0) | | | | Returns: 0 | With just one vertex, a single point suffices to draw the graph, so the area is 0. |
|
| 1) | | | | Returns: 0 | In this case, we can draw the vertices all in a line, again with area 0. |
|
| 2) | | | | Returns: 1 | Here, we can select any three corners of a 1x1 square. |
|
| 3) | | | {"FTTT",
"TFTT",
"TTFT",
"TTTF"} |
| Returns: 4 | |
| 4) | | | {"FTTTTTT",
"TFTTTFT",
"TTFFFFT",
"TTFFTFF",
"TTFTFTT",
"TFFFTFT",
"TTTFTTF"} |
| Returns: 15 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Note: this problem statement contains an image that may not display properly if viewed outside the applet.
When on a long flight, it is often helpful to be in an aisle seat (a seat adjacent to an aisle). This way you don't need to bother another passenger when you need to go to the restroom or take a walk. However, because large airliners are built to hold as many passengers as possible, only a limited number of seats can be aisle seats.
A typical arrangement of 10 seats in a single row with 2 aisles is as follows:
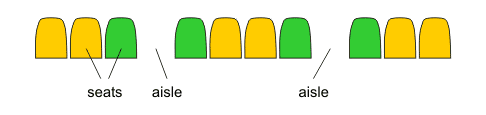
Aisle seats are colored green in the above example (there are four such seats), while center and window seats are colored orange.
All of the seats are equally wide and each aisle has the same width as a single seat. If an airplane's row is wide enough to fit width seats or aisles, and the airline wants exactly seats seats to be fitted in a row, find the arrangement which maximizes the number of aisle seats. A row should be formatted as a string of characters so that seats and aisles are represented by 'S' and '.' (dot) characters, respectively. If there are multiple arrangements which maximize the number of aisle seats, find the lexicographically smallest one (the dot character comes before 'S' in the lexicographical order).
You are to return the required arrangement (or part of it) as a String[] containing no more than 2 Strings:
- If width is 50 or less, return the entire arrangement as a single String inside the String[].
- If width is between 51 and 100 (inclusive), return the entire arrangement as two Strings, split after the first 50 characters.
- If width is more than 100, return two Strings containing the first and last 50 characters of the arrangement, respectively.
| | Definition | | Class: | AirlinerSeats | Method: | mostAisleSeats | Parameters: | int, int | Returns: | String[] | Method signature: | String[] mostAisleSeats(int width, int seats) | (be sure your method is public) |
| | | | Constraints | - | width will be between 1 and 100000, inclusive. | - | seats will be between 0 and width, inclusive. | | Examples | 0) | | | | Returns: {"..SS.S" } | All three seats can be made aisle seats and this is the lexicographically smallest such arrangement. |
|
| 1) | | | | Returns: {"S.SS.S" } | This is the only arrangement where all four seats are aisle seats. |
|
| 2) | | | | Returns: {"S.SS.SSSSSSS" } | The picture in the problem statement shows another arrangement with the maximum number of aisle seats, but this one is lexicographically smaller. |
|
| 3) | | | | Returns: {".SS.SS.SS.S" } | |
| 4) | | | | Returns: {"SSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSS", "SS" } | |
| 5) | | | | Returns:
{"..................................................",
"...............................................S.S" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
A caterpillar tree is a tree in which every node is on a central stalk or only one
graph edge away from the stalk. The figure below illustrates to the left a caterpillar tree
with 14 nodes (the stalk marked in blue), and on the right a non-caterpillar tree with 9 nodes.
Given the description of a tree, determine the least number of nodes that must be removed for
the tree to become a caterpillar tree. The tree will be described as a string of 0's and 1's.
Starting from some node in the tree, a '1' in the string traverses the tree to a previously
unvisited node, while a '0' backtracks to the previous node. The trees in the figure above
would be described as "11101011111010010001000100" and "1111100100110000", respectively,
if the traversals starts at node 1 and the nodes are visited in the numbered orders.
Create a class CaterpillarTree containing the method fewestRemovals which takes a String[]
tree containing the description of the tree (concatenate the elements to get the full description),
and returns an int containing the fewest number of nodes that must be removed for the tree
to become a caterpillar tree.
| | Definition | | Class: | CaterpillarTree | Method: | fewestRemovals | Parameters: | String[] | Returns: | int | Method signature: | int fewestRemovals(String[] tree) | (be sure your method is public) |
| | | | Constraints | - | tree will contain between 1 and 50 elements, inclusive. | - | Each element in tree will only contain the characters '0' and '1'. | - | Each element in tree will contain between 1 and 50 characters, inclusive. | - | tree will describe a valid tree. | | Examples | 0) | | | {"11101011111010010001000100"} |
| Returns: 0 | This is the leftmost picture above. Since it already is a caterpillar tree, no nodes have to be removed.
|
|
| 1) | | | | Returns: 1 | This is the rightmost picture. One of the leaf nodes to the left must be removed for it to become a caterpillar tree. |
|
| 2) | | | {"1111100000",
"1111100000",
"1111100000",
"1111100000",
"1111100000"} |
| Returns: 12 | This is a star graph, with one node in the center and five arms containing five nodes each.
If we delete four of the five nodes in three of the arms, we end up with a graph that
is a caterpillar tree.
|
|
| 3) | | | | 4) | | | {"11110100111100100111100110100110001110101001111000",
"1101100000011100110000111001101100010000"} |
| Returns: 23 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | If you look at a cross section of a tree you will notice that there are several
rings in it. The innermost ring represents the first year of the tree's growth,
and each year the tree adds one new ring. You will be given a bitmap of a
tree's cross section. Character j of element i represents the pixel at position
(j,i) and is either an 'X' or an '.'. The exact structure of the rings can be
defined recursively. The innermost ring consists of a group of connected 'X's.
The innermost ring is then surrounded by '.' characters, which are then
surrounded by 'X' characters. Each ring after is formed in the same way: all
previous rings are surrounded by '.' characters, which are then surrounded by
'X' characters. The group of '.' characters that surround previous rings are all connected in a circle
(horizontally or vertically),
as are the 'X' characters forming the new ring. The background of the image also consists of '.'
characters, and there may be no 'X's on the edges of the image. For example,
the following is a valid bitmap with 2 rings:
{".........",
".XXXXXXX.",
".X....XX.",
".X.XX.X..",
".X....XX.",
".XXXXXXX.",
"........."}
If the bitmap represents an image of rings as defined above, you are to return the number of rings in it (only the 'X's make rings). Otherwise, if the image is not valid in some way, you are to return -1.
| | Definition | | Class: | RingCount | Method: | count | Parameters: | String[] | Returns: | int | Method signature: | int count(String[] bitmap) | (be sure your method is public) |
| | | | Notes | - | Informally, a ring is connected in a circle if, for every pair of pixels in that ring, there is a path (of horizontal and vertical steps to pixels in the ring) going from one pixel to the other both in both clockwise and counterclockwise directions. | | Constraints | - | bitmap will contain between 1 and 50 elements, inclusive. | - | Each element of bitmap will contain the same number of characters. | - | Each element of bitmap will contain between 1 and 50 characters. | - | Each character in bitmap will be 'X' or '.'. | | Examples | 0) | | | {".........",
".XXXXXXX.",
".X....XX.",
".X.XX.X..",
".X....XX.",
".XXXXXXX.",
"........."} |
| Returns: 2 | |
| 1) | | | | 2) | | | {"...........",
".XXXXXXXXX.",
".X.......X.",
".X.XXXXX.X.",
".X.X...X.X.",
".X.X..XX.X.",
".X.X...X.X.",
".X.XXXXX.X.",
".X.......X.",
".XXXXXXXXX.",
"..........."}
|
| Returns: -1 | This is invalid because there is no innermost ring consisting of just 'X's. |
|
| 3) | | | {".......",
".XXXXX.",
".X...X.",
".X.X.X.",
".X...X.",
".XXXX..",
"......."} |
| Returns: -1 | The rings of 'X's and '.'s must be horizontally and vertically connected in a circle. In this example, the large ring of 'X's is connected, but not in a circle. |
|
| 4) | | | | Returns: -1 | There may be no 'X's on the edges. |
|
| 5) | | | {
".........",
".XXXXXXX.",
".X.....X.",
".X.X.X.X.",
".X.....XX",
".XXXXXXX.",
"........."} |
| Returns: -1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Travelling salesmen (and of course, travelling saleswomen, too) usually have lots of problems. And some of them are pretty hard to solve algorithmically. Let's take a look at one such problem.
In the country there are several towns. Let towns be their count. The towns are numbered from 0 to towns-1. Our travelling salesman wants to travel through this country. He starts his journey in the town origin and wants to end it in the town destination.
There are several means of transportation he may use. For each of them he knows the source and destination town and its cost. Furthermore, each time he enters a town, he will be able to sign some contracts there and make some money. This amount of money may differ from town to town, but for each town it is a fixed amount. He does sign contracts at the beginning of his journey (in the town origin). Of course, the salesman's goal is to maximize the amount of money he has at the end of his journey.
The various travel possibilities are given in a String[] travelCosts, and the profits for each town are given in a int[] profits. Each element of travelCosts is of the form "SOURCE DESTINATION COST", where SOURCE and DESTINATION are town numbers and COST is the cost of using this transportation. In profits there are exactly towns elements, and the i-th of them is the amount of money gained when entering town i.
If it is not possible to reach the destination town at all, return the String "IMPOSSIBLE".
If it is possible to reach the destination town with an arbitrarily large final profit, return the String "ENDLESS PROFIT".
Otherwise, return the String "BEST PROFIT: X", where X is the best profit he can make.
The value X must be printed with no unnecessary leading zeroes.
| | Definition | | Class: | SalesmansDilemma | Method: | bestRoute | Parameters: | int, int, int, String[], int[] | Returns: | String | Method signature: | String bestRoute(int towns, int origin, int destination, String[] travelCosts, int[] profits) | (be sure your method is public) |
| | | | Notes | - | We are interested in the amount the salesman earns during the journey, i.e., the difference between the balance of his account at the end and at the beginning of his journey. This difference may also be negative in case the salesman has to pay more than he earns during his journey. | - | The salesman may travel to the same town multiple times. He gains the profit from the town once for each visit he makes. | - | All means of transportation can only be used in the specified direction, i.e., they are one-way. The salesman may use each of them multiple times. | | Constraints | - | towns is between 1 and 50, inclusive. | - | origin and destination are between 0 and towns-1, inclusive. | - | profits has exactly towns elements. | - | Each element of profits is between 0 and 1,000,000, inclusive. | - | travelCosts has between 1 and 50 elements, inclusive. | - | Each element of travelCosts is of the form "SOURCE DESTINATION COST". | - | Each SOURCE and DESTINATION in travelCosts are integers between 0 and towns-1, inclusive, with no unnecessary leading zeroes. | - | Each COST in travelCosts is an integer between 1 and 1,000,000, inclusive, with no leading zeroes. | | Examples | 0) | | | 5 | 0 | 4 | {"0 1 10", "1 2 10", "2 3 10", "3 1 10", "2 4 10"} | {0, 10, 10, 110, 10} |
| Returns: "ENDLESS PROFIT" |
The profit in each town exactly covers the cost of travelling there. The single exception is town 3. Each time the salesman travels (from town 2) to town 3, he pays 10 for the travel and gains 110 from sales in town 3, resulting in a net gain of 100.
He is able to reach an arbitrarily large profit in the following way:
Start by travelling from 0 to 1, travel around the circle 1->2->3->1 sufficiently many times, then travel from 1 to 2 and from 2 to 4.
|
|
| 1) | | | 5 | 0 | 4 | {"0 1 13", "1 2 17", "2 4 20", "0 3 22", "1 3 4747", "2 0 10", "3 4 10"} | {0, 0, 0, 0, 0} |
| Returns: "BEST PROFIT: -32" | There is no profit here, so the salesman has to find the cheapest way of travelling. |
|
| 2) | | | 3 | 0 | 2 | {"0 1 10", "1 0 10", "2 1 10"} | {1000, 1000, 47000} |
| Returns: "IMPOSSIBLE" | The destination town is unreachable. |
|
| 3) | | | 2 | 0 | 1 | {"0 1 1000", "1 1 10"} | {11, 11} |
| Returns: "ENDLESS PROFIT" | |
| 4) | | | | Returns: "BEST PROFIT: 7" | Loops are not always useful. |
|
| 5) | | | 5 | 0 | 4 | {"0 1 13", "1 2 17", "2 4 20", "0 3 22", "1 3 4747", "2 0 10", "3 4 10"} | {8, 10, 20, 1, 100000} |
| Returns: "BEST PROFIT: 99988" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Some square tiles have been selected from a N x M chessboard,
building a pattern (not necessarily connected). You have the task of coloring
some of the selected tiles red and all of the others blue so that the pattern
composed by the blue tiles is a shifted copy of the
pattern composed by the red tiles.
One example is shown in the figure below (the white tiles in the left
image are the selected tiles, which are colored in the right image).
You will be given a String[] board containing N
elements, each of them M characters long, with a character '#'
indicating that the tile in the corresponding position belongs to the
selected tiles (i.e., to be colored, the white tiles in the example
above), a character '.' indicating a tile that shall be ignored
(the gray tiles in the example above).
Return a String[] representing the red pattern,
after
cropping the image to the smallest rectangle that contains all the red tiles.
Use the character '#' to denote tiles painted in red, '.' to denote other
tiles. If there are several ways to color the tiles so that two
equal patterns are created (one in red, one in blue), use the solution
in which the red pattern has the smallest width (horizontal
distance from the leftmost red tile to the rightmost red tile). If there
are several ways with the smallest width, use the solution among them
with the smallest height (vertical distance from the uppermost red tile
to the lowermost red tile).
If there is still a tie, use the one among the tied ones that comes first
lexicographically after you concatenate the Strings representing
the solution ('#' comes before '.').
If there is no solution, return an empty String[].
| | Definition | | Class: | AreaSplit | Method: | halfPattern | Parameters: | String[] | Returns: | String[] | Method signature: | String[] halfPattern(String[] board) | (be sure your method is public) |
| | | | Notes | - | Since the red and blue patterns are translated copies of the same pattern, the returned value would be the same if we asked for the blue pattern (since we crop the returned value to the width/height of the resulting pattern). | - | All elements of the returned String[] shall have the same length (the width of the red pattern). | | Constraints | - | board will contain between 1 and 50 elements, inclusive. | - | Each element of board will have between 1 and 50 characters, inclusive. | - | Each character of each element of board will be either '#' or '.'. | - | There will be at least one '#' character in at least one element of board. | | Examples | 0) | | | {".........",
".##......",
"..#.##...",
".#####...",
".#######.",
".#..###..",
"....#....",
"........."} |
| Returns: {"##..", ".#..", "####", "###.", "#..." } | The example from the problem statement. |
|
| 1) | | | | Returns: {"#", "#" } | Here, two different half-patterns are possible: the horizontal {"##"} (width 2, height 1) and the vertical {"#", "#"}
(width 1, height 2). We return the one with the smallest width. |
|
| 2) | | | | Returns: {".#", "##" } | Note that the pattern need not be connected. |
|
| 3) | | | {".#.##",
"####.",
"#...."} |
| Returns: {"#.##", "#..." } | Even if the pattern is connected, the solution may not be connected. |
|
| 4) | | | {".###.",
"##.#.",
"..###"} |
| Returns: { } | We can not split this pattern into two equal patterns. |
|
| 5) | | | | Returns: {"#.", ".#" } | Here, both {"#.", ".#"} and {".#", "#."} are possible solutions. We have to return the first
of these solutions (if we concatenate the two Strings
of that solution we get "#..#" which comes lexicographically
before ".##.", the concatenation of the Strings
of the second solution). |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Some dictionaries use a word pattern that consists of letters, '?' symbols which each denote exactly one letter, and '*' symbols which each denote zero or more letters.
Interestingly, some patterns represent the same set of words. For example, "*??*a" and "?*?a" (quotes for clarity only) patterns both represent all words that consist of three or more letters and end with 'a'.
You will be given a String pattern. Your method should return the shortest pattern that represents the same set of words as the given pattern. Return the lexicographically first in case of tie.
| | Definition | | Class: | PatternOptimizer | Method: | optimize | Parameters: | String | Returns: | String | Method signature: | String optimize(String pattern) | (be sure your method is public) |
| | | | Notes | - | Note that '*' comes before '?' in the lexicographical order. | | Constraints | - | pattern will contain between 1 and 50 characters, inclusive. | - | pattern will contain only letters ('a'-'z', 'A'-'Z'), '?' and '*'. | | Examples | 0) | | | | 1) | | | | 2) | | | | 3) | | | | 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | An undirected graph is defined as a set of vertices with undirected edges connecting some of the pairs of vertices.
You will be given such a graph as a String[] where character j of element i is a '1' if and only if vertices i and j are connected. Such a graph has as many vertices in it as there are elements in the String[]. Your task is to assign an integer between 1 and the total number of vertices, inclusive, to each of these vertices. You may not assign the same integer to multiple vertices. Your goal is to assign these integers such that vertices which are connected have integers which are close to each other numerically. More specifically, let max be the maximum difference between the two integers assigned to any two connected vertices. You want to assign the integers to the vertices such that max is minimized. You should return that minimum max. | | Definition | | Class: | GraphLabel | Method: | adjacentDifference | Parameters: | String[] | Returns: | int | Method signature: | int adjacentDifference(String[] graph) | (be sure your method is public) |
| | | | Constraints | - | graph will contain between 2 and 9 elements, inclusive. | - | Each element of graph will contain as many characters as graph has elements. | - | Each character in graph will be '0' or '1'. | - | Character j of element i of graph will be the same as character i of element j for all i and j. | - | Character i of element i of graph will be '0' for all i. | - | At least one character in graph will be '1'. | | Examples | 0) | | | {"010000",
"101111",
"010111",
"011010",
"011101",
"011010"} |
| Returns: 3 | One way to do this is to assign the label 1 to the first vertex (represented by element 0 of the input), 3 to the second vertex, 4 to the third, 2 to the fourth, 5 to the fifth, and 6 to the sixth. |
|
| 1) | | | {"01111001",
"10101000",
"11000101",
"10000111",
"11000111",
"00111000",
"00011000",
"10111000"} |
| Returns: 4 | The labels corresponding to the elements of the input are:
2 1 3 4 5 7 8 6 |
|
| 2) | | | {"011110101",
"100111000",
"100000111",
"110011011",
"110101001",
"010110110",
"101001010",
"001101101",
"101110010"} |
| Returns: 4 | |
| 3) | | | {"011111111",
"101111111",
"110111111",
"111011111",
"111101111",
"111110111",
"111111011",
"111111101",
"111111110"}
|
| Returns: 8 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | An undirected graph is defined as a set of vertices with undirected edges connecting some of the pairs of vertices. A clique in an undirected graph is a non-empty subset of vertices where there is a direct connection between every pair of vertices in the subset. A maximal clique is a clique that is not a proper subset of any other clique. You will be given a graph as a String[] where the jth character of the ith element is a '1' if and only if vertices i and j are connected. Your method should return the number of maximal cliques in the graph. | | Definition | | Class: | CliqueCount | Method: | countCliques | Parameters: | String[] | Returns: | int | Method signature: | int countCliques(String[] graph) | (be sure your method is public) |
| | | | Constraints | - | graph will contain between 1 and 20 elements, inclusive. | - | Each element of graph will contain as many characters as graph has elements. | - | Each character in graph will be '0' or '1'. | - | Character j of element i of graph will be the same as character i of element j for all i and j. | - | Character i of element i of graph will be '0' for all i. | | Examples | 0) | | | | Returns: 2 | If the vertices are 0, 1, and 2, corresonding to the elements of the input, then the two maximal cliques are {0,1} and {2}. |
|
| 1) | | | | Returns: 1 | All nodes are connected so there is just one big clique. |
|
| 2) | | | {"00010000000000100000",
"00110000000000000000",
"01011001000000011000",
"11101000000100010110",
"00110000001100000000",
"00000000010000000001",
"00000000000000011001",
"00100000000010000001",
"00000000000100011000",
"00000100000010000010",
"00001000000000000010",
"00011000100001000101",
"00000001010000000000",
"00000000000100000010",
"10000000000000000010",
"00110010100000000000",
"00100010100000000000",
"00010000000100000000",
"00010000011001100000",
"00000111000100000000"} |
| Returns: 28 | |
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You have won a collection of tickets on luxury cruisers. Each ticket can be
used only once, but can be used in either direction between the 2 cities printed
on the ticket. Your prize gives you free airfare to any city to start your
cruising, and free airfare back home from wherever you finish your cruising.
You love to sail and don't want to waste any of your free tickets. How many additional
tickets would you have to buy so that your cruise can use all of your tickets?
Create a class MaxTrip that contains a method minBuy that is given Strings
portA and portB and that returns the smallest number of
additional tickets that can be purchased to allow you to use all of your free tickets.
Each position in portA and portB corresponds to one free ticket, allowing you to travel either way between the cities denoted by the corresponding character
in portA and in portB.
| | Definition | | Class: | MaxTrip | Method: | minBuy | Parameters: | String, String | Returns: | int | Method signature: | int minBuy(String portA, String portB) | (be sure your method is public) |
| | | | Constraints | - | portA will contain between 1 and 50 characters, inclusive. | - | portB will contain the same number of characters as portA. | - | portA and portB will contain only uppercase letters ('A'-'Z'). | | Examples | 0) | | | | Returns: 1 |
You have 3 free tickets, one between A and C, one between A and B, and one
between X and Y. You can use all of these tickets if you purchase one
additional ticket.
One way is to buy a ticket between C and X. Now your cruise could start at B,
go from B to A using your 2nd free ticket, then from A to C using your first
free ticket, then from C to X using your purchased ticket, and finally from X
to Y using your 3rd free ticket.
|
|
| 1) | | | | Returns: 2 |
One plan is to cruise from C to A to B to Q (using a purchased ticket) to A
to X to A (using a purchased ticket) to Y.
|
|
| 2) | | | | Returns: 1 | Your 2 free tickets are circle cruises that end at the same port that they debark from. To use both of them, you will need to purchase a ticket that goes between A and B. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | It is a common mistake to sort numbers as strings. For example, a sorted sequence like {"1", "174", "23", "578", "71", "9"} is not correctly sorted if its elements are interpreted as numbers rather than strings.
You will be given a String[] sequence that is sorted in non-descending order using string comparison. Return this sequence sorted in non-descending order using numerical comparison instead.
| | Definition | | Class: | SequenceOfNumbers | Method: | rearrange | Parameters: | String[] | Returns: | String[] | Method signature: | String[] rearrange(String[] sequence) | (be sure your method is public) |
| | | | Constraints | - | sequence will contain between 2 and 50 elements inclusive. | - | Each element of sequence will contain between 1 and 9 characters inclusive. | - | Each element of sequence will consist of only digits ('0'-'9'). | - | Each element of sequence will not start with a '0' digit. | - | sequence will be ordered lexicographically. | | Examples | 0) | | | {"1","174","23","578","71","9"} |
| Returns: {"1", "9", "23", "71", "174", "578" } | |
| 1) | | | {"172","172","172","23","23"} |
| Returns: {"23", "23", "172", "172", "172" } | |
| 2) | | | {"183","2","357","38","446","46","628","734","741","838"} |
| Returns: {"2", "38", "46", "183", "357", "446", "628", "734", "741", "838" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You will be given a map of the bunker. The map will consist of bombs ('*'), empty cells ('.') and secret objects ('?') arranged on a regular grid. For security reasons, in case of danger, all secret objects in the bunker should be destroyed by the explosion of any one bomb. A bomb explosion destroys all objects in range D, where D depends on the bomb type. A bomb explosion also causes all other bombs in range D to explode. More formally, an item (a secret object or a bomb) will be affected by a bomb explosion if the distance between the centers of their cells is not greater than D.
You will be given a String[] field. Return the minimal value of D that will ensure that all secret objects will be destroyed by the explosion of any one bomb. | | Definition | | Class: | SecurityBunker | Method: | chooseBomb | Parameters: | String[] | Returns: | double | Method signature: | double chooseBomb(String[] field) | (be sure your method is public) |
| | | | Notes | - | The returned value must be accurate to 1e-9 relative or absolute. | | Constraints | - | field will contain between 1 and 50 elements, inclusive. | - | Each element of field will contain between 1 and 50 characters, inclusive. | - | Each element of field will contain the same number of characters. | - | field will contain only the characters '*', '.', and '?'. | - | field will contain between 1 and 100 '*' characters, inclusive. | - | field will contain between 1 and 100 '?' characters, inclusive. | | Examples | 0) | | | | Returns: 1.4142135623730951 | The answer is the distance from the secret object to any one of the bombs. |
|
| 1) | | | {"*****",
".?.?.",
"..?..",
".?.?.",
"*****"} |
| Returns: 3.0 | With D>=1, the explosion of any bomb will explode all other bombs in the same row. |
|
| 2) | | | {"*****",
"....*",
"....*",
".?..*",
"....*",
"*...*",
"*****"} |
| Returns: 2.23606797749979 | |
| 3) | | | | Returns: 2.0 | D=2 is enough to explode all bombs and the secret objects with them. |
|
| 4) | | | {"?*..*?....?",
"...........",
"....*......",
"...?.......",
".*....**?..",
"*......?...",
".......*...",
".......?.*.",
"..*.......*",
"?........?.",
"......?*?.."} |
| Returns: 5.0 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You were hired to manage a forthcoming tournament. Each player will play against each of all the other players. According to the rules, the matches can be played in any order, but the audience will want to know the current leader at any point in time.
You will be given a String[] matches. All elements of matches will be in the form "playerA defeats playerB" (quotes for clarity only). Your method should return the leader after this set of matches. The leader is a player who has played at least one match and has the highest ratio of winning matches to total matches played. In case of a tie, return the player whose name comes first lexicographically. The players' names are case sensitive (i.e. uppercase letters are lexicographically earlier than lowercase letters).
| | Definition | | Class: | TheTournament | Method: | findLeader | Parameters: | String[] | Returns: | String | Method signature: | String findLeader(String[] matches) | (be sure your method is public) |
| | | | Constraints | - | matches will contain between 1 and 50 elements inclusive. | - | Each element of matches will be in the form "playerA defeats playerB". | - | In each element of matches playerA will differ from playerB. | - | There will not be two elements of matches with the same players. | - | Each playerA and playerB will contain between 1 and 20 letters ('A'-'Z', 'a'-'z'), inclusive. | | Examples | 0) | | | {"Ted defeats Kate", "Kate defeats Billy", "Ted defeats Billy"} |
| Returns: "Ted" | Ted wins all his games, but Kate and Billy have at least one defeat. |
|
| 1) | | | {"Ted defeats Kate", "Kate defeats Billy", "Billy defeats Ted"} |
| Returns: "Billy" | All players have a 1/2 ratio of wins to total matches, so we should return the player whose name comes first lexicographically. |
|
| 2) | | | {"Ted defeats Kate", "Kate defeats Billy", "Ted defeats Billy", "Tommy defeats Ted"} |
| Returns: "Tommy" | Tommy's ratio is 1/1, Kate's and Billy's ratio is 1/2 and Ted's ratio is 1/3. So, Tommy's ratio is the best. |
|
| 3) | | | {"B defeats BA", "B defeats BB", "BC defeats B", "A defeats AB",
"A defeats AC", "A defeats AD", "A defeats AE", "AF defeats A",
"AG defeats A", "AB defeats AF", "AC defeats AG", "BB defeats BC"} |
| Returns: "A" | |
| 4) | | | {"defeats defeats def", "defeats defeats defe", "defe defeats ded", "defeat defeats defeats"} |
| Returns: "defeat" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A graph is called split if the vertices can be partioned into two non-empty sets A and B such that no two distinct vertices in A are adjacent, and all pairs of distinct vertices in B are adjacent. Considering all possible ways of removing 0 or more nodes from the given graph, return how many of the resulting subgraphs are split. Two subgraphs are considered distinct if the sets of removed nodes are distinct. Character i in element j of graph is '1' if nodes i and j are adjacent, and '0' if not. | | Definition | | Class: | SplitSubgraphs | Method: | howMany | Parameters: | String[] | Returns: | int | Method signature: | int howMany(String[] graph) | (be sure your method is public) |
| | | | Constraints | - | graph will contain between 1 and 20 elements, inclusive. | - | Each character in graph will be '0' or '1'. | - | Each element of graph will contain exactly N characters, where N is the number of elements in graph. | - | Character i of element i of graph will always be '0'. | - | Character i of element j of graph will equal character j of element i. | | Examples | 0) | | | | Returns: 4 | Every subgraph with at least 2 vertices is split. |
|
| 1) | | | | Returns: 0 | Note that A and B must both be non-empty. |
|
| 2) | | | {"0000",
"0000",
"0001",
"0010"
} |
| Returns: 11 | |
| 3) | | | {"0100",
"1000",
"0001",
"0010"
} |
| Returns: 10 | |
| 4) | | | {"01100",
"10110",
"11001",
"01001",
"00110"} |
| Returns: 24 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Suppose you have half of a chessboard as depicted below with 0 or more rooks placed on it. No two rooks can share a row or column.
Beginning with the lower left corner, we travel along the edge of the board. Below, the different positions we move through during this process are numbered.
At each corner, we count how many rooks are above and to the left of our position. Examples illustrating how the rooks are counted are given below.
By travelling from position to position we obtain a sequence of integers. For the above board, our sequence would be
0, 1, 0, 1, 1, 2, 1, 2, 1, 1, 0.
In this problem we reverse the process. Instead, you are given the sequence and must return the corresponding half-chessboard.
Given a sequence of 2n+1 integers, you will return a String[] with n elements. Element k (0-based), which corresponds to row k of the board, will contain precisely n-k characters. 'R' should be used to denote a rook and '.' should be used to denote an empty square. If more than one board is possible, choose the one where the rook in row 0 is furthest to the left (if such a rook exists). If multiple boards are still possible, choose the one where the rook in row 1 is furthest to the left, and so forth. If no board is possible, return an empty String[]. The sequence will be given in seqBeg and seqEnd. The elements of seqBeg all come before the elements of seqEnd. For example, if
seqBeg = { 0, 1, 0, 1, 1, 2, 1 }
seqEnd = { 2, 1, 1, 0}
then the resulting 11 element input sequence is the same as featured in the example above. | | Definition | | Class: | TriangleRooks | Method: | getBoard | Parameters: | int[], int[] | Returns: | String[] | Method signature: | String[] getBoard(int[] seqBeg, int[] seqEnd) | (be sure your method is public) |
| | | | Constraints | - | seqBeg and seqEnd will contain between 0 and 50 elements inclusive. | - | The combination of seqBeg and seqEnd will contain an odd number of elements. | - | The combination of seqBeg and seqEnd will contain at least 3 elements. | - | Each element of seqBeg and seqEnd will be between 0 and 100 inclusive. | | Examples | 0) | | | { 0, 1, 0, 1, 1, 2, 1 } | { 2, 1, 1, 0} |
| Returns: {".R...", "...R", "..R", "..", "R" } | From the problem statement. |
|
| 1) | | | | 2) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Over the years you have collected a great mass of music from various artists. These days you have very little time to listen to your whole collection. You decide to make a single compilation that contains the best hits from each artist. Your first attempt at the compilation was to place the best hits in a random order. However, this resulted in songs from the same artist being close or even next to each other. This is highly undesirable since you hate listening to the same artist over and over again.
After some further consideration you realize that there must be some kind of an ultimate order - i.e., an order where artists are 'spread out' across the compilation as much as possible. You then come up with a distance metric (D) that measures the 'spread' of artists across the compilation:
D(compilation) = Sum of all D(i), where D(i) is the distance of the song at position i.
D(i) = k-i, where k is the smallest integer greater than i, such that the songs at positions k and i are by the same artist. 0 if no such k exists.
Each element in artists will be formatted as "<artist name> <number of hits>". Your task is to make a String[] compilation of these artists such that D(compilation) is maximal. If there is more than one such compilation return the one which is lexicographically earliest. | | Definition | | Class: | MusicCompilation | Method: | makeCompilation | Parameters: | String[] | Returns: | String[] | Method signature: | String[] makeCompilation(String[] artists) | (be sure your method is public) |
| | | | Constraints | - | artists will contain between 0 and 50 elements inclusive. | - | Each element in artists will be formatted as "<artist name> <number of hits>". | - | <artist name> will contain between 1 and 10 letters ('a'-'z' and 'A'-'Z'), inclusive. | - | <artist name> is case-sensitive and may not appear more than once in artists. | - | <number of hits> will be an integer with no leading zeroes between 1 and 800 inclusive. | - | The sum of all <number of hits> will be between 0 and 800 inclusive. | | Examples | 0) | | | {"Shakira 1","Jamiroquai 3","Gorillaz 2"} |
| Returns: {"Gorillaz", "Jamiroquai", "Jamiroquai", "Shakira", "Gorillaz", "Jamiroquai" } | Songs 1, 2 and 3 have distances 4, 1 and 3 respectively. Songs 4, 5 and 6 have distances of 0 because they are not followed by songs from the same artist. Hence, the distance of the compilation is 4 + 1 + 3 = 8. Although there are other compilations with the same distance, this is the lexicographically earliest. |
|
| 1) | | | {"Radiohead 2","Spiderbait 3","LimpBizkit 4"} |
| Returns:
{"LimpBizkit",
"Radiohead",
"Spiderbait",
"LimpBizkit",
"LimpBizkit",
"Spiderbait",
"LimpBizkit",
"Radiohead",
"Spiderbait" } | |
| 2) | | | | Returns: {"Beatles", "ABBA", "Beatles" } | Call me old-fashioned, but I love these two bands! |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A group of people have participated in an online chat session. Element i (0-based) of numSeen contains the number of lines of chat seen by person i before leaving (everyone arrives at the same time). More precisely, integer j in element i of numSeen is the exact number of lines written by person j that are seen by person i before leaving. Integer i of element i will always be 0, and should be ignored. You will return a int[] containing the order in which each person left. If multiple orders are possible, return the one that occurs first lexicographically. If none are possible, return an empty int[]. | | Definition | | Class: | ChatExit | Method: | leaveOrder | Parameters: | String[] | Returns: | int[] | Method signature: | int[] leaveOrder(String[] numSeen) | (be sure your method is public) |
| | | | Notes | - | Order A comes lexicographically before order B if A has a lower value than B in the first position that they disagree. | | Constraints | - | numSeen will contain between 2 and 25 elements inclusive. | - | Each element of numSeen will contain between 3 and 50 characters inclusive. | - | Each element of numSeen will be a single space delimited list of integers. Each integer will be between 0 and 100 inclusive, and will have no extra leading zeros. | - | Each element of numSeen will contain exactly k integers, where k is the number of elements in numSeen. | - | Integer i in element i of numSeen will always be 0. | | Examples | 0) | | | {
"0 1 1",
"2 0 0",
"3 1 0"
} |
| Returns: {1, 0, 2 } | A possible sequence of events is:
Person 0 writes a line.
Person 1 writes a line.
Person 0 writes a line.
Person 1 leaves.
Person 2 writes a line.
Person 0 writes a line.
Person 0 leaves.
Person 2 leaves.
|
|
| 1) | | | {
"0 1 1",
"4 0 0",
"3 1 0"
} |
| Returns: { } | No order is possible here due to the following requirements:
- Person 1 must see 4 lines from person 0, but person 2 must only see 3 lines from person 0.
- Person 0 must see 1 line from person 2, but person 1 must not see any.
The first item above forces person 1 to leave after person 2. The second item forces person 1 to leave before person 2. |
|
| 2) | | | {
"0 100 100 100 100 100",
"100 0 100 100 100 100",
"100 100 0 100 100 100",
"100 100 100 0 100 100",
"100 100 100 100 0 100",
"100 100 100 100 100 0"
} |
| Returns: {0, 1, 2, 3, 4, 5 } | Everyone says exactly 100 lines. Any leaving order is possible. The lexicographically first order is returned. |
|
| 3) | | | {
"0 1 0 0",
"1 0 0 0",
"0 0 0 0",
"0 0 0 0"
} |
| Returns: {2, 3, 0, 1 } | |
| 4) | | | {
"0 0 0 0 0",
"0 0 0 0 0",
"0 0 0 0 0",
"0 0 0 0 0",
"0 0 0 0 0"
} |
| Returns: {0, 1, 2, 3, 4 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Sigh... If only the king could make up his mind. First he wanted rounds in the Royal Wrestling Tournament to last for 2 minutes, then he changed his mind to 3 minutes. He wanted periods in the Royal Hockey Tournament to last for 20 minutes, then he decided on 15. Your job as the Royal Time Keeper would be much easier if he would buy you a modern stopwatch, but he insists that you use the Royal Sand Timers, which have been in his family since the 14th century. You're worried that someday he is going to ask you to measure a time interval that simply can't be measured with the timers you have available.
Given a int[] timers of the times that each of your timers can measure, you want to determine which integral intervals between 1 minute
and maxInterval minutes (inclusive) cannot be measured.
You always begin with all the sand in the bottom of your timers. You turn over some or all of
your timers and the sand begins to fall. Whenever the sand runs out of a timer, you can again turn over some timers. You yell "Start" when you turn
over some timer and "Stop" when the sand runs out of some timer. The time between the yells is the interval you are measuring. Because the king's
patience is limited, you must yell "Stop" no later than maxTime minutes after turning over the first sand timer.
For example, if you had a 5-minute timer and a 7-minute timer, you could easily measure intervals of 5, 7, and 10 minutes. With a little more trouble you can measure intervals of 2, 3, 4, and 9 minutes. To measure 4 minutes, you start by flipping over both timers. When the 5-minute timer runs out, you flip it over and yell "Start", leaving the 7-minute timer running. When the 7-minute timer runs out two minutes later, you again flip over the 5-minute timer, which
has been running for two minutes. The 5-minute timer runs out two minutes later, and you yell "Stop". However, no matter how you try, you cannot
find a way to measure 1, 6, or 8 minutes, assuming the king's patience is limited to 10 minutes.
You will return a int[] of the intervals you cannot measure, arranged in increasing order.
Note that, after years of training, you are able to turn over a sand timer instantaneously.
However, tradition forbids you to ever lay a sand timer on its side.
| | Definition | | Class: | SandTimers | Method: | badIntervals | Parameters: | int[], int, int | Returns: | int[] | Method signature: | int[] badIntervals(int[] timers, int maxInterval, int maxTime) | (be sure your method is public) |
| | | | Constraints | - | timers contains between 1 and 3 elements, inclusive. | - | Each element of timers is between 1 and 20, inclusive. | - | maxInterval is between 1 and 360, inclusive. | - | maxTime is between 1 and 360, inclusive. | - | maxInterval is no greater than maxTime. | | Examples | 0) | | | | 1) | | | | Returns: {1, 3, 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, 25, 27, 29 } | All your timers are even, so you can't measure an odd number of minutes. |
|
| 2) | | | | Returns: { } | You can measure all possible intervals. |
|
| 3) | | | | Returns: {1, 2, 3, 5, 6, 7, 9, 10, 11, 13, 14, 15, 17, 18, 19, 21, 22, 23 } | |
| 4) | | | | Returns:
{1, 2, 3, 4, 5, 8, 9, 10, 11, 12, 15, 16, 17, 18, 19, 21, 22, 23, 24, 25, 28, 29, 30 } | |
| 5) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | An odd-digitable number is a positive integer which consists of only odd digits. For
example, 1, 7, 15, 91 and 73353 are odd-digitable numbers, but 2, 70, 94 and 72653 are not
odd-digitable.
You will be given integers N and M. Your method should return the smallest
odd-digitable number that equals M modulo N. Your method should
return "-1"(quotes for clarity only) if there are no such odd-digitable numbers.
| | Definition | | Class: | OddDigitable | Method: | findMultiple | Parameters: | int, int | Returns: | String | Method signature: | String findMultiple(int N, int M) | (be sure your method is public) |
| | | | Constraints | - | N will be between 2 and 100000, inclusive. | - | M will be between 0 and N-1, inclusive. | | Examples | 0) | | | | 1) | | | | 2) | | | | 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are working for a shipping company, TopShipper, that specializes in shipping products by air. Your cargo jets transport items between a set of airports. From each airport, you can travel directly to some subset of the other airports. (Some airports may be too far, or may not have a safe passageway.) The ability to travel from one airport to another does not guarantee the ability to travel directly in the opposite direction.
You are about to send out a cargo plane on a trip to pick up a large shipment of a certain product. Unfortunately, only one other airport has this product for pickup, and you aren't guaranteed that there is a safe, direct route to the other airport. You may have to travel through one or more other airports to get to your final destination. Nonetheless, you wish to ultimately end up at the airport that has your desired product, and which you can get to by travelling the shortest possible distance.
You are to return a double indicating the number of miles travelled by the cargo plane along the optimal route from the origin to the destination. If no such route exists, return -1.
Given two coordinates, (lat1, lon1) and (lat2, lon2), the shortest distance between them is across an arc known as a great circle. The arclength along a great circle, between two points on the earth can be calculated as:
radius * acos(sin(lat1) * sin(lat2) + cos(lat1) * cos(lat2) * cos(lon1 - lon2))
For purposes of this problem, the radius of the earth is 4000 miles.
You are given the latitude and longitude coordinates of each airport in latitude and longitude. The i-th element of latitude corresponds to the i-th element of longitude. You are also given String[] canTravel. The i-th element of canTravel is a space-delimited list of the 0-based indices of the airports that can be reached from airport i. Finally, you are given origin and destination, the indices of the airports at which you start and end your trip. | | Definition | | Class: | AirTravel | Method: | shortestTrip | Parameters: | int[], int[], String[], int, int | Returns: | double | Method signature: | double shortestTrip(int[] latitude, int[] longitude, String[] canTravel, int origin, int destination) | (be sure your method is public) |
| | | | Constraints | - | latitude, longitude, and canTravel will contain between 1 and 20 elements, inclusive. | - | latitude, longitude, and canTravel will each contain the same number of elements. | - | Each element of latitude will be between -89 and 89, inclusive. | - | Each element of longitude will be between -179 and 179, inclusive. | - | Each element of canTravel will be a space-delimited list of integers, with no leading zeroes. | - | Each integer represented in each element of canTravel will be between 0 and n - 1, where n is the number of elements in latitude. | - | origin and destination will be between 0 and n - 1, inclusive, where n is the number of elements in latitude. | - | No two airports will reside at the same latitude and longitude. | | Examples | 0) | | | {0, 0, 70} | {90, 0, 45} | {"2", "0 2", "0 1"} | 0 | 1 |
| Returns: 10612.237799994255 | Here, we are looking to travel from airport 0 to airport 1.
Using the given formula, we calculate that the distance from 0 to 1 is 6283, from 0 to 2 is 5306, and from 1 to 2 is 5306.
A direct route from airport 0 to 1 would be fastest, if such a route were allowed. Since it is not, we have to travel through airport 2. |
|
| 1) | | | {0, 0, 70} | {90, 0, 45} | {"1 2", "0 2", "0 1"} | 0 | 1 |
| Returns: 6283.185307179586 | Here, we have the same three airports, and there is a safe route between any two. Thus, we take the direct route, which is quickest. |
|
| 2) | | | {0, 30, 60} | {25, -130, 78} | {"1 2", "0 2", "1 2"} | 0 | 0 |
| Returns: 0.0 | We are free to travel as we wish, but since our destination is the same as our point of origin, we don't have much travel to do. |
|
| 3) | | | {0, 20, 55} | {-20, 85, 42} | {"1", "0", "0"} | 0 | 2 |
| Returns: -1.0 | Notice here that we could go from airport 2 to airport 0, but not from 0 to 2. Given the available routes, there is no way we can get from airport 0 to airport 2. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You are given a rectangular board consisting of width times height small squares. Return the number of different ways there are to cut a k-polyomino out of this board, so that the remaining part remains connected.
A k-polyomino is a connected set of k squares. The figure below shows as an example all possible pentominoes (5-polyominoes) ignoring any translations, rotations or reflections.
Connected means side-connected here (both in the polyomino definition and in the requirement for the remaining part of the board) - i.e., you must be able to go from any square to any other by only going through squares and square-sides but not square-corners.
For two ways of cutting a polyomino out of the grid to be different and be counted separately it suffices if at least one grid square is included in the first polyomino but not in the other.
The example below shows some polyomino cuts for k=7. The two on the left (red) are not counted, since they leave the remaining grid disconnected, while the one on the right (green) is counted.
| | Definition | | Class: | PolyominoCut | Method: | count | Parameters: | int, int, int | Returns: | int | Method signature: | int count(int k, int width, int height) | (be sure your method is public) |
| | | | Constraints | - | k will be between 1 and 8, inclusive. | - | width and height will be between k+1 and 800, inclusive. | | Examples | 0) | | | | Returns: 200 | There is only one 1-polyomino (called monomino), and this can be cut out anywhere on the grid. |
|
| 1) | | | | Returns: 480 |
There are in total 484 ways to cut a triomino (3-polyomino) out of a 10x10 square, but 4 of them leave a square in the corner not connected (see figure below), so only 480 satisfy the problem requirements.
|
|
| 2) | | | | Returns: 1704053350 | The worst case fits in an int. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are developing a new software calculator. You decided to add a new
feature - the three-digit button. Clicking on this button will add three digits into
the edit field. This feature is for fast typing of common digit combinations. The problem is to figure out which digits should be placed on this button. You have prepared some statistical data - a long sequence of digits. You have decided that the button will be chosen to minimize the quantity of clicking required for typing this sequence.
You will be given a String[] sequence. The data is divided into several rows only for convenience. You can assume that this is a solid array (i.e. the first character in the i-th row is directly after the last character in the (i-1)-th row). You should return a three-character String containing the digits on the button. If there are several solutions you should return the one which appears first lexicographically.
| | Definition | | Class: | CalcButton | Method: | getDigits | Parameters: | String[] | Returns: | String | Method signature: | String getDigits(String[] sequence) | (be sure your method is public) |
| | | | Constraints | - | sequence will have between 1 and 50 elements, inclusive. | - | Each element of sequence will contain between 1 and 50 characters, inclusive. | - | Each element of sequence will contain only digits ('0'-'9'). | | Examples | 0) | | | | Returns: "000" | We can type the sequence in 5 clicks (the extra button is used twice) |
|
| 1) | | | | Returns: "777" | We can type the entire sequence in 3 clicks |
|
| 2) | | | | Returns: "032" | A sequence can be divided into several rows. |
|
| 3) | | | {"0993034","6","4137","45959935","25939","93993","0","9358333"} |
| Returns: "993" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
We start with an integer and create a sequence of successors using the following procedure:
First split the decimal representation of the given number into several
(at least two) parts, and multiply the parts to get a possible successor.
With the selected successor, we repeat this procedure to get a third number,
and so on, until we reach a single-digit number.
For example, let's say we start with the number 234. The possible successors
are:
- 23 * 4 = 92,
- 2 * 34 = 68 and
- 2 * 3 * 4 = 24.
If we select 68 as the successor, we then generate
6 * 8 = 48 (the only possibility),
from this we generate
4 * 8 = 32 and finally
3 * 2 = 6. With this selection, we have generated
a sequence of 5 integers (234, 68, 48, 32, 6).
Given the starting number, start, return the length of
the longest sequence that can be generated with this procedure. In the
example, the given sequence would be the longest one since the other
selections in the first step would give the sequences: (234, 92, 18, 8)
and (234, 24, 8), which are both shorter than (234, 68, 48, 32, 6).
| | Definition | | Class: | NumberSplit | Method: | longestSequence | Parameters: | int | Returns: | int | Method signature: | int longestSequence(int start) | (be sure your method is public) |
| | | | Notes | - | There can not exist an infinite sequence. | - | Although we use no leading zeros in the decimal representation of the number we start with, the parts we get by splitting the number may have leading zeros (e.g. from 3021 we can get 3 * 021 = 63). | | Constraints | - | start is between 1 and 100000, inclusive. | | Examples | 0) | | | | Returns: 1 | A single-digit number is already the last number. |
|
| 1) | | | | Returns: 4 | For two-digit numbers, there is always only one possible sequence.
Here: 97 -> 63 -> 18 -> 8 (4 numbers). |
|
| 2) | | | | Returns: 5 | The example from the problem statement. |
|
| 3) | | | | Returns: 7 | Here, it is optimal to make a three way split in the first step - i.e. use 8*7*6=336 as the first successor. Although a two way split would lead to a larger number (87*6=522 or 8*76=608), both these choices would lead in the end to a shorter sequence. The optimal sequence is: 876, 8*7*6=336, 33*6=198, 19*8=152, 1*52=52, 5*2=10, 1*0=0. |
|
| 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | We are using aerial photography to estimate the number of snakes in a region.
We have a rectangular image of pixels with values 0 or 1, and we want to count the number
of snakes in the image. The 0's are background, and the 1's are snake-colored.
Because of the attributes of this type of snake (not
gregarious and not likely to curl up tightly),
we have adopted the following rules for identifying a snake:-
A snake is never adjacent to a snake-colored pixel that is not part of itself, not even diagonally.
-
There is a sequence of orthogonally adjacent pixels that traces out the entire snake from its head to its tail.
-
Two pixels of a snake cannot be orthogonally adjacent unless they are
connected in the snake (i.e., are neighbors in the sequence that traces out the snake).
-
A snake occupies between 3 and 20 pixels, inclusive.
As an example, consider the following picture. There are several ways to trace an orthogonal
connection from a head to a tail, but it is NOT a snake since whichever way you trace out
the snake, there are pixels that are orthogonally adjacent to each other but which are not neighbors in the sequence that traced out the snake.
11110
01110
Create a class SnakeCount that contains a method number that is given a
String[] image and that returns the number of snakes in the image.
| | Definition | | Class: | SnakeCount | Method: | number | Parameters: | String[] | Returns: | int | Method signature: | int number(String[] image) | (be sure your method is public) |
| | | | Notes | - | A pixel is "orthogonally" adjacent to another if it is directly above, below, left or right of the other pixel. | | Constraints | - | image will contain between 1 and 50 elements, inclusive. | - | Each element of image will contain exactly n characters, where n is between 1 and 50, inclusive. | - | Each character in each element of image will be '0' (zero) or '1' (one). | | Examples | 0) | | | {"11111111",
"00000010",
"11100000",
"00010001",
"10110011"} |
| Returns: 1 |
The possible snake at the top does not have a head to tail connection that
includes all its pixels. The 2 possible snakes of length 3 in the middle of the
image are eliminated because each is adjacent to a snake-colored pixel diagonally. The possible snake in
the lower left corner is too short. There is a snake in the lower right
corner.
|
|
| 1) | | | {"110111",
"110101",
"000110"} |
| Returns: 1 | The four pixels in the upper left do not make a snake -- the head of a snake cannot be orthogonally adjacent to its tail. The 7 snake-colored pixels in the right 3 columns do make a legitimate snake.
|
|
| 2) | | | | Returns: 0 | This is not a snake since the head cannot be orthogonally adjacent to the tail. |
|
| 3) | | | {
"11111111111111111111111111111111111111111111111111",
"00000000000000000000000000000000000000000000000001",
"11111111111111111111111111111111111111111111111111"
} |
| Returns: 0 | A snake can not occupy more than 20 pixels. |
|
| 4) | | | {
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"10101010101010101010101010101010101010101010101010",
"00000000000000000000000000000000000000000000000000",
"11011011011011011011011011011011011011011011011011",
"10010010010010010010010010010010010010010010010001"
} |
| Returns: 317 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A satellite image consists of a rectangular grid of 1x1 squares, each having
a value of 0 or 1. We want to
identify the longest "blob", where a blob is a collection of 0's that are
connected orthogonally. The length of a blob is defined to be the maximum
Euclidean distance between the centers of any two 0's in the blob. For example,
in the following image
0010
1001
0011
0111
there are 2 blobs. The length of the big blob is sqrt(1+9), the distance
between the 0 in the lower left corner and the one in row 0,
column 1. The length of the tiny blob is 0.
The difficulty is that the image may contain some noise in the form of 1's
that should be 0's. We do not want to underestimate the length of the
longest blob. So we will consider all different ways of choosing up to 4
different 1's and replacing them with 0's. Among all these altered images we
want the length of the longest blob.
Create a class LongBlob that contains a method maxLength that is given a
String[] image and that return the length of the longest blob when up to
4 of the 1's in image are regarded as noise.
| | Definition | | Class: | LongBlob | Method: | maxLength | Parameters: | String[] | Returns: | double | Method signature: | double maxLength(String[] image) | (be sure your method is public) |
| | | | Notes | - | A return value with either an absolute or relative error of less than 1.0E-9 is considered correct. | | Constraints | - | image will contain between 1 and 25 elements inclusive. | - | Each element of image will contain exactly n characters, where n is between 1 and 25 inclusive. | - | Each element of image will contain only the characters '0' (zero) and '1' (one). | | Examples | 0) | | | {"0010",
"1001",
"0011",
"0111"} |
| Returns: 4.242640687119285 |
This is the example given above but now by replacing 4 1's with 0's we can
get a blob that includes diagonally opposite corners. The distance between
two opposite corners is sqrt(9 + 9).
|
|
| 1) | | | | Returns: 7.0 |
Replace the first 4 1's with 0's. Then there is one long blob, whose length
is the distance between the first 0 (that used to be a 1) and the last 0.
|
|
| 2) | | | {"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101",
"0101010101010101010101010",
"1010101010101010101010101"} |
| Returns: 8.0 | |
| 3) | | | {"01011",
"11100",
"01110",
"11111",
"01011",
"11111"}
|
| Returns: 5.656854249492381 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | We have a circular track on which a number of loaded cars are located. Each
car has its own specified unloading position along the track. The cars can be
moved in either direction along the track, but cannot pass each other.
We want to make a worklist, specifying the order in which the cars should be
moved. The order of cars in the worklist must allow each car to be moved just
once all the way to its unloading position. How many different orders will
allow this?
The cars are each named with a lowercase letter, and their destinations with
the same letter but in uppercase. The positions of the cars and of their
destinations are given by a sequence of letters that is regarded as circular by
wrapping around the ends. So, for example, "BACacb" describes a situation in
which going clockwise around the track we encounter B, A, C, a, c, b, and then
return back to B. Here
there are 3 different orders in which the cars can be moved to their
destinations: a then c then b, a then b then c, or b then a then c.
Given the
original positions of the cars and destinations in a String circle,
return the number of different orders in which the
cars can be moved. If there are more than 2,000,000,000 orders return -1.
If no order is possible, your method should return 0.
| | Definition | | Class: | CircleCount | Method: | countOrder | Parameters: | String | Returns: | int | Method signature: | int countOrder(String circle) | (be sure your method is public) |
| | | | Constraints | - | circle will contain between 2 and 50 characters, inclusive. | - | Each character will be a letter ('a'-'z', 'A'-'Z'). | - | No character will appear more than once in circle. | - | If a letter appears in circle, it will appear both in uppercase and in lowercase. | | Examples | 0) | | | | Returns: 3 |
This is the example given above.
|
|
| 1) | | | | Returns: 0 |
We cannot move c first. If we move a first, then we can never move b to B, but
if we move b first we can never move a to A.
|
|
| 2) | | | | Returns: 1 |
We must move car x. We could move it to its destination either in a clockwise
or a counterclockwise direction but there is only one order for choosing
the cars to move.
|
|
| 3) | | | | Returns: 2 |
The possibilities are 1) a then b then c, or 2) c then b then a (moving the cars in the other direction).
|
|
| 4) | | | "AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPp" |
| Returns: -1 |
These 16 cars can be move in any order, so there are 16! orders which is
greater than 2,000,000,000 |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | In any field of research, there are many journals to which one can submit an article for publication. One criterion that is commonly used to choose between journals is the impact factor, a measure of the importance of a journal and the papers that are published there. The impact factor of a journal is defined as the average number of citations each paper in that journal receives from papers in other journals. Citations from papers in the same journal are not counted in order to prevent its editors from inflating their impact factor by preferentially accepting papers that cite other papers in their journal. Although impact factors are not a fair way to judge the quality of research, they do provide a quantitative method for comparing journals to each other.
Write a class AcademicJournal with a method rankByImpact that takes a String[] papers, a collection of published papers from which to calculate impact factors, and returns a String[] with the journal names sorted in decreasing order by impact factor. Each element of papers contains the information for a single paper, and is formatted with the name of the paper's journal (consisting of only uppercase English characters and spaces), followed by a period, followed by zero or more integers specifying the zero-based indices of the papers it cites. The citation indices contain no extra leading zeroes, and are separated from each other and from the period character by single spaces. If there is a tie in the impact factors, the journal with more papers comes first in the return value. If there is still a tie, the journal with the lexicographically earlier name comes first. | | Definition | | Class: | AcademicJournal | Method: | rankByImpact | Parameters: | String[] | Returns: | String[] | Method signature: | String[] rankByImpact(String[] papers) | (be sure your method is public) |
| | | | Notes | - | Although it is not supposed to happen, it is possible for two papers to reference each other due to delays in the editing and publishing process. | - | A sloppy author or editor of a paper might accidentally include multiple citations to another paper. In your calculation of the impact factors, count citations from one paper to another only once. | | Constraints | - | papers will contain between 1 and 50 elements, inclusive. | - | Each element of papers will contain between 2 and 50 characters, inclusive. | - | Each element of papers will be formatted as described in the problem statement. | - | Each index will be between 0 and the number of papers - 1, inclusive. | - | A paper will not contain a reference to itself. | | Examples | 0) | | | {"A.", "B. 0", "C. 1 0 3", "C. 2"} |
| Returns: {"A", "B", "C" } | The one paper in journal A is cited two times, so A's impact factor is 2/1. The one paper in journal B is cited once, so B's impact factor is 1/1. The two papers in journal C only receive citations from each other. Since citations from a paper in the same journal do not count, C's impact factor is 0/2. |
|
| 1) | | | {"RESPECTED JOURNAL.", "MEDIOCRE JOURNAL. 0", "LOUSY JOURNAL. 0 1",
"RESPECTED JOURNAL.", "MEDIOCRE JOURNAL. 3", "LOUSY JOURNAL. 4 3 3 4",
"RESPECTED SPECIFIC JOURNAL.", "MEDIOCRE SPECIFIC JOURNAL. 6", "LOUSY SPECIFIC JOURNAL. 6 7"} |
| Returns:
{"RESPECTED JOURNAL",
"RESPECTED SPECIFIC JOURNAL",
"MEDIOCRE JOURNAL",
"MEDIOCRE SPECIFIC JOURNAL",
"LOUSY JOURNAL",
"LOUSY SPECIFIC JOURNAL" } | There is an impact factor tie between the SPECIFIC and non-specific versions of each tier of journal. Since the non-specific ones have more papers, they win the tiebreaker. |
|
| 2) | | | {"NO CITATIONS.", "COMPLETELY ORIGINAL."} |
| Returns: {"COMPLETELY ORIGINAL", "NO CITATIONS" } | If there is a tie in impact factor and number of papers, the journal with the lexicographically earlier name comes first. |
|
| 3) | | | {"CONTEMPORARY PHYSICS. 5 4 6 8 7 1 9",
"EUROPHYSICS LETTERS. 9",
"J PHYS CHEM REF D. 5 4 6 8 7 1 9",
"J PHYS SOC JAPAN. 5 4 6 8 7 1 9",
"PHYSICAL REVIEW LETTERS. 5 6 8 7 1 9",
"PHYSICS LETTERS B. 6 8 7 1 9",
"PHYSICS REPORTS. 8 7 1 9",
"PHYSICS TODAY. 1 9",
"REP PROGRESS PHYSICS. 7 1 9",
"REV MODERN PHYSICS."} |
| Returns:
{"REV MODERN PHYSICS",
"EUROPHYSICS LETTERS",
"PHYSICS TODAY",
"REP PROGRESS PHYSICS",
"PHYSICS REPORTS",
"PHYSICS LETTERS B",
"PHYSICAL REVIEW LETTERS",
"CONTEMPORARY PHYSICS",
"J PHYS CHEM REF D",
"J PHYS SOC JAPAN" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You have a few very large files and they need to be sorted according to a total order that is defined for them. The only information you can use to sort them is the relative orders of pairs of files. Unfortunately, finding the relative order of a pair takes N*M minutes where N and M are the sizes of the two files being compared. Given the sizes of the files being sorted, your task is to find a comparison plan that guarantees you will find the correct order in the minimal amount of time. You should return that minimum. | | Definition | | Class: | BasicSorting | Method: | minSortTime | Parameters: | int[] | Returns: | int | Method signature: | int minSortTime(int[] sizes) | (be sure your method is public) |
| | | | Notes | - | No two files are 'equal' -- one of them always belongs strictly after the other in the ordering. | | Constraints | - | sizes will contain between 2 and 6 elements, inclusive. | - | Each element of sizes will be between 1 and 100, inclusive. | | Examples | 0) | | | | Returns: 11 | With 3 files, you must compare all 3 pairs of files to figure out the order. The total time is thus 1*2 + 1*3 + 2*3 = 11 minutes. |
|
| 1) | | | | Returns: 5 | One way to do this is to find the order on three of the files, which takes 3 minutes. Then, compare the fourth file to the middle one of those three files. Finally, if the fourth file comes after the middle file, compare it to the last file, while if it comes before the fourth file, compare it to the first file. |
|
| 2) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | One type of compression is run length encoding. In this case, a character may be preceded by an integer N, which indicates that the character should be repeated N times. For example, the String "3ABBC10D" would be decompressed as "AAABBCDDDDDDDDDD". A character that is not preceded by an integer is repeated just once. We are going to enrich this slightly be allowing a sequence of characters to be preceded by an integer N, indicating that the sequence should be repeated N times. In this case, the sequence of characters should be surrounded by parentheses. Additionally, you may nest the compressed sequences. Thus, the compressed sequence "X2(2A3(BC))X" would be decompressed as "XAABCBCBCAABCBCBCX". You will be given a String of uppercase letters and are to compress it in such a way that the result has as few characters as possible. If there are multiple ways to do this, choose the one that comes first lexicographically (using standard ASCII ordering). | | Definition | | Class: | RunLengthPlus | Method: | compress | Parameters: | String | Returns: | String | Method signature: | String compress(String s) | (be sure your method is public) |
| | | | Constraints | - | s will contain between 1 and 50 uppercase letters, inclusive. | | Examples | 0) | | | | 1) | | | | 2) | | | "ABCBACBABCBABCABACACBCBABACBCBBABACBACBCACBBAC" |
| Returns: "ABCBA2(CBAB)CABACACBCBABACBC2BA2(BAC)BCAC2BAC" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Given a positive integer n, you are to find the largest subset S of {1,...,n} such that no
member of S is divisible by another member of S. If there is more than one subset of the maximum size,
choose the lexicographically earliest such subset. A subset S is lexicographically earlier than a subset T if the smallest element that is a member of one of the subsets, but not both, is a member of S. Return the subset S as a int[] in increasing order.
| | Definition | | Class: | Indivisible | Method: | largestSubset | Parameters: | int | Returns: | int[] | Method signature: | int[] largestSubset(int n) | (be sure your method is public) |
| | | | Constraints | - | n is between 1 and 1000, inclusive. | | Examples | 0) | | | | Returns: {4, 5, 6, 7, 9} | There are several possible subsets of size 5, such as { 4,6,7,9,10 }, but { 4,5,6,7,9 } is the lexicographically earliest. |
|
| 1) | | | | 2) | | | | 3) | | | | Returns: {4, 6, 9, 10, 11, 13, 14, 15, 17, 19, 21, 23} | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Two people are within "shouting distance" if one can hear the other one shout.
We have a group of identical people located across the countryside.
We want to know how small the shouting distance can be and still allow a message to
originate with anyone and be heard by everyone else by a sequence of shouts.
The locations of the people are given by int[]'s x and y, with the location
of the i-th person given by the i-th element of x and the i-th element of y.
Create a class Shouting that contains a method shout that is given
x and y and that returns the smallest
shouting distance that will allow complete communication.
| | Definition | | Class: | Shouting | Method: | shout | Parameters: | int[], int[] | Returns: | double | Method signature: | double shout(int[] x, int[] y) | (be sure your method is public) |
| | | | Notes | - | The returned value must be accurate to within a relative or absolute value of 1E-9. | | Constraints | - | x and y will contain the same number of elements, between 1 and 50 inclusive. | - | Each element of x and y will be between -10,000 and 10,000 inclusive. | | Examples | 0) | | | | Returns: 0.0 |
These two people are standing in the same spot, so
there is no need to shout.
|
|
| 1) | | | {3,3,3,3,3,3,3} | {2,3,4,3,9,8,1} |
| Returns: 4.0 |
These people are standing in a line, and the biggest gap is between (3,4)
and (3,8) which can be bridged by a shout of length 4. |
|
| 2) | | | | Returns: 7.0710678118654755 |
These people are standing in a square. Shouting around the edges of the
square is the best way to communicate, and each edge has a length of 5*sqrt(2) |
|
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | It is expected that the more expensive the automobile, the more features are included and the less often it breaks down. While expected, this is not always true. Given a set of automobile specs, find the largest subset where the condition is reversed.
Write a class AutoMarket that contains a method maxSet, which accepts a int[] cost, int [] features and int[] fixTimes and returns the size of the largest subset that can be ordered such that each succeeding automobile costs more, has less features and must be fixed more often. All conditions are strict. The ith element in cost, features and fixTimes represents the ith car, which costs cost[i] dollars, has features[i] features and must be fixed fixTimes[i] times per year. | | Definition | | Class: | AutoMarket | Method: | maxSet | Parameters: | int[], int[], int[] | Returns: | int | Method signature: | int maxSet(int[] cost, int[] features, int[] fixTimes) | (be sure your method is public) |
| | | | Constraints | - | cost, features and fixTimes will each have between 1 and 50 elements, inclusive. | - | cost, features and fixTimes will have the same number of elements. | - | Each element of cost will be between 1 and 100000, inclusive. | - | Each element of features and fixTimes will be between 1 and 100, inclusive. | | Examples | 0) | | | {10000, 14000, 8000, 12000} | {1, 2, 4, 3} | {17, 15, 8, 11} |
| Returns: 3 | The largest set contains all elements except the first. |
|
| 1) | | | {1,2,3,4,5} | {1,2,3,4,5} | {1,2,3,4,5} |
| Returns: 1 | |
| 2) | | | {9000, 6000, 5000, 5000, 7000} | {1, 3, 4, 5, 2} | {10, 6, 6, 5, 9} |
| Returns: 4 | |
| 3) | | | {1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20} | {20,19,18,17,16,15,14,13,12,11,10,9,8,7,6,5,4,3,2,1} | {1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20} |
| Returns: 20 | |
| 4) | | | {1000, 1000, 1000, 1000, 2000} | {3,3,4,3,3} | {3,3,3,4,3} |
| Returns: 1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | The toy company "I Can't Believe It Works!" has hired you to help develop educational toys. The current project is a word toy that displays four letters at all times. Below each letter are two buttons that cause the letter above to change to the previous or next letter in alphabetical order. So, with one click of a button the letter 'c' can be changed to a 'b' or a 'd'. The alphabet is circular, so for example an 'a' can become a 'z' or a 'b' with one click.
In order to test the toy, you would like to know if a word can be reached from some starting word, given one or more constraints. A constraint defines a set of forbidden words that can never be displayed by the toy. Each constraint is formatted like "X X X X", where each X is a string of lowercase letters. A word is defined by a constraint if the ith letter of the word is contained in the ith X of the contraint. For example, the constraint "lf a tc e" defines the words "late", "fate", "lace" and "face".
You will be given a String start, a String finish, and a String[] forbid. Calculate and return the minimum number of button presses required for the toy to show the word finish if the toy was originally showing the word start. Remember, the toy must never show a forbidden word. If it is impossible for the toy to ever show the desired word, return -1. | | Definition | | Class: | SmartWordToy | Method: | minPresses | Parameters: | String, String, String[] | Returns: | int | Method signature: | int minPresses(String start, String finish, String[] forbid) | (be sure your method is public) |
| | | | Constraints | - | start and finish will contain exactly four characters. | - | start and finish will contain only lowercase letters. | - | forbid will contain between 0 and 50 elements, inclusive. | - | Each element of forbid will contain between 1 and 50 characters. | - | Each element of forbid will contain lowercase letters and exactly three spaces. | - | Each element of forbid will not contain leading, trailing or double spaces. | - | Each letter within a group of letters in each element of forbid will be distinct. Thus "aa a a a" is not allowed. | - | start will not be a forbidden word. | | Examples | 0) | | | "aaaa" | "zzzz" | {"a a a z", "a a z a", "a z a a", "z a a a", "a z z z", "z a z z", "z z a z", "z z z a"} |
| Returns: 8 | |
| 1) | | | | Returns: 4 | Simply change each letter one by one to the following letter in the alphabet. |
|
| 2) | | | | Returns: 50 | Just as in the previous example, we have no forbidden words. Simply apply the correct number of button presses for each letter and you're there. |
|
| 3) | | | "aaaa" | "zzzz" | {"bz a a a", "a bz a a", "a a bz a", "a a a bz"} |
| Returns: -1 | Here is an example where it is impossible to go to any word from "aaaa". |
|
| 4) | | | "aaaa" | "zzzz" | {"cdefghijklmnopqrstuvwxyz a a a",
"a cdefghijklmnopqrstuvwxyz a a",
"a a cdefghijklmnopqrstuvwxyz a",
"a a a cdefghijklmnopqrstuvwxyz"} |
| Returns: 6 | |
| 5) | | | | 6) | | | "zzzz" | "aaaa" | {"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk",
"abcdefghijkl abcdefghijkl abcdefghijkl abcdefghijk"} |
| Returns: -1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | In a parking lot there are a lot of cars and parking spots and all cars want to drive to a parking spot. Due to the traffic regulations cars may only drive parallel to the boundaries of the parking lot and only at the speed of one square per unit of time.
Usually all cars drive to the nearest available parking spot, but that might turn out badly for some cars. Consider for example the following car park
.C.....P.X...
XX.......X..P
XX.....C.....
(here 'C' stands for car, 'P' for parking spot, 'X' for wall and '.' for empty spot)
If the car on the bottom drives to its nearest parking spot, the upper left car must drive all the way to the right, taking 13 units of time. If, however, the car on the bottom drives to the parking spot on the right, it will take 6 units of time for both cars to find a parking spot.
Return the minimal amount of time it takes before every car can have a parking spot (assuming that the cars act socially like above). All cars start on an empty spot. Cars are small and any number of them can drive on the same square simultaneously. They can drive over empty spots and parking spots, but not through walls. Each car has to end on a separate parking spot.
If it is impossible for each car to drive to a parking place, return -1.
| | Definition | | Class: | Parking | Method: | minTime | Parameters: | String[] | Returns: | int | Method signature: | int minTime(String[] park) | (be sure your method is public) |
| | | | Constraints | - | park will contain between 1 and 50 elements, inclusive. | - | All elements of park have equal length. | - | Each element of park has length between 1 and 50, inclusive. | - | Each character in park is either 'C', 'P', 'X' or '.'. | - | There will be no more than 100 cars and 100 parking places in park. | | Examples | 0) | | | {"C.....P",
"C.....P",
"C.....P"} |
| Returns: 6 | Every car just drives to the opposite parking spot. |
|
| 1) | | | {"C.X.....",
"..X..X..",
"..X..X..",
".....X.P"} |
| Returns: 16 | The slalom takes the car 16 units of time. |
|
| 2) | | | {"XXXXXXXXXXX",
"X......XPPX",
"XC...P.XPPX",
"X......X..X",
"X....C....X",
"XXXXXXXXXXX"}
|
| Returns: 5 | This would take 11 instead of 5 units of time if the car on the bottom drove to its nearest parking spot. |
|
| 3) | | | {".C.",
"...",
"C.C",
"X.X",
"PPP"} |
| Returns: 4 | While driving, the cars can be on the same empty spot or parking spot, but they have to finish on different parking spots. |
|
| 4) | | | {"CCCCC",
".....",
"PXPXP"}
|
| Returns: -1 | There are not enough parking spots for all the cars. |
|
| 5) | | | {"..X..",
"C.X.P",
"..X.."} |
| Returns: -1 | The car can't reach the parking spot. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Square City got a new snow plow. Unfortunately, it is impossible for the plow to do a 180 degree turn at an intersection, and due to traffic rules it is not allowed to drive backwards. Soon it was realized that some streets can't be reached by the new snow plow and it is your job to calculate how many.
You are given a map of the city, consisting of '|', '+', '-' and ' '. For example, a possible map may look like this:
+-+-+-+-+-+-+
| | | | | | |
+-+ +-+ +-+ +
| | |
+-+-+-+-+-+-+
Each '+' stands for an intersection, whereas '-' or '|' denotes a single two-way street.
In addition to the map, you are given the row number (counted from the top) and column number (counted from the left) of the snow plow's garage (both indexed from 1), where the plow starts its snow clearing tours. Note that only the rows and columns containing intersections are counted. After the snow clearing, the plow must be able to get back to the garage. Therefore, a street forming a dead end is not reachable because at the end intersection, the only possible way back would be using the same street, and a 180 degree turn is not possible. The only exception to this is that the plow can turn in any direction at the garage, so it is not important in which direction the plow is initially facing. Determine the number of streets which can't be cleared by the snow plow because there is no way back to the garage. In the example above, if the garage is at (2,7), there are two streets which can't be cleared: the streets connecting (3,5) with (3,6) and (3,6) with (3,7). | | Definition | | Class: | SnowClearing | Method: | unreachable | Parameters: | String[], int, int | Returns: | int | Method signature: | int unreachable(String[] citymap, int row, int column) | (be sure your method is public) |
| | | | Notes | - | If citymap contains n elements, and each element has length m, then there are (n+1)/2 * (m+1)/2 intersections. | | Constraints | - | citymap contains an odd number (between 3 and 49, inclusive) of elements. | - | Each element of citymap contains the same number of characters. | - | Every second element of citymap consists of an odd number (between 3 and 49, inclusive) of characters from the set {'|',' '}, where character '|' can only appear at even positions in the string (positions 0, 2, ...). | - | All other elements of citymap consists of an odd number (between 3 and 49, inclusive) of characters from the set {'+','-',' '}, where character '+' appears at every even position in the string (position 0, 2, ...). | - | row will be between 1 and (1 + number of elements in citymap)/2, inclusive. | - | column will be between 1 and (1 + length of elements in citymap)/2, inclusive. | - | There will always be a path between each pair of intersections using the available streets. | | Examples | 0) | | | {"+-+-+-+-+-+-+"
,"| | | | | | |"
,"+-+ +-+ +-+ +"
,"| | | "
,"+-+-+-+-+-+-+"} | 2 | 7 |
| Returns: 2 | This is the example from above. The snow plow starts at the middle intersection, on the far right of the city. Since the snow plow can turn at the garage, it can do several tours and clear all streets but the two streets connecting (3,5) to (3,6) and (3,6) to (3,7). |
|
| 1) | | | | Returns: 3 | In this example, the snow plow can't clear any street, since it would get stuck at (2,1) or (2,2). |
|
| 2) | | | {"+-+-+"
,"| | |"
,"+-+ +"} | 1 | 3 |
| Returns: 1 | Note that it is not important in which direction the snow plow is initally facing. Therefore the only street it can't clear is the street connecting (1,3) to (2,3). |
|
| 3) | | | {"+-+-+"
,"| |"
,"+-+-+"} | 2 | 2 |
| Returns: 0 | Here the snow plow can clear every street. |
|
| 4) | | | {"+ +-+ +-+-+ +-+-+ +-+-+-+-+-+-+-+-+-+-+ +-+-+-+ +"
,"| | | | | | | | | | | |"
,"+ +-+-+-+ +-+-+-+ +-+ +-+-+-+-+ +-+-+-+ +-+ +-+-+"
,"| | | | | | | | | | | | | "
,"+ +-+-+ +-+-+ +-+-+-+-+-+-+-+ +-+ +-+ +-+ +-+ +-+"
,"| | | | | | | | | | | |"
,"+-+-+ +-+-+-+ +-+ +-+ + +-+-+-+-+-+ +-+-+ +-+-+-+"
,"| | | | | | | | | |"
,"+-+-+-+ +-+-+-+-+-+-+-+-+-+-+ +-+ +-+ +-+-+-+-+-+"
," | | | | | | | | | | | |"
,"+ +-+-+-+-+ + +-+ +-+ +-+ +-+-+ +-+-+-+-+-+ +-+-+"
,"| | | | | | | | | | | | |"
,"+-+-+-+-+-+ +-+-+ +-+-+-+ +-+-+ +-+-+ +-+-+ +-+-+"
," | | | | | | "
,"+-+-+-+-+-+-+ +-+-+-+ + +-+-+ +-+ +-+-+-+ +-+-+ +"
,"| | | | | | | | | |"
,"+ +-+-+-+-+-+-+ + +-+-+-+-+-+-+-+-+ +-+-+-+ + +-+"
,"| | | | | | | | | | | | | | | | |"
,"+-+-+-+ +-+-+-+-+ +-+ + +-+ +-+-+-+ +-+-+ +-+ +-+"
," | | | | | | | | "
,"+-+ +-+-+-+-+-+ +-+ + +-+-+-+-+-+-+ + + +-+-+ +-+"
," | | | | | | | | | | | | | | | | | "
,"+-+-+ + +-+ +-+ +-+-+-+-+ + + + +-+-+ +-+-+-+-+-+"
," | | | | | | | | | | | | | "
,"+ +-+ + + +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ +-+-+"
,"| | | | | | | | | | | | |"
,"+-+-+ +-+-+-+-+ +-+ + +-+-+-+-+ +-+ +-+-+-+-+-+-+"
," | | | | | | | | | | | "
,"+-+ + +-+-+-+-+ + +-+-+-+ +-+-+-+-+ +-+ +-+-+-+-+"
," | | | | | | | | | | | | | | | "
,"+-+-+-+-+-+-+ +-+-+-+-+-+-+ +-+ +-+ +-+ +-+-+ +-+"
,"| | | | | | | | | | | | | | |"
,"+-+-+-+-+-+-+ + +-+-+-+-+-+ +-+-+-+-+ +-+ + +-+-+"
,"| | | | | | | | | | | | | | | |"
,"+ +-+ +-+-+ + + +-+-+ + + +-+-+-+-+ +-+ +-+-+-+-+"
," | | | | | | | | | | | | |"
,"+ +-+ +-+-+-+ +-+-+-+-+ +-+-+-+ +-+-+-+ +-+-+ + +"
,"| | | | | | | | | | | | "
,"+-+-+-+-+-+-+-+ +-+-+ +-+-+-+-+-+ +-+-+ +-+-+ +-+"
,"| | | | | | | | | | | "
,"+-+-+-+ +-+-+-+-+-+-+-+-+ +-+ +-+ +-+-+ +-+-+-+-+"
," | | | | | | | | | | | | | | | | |"
,"+-+-+-+-+-+-+-+ +-+-+ +-+-+ +-+-+-+ +-+ + +-+-+ +"
," | | | | | | | | | | | |"
,"+ +-+-+-+-+-+ +-+ +-+ +-+-+-+ +-+-+-+-+-+ + +-+-+"
,"| | | | | | | | | | | | | |"
,"+-+-+-+-+-+-+-+ + +-+-+-+ + +-+-+-+-+-+-+-+ +-+ +"
," | | | | | | | | | | | | |"
,"+-+-+-+-+-+-+-+-+-+ +-+-+-+-+ +-+-+-+ + + +-+-+ +"} | 10 | 12 |
| Returns: 160 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | We have a program segment and want to analyze the control flow through it.
We have already replaced the actual code with simpler code that captures just
the control logic. The code we want to analyze consists of a sequence of
statements in which each statement is one of the following two types:-
IF target1 ELSE target2
-
RETURN
Execution of an IF statement is followed by execution of one of its two targets.
Each target is an integer referring to a zero-based position in the code sequence.
The two targets may be identical. Execution of a RETURN statement ends the execution.
Execution of the program segment starts at the first
statement (statement 0) and concludes when it reaches a RETURN statement. We call such a
sequence an "execution path."
In order to optimize the code, we want to find
the smallest loop in the program segment that can be executed.
A loop is defined to be a set of statements such that -
Only one statement in the loop (the entry point) may be immediately
preceded in an execution path by
a statement that is not in the loop.
-
If a loop contains statement 0, then statement 0 must be the entry point for that loop.
-
If a statement S is in the loop, then there is an execution path that
executes S and then, without executing any statement outside the loop,
executes every statement (including S) in the loop at least once.
Create a class Loopy that contains a method minLoop that is given a String[]
code containing the sequence of statements and that returns the smallest number
of statements in code that form a loop. If code contains no loop, return 0.
| | Definition | | Class: | Loopy | Method: | minLoop | Parameters: | String[] | Returns: | int | Method signature: | int minLoop(String[] code) | (be sure your method is public) |
| | | | Constraints | - | code will contain between 1 and 50 elements inclusive. | - | Each element of code will be one of the two forms above. | - | Each RETURN statement has no spaces. | - | Each IF statement has exactly 3 spaces. | - | Each target1 and target2 will be an integer with no extraneous leading zeroes. | - | Each target1 and target2 will be between 0 and n-1 inclusive, where n is the number of elements in code. | | Examples | 0) | | | {"RETURN", "IF 0 ELSE 1"} |
| Returns: 0 |
Execution immediately returns, so there is no loop.
|
|
| 1) | | | {"IF 1 ELSE 2","IF 1 ELSE 2","RETURN"} |
| Returns: 1 |
Statement 1 forms a loop. |
|
| 2) | | | {"IF 1 ELSE 2","RETURN", "IF 3 ELSE 2", "IF 2 ELSE 3"} |
| Returns: 0 |
No execution path that includes
either statement 2 or 3 can ever reach a RETURN statement. The only legal execution
path is statement 0 followed by statement 1 so there is no loop. |
|
| 3) | | | {"IF 1 ELSE 2","IF 3 ELSE 3","IF 4 ELSE 1",
"IF 4 ELSE 5","RETURN","IF 0 ELSE 6",
"IF 6 ELSE 6","IF 7 ELSE 2"} |
| Returns: 5 |
Statements 0, 1, 2, 3, and 5 form a loop whose entry point is statement 0. Note that
no execution path contains statement 7, so statement 2 is never preceded
by a non-loop statement.
|
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | When a paragraph is justified, the widths of the spaces on each line except the
last are adjusted so that each line except the last has exactly the same width.
This is done in such a way that each space within a line has the same width (spaces can have any width, not just integral ones).
This can lead to some lines with undesirably wide spaces. For example, if each
line is 20 characters long, and one line contains 10 characters and 1 space,
while the next contains 15 characters and 3 spaces, the space in the first line
will be far wider than the spaces in the next line.
Your task is to find a way to place the words in a paragrah so that the width
of the widest space is as small as possible. You may assume that each letter
has the same width, and that each space must be at least as wide as one letter.
You will be given a String[], paragraph, representing the
text to be justified, and an int width, indicating the
width (in letters) that each line except the last must have. You should always
place at least two words on each line except the last (the constraints ensure
this is possible). The constraints will ensure that the return is unique.
You should return a String[], each
element of which represents a single line. Keep in mind that the last line of
the return will not be justified. Words within a line should be separated by
single spaces, and there should be no leading or trailing spaces.
| | Definition | | Class: | Justify | Method: | justify | Parameters: | String[], int | Returns: | String[] | Method signature: | String[] justify(String[] paragraph, int width) | (be sure your method is public) |
| | | | Notes | - | A word is a sequence of non-space characters that is not a substring of any other sequence of non-space characters. | | Constraints | - | paragraph will contain between 1 and 50 elements, inclusive. | - | Each element of paragraph will contain between 1 and 50 characters ('a'-'z', 'A'-'Z', '.', ',', '-' and ' '). | - | No element of paragraph will contain leading, trailing, or double spaces. | - | width will be between 10 and 80, inclusive. | - | There will be a way to place the words in paragraph such that each line except the last has at least two words. | - | The best return will be unique. | | Examples | 0) | | | {"the quick brown fox jumps over the lazy dog"} | 13 |
| Returns: { "the quick", "brown fox", "jumps over", "the lazy dog" } | With the width this small, the best we can do is to have some spaces that are 5 times as wide as letters. In the first line, for instance, there are 8 letters, and only one space. Note that the last line need not be justified. |
|
| 1) | | | {"the quick brown fox", "jumps over the lazy dog"} | 20 |
| Returns: { "the quick brown fox", "jumps over the lazy", "dog" } | We can do much better with the same words, but a larger width. In this case, the widest space is only 1.3333 times as wide as a letter. |
|
| 2) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
An important metric in the theory of sorting is the number of inversions in a sequence.
An inversion is any pair of elements that are out of order, meaning that the earlier element in the sequence has a larger value than the later element in the sequence. For example, the sequence
3 1 4 2 5
has three inversions: 3-1, 3-2, and 4-2.
Given integers n and inv, you are to return a permutation of the sequence 1,...,n that has
exactly inv inversions. If it is not possible to create a permutation that has inv inversions, return the empty sequence. If there is more than one permutation that has exactly inv inversions, return the lexicographically earliest.
A permutation P is lexicographically earlier than a permutation Q if, at the first index at which the permutations differ, the element in P is smaller than the element in Q.
| | Definition | | Class: | Inversions | Method: | createExample | Parameters: | int, int | Returns: | int[] | Method signature: | int[] createExample(int n, int inv) | (be sure your method is public) |
| | | | Constraints | - | n is between 1 and 1000, inclusive. | - | inv is between 0 and 1000000, inclusive. | | Examples | 0) | | | | Returns: { 1, 2, 3 } | The only way for the sequence to have no inversions is for it to be in increasing order. |
|
| 1) | | | | Returns: { 4, 3, 2, 1 } | A sequence in decreasing order. |
|
| 2) | | | | Returns: { } | There is no way to get 10 inversions with only 4 elements. |
|
| 3) | | | | Returns: { 1, 2, 3, 4, 5, 6, 7, 14, 13, 12, 11, 10, 9, 8 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Shipping is a complicated business, particularly when you are shipping fragile items. To do well, you need to deliver packages quickly, at a low cost, and without breaking them. To this end, you would like software to help you send a package, given the package's cost and the maximum delivery time. There are a number of different routes that are continually traversed by your fleet of trucks. Each route takes a certain amount of time, and it costs a certain amount to send a package along that route. Furthermore, there is a probability associated with each route indicating the percent chance that a package will be damaged along that route.
As the shipping company, you must pay the cost of an item if it is damaged during shipping. Your goal is to find the way to ship an item with the lowest expected cost. For example, if one shipping plan has a cost of X, but a 50% probability of damaging an item, it might end up with a higher expected cost than a plan that has a cost of 2X but only a 5% chance of damaging an item.
You will be given a String[], routes, each element of which represents a single route and will be formatted as "ORIGIN DESTINATION TIME COST PROBABILITY" where ORIGIN and DESTINATION are city codes (sequences of uppercase letters), TIME is an integer between 1 and 50, COST is an integer between 1 and 20, and PROBABILITY is a real number between 0 and 10, all ranges inclusive. The inputs origin and destination are the starting and ending points for the package to be delivered. time is the maximum allowed delivery time, and is in the same units as TIME in routes. cost is the cost of the package, and is in the same units as COST in routes. You should find the shipping plan with the lowest expected cost, and return that cost as a double. | | Definition | | Class: | PackageShipping | Method: | ship | Parameters: | String[], String, String, int, int | Returns: | double | Method signature: | double ship(String[] routes, String origin, String destination, int time, int packageCost) | (be sure your method is public) |
| | | | Notes | - | A route from A to B does not imply a route from B to A. | - | You may assume that there is no waiting time when a package is transferred from one route to another. | - | You won't know that a package is damaged until it reaches its destination, so you must still send it all the way to the destination, even if it is damaged at the beginning of the trip. | | Constraints | - | routes will contain between 1 and 50 elements, inclusive. | - | Each element of routes will be formatted as "ORIGIN DESTINATION TIME COST PROBABILITY", with no double, leading or trailing spaces. | - | Each element of routes will contain between 9 and 50 characters, inclusive. | - | ORIGIN and DESTINATION will each be sequences of uppercase letters ('A'-'Z') | - | In no element of routes will ORIGIN equal DESTINATION. | - | TIME will be an integer between 1 and 50, inclusive, with no leading zeros. | - | COST will be an integer between 1 and 20, inclusive, with no leading zeros. | - | PROBABILITY will be between 0 and 10, inclusive, and will be formatted as a sequence of 1 or more digits ('0'-'9') and an optional decimal point (extra leading/trailing zeros allowed). | - | origin will be a sequence of uppercase letters matching an ORIGIN in some element of routes. | - | destination will be a sequence of uppercase letters matching a DESTINATION in some element of routes. | - | time will be between 1 and 100, inclusive. | - | packageCost will be between 1 and 1,000,000,000, inclusive. | - | There will be some way to deliver the package in the given amount of time or less. | - | No two elements of routes will have the same origin and destination. | - | origin and destination will not be the same. | | Examples | 0) | | | {"SANFRAN CHICAGO 20 3 0.4",
"SANFRAN MEMPHIS 30 5 1.0",
"CHICAGO NEWYORK 15 2 2.0",
"MEMPHIS NEWYORK 8 6 0.1"} | "SANFRAN" | "NEWYORK" | 100 | 100 |
| Returns: 7.392000000000005 | There are two possibilities here. We can ship the package through either CHICAGO or MEMPHIS. If we ship the package through CHICAGO, it will cost us 5, and there will be a 2.392% chance that it will be damaged. If we ship through MEMPHIS, it will cost 11, but there will only be a 1.099% chance of damage. Since the package has a cost of 100, the first option will have an expected cost of 7.392, while the second will have an expected cost of 12.099. |
|
| 1) | | | {"SANFRAN CHICAGO 20 3 0.4",
"SANFRAN MEMPHIS 30 5 1.0",
"CHICAGO NEWYORK 15 2 2.0",
"MEMPHIS NEWYORK 8 6 0.1"} | "SANFRAN" | "NEWYORK" | 100 | 10000 |
| Returns: 120.90000000000055 | Here we have the same shipping routes as example 0, but for a more expensive package. Shipping through CHICAGO will have an expected cost of 5 + 239.2 = 244.2, while MEMPHIS will have an expected cost of 11 + 109.9 = 120.9. |
|
| 2) | | | {"SANFRAN CHICAGO 20 3 0.4",
"SANFRAN MEMPHIS 30 5 1.0",
"CHICAGO NEWYORK 15 2 2.0",
"MEMPHIS NEWYORK 8 6 0.1"} | "SANFRAN" | "NEWYORK" | 36 | 10000 |
| Returns: 244.20000000000053 | In this case, the time constraint forces us to send the package along the more expensive route through CHICAGO. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
The spirit of Christmas is most apparent in the exchanging of presents! Santa
Claus would like software to make this unnecessary. He has already decided
which present should be given to each person, but he is wondering if he could make
adjustments.
Specifically he has estimated the intrinsic value of each present to each
person. He wants software to determine if there is a group of people whose
total value would be increased by redistributing the gifts within the group.
Some people might end up with a worse present, but the total value is what
Santa must consider. If such a group exists, Santa would like to know the
size of the smallest such group, and how much the total value of their
presents can be increased by.
The intrinsic value of the presents to each person is given in a String[] value.
Each character in each element of value is an uppercase letter,
with 'A' signifying a value of 1,
'B' a value of 2, etc. The j-th character in the i-th element of value is the
value of the j-th present to the i-th person.
The original intent of Santa was to give the first present to the first person, the second present to the second person, etc.
Create a class SantaClaus that contains a method exchange that is given the String[]
value and returns a int[] containing two elements: the first element is the size of
the smallest improvable group, and the second is the amount by which their total value can
be increased. Report the largest possible increase in value for a group of that
size. If there is no improvable group, return 0 in both elements.
| | Definition | | Class: | SantaClaus | Method: | exchange | Parameters: | String[] | Returns: | int[] | Method signature: | int[] exchange(String[] value) | (be sure your method is public) |
| | | | Constraints | - | value will contain n elements, where n is between 2 and 50 inclusive. | - | Each element of value will contain n characters, each being an uppercase letter 'A'-'Z'. | | Examples | 0) | | | {"ABCDE","ABCDE","ABCDE","ABCDE","ABCDE"} |
| Returns: {0, 0 } |
Each present has the same value to everybody, so there is no way to increase
the total value by reassigning the presents.
|
|
| 1) | | | | Returns: {2, 26 } |
The third person would really like the first present. So we could exchange
presents between the first person and the third person. Previously, the values
were 1 (first person) + 2 (third person) = 3. After the exchange the values
become 3 (first person) + 26 (third person) = 29. So the net gain is 29-3 = 26.
|
|
| 2) | | | {"BCAAA","ADEAA","AAXYA","AAAKL","EAAAD"} |
| Returns: {3, 1 } |
The first, fourth, and fifth persons can exchange presents, giving up values
of 'B', 'K', and 'D' and acquiring values of 'A', 'L', and 'E'. The first
person loses net value of 1, but each of the others gains 1.
|
|
| 3) | | | {"VWAAA","ADEAA","AAXYA","AAAKL","EAAAD"} |
| Returns: {5, 5 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You are planning a business trip in which you need to visit clients in three cities.
The airline charges a separate rate for each individual leg of your journey.
For example, if you are flying from city A to city C, and
you land in city B along the way, then you will pay the fare for a flight
from A to B plus the fare for a flight from B to C.
The airline only sells round-trip tickets, so if you pay for a flight from A to B, then you
get a flight from B to A for free, whether you intend to use it or not. You want to calculate the minimum cost
needed to fly to your three destination cities and return home.
The prices of each possible flight are given as a String[] fares. The i-th element of
fares represents the prices of flights between city i and each of the other cities. In particular, character j of element i represents the price of a flight between city i and city j. Characters with ASCII
values between 49 (the character '1') and 122 (the character 'z') represent costs between $10 and $740, respectively. In particular, a character with ASCII value VAL represents the cost 10*(VAL - 48), measured in dollars. The special character '-' indicates that no flights are available between those two cities. For example, if fares contains the Strings
"-7-"
"7-A"
"-A-"
then it costs $70 to travel between cities 0 and 1, and $170 to travel between cities 1 and 2 ('A' has ASCII value 65). There are no direct flights between cities 0 and 2. fares is always symmetric; the cost to travel from city i to city j is always the same as the cost to travel from city j to city i.
The indices of the cities you intend to visit are given in a int[] destinations, which always contains exactly 3 elements. It is possible that more than one client lives in the same city, so the elements of destinations are not necessarily distinct. You live in city 0, and must begin and end your trip there.
Calculate and return the minimum cost to visit all three clients, or -1 if it is impossible to visit one or more of the clients.
| | Definition | | Class: | Triptych | Method: | minCost | Parameters: | String[], int[] | Returns: | int | Method signature: | int minCost(String[] fares, int[] destinations) | (be sure your method is public) |
| | | | Constraints | - | fares contains between 3 and 50 elements, inclusive. | - | Each element of fares contains exactly N characters, where N is the number of elements in fares. | - | Each character in fares is either '-' or has an ASCII value between 49 ('1') and 122 ('z'), inclusive. | - | The character '\' (ASCII 92) does not appear in fares. | - | Character i of element j of fares is equal to character j of element i, for all indices i and j. | - | Character i of element i of fares is '-', for all indices i. | - | destinations contains exactly 3 elements. | - | Each element of destinations is between 0 and N-1, inclusive. | | Examples | 0) | | | { "-7-",
"7-A",
"-A-" }
| { 1, 2, 1 } |
| Returns: 240 | You fly from city 0 to city 1 for $70, and visit both client 0 and client 2.
Then you fly from city 1 to city 2 for $170, and visit client 1. Finally, you
return home to city 0, with a layover in city 1. |
|
| 1) | | | { "-8--9",
"8---7",
"---5-",
"--5--",
"97---" } | { 1, 3, 4 } |
| Returns: -1 | You can reach cities 1 and 4, but you can't get to city 3. |
|
| 2) | | | { "-5777",
"5-555",
"75-88",
"758-8",
"7588-" } | { 2, 3, 4 } |
| Returns: 200 | You could fly directly to each client's city and return home in between visits for a cost of $210.
However, you can make all the visits for only $200 by flying to city 1 and using that as a hub to fly to the other cities. |
|
| 3) | | | { "-123",
"1-45",
"24-6",
"356-" } | { 0, 0, 0 } |
| Returns: 0 | All the clients live in your own city, so no flights are necessary. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Johnny has to walk home from school, and wants to map out the best route to take, so that he has to cross as few streets as possible.
You are given a String[] map that maps the roadway layout of Johnny's
town. The location of Johnny's school is designated with a 'S' character, and the location of
Johnny's home is designated with a 'H' character. North-South roads are denoted by a '|' character, while East-West roads are denoted by a '-' character.
Intersections, which can never be crossed, are indicated by '*' characters. Fences, indicated by
a 'F' character, can also never be crossed. All other areas are indicated by '.' characters; Johnny can walk freely over these areas.
For maximum safety, Johnny may only walk directly across a road, perpendicular to the traffic, never diagonally. All of Johnny's movements, onto and off of a road, and walking around town, should be in one of the four cardinal directions. Johnny may, however, cross roads that are multiple lanes wide, and doing so only counts as a single crossing. Two or more adjacent || characters are always considered to be a single road, and this works similarly for '-' characters that appear adjacent vertically.
For instance, the following requires only a single crossing, since it's a single two-lane road:
S.||.H
Also, a situation such as the following leaves Johnny with no safe way to walk home, since he cannot cross the road diagonally, and can only step onto and off a road in a direction perpendicular to the road:
S||
||H
Also notice that because Johnny can never move diagonally, in the following case, Johnny cannot get home:
S.F
.F.
F.H
You are to return an int indicating the fewest number of times Johnny must cross
the street on his way home. If there is no safe way for Johnny to get home, return -1. | | Definition | | Class: | WalkingHome | Method: | fewestCrossings | Parameters: | String[] | Returns: | int | Method signature: | int fewestCrossings(String[] map) | (be sure your method is public) |
| | | | Notes | - | If a street is more than one unit wide, it still only counts as a single crossing. | | Constraints | - | map will contain between 1 and 50 elements, inclusive. | - | Each element of map will contain between 1 and 50 characters, inclusive. | - | Each element of map will contain only the characters '.', '-', '|', '*', 'F', 'S', 'H'. | - | There will be exactly one occurrence each of 'S' and 'H' in map. | - | Each element of map will contain the same number of characters. | | Examples | 0) | | | | Returns: 1 | Here, Johnny lives right across the street from the school, so inevitably, he's crossing the street once to get home. |
|
| 1) | | | {"S.|..",
"..|.H",
"..|..",
"....."} |
| Returns: 0 | Similar to above, but since the road has a dead end (maybe even a cul-de-sac at the end), Johnny can get home without actually having to cross the road. |
|
| 2) | | | {"S.||...",
"..||...",
"..||...",
"..||..H"} |
| Returns: 1 | Notice here that even though it's a 2-lane highway, it only counts as a single crossing. |
|
| 3) | | | {"S.....",
"---*--",
"...|..",
"...|.H"} |
| Returns: 1 | Here, Johnny could go down across the street and then right across another street to his house. However, if he first goes to the right before crossing down, he will only cross 1 street. |
|
| 4) | | | {"S.F..",
"..F..",
"--*--",
"..|..",
"..|.H"} |
| Returns: 2 | Similar to above, but because there's a fence around the school, Johnny has no choice but to cross twice. |
|
| 5) | | | {"H|.|.|.|.|.|.|.|.|.|.|.|.|.",
"F|F|F|F|F|F|F|F|F|F|F|F|F|-",
"S|.|.|.|.|.|.|.|.|.|.|.|.|."} |
| Returns: 27 | Poor Johnny lives so close to school, but that fence makes him cross the street quite a bit just to get home. |
|
| 6) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Given the elevations of a plot of land, determine the deepest lake that could exist on the plot. Assume that water will flow off the edge of the plot, and therefore a lake will not cover any land on the border. A lake could form at a location up to level h so long as there is no path of horizontal or vertical steps from the lake to the edge of the plot that is completely below h. For example, consider the following plot:
5255
5225
5525
5515
5555
A lake will form up to level 2 where the 1 is.
You will be given a String[], plot, where the ASCII value of plot[i][j] represents the height of the land at location (i,j). Your task is to determine the deepest lake that could form in the plot, where the depth of a lake is the largest difference between the lake height and the height of the land under the water. | | Definition | | Class: | LakeDepth | Method: | depth | Parameters: | String[] | Returns: | int | Method signature: | int depth(String[] plot) | (be sure your method is public) |
| | | | Notes | - | If no lake can form, return 0. | | Constraints | - | plot will contain between 3 and 50 elements, inclusive. | - | Each element of plot will contain between 3 and 50 characters, inclusive. | - | Each element of plot will be the same length. | - | Each character in plot will have an ASCII value between 32 and 126, inclusive. | | Examples | 0) | | | {"5255",
"5225",
"5525",
"5515",
"5555"} |
| Returns: 1 | This is the example above. |
|
| 1) | | | {"55555",
"59995",
"59595",
"59195",
"59995",
"55555"} |
| Returns: 8 | A lake of height '9' will form in the center of this plot. |
|
| 2) | | | {"55555",
"59995",
"59A95",
"59A95",
"59995",
"55555"} |
| Returns: 0 | No lake may form here, as 'A' has a higher value that '9'. |
|
| 3) | | | {"55555",
"52335",
"53315",
"45555"} |
| Returns: 4 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Given a connected graph with nodes nodes, where each edge is undirected and has both a length and a cost, your task is to pick a subset of the edges such that the graph is still connected, the minimum distance between each pair of nodes is less than or equal to threshold, and the total cost is minimized. You should return this minimum cost. The graph will be given as a String[], g, each element of which represents an edge in the form "u v length cost", where u and v are the zero-based indices of the two nodes connected by this edge. For example, consider the following input:
nodes = 3
threshold = 5
g = {"0 1 4 1","0 2 3 2","1 2 1 4"}
If we select the first and second edges, then the distance between nodes 1 and 2 ends up being 7, greater than our threshold. However, if we pick the first and third edges, the distance between all pairs of nodes is 5 or less, and the cost is minimized (picking the second and third edges would cost more). Thus, we return the cost of these two edges, 5. | | Definition | | Class: | Spanning | Method: | cost | Parameters: | String[], int, int | Returns: | int | Method signature: | int cost(String[] g, int nodes, int threshold) | (be sure your method is public) |
| | | | Constraints | - | g will contain between 1 and 18 elements, inclusive. | - | nodes will be between 2 and 10, inclusive. | - | threshold will be between 1 and 1000, inclusive. | - | Each element of g will be formatted as "u v length cost", where u, v, length and cost are all integers with no extra leading zeros. | - | Each u and v will be between 0 and nodes-1, inclusive. | - | Each length and cost will be between 1 and 100, inclusive. | - | No two elements of g will refer to edges between the same pair of nodes. | - | In each element of g, u will not equal v. | - | If you use all of the edges, the graph will be connected, and the minimum distance between each pair of nodes will be less than or equal to threshold. | | Examples | 0) | | | {"0 1 4 1","0 2 3 2","1 2 1 4"} | 3 | 5 |
| Returns: 5 | |
| 1) | | | {"2 3 7 1",
"3 1 9 1",
"1 0 8 1",
"3 0 1 5",
"1 2 5 7",
"0 2 8 4"} | 4 | 1000 |
| Returns: 3 | With the threshold this high, we just need to find the cheapest way to connect all the nodes. It turns out that the first, second and third edges do this cheapest. |
|
| 2) | | | {"2 3 7 1",
"3 1 9 1",
"1 0 8 1",
"3 0 1 5",
"1 2 5 7",
"0 2 8 4"} | 4 | 10 |
| Returns: 14 | With the same graph, but a lower threshold, the best edges to use are the first, third, fourth, and fifth. |
|
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A "combo box", "choice", or "select box" is a graphical component found in many applications where the current selection is displayed with an arrow on the right side that you can push on to see all the options that can be selected. You probably selected an item from a combo box to open this problem.
A common behavior when you try to use a combo box with your keyboard is that the first element in the combo box that starts with the typed character (case insensitive) after the currently selected item becomes selected. If there are no elements that start with that character after the currently selected item but there is one before it, the first element in the combo box that starts with that character is selected. If no items in the combo box start with that letter, the currently selected element stays selected.
A well-designed combo box will allow you to navigate by keyboard from any selection to any other selection in a minimal number of keystrokes.
You will be given the selectable contents of the combo box as a String[] called elements. You are to write a program to find the maximum number of keystrokes absolutely necessary to get to any element from any element in the combo box. | | Definition | | Class: | ComboBoxKeystrokes | Method: | minimumKeystrokes | Parameters: | String[] | Returns: | int | Method signature: | int minimumKeystrokes(String[] elements) | (be sure your method is public) |
| | | | Notes | - | The elements in elements may not be unique. | - | It takes zero keystrokes to get from an element to itself. | - | The keystrokes made are case-insensitive, meaning that if 'a' is hit, the first 'a' or 'A' after the current selection will be selected, whichever occurs first. | - | In most real applications, you could use the arrow keys as well as the letter keys. However, in this problem, you may only use letter keys. | | Constraints | - | elements will have between 1 and 50 elements, inclusive. | - | There will be between 1 and 50 characters in each element of elements, inclusive. | - | Each element in elements will consist of upper- and lower-case letters and spaces, but will not start with a space. | | Examples | 0) | | | {"alpha","beta","gamma","delta"} |
| Returns: 1 | Since all the elements in the combo box start with different letters, you can get to any element with one keystroke! |
|
| 1) | | | {"kyky","kalinov","kalmakka","Kavan","Kawigi","kaylin","Klinck","krijgertje","kupo"} |
| Returns: 8 | This list of fine coders can only be traversed one at a time, because all elements start with "K". |
|
| 2) | | | {"a","b","c","b","d","b","e","b","f"} |
| Returns: 2 | The b's in this list would be an inconvenience, if you couldn't always instantly skip to the entry before them, making them all no more than 2 keystrokes away. |
|
| 3) | | | {"apple","Boy","CaRD","Dog","egG","FruiT",
"Grape","hand","Ant","eagle","ice cream",
"air","East","Exit","Door","camerA","bad",
"fast","Zealot","internAtional","Bead",
"Bread","Exit","fast", "actiVe","Cats",
"beast","Alligator","drag","castle",
"clean","iLlEgAl","crab","Free Willy",
"first","dean","Fourth of July","King",
"fellow","FrEaK","Elephant","bird","Bible",
"creep","create","Deal","eEl","entrance",
"cream","Fleece"} |
| Returns: 4 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Steganography is a method of cryptography where a message or entire document can be hidden inside of another file or image which shows no evidence that there is data hidden in it. Typically, the message or document to be sent is first encrypted and compressed, and then combined with an existing file in the bits that are less significant.
For example, the encrypted, compressed file could be combined with a bitmap image, and each byte of the file could be encrypted into the lowest two bits of 4 pixel values. The result would be that there is a 1/4 chance that a given pixel is completely unchanged, and a 3/4 chance that it is changed, but by such a small amount that it is essentially undetectable.
While it is not as secure or efficient, you could quite easily encrypt a message into a bitmap image with no encryption or compression, and your message would be protected by the fact that the image has no distinguishable traits that suggest that a message is hidden in it. You will be given an "image" and you will encode a given message into it and return the new image. The returned image should be in the same format as the original image.
The image will be in the format of a String[] where each three digits represent a number from 0 to 255, inclusive (leading zeros will be added as necessary), which is a pixel value in the image. You will also be given a String, message which contains the message you would like to encode into the image. You will first encode the message into numbers representing the characters in the message - spaces will be 0, 'A'-'Z' will be 1-26, 'a'-'z' will be 27-52, '0'-'9' will be 53-62, and 63 will be used for any space after the message. All these numbers can be represented in binary with 6 digits. You will put each pair of bits (representing a number between 0 and 3) into the lowest two bits of the values in the image. For each character, you will put in the lowest two bits, then the middle two, then the highest two, and then continue to the next character. You will put them in the lowest two bits of the first pixel on the first row, then the second pixel on the first row, and so on until you get to the end of the first row, then the first pixel on the second row, and so on. Once you are out of characters, continue substituting the lowest two bits of each pixel value as if the current character were represented by number 63. | | Definition | | Class: | ImageSteganography | Method: | encode | Parameters: | String[], String | Returns: | String[] | Method signature: | String[] encode(String[] image, String message) | (be sure your method is public) |
| | | | Notes | - | The number of pixels in image may not be a multiple of 3, but you should continue inserting the message as if there was room to finish the last character. | | Constraints | - | image will have between 1 and 50 elements, inclusive. | - | The number of characters in each element of image will be a multiple of 3 between 3 and 48, inclusive. | - | message will have between 1 and 50 characters, inclusive. | - | There will be enough room in image to encode message. | - | Each element of image will be made up of 3-digit numbers between 0 and 255, inclusive, padded with zeros if necessary. | - | The characters in message will be spaces, numbers, and upper- and lower-case letters. | | Examples | 0) | | | {"255123212001201222"} | "hi" |
| Returns: { "254120214003200222" } | This single-pixel-thick image is still big enough to encode this message. |
|
| 1) | | | {"255123212","001201222"} | "hi" |
| Returns: { "254120214", "003200222" } | Same message, but with the second letter on a different row. |
|
| 2) | | | {"123234213001023213123145",
"222111121101213198009",
"121122123124125",
"132212093039",
"213110"} | "Hello 1" |
| Returns:
{ "120234212003023213122145",
"222110121102213198010",
"120120120126125",
"135215095039",
"215111" } | Notice that the image doesn't strictly need to be rectangular, letters from the message might need to wrap to multiple lines, and the whole image may not have a multiple of 3 "pixels". |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Your friend was born on "leap day" and has always been troubled by the fact that he just turned 24 this year on his 5th birthday. You would like to write him a nice program for his birthday to help him and other leap-day-born people keep track of how many birthdays they've had so far.
You will be given two years as ints, year represents the current year and born represents the year the person was born. You are to return the number of leap days that have occurred in that time span, not including the first year if it is a leap year, but including the current year if it's a leap year.
A leap year is any year that is a multiple of 4, unless it is divisible by 100 and not 400. For example, 1984 was a leap year, but 1900 wasn't, because it was divisible by 100 and not 400. 2000 was a leap year, because it was divisible by both 100 and 400. | | Definition | | Class: | LeapAge | Method: | getAge | Parameters: | int, int | Returns: | int | Method signature: | int getAge(int year, int born) | (be sure your method is public) |
| | | | Notes | - | It is possible that year and born are not actually leap years. | | Constraints | - | year and born will both be between 1582 and 10000 (1582 is when the Gregorian Calendar started, so there were no leap years before then). | - | year will be greater than born. | | Examples | 0) | | | | Returns: 6 | This is the case in the first paragraph of the problem statement. |
|
| 1) | | | | Returns: 2042 | This is about as old as you get in this problem. Note that while the Gregorian Calendar (and therefore, leap years) started in 1582, 1582 wasn't a leap year. |
|
| 2) | | | | Returns: 6 | You really don't know why your lying friend asked you to write this... |
|
| 3) | | | | 4) | | | | 5) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | As the manager of a large company, you have a number of factories, and a number of retail stores. Each factory has a location given as (x,y), and some output capacity. Each store also has a location given as (x,y), and a demand specifying the number of products it must receive. Products are produced at the factories, and sent to the stores, and it is important that the stores receive the number of products they ask for. Recently, the factories have not been able to keep up with demand, so you are planning to build a new one. However, you are not sure where the best place to build it is. There is a constant cost of shipping per product, per unit of distance, which we'll call C. Hence, shipping 3 products from (0,0) to (3,4) costs 3*sqrt(3*3+4*4)*C = 15*C. Your new factory will have an output capacity exactly equal to the current difference between the sum of the capacities of all the factories, and the sum of the demands of all the stores. Your task is, assuming an optimal shipping plan, determine the best location to place the new factory.
A String[], factories, will specify the location and capacity of each factory in the format "X Y CAPACITY", where X, Y and CAPACITY are integers. Another String[], stores, will specify the locations and demands of the stores in the format "X Y DEMAND", where X, Y and DEMAND are integers. You should return the optimal factory location as a int[] with two elements, x and y, in that order. | | Definition | | Class: | Supply | Method: | newFactory | Parameters: | String[], String[] | Returns: | int[] | Method signature: | int[] newFactory(String[] factories, String[] stores) | (be sure your method is public) |
| | | | Notes | - | The location of the new factory must have integral coordinates. | - | There may be multiple factories or stores at the same location. | - | The constraints ensure that the return is unique. | | Constraints | - | stores will contain between 1 and 10 elements, inclusive. | - | factories will contain between 1 and 4 elements, inclusive. | - | Each X and Y in stores and factories will be between 0 and 10, inclusive. | - | Each CAPACITY and DEMAND will be between 1 and 10, inclusive. | - | There will be no extraneous leading zeros or extra spaces in either input. | - | The total DEMAND will exceed the total CAPACITY. | - | The location providing the minimum cost will be unique, and there will be no other locations that have costs within 1E-3*C of that minimum. | | Examples | 0) | | | {"4 0 10"} | {"5 0 7","10 0 8"} |
| Returns: { 10, 0 } | There are two stores, with demands of 7 and 8. The single existing factory has a capacity of 10, so our new factory must have a capacity of 5. The best place to put the factory is (10,0), the same spot as the store. Then, we have to ship 3 units from the existing factory to (10,0), and 7 units to (5,0). Thus, our total cost is 7*1*C + 3*6*C = 25*C. |
|
| 1) | | | {"0 0 1"} | {"5 5 7","10 10 8","10 0 5"} |
| Returns: { 7, 6 } | Notice that the optimal factory placement is not always at the same location as a store. The total cost here is about 92.485*C. |
|
| 2) | | | {"0 0 10","0 10 10","10 0 10","10 10 10"} | {"0 0 8",
"1 1 8",
"2 2 4",
"3 3 9",
"4 4 9",
"5 5 8",
"6 6 1",
"7 7 5",
"8 8 2",
"9 9 10"} |
| Returns: { 3, 3 } | |
| 3) | | | {"3 3 10","10 7 7"}
| {"6 2 10","2 6 2","7 1 9"} |
| Returns: { 7, 1 } | |
| 4) | | | {"2 8 10","0 1 9","6 4 10","4 8 10"}
| {"8 10 9","8 5 2","5 4 7","6 6 7","9 10 3",
"6 8 7","5 2 6","6 9 2","6 3 9","10 10 9"}
|
| Returns: { 9, 10 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You have collected a number of data points, each consisting of an ordered triple of three integers. Unfortunately, you've lost the original data. However, before you lost the data, you calculated the Euclidean distances between each pair of points and recorded the square of each distance. Given the distances as a String[], dists, you are to find the original data points, if possible. Each element of dists will be formatted as a single space delimited list of integers. The ith integer of the jth element of dists will represent the square of the distance between the ith and jth points.
You should return a String[], the ith element of which represents the the ith data point as 3 single space delimited integers. Since distances are preserved under translation and rotation, the first element of the return should always be "0 0 0", and the second element should consist of 3 non-negative integers sorted in non-descending order. If there are multiple such returns, pick the one that is lexicographically first. For the purposes of this problem, one return is before another lexicographically if it has a lower integer in the first location for which the two differ. In other words, find the first element of the return that is different between the two, and then find the first integer in the two elements that differ, and compare them. If there is no set of points that could generate the given distances, return an empty String[]. | | Definition | | Class: | Reconstruct | Method: | findPoints | Parameters: | String[] | Returns: | String[] | Method signature: | String[] findPoints(String[] dists) | (be sure your method is public) |
| | | | Constraints | - | dists will contain between 1 and 20 elements, inclusive. | - | Each element of dists will contain between 1 and 50 characters, inclusive. | - | Each number in dists will be an integer between 0 and 1000, inclusive, with no extra leading zeros. | - | Each element of dists will contain the same number of integers as there are elements of dists, separated by single spaces. | - | In dists, integer i of element j will equal integer j of element i. | - | In dists, integer i of element i will equal 0. | | Examples | 0) | | | | Returns: { "0 0 0", "0 0 1" } | |
| 1) | | | {"0 2 2","2 0 2","2 2 0"} |
| Returns: { "0 0 0", "0 1 1", "-1 0 1" } | |
| 2) | | | {"0 33 25","33 0 84","25 84 0"} |
| Returns: { "0 0 0", "1 4 4", "3 -4 0" } | |
| 3) | | | | Returns: { } | There are no three integers the sum of whose squares is 15. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Given a String s, we will first collect all distinct positive length subsequences of s, and place them in a list L. A subsequence of s is obtained by deleting 0 or more characters. Next, sort L into ascending order by length. Where ties occur, break them lexicographically. Here uppercase letters occur before lowercase letters. Finally, return the String in position pos % k (0-based), where k is the number of elements in L, and % is the modulus operator. | | Definition | | Class: | GetSubsequence | Method: | getAt | Parameters: | String, String | Returns: | String | Method signature: | String getAt(String s, String pos) | (be sure your method is public) |
| | | | Constraints | - | s will contain between 2 and 50 characters inclusive. | - | Each character in s will be a letter ('A'-'Z','a'-'z'). | - | pos will be an integral value between 0 and 2^63 - 1 inclusive. | - | pos will not have extra leading zeros. | | Examples | 0) | | | | Returns: "ABCD" | "ABCD" is the last of the 15 subsequences in L. |
|
| 1) | | | | Returns: "ABD" | 19203410239121 % 15 = 11. |
|
| 2) | | | | 3) | | | "AbcdAbcdAbcd" | "39283423984923" |
| Returns: "AbAbdbd" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | In a Context-Free Grammar, strings are transformed into other strings during a process called a derivation. In our notation, one step of a derivation occurs when an uppercase letter ('A'-'Z') is turned into a string (possibly empty) of uppercase and lowercase letters ('A'-'Z', 'a'-'z'). For example,
ZaBcD -> ZagHgcD -> ZagHgcF -> ZaggcF -> Zaggcq
is a derivation transforming ZaBcD into Zaggcq. A derivation is called leftmost if every derivation step replaces the leftmost uppercase letter. Assuming all valid derivation steps are at your disposal, return the leftmost derivation with the smallest number of steps transforming start into finish. Since all possible derivation rules can be used, during each step, choose the leftmost capital letter, and change it to whichever string you want. If there are multiple possible smallest leftmost derivations, return one that comes first lexicographically. Here smallest is measured by the number of steps. To compare two derivations lexicographically, concatenate all of their intermediate strings, and then compare them. As in ASCII, uppercase letters occur lexicographically before lowercase letters. The returned value should be a String[] containing the sequence of intermediate strings, in the order they are created. Element 0 must be start, and the last element must be finish (see the examples for further clarification). If no leftmost derivation is possible, return an empty String[]. | | Definition | | Class: | LeftmostDerivation | Method: | getDeriv | Parameters: | String, String | Returns: | String[] | Method signature: | String[] getDeriv(String start, String finish) | (be sure your method is public) |
| | | | Constraints | - | start will contain between 1 and 50 characters inclusive. | - | Each character in start will be an uppercase or lowercase letter ('A'-'Z','a'-'z'). | - | finish will contain between 1 and 50 characters inclusive. | - | Each character in finish will be an uppercase or lowercase letter ('A'-'Z','a'-'z'). | - | start and finish will be distinct. | | Examples | 0) | | | | Returns: { } | Although a derivation was shown in the problem statement, there is no leftmost derivation that works. |
|
| 1) | | | | Returns: { "AH", "ABCDEFGH" } | Here we replace A with ABCDEFG. |
|
| 2) | | | | Returns: { "ABC", "BC", "C", "abc" } | There are numerous leftmost derivations that take 3 steps, so return the one that occurs first lexicographically. |
|
| 3) | | | | Returns: { "AaA", "aA", "aAaa" } | Firstly, we delete the leftmost A by replacing it with the empty string. Next we replace the remaining A with Aaa. |
|
| 4) | | | "AaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa" | "aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa" |
| Returns:
{ "AaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaaaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaaaaAaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaaaaaAaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaaaaaaAaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaaaaaaaAaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaaaaaaaaAaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaaaaaaaaaAaAaAaAaAaAaAaAaAaAa",
"aaaaaaaaaaaaaaaAaAaAaAaAaAaAaAaAa",
"aaaaaaaaaaaaaaaaAaAaAaAaAaAaAaAa",
"aaaaaaaaaaaaaaaaaAaAaAaAaAaAaAa",
"aaaaaaaaaaaaaaaaaaAaAaAaAaAaAa",
"aaaaaaaaaaaaaaaaaaaAaAaAaAaAa",
"aaaaaaaaaaaaaaaaaaaaAaAaAaAa",
"aaaaaaaaaaaaaaaaaaaaaAaAaAa",
"aaaaaaaaaaaaaaaaaaaaaaAaAa",
"aaaaaaaaaaaaaaaaaaaaaaaAa",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
XML documents are widely used today to describe many different kinds
of data. The primary purpose of XML is to allow people to share
textual and numerical data in a structured format across the Internet.
Note that the XML document format described in this problem is a simplified version
of the actual XML format.
An XML document contains tags and plain text. Tags are used to define blocks within
the XML document. A block always begins with a start-tag and ends with a
corresponding end-tag. The format of these tags are <tag-name>
and </tag-name>, respectively.
The tag-name for an end-tag is the same (including case) as the start-tag
for the block it closes. All plain text data must be inside at least one block.
The plain text will not contain the characters '<', '>' and '/'.
Blocks may be nested, but cannot overlap. So for instance,
"<root><data>Hello world</data></root>"
and
"<root>Hello</root><root>world</root>"
are valid XML documents, while
"<root><data>Hello world</root></data>"
and
"<root>Hello</root> <root>world</root>"
are not; the first one has overlapping blocks (the tag <data> must end
before the outer tag <root> ends), the second has text - a space - outside
all blocks (in this problem, spaces are treated just like any other character, see example 2).
Your task is to write a program which formats an XML document, according to the following
rules: If a block contains other blocks, the start- and end-tags for that block should
be on lines by themselves, and all tags and text inside this block should be indented
by 3 spaces per open tag. Otherwise the start- and end-tags should be on the same line, with the textual
content of the block (if any) between the tags (and nothing else, except indentation,
may appear on this line). See example 0 for clarifications.
Create a class BadXML containing the method
format which takes a String[] doc, the XML document,
and returns a String[] containing the properly indented XML document.
Concatenate the elements in doc to get the full XML document.
| | Definition | | Class: | BadXML | Method: | format | Parameters: | String[] | Returns: | String[] | Method signature: | String[] format(String[] doc) | (be sure your method is public) |
| | | | Notes | - | Spaces are treated like any other character, see example 2. | | Constraints | - | doc will contain between 1 and 50 elements, inclusive. | - | Each element in doc will contain between 0 and 50 characters, inclusive. | - | The characters in the elements of doc will have ASCII values between 32 and 126, inclusive. | - | The characters '<', '>' and '/' will only be used in tags. | - | A tag-name will not contain any of the characters '<', '>', '/' or space. | - | A tag-name will contain at least one character. | - | doc will describe a valid XML document according to the description above, and will contain at least one block. | - | The return value will contain at most 100 elements, and no element will contain more than 80 characters. | | Examples | 0) | | | {"<article>",
"<author>writer</author>",
"<headline>TopCoder",
" ",
"Open 2004</headline>",
"<ingress>",
"</ingress>",
"<paragraph>",
"TopCoder Open is being held at <st:hotel>",
"Santa Clara Marriott</st:hotel>",
"which lies in the northern part of ",
"<st:state>California</st:state>.",
"</paragraph>",
"<paragraph>",
"&lbr;Image&rbr;",
"</paragraph>",
"</article>"}
|
| Returns:
{ "<article>",
" <author>writer</author>",
" <headline>TopCoder Open 2004</headline>",
" <ingress></ingress>",
" <paragraph>",
" TopCoder Open is being held at ",
" <st:hotel>Santa Clara Marriott</st:hotel>",
" which lies in the northern part of ",
" <st:state>California</st:state>",
" .",
" </paragraph>",
" <paragraph>&lbr;Image&rbr;</paragraph>",
"</article>" } | The block surrounded by the paragraph tags contain two nested blocks as well as three plain text strings. All these end up on separate lines. The other plain text strings end up on the same line as the tags in the blocks they are surrounded by. |
|
| 1) | | | {"<ro","ot>A roo","","t node</r","oot><","root>Anot","her root node</ro","ot>",""} |
| Returns: { "<root>A root node</root>", "<root>Another root node</root>" } | An XML document may contain several blocks at the top level. |
|
| 2) | | | {"<outer_tag>",
" <inner_tag>",
" Some text",
" </inner_tag>",
"</outer_tag>"}
|
| Returns:
{ "<outer_tag>",
" ",
" <inner_tag> Some text </inner_tag>",
"</outer_tag>" } | The indentation in the input is treated as space characters and is not removed.
|
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Note: This problem statement includes images that may not appear
if you are using a plugin. For best results, use the Arena editor.
In one mode of the grafix software package, the user blocks off portions
of a masking layer using opaque rectangles. The bitmap used as the masking
layer is 400 pixels tall and 600 pixels wide. Once the rectangles have
been blocked off, the user can perform painting actions through the
remaining areas of the masking layer, known as holes. To be
precise, each hole is a maximal collection of contiguous pixels that
are not covered by any of the opaque rectangles. Two pixels are contiguous
if they share an edge, and contiguity is transitive.
You are given a String[] named rectangles, the
elements of which specify the rectangles that have been blocked off
in the masking layer. Each String in rectangles consists of four
integers separated by single spaces, with no additional spaces in the
string. The first two integers are the window coordinates of the
top left pixel in the given rectangle, and the last two integers are
the window coordinates of its bottom right pixel. The window coordinates
of a pixel are a pair of integers specifying the row number and column
number of the pixel, in that order. Rows are numbered from top to bottom,
starting with 0 and ending with 399. Columns are numbered from left to
right, starting with 0 and ending with 599.
Every pixel within and along the border of the rectangle defined by these
opposing corners is blocked off.
Return a int[] containing the area, in pixels, of every
hole in the resulting masking area, sorted from smallest area to greatest.
| | Definition | | Class: | grafixMask | Method: | sortedAreas | Parameters: | String[] | Returns: | int[] | Method signature: | int[] sortedAreas(String[] rectangles) | (be sure your method is public) |
| | | | Notes | - | Window coordinates are not the same as Cartesian coordinates. Follow the definition given in the second paragraph of the problem statement. | | Constraints | - | rectangles contains between 1 and 50 elements, inclusive | - | each element of rectangles has the form "ROW COL ROW COL", where: "ROW" is a placeholder for a non-zero-padded integer between 0 and 399, inclusive; "COL" is a placeholder for a non-zero-padded integer between 0 and 599, inclusive; the first row number is no greater than the second row number; the first column number is no greater than the second column number | | Examples | 0) | | | | Returns: { 116800, 116800 } | The masking layer is depicted below in a 1:4 scale diagram.
|
|
| 1) | | | {"48 192 351 207", "48 392 351 407", "120 52 135 547", "260 52 275 547"} |
| Returns: { 22816, 192608 } | |
| 2) | | | {"0 192 399 207", "0 392 399 407", "120 0 135 599", "260 0 275 599"} |
| Returns: { 22080, 22816, 22816, 23040, 23040, 23808, 23808, 23808, 23808 } | |
| 3) | | | {"50 300 199 300", "201 300 350 300", "200 50 200 299", "200 301 200 550"} |
| Returns: { 1, 239199 } | |
| 4) | | | {"0 20 399 20", "0 44 399 44", "0 68 399 68", "0 92 399 92",
"0 116 399 116", "0 140 399 140", "0 164 399 164", "0 188 399 188",
"0 212 399 212", "0 236 399 236", "0 260 399 260", "0 284 399 284",
"0 308 399 308", "0 332 399 332", "0 356 399 356", "0 380 399 380",
"0 404 399 404", "0 428 399 428", "0 452 399 452", "0 476 399 476",
"0 500 399 500", "0 524 399 524", "0 548 399 548", "0 572 399 572",
"0 596 399 596", "5 0 5 599", "21 0 21 599", "37 0 37 599",
"53 0 53 599", "69 0 69 599", "85 0 85 599", "101 0 101 599",
"117 0 117 599", "133 0 133 599", "149 0 149 599", "165 0 165 599",
"181 0 181 599", "197 0 197 599", "213 0 213 599", "229 0 229 599",
"245 0 245 599", "261 0 261 599", "277 0 277 599", "293 0 293 599",
"309 0 309 599", "325 0 325 599", "341 0 341 599", "357 0 357 599",
"373 0 373 599", "389 0 389 599"} |
| Returns:
{ 15, 30, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 45, 100, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 115, 200, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 230, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 300, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345, 345 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Note: This problem statement includes an image that may
not appear if you are using a plugin. For best results, use the Arena
editor.
You have been hired to work on a graphics application called grafix. The
interface includes several buttons that the user can click on during
a session. One of these is the Save button, which appears in the
application window as a rectangle composed of pixels. The location of
each pixel is given by a pair of integers that specify the pixel's row
number and column number, in that order. Such an integer pair is called
the window coordinates of a pixel. Rows are numbered from top to
bottom, and columns are numbered from left to right.
The top left corner of the Save button has window coordinates (20, 50),
meaning that it is a pixel occupying the 20th row from the top and the
50th column from the left. The bottom right corner of the Save button
has window coordinates (39, 99). If the user clicks her mouse on any
pixel within or on the border of the rectangle defined by these points,
she is considered to have activated the Save button. Below is a magnified
image of the Save button, showing the numbers of its rows and columns.
Given a sequence of mouse clicks, your job is to determine which ones have
activated the Save button. For each mouse click, the int[]
mouseRows contains the row number of its window coordinates,
while the int[] mouseCols contains its column
number. The values in each int[] are in corresponding order,
so the nth element of mouseRows and the nth element
of mouseCols make up the window coordinates of the nth
mouse click. You are to return a int[] containing, in ascending
order, the zero-based index of each mouse click that activates the
Save button.
| | Definition | | Class: | grafixClick | Method: | checkSaveButton | Parameters: | int[], int[] | Returns: | int[] | Method signature: | int[] checkSaveButton(int[] mouseRows, int[] mouseCols) | (be sure your method is public) |
| | | | Notes | - | Window coordinates are not the same as Cartesian coordinates. Follow the definition given in the first paragraph of the problem statement. | | Constraints | - | mouseRows contains between 1 and 50 elements, inclusive | - | mouseRows and mouseCols each contain the same number of elements | - | each element in mouseRows is between 0 and 399, inclusive | - | each element in mouseCols is between 0 and 599, inclusive | | Examples | 0) | | | {20, 39, 100} | {99, 50, 200} |
| Returns: { 0, 1 } | The first click, with window coordinates (20, 99), falls on the top right corner of the Save button. The second click is on the bottom left corner at (39, 50). The third click has window coordinates (100, 200) and falls outside the button. |
|
| 1) | | | {0, 100, 399} | {0, 200, 599} |
| Returns: { } | None of these clicks activate the Save button. |
|
| 2) | | | | 3) | | | {10, 20, 30, 30, 30, 30, 34, 35, 345} | {10, 20, 30, 50, 60, 80, 34, 35, 345} |
| Returns: { 3, 4, 5 } | |
| 4) | | | {57, 28, 18, 25, 36, 12, 33, 44, 13, 32,
32, 51, 11, 27, 8, 51, 17, 34, 10, 16,
47, 57, 20, 57, 32, 14, 13, 37, 10, 16,
49, 37, 52, 8, 18, 44, 50, 43, 11, 1,
21, 22, 17, 35, 28, 53, 56, 16, 11, 44} | {146, 22, 41, 88, 123, 99, 43, 110, 25, 64,
141, 110, 70, 34, 99, 103, 60, 64, 142, 109,
133, 144, 72, 68, 25, 32, 21, 140, 30, 105,
32, 72, 114, 97, 35, 131, 103, 110, 133, 81,
125, 36, 76, 78, 77, 47, 50, 94, 22, 20} |
| Returns: { 3, 9, 17, 22, 31, 43, 44 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are working on the backend of a system that allows people to upload files. For security reasons, you want to restrict the file extensions of the files they upload. You will be given a String[], extensions, containing a list of allowed extensions. You will also be given a String[], files, containing the names of a number of files. Your task is to return a String[], each element of which is either "ALLOW" or "DENY", corresponding to the elements of files with the same indices. If a filename ends with a period ('.') followed by an allowed extension, ignoring case, then it is to be allowed, otherwise it should be denied. | | Definition | | Class: | FileFilter | Method: | filter | Parameters: | String[], String[] | Returns: | String[] | Method signature: | String[] filter(String[] files, String[] extensions) | (be sure your method is public) |
| | | | Constraints | - | files will contain between 0 and 50 elements, inclusive. | - | extensions will contain between 0 and 50 elements, inclusive. | - | Each element of files will contain between 2 and 50 letters ('a'-'z' and 'A'-'Z') and periods. | - | The last character of each element of files will be a letter. | - | Each element of files will contain at least one period. | - | Each element of extensions will contain between 1 and 50 lowercase letters ('a'-'z'). | | Examples | 0) | | | {"algorithm.txt","algorithms.html","wingl.exe","yourDoc.PIF","graph.gIf"} | {"txt","html","gif","doc"} |
| Returns: { "ALLOW", "ALLOW", "DENY", "DENY", "ALLOW" } | |
| 1) | | | | 2) | | | {".bashrc","bash.rc"} | {"rc"} |
| Returns: { "DENY", "ALLOW" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | In genetics, most large animals have two copies of every gene - one from each
parent. In the simplest genetic model, each of the genes takes on one of two
forms, dominant or recessive, usually represented by an uppercase
and lowercase letter of the same value, respectively ('A' and 'a', for example).
The pair of genes typically contributes to the external qualities of the animal
in one of two ways. If one or two genes are uppercase, the dominant gene is
said to be expressed. If both genes are lowercase, then the recessive
gene is said to be expressed.
Additionally, there may be some gene dependencies. If one pair of genes is
dependent on another pair of genes, then the first gene may only be expressed
dominantly if the gene it depends on is also expressed dominantly. These
dependencies are indicated by a int[], dependencies. If
element i of dependencies is j, it means that gene i can only be
expressed dominantly if gene j also is (both i and j are indexed from 0). If gene j is expressed recessively,
then gene i must be also, regardless of the actual genes at position i. If an
element of dependencies is -1, it means that the gene is not dependent on
any other gene, and is expressed as described in the first paragraph. Chains
may exist within dependencies where i depends on j, which in turn depends
on k. However, a gene may not depend on itself, and there will be no looping
dependencies.
In this problem you are to predict a certain quality about an animal, given N
pairs of genes from each of its parents, and some information about those N
genes. The first parent's two copies of the genes are given by p1a and p1b,
while the second parent's are given by p2a and p2b. For each of the N genes, each parent contributes one of its two genes to its children (characters with the same indices in p1a, p1b, p2a, and p2b correspond to the same gene). For example, if p1a = "ABC" and p1b = "abc", the first parent would contribute an 'A' or an 'a' to its child's first pair of genes, a 'B' or a 'b' to its child's second pair and so on. Similarly, the second parent would also contribute a single gene to its child's first pair of genes, one to its second pair and so on.
Thus, the offspring end up with N genes from each parent. Each pair of
corresponding genes contributes in some way to a certain quality we are
interested in. If the ith pair of genes is expressed dominantly, we
add dom[i] to a running sum (which is initialized to 0), otherwise we add
rec[i]. Given that each parent contributes one of its two genes entirely
at random (with a 50% chance of either), you are to determine the expected value
the offspring will have for the quality we are interested in (the running sum), and return that
value.
| | Definition | | Class: | GeneticCrossover | Method: | cross | Parameters: | String, String, String, String, int[], int[], int[] | Returns: | double | Method signature: | double cross(String p1a, String p1b, String p2a, String p2b, int[] dom, int[] rec, int[] dependencies) | (be sure your method is public) |
| | | | Notes | - | Your return must have a relative or absolute error less than 1e-9. | | Constraints | - | Let N be the number of genes, where N is between 1 and 50, inclusive. | - | p1a, p1b, p2a and p2b will each contain exactly N characters. | - | dom, rec, and dependencies will each contain exactly N elements. | - | Corresponding characters of p1a, p1b, p2a and p2b will have the same value (but may be uppercase or lowercase). | - | Each element of dependencies will be between -1 and N-1, inclusive. | - | dependencies will not contain any cycles. | - | Each element of dom and rec will be between -100 and 100, inclusive. | | Examples | 0) | | | "AaaAA" | "AaaAA" | "AaaAA" | "AaaAA" | {1,2,3,4,5} | {-1,-2,-3,-4,-5} | {-1,-1,-1,-1,1} |
| Returns: -5.0 | Since every copy is the same, the animal is guaranteed to have two pairs of "AaaAA". This means that the first and fourth genes will be dominantly expressed, while the others will be recessively expressed. Note that the last gene is dependent on gene 1, which is recessively expressed. |
|
| 1) | | | "AbegG" | "ABEgG" | "aBEgg" | "abegG" | {5,5,5,5,5} | {1,1,1,1,1} | {-1,0,1,2,3} |
| Returns: 14.25 | Here, we have a chain of dependencies, where gene 1 depends on gene 0, 2 depends on 1, and so on. For the first
gene, the animal is guaranteed to get an 'A' from its first parent, and an 'a' from its second parent. This means
that gene 0 will be expressed dominantly, contributing 5 to the sum. The next gene has a 75% chance of being
expressed dominantly (it will only be expressed recessively if a 'b' comes from each parent). The third gene also
has a 75% chance of being expressed dominantly, assuming that the second gene was expressed dominantly. The fourth
gene is guaranteed to be expressed recessively, and hence so is the final gene.
This gives us 3 possible scenarios, denoted below, where a 'D' indicates a gene is expressed dominantly, and an 'R'
means recessively:
Genes | Sum | Prob
------+-----+-------
DDDRR | 17 | 0.5625
DDRRR | 13 | 0.1875
DRRRR | 9 | 0.25
The expected value is thus 17*0.5625+13*0.1875+9*.25 = 14.25 |
|
| 2) | | | "XyMpdnVsbinDvqBpcWGDLfsmQtZpeirvTmoRmBASfqqrFS" | "xYmpdnVsBINdvQBPCwgDlFSmQTzpEIrVtmoRmbaSfqQRfS" | "XYMpdnvsBINdVQbpCWgDlfSMqTzPeIrVTMOrmbaSfQqrFs" | "XYMpDnVSBIndvQBPCWGDlFsMqtzpEiRVTMORMBASFqQrfS" | {-82,-35,-51,52,87,25,8,27,-12,-10,-63,-36,-95,-35,-98,95,-76,7,36,-35,92,23,-94,
-31,-30,36,51,-49,-19,-3,19,32,58,82,-36,-87,-54,-17,-40,32,-91,-56,0,-16,70,42} | {-36,77,90,50,83,66,-94,-66,-22,-83,-86,-89,-55,-3,-51,18,-41,-91,91,32,-25,-60,
5,79,100,85,60,98,55,95,-67,-46,-26,48,-64,16,-36,-95,-54,19,96,79,78,-91,-12,35} | {-1,-1,1,-1,3,-1,-1,1,3,5,4,0,-1,-1,2,8,5,4,-1,10,11,14,3,-1,
15,-1,7,-1,8,-1,-1,15,-1,-1,30,-1,26,26,-1,-1,-1,-1,-1,31,0,3} |
| Returns: -356.875 | |
| 3) | | | "fOai" | "Foai" | "fOAI" | "FOAi" | {96,21,-34,-81} | {77,-2,20,25} | {-1,0,-1,-1} |
| Returns: 44.5 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are responsible for fabricating a circuit board for an engineering firm. The circuit consists of pinholes (where pins of various components will be inserted) connected by metal traces. For this problem, assume that pinholes can be treated as points in a plane and metal traces can be treated as straight line segments connecting two pinholes.
A highly simplified description of the fabrication process follows. The circuit pattern is cut out of a sheet of opaque material, forming a mask. A sheet of metal is covered with a thin film of material, called negative photoresist, that hardens when exposed to ultraviolet light. The mask is placed over the photoresist and illuminated with ultraviolet light, hardening the photoresist where the light passes through the mask. Finally, the metal sheet is bathed in an etching solution, which destroys the metal except where it is protected by hardened photoresist. The remaining metal forms the circuit.
After a long period of carefully laying out the circuit board, you have the mask prepared. Just before you illuminate the photoresist with ultraviolet light, your boss rushes in and gives you some last minute requirements: the sum of the lengths of the shortest metal trace paths between each pair of pinholes must be under a certain limit, and the board has to be ready by tomorrow. In other words, if you find the shorest path between every pair of connected pins, the sum of the lengths of all these shortest paths must be under the limit. There is no way you can redesign everything in one day, so you decide to improvise. By tilting the mask around its x-axis before you illuminate the photoresist, the circuit will be shrunk along the y-direction; only the projection of the mask on the plane of the photoresist is important since you are shining light through it (assume the light rays are parallel to the z-axis and ignore diffraction effects). Since this also moves the locations of the pins, you want to tilt the mask as little as possible.
Write a class NegativePhotoresist with a method minimumTilt that takes a String[] pinConnections specifying for each pinhole its mask-plane coordinates and its connections to other pinholes and an int limit, and returns as a double the minimum non-negative angle (in radians) the mask must be rotated around the x-axis such that the sum of the lengths of the shortest paths between each pair of pinholes is less than limit. If there is no path between a pair of pinholes, omit that pair from the sum.
Each element of pinConnections corresponds to one pinhole. Element i will begin with a pair of integers specifying the x and y coordinates of pinhole i separated by a single comma. Following this is a space-separated list of zero or more integers specifying the zero-based indices of the pinholes that i connects to. All integers are non-negative and may have leading zeroes. For this problem, pinholes are directly connected if and only if they are specified to connect in pinConnections. In other words, overlapping or coinciding metal traces do not create a connection. | | Definition | | Class: | NegativePhotoresist | Method: | minimumTilt | Parameters: | String[], int | Returns: | double | Method signature: | double minimumTilt(String[] pinConnections, int limit) | (be sure your method is public) |
| | | | Notes | - | A return value with either an absolute or relative error of less than 1e-9 radians is considered correct. | - | There can only be up to one connection between a pair of pinholes. If an index j appears multiple times in element i of pinConnections, there is still only one metal trace between pinholes i and j. | - | The sum over all pairs of the shortest path lengths will be minimized when the mask is rotated by Pi / 2 radians. | - | Pairs are unordered. The pair (pinhole i, pinhole j) is the same as (pinhole j, pinhole i). | | Constraints | - | The correct answer will be between 0.01 and 1.55, inclusive. | - | pinConnections will contain between 2 and 50 elements, inclusive. | - | Elements of pinConnections will contain at most 50 characters. | - | Elements of pinConnections will be formatted "X,Y P1 P2 P3 ..." (quotes are for clarity) where X and Y are between 0 and 100000 inclusive, and each of the indices Pj will be separated by a single space and will be between 0 inclusive and the number of elements in pinConnections exclusive. | - | If pinhole i connects to pinhole j, pinhole j will also connect to pinhole i. | - | A pinhole will not connect to itself. | - | limit will be between 1 and 1,000,000,000, inclusive. | | Examples | 0) | | | {"3,0 2 3",
"13,0 2 3",
"0,2 0 1",
"8,50 0 1",
"3,100000 5",
"100000,3 4",
"8432,221"} | 100835 |
| Returns: 1.4454078077996135 | Pinholes 0, 2, 1, and 3 form a cycle (in that order). When the mask is laid flat, the shortest path between pinholes 0 and 1 goes through pinhole 2. However, if the mask is tilted by more than 1.4454039026103331 radians, the shortest path between pinholes 0 and 1 switches to go through pinhole 3. The shortest path between pinholes 2 and 3 always goes through pinhole 0, regardless of the angle. Pinholes 4 and 5 form a separate component that contains only one connection. Pinhole 6 is not connected to anything, and therefore does not influence the total path length. |
|
| 1) | | | {"3,0 2 3",
"13,0 2 3",
"0,2 0 1",
"8,50 0 1",
"3,100000 5",
"100000,3 4",
"8432,221"} | 141601 |
| Returns: 0.010604469396671205 | This is the same mask as in Example 0. It only has to be tilted slightly to achieve a total shortest path length of 141601. |
|
| 2) | | | {"3,0 2 3",
"13,0 2 3",
"0,2 0 1",
"8,50 0 1",
"3,100000 5",
"100000,3 4",
"8432,221"} | 100065 |
| Returns: 1.5491503047781332 | This is the same mask as in Example 0. It has to be tilted until it is almost standing on its edge to achieve a total shortest path length of 100065. |
|
| 3) | | | {"76492,66181 1",
"74004,26736 0 14",
"22967,14922",
"5781,62226",
"82836,93961 23",
"87218,72769 19",
"93356,54263 21 23",
"31487,92953",
"4108,40237 25",
"90459,36018 18",
"86769,8014",
"6311,25772 13",
"9507,63470 20",
"30653,48087 11 15",
"84214,63941 1",
"87079,8036 13",
"10892,10164 25 23",
"31650,57394",
"12181,22580 9",
"8820,66849 5",
"63222,10057 12",
"85163,78521 6",
"73264,56781",
"45982,63119 4 6 16",
"96653,33496",
"4728,75705 8 16",
"93821,30582",
"9948,22812"} | 2198136 |
| Returns: 1.078664288678656 | |
| 4) | | | {"26749,31005 7 12 37 22 3 31 18 14 22 43 18 47 27",
"89443,23479 37 20 18 45 4 28 45 48 45 48 42 43",
"26624,91482 8 10 9 47 38 7 26 38 7 15 24 33 13 19",
"74780,3281 5 35 24 0 32 6 7 40 16 44 27 29 27 42",
"64491,34646 12 42 9 10 25 1 14 22 32 28 16 24 49",
"57611,97350 3 6 16 38 37 26 7 46 47 9 34 17 36 33",
"54900,19650 5 41 17 21 3 41 45 9 45 24 7 29 49 33",
"37779,84466 30 37 0 43 36 2 5 45 31 2 3 13 17 6 27",
"68486,19205 2 14 42 44 17 34 25 41 38 43 10 49 11",
"86915,28119 21 31 2 40 31 12 49 41 4 22 5 34 6 46",
"81402,18667 40 2 17 17 18 8 4 12 38 12 15 26 42 12",
"76982,50425 29 29 13 8 36 49 16 27 26 28 27 34 46",
"43766,59005 15 27 0 9 4 22 10 10 49 44 37 10 34 24",
"71619,57051 36 47 48 39 11 45 32 42 30 7 29 2 34",
"95436,51350 8 18 23 22 17 23 22 16 0 48 19 4 24 19",
"18419,71778 29 12 31 23 24 30 36 26 10 18 45 36 2",
"58370,12207 48 33 5 14 19 47 11 28 44 3 18 32 4 46",
"93954,84580 33 21 10 8 47 35 32 6 10 14 43 22 5 7",
"37005,46508 14 29 22 1 10 0 23 37 15 0 31 16 31 41",
"25700,33156 20 25 35 40 38 16 14 22 14 23 27 25 2",
"87882,64415 19 35 35 33 1 24 26 24 34 41 34 31 29",
"80610,20042 9 27 35 28 46 17 37 28 36 37 6 41 34",
"55546,68119 39 18 34 14 12 0 14 9 0 17 39 4 19 27",
"12701,80882 30 32 15 14 37 42 14 47 18 33 47 19 46",
"14048,1355 15 20 20 3 28 42 14 12 2 49 45 25 6 4",
"27482,63293 49 38 45 19 8 39 4 38 47 38 46 19 24",
"48215,66574 35 41 32 30 20 41 5 2 15 35 10 11 49",
"53613,76037 21 12 40 11 42 46 0 3 49 11 19 3 22 7",
"90922,15011 21 30 40 40 40 21 44 24 1 16 4 11 49",
"67445,91022 15 11 35 47 18 11 38 20 13 38 3 32 6",
"93451,40067 41 23 7 28 26 47 42 15 44 49 31 13 31",
"25947,11589 9 15 9 40 39 0 30 7 18 20 30 35 43 18",
"98403,23751 47 37 44 26 23 17 3 13 48 4 16 42 29",
"32918,74706 17 16 39 47 41 20 23 36 5 35 2 6 43 43",
"9851,96894 8 22 44 36 20 21 5 20 12 9 43 13 46 11",
"86305,55072 37 21 26 29 20 3 20 17 19 46 26 33 31",
"66150,16273 45 13 39 7 21 34 15 11 45 39 15 33 5",
"95275,37494 1 35 32 43 7 0 21 23 5 39 44 21 18 12",
"16184,79884 40 25 39 5 8 2 19 10 47 2 25 29 25 29",
"77000,48925 33 22 36 38 25 37 13 31 40 46 40 36 22",
"19246,92639 10 38 9 28 19 28 28 48 31 27 39 39 3",
"37419,96699 30 26 33 6 9 8 45 26 21 6 20 46 18 43",
"65611,72059 8 30 23 4 44 24 10 13 27 3 32 1 48 48",
"78307,80168 37 7 8 17 0 31 34 41 48 33 33 48 1 48",
"35276,57349 8 32 45 34 30 37 28 42 12 46 16 49 3",
"10106,992 36 25 44 41 1 7 36 13 15 6 6 1 24 49 1",
"43647,68652 21 5 35 44 39 27 41 9 25 16 34 23 11",
"7588,43383 32 33 29 2 17 13 30 38 23 16 25 5 23 0",
"39549,20275 16 13 40 14 32 43 1 1 42 43 42 43",
"49470,43071 25 9 8 12 30 11 44 26 28 27 24 45 4 6"} | 45315043 |
| Returns: 1.4635199471353126 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A computer connected to the internet is identified by an IP address. The most common way of displaying an IP address is the dotted quad method: four eight-bit (0-255 in base ten) numbers separated by periods.
Someone has given you a possible IP address, but the periods have been removed, leaving only a string of digits. Write a class IPConverter with a method possibleAddresses that takes a String ambiguousIP containing the digits and returns a String[] containing all the possible IP addresses that can be formed from those digits by inserting three periods to form a dotted quad. Sort the elements of the return value lexicographically, using their string ordering (the period character precedes all digit characters).
The numbers in each of the four positions can have any integer value between zero and 255, inclusive. However, a number may not have leading zeroes. For example, the digits 1902426 can form 1.90.24.26, 19.0.24.26, 190.2.4.26, and other IP addresses (see Example 0). However, it cannot form 19.02.4.26. | | Definition | | Class: | IPConverter | Method: | possibleAddresses | Parameters: | String | Returns: | String[] | Method signature: | String[] possibleAddresses(String ambiguousIP) | (be sure your method is public) |
| | | | Constraints | - | ambiguousIP will contain between 0 and 50 characters, inclusive. | - | Each character of ambiguousIP will be between '0' and '9', inclusive. | | Examples | 0) | | | | Returns:
{ "1.90.24.26",
"1.90.242.6",
"19.0.24.26",
"19.0.242.6",
"190.2.4.26",
"190.2.42.6",
"190.24.2.6" } | This is the example from the problem statement. |
|
| 1) | | | | 2) | | | | 3) | | | | Returns: { "0.18.62.90", "0.186.2.90", "0.186.29.0" } | |
| 4) | | | | Returns:
{ "1.1.111.111",
"1.11.11.111",
"1.11.111.11",
"1.111.1.111",
"1.111.11.11",
"1.111.111.1",
"11.1.11.111",
"11.1.111.11",
"11.11.1.111",
"11.11.11.11",
"11.11.111.1",
"11.111.1.11",
"11.111.11.1",
"111.1.1.111",
"111.1.11.11",
"111.1.111.1",
"111.11.1.11",
"111.11.11.1",
"111.111.1.1" } | |
| 5) | | | "3082390871771742784899852251737850570843857369760" |
| Returns: { } | |
| 6) | | | | Returns: { "2.56.255.255", "25.6.255.255", "25.62.55.255" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Suppose you are standing at the highest point, called M0, of a mountainous landscape. As you look around, you wonder how many different points you could walk to starting from your lofty position without ever going uphill. The set of these points is called peak P0, and the entire landscape can be divided into peaks according to a similar definition. For i > 0, let Mi be the highest point of the landscape not contained in peaks P0 through Pi - 1, and let peak Pi be the set of points to which there is a path from Mi that never goes uphill (but may remain level) and never touches points already contained in P0 through Pi - 1. The number of peaks in the landscape is the smallest value of n for which all points of the landscape are contained in P0 through Pn - 1.
You have a topographical map of a rectangular landscape, and you are interested in the area of its peaks. Write a class TopographicalImage with a method calcPeakAreas that takes a String[] topoData containing the height of the landscape at each x and y position and returns a int[] with the areas of each peak. The ASCII value of character x of element y of topoData is the height of the landscape at point (x,y). You can walk from a point to each of its vertical, horizontal, and diagonal neighbors. The return value should have a number of elements equal to the number of peaks in the landscape, and element i should be the number of points in Pi. If there is a tie between multiple points for maximum height when choosing Mi, choose the point with the smallest y-coordinate. If there is still a tie between points with the same y-coordinate, choose the point with the smallest x-coordinate. | | Definition | | Class: | TopographicalImage | Method: | calcPeakAreas | Parameters: | String[] | Returns: | int[] | Method signature: | int[] calcPeakAreas(String[] topoData) | (be sure your method is public) |
| | | | Notes | - | Point Mi is always contained in peak Pi, so the area of a peak is always at least 1. | | Constraints | - | topoData will contain between 1 and 50 elements, inclusive. | - | Each element of topoData will contain between 1 and 50 characters, inclusive. | - | Each element of topoData will contain the same number of characters. | - | Each element of topoData will contain only characters with ASCII value between 33 and 126, inclusive. | | Examples | 0) | | | {
"............",
"....i..i....",
"....i..i....",
".o..i..i..o.",
".o........o.",
"..oooooooo..",
"............"
} |
| Returns: { 78, 3, 3 } | |
| 1) | | | {
"............",
"....i..i....",
"....i..i....",
".S..i..i..Y.",
".M........E.",
"..ILEYSMIL..",
"............"
} |
| Returns: { 69, 3, 2, 5, 3, 1, 1 } | |
| 2) | | | {
"zzzzzzzzzzzzz",
"z...........z",
"z...c.b.c...z",
"z....bab.b..z",
"z...c.b.c...z",
"z...........z",
"zzzzzzzzzzzzz"
} |
| Returns: { 81, 6, 2, 1, 1 } | |
| 3) | | | | 4) | | | {
"AAAAAAABBBBCCCDEFGHHIIJIIHGFEDDCCCBBBBBBBBBBAAAAAA",
"AAAAABBBBBCCDDEEFGHIJJJJIIHGFEDDCCCCCCCCCBBBBBAAAA",
"AAAABBBBCCCDDEEFGHIIJJJJJIHGFEDDDDDDDDDCCCCBBBBAAA",
"AAABBBBCCDDEEFFGHHIJJJJJJIHGFEEDDDDDEEDDDDCCBBBBAA",
"AABBBCCDDEEFFGGHHIIJJJJJIHHGFEEEEEEEFFFEEDDCCBBBAA",
"BBBBCCDDEFFGHHHIIIIJJJIIIHGFFEEEEFFGGGGGFEEDCCBBBA",
"BBBCCDEEFGHIIIJJJJIIIIIHHGGFFEEFFGGHHHHHGGFEDCCBBB",
"BBCCDEEGHIJJKKKKJJJIIHHGGFFEEEEFGGHIIJJIIHGFEDCCBB",
"CCCDEEFHIJKLMMMLKKJIHHGGFFEEEEFFGHIJJKKJJIHGFEDCBB",
"CDDEEFHIJLMNNNNMLKJIHGFFEEEDEEFFGIJKKLLLKJIHFEDCCB",
"DDEFFGIJLMNOPPONMLJIHGFEEDDDDEFGHIJKLMMMLKJIGFEDCB",
"EEFFGHIKMNOQQQPONLKIHFEEDDDDDEFGHIKLMMNMMLKIHGEDCC",
"FFGGHIJLMOPQRRQPNMKIGFEDDCCDDEFGHIKLMNNNNMLJIGFEDC",
"GHHHIJKLNOQRRRQPOMKIGFEDDCCDDEFGHIKLMNNNNMLKIHFEDC",
"HIIIJJKLNOPQRRQPNLKIGFEDDCCDDEFGHJKLMNOONNMKJHGFDC",
"IJJJJJKLMOPQQQPONLJHGFEDDDDDEEFGIJKLMNOONNMLJIGFED",
"JJJJJKKLMNOOPPONMKJHGFEDDDDEEFGHIJKLMNNONNMLJIGFED",
"JKKJJJKKLMMNNNNMLJIHFFEEEEEFGGHIJKLMMNNNNMMKJIGFED",
"KKKJJJJJKKLLMLLKJIHGFFEEEFFGHIJKKLMMNNNNNMLKJHGFED",
"JJJJIIIIIJJJKKJJIIHGFFFFFGHIJKLMMNNNNNNMMLKJIHGEDC",
"JJJIIHHHHHHIIIIIHHGGGGGGHIJKLMNOOOOONNMMLKJIHGFEDC",
"IIIHHGGGGGGGGHHHGGGGGGHIIJLMNOPQQQQPONMLKJIHGFEDDC",
"HHHGGFFFFFFFFFGGGGGGHHIJKMNOQRSSSSRQPNMLKIHGFFEDCC",
"GGGFFEEEEEEEEFFFGGGHIJKLMOPRSTUUUTSRPNMKJHGFFEDCCB",
"FFFEEEEDDDDEEEEFGGHIJKLNOQRTUVWWWVTRPNLJIHFEEDCCBB",
"EEEEDDDDDDDDEEEFGHIJKLNOQRTVWXYYXWUSPNLJHGFEDCCBBB",
"DDDDDDDDDDDEEEFFGHIKLNOQRTVWXYZYYWURPMKIGFEDCCBBBB",
"CDDDDDDEEEEEEFFGHIJKMOPRSUWXYZZZXWTROMJHGEDCCBBBBA",
"CCDDDEEEFFFFFGGHHJKLNOQRTVWXYZZYXVTQNLIGFEDCBBBAAA",
"CCDDEFFGGGGHHHHIIJKMNPQSTVWXYYYXVURPMKIGEDCBBBAAAA",
"CDDEFGGHIIIIIIIJJKLMOPQSTUVWWXWVUSQNLJHFECCBBBAAAA",
"CDEFGHIJKKKKKKKKKLMNOPQRSTUVVVUTSQOMJHGEDCBBBAAAAA",
"CDEGHIKLMMMMMMLLLMMNOPQRSSTTTTSRQOMKIGFDCCBBAAAAAA",
"DEFGIKLMNOOOONNMMMNNOPQQRRRRRRQPNMKIHFEDCBBBAAAAAA",
"DEGHJLMOPQQQPPOONNNOOPPPQQQPPONMLKIHFEDCBBBAAAAAAA",
"DEGIKMNPQRRRRQPOOOOOOOPPPOOONMLKJIHFEDCCBBAAAAAAAA",
"DFGIKMOQRSSSRRQPOOOOOOOOONMMLKJIHGFEDCCBBBAAAAAAAA",
"DFGIKMOQRSSSRRQPOOOOONNNMMLKJIIHGFEDCCBBBAAAAAAAAA",
"DEGIJLNPQRRRRQPOONNNNNMMLLKJIHGFEEDCCBBBAAAAAAAAAA",
"DEFHJKMOPQQQQPOONNMMMMLLKJIHGGFEDDCCBBBAAAAAAAAAAA",
"CDFGIJLMNOOOONNMMLLLLLKKJIHGFEEDCCCBBBAAAAAAAAAAAA",
"CDEFGIJKLMMMMMLLKKKKKJJIIHGFEDDCCBBBBAAAAAAAAAAAAA",
"CCDEFGHIJKKKKKJJJIIIIIHHGGFEDDCCBBBBAAAAAAAAAAAAAA",
"BCCDEFGHHIIIIIHHHHHHHGGGFFEDDCCBBBAAAAAAAAAAAAAAAA",
"BBCCDEEFFGGGGGGFFFFFFFFEEDDCCCBBBAAAAAAAAAAAAAAAAA",
"BBBCCDDEEEEEEEEEEEEEEEEDDDCCBBBBAAAAAAAAAAAAAAAAAA",
"ABBBCCCCDDDDDDDDDDDDDDDCCCCBBBBAAAAAAAAAAAAAAAAAAA",
"AABBBBBCCCCCCCCCCCCCCCCCCBBBBBAAAAAAAAAAAAAAAAAAAA",
"AAABBBBBBBBBBBBBBBBBBBBBBBBBAAAAAAAAAAAAAAAAAAAAAA",
"AAAAAABBBBBBBBBBBBBBBBBBBBAAAAAAAAAAAAAAAAAAAAAAAA"
} |
| Returns: { 1918, 65, 483, 5, 5, 24 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Many systems of information are naturally organized as hierarchical trees. For example, a computer file system has a hierarchical structure of nested directories, and a textbook could be successively divided into chapters, sections, and subsections. In a hierarchical tree, the root node has no parent, and all other nodes are descendants of the root with exactly one parent each. Therefore, even though a node can have any number of children (zero or more), it is possible to describe the structure of a tree by specifying just the parent of each node.
You have a list of node-parent relationships and you want to analyze the structure of the hierarchical tree. All nodes are named with strings of lowercase alphabetical characters, except for the root node which is named ROOT. Write a class HierarchicalTree with a method countDescendants that takes a String[] parentData containing the nodes and their parents and returns a String[] listing the number of descendants of each node. If parentData describes a structure that is not a hierarchical tree, return an empty String[].
The elements of parentData should be concatenated, in order, onto an initially empty string. The resulting string will contain pairs of the form NODE,PARENT separated by exactly one space character. NODE represents the name of a node, and PARENT represents the name of NODE's parent. A name will either consist of all lowercase English characters ('a' to 'z') or be ROOT. The elements of the return value should be formatted "NODE: DESCENDANT_COUNT" (quotes are for clarity) with exactly one space character between the colon and the number of descendants, which should contain no extra leading zeroes. The elements of the return value should be sorted in lexicographical order by NODE. Since ROOT is capitalized, it should always precede the other nodes in the returned array. | | Definition | | Class: | HierarchicalTree | Method: | countDescendants | Parameters: | String[] | Returns: | String[] | Method signature: | String[] countDescendants(String[] parentData) | (be sure your method is public) |
| | | | Notes | - | The ROOT node always exists. | - | The name of a node uniquely identifies it. There cannot be multiple nodes with the same name, even if they have different parents. | - | A node does not count as its own descendant. | | Constraints | - | parentData will contain between 0 and 50 elements, inclusive. | - | Each element of parentData will contain between 0 and 50 characters, inclusive. | - | When the elements of parentData are concatenated, the resulting string will be a list of name pairs with no leading or trailing spaces. Members of a pair will be separated by a single comma, and pairs will be separated by a single space. Names will be lowercase alphabetical English characters ('a' to 'z') or ROOT. | | Examples | 0) | | | {"bin,ROOT tty,dev dev,ROOT passwd,etc etc,ROOT lib",
",ROOT mnt,ROOT proc,ROOT tmp,ROOT usr,ROOT var,RO",
"OT libc,lib screens,tmp kernel,usr log,var tty,de",
"v"} |
| Returns:
{ "ROOT: 15",
"bin: 0",
"dev: 1",
"etc: 1",
"kernel: 0",
"lib: 1",
"libc: 0",
"log: 0",
"mnt: 0",
"passwd: 0",
"proc: 0",
"screens: 0",
"tmp: 1",
"tty: 0",
"usr: 1",
"var: 1" } | A node doesn't have to be specified as a child before it is specified as a parent. Also, redundant specifications may exist (tty,dev appears twice). |
|
| 1) | | | | 2) | | | {"disconnectb,disconnecta cyclea,ROOT intermediatea",
",cyclea cycleb,intermediatea cyclea,cycleb ROOT,r",
"ootparent"} |
| Returns: { } | There are three things wrong with this tree. First, disconnecta and disconnectb are not descendants of ROOT. Second, cyclea has two parents. Third, rootparent is specified as the parent of ROOT. |
|
| 3) | | | {"sapiens,homo homo,hominidae hominidae,primates pri",
"mates,mammalia mammalia,chordata chordata,animalia",
" animalia,eukarya eukarya,ROOT ",
"protista,eukarya fungi,eukarya plantae,eukarya ",
"porifera,animalia cnidaria,animalia platyhelminthe",
"s,animalia nematoda,animalia mollusca,animalia ann",
"elida,animalia arthropoda,animalia echinodermata,a",
"nimalia ",
"agnatha,chordata chondrichthyes,chordata osteichth",
"yes,chordata amphibia,chordata reptilia,chordata a",
"ves,chordata ",
"artiodactyla,mammalia carnivora,mammalia cetacea,m",
"ammalia chiroptera,mammalia dermoptera,mammalia hy",
"racoidea,mammalia insectivora,mammalia lagomorpha,",
"mammalia macroscelidea,mammalia notoryctemorphia,m",
"ammalia perissodactyla,mammalia pholidota,mammalia",
" proboscidea,mammalia rodentia,mammalia scandentia",
",mammalia sirenia,mammalia tubulidentata,mammalia ",
"xenarthra,mammalia ",
"lemuridae,primates cheirogaleidae,primates indrida",
"e,primates daubentoniidae,primates galagonidae,pri",
"mates loridae,primates megaladapidae,primates tars",
"iidae,primates cebidae,primates cercopithecidae,pr",
"imates callitrichidae,primates hylobatidae,primate",
"s ",
"gorilla,hominidae pan,hominidae pongo,hominidae ",
"domain,ROOT kingdom,domain phylum,kingdom class,ph",
"ylum order,class family,order genus,family species",
",genus ninja,mammalia"} |
| Returns:
{ "ROOT: 67",
"agnatha: 0",
"amphibia: 0",
"animalia: 54",
"annelida: 0",
"arthropoda: 0",
"artiodactyla: 0",
"aves: 0",
"callitrichidae: 0",
"carnivora: 0",
"cebidae: 0",
"cercopithecidae: 0",
"cetacea: 0",
"cheirogaleidae: 0",
"chiroptera: 0",
"chondrichthyes: 0",
"chordata: 45",
"class: 4",
"cnidaria: 0",
"daubentoniidae: 0",
"dermoptera: 0",
"domain: 7",
"echinodermata: 0",
"eukarya: 58",
"family: 2",
"fungi: 0",
"galagonidae: 0",
"genus: 1",
"gorilla: 0",
"hominidae: 5",
"homo: 1",
"hylobatidae: 0",
"hyracoidea: 0",
"indridae: 0",
"insectivora: 0",
"kingdom: 6",
"lagomorpha: 0",
"lemuridae: 0",
"loridae: 0",
"macroscelidea: 0",
"mammalia: 38",
"megaladapidae: 0",
"mollusca: 0",
"nematoda: 0",
"ninja: 0",
"notoryctemorphia: 0",
"order: 3",
"osteichthyes: 0",
"pan: 0",
"perissodactyla: 0",
"pholidota: 0",
"phylum: 5",
"plantae: 0",
"platyhelminthes: 0",
"pongo: 0",
"porifera: 0",
"primates: 18",
"proboscidea: 0",
"protista: 0",
"reptilia: 0",
"rodentia: 0",
"sapiens: 0",
"scandentia: 0",
"sirenia: 0",
"species: 0",
"tarsiidae: 0",
"tubulidentata: 0",
"xenarthra: 0" } | The phylogeny of living organisms can be represented as a hierarchical tree. |
|
| 4) | | | {"duke,ROOT arizona,duke maryland,duke michiganst,a",
"rizona usc,duke stanford,maryland temple,michigan",
"st illinois,arizona ucla,duke kentucky,usc cincin",
"nati,stanford georgetown,maryland gonzaga,michiga",
"nst pennst,temple kansas,illinois mississippi,ari",
"zona missouri,duke utahst,ucla bostoncollege,usc ",
"iowa,kentucky stjosephs,stanford kentstate,cincin",
"nati georgiast,maryland hampton,georgetown fresno",
"st,michiganst indianast,gonzaga florida,temple no",
"rthcarolina,pennst charlotte,illinois syracuse,ka",
"nsas notredame,mississippi butler,arizona monmout",
"h,duke georgia,missouri ohiost,utahst hofstra,ucl",
"a oklahomast,usc southernutah,bostoncollege creig",
"hton,iowa holycross,kentucky ncgreensboro,stanfor",
"d georgiatech,stjosephs byu,cincinnati indiana,ke",
"ntstate wisconsin,georgiast georgemason,maryland ",
"arkansas,georgetown iowast,hampton alabamast,mich",
"iganst california,fresnost virginia,gonzaga oklah",
"oma,indianast texas,temple westernky,florida prov",
"idence,pennst princeton,northcarolina northwester",
"nst,illinois tennessee,charlotte hawaii,syracuse ",
"csnorthridge,kansas xavier,notredame iona,mississ",
"ippi wakeforest,butler easternill,arizona winthro",
"p,northwesternst"} |
| Returns:
{ "ROOT: 65",
"alabamast: 0",
"arizona: 32",
"arkansas: 0",
"bostoncollege: 1",
"butler: 1",
"byu: 0",
"california: 0",
"charlotte: 1",
"cincinnati: 3",
"creighton: 0",
"csnorthridge: 0",
"duke: 64",
"easternill: 0",
"florida: 1",
"fresnost: 1",
"georgemason: 0",
"georgetown: 3",
"georgia: 0",
"georgiast: 1",
"georgiatech: 0",
"gonzaga: 3",
"hampton: 1",
"hawaii: 0",
"hofstra: 0",
"holycross: 0",
"illinois: 8",
"indiana: 0",
"indianast: 1",
"iona: 0",
"iowa: 1",
"iowast: 0",
"kansas: 3",
"kentstate: 1",
"kentucky: 3",
"maryland: 15",
"michiganst: 15",
"mississippi: 3",
"missouri: 1",
"monmouth: 0",
"ncgreensboro: 0",
"northcarolina: 1",
"northwesternst: 1",
"notredame: 1",
"ohiost: 0",
"oklahoma: 0",
"oklahomast: 0",
"pennst: 3",
"princeton: 0",
"providence: 0",
"southernutah: 0",
"stanford: 7",
"stjosephs: 1",
"syracuse: 1",
"temple: 7",
"tennessee: 0",
"texas: 0",
"ucla: 3",
"usc: 7",
"utahst: 1",
"virginia: 0",
"wakeforest: 0",
"westernky: 0",
"winthrop: 0",
"wisconsin: 0",
"xavier: 0" } | The results of single-elimination tournaments can also be expressed as hierarchical trees. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You are developing the matchmaking component of an online dating site. Prospective
members must fill out a questionnaire consisting of
binary questions such as Do you prefer to vacation (a)
in the mountains or (b) at the seaside? and Would you rather
travel (a) by plane or (b) by train?
You are to match up men with women by maximizing the number of answers
each couple has in common. A man and a woman have an answer in common
whenever they give the same answer to the same question. Conflicts
can easily arise due to numerical ties,
but you will be able to resolve all such conflicts using
the following procedure. Note that there will be equal numbers of men and
women, with names being unique in each sex.
Take the woman whose name comes earliest
in lexicographic order, and consider the men with whom she has the greatest number of
answers in common. Among these men, pick the one whose name comes earliest in lexicographic
order. You have found the woman's best match. Remove this couple from
the dating pool, and repeat the matching procedure until there are no
more singles left.
You are given a String[], namesWomen, containing
the names of single women, and another String[],
answersWomen, containing their answers. The kth
element of answersWomen lists the answers of the woman whose name
is the kth element of namesWomen. If there are n
questions in the questionnaire, then every element of answersWomen
consists of n characters, each of which is either 'a' or
'b'. The answers are always given in the fixed questionnaire order. You
are similarly given the String[]s namesMen and
answersMen for the single men. Lastly, you are given
a String, queryWoman, containing the name of a woman.
Return the name of the man to whom she is matched after you have formed
all couples according to the above rules.
| | Definition | | Class: | MatchMaking | Method: | makeMatch | Parameters: | String[], String[], String[], String[], String | Returns: | String | Method signature: | String makeMatch(String[] namesWomen, String[] answersWomen, String[] namesMen, String[] answersMen, String queryWoman) | (be sure your method is public) |
| | | | Notes | - | Lexicographic order is like dictionary order, with the difference that case matters. All uppercase letters take precedence over all lowercase letters. Thus, "boolean" comes before "boot"; "boo" comes before "boolean"; "Boot" comes before "boo"; "Zoo" comes before "boo". | | Constraints | - | namesWomen contains between 1 and 50 elements, inclusive | - | if namesWomen consists of n elements, then answersWomen, namesMen, and answersMen consist of n elements each | - | each element of namesWomen and each element of namesMen is between 1 and 50 characters long, inclusive | - | the only characters that may appear in namesMen and namesWomen are 'a' to 'z' and 'A' to 'Z' | - | no two elements of namesWomen are alike | - | no two elements of namesMen are alike | - | the first element of answersWomen is between 1 and 50 characters long, inclusive | - | if the first element of answersWomen consists of m characters, then each element of answersWomen and of answersMen is m characters long | - | the only characters that may appear in answersWomen and answersMen are 'a' and 'b' | - | queryWoman is one of the Strings in namesWomen | | Examples | 0) | | | {"Constance", "Bertha", "Alice"} | {"aaba", "baab", "aaaa"} | {"Chip", "Biff", "Abe"} | {"bbaa", "baaa", "aaab"} | "Bertha" |
| Returns: "Biff" | Alice has two answers in common with Chip and three answers in common with both Abe and Biff; Abe gets lexicographic preference. Bertha also has two answers in common with Chip and three answers in common with both Abe and Biff. Since Abe has already been matched to Alice, Bertha lands Biff. |
|
| 1) | | | {"Constance", "Bertha", "Alice"} | {"aaba", "baab", "aaaa"} | {"Chip", "Biff", "Abe"} | {"bbaa", "baaa", "aaab"} | "Constance" |
| Returns: "Chip" | We are dealing with the same names and answers as before. Constance is the last to go. Although she has two answers in common with Abe and Biff, they are both taken. She ends up with Chip, with whom she has only one answer in common. |
|
| 2) | | | {"Constance", "Alice", "Bertha", "Delilah", "Emily"} | {"baabaa", "ababab", "aaabbb", "bababa", "baabba"} | {"Ed", "Duff", "Chip", "Abe", "Biff"} | {"aabaab", "babbab", "bbbaaa", "abbbba", "abaaba"} | "Constance" |
| Returns: "Duff" | |
| 3) | | | {"Constance", "Alice", "Bertha", "Delilah", "Emily"} | {"baabaa", "ababab", "aaabbb", "bababa", "baabba"} | {"Ed", "Duff", "Chip", "Abe", "Biff"} | {"aabaab", "babbab", "bbbaaa", "abbbba", "abaaba"} | "Delilah" |
| Returns: "Chip" | |
| 4) | | | {"Constance", "Alice", "Bertha", "Delilah", "Emily"} | {"baabaa", "ababab", "aaabbb", "bababa", "baabba"} | {"Ed", "Duff", "Chip", "Abe", "Biff"} | {"aabaab", "babbab", "bbbaaa", "abbbba", "abaaba"} | "Emily" |
| Returns: "Ed" | |
| 5) | | | {"anne", "Zoe"} | {"a", "a"} | {"bob", "chuck"} | {"a", "a"} | "Zoe" |
| Returns: "bob" | |
| 6) | | | {"F", "M", "S", "h", "q", "g", "r", "N", "U", "x", "H", "P",
"o", "E", "R", "z", "L", "m", "e", "u", "K", "A", "w", "Q",
"O", "v", "j", "a", "t", "p", "C", "G", "k", "c", "V", "B",
"D", "s", "n", "i", "f", "T", "I", "l", "d", "J", "y", "b"} | {"abaabbbb", "bbaabbbb", "aaabaaab", "aabbaaaa", "baabbaab",
"aaababba", "bbabbbbb", "bbbabbba", "aaabbbba", "aabbbaaa",
"abbabaaa", "babbabbb", "aaaaabba", "aaaabbaa", "abbbabaa",
"babababa", "abbaaaaa", "bbababba", "baaaaaba", "baaaaabb",
"bbbbabba", "ababbaaa", "abbbabab", "baabbbaa", "bbbaabbb",
"aababbab", "ababbabb", "abbaabba", "baabbabb", "aaabaaab",
"aabbbaba", "aabaaabb", "abababba", "aabbaaaa", "aabbabaa",
"bababaaa", "aabaaaab", "bbbbaabb", "baaababb", "abaabbab",
"aabbbaaa", "baabbaba", "bbabbbaa", "aabbbbaa", "abbbaaab",
"abababbb", "ababaaba", "bababaaa"} | {"f", "C", "v", "g", "Q", "z", "n", "c", "B", "o", "M", "F",
"u", "x", "I", "T", "K", "L", "E", "U", "w", "A", "d", "t",
"e", "R", "D", "s", "p", "q", "m", "r", "H", "j", "J", "V",
"l", "a", "k", "h", "G", "y", "i", "P", "O", "N", "b", "S"} | {"bbbaabab", "bbabaabb", "ababbbbb", "bbbababb", "baababaa",
"bbaaabab", "abbabbaa", "bbbabbbb", "aabbabab", "abbababa",
"aababbbb", "bababaab", "aaababbb", "baabbaba", "abaaaaab",
"bbaababa", "babaabab", "abbabbba", "ababbbab", "baabbbab",
"babbaaab", "abbbbaba", "bbabbbba", "baaabaab", "ababbabb",
"abbbaabb", "bbbbaabb", "bbbaaabb", "baabbaba", "bbabaaab",
"aabbbaab", "abbbbabb", "bbaaaaba", "bbbababa", "abbaabba",
"bababbbb", "aabaaabb", "babbabab", "baaaabaa", "ababbaba",
"aaabaabb", "bbaaabaa", "baaaaabb", "bbaabaab", "bbababab",
"aabaaaab", "aaaaabab", "aabbaaba"} | "U" |
| Returns: "x" | |
| 7) | | | {"q", "M", "w", "y", "p", "N", "s", "r", "a", "H", "o", "n",
"F", "m", "l", "b", "D", "j", "C", "u", "f", "I", "g", "L",
"i", "x", "A", "G", "O", "k", "h", "d", "c", "E", "B", "v",
"J", "z", "K", "e", "t"} | {"aabbaaabb", "baabababb", "bbaababba", "bbbaaaaaa", "abaaaabaa",
"bababbbab", "abbaabbaa", "aabababbb", "bababaaaa", "abbababaa",
"aabbbbbba", "bbabbabab", "babaabbba", "babbabbbb", "baaabbbbb",
"baaabaaaa", "aaabbaaab", "abbaabbbb", "abbabbbab", "bbaaaabba",
"babbaaabb", "aabbabbab", "baaababba", "ababaabab", "bbbaabbab",
"aaaabbabb", "babaaaaaa", "abbbbaaab", "aabaaabba", "bbbaaaaba",
"bbbbbbaab", "aabbaaabb", "aabaabbab", "aababaaba", "bbabbbbab",
"abbabaaab", "babaaabbb", "bababbaaa", "aabbaabaa", "baaabbabb",
"bbbbbbbbb"} | {"m", "k", "n", "q", "L", "E", "M", "l", "w", "x", "g", "e",
"i", "z", "F", "r", "a", "h", "f", "D", "J", "K", "j", "v",
"A", "t", "N", "y", "s", "c", "o", "p", "d", "b", "B", "G",
"O", "I", "u", "C", "H"} | {"bbaaabbba", "bbaaaaaab", "abaaababb", "baaaabbbb", "abbbababa",
"baaaaaaaa", "aabbbbbab", "aaaaabbba", "baabababb", "babaaabab",
"baaababaa", "bbbbaabba", "bbaabbabb", "bbaaababb", "abbabbaba",
"aababaaab", "abbbbbbaa", "aabbaabaa", "bbbaabbba", "abbabbaba",
"aaabbbaaa", "bbaabaaaa", "aabababbb", "abbbbabab", "baaabbbba",
"bababbbba", "aababbaab", "bbaabbaab", "bbbaaabbb", "babbbbabb",
"ababababb", "babaaabab", "bbaaaaaba", "aaaaabaaa", "abbaaabbb",
"bbbbababb", "baabababb", "bbaabaaaa", "aaababbbb", "abbbbbbba",
"bbaabbaaa"} | "o" |
| Returns: "C" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are a student advisor at TopCoder University (TCU). The graduation requirements at TCU are somewhat complicated. Each requirement states that a student must take some number of classes from some set, and all requirements must be satisfied for a student to graduate. Each class is represented as a single distinct character. For example, one requirement might be "Take any 2 classes of B, C, D, or F." Further complicating the matter is the fact that no class can be used to satisfy more than one requirement. And so students come to you all the time, dazed and confused, because they don't know how close they are to satisfying the requirements so that they can graduate!
Each class is represented as a distinct single character whose ASCII value is between 33 and 126, inclusive, but is also not a numeric character ('0'-'9'). You will be given a String classesTaken, which represents the classes that a given student has taken. You will also be given a String[] requirements, which lists the requirements needed to graduate. Each String in requirements will start with a positive integer, followed by some number of classes. For example, the requirement "Take any 2 classes from B, C, D, or F" would be represented in requirements as "2BCDF".
Your method should return a String representing the classes that the student needs to take in order to graduate, in ASCII order. Classes may not be taken more than once. If there is more than one set that will allow the student to graduate, return the smallest set. If there are multiple smallest sets, return the lexicographically smallest of these. Finally, if there is no set that will enable this student to graduate, return "0".
| | Definition | | Class: | Graduation | Method: | moreClasses | Parameters: | String, String[] | Returns: | String | Method signature: | String moreClasses(String classesTaken, String[] requirements) | (be sure your method is public) |
| | | | Notes | - | Classes may not be taken more than once. | | Constraints | - | classesTaken will be between 0 and 50 characters in length, inclusive. | - | each character in classesTaken will be a valid class (ASCII value between 33 to 126 inclusive and not a digit). | - | there will be no duplicate classes in classesTaken. | - | requirements will contain between 1 and 50 elements, inclusive. | - | each element of requirements will contain a positive integer with no leading zeros between 1 and 100, inclusive, followed by some number of valid classes. | - | each element of requirements will be between 1 and 50 characters in length, inclusive. | - | there will be no duplicate classes in any given element of requirements | | Examples | 0) | | | | Returns: "BCD" | The student must take two classes from {A,B,C}, and two from {C,D,E}. He has already taken A. |
|
| 1) | | | "+/NAMT" | {"3NAMT","2+/","1M"} |
| Returns: "" | The student has already fulfilled all the requirements - you should congratulate him for his achievement! |
|
| 2) | | | | Returns: "0" | No matter how hard you try, you can't take 100 classes out of 6. TCU had better fix their policies quick. |
|
| 3) | | | | 4) | | | "CDH" | {"2AP", "3CDEF", "1CDEFH"} |
| Returns: "AEP" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are the famous explorer Indiana Jones, or Lara Croft, take your pick. You are exploring the ruins of the dungeons beneath King Lockumup IV the Good's castle in Flatlandia. Of course, the dungeon layout is two-dimensional (like your character), East-West and Up-Down in this case, in a regular grid.
Surface
| | | | | | | | |
level 0 -R-R-R-R-R-R-R-R-R-
| | | | | | | | |
level 1 <- West -R-R-R-R-R-R-R-R-R- East ->
| | | | | | | | |
level 2 -R-R-R-R-R-R-R-R-R-
| | | | | | | | |
Depths of Despair
"R" indicates a room. "-" indicates an east-west passageway. "|" indicates an up-down passageway.
Because it is rough going in the passageways between the rooms (there has been no dungeon maintenance for centuries), it is frequently easier to travel through a passageway in a particular direction than in the opposite direction. Each room has four passageways leaving in the directions East (right), West (left), Up, and Down which lead to adjacent rooms (except the Down in the bottom-most rooms, the East in the east-most rooms, and the West in the west-most rooms, which have dead-end passageways due to ancient budget cuts, and Up in the topmost rooms which lead to the surface). The time it takes to travel through a passageway from a given room to the adjacent rooms is given in four String[]s depending on your direction of travel. A digit between 0 and 9 indicates how many time units (in the local system of decimillifortnights, dmfs) are taken to leave the room in that direction and travel through the passageway to the adjacent room. An 'x' character indicates that the travel in that direction is too difficult and can not be done. The dead end passageways (at the edges of the dungeon) have time values, or 'x', specified (because they were in the original plans for the dungeon and we have an old map), but we can not actually travel through these passageways in this problem. The dungeon does *not* wrap in any direction (you are probably thinking of the castle of Queen Mobius the One Sided, the former stripper).
In other words, if you are in room (i,j), where i is the up-down level and j is your easting (ie. how far east you are) coordinate,
then
- up[i][j] tells how many dmfs it takes to get to (i-1,j).
- down[i][j] tells how many dmfs it takes to get to (i+1,j).
- east[i][j] tells how many dmfs it takes to get to (i,j+1).
- west[i][j] tells how many dmfs it takes to get to (i,j-1).
If it is obvious to you how these four directional time value arrays map to
a directed graph of the dungeon, then skip this next section of the problem
description, which goes into detail, and continue reading below for more
of the important problem description information.
-
For example if given the inputs up and west (shown below)
up = {"123", west = {"222",
"111", "131",
"121"} "444"}
You would have the following time values for each passageway while going up or west.
Surface
1 2 3
level 0 2R2R2R- Up and West going
1 1 1 Passageway times
level 1 West 1R3R1R- East
1 2 1
level 2 4R4R4R-
| | |
Depths of Despair
The dead-end passageways on the far west with times {2, 1, 4} are useless and can be ignored.
Similarly if you have the inputs down and east (shown below)
down = {"987", east = {"222",
"111", "3x3",
"121"} "111"}
You would have the following time values for each passageway while going down or east.
Surface
| | |
level 0 -R2R2R2 Down and East going
9 8 7 Passageway times
level 1 West -R3RxR3 East
1 1 1
level 2 -R1R1R1
1 2 1
Depths of Despair
The dead-end passageways on the far east with times {2, 3, 1} and the very bottom with times {1, 2, 1} are also
useless to you and can be ignored.
We are back from the boring details, here is some more important information.
Unfortunately for you, Dr. Jones or Dr. Croft, you have just triggered an ancient trap, and the dungeon is beginning to to fill with water. First the lowest level fills with water. If the East-West width of the dungeon is n rooms, then each level of the dungeon takes n decimillifortnights to fill. Once full of water, the rooms on that level are no longer accessible. While partly full of water, they are still fully accessible. Time starts at time = 0, at time = n the lowest level becomes inaccessible, at time = 2n the second lowest level becomes inaccessible, etc. So if you are in, or pass through, a room on the lowest level at time >= n, you are dead.
For simplicity, we will only consider if the room is completely filled with water when you enter. So if you leave a nearly filled room, going up through a slow
passageway, and arrive somewhat later in a now nearly filled room one level up, this is ok. We will ignore the physics which would lead us to think the passageway would fill with water before the room above it. Only check for drowning when you enter the room. Also the surface (above level 0) never fills with water (we run out of water before then), so you can not drown on the surface.
Your goal is to get to the surface as fast as possible. You start at the location (startLevel, startEasting). "Easting" is how far east you are in the local coordinate system. Rooms have Easting coordinates between 0 and n-1 inclusive, where
n is the number of rooms on each level. Return the number of time units (decimillifortnights) it takes to escape, or -1 if escape is impossible.
For example:
up = {"0x4", down = {"0x9", east = {"1x9", west = {"0x9",
"0x3", "009", "0x9", "0x0",
"0x3"} "0x9"} "009"} "009"}
startLevel = 2, startEasting = 2
We start in the lower right corner. If water were not an issue, we could reach each of the various rooms
with various paths, and the earliest possible times in which we could reach each room are shown below. If we go straight up,
the passageways take 3, 3 and 4 dmfs reaching the surface in 10 dmfs. We could also follow the path: up (3 dmfs), west (0 dmfs),
down (0 dmfs), west (0 dmfs), up (0 dmfs), up (0 dmfs), and up (0 dmfs) reaching the surface in 3 dmfs. The diagram
below shows the minimum time in which we could first get to each room (if water were not an issue), and the
passageways used are shown with '|' for up-down, and '-' for east-west.
3 10 - surface
| | -------------
3-4 6 - level 0
| |
3 3-3 - level 1
| | |
3-3 0 - level 2
But since level 2 fills with water at time 3, we can not move from (1,1) down to (2,1) at time = 3 dmfs. The
actual rooms we can reach, considering flooding, are shown below, where 'w' represents a room which is full of water
when we first could move into it, and 'x' represents a room we can never reach:
10 - surface
| ------------
x w-6 - level 0
|
x 3-3 - level 1
| |
x w 0 - level 2
In this example we can reach the surface in 10 dmfs by going straight up, and this is the minimum,
so return 10.
| | Definition | | Class: | DungeonEscape | Method: | exitTime | Parameters: | String[], String[], String[], String[], int, int | Returns: | int | Method signature: | int exitTime(String[] up, String[] down, String[] east, String[] west, int startLevel, int startEasting) | (be sure your method is public) |
| | | | Constraints | - | up, down, east and west have the same constraints, only up is described. | - | up will contain between 1 and 50 elements inclusive. | - | each element of up will contain between 1 and 50 characters inclusive. | - | each character in each element of up will be a digit between '0' and '9' inclusive or 'x'. | - | up, down, east and west will all have exactly the same number of elements in each. | - | All elements of up, down, east and west will contain the same number of characters. | - | startLevel will be between 0 and (the number of elements in up) - 1 inclusive. | - | startEasting will be between 0 and (the number of characters in up[0]) - 1 inclusive. | | Examples | 0) | | | {"0x4",
"0x3",
"0x3"} | {"0x9",
"009",
"0x9"} | {"0x9",
"1x9",
"009"} | {"0x9",
"0x0",
"009"} | 2 | 2 |
| Returns: 10 | |
| 1) | | | {"xxxxxxxxx1",
"1xxxxxxxxx",
"xxxxxxxxx1"} | {"xxxxxxxxxx",
"xxxxxxxxxx",
"xxxxxxxxxx"} | {"1111111111",
"xxxxxxxxxx",
"1111111111"} | {"xxxxxxxxxx",
"1111111111",
"xxxxxxxxxx"} | 2 | 0 |
| Returns: 30 | Only one serpentine path out, just avoiding the water. |
|
| 2) | | | {"xxxxxxxxx1",
"xxxx999991",
"x999999991"} | {"1111111111",
"1111111111",
"1111111111"} | {"1111122242",
"2222222241",
"2111111111"} | {"xxxxxxxxx1",
"1111111111",
"xxxxxxxxx1"} | 2 | 0 |
| Returns: -1 | No way out that is fast enough, glub, glub... |
|
| 3) | | | {"1x2x3x4x5x6x7x8x9",
"00000000000000000",
"98765432223456789",
"12345678987654321"} | {"00000000000000000",
"00000000000000000",
"00000000000000000",
"00000000000000000"} | {"xxxxxxxxxxxxxxxxx",
"xxxxxxxxxxxxxxxxx",
"22222222222222222",
"33333333333333333"} | {"xxxxxxxxxxxxxxxxx",
"xxxxxxxxxxxxxxxxx",
"22222222222222222",
"33333333333333333"} | 3 | 12 |
| Returns: 17 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
You are a traveler in a chain of tropical islands in the South Pacific. Transportation between these islands is provided by competing ferry services. One-way tickets for the ferries can be purchased on each of the islands. The tickets are only accepted by the ferry service for which the ticket was purchased. Each ticket entitles you to ride any single leg offered by that ferry service, where a leg is a trip between any two islands.
Ticket prices vary from island to island, and are published daily in the local newspaper. Armed with the current prices, you wish to decide which island to visit next. Your task is to determine for each island the cheapest path to journey from your current location to that island. You are not concerned with the number of ferries you must ride, only with the cost of the tickets required to make each journey.
Anti-competitive regulations prohibit you from carrying more than one ticket for the same ferry service, and from carrying more than three tickets total. Thus, after stepping off a ferry onto an island, you will have zero, one, or two tickets in your possession, and any tickets you are carrying will not be for the ferry you just rode. You begin with no tickets in your possession, so the first step of any journey must be to purchase one, two, or three tickets on the initial island (island 0).
The available legs will be given as a String[] legs, with each element giving the legs offered by a particular ferry service. The size of legs gives you the number of ferry services. Each element of legs will be formatted as a list of "<island1>-<island2>" (representing a one-way leg from <island1> to <island2>), each separated by a single space.
The prices of ferry tickets sold on each island is given as a String[] prices, with each element giving the prices for tickets on a particular island. The size of prices gives you the number of islands. Each element of prices will be a space-separated list of integers, corresponding to the ticket prices for ferries in the same order they are given in legs.
There will be up to 40 islands and 10 ferry services. Given the list of legs offered by each ferry service and the prices of tickets on each island, for each island compute the cost of traveling there from your initial island (island 0), and return the costs as a int[]. The size of your returned int[] should be one less than the number of islands. If a given island is unreachable, return -1 for the cost to that island.
| | Definition | | Class: | IslandFerries | Method: | costs | Parameters: | String[], String[] | Returns: | int[] | Method signature: | int[] costs(String[] legs, String[] prices) | (be sure your method is public) |
| | | | Notes | - | There is not necessarily a route to all islands. | - | The cheapest route may including visiting an island more than once (see example 2 below). | | Constraints | - | legs will contain between 1 and 10 elements, inclusive. The size of legs gives you F, the number of ferry services. | - | prices will contain between 2 and 40 elements, inclusive. The size of prices gives you I, the number of islands. | - | Each element of legs will contain between 1 and 50 characters, inclusive. | - | Each element of prices will contain between 1 and 50 characters, inclusive. | - | Each <island> in legs will be an integer between 0 and I-1, inclusive. | - | Each element of prices will contain F integers between 1 and 1000, inclusive. | - | There will be no duplicates within a given element of legs. | - | The jth element in the list of prices[i] gives the price of a ticket for ferry service j sold on island i. | - | No integers will have leading zeros. | | Examples | 0) | | | { "0-1 0-3", "0-2" } | { "5 7", "1000 1000", "1000 1000", "1000 1000" } |
| Returns: { 5, 7, 5 } | You need an A ticket (cost of 5) to get to islands 1 and 3, and a B ticket (cost of 7) to get to island 2.
|
|
| 1) | | | { "0-1 1-2 2-3", "0-1 2-3" } | { "1 10", "20 25", "50 50", "1000 1000", "1000 1000" } |
| Returns: { 1, 11, 31, -1 } |
There are 5 islands and 2 ferry services. Referring to the ferry services as A and B, the cheapest way to get to island 1 is to purchase an A ticket on island 0 for a cost of 1 and take the A ferry to island 1.
The cheapest way to get to island 2 is to purchase A and B tickets on island 0 for a total cost of 11. Use the B ticket to get to island 1 and the A ticket to get to island 2.
The cheapest way to get to island 3 is to purchase A and B tickets on island 0 (cost of 11), use the A ticket to get to island 1, purchase another A ticket on island 1 (cost of 20), use it to get to island 2, and then use the B ticket to get to island 3. The total cost is 31.
There is no path to island 4.
|
|
| 2) | | | { "0-1", "1-0", "0-2", "2-3" } | { "1 1 1000 1000", "1000 1000 10 100", "1000 1000 1000 1000", "1000 1000 1000 1000" } |
| Returns: { 1, 12, 112 } | The cheapest way to get to island 3 is to purchase A and B tickets on island 0, take the A ferry to island 1, purchase C and D tickets on island 1, take the B ferry back to island 0, then the C ferry to island 2, and then the D ferry to island 3. |
|
| 3) | | | { "1-0" }
| { "793", "350" }
|
| Returns: { -1 } | |
| 4) | | | {"8-18 4-11 15-5 7-12 11-8 0-15 15-2 3-11 4-18 2-3",
"16-2 18-3 15-18 11-19 18-2 18-7 19-17 3-15 12-19",
"2-17 0-12 1-2 14-12 6-2 4-2 11-5 4-11 11-6 17-16",
"0-18 13-18 16-0 3-7 14-12 3-1 19-18 3-19 10-3 8-15",
"18-19 3-16 13-6 0-19 12-14 5-17 1-12 7-13 9-14 1-2",
"14-5 17-9 2-10 16-13 11-15 10-17 14-10 0-14 2-13",
"4-5 0-17 6-9 17-7 12-6 5-6 6-18 10-18 0-2 13-0 8-4",
"3-12 4-11 10-17 13-12 3-0 3-7 13-0 9-15 10-9 0-10" }
| {"592 219 88 529 324 86 503 610",
"2 94 8 600 34 95 6 494",
"638 301 10 246 290 97 85 74",
"118 8 939 393 450 79 317 99",
"99 806 698 740 2 26 525 818",
"95 9 615 972 23 23 5 666",
"6 448 440 710 83 4 419 496",
"4 47 182 4 185 70 718 770",
"3 321 6 855 968 65 10 6",
"173 224 300 3 79 2 707 49",
"21 10 7 10 4 9 5 8",
"6 600 4 724 7 1 960 247",
"83 16 901 50 437 780 658 2",
"763 923 125 576 45 423 3 1",
"7 324 391 898 8 59 281 973",
"9 44 49 364 78 744 43 5",
"10 62 75 418 216 90 32 919",
"764 534 778 605 80 647 821 74",
"65 449 102 867 421 94 6 7",
"67 155 362 789 189 316 107 595" }
|
| Returns:
{ 170, 160, 155, 173, 145, 150, 158, 168, 153, 145, 162, 88, 162, 86, 163, 159, 153, 150, 104 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Being second best usually warrants respect and some degree of popularity. Unfortunately, sometimes being second is worse than being last. This phenomenon can be seen in graph theory path-finding algorithms. No one ever seems to pay attention to second best paths! This is unfair and it leaves many almost optimal paths unseen. You wish to change all this by writing an algorithm that detects the second shortest path length in a directed graph, which is not equal to the optimal shortest path length. For the purpose of this problem, a path does not need to have distinct nodes and the length of a path is the sum of all edge weights from the starting node to the ending node.
Write a class Paths that contains the method secondBest, which takes a String[] graph, int from and int to and returns the length of the second shortest path from the node from to the node to. graph will contain digits that represent the weights of edges or 'X', which means there is no edge at all. The jth character of the ith element in graph represents the weight of the edge from node i to node j (i and j are zero-indexed). If no second best path exists, return -1.
| | Definition | | Class: | Paths | Method: | secondBest | Parameters: | String[], int, int | Returns: | int | Method signature: | int secondBest(String[] graph, int from, int to) | (be sure your method is public) |
| | | | Notes | - | The weight of an edge from node j to node i need not be the same as the weight of an edge from node i to node j. | - | Nodes may have an edge pointing to themselves with non-zero weights. | | Constraints | - | graph will contain between 1 and 50 elements, inclusive. | - | Each element of graph will contain the same number of characters as the number of elements in graph. | - | Each element of graph may contain only digits or 'X'. | - | from will be between 0 and the number of elements in graph-1, inclusive. | - | to will be between 0 and the number of elements in graph-1, inclusive. | | Examples | 0) | | | {"01111",
"10111",
"11011",
"11101",
"11110"} | 0 | 0 |
| Returns: 2 | In this case, the best path may be no path at all or any number of moves from node 0 back to itself. In either case, the best path is 0. The second best path has a length of 2 and can be achieved by moving from node 0 to any other node, and then back to node 0. |
|
| 1) | | | | Returns: 1 | Since the starting node is the ending node, the best path is 0. The second best path is to move once from node 0 back to itself. |
|
| 2) | | | {"X1119",
"1X119",
"11X19",
"111X1",
"9111X"} | 0 | 4 |
| Returns: 3 | |
| 3) | | | {"X1110",
"1X111",
"11111",
"111X1",
"0111X"} | 0 | 4 |
| Returns: 2 | |
| 4) | | | {"X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"XX9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X9X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X99X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X9999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X99999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X9999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X99999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X9999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X99999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X9999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X99999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X999999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X9999999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X99999999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X999999999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X9999999999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X99999999999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXXX",
"X999999999999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXXX",
"X9999999999999999999999X9XXXXXXXXXXXXXXXXXXXXXXXXX",
"X99999999999999999999999X9XXXXXXXXXXXXXXXXXXXXXXXX",
"X999999999999999999999999X9XXXXXXXXXXXXXXXXXXXXXXX",
"X9999999999999999999999999X9XXXXXXXXXXXXXXXXXXXXXX",
"X99999999999999999999999999X9XXXXXXXXXXXXXXXXXXXXX",
"X999999999999999999999999999X9XXXXXXXXXXXXXXXXXXXX",
"X9999999999999999999999999999X9XXXXXXXXXXXXXXXXXXX",
"X99999999999999999999999999999X9XXXXXXXXXXXXXXXXXX",
"X999999999999999999999999999999X9XXXXXXXXXXXXXXXXX",
"X9999999999999999999999999999999X9XXXXXXXXXXXXXXXX",
"X99999999999999999999999999999999X9XXXXXXXXXXXXXXX",
"X999999999999999999999999999999999X9XXXXXXXXXXXXXX",
"X9999999999999999999999999999999999X9XXXXXXXXXXXXX",
"X99999999999999999999999999999999999X9XXXXXXXXXXXX",
"X999999999999999999999999999999999999X9XXXXXXXXXXX",
"X9999999999999999999999999999999999999X9XXXXXXXXXX",
"X99999999999999999999999999999999999999X9XXXXXXXXX",
"X999999999999999999999999999999999999999X9XXXXXXXX",
"X9999999999999999999999999999999999999999X9XXXXXXX",
"X99999999999999999999999999999999999999999X9XXXXXX",
"X999999999999999999999999999999999999999999X9XXXXX",
"X9999999999999999999999999999999999999999999X9XXXX",
"X99999999999999999999999999999999999999999999X9XXX",
"X999999999999999999999999999999999999999999999X9XX",
"X9999999999999999999999999999999999999999999999X9X",
"X99999999999999999999999999999999999999999999999X9",
"XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"} | 0 | 49 |
| Returns: 459 | |
| 5) | | | | Returns: -1 | A second best path does not exist in this graph. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Every spring, your grandmother plants a garden. Since she is in her later years, she often needs help with watering the plants. Of course, you always offer a helping hand but you also have a more permanent solution. You call your invention "I can't believe it works!", and it is about to bring a revolution to the garden-watering industry. Your creation is a robot that will water the garden, taking the least time possible.
Your job is to implement part of the software that will guide the robot. The robot may only move and see in the four cardinal directions: North, East, West or South. The garden will be represented by the String[] garden, which consists of digits, dots, a single 'R' and a single 'W' (quotes for clarity). Each digit will represent how many units of water a plant in that location needs. The character '.' marks an empty location, 'R' marks the robot's current location and 'W' marks the location of a well (quotes for clarity). During the robot's travels, it may not step on any plants (that would only upset your grandmother) or the well (that would really make you angry).
Given garden and carryLimit, calculate and return the minimum amount of time needed to water all plants if the robot may only carry at most carryLimit units of water at any time. It takes n time units to water a plant that needs n units of water. In addition, m time units are needed to get m units of water from the well and each step in any direction takes 1 unit of time. The robot can change the direction in which it is facing instantly, taking zero time units. The robot may only water a plant if it is directly next to it; the same requirement must be met when taking water from the well. Finally, the robot may water only one plant at a time. If it is impossible to water all plants, return -1. | | Definition | | Class: | WaterBot | Method: | minTime | Parameters: | String[], int | Returns: | int | Method signature: | int minTime(String[] garden, int carryLimit) | (be sure your method is public) |
| | | | Notes | - | Initially, the robot is holding zero units of water. | - | The robot may not step out of the garden at any time. | | Constraints | - | garden will contain between 1 and 50 elements, inclusive. | - | Each element in garden will contain between 1 and 50 characters, inclusive. | - | All elements in garden will be of the same size. | - | Each element in garden may only contain the following characters: 'W', 'R', '.', '1', '2', '3', '4', '5' (quotes for clarity). | - | garden will contain exactly one occurrence of 'R' and 'W'. | - | garden will contain between 0 and 4 digits, inclusive. | - | carryLimit will be between 1 and 5, inclusive. | | Examples | 0) | | | {"5....",
".....",
".....",
".....",
"...RW"} | 5 |
| Returns: 16 | The robot begins its journey next to the well. It takes 5 units of time to collect 5 units of water. Then, it takes 6 units of time to move to the only plant that needs watering. At last, the robot will water the plant for 5 units of time. The total time required is 16 units. |
|
| 1) | | | {"3.2..",
".....",
".....",
"....R",
"....W"} | 5 |
| Returns: 16 | Once again, the robot is next to the well. The most efficient schedule is to collect 5 units of water, then water both plants without returning to the well. |
|
| 2) | | | {".5555",
".....",
".....",
"....R",
"....W"} | 3 |
| Returns: 85 | |
| 3) | | | {"R.....55",
"......55",
"........",
"........",
"........",
".......W"} | 2 |
| Returns: -1 | The robot cannot reach the top-right plant without stepping on at least one other plant. |
|
| 4) | | | {"R.......",
"........",
"....5...",
"...5W5..",
"....5...",
"........"} | 4 |
| Returns: -1 | The robot cannot get any water from the well. |
|
| 5) | | | {".1",
".2",
".3",
".4",
"R.",
"W."} | 5 |
| Returns: 28 | |
| 6) | | | {"R....",
".....",
".....",
".....",
"....W"} | 5 |
| Returns: 0 | There are no plants to water here. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A matrix is a rectangular (two-dimensional) array of numbers. A vector is a linear sequence of numbers, a one dimensional array.
There are row vectors and column vectors. We use only column vectors in this problem. How to multiply a matrix by a column vector (vector on the right) to yield another column vector is described in the notes section.
A right eigenvector, X, of a square matrix, A, is a (nonzero) column vector that satisfies AX=kX, where k is a nonzero scalar constant called an eigenvalue (usually represented by lambda). In other words, multiplying the matrix by the eigenvector yields a vector which is proportional to the eigenvector. For the vectors to be proportional by the factor k, each element of the resulting vector is k times the corresponding element of X, k*X[i].
Given a matrix, find the smallest right eigenvector of that matrix where each element of the eigenvector is an integer. Smallest is defined by the L0 norm, which is the sum of the absolute values of the elements of the vector. The first nonzero element of the eigenvector should be positive (if it is not positive, multiply all the elements by -1). If there are equally small integer eigenvectors, return the lexicographically first eigenvector. A vector v1 comes before v2 lexicographically if v1 has a smaller element in the first position for which the two vectors differ.
To simplify this problem, the input matrix, A, will be at most 5 by 5 and will always have an integer eigenvector with L0 norm between 1 and 9 inclusive.
Some valid vectors (L0 = 5, in lexicographic order):
{0,0,0,5}
{0,0,2,-3}
{1,0,-2,2}
{1,0,1,3}
Some invalid vectors:
{0,0,0} vector must be nonzero
{0,0,-1} first nonzero element must be positive
{0,-1,3} first nonzero element must be positive
{-1,2,3} first nonzero element must be positive
For example:
{"1 0 0 0 0",
"0 1 0 0 0",
"0 0 1 0 0",
"0 0 0 1 0",
"0 0 0 0 1"}
The identity matrix, I, is unusual in terms of eigenvalues and eigenvectors. All the eigenvalues are 1, and every nonzero vector is an eigenvector. The first one in our ordering is {0,0,0,0,1}. | | Definition | | Class: | EigenVector | Method: | findEigenVector | Parameters: | String[] | Returns: | int[] | Method signature: | int[] findEigenVector(String[] A) | (be sure your method is public) |
| | | | Notes | - | To calculate matrix-column vector multiplication, AX = Y, where A is an n by n matrix, and X and Y are length n column vectors, use something like: for(i) { Y[i] = 0 ; for(j) { Y[i] = Y[i] + A[i,j] * X[j] ; } } | | Constraints | - | A will be the string representation of an n by n integer matrix where n is between 2 and 5 inclusive. Each element of A represents a row of the matrix with n integer elements in each row. | - | A will contain exactly n elements (rows). | - | Each element of A will contain exactly n integers (characters between '0' and '9' inclusive, optionally preceded by a minus sign '-'), each separated by a single space. | - | There will be no leading or trailing spaces in each element of A. For example: "10 2 0 -4" (quotes for clarity) | - | Each of the integers in A will be between -1000 and 1000 inclusive, with no leading zeros. | - | The matrix A will have at least one integer right eigenvector having an L0 norm between 1 and 9 inclusive. | | Examples | 0) | | | {"1 0 0 0 0",
"0 1 0 0 0",
"0 0 1 0 0",
"0 0 0 1 0",
"0 0 0 0 1"} |
| Returns: { 0, 0, 0, 0, 1 } | The example from above, the identity matrix. |
|
| 1) | | | {"100 0 0 0",
"0 200 0 0",
"0 0 333 0",
"0 0 0 42"} |
| Returns: { 0, 0, 0, 1 } | All diagonal matrices (including the identity matrix) will have the same answer: n-1 zeros followed by a one. |
|
| 2) | | | {"10 -10 -10 10",
"20 40 -10 -10",
"10 -10 10 20",
"10 10 20 5"} |
| Returns: { 1, -5, 2, 0 } | |
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
If you've driven much on North American highways, especially west of the
prairies, you're familiar with the sight of trucks hauling great stacks of
logs. In the old days, before internal combustion was a going concern,
logs were transported at a more sedate pace by floating them down a
river. This was all very picturesque until the logs jammed together so
tightly that no movement was possible. Then they had to send someone out
on the river to loosen up the works with dynamite. Skipping nimbly from
log to log, a lumberjack would find the crux of the logjam and set an
explosive charge. After lighting the fuse, he had to skip quickly back
to shore. The lumberjack was prepared to plunge briefly into the river
as a shortcut, but he really didn't like to get wet. The main thing was
to get away quickly.
You are given a String[] describing a stretch of river in
which the character '|' stands for a log, '.' stands for water, and '+'
is where the lumberjack is standing on a log. The lumberjack can reach
safety by stepping up, down, left, or right between adjacent logs until he
reaches the far left or far right, at which point he is immediately safe
from the blast. (The "far left" is the first character in each String,
and the "far right" is the last character in each String.) Stepping up or
down onto a log takes him one second, while stepping left or right onto
a log takes two seconds. The lumberjack may step on no more than one
square of water in his entire trip, at a cost of three seconds instead
of the usual one or two. Return the minimal number of seconds it will
take the lumberjack to reach safety, or -1 if he cannot do so.
| | Definition | | Class: | LumberjackHack | Method: | timeToShore | Parameters: | String[] | Returns: | int | Method signature: | int timeToShore(String[] riverMap) | (be sure your method is public) |
| | | | Constraints | - | riverMap contains between 1 and 50 elements, inclusive | - | the first element of riverMap is between 3 and 50 characters long | - | every element of riverMap has the same length as the first element of riverMap | - | for every element of riverMap, each character must be '|', '.', or '+' | - | riverMap contains exactly one occurrence of the character '+' | | Examples | 0) | | | | Returns: 3 | The lumberjack can reach safety in 1+2 = 3 seconds by stepping down and to the left. |
|
| 1) | | | | Returns: 0 | The lumberjack is already safe at the right edge. |
|
| 2) | | | {"....|||",
"....|..",
"...||..",
"||.+...",
"...|...",
"...||||"} |
| Returns: 7 | The lumberjack could reach the upper right corner in 1+2+1+1+2+2 = 9 seconds or the lower right in 1+1+2+2+2 = 8 seconds, but his best choice is to reach the left border in 3+2+2 = 7 seconds. |
|
| 3) | | | {"||.|....",
"........",
".|.+|..|",
"...|....",
"|..|.|.|"} |
| Returns: -1 | The lumberjack had better not light that fuse. |
|
| 4) | | | {".........|.|.|.|.|..||...||.|..|.||...|.|.|||...||",
".||.||...||..|||.....|.||||...|.|.|.|.|..|...|.|||",
"||.|.|..||.||....|.....|.||.|||||.|.|.||.|||||.|..",
"|.....|.|.||||.||..|.|..|..|.|||||.....||.|.||....",
"..|..||...||.|.......|||+||.||||....||||.....|..||",
"...||..||||.|......||..|.|||||.|.|||||.||..|||...|",
"|||...|..|..|.|||.||.|..|...||.|||..|..||.|.||....",
"|..|||||||||.||.....|..|.|.|||||...||...|.|.|||||.",
".|..||...|||............|.|..|||.||.|||.||..||.|||",
".|.|||...||..|..|...||.||..|..|||||.|.|...||..||.|",
"||||.|||.|..||||..|..||...|..||.|.||||...|...|.|..",
".||..||...|.....|||.|||||..||......|.||.||.|..||..",
"|.|||....|||||.|..|..|.|||..|.||.||.|.|.|.|..||...",
".||.||||||...||||||..||....|..||.|||..||...|.|||||",
".||||.|....|...|.||..||..|||.|||||....|...||.|.||.",
".|...|..||....||...|.||||.....||||.||.|||||||..|||",
".||||...|...|..||...|...|...|.|..|.|.|..|..|||.||.",
".|.|||..||||||||........|||||||||.|.|........|||||",
".....|...||.||...|||...||||..|||...||....||..||.||",
"||...||..||.||...||...||||..|.|..|...|||..||..|||.",
"|..||||.||..|...|....||||||...|||.|.|||||..|||...|",
".....|||..||.|||.....||..||||...|||||.||.|.||..|||",
"|..|.||..|..||..||..|...|..|.||..||||..|...||..|..",
"||.|..|.|||||...|...|.|..|||||...|.......|.||.||||",
".|.....|.|||||.....|||...|..|||||...|.||..||.|||.|",
".|...||....|||...|||.||.|.|......|........|.||||||",
"..|.|.|.|||||..|||..|.........|...|.|.|...||.....|",
".|.|.|.|..|.|||||||||||.|.|||....|||||...|.||||.|.",
"|.|||....|.||||..||......|..|||||.....||.|..||..|.",
"||.||.|||.|......|..|.|...|..||.|||..||.....|.|..|",
".||||..|.|.||||.|||.||.||.....|....|....||...|..||",
".....|||...||.||.||....|.||..||....|....|||||.|..|",
"|.|.|||||...|.||..|..|..|.|..|.|........||..|.|.||",
"....|..|.|..|.||||||....||||.|.||||||.|.|.|.......",
"||||....|..|...||..||||||||...|.|||||.|.|||.|...||",
"|...|.|..|..|..|....|..||..|||....||..||..|..|..|.",
"|||||....|.||.|..|.||..|||..|.|.|..||.|...|.|..||.",
"..|.|||.|.||..|...|||.|..|||..||...||...||||.....|",
"..||||.|.|.....|||..||||..|.||||..|..|||.....||.||",
"|..|||||....|........|.||||.||..||||.|....|||||||.",
".|...||.|.||..|.|....|.||..|.|....|.|.||.||.||.|..",
".|..|..|.||||.||||....|||.....|.|...|.|...|...||..",
"|..|||..|.||.|||..||.....|.|..|.|.|...|.....|.....",
"||..||.|...|.||...|..|..||.|||.||.|.||...|....|||.",
".|....|.|||.|..|||..|.....|.||.||...|...||.......|",
".||..|||.|.|....|||...|..|.||.||.|.|...|||||.|.|.|",
"|.|.||.||.|.|.||.|||.||....||.|||||.||.|.|||......",
"|...|||...|.||||....|.||.||.|.........|..||.|..||.",
".|.....|.|.|....||.||...|||.|..||...||.|||.||.|.|.",
"||.||.|||.|||..||......|......||..||||.|..||.||||."} |
| Returns: 63 | |
| 5) | | | | 6) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Consider a simple Input/Output system. The system input consists of a button that you can press, while the system output consists of an audible beep. The system logic consists of a state machine that transitions from one state to the next every time the button is pressed. At the last state, the machine transitions to the first state when the button is pressed. On some state transitions, the audible beep is sounded, and on others it is not. The pattern of beeps mapped to states is known ahead of time.
You are given a String pattern of beeps for state transitions. The i-th character of pattern (0-based index) will be a 'B' if there is a beep when state i is entered, and will be a 'N' if there is no beep. You are also given an int targetState to achieve, but not the starting state. Return the worst case scenario of the most number of times you must press the button to guarantee the machine is in the targetState. If it is not possible to always ensure you can end on the targetState, return -1.
| | Definition | | Class: | SimpleIO | Method: | worstCase | Parameters: | String, int | Returns: | int | Method signature: | int worstCase(String pattern, int targetState) | (be sure your method is public) |
| | | | Constraints | - | pattern will have between 2 and 50 characters, inclusive. | - | pattern will only contain the characters 'B' and 'N'. | - | targetState will be between 0 and the length of pattern - 1, inclusive. | | Examples | 0) | | | | Returns: 4 | In the worst case, the first state is state 2. In this case, you press the button 2 times before we narrow down that the state is now state 1. You must now press the button 2 more times to get back to state 0. |
|
| 1) | | | | Returns: -1 | In this case, we can only tell if we are on an even or odd state, we cannot determine the exact state. |
|
| 2) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | This problem contains HTML superscripts and images which will not display correctly for plugin users
Given two real numbers x1 and x2, calculate an approximation to the integral of e-x^2 evaluated between the limits from x1 to x2, which is accurate to the nearest 0.00001.
Return the answer in a String, as a fixed point number with exactly five digits to the right of the decimal point and exactly one digit to the left of the decimal point.
For example: x1 = -0.5 and x2 = 0.5 returns "0.92256" | | Definition | | Class: | NumericalIntegral | Method: | integrate | Parameters: | double, double | Returns: | String | Method signature: | String integrate(double x1, double x2) | (be sure your method is public) |
| | | | Notes | - | - e-x^2 can be calculated in C++ with exp(-x*x) in math.h.
- e-x^2 can be calculated in C# with Math.Exp(-x*x). The Math class is in the System namespace.
- e-x^2 can be calculated in Java with Math.exp(-x*x).
- e-x^2 can be calculated in Visual Basic with Exp(-x*x) in the System.Math namespace.
| - | 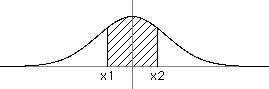 | - | The integral of a function is the area inside the closed figure formed by (on the top) the function between the limits of x=x1 and x=x2, (on the sides) vertical line segments at x=x1 and x=x2, and (on the bottom) the portion of the x axis between x=x1 and x=x2. This is shown by the shaded area above (the graph shows the function we are integrating, e-x^2). | - | The integral of e-x^2 is known to have no closed form, so don't waste time looking in a table of integrals for an exact formula. | - | Because of the 2e-6 constraint, about 40% of randomly chosen x1 and x2 values will be too close to a possible rounding error and will be rejected. This is not an error. It gives you more room for numerical errors. | | Constraints | - | x1 will be less than x2. | - | x2-x1 will be between 0.00001 and 1.00000 inclusive. | - | x1 will be between -10.0 and 10.0 inclusive. | - | x2 will be between -10.0 and 10.0 inclusive. | - | To avoid rounding errors the inputs x1 and x2 must be chosen so that the answer is not within 2e-6 of 0.000005 + a multiple of 0.00001 | | Examples | 0) | | | | Returns: "0.92256" | The example from above. This is the largest possible answer given the constraints of this problem. |
|
| 1) | | | | 2) | | | | Returns: "0.00000" | Values are very small out here. |
|
| 3) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
The city of Konigsberg is famous for its bridges. The Tour de Konigsberg
is an annual bicycle race over a route that crosses each bridge exactly once.
You are responsible for planning the route for this year's race.
A series of checkpoints numbered from 0 to N-1 have been set up around the city, with
exactly one bridge between each pair of neighboring checkpoints.
For example, here is a picture of the bridges and checkpoints from last year's race.
0-------1
| |
| |
| |
2-------3
|\ /|
| \ / |
| \ / |
4---5---6
(There has been substantial construction since last year, however, so the bridges and checkpoints for this year's race
may be completely different.)
The route for the race must begin and end at checkpoint 0, and must proceed across the bridges from checkpoint
to checkpoint, crossing each bridge exactly once. If the checkpoints are placed poorly, it might not be possible
to come up with such a route, so the planner for the first Tour de Kongisberg, Leonhard Euler, was careful
to leave instructions for how to lay out the checkpoints to guarantee that a successful race route will be possible.
He decreed that the checkpoints should be placed with an even number of bridges (greater than zero) leaving each checkpoint,
and furthermore that every checkpoint must be reachable from checkpoint 0. The checkpoints for this year's race
were placed with Euler's instructions in mind. Now your task is to plan the race route. Fortunately, Euler also
left notes telling you how.
You begin by laying out a tentative route that begins and ends at checkpoint 0. At every step
along the way, this initial route leaves the current checkpoint via the unused bridge that leads to the lowest
numbered checkpoint. When the tentative route returns to checkpoint 0, it may or may not have used all the bridges. If it has, you
are done. Otherwise, you need to extend the route to use more bridges as follows.
Beginning at checkpoint 0, you follow the tentative route until
you reach a checkpoint--call it checkpoint K--that has an unused bridge leading
out from it. (Note that K could be 0.) Lay out a new route by starting at checkpoint K and
repeatedly taking the unused bridge that leads to the lowest numbered checkpoint until you return to checkpoint K.
Now splice this new route into the tentative route after the first occurrence of K.
Repeat this extension process on the new larger tentative route until all the bridges have been used. Note that
you always restart the search for a checkpoint with an unused bridge back at the beginning of the tentative route;
you do not continue from where you left off.
For example, in last year's race, the initial tentative route travelled from checkpoint 0 to 1 to 3 to 2.
Marking the used bridges with x's we get
0-x-x-x-1
| |
x x
| |
2-x-x-x-3
|\ /|
| \ / |
| \ / |
4---5---6
Some bridges have not been used, so we retrace the tentative route until we reach checkpoint 3.
Then we lay out a new route from checkpoint 3 to 5 to 2 to 4 to 5 to 6 to 3. Splicing this new
route back into the original route, we end up with the final race route: 0-1-3-5-2-4-5-6-3-2-0.
You will be given a String[] bridges that contains information about which checkpoints are
connected by bridges. The i-th character of element j of bridges is '1' if there is a bridge between
checkpoints i and j. Otherwise, it is '0'. Note that both i and j are zero based. All bridges are two-way so
a bridge between checkpoints i and j appears in bridges in both directions, from i to j and from j to i.
You will return a int[] containing the sequence of checkpoints visited by the race route.
| | Definition | | Class: | EulerianRace | Method: | planRoute | Parameters: | String[] | Returns: | int[] | Method signature: | int[] planRoute(String[] bridges) | (be sure your method is public) |
| | | | Constraints | - | bridges contains between 3 and 30 elements, inclusive. | - | Every element of bridges contains the same number of characters as elements in bridges. | - | Every element in bridges contains only the characters '0' and/or '1'. | - | Every element in bridges contains a positive even number of '1's. | - | Character i of element i of bridges is '0'. | - | Character i of element j of bridges is equal to character j of element i of bridges. | - | Every checkpoint is reachable from checkpoint 0. | | Examples | 0) | | | { "0110000",
"1001000",
"1001110",
"0110011",
"0010010",
"0011101",
"0001010" } |
| Returns: { 0, 1, 3, 5, 2, 4, 5, 6, 3, 2, 0 } | The example from last year's race. |
|
| 1) | | |
{ "0101000000",
"1010110000",
"0101000000",
"1010000011",
"0100011100",
"0100100000",
"0000100100",
"0000101000",
"0001000001",
"0001000010" } |
| Returns: { 0, 1, 4, 6, 7, 4, 5, 1, 2, 3, 8, 9, 3, 0 } | In this example, the bridges and checkpoints are arranged as follows:
0-------1---4---6
| |\ |\ |
| | \ | \ |
| | \| \|
3-------2 5 7
|\
| \
| \
8---9
The initial tentative route is 0-1-2-3-0, which is then extended with 1-4-5-1 into 0-1-4-5-1-2-3-0. Next, it is extended with 4-6-7-4 into 0-1-4-6-7-4-5-1-2-3-0. Finally,
it is extended with 3-8-9-0 into 0-1-4-6-7-4-5-1-2-3-8-9-3-0. |
|
| 2) | | | { "01111",
"10111",
"11011",
"11101",
"11110" } |
| Returns: { 0, 3, 2, 4, 3, 1, 4, 0, 1, 2, 0 } | A bridge between every possible pair of checkpoints. |
|
| 3) | | | { "01000000001",
"10100000000",
"01010000000",
"00101000000",
"00010100000",
"00001010000",
"00000101000",
"00000010100",
"00000001010",
"00000000101",
"10000000010" } |
| Returns: { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 0 } | The bridges and checkpoints are arranged in a big circle. |
|
| 4) | | | { "00011",
"00101",
"01001",
"10001",
"11110" } |
| Returns: { 0, 3, 4, 1, 2, 4, 0 } | |
| 5) | | | {"011111111",
"101000000",
"110000000",
"100010000",
"100100000",
"100000100",
"100001000",
"100000001",
"100000010"} |
| Returns: { 0, 7, 8, 0, 5, 6, 0, 3, 4, 0, 1, 2, 0 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
This problem statement contains an image which will not display correctly for plugin users.
You will be given an undirected graph which may contain several smaller copies of itself. These copies, or "children", will in turn recursively contain the same number of children, creating a graph with an infinite number of nodes. You are to find the shortest path through this graph.
At each level down, the edges of children have half the weight of their parent, rounded down. Once you recurse deep enough into the graph, all edges eventually have a weight of zero.
For example, consider the graph pictured above with 4 top-level nodes (A, B, C, and D), and 2 recursive children. Notice that each child has two smaller copies inside of it, and each of those children has two smaller copies inside of it, repeating infinitely. In this graph, there is a direct path from node A to B with distance 800. However, there is a shorter path from A to B that goes through C and D in the left-side child of the top-level graph. Within the left-side child, the path from C to D goes through A and B in its right-side child. The length of this 5-edge path is 4 + 2 + 200 + 2 + 4 = 212.
However, there is an even shorter path, bypassing the third-level edge from A to B with cost 200. By replacing this edge with the same 5-edge path two levels down, there is a 9-edge path with distance 4 + 2 + 1 + 0 + 50 + 0 + 1 + 2 + 4 = 64.
Similarly, the edge with cost 50 can be bypassed again by recursing farther into the graph. By going down deep enough to the point where all edges have zero weight, the edge cost can be bypassed completely, resulting in a shortest path from A to B of length 4 + 2 + 1 + 0 + ... + 0 + 1 + 2 + 4 = 14.
Graphs will contain up to 10 top-level nodes ('A' through 'J'), and each parent graph at each level may contain up to 9 child graphs, smaller copies of itself, numbered ('1' through '9'). The graph will be specified by a list of edges in the top-level graph. Edges are specified by two nodes, followed by a weight. Either node may optionally be followed by a single digit, representing a connection to a node in the corresponding recursive copy of the graph.
The graph pictured above would be specified as:
"A B 800" (connecting A and B in every copy of the graph)
"A C1 4" (connecting A in every copy to C in its first child)
"D1 B 4" (connecting B in every copy to D in its first child)
"C A2 4" (connecting C in every copy to A in its second child)
"B2 D 4" (connecting D in every copy to B in its second child)
There will be no edge that directly connects two nodes of the same child. For example, the edge "A3 B3 10" would be illegal, but the edge "A4 B5 20" is allowed. A top-level edge implies corresponding edges in all copies of the graph at all levels. Likewise, an edge connecting a top-level node to a copy implies corresponding connections between parents and children at all levels, and an edge connecting nodes of two different children implies corresponding edges between nodes in those two children at all levels.
Given a list of edges, a starting node, and an ending node, return the length of the shortest path between the starting and ending nodes. Return -1 if there is no such path, or if the distance of the shortest path is greater than 1,000,000,000.
| | Definition | | Class: | RecursiveGraph | Method: | shortestPath | Parameters: | String[], char, char | Returns: | int | Method signature: | int shortestPath(String[] edges, char start, char end) | (be sure your method is public) |
| | | | Notes | - | The shortest path may descend recursively down many levels. | - | All edges are undirected. The order of a pair of nodes in the input is not significant. | - | There can be no edges that connect nodes between levels with depths that differ by more than 1. | | Constraints | - | edges will contain between 1 and 50 elements, inclusive. | - | Each element of edges will be formatted as "<node> <node> <weight>". | - | Each <node> is a character between 'A' and 'J', inclusive, optionally followed by a digit between '1' and '9', inclusive. | - | Each <weight> is an integer from 0 to 1000, inclusive, and will not have any leading zeros. | - | There will be at most 10 top-level nodes (letters 'A' through 'J'). | - | At each level, there will be at most 9 copies of the graph embedded directly in itself (nodes with digits '1' through '9'). | - | There will not be more than one edge between the same two nodes. | - | There will not be an edge connecting two nodes of the same recursive copy, i.e. "A1 B1 5" would be illegal input. | - | start and end will each be a character between 'A' and 'J', inclusive, and will be nodes which appear in edges. | | Examples | 0) | | | {"A B 20",
"C D 13",
"A C1 1",
"D1 C2 2",
"D2 B 3"} | 'A' | 'B' |
| Returns: 18 | This graph has 4 top-level nodes, plus two copies of itself. There are two paths from A to B. The direct path across the first edge has a cost of 20. However, the path through the two copies (A->C1->D1->C2->D2->B) has a weight of 1 + 6 + 2 + 6 + 3 = 18. Remember, the edges from C to D in the children have half the weight (rounded down) of the edge from C to D in their parent. |
|
| 1) | | | {"A B 800",
"A C1 4",
"D1 B 4",
"C A2 4",
"B2 D 4"} | 'A' | 'B' |
| Returns: 14 | This is the example from the problem statement. |
|
| 2) | | | {"A A1 1",
"B B1 1"} | 'A' | 'B' |
| Returns: -1 | No matter how far down you recurse, there is no path from A to B. |
|
| 3) | | | { "A E1 10", "H2 J 11",
"E F 50", "F1 G2 5", "G H 60",
"A C4 12", "D4 J 13",
"C E1 14", "F1 D 15",
"E G8 6", "F H8 8" } | 'J' | 'A' |
| Returns: 49 | |
| 4) | | | { "E H 3", "C J 3", "D E9 0", "H9 A 0", "A C3 0", "J3 F 0" } | 'D' | 'F' |
| Returns: 2 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
On a plotter, the mechanical arm that draws on paper with a pen has a motor that can move it back and forth across the paper sideways. The plotter also has a paper motor, which can move the paper orthogonally to the plotter's arm, which effectively is like moving the pen up and down the paper. The paper is square, and is oriented so its lower edge is parallel to the arm. This allows the plotter to move to any coordinates using the two motors. Both motors can be active simultaneously, and each motor can move the pen up to one pixel per millisecond in relation to the paper (it's possible for a motor to run slower).
Given the list of lines that the plotter must put on the paper, return the minimum time it takes to plot the given lines, in milliseconds. The plotter's pen starts at the coordinates (0,0), and after drawing all the lines, must return to (0,0). Note that the plotter cannot draw partial lines. Each element of lines will be in the form "x1 y1 x2 y2". This represents a line from point (x1,y1) to point (x2,y2). It takes 0 time to lower the pen down on the paper, and to raise it off the paper.
| | Definition | | Class: | LinePlotter | Method: | timeToPlot | Parameters: | String[] | Returns: | int | Method signature: | int timeToPlot(String[] lines) | (be sure your method is public) |
| | | | Notes | - | There can be 0-length lines, which are drawn by moving to the designated location, lowering the pen and without moving, raising the pen. | | Constraints | - | lines will contain between 1 and 15 elements, inclusive. | - | Each element of lines will be of the form "x1 y1 x2 y2", where each value is an integer between 0 and 9999, inclusive. | - | There will be no extra leading zeros in any of the numbers in lines. | - | There will be no repeated elements in lines. This includes lines which are reversed. For example, if "x1 y1 x2 y2" appears, then "x2 y2 x1 y1" cannot. | - | No two lines can intersect at more than one point. | | Examples | 0) | | | | Returns: 3 | This is a line which draws a diagonal across the bottom corner of the paper. The line can be drawn by first running the arm motor to bring the pen over to (1,0) in 1 millisecond. Then the paper motor can be run simultaneously with the lateral motor to draw the line from (1,0) to (0,1) in one more millisecond. Finally, the paper motor brings the pen back to (0,0) in one more millisecond. |
|
| 1) | | | {"0 0 5 5", "5 5 0 5", "0 100 0 4"} |
| Returns: 205 | The best path first draws the line from (0,0) to (5,5). Since both motors can move at top speed, this line takes 5 milliseconds to draw. Without even picking up the pen, we can draw the line from (5,5) to (0,5) in 5 milliseconds. Finally, to draw the final line, we lift the pen, go all the way up to (0,100), and draw the line to (0,4). This adds 95 + 96 = 191 milliseconds. Finally, we lift the pen and move back to (0,0), adding an additional 4 milliseconds. The total time is 5 + 5 + 95 + 96 + 4 = 205 milliseconds. |
|
| 2) | | | {"4647 8139 5695 458", "346 6987 5003 8789", "4955 4552 8216 4667",
"1676 5564 9137 703", "2722 911 3594 2734", "1718 6222 4828 6335",
"1265 5243 3677 2489", "2809 718 6675 9926", "5626 9254 8843 2486",
"4938 6286 9083 1621", "2058 9050 3421 6083", "1648 1890 6058 2896",
"6598 6029 8159 4543", "1279 634 5472 6714", "2295 4885 9765 5706"} |
| Returns: 92635 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
NOTE: This problem statement contains subscripts and superscripts which may not display properly for plugin users.
The elements of a two-dimensional matrix with M rows and N columns are usually stored as a linear array of length M*N. Assuming "row-major" order, elements are stored left-to-right, then top-to-bottom (the same order that we read English text on a written page). For example, the following matrix A, with M=3 and N=3:
0 1 2
3 4 5
6 7 8
is stored as: { 0, 1, 2, 3, 4, 5, 6, 7, 8 }.
The transpose of a matrix A (denoted AT) is obtained by exchanging its rows and columns. Element ATij equals Aji , and if A has M rows and N columns, AT will have N rows and M columns. For example, the transpose of the above matrix is:
0 3 6
1 4 7
2 5 8
and is stored as: { 0, 3, 6, 1, 4, 7, 2, 5, 8 }.
Computing the transpose of the square matrix "in place" (overwriting the original matrix) in this example is easy: it involves swapping 3 pairs of elements: 1 and 3, 2 and 6, and 5 and 7. Elements 0, 4, and 8 do not need to be moved.
It is a bit more tricky when the matrix is not square. For example, the matrix:
12 58 23
81 74 96
is stored as { 12, 58, 23, 81, 74, 96 }. Its transpose is:
12 81
58 74
23 96
and is stored as: { 12, 81, 58, 74, 23, 96 }. This also requires 3 swaps. (See example 1 below.)
Given M and N, return the minimum number of swaps necessary to take the transpose of a matrix of that size in place.
| | Definition | | Class: | Transpose | Method: | numSwaps | Parameters: | int, int | Returns: | int | Method signature: | int numSwaps(int M, int N) | (be sure your method is public) |
| | | | Notes | - | Assume that you know nothing about the actual values of the elements in the matrix, and that you cannot reduce the number of swaps by looking for pairs of identical elements. | | Constraints | - | M and N will be between 1 and 100, inclusive. | | Examples | 0) | | | | Returns: 3 | This is the example above. |
|
| 1) | | | | Returns: 3 | Initial array: { a, b, c, x, y, z }
Swap positions 1 and 2: { a, c, b, x, y, z }
Swap positions 1 and 3: { a, x, b, c, y, z }
Swap positions 3 and 4: { a, x, b, y, c, z }
After 3 swaps, the array has been transposed in place. |
|
| 2) | | | | 3) | | | | 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are arranging a weird game for a team building exercise. In this game there are certain locations that people can stand at, and from each location there are paths that lead to other locations, but there are not necessarily paths that lead directly back. You have everything set up, but you need to know two important numbers. There might be some locations from which every other location can be reached. There might also be locations that can be reached from every other location. You need to know how many of each of these there are.
Create a class TeamBuilder with a method specialLocations that takes a String[] paths that describes the way the locations have been connected, and returns a int[] with exactly two elements, the first one is the number of locations that can reach all other locations, and the second one is the number of locations that are reachable by all other locations. Each element of paths will be a String containing as many characters as there are elements in paths. The i-th element of paths (beginning with the 0-th element) will contain a '1' (all quotes are for clarity only) in position j if there is a path that leads directly from i to j, and a '0' if there is not a path that leads directly from i to j.
| | Definition | | Class: | TeamBuilder | Method: | specialLocations | Parameters: | String[] | Returns: | int[] | Method signature: | int[] specialLocations(String[] paths) | (be sure your method is public) |
| | | | Constraints | - | paths will contain between 2 and 50 elements, inclusive. | - | Each element of paths will contain N characters, where N is the number of elements of paths. | - | Each element of paths will contain only the characters '0' and '1'. | - | The i-th element of paths will contain a zero in the i-th position. | | Examples | 0) | | | | Returns: { 1, 1 } | Locations 0 and 2 can both reach location 1, and location 2 can reach both of the other locations, so we return {1,1}. |
|
| 1) | | | {"0010","1000","1100","1000"} |
| Returns: { 1, 3 } | Only location 3 is able to reach all of the other locations, but it must take more than one path to reach locations 1 and 2. Locations 0, 1, and 2 are reachable by all other locations. The method returns {1,3}. |
|
| 2) | | | {"01000","00100","00010","00001","10000"} |
| Returns: { 5, 5 } | Each location can reach one other, and the last one can reach the first, so all of them can reach all of the others. |
|
| 3) | | | {"0110000","1000100","0000001","0010000","0110000","1000010","0001000"} |
| Returns: { 1, 3 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are a magician who has two different shows. You are planning a tour of a series of cities. Given the population of each city and the roads between cities, you must plan the tour such that no two cities directly connected by a road are assigned the same show and such that the difference between the number of people who see show 1 and those who see show 2 is minimized. You have to return the difference between the number of people who will see show 1 and those who will see show 2.
The roads between the cities are specified in the String[] roads. roads[i][j] is '1' if there is a road between the ith and jth cities and is '0' if there is no road. You are guaranteed that you can pull this off. (You can schedule the shows such that no two adjacent cities are assigned the same show.) Keep in mind that each city must be assigned one of the two shows. The population of each city is specified by the int[] populations whose ith element is the population of the ith city. | | Definition | | Class: | MagicianTour | Method: | bestDifference | Parameters: | String[], int[] | Returns: | int | Method signature: | int bestDifference(String[] roads, int[] populations) | (be sure your method is public) |
| | | | Notes | - | You, the magician, can travel between two cities regardless of whether or not they are connected by a road. | | Constraints | - | populations contains between 1 and 50 elements, inclusive. | - | roads contains exactly n elements, each of which has exactly n characters, where n is the number of elements in populations. | - | Each element of populations will be between 0 and 20, inclusive. | - | Each element of roads will only contain the characters '0' and '1'. | - | You are guaranteed that you can schedule the shows such that no two adjacent cities are assigned the same show. | - | No city will have a road to itself. | - | The graph is undirected. Hence, if there is a road from city a to city b then there has to be a road from city b to city a. As a result, roads[i][j] and roads[j][i] must be the same. | | Examples | 0) | | | | Returns: 5 | There are two cities of populations 15 and 20. Perform show 1 in the first and show 2 in the second or vice versa. |
|
| 1) | | | {"0100",
"1000",
"0001",
"0010"} | {2,4,2,4} |
| Returns: 0 | There are four cities of populations 2, 4, 2 and 4. Perform show 1 in the first and fourth cities and perform show 2 in the second and third cities. |
|
| 2) | | | {"0010",
"0001",
"1000",
"0100"} | {2,2,2,2} |
| Returns: 0 | |
| 3) | | | {"000",
"000",
"000"} | {6,7,15} |
| Returns: 2 | There are no roads! To keep it balanced, perform show 1 in the first and second cities and show 2 in the third city. |
|
| 4) | | | {"0000",
"0010",
"0101",
"0010"} | {8,10,15,10} |
| Returns: 3 | |
| 5) | | | {"010",
"101",
"010"} | {5,1,5} |
| Returns: 9 | |
| 6) | | | {
"01000000000000000000000000000000000",
"10100000000000000000000000000000000",
"01010000000000000000000000000000000",
"00101000000000000000000000000000000",
"00010100000000000000000000000000000",
"00001010000000000000000000000000000",
"00000101000000000000000000000000000",
"00000010100000000000000000000000000",
"00000001010000000000000000000000000",
"00000000101000000000000000000000000",
"00000000010100000000000000000000000",
"00000000001010000000000000000000000",
"00000000000101000000000000000000000",
"00000000000010100000000000000000000",
"00000000000001010000000000000000000",
"00000000000000101000000000000000000",
"00000000000000010100000000000000000",
"00000000000000001010000000000000000",
"00000000000000000100000000000000000",
"00000000000000000000000000000000000",
"00000000000000000000010000000000000",
"00000000000000000000101000000000000",
"00000000000000000000010100000000000",
"00000000000000000000001010000000000",
"00000000000000000000000101000000000",
"00000000000000000000000010100000000",
"00000000000000000000000001010000000",
"00000000000000000000000000101000000",
"00000000000000000000000000010100000",
"00000000000000000000000000001010000",
"00000000000000000000000000000101000",
"00000000000000000000000000000010100",
"00000000000000000000000000000001010",
"00000000000000000000000000000000101",
"00000000000000000000000000000000010"
} | {8,15,12,9,12,6,4,6,16,1,15,3,18,15,14,8,6,6,12,13,14,15,17,15,3,8,7,8,3,19,12,9,14,19,9} |
| Returns: 21 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are arranging a weird game for a team building exercise. In this game there are certain locations that people can stand at, and from each location there are paths that lead to other locations, but there are not necessarily paths that lead directly back. You want the paths set up such that regardless of where someone starts, there will be at least one path they can take that will return to their starting location. You already have the locations set up, and some paths connecting them. You want to know the fewest paths that you have to add such that everything is set up the way you need it.
Create a class TeamBuilding with a method fewestPaths that takes a String[] paths that describes the way the locations are currently connected, and returns an int that is the fewest number of paths that must be added. Each element of paths will be a String containing as many characters as there are elements in paths. The i-th element of paths (beginning with the 0-th element) will contain a '1' (all quotes are for clarity only) in position j if there is a path that leads directly from i to j, and a '0' if there is not a path that leads directly from i to j.
| | Definition | | Class: | TeamBuilding | Method: | fewestPaths | Parameters: | String[] | Returns: | int | Method signature: | int fewestPaths(String[] paths) | (be sure your method is public) |
| | | | Notes | - | There may be paths that leads directly from one location to that same location. | | Constraints | - | paths will contain between 2 and 20 elements, inclusive. | - | Each element of paths will contain only the characters '0' and '1'. | - | Each element of paths will contain as many characters as there are elements in paths. | | Examples | 0) | | | | Returns: 1 | There are paths leaading from location 0 to location 1, and from location 1 to location 0. By adding a path from location 2 to itself, all locations will be able to follow a set of paths that lead back to themselves. |
|
| 1) | | | {"0110",
"0001",
"0101",
"1000"} |
| Returns: 0 | There already exists a set of paths for each location that leads back to itself. |
|
| 2) | | | {"00101",
"00010",
"00010",
"10000",
"00000"} |
| Returns: 1 | |
| 3) | | | {"001000000",
"000000010",
"000001000",
"000010010",
"000000000",
"000000010",
"000000000",
"100000001",
"010000100"} |
| Returns: 2 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
In some popular word puzzles from newspapers, you are to convert a word into another word by either swapping letters, or changing one letter to another. However, one constraint is that the characters formed by each move MUST be a real word.
Your method will be given a String source that should be converted to a String target. You will also be given a String[] dictionary, where each element is a word you are allowed to use in the conversion. At each step, you can either swap two (not necessarily adjacent) characters in a word, or change one letter in a word. The new word must be either target or one of the words from dictionary. The return value will be a String[] containing the words used to do the conversion, in the order they were used. The first element in the return value should be source, the last element should be target. If multiple ways exist to convert from one word to the other, you should return the one with the smallest number of intermediate words. If two sequences exist that have the same number of intermediate words, return the sequence which is smallest lexicographically. Sequence A is lexicographically smaller than sequence B if the first string A[i] that differs from B[i] is lexicographically smaller than B[i]. String A[i] is lexicographically smaller than B[i] if the first character A[i][j] that differs from B[i][j] comes before B[i][j] alphabetically. All words in the input will be the same length. If no conversion is possible, return an empty String[].
| | Definition | | Class: | WordPuzzle | Method: | getConversions | Parameters: | String[], String, String | Returns: | String[] | Method signature: | String[] getConversions(String[] dictionary, String source, String target) | (be sure your method is public) |
| | | | Notes | - | The words in the input might or might not be actual English words or words from any other language. See example 3. | | Constraints | - | dictionary has between 1 and 50 elements, inclusive. | - | source has between 4 and 50 characters, inclusive. | - | target has the same number of characters as source. | - | All elements in dictionary have the same number of characters as source. | - | source, target, and all elements of dictionary will consist only of lowercase letters ('a'-'z'). | - | source will not be the same as target. | - | source and target will not appear in dictionary. | - | There will be no repeated elements in dictionary. | | Examples | 0) | | | {"fowl","foal","loaf","load","loan","loon","toon"} | "foul" | "tool" |
| Returns: { "foul", "foal", "loaf", "loan", "loon", "toon", "tool" } | Note that although in the given list of words, each element can achieve the next, you can skip over "fowl" and "load" since you can go directly from "foul" to "foal", and directly from "loaf" to "loan". |
|
| 1) | | | {"stew","wets"} | "stow" | "west" |
| Returns: { } | Only two letters can be swapped at once. |
|
| 2) | | | {"flew", "slew", "stew", "stow"} | "flow" | "slow" |
| Returns: { "flow", "slow" } | In this conversion, no words from the dictionary were necessary. |
|
| 3) | | | {"tsray","trsay","trasy"} | "stray" | "trays" |
| Returns: { "stray", "tsray", "trsay", "trasy", "trays" } | Note that you cannot simply remove the 's' and insert it at the end. |
|
| 4) | | | {"toon","loot"} | "loon" | "tool" |
| Returns: { "loon", "loot", "tool" } |
The following is also a conversion with three words, but is lexicographically larger than the answer:
loon -> toon -> tool
|
|
| 5) | | | {"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaay",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaax",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaw",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaav",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaau",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaat",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaas",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaar",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaq",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaap",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaao",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaan",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaam",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaal",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaak",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaj",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaai",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaah",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaag",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaf",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaae",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaad",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaac",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"yaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"xaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"waaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"vaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"uaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"taaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"saaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"raaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"qaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"paaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"oaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"naaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"maaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"laaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"kaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"jaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"iaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"haaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"gaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"faaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"eaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"daaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"caaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"baaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"zaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"caaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaz"} | "aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa" | "zaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaz" |
| Returns:
{ "aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa",
"aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"zaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaab",
"zaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaz" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A Randomized Finite Automaton (RFA) works as follows. The RFA maintains a variable, called its state, which is initially set to 0. When an input character ('a', 'b', or 'c') is fed into the RFA, it must determine which new value is to be assigned to its state. This determining process is governed by a list of rules stored in the RFA. For example, if the RFA is in state 2 and has received an 'a' as input, the RFA would consult element 2 of rulesa. Each element of rulesa is of the form "st:prob st:prob ...", where st denotes a value to be assigned to the state variable, and prob denotes the percentage (out of 100) of such an assignment occurring. If the given probabilities do not add up to 100, the remaining probability is the chance of changing state to 999. If a state (not 999) is missing from a rule, then its prob is assumed to be 0. Once state is 999, regardless of future input, it remains on 999. The machine has analogously defined rulesb, and rulesc defining what to do on 'b' or 'c' accordingly.
When processing an input string, each character is processed in order, left to right, one at a time. Assuming all input strings of length maxLength or fewer (including the string of length 0) are equally likely, return the probability the RFA will be on state finalState once the string is completely processed. The probability returned will be a double, between 0 and 1 inclusive. Results must be accurate to 1e-9. | | Definition | | Class: | RandomFA | Method: | getProbability | Parameters: | String[], String[], String[], int, int | Returns: | double | Method signature: | double getProbability(String[] rulesa, String[] rulesb, String[] rulesc, int finalState, int maxLength) | (be sure your method is public) |
| | | | Constraints | - | rulesa will contain between 1 and 50 elements inclusive. | - | rulesa, rulesb, and rulesc all contain the same number of elements. | - | Each element of rulesa, rulesb, and rulesc contain between 0 and 50 characters inclusive. | - | Each element of rulesa, rulesb, and rulesc is a single space delimited list of pairs. Each pair is of the form (quotes for clarity) "st:prob" where, st is an integer, with no extra leading zeros, between 0 and (size of rulesa-1) inclusive, and prob is a positive integer, with no leading zeros. | - | The prob values in a single element of rulesa, rulesb, or rulesc will sum up to a value between 0 and 100 inclusive. | - | No 2 pairs in a single element of rulesa, rulesb, or rulesc will have the same st value. | - | finalState will be between 0 and (size of rulesa-1) inclusive, or 999. | - | maxLength will be between 0 and 10 inclusive. | | Examples | 0) | | | | Returns: 0.5 | The 4 strings that need to be considered are (quotes for clarity) "", "a", "b", and "c". Of those 4, the first 2 will end up on state 0, while the latter 2 will end up on state 999. 2 out of 4 produces a probability of .5 . |
|
| 1) | | | {"1:100","0:100"} | {"1:100","0:100"} | {"1:100","0:100"} | 1 | 3 |
| Returns: 0.75 | Here all even length strings will result in state 0, and odd length strings will result in state 1. Of the 40 strings considered, 1 has length 0, 3 have length 1, 9 have length 2, and 27 have length 3. Thus 30/40=.75 of them have odd length. |
|
| 2) | | | {"1:25 2:25 3:25 4:25","0:100","0:100","0:100","0:100"} | {"","","","",""} | {"","","","",""} | 3 | 5 |
| Returns: 0.0020604395604395605 | All strings that contain a 'b', or 'c' are sent to 999. The strings containing only 'a's begin on state 0, and are evenly distributed between states 1,2,3 and 4 after the first 'a'. After the second 'a' they are all returned to state 0. Examining this pattern, we see that strings that end on state 3 must contain only 'a's and have odd length. Of those, only a quarter of them actually make it to state 3. There are 3 strings consisting of only 'a's that have odd lengths less than or equal 5. This makes 3 out of the total 364 strings. The returned probability is thus 3/364*.25 = .0021 approx. |
|
| 3) | | | {"1:25 2:25 3:25 4:25","0:100","0:100","0:100","0:100"} | {"","","","",""} | {"","","","",""} | 999 | 5 |
| Returns: 0.9835164835164837 | The same problem as before, but now we want the strings that will end on 999. This will be any string of positive length that has a 'b' or 'c'. There are 358 of these, resulting in a probability of 358/364 = .984 approx. |
|
| 4) | | | {"2:9 0:7 3:4 1:2 5:35","2:9 0:7 3:4 1:2 5:35",
"2:9 0:7 3:4 1:2 5:35","2:9 0:7 3:4 1:2 5:35",
"2:9 0:7 3:4 1:2 5:35","2:9 0:7 3:4 1:2 5:35"} | {"0:10 4:7 5:1","0:10 4:7 5:1","0:10 4:7 5:1",
"0:10 4:7 5:1","0:10 4:7 5:1","3:79 2:10"} | {"1:100","2:100","3:100","4:100","5:100","0:100"} | 3 | 10 |
| Returns: 0.002676338903044717 | |
| 5) | | | {"2:9 0:7 3:4 1:2 5:35","2:9 0:7 3:4 1:2 5:35",
"2:9 0:7 3:4 1:2 5:35","2:9 0:7 3:4 1:2 5:35",
"2:9 0:7 3:4 1:2 5:35","2:9 0:7 3:4 1:2 5:35"} | {"0:10 4:7 5:1","0:10 4:7 5:1","0:10 4:7 5:1",
"0:10 4:7 5:1","0:10 4:7 5:1","3:79 2:10"} | {"1:100","2:100","3:100","4:100","5:100","0:100"} | 3 | 0 |
| Returns: 0.0 | Same as before, but only the empty string is considered. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
An OCR program (optical character recognition) needs to perform
other things than just recognizing letters. One of these things
is mapping out the page layout. In this problem, you should solve
the similar, but easier task, of concatenating text spread across
several text columns.
The input text will be given as a String[] and
split up into several text columns. Consider for instance the
text below: (quotes are for clarity only)
{" This is put it that ",
" a text all is ",
"in several together nicely ",
"columns. into a formatted. ",
" single ",
" Your job text ",
"is to Good luck! "}
The text above obviously has three text columns. Formally,
text columns are always separated where there are three or more consecutive
empty character columns (i.e. the only character in those columns are space).
Furthermore, text columns can't be empty (see example 3).
You should also find all paragraphs. The paragraph splits occur
on blank lines within a text column. Only blank lines between
the first and last row used in a text column should be considered.
In the text above, the last line in column two is empty, but
this is not a paragraph split because there is no text below
this line in the same text column.
The return value should contain the same text, in a String[],
but where each element corresponds to one paragraph. Empty paragraphs
should be ignored (that is, there should be no empty strings).
Leading and trailing spaces should be removed in each paragraph,
and consecutive spaces should be replaced by a single space.
Line breaks within a paragraph should also be replaced by a single
space so the last word on a line is not concatenated
with the first word on the next line.
Create a class TextColumns containing the method formatText which takes
a String[] text containing the text and which
returns a String[], containing the formatted text in
the format described above. See the examples for further explanations.
| | Definition | | Class: | TextColumns | Method: | formatText | Parameters: | String[] | Returns: | String[] | Method signature: | String[] formatText(String[] text) | (be sure your method is public) |
| | | | Constraints | - | text will contain between 1 and 50 elements inclusive. | - | Each element in text will contain between 1 and 50 characters, inclusive. | - | All elements in text will contain the same number of characters. | - | text will only contain the characters 'A'-'Z', 'a'-'z', '0'-'9', '.', ',', '!', '?' and space. | - | At least one character in text will not be a space. | | Examples | 0) | | | {" This is put it that ",
" a text all is ",
"in several together nicely ",
"columns. into a formatted. ",
" single ",
" Your job text ",
"is to Good luck! "} |
| Returns:
{ "This is a text in several columns.",
"Your job is to put it all together into a single text that is nicely formatted.",
"Good luck!" } | This is the example in the problem statement.
|
|
| 1) | | | {"This is ",
"just one ",
"column ",
" ",
"a l ",
" b m ",
" c n ",
" d o ",
" e p ",
" f q ",
" g r ",
" h s ",
" i t ",
" j u ",
"k v "} |
| Returns: { "This is just one column", "a l b m c n d o e p f q g r h s i t j u k v" } | |
| 2) | | | {" ",
" ",
" Some text ",
" here and ",
" some ",
" ",
" new ",
" over",
" here ",
" text ",
" there ",
" ",
" ",
" And ",
" something ",
" ",
" ",
" Finito ",
" "} |
| Returns:
{ "Some text here and some text there",
"And something new over here",
"Finito" } | |
| 3) | | | {" Column 1 ",
" Column 2 ",
" Column 3 "}
|
| Returns: { "Column 1 Column 2 Column 3" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Bomb Man is trapped inside a maze shaped like a grid.
You must help him find the exit as fast as possible.
The maze will be given as a String[] where each element
corresponds to a row in the grid and each character in an element
corresponds to a cell in that row. '#' marks a wall,
'.' an empty cell, 'B' the start position of Bomb Man
and 'E' the exit. Below is an example of a maze in this format,
given as a String[]:
{".....B.",
".#####.",
".#...#.",
".#E#.#.",
".###.#.",
"......."}
In each time unit, Bomb Man can move one cell up, down, left or right.
Thus, in the maze above, he can reach the exit in 14 time units by just walking.
To be able to reach the exit quicker, Bomb Man is in possession
of a number of bombs, each of which can blow a hole in a wall and thus open
up new passages. Instead of moving in any of the four cardinal directions,
Bomb Man can (if he has bombs left) blow a hole in a wall located in any of
the four neighboring squares. The bomb will go off in the time unit after he has placed the bomb, creating
an empty cell that Bomb Man can enter in the time unit after that. That is,
if he places a bomb in time unit t, he can enter the cell earliest in time unit t+2.
In the maze above, Bomb Man can then reach the exit in 8 time units by first walking
left, placing a bomb, waiting for the bomb to explode, and then walking down, down,
left, left and down.
Create a class BombMan containing the method shortestPath which
takes a String[] maze, containing the maze in the
format described above, and an int bombs, the number
of bombs in Bomb Man's possession. The method should return an int, the
least number of time units required for Bomb Man to reach the exit.
If it's not possible for Bomb Man to reach the exit, return -1 (see example 1).
| | Definition | | Class: | BombMan | Method: | shortestPath | Parameters: | String[], int | Returns: | int | Method signature: | int shortestPath(String[] maze, int bombs) | (be sure your method is public) |
| | | | Constraints | - | maze will contain between 1 and 50 elements, inclusive. | - | Each element in maze will contain between 1 and 50 characters, inclusive. | - | Each element in maze will contain the same number of characters. | - | maze will only contain the characters '#', '.', 'B' and 'E'. | - | Exactly one character in maze will be a 'B'. | - | Exactly one character in maze will be a 'E'. | - | bombs will be between 0 and 100, inclusive. | | Examples | 0) | | | {".....B.",
".#####.",
".#...#.",
".#E#.#.",
".###.#.",
"......."}
| 1 |
| Returns: 8 | This is the example mentioned in the problem statement. |
|
| 1) | | | | Returns: -1 | Bomb Man can only blow through the first two walls, but not the third. Hence the method returns -1. |
|
| 2) | | | {"..#####",
"B.#####",
"..#####",
"#######",
"####...",
"####.E."}
| 4 |
| Returns: 17 | Here Bomb Man has just enough bombs to reach the exit. One way to do this is to walk
down, right, down (bomb), down (bomb), right (bomb), right (bomb), right, right, down.
This takes 1+1+3+3+3+3+1+1+1 = 17 time units. |
|
| 3) | | | {".#.#.#.#B#...#.#...#.#...#.#...#.#...#.#.#.......",
".#.#.#.#.#.###.###.#.###.#.#.###.###.#.#.#.###.##",
".#.#.#...#.#.#.#.#.#...#.....#.#.#...#...#.#.#...",
".#.#.###.#.#.#.#.#.###.#.#####.#.###.###.#.#.###.",
".............#.#...#...#.....#.#.#...#.#.#.....#.",
"##.#######.###.#.#####.#.#####.#.###.#.#.#.#.####",
".#.#.....#...#...#.#...#...#.#.#...#...#...#.....",
".#######.#.#####.#.#.#.#.###.#.###.#.#####.#.####",
".#.#.#.#...#.#.#.#.#.#.......#...#.#...#.#.#.....",
".#.#.#.###.#.#.#.#.#####.#####.###.###.#.#.######",
".....#...#.#...#...#...#...#...#...#.#.#.........",
"####.###.#.###.###.#.###.#.#.###.###.#.#.########",
".......#.........#.#.#.#.#.#.#.#.........#...#...",
".#.###.#########.#.#.#.#.###.#.#####.#.#.#.###.##",
".#.#.........#.#.#.#.#.....#.#.#.....#.#.........",
"############.#.#.#.#.#.#####.#.#.################",
".#...........#...#.#.#.#...#.#.#...#.#.#.....#...",
".#####.#####.###.#.#.#.#.###.#.#.###.#.#.#####.##",
".......#...#.#.#.....#...#...#.#.#.#.#...........",
"##########.#.#.#####.#.###.###.#.#.#.#.##########",
".....#...#.....#.#...#.......#.#...#.......#.....",
"##.#.###.#.###.#.#.#.#.#####.#.#.###.#######.####",
"...#...#...#.......#.....#.#...#...#.......#.....",
"####.#.#.#########.#.###.#.#####.###.#.#######.##",
".#...#...#.........#.#.....#.........#.#.#.#.....",
".#####.#.#.###.#######.#.###.#.#########.#.#.####",
".......#.#.#...#.......#.....#.#.#.......#.#.#.#.",
"########.#.#.#.#####.#.###.#.###.#.#######.#.#.#.",
".........#.#.#.#.....#...#.#.........#.#.........",
"################.#.#.#.#.#.#.#.#######.#.########",
".................#.#.#.#.#.#.#...........#.......",
"########.#####.#.###.#.#.#####.###.#.#####.###.##",
".........#...#.#...#.#.#...#.....#.#.........#...",
".#####.#####.#.###.#.###.#.#.#.#.#.#####.#.###.#.",
".#.....#.........#.#.#...#.#.#.#.#.#.....#...#.#.",
"####.#####.###.#.#.#.#.#.#.###.###.#.#.#.#.#####.",
".....#.....#.#.#.#.#.#.#.#.#...#...#.#.#.#...#...",
"####.#.#.###.#.#.###.#.###.#.#.#####.#.#.#.######",
".....#.#.#.#...#...#.#...#.#.#...#...#.#.#.......",
"##########.#.#.#.#####.###.#.#.###.#.###.#####.##",
"...#.#...#...#.#.....#.#...#.#...#.#.#.......#...",
".#.#.#.#.#.#.#.#.#.#.###.#.#########.###.#.#.#.#.",
".#.#...#...#.#.#.#.#...#.#...#.......#...#.#.#.#.",
"##.###.#.#.###.#.#.#.#.#####.#.#.#.###.#.########",
".......#.#...#.#.#.#.#.#.....#.#.#...#.#.........",
"####.#######.#.#####.#.###.#.#.###.#.#.#.########",
"E..#.......#.#.....#.#.#.#.#.#.#...#.#.#.........",
"##.#.#.#.###.###.###.###.#.#.###.#.#.#.#.#######.",
".....#.#...#.#.....#.#.....#...#.#.#.#.#.....#..."} | 3 |
| Returns: 76 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Throughout the term in computer science class, Professor Smith's exams consisted entirely of true or false questions. The reason? He was too lazy to grade things by hand, so after a test was over, he would input each student's answers into his computer, and the grades would be automatically calculated. Easy!
It is the end of the term now, and grades are due tomorrow. As luck would have it, Smith's computer has crashed, and he has no time to try and fix it. All he can do now is to try and dig up old test papers and regrade them by hand.
The problem is, the answer keys for all the tests were on the computer. So now, he must try and reconstruct the answer keys so that he can grade things. All is not lost, however. He has all of the papers stored, and he can remember the number of correct answers for certain students. In addition, he is sure each question was answered correctly by at least one student. However, he still hasn't been able to figure out the answer keys for some of them, and he's asked you for help.
For some given test, the papers for which he can remember grades are given as a String[] graded. Each String represents a single student's graded test paper for this specific test, and will start off with a non-negative integer without extra leading zeros indicating the number of questions that student got correct. Then that number is followed by a single space, and then a sequence of uppercase 'T's and 'F's, where the first represents that student's answer to the first question ('T' = true, 'F' = false), and so on. Your method should return the lexicographically earliest answer key that is consistent with the grades he remembers as a String, with the first character being the correct answer to the first question, the second character being the correct answer to the second, and so on. If there is no answer key that works, your method should return the String "inconsistent" (without the double quotes). | | Definition | | Class: | TrueFalse | Method: | answerKey | Parameters: | String[] | Returns: | String | Method signature: | String answerKey(String[] graded) | (be sure your method is public) |
| | | | Notes | - | Each question has been answered correctly by at least one student. (See example 0.) | | Constraints | - | graded will contain between 1 and 50 elements, inclusive. | - | each element of graded will be between 3 and 18 characters in length, inclusive. | - | each element of graded will contain a number N without extra leading zeros, followed by one space and then a sequence of 'T's and 'F's. | - | each element of graded will have the same number of letters following the number. | - | for each element of graded, N will be between 0 and the number of letters in each element, inclusive. | | Examples | 0) | | | {"2 TTF",
"1 FTF",
"2 FTT"} |
| Returns: "TTT" | Since the second question was answered 'T' by all of them, it must be right, since every question was answered correctly by at least one student. Then the second person's test paper tells us that the only possible correct answer key is "TTT", which is consistent with the other two students' papers. |
|
| 1) | | | | Returns: "TTFFTFT" | Only one person, and he had a perfect score. |
|
| 2) | | | {"9 TTTFFFFTTFFTTFT",
"7 FFFFFFFFFFFFFFF"} |
| Returns: "inconsistent" | |
| 3) | | | {"9 TTTFFFFTTFFTTFT",
"7 FFFFFFFFFFFFFFF",
"8 TTTTTTTTTTTTTTT"} |
| Returns: "FFFFFFFTTTTTTTT" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Warning: Embedded in this problem statement are images that may
not be visible if you are using a plugin. For best results, view this
problem in the standard Arena editor.
"To heck with this quipu!" exclaimed Tupac the Incan philosopher. He
was trying to record the contents of his household using the knot
language of Peru, but the rules were too complicated. Who besides a
tax collector could keep track of all the fixed knots, transient knots,
and rhetorical knots?
After some thought, Tupac contrived a simpler scheme that would encode
each inventory item on a length of string using a sequence of square
knots and bow knots. For one particular collection of four items, he
tied the following four strings, where 's' stands for a square knot and
'b' for a bow knot: {"bsb", "bbs", "sbbs", "sbs"}.
This took 13 knots. Tupac now gathered the strings into a quipu by tying
them at one end with a top knot, making a grand total of 14 knots. The
result of his efforts is depicted in the following image, where the bow
knots are colored blue; the square knots, red; and the top knot, black.
"The top knot is important," explained Tupac to his wife, Cuxi, "because
it shows me where to start reading each item."
"Let me see that," said Cuxi, reaching for the quipu. "I can record the
same four items using fewer knots."
Once Cuxi had cut off some parts of the quipu and reknotted a few
others, it emerged like so.
Her husband goggled at this feat. "Three knots fewer!" he cried. "And
it encodes exactly the same inventory!"
Tupac saw that if he began from the top knot and followed a path through
the quipu from left to right until the end of a string, he could spell out
any inventory item, but nothing that wasn't in the inventory. This was an
improvement over the original quipu, in which he sometimes found himself
reading the wrong item and therefore having to backtrack to the top knot.
"I can do better yet," said Cuxi, "if I join some strings at the other
end of the quipu. Watch this."
After some more cutting and reknotting, she produced the following
configuration.
Cuxi stated emphatically that you weren't supposed to pursue a looping
path through the quipu. "Follow the grain of the string from beginning
to end," she said, "and make sure you don't double back. The quipu only
works in one direction."
She also pointed out that the paths were deterministic: at every
knot where the quipu bifurcated, there was a choice of two different
knots. This guaranteed that any inventory item could be found without
backtracking or pursuing several paths at once.
Tupac was pleased to confirm that Cuxi's abbreviated quipu was
functionally equivalent to the original. He could begin reading from
the top knot and proceed strictly from left to right through the knots,
following one of four distinct paths that spelled out exactly the items
of the inventory.
"I can't make it any smaller," said Cuxi, "but a quipu with eight knots
is an improvement over the original fourteen. So I'm pretty good. I'm
only worried about cases where one item in the inventory is a prefix
of another."
"There will never be such a case," said Tupac. "As it happens, my
inventory encoding has the property that no item can be a prefix of
another."
You are given a String[] describing a collection of inventory
items encoded under Tupac's system. Calculate the smallest number of
knots, including the top knot, that must be tied to make a deterministic
binary quipu for this inventory.
| | Definition | | Class: | BinaryQuipu | Method: | fewestKnots | Parameters: | String[] | Returns: | int | Method signature: | int fewestKnots(String[] inventory) | (be sure your method is public) |
| | | | Notes | - | Since Tupac's encoding precludes any inventory where one item is a prefix of another, there will be no duplicates. | | Constraints | - | inventory contains between 1 and 50 elements, inclusive | - | each element of inventory is between 1 and 50 characters long, inclusive | - | no element of inventory is a prefix of any other element | - | each character in every element of inventory is either 's' or 'b' | | Examples | 0) | | | {"bsb", "bbs", "sbbs", "sbs"} |
| Returns: 8 | This is the example shown above. |
|
| 1) | | | | 2) | | | | 3) | | | | 4) | | | {"bssbs", "ssbs", "sbb", "bbs", "sbs", "ssbb"} |
| Returns: 10 | |
| 5) | | | {"bbbsssbbsbbssbbbbs",
"bssssbbbbsbbsbbbbbbsbbsbsssbbbsbbbbbsbbssbsb",
"sbbbbbsbbbsbsssbssssbssbbsssssssbbssss",
"sbbbbbsbsbssbssbsssbsbbsbssbsbbbsbsbs",
"bsbbbbbssbsbbbbsbbs",
"bbsbbsbsssbsbbsbbssbbbsbsssbsbbsbsbssbsbsssbsbsbs"} |
| Returns: 181 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Name, age, and id. That is the information that we are supposed to have for
all our workers, but what we have is a mess. Our data is a collection of records,
but some workers have multiple records, and some of the records are missing
some of the information. We would like to untangle the mess. At least, we
would like to figure out how many different workers we have!
Each worker has his own unique id that is a string of digits, the first of
which is always zero ('0'). Each worker has a name consisting of uppercase
letters 'A'-'Z', and has an age that is an integer with no leading zeros. Neither
name nor age can be relied upon to be unique. Each data
record contains information for a single worker, and is formatted to contain
no leading or trailing spaces. It may contain 1, 2, or all 3 of the
fields (name, age, id) in any order. The fields within a record will be
separated by one or more spaces.
Create a class DataFilter that contains a method untangle that is
given a String[] data that contains the
records and returns the number of different workers we have.
Each element of
data consists of one or more records, separated from each other by a single semicolon (';'). If there is more
than one way to untangle the data, return the smallest possible
number of workers. If there is no consistent interpretation of the data,
return -1.
| | Definition | | Class: | DataFilter | Method: | untangle | Parameters: | String[] | Returns: | int | Method signature: | int untangle(String[] data) | (be sure your method is public) |
| | | | Notes | - | Ids should be treated as strings, not numbers. | | Constraints | - | data will contain between 1 and 50 elements inclusive. | - | Each element of data will contain between 1 and 50 characters inclusive. | - | Each element of data will consist of 1 or more records, separated from each other by a single ';' | - | Each record in each element of data will contain no leading or trailing spaces. | - | Each record will contain exactly 1, 2, or 3 fields separated by (possibly multiple) spaces. | - | No record will contain more than one age, name, or id. | - | Each age will be between 1 and 150 inclusive, and will not have a leading '0' | - | Each id will contain between 1 and 10 characters inclusive, all digits and having a leading '0' | - | Each name will contain between 1 and 10 characters inclusive, all uppercase letters 'A'-'Z' | | Examples | 0) | | | {"BOB 22 013","17 BOB;22","0234","16"} |
| Returns: 3 |
data consists of 5 records (one of the elements of data contains 2 records, separated by a ';'). One of the records has all 3 fields, one of the records has 2 fields, and the other records each have just a single field.
There are at least 3 different workers since there are 3 different ages.
[BOB 22 013],[BOB 17 0234],[TOM 16 099] is one possibility that is consistent with these 5 data records. |
|
| 1) | | | {"2 01;02 B;B;B 02;2 02;C 02"} |
| Returns: -1 | One record has the employee with id 02 being named B, while another record claims that 02 is named C. So these records are inconsistent. |
|
| 2) | | | {"A 21","B 21","B 23","A 23","01 A","02 21","B 03","04 B"} |
| Returns: 4 | One way to untangle these 8 records into just 4 employees is [A 23 01], [B 21 03], [B 23 04], [A 21 02]. |
|
| 3) | | | | 4) | | | | Returns: 2 | Id's 0 and 00 are different, so these two cannot be combined. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Given a starting point and a finishing point on a square grid, along with some
grid points that are forbidden, determine the length of the shortest path
from start to finish. In one step you may move one unit horizontally,
vertically, or diagonally (but not onto a forbidden point).
Create a class ShortPath with a method steps that is given int[] x and int[] y as input. Corresponding elements of x and y give the coordinates of a point. The first elements of
x and y give the start, the second elements give the finish, and additional corresponding
pairs of elements from x and y specify forbidden points. Your method should return the minimum
number of steps required to go from start to finish, or -1 if it is not possible to go from start
to finish.
| | Definition | | Class: | ShortPath | Method: | steps | Parameters: | int[], int[] | Returns: | int | Method signature: | int steps(int[] x, int[] y) | (be sure your method is public) |
| | | | Constraints | - | x will have between 2 and 50 elements inclusive. | - | y will have the same number of elements as x. | - | Each element of x will be between -1,000,000,000 and 1,000,000,000 inclusive. | - | Each element of y will be between -1,000,000,000 and 1,000,000,000 inclusive. | - | The start and finish points will be distinct from each other and from all other points given by x and y. | | Examples | 0) | | | | Returns: 3 |
3 --|--|--|--|--|--|--
2 --|--|--F--|--|--|--
1 --|--X--|--|--|--|--
0 --S--|--|--|--|--|--
0 1 2 3 4 5
In the diagram, S is the start point, F is the finish, and X is forbidden.
There are two different paths of length 3, one of which is S to (0,1) to (1,2) to F.
The direct path of length 2 is blocked by the forbidden point.
|
|
| 1) | | | | Returns: 1 |
Take a single diagonal step from (0,0) to (1,1). The second appearance of (2,2) on the forbidden list is legal but never matters. |
|
| 2) | | | {1,100000,0,0,0,2,2,2,1,1} | {1,200000,0,1,2,0,1,2,0,2} |
| Returns: -1 | The start point is trapped in a square of forbidden points. |
|
| 3) | | | {1,100000,0,0,0,2,2,2,1} | {1,200000,0,1,2,0,1,2,0} |
| Returns: 199999 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | The pipe game is played on a grid with three kinds of pipes. The straight pipe '-' allows water to flow straight. The elbow pipe 'L' diverts water either to the left or to the right. The cross pipe '+' has the same effect as a straight pipe. Unlike the other two pipes, the cross pipe allows water to flow through it a second time in a direction perpendicular to the first pass. The water source is represented by one of: 'N', 'S', 'W' or 'E' indicating whether the water will begin flowing north, south, west or east respectively. You are allowed to rotate each pipe in the grid by 90 degree increments with the objective of maximizing the number of pipes connected to the water source.
Given a String[] pipes that represents the game grid, determine the length of the longest possible flow of water. Each pipe that the water flows through adds one to the total length. A cross pipe through which water passes twice contributes two to the overall length. The starting water source is not a pipe, does not count towards the length, and may not be rotated.
{"LL-L-",
"L+L+L",
"--NL-",
"L+--L",
"LL+L-"}
This is a graphical representation of the puzzle above.
| | Definition | | Class: | PipePuzzle | Method: | longest | Parameters: | String[] | Returns: | int | Method signature: | int longest(String[] pipes) | (be sure your method is public) |
| | | | Notes | - | In each grid, south is the direction of increasing index within pipes, and north that of decreasing index. East is the direction of increasing index within an element of pipes, and west that of decreasing index. | | Constraints | - | pipes will have between 1 and 20 elements, inclusive. | - | Each element of pipes will have length between 1 and 20, inclusive. | - | Every element of pipes will have the same length. | - | pipes will only contain the characters ('-', 'L', '+', 'N', 'S', 'E', 'W'). | - | pipes will have exactly one water source. | - | pipes will contain between 0 and 20 elbow 'L' pipes, inclusive. | | Examples | 0) | | | {"LL-L-",
"L+L+L",
"--NL-",
"L+--L",
"LL+L-"} |
| Returns: 19 | |
| 1) | | | {"ELLL",
"LLLL",
"LLLL",
"LLLL"}
|
| Returns: 13 | |
| 2) | | | {"ELLLLL+",
"++++++L",
"L+++++L",
"L+++++L",
"L+++++L",
"L+++++L",
"+LLLLLL"} |
| Returns: 71 | |
| 3) | | | | 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Graphical user interfaces rely on text routines to properly display words on various GUI components. Being able to determine the width in pixels of a line of text is useful for centering the text in a window. You will be given a sentence of letters and spaces. You will also be given the letter widths for both uppercase and lowercase letters of a particular font. You must return the width of the sentence.
Both uppercase and lowercase contain 26 elements. The first element of uppercase is the width of 'A' and the last is the width of 'Z'. The first element of lowercase is the width of 'a' and the last is the width of 'z'. The width of the space character is always 3 pixels. When a line of text is rendered, there is a gap of 1 pixel between each pair of adjacent characters.
| | Definition | | Class: | FontSize | Method: | getPixelWidth | Parameters: | String, int[], int[] | Returns: | int | Method signature: | int getPixelWidth(String sentence, int[] uppercase, int[] lowercase) | (be sure your method is public) |
| | | | Constraints | - | sentence will contain between 1 and 50 characters, inclusive. | - | sentence will only contain uppercase letters ('A'-'Z'), lowercase letters ('a'-'z'), and spaces. | - | uppercase will contain exactly 26 elements. | - | lowercase will contain exactly 26 elements. | - | Each value in uppercase and lowercase will be between 1 and 36, inclusive. | | Examples | 0) | | | "Dead Beef" | {6,6,6,7,7,7,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9} | {5,5,5,4,4,4,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9} |
| Returns: 49 |
D e a d (space) B e e f
7+1+4+1+5+1+4+1 + 3 + 1+6+1+4+1+4+1+4 = 49
|
|
| 1) | | | "Hello World" | {7,8,8,8,7,8,8,8,7,8,8,8,8,8,7,8,8,8,8,8,7,8,8,8,8,8} | {5,6,6,6,5,6,6,6,5,6,6,6,6,6,5,6,6,6,6,6,5,6,6,6,6,6} |
| Returns: 74 | |
| 2) | | | "Hello World" | {7,7,7,7,7,7,7,8,7,7,7,7,7,7,7,7,7,7,7,7,7,7,9,7,7,7} | {5,5,5,6,6,5,5,5,5,5,5,1,5,5,6,5,5,6,5,5,5,5,5,5,5,5} |
| Returns: 63 | |
| 3) | | | "ThE qUiCk BrOwN fOx JuMpEd OvEr ThE lAzY dOg" | {36,35,34,33,32,31,30,29,28,27,26,25,24,23,22,21,20,19,18,17,16,15,14,13,12,11} | {1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26} |
| Returns: 778 | |
| 4) | | | "two spaces" | {9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9,9} | {3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3} |
| Returns: 43 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Your company controls the flow of oil through a network of pipes. The network is composed of N waypoints, each of which has a number between 0 and N-1 inclusive. Some of the waypoints are connected by pipes. You will be given String[]s caps and costs describing these pipes. Each element of caps and costs will be formatted as follows (quotes for clarity):
"x1,y1 x2,y2 x3,y3 ..."
Each x,y pair is called an indicator. Element i of caps will always contain the same number of indicators as element i of costs. Furthermore, the jth indicator of element i of caps will correspond to the jth indicator of element i of costs. If element i of caps contains the indicator x,y it means there is a pipe that allows y units of flow from waypoint i to waypoint x. The corresponding pair x,z in element i of costs gives the cost z attributed to using this pipe.
In this network, one waypoint has been designated as the source, and another as the sink. Your business would like to send oil from the source to the sink but it also wants to save money. For this reason, only 1 path will be activated. Your job is to find the path from the source to the sink that maximizes the ratio capacity/cost. For the purposes of this problem, a path will consist of an ordered sequence of distinct waypoints, from the source to the sink, where each consecutive pair of waypoints are connected by a pipe. The pipes on the path must allow oil to flow in the direction of the path (source to sink). No flow of oil may enter the source or leave the sink. The cost of a path is the sum of all the costs of the pipes on the path. The capacity is the minimum capacity over all the pipes on the path. Note that the pipes are directed, so you can push oil from waypoint i to waypoint j only if there is a pipe allowing such a flow in that direction. You will return the maximum value of capacity/cost accurate to 1e-9 absolute or relative. If there is no path of pipes from source to finish to push oil through, return 0. | | Definition | | Class: | PipePath | Method: | capCost | Parameters: | String[], String[], int, int | Returns: | double | Method signature: | double capCost(String[] caps, String[] costs, int source, int sink) | (be sure your method is public) |
| | | | Notes | - | A pipe from waypoint i to waypoint j does not imply there is a pipe from j to i. | - | There can be multiple pipes carrying oil from waypoint i to waypoint j. | | Constraints | - | caps will contain between 2 and 50 elements inclusive. | - | caps and costs will contain the same number of elements. | - | Each element of caps and costs will contain between 0 and 50 characters inclusive. | - | Each element of caps and costs will be a single space delimited list of indicators. Each indicator will take the form (quotes for clarity) "x,y". x will have no extra leading zeros, and will be between 0 and N-1 inclusive, where N is the number of elements in caps. y will have no extra leading zeros, and will be between 1 and 20000000 inclusive. | - | Element i of caps will not contain the indicator "i,y". | - | Each element of caps and costs will have no leading or trailing whitespace. | - | Element i of caps will have the same number of indicators as element i of costs. | - | The jth indicator of element i of caps will have the same first value as the jth indicator of element i of costs. | - | source and sink will be between 0 and N-1 inclusive, where N is the number of elements in caps. | - | source and sink will not be equal. | | Examples | 0) | | | {"1,10 2,9","","1,100"} | {"1,100 2,50","","1,50"} | 0 | 1 |
| Returns: 0.1 | The 2 paths from waypoint 0 to waypoint 1 have a cost of 100. Since one has capacity 9, and the other has capacity 10, we choose the capacity 10 path. |
|
| 1) | | | {"1,3 3,5","3,2 2,6","3,2","1,1 2,4 4,6",
"0,3 2,7"} | {"1,3 3,5","3,2 2,6","3,2","1,1 2,4 4,6",
"0,3 2,7"} | 0 | 4 |
| Returns: 0.45454545454545453 | |
| 2) | | | {"1,15 1,20","2,10",""} | {"1,10 1,10","2,15",""} | 0 | 2 |
| Returns: 0.4 | There are multiple pipes from waypoint 0 to waypoint 1. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
With the advent of text messaging on cell phones, a new method for typing has evolved, called T9. The way T9 works is it has a dictionary of words, where each letter is mapped to a number 2-9. The number-letter mappings are fixed, and are printed on the number keys of the phone. For example, 2 corresponds to 'a', 'b', and 'c'. A word is defined as a sequence of lowercase letters. Since most words use different digits for their characters, it is possible to determine which word a user is typing by matching the digit sequence to the letter sequence. Below are all the mappings for the characters used in the problem:
2 - abc
3 - def
4 - ghi
5 - jkl
6 - mno
7 - pqrs
8 - tuv
9 - wxyz
# - <space>
0 - <next word>
So for example, to type "hello world", you would press "43556#96753". A conflict arises when two words have the same digit sequence. So for example, "the" and "tie" both have the same digit sequence - "843". The way T9 handles these conflicts is to display the word that comes first alphabetically. Then, if the word isn't desired, the user must press 0 to get the next word alphabetically, and repeat until the desired word is shown. If the user wishes to enter another word, he/she presses # to insert a space and starts entering the next word. So to input "the tie", you would type "843#8430". It is illegal to press a non-zero digit immediately after pressing '0', the only legal keys you can press after pressing '0' are '0' and '#'.
You will be given a String[] messages, which consists of messages you are to type into your cell phone. messages will only contain spaces and words made entirely of lowercase letters. Assume that the words contained in all of the messages are exactly the words contained in the T9 dictionary. Return a String[] where each element i contains the numeric keypresses you would have to type for element i of messages. Note that messages do not necessarily have to contain words, and can contain sequences of multiple spaces which must be preserved.
| | Definition | | Class: | T9Input | Method: | getKeypresses | Parameters: | String[] | Returns: | String[] | Method signature: | String[] getKeypresses(String[] messages) | (be sure your method is public) |
| | | | Constraints | - | messages will have between 1 and 50 elements, inclusive. | - | Each element of messages will have between 0 and 50 characters, inclusive. | - | Each element of messages will contain only lowercase letters ('a'-'z') and spaces. | - | There will not be more than 7 words which have the same digit sequence. | | Examples | 0) | | | | Returns: { "843#8430", "843#8430" } | The example from the problem statement. |
|
| 1) | | | | Returns: { "#439#563###", "###" } | Don't forget to preserve multiple spaces, and to handle messages where there are no words. |
|
| 2) | | | {"cba cba cba cba cba cba cba cba", "abc acb bac bca cab cba"} |
| Returns:
{ "22200000#22200000#22200000#22200000#22200000#22200000#22200000#22200000",
"222#2220#22200#222000#2220000#22200000" } | Remember that the dictionary contains all words from all the messages, not just the message you are working on. |
|
| 3) | | | { "the longest case for t nine is probably when",
"you enter seven two letter sequences from the",
"same key and then repeat the alphabetically",
"last case over and over again for the entire",
"list of messages",
"",
"these paragraphs demonstrate how efficient t",
"nine is since there is only one time where you",
"must use the zero key"} |
| Returns:
{ "843#5664378#2273#367#8#6463#47#77622259#9436",
"968#36837#73836#896#538837#737836237#3766#843",
"7263#539#263#8436#737328#843#25742238422559",
"5278#2273#6837#263#6837#24246#367#843#368473",
"5478#63#63772437",
"",
"843730#7272472747#33666787283#469#333424368#8",
"6463#47#74623#84373#47#6659#663#8463#94373#968",
"6878#873#843#9376#539" } | |
| 4) | | | {"cca ccc c cccb ccca cccc"} |
| Returns: { "222#2220#2#22220#2222#222200" } | Note that to make "cccc", you cannot use "22202". Pressing '2' after pressing '0' is illegal. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Given a map of a hill, there are many ways for an agent to get from one point to another point. Many different algorithms have been developed to find the best, or reasonably good ways to get from a starting location to an ending location. One such method is the breadth-first-search (BFS) algorithm, where the agent always expands the location with the least accumulated cost. Another method is called A*, which involves predicting the total cost to the goal based on the cost so far, and a heuristic which calculates the expected cost to come, and then expanding the nodes in a BFS-fashion based on this expected, rather than already accumulated, cost.
When a search method expands a node, it assigns a new cost to each neighbor, unless that neighbor already has an equal or lesser cost assigned. In BFS, this cost is the cost to get to the current node plus the cost of moving from the current node to the given neighboring node. In A*, this is the total cost as in BFS plus the predicted cost based on the heuristic function. The search ends when the goal node is expanded, and not when its cost is calculated. This is because different neighboring nodes can assign different costs to the goal, so terminating before the goal node itself is expanded can give a sub-optimal solution. Once the goal is expanded, however, you are guaranteed that no neighbor will assign it a lower cost than the one it currently has, and thus it is optimal.
BFS (for reference): For BFS, the agent starts at the starting location (hereafter referred to as S) with a cost of 0. The agent expands this location (also referred to as a node), calculating the cost for all of its neighbors. It then expands all nodes with a calculated cost of 1, then all nodes with a calculated cost of 2, and so forth, until it has expanded the goal node. Notice that a given node may be assigned different costs by different neighbors, but this is okay because we assume that the cost is always increasing, so it should be assigned the lowest cost possible and expanded in turn.
A*: For A*, the agent starts at S, with a so-far cost of 0. The heuristic function our agent uses will be the "Manhattan Distance" from the current node to the goal node (hereafter referred to as G), that is, the positive difference in X values plus the positive difference in Y values. The agent expands S, assigning all of its neighbors a cost as with BFS, but with an additional predicted cost (the Manhattan Distance to the goal). It then expands the nodes in a BFS-fashion using these total predicted costs. A* should NOT expand a node that it has already expanded previously.
For this problem, the agent can move across the hill in any of the four cardinal directions (north, east, west and south.) The cost of moving down the hill is 1, of moving across a plateau (between 2 squares of equal altitude) is 2, and of moving up the hill is 3. The hill is represented by a String[] hill, which represents the altitudes as 1 through 9 (with 9 being the highest altitude). The x and y coordinates of S and G are represented respectively by int sx sy gx and gy. The y coordinate is the 0-indexed element in hill, and the x coordinate is the 0-indexed location in the element of hill. Use the A* method to search for G starting from S, and return the number of nodes expanded. Expand all nodes that share the same predicted cost as G. For example, if you expand G with a predicted cost of 10, make sure that all other nodes with a predicted cost of 10 are also expanded, to avoid ambiguities.
| | Definition | | Class: | AlgoHill | Method: | astar | Parameters: | String[], int, int, int, int | Returns: | int | Method signature: | int astar(String[] hill, int sx, int sy, int gx, int gy) | (be sure your method is public) |
| | | | Constraints | - | hill will contain between 5 and 50 elements, inclusive. | - | each element of hill will have between 5 and 50 characters, inclusive. | - | each element of hill will have the same number of characters as every other element of hill. | - | each element of hill will contain only the characters '1' through '9'. | - | sy and gy will each be between 0 and one less than the number of elements in hill, inclusive. | - | sx and gx will each be between 0 and one less than the length of the elements in hill, inclusive. | | Examples | 0) | | | {"34567",
"23456",
"12345",
"23456",
"34567"} | 0 | 2 | 2 | 2 |
| Returns: 5 | A* will assign the following costs:
10 * * * *
6 8 10 * *
2 4 6 10 *
6 8 10 * *
10 * * * *
All nodes with a predicted cost less than or equal to 6 will be expanded (5 nodes).
|
|
| 1) | | | {"55555",
"54555",
"55355",
"55525",
"55551"}
| 0 | 0 | 4 | 4 |
| Returns: 25 | In this case, A* expands all nodes.
Also, note that several times in this example, a node of cost C expands to form another node with cost C, and this new node must also be expanded before going on to expand nodes of cost greater than C.
|
|
| 2) | | | {"55555",
"54445",
"54345",
"54445",
"55555"} | 0 | 1 | 4 | 3 |
| Returns: 21 | |
| 3) | | | {"99999",
"89992",
"76543",
"99992",
"99991"}
| 0 | 0 | 4 | 0 |
| Returns: 13 | Note that the node at (4,1) gets expanded by A*. Prior to expanding nodes with a predicted cost of 8, this node has no cost. After expanding the node at (4,2), this is assigned a predicted cost of 8 (the same as the goal) and therefore should be expanded. |
|
| 4) | | | {"68233335836531",
"57244363483169",
"92744511826738",
"76864574378195",
"39927887799237",
"89447973623642",
"19758793475135",
"33857155647757",
"82987352116383",
"18813823791825",
"91772225881964",
"46692256258431",
"41961397519198",
"48265328441524",
"31422961925492",
"17844758382392",
"47549368526297",
"27955796939522",
"59699922792416",
"56366572279148",
"26884644728715",
"98234493558879",
"38984599399327",
"66812381124583",
"73833939626911",
"13681831986111",
"69237772565286",
"34347792857462"} | 8 | 2 | 8 | 2 |
| Returns: 1 | The start node is the goal node, and is the only node that you expand. |
|
| 5) | | | {"44435",
"44425",
"44445",
"32445",
"55555"} | 0 | 0 | 2 | 2 |
| Returns: 13 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You work for a company that stores its goods in a large underground vault. There have been flood warnings, and it may be necessary to seal the vault to protect it from flood waters. The vault is a series of rooms that are connected by doors, each of which can only be sealed from one of the rooms it connects. To ensure that the rooms are as safe as possible, you want to seal every individual door in the vault. You can't do this, however, just by sealing any door you see, because you could close off a section of the vault and be unable to seal doors in that section, or you could seal yourself in the vault!
You begin (and must end) in room 0. When you seal a room, you close all doors in that room that must be sealed from that side. If there are other doors that go to that room that must be sealed from the other side, they remain open (but the room is still considered sealed). Given a String[], rooms, describing the vault, return a int[] that gives the order in which to seal the rooms. If there exist multiple solutions, return the one that is lexicographically first. When ordered lexicographically, int[] A comes before int[] B if and only if for the smallest i such that A[i]!=B[i], A[i]<B[i]. Keep in mind that every room must be sealed, even though in some cases sealing a room does not close any doors.
Each element of rooms will be a comma delimited list of numbers. The i-th element of rooms will list all rooms adjacent to room i that must be sealed from room i. For any two rooms i and j, there will be at most one door connecting them. If there is a door connecting them, i will be listed as adjacent to j, or vice versa, but not both. | | Definition | | Class: | UndergroundVault | Method: | sealOrder | Parameters: | String[] | Returns: | int[] | Method signature: | int[] sealOrder(String[] rooms) | (be sure your method is public) |
| | | | Notes | - | There can be cycles. For example: room i has a door to room j that must be sealed from room i, room j has a door to room k that must be sealed from room j, and room k has a door to room i that must be sealed from room k. | | Constraints | - | rooms will contain between 1 and 50 elements, inclusive. | - | Each element of rooms will contain only the digits '0'-'9' and commas. | - | Each element of rooms will only contain values between 0 and the number of elements in rooms - 1, inclusive, and no value will have leading zeros. | - | No element of rooms will contain leading or trailing commas, or more than one commma between values. | - | Element i of rooms will not contain the value i. | - | No element of rooms will contain the same value more than once. | - | If element i of rooms contains j, element j of rooms will not contain i. | - | There will be a way to seal all the rooms and end in room 0. | | Examples | 0) | | | | Returns: { 2, 1, 0 } | We can't seal room 0 first, because then we won't be able to reach any other rooms.
We can't seal room 1 first either because then we can't reach room 2 to seal it.
The only way is to seal room 2, then 1, then finally 0. |
|
| 1) | | | | Returns: { 3, 2, 1, 0 } | Rooms 1, 2, and 3 form a cycle. Each one must seal a door to the next one
in the cycle. The only way to seal all the rooms is to go to room 3, seal it,
and then work backwards through the cycle. |
|
| 2) | | | {"3,5","2","8","","","6,7","","1,8","4"} |
| Returns: { 2, 1, 3, 4, 6, 8, 7, 5, 0 } | |
| 3) | | | {"1,2","3","3,5","4","",""}
|
| Returns: { 1, 4, 3, 5, 2, 0 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Given a summary of events occurring during a TopCoder match in a single room, return the room summary for that room.
The room summary is a list of coders' handles with their point totals, sorted in decreasing order by points. Ties are broken by listing lexicographically lower handles first (according to ASCII values).
The room summary is a String[], where each element is the result of a coder's performance in the format "<handle> <points>" (quotes for clarity) where <handle> is the coder's handle and <points> is the coder's point total (<points> must contain exactly 2 decimal places and have no extra leading zeros), eg. "SnapDragon 1201.30"
The Problems
The name of the three problems are given in the String[] problems.
The Coders
The coder's handles are given in the String[] handles.
I. Coding Phase
Each event during the Coding Phase is a submission, which is given as an element of the String[] submissions in the following format:
"<handle> <problem> <points>" (quotes for clarity), where
- <handle> is a coder's handle that corresponds to an element of handles,
- <problem> is a problem's name that corresponds to an element of problems,
- <points> is the points the coder receives for the submission of the respective problem, with exactly 2 decimal places and no extra leading zeros, eg. "612.34" (quotes for clarity).
This means that coder <handle> submits problem <problem> for <points> points.
If a coder submits a problem more than once (i.e. resubmits), only the last submission made (i.e. the one with the higher index in submissions) is to be considered.
Points are between 60.00 and 1000.00, inclusive.
II. Challenge Phase
Each event during the Challenge Phase is a challenge, which is given as an element of challenges in the following format:
"<handle1> <handle2> <problem> <result>" (quotes for clarity), where
- <handle1> is a coder's handle that corresponds to an element of handles,
- <handle2> is a coder's handle that corresponds to a different element of handles,
- <problem> is a problem's name that corresponds to an element of problems,
- <result> is the result of the challenge, either "successful" or "unsuccessful" (quotes for clarity).
This means that coder <handle1> challenges problem <problem> of coder <handle2> with the result <result>.
For a successful challenge, <handle1> receives 50 points and <handle2> loses all points for his submission of <problem>.
For an unsuccessful challenge, <handle1> loses 50 points (possibly getting a negative score). Challenges occur in the order listed in challenges.
Coders with a non-positive score are still allowed to challenge (unlike in real TopCoder matches).
III. System Testing Phase
Each element in failed is a result of the System Testing Phase in the following format:
"<handle> <problem>" (quotes for clarity), where
- <handle> is a coder's handle that corresponds to an element of handles,
- <problem> is a problem's name that corresponds to an element of problems.
This means that problem <problem> of coder <handle> failed the system tests and <handle> loses all points for his submission of <problem>.
All submissions that pass the system tests are not listed within failed.
| | Definition | | Class: | RoomSummary | Method: | generate | Parameters: | String[], String[], String[], String[], String[] | Returns: | String[] | Method signature: | String[] generate(String[] problems, String[] handles, String[] submissions, String[] challenges, String[] failed) | (be sure your method is public) |
| | | | Notes | - | Problem names and coder handles are case sensitive. | | Constraints | - | problems contains exactly 3 elements. | - | Each element of problems contains between 1 and 15 alphanumeric characters ('A'-'Z', 'a'-'z', '0'-'9'). | - | There are no duplicates within problems. | - | handles contains between 1 and 20 elements, inclusive. | - | Each element of handles contains between 1 and 10 alphanumeric characters ('A'-'Z', 'a'-'z', '0'-'9'). | - | There are no duplicates within handles. | - | submissions contains between 0 and 50 elements, inclusive. | - | challenges contains between 0 and 50 elements, inclusive. | - | failed contains between 0 and 50 elements, inclusive. | - | All elements of submissions, challenges and failed are formatted as described in the problem statement. | - | Points for submissions are between 60.00 and 1000.00, inclusive. | - | A resubmission results in a lower score than a previous submission or in the minimal possible score of 60.00. | - | A coder will not challenge his own problems or another coder's problem that was previously unsuccessfully challenged by him or successfully challenged by somebody else. Only problems that have been submitted will be challenged. | - | failed only contains problems that have been submitted and have not been successfully challenged. | | Examples | 0) | | | { "EasyP", "MediumP", "HardP" } | { "Andrea", "Billy", "Chris", "eddy", "David", "Feliza" } | { "Andrea EasyP 220.31",
"Billy EasyP 213.24",
"Chris EasyP 194.24",
"Chris EasyP 75.00",
"Andrea MediumP 472.23",
"Billy MediumP 428.34",
"Andrea HardP 823.60" } | { "Chris Andrea EasyP unsuccessful",
"Chris Billy EasyP unsuccessful",
"Billy Andrea HardP successful" } | { "Billy MediumP" } |
| Returns:
{ "Andrea 692.54",
"Billy 263.24",
"David 0.00",
"Feliza 0.00",
"eddy 0.00",
"Chris -25.00" } | Andrea has 1516.14 (220.31 + 472.23 + 823.60) points after the coding phase. She loses 823.60 points during the challenge phase because her submission of HardP was successfully challenged. Her final score is 692.54.
Billy has 641.58 (213.24 + 428.34) points after the coding phase. He gains 50.00 points during the challenge phase and loses 428.34 points because his MediumP fails the system tests. His final score is 263.24.
Chris receives 75.00 points for his submission of EasyP (which was a resubmission), but loses 100.00 points due to two unsuccessful challenges. His final score is -25.00.
David, eddy and Feliza are not involved in any submission or challenge, thus all have a final score of 0.00. They are listed in lexicographical order (lower ASCII values first). |
|
| 1) | | | { "EasyP", "MediumP", "HardP" } | { "Andrea", "Billy", "Chris" } | { "Billy EasyP 240.31",
"Billy MediumP 425.23",
"Chris HardP 831.42",
"Andrea EasyP 89.12" } | { "Andrea Billy EasyP unsuccessful",
"Andrea Chris HardP unsuccessful" } | {} |
| Returns: { "Chris 831.42", "Billy 665.54", "Andrea -10.88" } | |
| 2) | | | { "250pointer", "500pointer", "1000pointer" } | { "Andrea", "Billy", "Chrissy", "Chris" } | { "Billy 250pointer 244.32",
"Andrea 250pointer 241.42",
"Andrea 500pointer 432.39",
"Billy 500pointer 372.40",
"Billy 250pointer 100.42" } | { "Billy Andrea 250pointer unsuccessful",
"Andrea Billy 500pointer successful",
"Billy Andrea 500pointer unsuccessful" } | {} |
| Returns: { "Andrea 723.81", "Billy 0.42", "Chris 0.00", "Chrissy 0.00" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You have just received a int[] sampleData from the laboratory. You know that the variance of this data should be varNum/varDen, where varNum and varDen are ints. Note that varNum/varDen need not be an integer. Your method will return which nonempty subset of the elements from sampleData produces a variance closest to the variance you expected. This subset may be the entire sampleData. The variance of a subset of the sampleData can be calculated via the following formula.
Let s(i) denote the ith element of the subset (assuming some method of indexing into the subset), and let n denote the size of the subset. Then
mean = ( s(0) + s(1) + s(2) + ... + s(n-1) )/n
varsum = (mean-s(0))^2 + (mean-s(1))^2 + ... + (mean-s(n-1))^2
variance = varsum/n
The subset will be returned as a int[], whose elements are the values of the subset, sorted in nondescending order. If two subsets are equally far from the expected variance, choose the one whose return value comes first lexicographically. A int[] X comes lexicographically before a int[] Y if there is a position j such that, X[i]=Y[i] for all i<j, and X[j]<Y[j]. If X is a proper prefix of Y, it occurs lexicographically before it. | | Definition | | Class: | WhichData | Method: | bestVariance | Parameters: | int[], int, int | Returns: | int[] | Method signature: | int[] bestVariance(int[] sampleData, int varNum, int varDen) | (be sure your method is public) |
| | | | Notes | - | doubles will provide enough precision to properly solve this problem. Given two subsets, s1 and s2, the difference between |variance(s1)-varNum/varDen| and |variance(s2)-varNum/varDen| will be either 0, or greater than 1e-9, where variance(x) is the variance of subset x. | | Constraints | - | varNum will be between 0 and 10000 inclusive. | - | varDen will be between 1 and 10000 inclusive. | - | sampleData will contain between 2 and 16 elements inclusive. | - | Each element of sampleData will be between -10000 and 10000 inclusive. | | Examples | 0) | | | | Returns: { 1, 2, 3, 4, 5 } | The variance should be 40/20 = 2. The set of numbers {1,2,3,4,5} has
mean = (1+2+3+4+5)/5 = 3
varsum = 2^2 + 1^2 + 0^2 + 1^2 + 2^2 = 10
variance = 10/5 = 2
|
|
| 1) | | | | Returns: { 1, 2, 4, 5, 8 } | |
| 2) | | | {-10000,10000,-9999,9999,-9998,9000} | 10000 | 1 |
| Returns: { -10000, -9998 } | |
| 3) | | | {-10000,10000,-9999,9999,-9998,9998,1,1,2,2} | 9999 | 10000 |
| Returns: { -10000, -9998 } | |
| 4) | | | {500,500,500,500,500,500,500,580,
100,100,100,100,100,100,100,180} | 700 | 1 |
| Returns: { 100, 100, 100, 100, 100, 100, 100, 180 } | |
| 5) | | | | 6) | | | {2,5,8,15,-14,0,-2,3,0,-10,-3,-9,6,-13,4,-1} | 5787 | 170 |
| Returns: { -14, -10, -3, -1, 0, 0, 2, 3, 4, 5 } | |
| 7) | | | {-14,-3,-1,10,-5,0,13,6,11,9,5,6,3,-2,0,2} | 5061 | 225 |
| Returns: { -5, -3, -2, -1, 0, 2, 5, 6, 6, 11 } | |
| 8) | | | {0,-13,15,5,5,-7,-6,-7,-8,4,-12,-13,14,9,-3,-1} | 9262 | 197 |
| Returns: { -13, -13, -12, -7, -7, -6, -3, 4, 5, 5 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You have been given a rows-by-cols chessboard, with a list of squares cut out. The list of cutouts will be given in a String[] cutouts. Each element of cutouts is a comma-delimited lists of coords. Each coord has the form (quotes for clarity) "r c". If coord "r c" appears in an element of cutouts, it means that the square at row r column c (0-based) has been removed from the chessboard. This problem will involve placing rooks on a chessboard, so that they cannot attack each other. For a rook to attack a target piece, it must share the same row or column as the target. Your method will return an int that will be the maximum number of rooks that can be placed on the chessboard, such that no pair of rooks can attack each other. Rooks cannot be placed on cut out squares. The cut out squares do not affect where the rooks can attack. | | Definition | | Class: | RookAttack | Method: | howMany | Parameters: | int, int, String[] | Returns: | int | Method signature: | int howMany(int rows, int cols, String[] cutouts) | (be sure your method is public) |
| | | | Constraints | - | rows will be between 1 and 300 inclusive. | - | cols will be between 1 and 300 inclusive. | - | cutouts will contain between 0 and 50 elements inclusive. | - | Each element of cutouts will contain between 3 and 50 characters inclusive. | - | Each element of cutouts will be a comma delimited list of coords. Each coord will be of the form "r c", where - r and c are integers, with no extra leading zeros,
- r is between 0 and rows-1 inclusive,
- and c is between 0 and cols-1 inclusive.
| - | Each element of cutouts will not contain leading or trailing spaces. | | Examples | 0) | | | | Returns: 8 |
........
........
........
........
........
........
........
........
|
|
| 1) | | | 2 | 2 | {"0 0","0 1","1 1","1 0"} |
| Returns: 0 | |
| 2) | | | 3 | 3 | {"0 0","1 0","1 1","2 0","2 1","2 2"} |
| Returns: 2 | |
| 3) | | | | 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Given a sequence of uppercase letters, we want to remove all but one occurrence of each
letter, doing it in such a way that the
remaining letters are in alphabetical order. Of course, there may be no way to
do this, but if there is, we want to know which letters to remove.
Create a class MakeUnique that contains a method eliminated that is given a
String original, and returns original with the
eliminated letters replaced with periods ('.'). The remaining letters must
be in alphabetical order.
If there is no way to do this, return a String with length 0.
If there are several ways to do this, choose the one with the shortest length
between the first and last remaining letters. If there are still several ways,
return the String among these that comes earliest lexicographically ('.' comes
earlier than any letter in the ASCII sequence).
| | Definition | | Class: | MakeUnique | Method: | eliminated | Parameters: | String | Returns: | String | Method signature: | String eliminated(String original) | (be sure your method is public) |
| | | | Constraints | - | original will contain between 1 and 50 characters inclusive | - | each character in original will be an uppercase letter 'A'-'Z' | | Examples | 0) | | | | Returns: ".......ABCX" |
The 4 letters ABCX must remain, and in that order. "AB...CX...." would also leave these letters in the
appropriate order,
but the length between the first and last remaining letters
would be longer than in the given answer.
|
|
| 1) | | | | Returns: "A..B.CX...." |
"AB...CX...." and "A.B..CX...." are also possible and have the same
length between first and last remaining letters, but they come later
lexicographically than the given answer.
|
|
| 2) | | | | Returns: "" |
There is no way to eliminate letters and end up with C before X. |
|
| 3) | | | | 4) | | | "CABDEAFDEGSDABCDEADFGSEFBGS" |
| Returns: "............ABCDE..FGS....." | |
| 5) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Graphs are relatively common data structures in computer science. To visualize a graph, we often display it as a bitmap like this one:
However, an image is not very easy to work with for most purposes. It is usually much easier to perform common tasks (like finding a shortest path) if the graph is represented as a set of nodes and edges. In this problem, you will be given a graph as a bitmap, and are to convert it to a set of nodes and edges.
You have some information about the bitmap to make this task easier. First, you know that all nodes in the graph are represented by a single pixel of a certain color, which will be represented by an 'N' in the input. Furthermore, each edge will be a single color, represented by an 'E' in the input. The rest of the pixels will be represented by '.'s. After examining the images, you've come up with the following sketch for an algorithm to find and follow edges:
- First, find an 'N' that is adjacent to an 'E' (either orthogonally, or diagonally).
- Start following the edge in the same direction as you went to get from the 'N' to the 'E'.
- Then, as long as the edge has not terminated at an 'N', follow the edge ('E' pixels) by either continuing in the same direction, or if you cannot continue straight, by turning 45 degrees and continuing. You should always go straight when possible, but if you cannot go straight, you will be able to go either 45 degrees left, or 45 degrees right, though not both. In other words, if you cannot go straight, it will be unambiguous which way to turn (this is ensured by the constraints).
- If, at any point, there is an 'N' straight ahead, or there is not an edge straight ahead and there is an 'N' 45 degrees off from straight ahead, then the edge you are following terminates at that node. Again, this will not be ambiguous, so if there is neither an 'E' nor an 'N' straight ahead, then exactly one of the pixels 45 degrees off from straight will be 'N' or 'E'.
Your task is to write a method parse, which takes a String[], bitmap, each of whose elements represents a row in the bitmap, and returns a String[], each of whose elements represents a single node. To do this, you should first number all of the nodes, starting with the most upper most nodes, and breaking ties by doing the left most first. Your numbering should start at 0. Then, the ith element of the return should be a comma delimited list of all the <edge>s coming out of the ith node. Each <edge> should be formatted as <dest>:<length>, where <dest> is the number of the node to which this edge connects, and <length> is the number of 'E's in the edge. The list of edges in each element should be sorted in ascending order by the index of the element they connect to. Ties should be broken by sorting the edges in ascending order by length. | | Definition | | Class: | BitmapToGraph | Method: | parse | Parameters: | String[] | Returns: | String[] | Method signature: | String[] parse(String[] bitmap) | (be sure your method is public) |
| | | | Notes | - | Only list individual loops (edges from a node to itself) once. See example 4. | | Constraints | - | bitmap will contain between 1 and 50 elements, inclusive. | - | Each element of bitmap will contain the same number of characters. | - | Each element of bitmap will contain between 1 and 50 characters, inclusive. | - | Each character of bitmap will be an 'E', an 'N', or a '.'. | - | If you follow each edge as described in the problem statement, each edge will terminate at a node. | - | There will not be two adjacent 'N's. | - | All of the edges will be traversed in the same manner in both directions. In other words, if there is an edge from node i to node j, there will also be an edge from node j to node i which uses all of the same pixels. | - | There will be no ambiguity as to which way to go when a 45 turn must be made. | | Examples | 0) | | | {"NEEE.....N",
"....EEEEE.",
".........."} |
| Returns: { "1:8", "0:8" } | The upper left 'N' is node 0, and the upper right 'N' is node 1. There is an edge with 8 'E's connecting them, so element 0 of the return is "1:8" since the edge from 0 to 1 is of length 8. Similarly, element 1 of the return is "0:8" |
|
| 1) | | | | Returns: { "3:1", "2:1", "1:1", "0:1" } | The numbers of the nodes are as follows:
0.1
...
2.3
Thus, 0 is connected to 3, and 1 is connected to 2. |
|
| 2) | | | {"N..N..N",
".E.E.E.",
"..EEE..",
"NEEEEEN",
"..EEE..",
".E.E.E.",
"N..N..N"} |
| Returns: { "7:5", "6:5", "5:5", "4:5", "3:5", "2:5", "1:5", "0:5" } | |
| 3) | | | {".NE....NE..N",
"E..E...E.E..",
"E..E...E.E.E",
".EE....NE..E"}
|
| Returns: { "0:7", "3:2,3:4", "", "1:2,1:4" } | Notice that the loop from node 0 to itself is only listed once. Also note that when there are multiple edges from one node to another, they are sorted by length. Finally, note that there may be edge pixels that are not part of any edge. |
|
| 4) | | | {".EE....",
"E..E...",
"E..E...",
"NEEEEE.",
"...E..E",
"...E..E",
"...E..E",
"....EE."} |
| Returns: { "0:20" } | |
| 5) | | | | Returns: { "1:2,1:2", "0:2,0:2" } | |
| 6) | | | {"N..N.........",
".E.E.........",
"..EE....EN...",
"...E.N.E.....",
"...NEEEEEN...",
"...E.N.E.....",
"..EE....EN...",
".E.E.........",
"N..N........."} |
| Returns:
{ "6:4",
"4:3",
"6:3",
"6:1,7:3,8:4",
"1:3,5:5,9:3",
"4:5",
"0:4,2:3,3:1",
"3:3",
"3:4",
"4:3" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Often, a large task can be broken down into a number of smaller tasks, some of which are dependent on others. For example, developing a substantial piece of software might be broken down into 4 tasks: the database component, the server component, the client component, and integration of the three components. Each component can be written independently, but the final task of integrating the three components cannot be started until each of the components is finished.
We can think of these dependencies as a directed acyclic graph (DAG), where each node represents a task, and each directed edge represents a dependency. For example, the following graph represents the above example:
DB------>--\
\
Server------>Integration
/
Client-->--/
Your task is to determine how fast some set of tasks can be completed, given that you can work on at most 2 tasks at a time, and once you start working on a task, you must work on that task until it is finished. You are to write a method, fastest, which will take a DAG representing the dependencies of the tasks and the amount of time that each task takes to complete, and should return the smallest amount of time in which every task may be finished.
The DAG will be given to you as a String[], dag. Each element of dag will be formatted as "<time>:<d0>,<d1>,...", where each <di> indicates that the task represented by this element of dag is dependent on the task represented by the dith element of dag.
| | Definition | | Class: | Scheduling | Method: | fastest | Parameters: | String[] | Returns: | int | Method signature: | int fastest(String[] dag) | (be sure your method is public) |
| | | | Constraints | - | dag will contain between 1 and 12 elements, inclusive. | - | Each element of dag will be formatted as "<time>:<d0>,<d1>,...", where each <di> is between 0 and the number of elements in dag-1, inclusive (with no extra leading 0's anywhere). | - | Each <time> will be between 1 and 10, inclusive. | - | No element of dag will contain more than one occurrence of the same <di>. | - | Each element of dag will contain between 2 and 50 characters, inclusive. | - | dag will represent a directed acyclic graph. | | Examples | 0) | | | {"3:","2:","4:","7:0,1,2"} |
| Returns: 12 | This represents the following DAG (numbers represent the time it takes to complete a task represented by a node).
3-->--\
\
2------->7
/
4-->--/
This is similar to the example in the problem statement. Task 3 may not be started until tasks 0, 1, and 2 are all completed. So, the best way to do this is to start by doing both tasks 0 and 2. Then, at time 3, task 0 is completed, and you can start working on task 1. At time 4, you complete task 2, but there is nothing more to do, so at that point you are only working on one task. Then, at time 5, task 1 completes, and you can work on task 3, which completes at time 12.
Here is a table showing a timeline for one of the best ways to do it:
| Tasks being
Time | worked on or
| started
------+------
0 | 0,2
3 | 1,2
4 | 1
5 | 3
12 | done
|
|
| 1) | | | {"9:","8:","6:","4:","7:","7:0,1,2,3,4","3:2,3"} |
| Returns: 24 | This one is a little more complicated. It represents the following DAG (plugin users - please view the image in the applet):
|
|
| 2) | | | { "10:", "5:", "5:1", "5:1", "5:2,3" } |
| Returns: 20 | |
| 3) | | | {"1:","2:","4:","8:","6:","3:","7:","5:","9:","5:","10:","3:"} |
| Returns: 32 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Spelling in the English language doesn't make sense. Congress has enacted a plan for orthographical reform (loosely based on Mark Twain's plan for the improvement of English spelling) which will change the spelling of words in the English language gradually over the next 7 years. The plan is as follows:
Year 1:
- - If a word starts with "x", replace the "x" with a "z".
- - Change all remaining "x"s to "ks"s.
Year 2:
- - Change all "y"s to "i"s.
Year 3:
- - If a "c" is directly followed by an "e" or "i", change the "c" to an "s".
Year 4:
- - If a "c" is directly followed by a "k", remove the "c". Keep applying this rule as necessary (Example: "cck" becomes "k".)
Year 5:
- - If a word starts with "sch", change the "sch" to a "sk".
- - If a "ch" is directly followed by an "r", change the "ch" to a "k".
- - After applying the above rules, change all "c"s that are not directly followed by an "h", to a "k". (This includes all "c"s that are the last letter of a word.)
Year 6:
- - If a word starts with "kn" change the "kn" to an "n".
Year 7:
- - Change all double consonants of the same letter to a single consonant. A consonant is any letter that is not one of "a, e, i, o, u." (Example: "apple" becomes "aple"). Keep applying this rule as necessary (Example: "zzz" becomes "z".)
The plan requires that rules for each year are followed in the order they are presented, and changes for each year occur after all the changes from previous years.
Write a class Twain, which contains a method getNewSpelling. getNewSpelling takes as parameters an int year representing the number of years that have passed since the plan to improve the English language began, and a String phrase representing the English phrase to convert. For the purposes of the plan, a word is a sequence of lowercase letters ('a'-'z') bounded by spaces or the start/end of phrase. The method returns a String representing the converted phrase.
| | Definition | | Class: | Twain | Method: | getNewSpelling | Parameters: | int, String | Returns: | String | Method signature: | String getNewSpelling(int year, String phrase) | (be sure your method is public) |
| | | | Constraints | - | year will be between 0 and 7, inclusive | - | phrase will be between 0 and 50 characters, inclusive | - | phrase will contain only lowercase letters ('a'-'z') and spaces (' '). | - | phrase will not contain three or more of the same consonant in a row | | Examples | 0) | | | 1 | "i fixed the chrome xerox by the cyclical church" |
| Returns: "i fiksed the chrome zeroks by the cyclical church" | In year 1, the first "x" in "xerox" is changed to a "z". Then, the "x"s in "fixed" and "zerox" are changed to "ks"s. |
|
| 1) | | | 2 | "i fixed the chrome xerox by the cyclical church" |
| Returns: "i fiksed the chrome zeroks bi the ciclical church" | In year 2, the "y"s in "by" and "cyclical" are changed to "i"s. |
|
| 2) | | | | Returns: "this is unchanged" | Since the year is 0, no changes occur. |
|
| 3) | | | 7 | "sch kn x xschrx cknnchc cyck xxceci" |
| Returns: "sk n z zskrks nchk sik zksesi" | In year 1, the phrase becomes "sch kn z zschrks cknnchc cyck zksceci" due to rules concerning the letter "x". In year 2, all "y"s are changed to "i"s yielding "sch kn z zschrks cknnchc cick zksceci". In year 3, "ce" and "ci" are changed to "se" and "si" yielding "sch kn z zschrks cknnchc sick zkssesi". In year 4, "ck" is changed to "k" and the phrase becomes "sch kn z zschrks knnchc sik zkssesi". In year 5, words that begin with "sch" are made to begin with "sk", "chr" is changed to "kr", and all "c"s not followed by an "h" are changed to "k" yielding "sk kn z zskrks knnchk sik zkssesi". In year 6, words that start with "kn" now start with "n" and the phrase becomes "sk n z zskrks nnchk sik zkssesi". Finally, in year 7, double consonants are removed yielding the final result. |
|
| 4) | | | 7 | " concoction convalescence cyclical cello " |
| Returns: " konkoktion konvalesense siklikal selo " | |
| 5) | | | | Returns: "" | Don't forget the empty case. |
|
| 6) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You will be given a int[] twoShuffle with k elements denoting a method for shuffling a deck of cards. The deck in question will have k cards numbered 1 through k inclusive. Initially, all of the cards in the deck are arranged in ascending order. The ith (1-based) element of twoShuffle describes which card is in position i (1-based) after 2 identical shuffling procedures have completed. You will return a int[] that is exactly like the input, except it describes what a single shuffle would do. A shuffling procedure describes how the relative positions of the cards will change due to the shuffle. More precisely, if element s of a shuffling procedure is p, then the card that was in position p ends up in position s (again, all 1-based). For example,
twoShuffle = {3,4,1,2}
means that after two shuffles
the deck 1,2,3,4 would become 3,4,1,2.
You would return {2,3,4,1} since:
the deck 1,2,3,4 - after one shuffle - 2,3,4,1
- after another shuffle - 3,4,1,2.
If there are no possible solutions, return an empty int[]. If there is more than 1 possible solution, return the one that comes first lexicographically. A shuffle X comes lexicographically before a shuffle Y if there is a position j such that, X[i]=Y[i] for all i<j, and X[j]<Y[j]. | | Definition | | Class: | ShuffleMethod | Method: | oneTime | Parameters: | int[] | Returns: | int[] | Method signature: | int[] oneTime(int[] twoShuffle) | (be sure your method is public) |
| | | | Constraints | - | twoShuffle will contain between 3 and 50 elements inclusive. | - | Each element of twoShuffle will be between 1 and k inclusive, where k is the number of elements in twoShuffle. | - | twoShuffle will contain no duplicate elements. | | Examples | 0) | | | | 1) | | | | Returns: { 1, 2, 3, 4 } | The cards are unshuffled. Since 1,2,3,4 is a valid solution, and is lexicographically first, it is the return value. 2,1,4,3 is another valid solution, but it does not come before 1,2,3,4 lexicographically. |
|
| 2) | | | | Returns: { 3, 4, 5, 1, 2 } | Using the shuffle 3,4,5,1,2 twice we see that
1 -> 3 -> 5
2 -> 4 -> 1
3 -> 5 -> 2
4 -> 1 -> 3
5 -> 2 -> 4
In other words, the deck is transformed as follows:
1,2,3,4,5 -> 3,4,5,2,3 -> 5,1,2,3,4 |
|
| 3) | | | {2,4,6,5,1,8,10,9,3,12,11,13,7,15,16,17,14} |
| Returns: { 3, 6, 2, 8, 9, 4, 14, 5, 1, 15, 11, 16, 17, 10, 12, 13, 7 } | |
| 4) | | | {2,4,6,5,1,8,10,9,3,12,11,13,7} |
| Returns: { } | |
| 5) | | | {10,9,12,8,13,3,4,1,5,11,6,2,7} |
| Returns: { 9, 1, 4, 12, 11, 7, 3, 2, 10, 5, 13, 8, 6 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | There are various cities at points around the x-y plane that are separated by great geographical distances, and thus are hard to travel between. The mayors of the cities have decided that they will undertake a road-paving plan to connect all of the cities. However, they do not know how long this will take.
At time zero, the roads are of zero length. Each time unit, the roads leading out of each city in the four cardinal directions (north, east, south, and west) will be extended by one unit. When roads from two different cities both contain at least one point in common, their two cities are connected, and all cities connected to those two cities are also considered connected. Your job is to figure out how long the construction job will take.
You will be given a int[] x and a int[] y, where the location of the i-th city is at (x[i], y[i]). Given the locations of all the cities in the x-y plane, your method should return the first time unit at which all the cities are connected to each other. | | Definition | | Class: | CityLink | Method: | timeTaken | Parameters: | int[], int[] | Returns: | int | Method signature: | int timeTaken(int[] x, int[] y) | (be sure your method is public) |
| | | | Notes | - | Connectedness is transitive; i.e., if city A is connected to city B, and B is connected to C, then A is connected to C. | | Constraints | - | x will contain between 1 and 50 elements, inclusive. | - | y will contain the same number of elements as x. | - | Each element of x will be between -1000000 and 1000000, inclusive. | - | Each element of y will be between -1000000 and 1000000, inclusive. | - | No two cities will be at the same coordinates. | | Examples | 0) | | | | Returns: 5 | The road north from (0,0) will intersect the road west from (5,5) at t = 5. |
|
| 1) | | | | 2) | | | {0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,
27,28,29,30,31,32,33,34,35,36,37,38,39,40,41,42,43,44,45,46,47,48,49} | {0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,
27,28,29,30,31,32,33,34,35,36,37,38,39,40,41,42,43,44,45,46,47,48,49} |
| Returns: 1 | |
| 3) | | | | 4) | | | {1593,-88517,14301,3200,6,-15099,3200,5881,-2593,11,57361,-92990} | {99531,-17742,-36499,1582,46,34001,-19234,1883,36001,0,233,485} |
| Returns: 73418 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
A palindrome is a String that is spelled the same forward and backwards. Given a String
base that may or may not be a palindrome, we can always force base to be a palindrome by adding
letters to it. For example, given the word "RACE", we could add the letters "CAR" to its back to get "RACECAR" (quotes for clarity only). However, we are not restricted to adding letters at the back. For example, we
could also add the letters "ECA" to the front to get "ECARACE". In fact, we can add letters anywhere in the word, so we
could also get "ERCACRE" by adding an 'E' at the beginning, a 'C' after the 'R', and another 'R' before the final 'E'.
Your task is to make base into a palindrome by adding as few letters
as possible and return the resulting String. When there is more than one palindrome of minimal length that can be made, return the lexicographically earliest (that is, the one that occurs first in alphabetical order).
| | Definition | | Class: | ShortPalindromes | Method: | shortest | Parameters: | String | Returns: | String | Method signature: | String shortest(String base) | (be sure your method is public) |
| | | | Constraints | - | base contains between 1 and 25 characters, inclusive. | - | Every character in base is an uppercase letter ('A'-'Z'). | | Examples | 0) | | | | Returns: "ECARACE" | To make "RACE" into a palindrome, we must add at least three letters. However, there are
eight ways to do this by adding exactly three letters:
"ECARACE" "ECRARCE" "ERACARE" "ERCACRE"
"RACECAR" "RAECEAR" "REACAER" "RECACER"
Of these alternatives, "ECARACE" is the lexicographically earliest.
|
|
| 1) | | | | 2) | | | | 3) | | | | 4) | | | "ALRCAGOEUAOEURGCOEUOOIGFA" |
| Returns: "AFLRCAGIOEOUAEOCEGRURGECOEAUOEOIGACRLFA" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | It is time to arrange the seating around a circular table for a party. We
want to alternate boys and girls around the table. We have a list of all
the attendees and their genders. Each attendee is specified by a String that
consists of the name, followed by one space, followed by either "boy" or "girl".
In addition to the attendees, we need to seat
the HOST (a boy) and the HOSTESS (a girl) with the HOSTESS directly across from
the HOST. That means that half the attendees should be on the HOST's left, and half on his right.
Create a class PartySeats that contains a method seating that is given a
String[] attendees that lists all the attendees and their genders. The method
returns a String[] that gives the seating plan, starting with "HOST" and proceeding
clockwise around the table, including all the attendees and the HOSTESS.
If there is more than one possible seating plan,
return the one that comes first lexicographically. "First lexicographically" means that each successive element in the return should be chosen to be the earliest alphabetically that is consistent with a legal seating plan. If there is no legal seating
plan, the return should contain 0 elements.
| | Definition | | Class: | PartySeats | Method: | seating | Parameters: | String[] | Returns: | String[] | Method signature: | String[] seating(String[] attendees) | (be sure your method is public) |
| | | | Constraints | - | attendees will contain between 1 and 50 elements inclusive | - | each element of attendees will consists of a name followed by a single space followed by either "boy" or "girl". There will be no leading or trailing spaces. | - | each name will contain between 1 and 20 characters inclusive | - | each name will contain only uppercase letters 'A'-'Z' | - | no name will be "HOST" or "HOSTESS" | | Examples | 0) | | | {"BOB boy","SAM girl","DAVE boy","JO girl"} |
| Returns: { "HOST", "JO", "BOB", "HOSTESS", "DAVE", "SAM" } | A girl must follow the HOST, and JO comes earliest lexicographically. Then comes
a boy, and BOB is the earliest lexicographically. HOSTESS must come next so
she can be opposite the HOST and then DAVE and SAM must follow in that order
to honor the alternating gender requirement.
|
|
| 1) | | | | Returns: { } |
There are more boys than girls so we cannot alternate.
|
|
| 2) | | | {"JOHN boy","CARLA girl"} |
| Returns: { } |
There is no way to alternate gender and also have the HOST sit directly
across from the HOSTESS |
|
| 3) | | | {"BOB boy","SUZIE girl","DAVE boy","JO girl",
"AL boy","BOB boy","CARLA girl","DEBBIE girl"} |
| Returns:
{ "HOST",
"CARLA",
"AL",
"DEBBIE",
"BOB",
"HOSTESS",
"BOB",
"JO",
"DAVE",
"SUZIE" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
On most modern PC systems, there is a 2-wire bus called the System Management Bus, or SMBus, which is based on the I2C protocol. This bus is used to talk to multiple devices on the system such as temperature sensors, or batteries. The most significant achievement of the I2C protocol is that it requires no more than 2 wires, and is not susceptible to collisions (unlike other hardware protocols, like ethernet). Collisions occur when two masters (devices which transmit messages) try to transmit at the same time and the resulting data is invalid. For I2C, the first bit transmitted by one master which is different than the bit transmitted by another master causes an "arbitration" to occur. The data being transmitted on the bus is the logic and-ed value of all the data being transmitted by the masters. Therefore, if one master is transmitting a '1' and another is transmitting a '0', the '0' will be transmitted and the '1' will not. The master transmitting the '1' detects that it is not able to transmit, and stops transmitting. The arbitration is not detected by the master transmitting the '0', or by the slave receiving the '0', so the message can continue without any collisions.
Since '0' bits that occur earlier in messages always win, the master transmitting the lowest byte always wins arbitration. For example, if one master was transmitting "01234", and another master was transmitting "01245", both masters would transmit the first three bytes, but the first master would win arbitration on the fourth data byte, since '3' is less than '4'.
Multiple masters can start transmissions at the same time, but if a transmission has already started, another master cannot start one in the middle of the transmission. Therefore, if one master is transmitting "01234", and has already transmitted the '0', another master wanting to transmit "1234" cannot start its transmission until the first master is finished.
The speed of transmission of each master is also allowed to vary. The speed at which each byte is transferred is determined by the slowest transmitting master. If a master loses arbitration of the bus, it continues to drive the clock signal (which determines the speed) for the remainder of the current byte, but then stops driving the clock for the rest of the transmission. In other words, the speed that each byte is transmitted at is determined by the slowest master attempting to transmit during that byte, even if the master loses arbitration during that byte.
Given the description of the I2C protocol above, you are to simulate multiple masters transmitting messages on the bus, and return how long it takes to transmit them. You will be given a String[] messages, where each element is a message to be transmitted by a master device, and the messages consist of numeric digits '0'-'9'. You will also be given a int[] times, where each element is the number of milliseconds it takes for the corresponding master to transmit a byte. The master transmitting element i of messages is described by element i of times. Each master that loses arbitration will retry transmitting its message after the current message is finished. Note that all masters try transmitting their messages at the very beginning of the simulation.
| | Definition | | Class: | SMBus | Method: | transmitTime | Parameters: | String[], int[] | Returns: | int | Method signature: | int transmitTime(String[] messages, int[] times) | (be sure your method is public) |
| | | | Constraints | - | messages will contain between 1 and 50 elements, inclusive. | - | Each element of messages will contain between 1 and 50 characters, inclusive. | - | Each element of messages will consist only of numeric digits '0'-'9', inclusive. | - | No element of messages will be a prefix of, or be exactly the same as, any other element of messages. | - | times will contain the same number of elements as messages. | - | Each element of times will be between 1 and 100, inclusive. | | Examples | 0) | | | {"123","124","134"} | {1,2,3} |
| Returns: 25 |
Here is a graph of the bytes that are transmitted, which masters are transmitting for each byte, and how long each byte takes to transmit. '-' means the master is transmitting, and '+' means the master was driving clock, but lost arbitration:
Data: 123124134
Master1: ---
Master2: --+---
Master3: -+ -+ ---
Time taken: 332332333
For the first message, all masters successfully transmit the first byte. Since the slowest is 3 milliseconds, the first byte takes 3 milliseconds to transfer. On the second byte, the third master is arbitrated out since the other two transmit a lower byte. However, the third master still drives the clock for the byte, so it is transmitted at 3 milliseconds. The third byte is transmitted by the first two masters, and even though the second master loses arbitration, it drives the clock at 2 milliseconds. The total time for the first message is therefore 3+3+2 = 8 milliseconds long.
At this point, the second and third master still haven't transmitted their messages. The second message again takes 8 milliseconds because the third master is arbitrated out on the second byte.
The final message is transmitted only by the third master, and therefore takes 9 milliseconds to transmit. The total time for the entire sequence is 8+8+9=25 milliseconds.
|
|
| 1) | | | {"012", "1234", "1233", "1223", "1123"} | {4,1,5,2,9} |
| Returns: 94 |
Again, here is a graph which displays the bytes transmitted, which masters are transmitting, and the resulting times for each byte. '-' means the master is transmitting and '+' means the master is driving clock, but lost arbitration:
Data: 0121123122312331234
Master1: ---
Master2: + -+ --+ ---+----
Master3: + -+ --+ ----
Master4: + -+ ----
Master5: + ----
Time taken: 9449999555255551111
|
|
| 2) | | | {"0002019289019101039663222896280025447",
"00201873554718989597528841094293294387326",
"010699029378761", "0110118", "011143279122561420",
"001046384966198", "00200570375817801109530240012",
"0003163277589822", "01100240744590150136673219652442108",
"012033596872642679096489479354", "0121059494098",
"00001002303019117948961792176", "00216399923558", "02014",
"00224999120803846121427603894967637446889670369",
"01101009414735635151893037596051740080475886",
"0000101211809647472761605153430927981533514",
"176845042961739039392207791589",
"02000047506929386333221966659552927385017901842706",
"021001117450487502127241076595509511",
"021010776262723557108100899515",
"0210114830738951774606917781619656",
"0211798433083855430", "00000005842153604632996228135814",
"0001000766929248550736138555144997170272757787",
"0001010247593342056062432721557",
"01100004828618452515832113396660046634951",
"0132559984733529872911444204991646138116334788",
"0224149857349431864906523152249992",
"00001142929552573133212195441932219",
"0011090498947092002457447355036811372647896489218",
"000001275414757476198997466", "00010014",
"00111025861963467834393486017602553072649743",
"000102085", "00120882661783539",
"01100018938145727291185020",
"01100191373790478446634214244459341793", "0001129060",
"001111287431066271555393813846448",
"011010160778408696098486370196313", "0002125146381515395"} | {42, 86, 47, 86, 32, 95, 2, 98, 17, 58, 31, 32, 85, 77,
87, 61, 1, 20, 15, 80, 20, 95, 55, 87, 52, 14, 55, 68,
2, 66, 67, 3, 28, 97, 100, 67, 65, 20, 28, 77, 93, 64} |
| Returns: 71048 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Your boss, the absent-minded biologist, has gone too far. It's one thing if he chills his lemonade in the lab refrigerator, but this morning he inadvertently rearranged the petri dishes. Now the old bird is calling for Culture X. You've found a dish of amoebae that looks like it might be Culture X, and you even have a photograph of Culture X taken yesterday, but these critters move around freely. They spin and glide through the dish and even flip over, making it hard to tell whether you're still looking at the same culture.
You are given a String[] describing a known state of Culture X. Consider it as a rectangular grid of squares, each of which is marked either '.' or 'X'. An amoeba is a component formed of directly adjacent 'X' squares. If an amoeba is composed of more than one 'X' square, then each of its 'X' squares shares an edge with at least one other 'X' square in the same amoeba. No two 'X' squares belonging to different amoebae may share an edge, but they are permitted to neighbor each other diagonally. An amoeba may contain holes. For example, the dish below contains five amoebae. Observe that one of them comprises exactly two squares, while another consists of a single square.
...XXXXXXX.......
....X.X.X........
...XXXXXX........
...............XX
......X.....XX..X
.XXXXX........X.X
.X.XXX.......XXXX
Given a second String[] with the same dimensions, decide whether it depicts the same collection of amoebae. Amoebae are considered identical under rotation (at right angles), reflection (in the horizontal or vertical axis), and translation (to any extent, as long as the amoeba remains wholly within the petri dish). Return 0 if both dishes contain the same amoebae. Otherwise, return the sum of these two figures: (a) the number of amoebae in Culture X that are not present in the candidate dish and (b) the number of amoebae in the candidate dish that are not present in Culture X.
| | Definition | | Class: | Amoebae | Method: | cultureX | Parameters: | String[], String[] | Returns: | int | Method signature: | int cultureX(String[] known, String[] candidate) | (be sure your method is public) |
| | | | Constraints | - | known and candidate have the same number of elements | - | known and candidate each have between 1 and 50 elements, inclusive | - | all elements of known and candidate are equally long | - | elements of known and candidate are each between 1 and 50 characters long, inclusive | - | all characters in known and candidate must be either '.' or 'X' | | Examples | 0) | | | {"...XXXXXXX.......",
"....X.X.X........",
"...XXXXXX........",
"...............XX",
"......X.....XX..X",
".XXXXX........X.X",
".X.XXX.......XXXX"} | {"X.X..........XXXX",
"XXX...XX......X.X",
"X.X...XX........X",
"XXX...XX.......XX",
"X.X....X.X.......",
"XXX...XX.X.......",
"X.......X........"}
|
| Returns: 0 | You've found Culture X! All the amoebae have been translated since yesterday. The one that was in the lower right corner has also been reflected in the horizontal axis. The one at lower left has been reflected and rotated, as has the big ugly one with two holes. The amoeba formed of two squares has been rotated. |
|
| 1) | | | {"...XXXXXXX.......",
"....X.X.X........",
"...XXXXXX........",
"...............XX",
"......X.....XX..X",
".XXXXX........X.X",
".X.XXX.......XXXX"}
| {"X.X..........XXXX",
"XXX...XX......X.X",
"X.X...XX........X",
"......XX.......XX",
"X.X....X.X.......",
"XXX...XX.X.......",
"X.......X........"} |
| Returns: 3 | Four amoebae from Culture X are present in the candidate petri dish, but the big ugly one with two holes is missing. Furthermore, the candidate dish has two amoebae that were not present in Culture X. In total, this makes a difference of 1+2=3. |
|
| 2) | | | {"......................",
"....XXX...............",
"..X...X......XX.XX....",
"..XX.........XX.XX....",
"...XX.................",
"..........X...X..XXXX.",
"..XXX.....XX.XX.XX..XX",
"..X........X.X..X....X",
".....XX....XXX..X....X",
".....XX.....X...XX..XX",
"............X....XXXX."} | {"....X......XX.XX.XX...",
"....XXX....XX.XX.XX...",
".........XX...........",
".........XX...........",
"......................",
"..X....XXXX....X......",
"..X...XX..XX...X......",
".XXX..X....X..XXX.....",
".X.X..X....X..X.X.....",
"XX.XX.XX..XX.XX.XX....",
"X...X..XXXX..X...X...."} |
| Returns: 4 | Compared to Culture X, the candidate dish has an extra Y-shaped amoeba and an extra two-by-two amoeba, but it's missing a small L-shaped amoeba, and it doesn't have the squiggly one at all. That makes a symmetric difference of 4. |
|
| 3) | | | {".XXXX.X...X...XXXX..",
"X..XXXXXXX.X.....XX.",
".X.X.XX....X..XXX..X",
"..XXX.X.X....X......",
".XXXXX...X.....XXXX.",
"..X.XXX..XX....XXX.X",
".......XXX..X.X...XX",
"XX....X.....X...X.X.",
".....X.....XX..XXX.X",
"X...XX.X.X..X....X.X",
".X........X.........",
".X..X.........X.....",
"..X.X..X..........XX",
"........X.X...X.XX..",
"....X.......X.X..X.X",
"....XX..X..X.X.X...X",
"X.....X.........X.X.",
"X.X...X.............",
"..XXX.X...X..X..XX..",
"X.......XX...X......"} | {".X.....X.X......XX.X",
"X.X.X..X..XX........",
"X..XXX......X....XX.",
"XXXXX.............X.",
"XX.XXX...X.XX.XX..X.",
".XX.XX..XX.....X....",
"XXXX.X.X........XXX.",
".X....X..X..X.......",
".X.X..X......X.X...X",
".X..XXX..X.........X",
"X....X....X..X....X.",
".XX.....X......X....",
"......XXXX....X.....",
"...X...........X..XX",
"X.X...X....X.XX.....",
"X.X.XX..X......X....",
"X.X.XX.XX....X..X.X.",
"XX..XX..XX...XX...X.",
".X..X.XX....X...X...",
"..X..XX.XX..X.XX...."} |
| Returns: 0 | |
| 4) | | | {".....XXX...X.....XX.....XX.XX..XX..",
"..X.XX.XX.X....XXX....X......X.....",
".....XX...X....X.X....X.X.X........",
"X.....X.......XXX.X....X..XX.X.....",
".X.X....X.X........X..........XX...",
"........XX........X.....X..X.X.....",
".X..X...X...XX.X..X.....X..........",
"..............X......X...X....X..X.",
"XX..X.....XX..X...X...X.X..X....X..",
".X....XX..X...............X.X.XXXX.",
"...XXXXX.....X...X....X.XX..XXX..X.",
".........X..XX....X.X......XXXX....",
"..XXXX.X..XXX.X...........X..X.XX..",
"X...X...........X..X.X..X.XX..X.X..",
"..X....X.....X..X.X....X..X.X..XXXX",
"X..XXX..X..XX.XX.X..X...X...X..X.XX",
"....X....X.X...X..X..X..XXX...XXXX.",
".X..XX.XX.....X..XX..............XX",
"X..........X..X......XXXX.....X..X.",
".X.XXX...X..X..XX.X.X.X.X..X.......",
"X....X.........X....XXXX..X....X..X",
"..X.XX...X......X.X.......XX....X..",
"X.XXXX....X.XXX..XX..XXXX.X.XX.....",
"XXX.X........X.X..X..X....X..X.....",
"......X..X..XX..X.....XX........X..",
"...X.....XX..X..X..X.X......XX..X.X",
"..X..X.......XX......X......X...XX.",
"..X.XX......XX.X..X.....X...X.X..X.",
".X.X.XX.XXX.X..X.X.................",
".......X.XX..X..X..X.....X.X..XX..X",
"X...XX.......X...X...X.X....X......",
"X.......X.X......X.X.X......X.....X",
"....X.X.X.X....XXX..XX.....XX.X..X.",
"...X..X.X.XX......X.....XX.XXXX....",
"..X......X...X.X...X...X.X.XXXX...X"} | {"..XX......X..XX.X....X..X.XX..X....",
"...X.X.X.......X..X....X...........",
".XXX.X.XXX.....X.X.X..XX.XX.X.XX.XX",
"X...X......X.X........X...X.X.....X",
"X.....X....X...XX....X.X........XX.",
"..XXX...XXX............X.XX..XX..XX",
"....X.X..X..X.X...X.X....X.XX...X..",
".XX..X........XX..X......XX..X....X",
"...X......X...X..X...XX...XXX..X.X.",
"..XX...X..X...X...X.XX.....X.....X.",
".XX.X........X..X..X......XX.X.XX..",
".X.X..XX..X.XX......X....X.........",
"X..X.X..X........X.....X..X..XX...X",
"X.X.........X.XXX..XX..X.........X.",
".........X......XX.....X.....X..X.X",
"X..X.X.XXX.X.....X...X...X...X..X.X",
"X......X..X.XX.X......X....XX.....X",
"X.X...................X...X..X..XX.",
".........X........X..XX..X......X.X",
".X....X.X.X.......X..XX..XX.XXX...X",
".................XXX...XX...X..X...",
"..X...X...XX.....X.....X..XX....XX.",
".......XXX.XX....X...XX..XXX.X....X",
"........X...XXXX..X..........X.....",
"..........XX.X.XX.X.........XX....X",
"...X.....X.X.................X....X",
"...XX.X.....X.....XXXX...X...X.X...",
".............X.....X..X..X.X.X..X..",
"...............X....X....X..X.X.X.X",
".XX..XX........X.....X.XX....X....X",
"...X....X..X...XXX..X........X.....",
"X.XXX.XX..X......XX.X......X.X.X...",
".XX.X....X..X..X.X.................",
"....XXX.X.X......XX.....XX...X.XX..",
"......X....XX..X.....X...XX.X.....X"} |
| Returns: 64 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You will be given two int[]s lows and highs describing a group of integer ranges. lows[i] and highs[i] represent the lower and upper inclusive bounds for the ith range of numbers. None of the ranges will overlap. The total group of numbers used in this problem will be the combination of the ranges given in lows and highs.
You will also be given a numeric String lexPos and a String[] retInts containing numeric Strings.
Assume you listed all
possible permutations of the given group of numbers in ascending lexicographical order (no repeated permutations). Find
the permutation A that is in position lexPos lexicographically. Return a int[] with the same
number of elements as retInts whose kth element is A[retInts[k]]. lexPos is a 0-based
index into the list of possible permutations. Each element of retInts is a 0-based index into the relevant permutation.
In a lexicographical ordering, permutations are ordered by their first
elements, with ties broken by their second elements, further ties broken by their third elements, and so forth. If
lexPos is greater than the total possible number of lexicographical orderings (without repeats) T, then use
lexPos%T instead of lexPos (% denotes mod). | | Definition | | Class: | PermutationValues | Method: | getValues | Parameters: | int[], int[], String, String[] | Returns: | int[] | Method signature: | int[] getValues(int[] lows, int[] highs, String lexPos, String[] retInts) | (be sure your method is public) |
| | | | Notes | - | Each element of retInts and lexPos will fit in a long. | | Constraints | - | lows will contain between 1 and 50 elements inclusive | - | highs will contain the same number of elements as lows | - | lexPos will be between 0 and (2^63)-1 inclusive | - | lexPos will contain only digits (0-9), will not have extra leading zeros, and will not contain whitespace | - | lexPos will contain between 1 and 50 characters inclusive | - | retInts will contain between 1 and 50 elements inclusive | - | Each element of lows will be between -2^31 and (2^31)-1 inclusive | - | Each element of highs will be between -2^31 and (2^31)-1 inclusive | - | Element k of lows will not be greater than element k of highs | - | No two ranges will overlap | - | Each element of retInts will contain only digits (0-9), will not have extra leading zeros, and will not contain whitespace | - | Each element of retInts will contain between 1 and 50 characters inclusive | - | Each element of retInts will be between 0 and tot-1 inclusive, where tot is the total amount of numbers in all ranges combined | | Examples | 0) | | | {1} | {4} | "0" | {"0","1","2","3"} |
| Returns: { 1, 2, 3, 4 } | The 0th permutation lexicographically is the sequence 1,2,3,4. |
|
| 1) | | | | Returns: { 3, 2, 1 } | The 6 possible permutations, in order, are :
0) 1, 2, 3
1) 1, 3, 2
2) 2, 1, 3
3) 2, 3, 1
4) 3, 1, 2
5) 3, 2, 1
lexPos is 5 so you return the 0th, 1st, and 2nd elements of permutation 5
|
|
| 2) | | | {1,16} | {5,20} | "1000000" | {"0","1","2","3","4","5","6","7","8","9","1","2","3"} |
| Returns: { 3, 18, 19, 4, 20, 2, 16, 17, 1, 5, 18, 19, 4 } | Notice the repeated elements in retInts |
|
| 3) | | | {1} | {5} | "100000000000001" | {"0","1","2","3","4"} |
| Returns: { 2, 4, 5, 3, 1 } | |
| 4) | | | {-1000000000,500000} | {0,2000000000} | "99999999999999999" | {"2999500000","1234123","123344","9293939","2999500001","2999499950"} |
| Returns: { 1999999987, -998765877, -999876656, -990706061, 1999999982, 1999999949 } | |
| 5) | | | | 6) | | | {0,-100,101} | {99,-11,100000000} | "100000000000009" | {"4","100000087","7"} |
| Returns: { -96, 99999993, -93 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | In Chapter 7 of W.V. Quine's book "Mathematical Logic", Quine speaks about the necessity of parentheses but also of their obsfucating nature. To combat this he introduces "a graphical notation of dots". Where parentheses determine the outside boundaries of operands by marking them directly, dot notation does this by placing dots on each side of the operator. The left operand of the operator is determined by the number of dots prefixed to the operator; the operand is the substring with its end at the beginning of the prefixed dots and with its beginning at the end of the next larger grouping of dots suffixed to another operator, or at the beginning of the expression whichever comes first. The right side of the operator is determined likewise; it is the substring starting at the end of the suffixed dots and has its end at the beginning of the next larger grouping of dots prefixed to another operator or at the end of the expression, whichever comes first.
To be specific, all dot notation will be a <DotNotation> of this form (quotes added for clarity):
<DotNotation> := <Number> | <DotNotation><Dots><Operator><Dots><Number>
<Dots> := "" | <Dots>"."
<Operator> := "+" | "-" | "*" | "/"
<Number> := exactly one of "0123456789"
If an operator's operands reach across the entire expression, the operator is said to be dominant. Evaluation in dot notation involves finding the dominant operator, evaluating the left and right operands of that operator, and then evaluating that operator last. For example: in the dot notation expression "2*.1+3", "2" is the left operand of the '*' (consider no dots as a grouping of 0 dots) and "1+3" is the right operand of the '*', so this can be read with parentheses as "2*(1+3)", the '*' is dominant, and the expression evaluates to 8. Likewise, "2*.1..+3" refers to the expression "(2*1)+3", the '+' is dominant, and the expression evaluates to 5.
Dot notation can be ambiguous if there is more than one dominating operator. For example, in the expression "3+.5.*7" either operator may be dominant (both operators reach across the entire expression unopposed) so this could be parenthesized as either "3+(5*7)" or "(3+5)*7" and the expression could evaluate to either 38 or 56 respectively. There are even expressions that have no dominating operator, such as "1+...2....*.8..+7". Since there is no way to parenthesize this expression consistent with its dot notation, it cannot be legally evaluated.
You will be given a String in dot form, and you'll need to return the number of unique integers that the expression can evaluate to. For this problem, a specific evaluation is illegal if any part of the evaluation involves division by 0, any operand evaluates to a value that is less than -2000000000 or greater than 2000000000, or if the expression has no dominating operator thus preventing evaluation. If all possible evaluations of the dot notation are illegal return 0. | | Definition | | Class: | DotNotation | Method: | countAmbiguity | Parameters: | String | Returns: | int | Method signature: | int countAmbiguity(String dotForm) | (be sure your method is public) |
| | | | Notes | - | There is no normal order of operations in this problem; thus a+b*c is ambiguous. | | Constraints | - | dotForm will be between 1 and 25 characters long, inclusive. | - | dotForm will be a <DotNotation> as described above. | | Examples | 0) | | | | Returns: 1 | A single digit by itself has only one interpretation. |
|
| 1) | | | | Returns: 2 | This could be read (9+5)*3 or as 9+(5*3). Keep in mind that no order of operations is to be assumed. |
|
| 2) | | | | Returns: 1 | Here, the dot prefixed to the * gives it dominance, and this can only be read as (9+5)*3. |
|
| 3) | | | | Returns: 1 | The * is dominant here, so this is read as (1+2)*(3+4), and 21 is the only number it can be evaluated to. |
|
| 4) | | | | 5) | | | | Returns: 0 | Notice here how none of the operators in the expression can be dominating. Since none of these operators are dominating, none of these operators may be evaluated last, meaning that there is no valid interpretation of this expression. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You work for an electric company, and the power goes out in a rather large apartment complex with a lot of irate tenants. You isolate the problem to a network of sewers underneath the complex with a step-up transformer at every junction in the maze of ducts. Before the power can be restored, every transformer must be checked for proper operation and fixed if necessary. To make things worse, the sewer ducts are arranged as a tree with the root of the tree at the entrance to the network of sewers. This means that in order to get from one transformer to the next, there will be a lot of backtracking through the long and claustrophobic ducts because there are no shortcuts between junctions. Furthermore, it's a Sunday; you only have one available technician on duty to search the sewer network for the bad transformers. Your supervisor wants to know how quickly you can get the power back on; he's so impatient that he wants the power back on the moment the technician okays the last transformer, without even waiting for the technician to exit the sewers first.
You will be given three int[]'s: fromJunction, toJunction, and ductLength that represents each sewer duct. Duct i starts at junction (fromJunction[i]) and leads to junction (toJunction[i]). ductlength[i] represents the amount of minutes it takes for the technician to traverse the duct connecting fromJunction[i] and toJunction[i]. Consider the amount of time it takes for your technician to check/repair the transformer to be instantaneous. Your technician will start at junction 0 which is the root of the sewer system. Your goal is to calculate the minimum number of minutes it will take for your technician to check all of the transformers. You will return an int that represents this minimum number of minutes. | | Definition | | Class: | PowerOutage | Method: | estimateTimeOut | Parameters: | int[], int[], int[] | Returns: | int | Method signature: | int estimateTimeOut(int[] fromJunction, int[] toJunction, int[] ductLength) | (be sure your method is public) |
| | | | Constraints | - | fromJunction will contain between 1 and 50 elements, inclusive. | - | toJunction will contain between 1 and 50 elements, inclusive. | - | ductLength will contain between 1 and 50 elements, inclusive. | - | toJunction, fromJunction, and ductLength must all contain the same number of elements. | - | Every element of fromJunction will be between 0 and 49 inclusive. | - | Every element of toJunction will be between 1 and 49 inclusive. | - | fromJunction[i] will be less than toJunction[i] for all valid values of i. | - | Every (fromJunction[i],toJunction[i]) pair will be unique for all valid values of i. | - | Every element of ductlength will be between 1 and 2000000 inclusive. | - | The graph represented by the set of edges (fromJunction[i],toJunction[i]) will never contain a loop, and all junctions can be reached from junction 0. | | Examples | 0) | | | | Returns: 10 | The simplest sewer system possible. Your technician would first check transformer 0, travel to junction 1 and check transformer 1, completing his check. This will take 10 minutes. |
|
| 1) | | | | Returns: 40 | Starting at junction 0, if the technician travels to junction 3 first, then backtracks to 0 and travels to junction 1 and then junction 2, all four transformers can be checked in 40 minutes, which is the minimum. |
|
| 2) | | | {0,0,0,1,4} | {1,3,4,2,5} | {10,10,100,10,5} |
| Returns: 165 | Traveling in the order 0-1-2-1-0-3-0-4-5 results in a time of 165 minutes which is the minimum. |
|
| 3) | | | {0,0,0,1,4,4,6,7,7,7,20} | {1,3,4,2,5,6,7,20,9,10,31} | {10,10,100,10,5,1,1,100,1,1,5} |
| Returns: 281 | Visiting junctions in the order 0-3-0-1-2-1-0-4-5-4-6-7-9-7-10-7-8-11 is optimal, which takes (10+10+10+10+10+10+100+5+5+1+1+1+1+1+1+100+5) or 281 minutes. |
|
| 4) | | | {0,0,0,0,0} | {1,2,3,4,5} | {100,200,300,400,500} |
| Returns: 2500 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | There are several distinct points located on the non-negative side of the x-axis. One of those points is at the origin (at 0). For each unique pair of points we write down the distance between them. Note that pairs (a, b) and pairs (b, a) are not unique pairs.
Given a int[] of those distances, return a int[] containing the locations of all points arranged in ascending order. If more than one set of locations is possible then return the set (represented as a int[]) which is lexicographically earliest (see example 1). A int[], n, comes before a int[], m, lexicographically if and only if, for the first element in which they differ, the element of n is smaller. If no set of locations is possible then return an empty int[].
For example: distances = {5, 2, 1, 6, 2, 3, 3, 4, 5, 6, 3, 9, 1, 4, 1}. This in order is {1, 1, 1, 2, 2, 3, 3, 3, 4, 4, 5, 5, 6, 6, 9}. The only possible arrangement of points is the following:
0 1 2 3 4 5 6 7 8 9
--x----|----|----x----x----x----x----|----|----x--
Here 'x' represents a point. Thus the method should return {0, 3, 4, 5, 6, 9} | | Definition | | Class: | PointsOnAxis | Method: | findPoints | Parameters: | int[] | Returns: | int[] | Method signature: | int[] findPoints(int[] distances) | (be sure your method is public) |
| | | | Notes | - | There cannot be more than one point at the same location, because elements in distances are never 0. | | Constraints | - | the number of elements in distances will be N(N-1)/2, where N is the number of points. | - | N will be between 2 and 10 inclusive. | - | each element in distances will be between 1 and 1000000 inclusive. | | Examples | 0) | | | {5, 2, 1, 6, 2, 3, 3, 4, 5, 6, 3, 9, 1, 4, 1} |
| Returns: { 0, 3, 4, 5, 6, 9 } | |
| 1) | | | | Returns: { 0, 20, 120 } | The possible sets of locations are {0,100,120} and {0,20,120}. Since 20 comes before 100, the method should return {0,20,120}. |
|
| 2) | | | | 3) | | | | Returns: { } | There are no possible sets of locations. |
|
| 4) | | | {237601, 843904, 56786, 429289, 52254, 83576, 220417,
606303, 180815, 191688, 185347, 154025, 17184, 787118,
414615, 791650, 760328, 623487, 372503, 4532, 26790,
163631, 377035, 345713, 208872, 31322, 168163, 136841} |
| Returns: { 0, 52254, 56786, 83576, 220417, 237601, 429289, 843904 } | |
| 5) | | | {1, 1, 1, 1, 2, 2, 3, 4, 4, 5, 5, 5, 6, 6, 7} |
| Returns: { 0, 1, 2, 5, 6, 7 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Julius Caesar used a system of cryptography, now known as Caesar Cipher, which shifted each letter 2 places further through the alphabet (e.g. 'A' shifts to 'C', 'R' shifts to 'T', etc.). At the end of the alphabet we wrap around, that is 'Y' shifts to 'A'.
We can, of course, try shifting by any number. Given an encoded text and a number of places to shift, decode it.
For example, "TOPCODER" shifted by 2 places will be encoded as "VQREQFGT". In other words, if given (quotes for clarity) "VQREQFGT" and 2 as input, you will return "TOPCODER". See example 0 below.
| | Definition | | Class: | CCipher | Method: | decode | Parameters: | String, int | Returns: | String | Method signature: | String decode(String cipherText, int shift) | (be sure your method is public) |
| | | | Constraints | - | cipherText has between 0 to 50 characters inclusive | - | each character of cipherText is an uppercase letter 'A'-'Z' | - | shift is between 0 and 25 inclusive | | Examples | 0) | | | | 1) | | | "ABCDEFGHIJKLMNOPQRSTUVWXYZ" | 10 |
| Returns: "QRSTUVWXYZABCDEFGHIJKLMNOP" | |
| 2) | | | | 3) | | | | 4) | | | | 5) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | We have a list of airline flights (directed edges between two nodes in a graph), and want to determine how many distinct directed quadrilaterals (directed cycles of length 4, which contain 4 distinct nodes) there are in the flight graph such that the nodes on opposite corners of the quadrilateral are not connected in either direction. Thus, we would count the graph on the left, but not the one on the right (the diagonal edge can be directed either way, or both ways - it doesn't matter):
o--->o o--->o
^ ^ ^\ ^
| | | \ |
| v | \ v
o<-->o o<-->o
You will be given a String[] flights representing the flights. Element i of flights is a single space delimited list of integers (without extra leading 0's or leading/trailing spaces), where each integer j in the list indicates that there is an edge from i to j. An integer, j may appear more than once in element i meaning that there are multiple flights from i to j, each of which should be considered distinct. However, regardless of how many flights there are from one node to another, a quadrilateral must contain exactly 4 distinct nodes. Two quadrilaterals are considered distinct if and only if the sets of flights that they use are distinct. Thus, there may be many quadrilaterals that use the same four nodes, but different edges. Your task is to write a class DQuads, with a method count, which returns the total number of distinct quadrilaterals.
| | Definition | | Class: | DQuads | Method: | count | Parameters: | String[] | Returns: | int | Method signature: | int count(String[] flights) | (be sure your method is public) |
| | | | Constraints | - | flights will contain between 4 and 50 elements, inclusive. | - | Each element of flights will be a single space delimited list of integers, with no extra leading 0's and no leading or trailing spaces. | - | Each integer in each element of flights will be between 0 and the length of flights - 1, inclusive. | - | Each element of flights will contain between 0 and 50 characters, inclusive. | - | Element i of flights will not contain the integer i as one of its terms. | | Examples | 0) | | | {"1 1 1 1 1 1 1 1 1 1","2","3","0"} |
| Returns: 10 | This is just a single directed square. Since there are 10 edges from 0 to 1, there are 10 distinct, directed quadrilaterals. |
|
| 1) | | | {"1 1 1 1 1 1 1 1 1 1","2","3","0 1"} |
| Returns: 0 | This is the same as the last example, except with a cross edge from 3 to 1 included. The cross edge is in all the quadrilaterals from the previous example, and so none of them count any more. |
|
| 2) | | | {"","6 0 2","","6 6 4","6 5 5 0","",""} |
| Returns: 0 | |
| 3) | | | | 4) | | | {"3 4 8 3 7","0","4 0","","1 7 7 0","0 0 2","5 4 8 3 8","5 4 5 5 6",""} |
| Returns: 6 | |
| 5) | | | {"3 3 6","0","5 5 6 3","2 4 1 4","6 3 6 6","3","5 0 2"} |
| Returns: 26 | |
| 6) | | | {"8 1 2 4 8 12 4 5 11 10 6 2","5 3 15 7 15 15 12 0 8 9 2 0 13 9 8 7 4 7 9 11 11",
"6 7 11 9 10 1 12 9","6 6 9 7 6 1 14 1 6 7 10 6 15 6 14 16 10 11 13 4 7",
"14 15 16 0 13 2 5 16 6","2 7 16 13 16 10 16 0 8 6 0 2 6",
"8 8 4 11 3 14 9 14 14 0 5 10 13 3 11 9 5 7","13 15 6 1 3 13 6 6 8 9 6 4 10",
"4 0 1 4 12 1 2 0 14 9 6 4 16 10 7 6 9 7 13 14","11 12 4 12",
"6 4 4 9 3 1 8 0 14 14 9 14 16 5 8 16 5 12 4 5 1 12",
"14 7 14 8 4 16 6 3 13 6 10 7 13 3","15 4","12 14 14 0 8 12 11 4 3 1 12 1",
"13 4 4 6 12 0","9 0 2 9 5 10","6 15 6 13 4 5 1 6"} |
| Returns: 140 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | NOTE: There are images in the examples section of this problem statement that help describe the problem. Please view the problem statement in the HTML window to view them.
Given a picture composed entirely of horizontal and vertical line segments, calculate the minimum number of times you must lift your pen to trace every line segment in the picture exactly n times.
Each line segment will be of the form "<x1> <y1> <x2> <y2>" (quotes for clarity), representing a segment from (x1,y1) to (x2,y2). Segments may cross each other. Segments may also overlap, in which case you should count the overlapping region as appearing in the drawing only once. For example, say the drawing were composed of two lines: one from (6,4) to (9,4), and one from (8,4) to (14,4). Even though they overlap from (8,4) to (9,4), you should treat the drawing as if it were a single line from (6,4) to (14,4). You would not need to lift your pen at all to trace this drawing. | | Definition | | Class: | PenLift | Method: | numTimes | Parameters: | String[], int | Returns: | int | Method signature: | int numTimes(String[] segments, int n) | (be sure your method is public) |
| | | | Notes | - | The pen starts on the paper at a location of your choice. This initial placement does not count toward the number of times that the pen needs to be lifted. | | Constraints | - | segments will contain between 1 and 50 elements, inclusive. | - | Each element of segments will contain between 7 and 50 characters, inclusive. | - | Each element of segments will be in the format "<X1>_<Y1>_<X2>_<Y2>" (quotes for clarity). The underscore character represents exactly one space. The string will have no leading or trailing spaces. | - | <X1>, <Y1>, <X2>, and <Y2> will each be between -1000000 and 1000000, inclusive, with no unnecessary leading zeroes. | - | Each element of segments will represent a horizontal or vertical line segment. No line segment will reduce to a point. | - | n will be between 1 and 1000000, inclusive. | | Examples | 0) | | | {"-10 0 10 0","0 -10 0 10"} | 1 |
| Returns: 1 | This picture looks like a plus sign centered at the origin. One way to trace this image is to start your pen at (-10,0), move right to (10,0), lift your pen and place it at (0,-10), and then move up to (0,10). There is no way to trace the picture without lifting your pen at all, so the method returns 1. |
|
| 1) | | | {"-10 0 0 0","0 0 10 0","0 -10 0 0","0 0 0 10"} | 1 |
| Returns: 1 | The picture is the same as the previous example, except that it has been described with four line segments instead of two. Therefore, the method still returns 1. |
|
| 2) | | | {"-10 0 0 0","0 0 10 0","0 -10 0 0","0 0 0 10"} | 4 |
| Returns: 0 | You are now required to trace each segment exactly 4 times. You can do so without lifting your pen at all. Start at (0,0). Move your pen left to (-10,0), then back right to (0,0), then left again to (-10,0), then right again to (0,0). You have now traced the first line segment 4 times. Repeat this process for the other three segments as well. Since no pen lifts were required, the method returns 0. |
|
| 3) | | | {"0 0 1 0", "2 0 4 0", "5 0 8 0", "9 0 13 0",
"0 1 1 1", "2 1 4 1", "5 1 8 1", "9 1 13 1",
"0 0 0 1", "1 0 1 1", "2 0 2 1", "3 0 3 1",
"4 0 4 1", "5 0 5 1", "6 0 6 1", "7 0 7 1",
"8 0 8 1", "9 0 9 1", "10 0 10 1", "11 0 11 1",
"12 0 12 1", "13 0 13 1"} | 1 |
| Returns: 6 | The picture looks like this:

To trace the picture using the minimum number of pen lifts, refer to the following diagram:

Start by placing your pen at the yellow dot. Trace the yellow square. Now lift your pen and place it on the red dot. Move downward, tracing the vertical line segment, and then around the perimeter of the red rectangle. Lift your pen again and place it on the green dot. Trace the green lines using the same method as you did for the red lines. Lift your pen a third time, placing it on the magenta dot. Trace the magenta lines in a similar fashion. You will need to lift your pen three more times to trace each of the leftover white segments, for a grand total of 6 pen lifts. |
|
| 4) | | | {"-2 6 -2 1", "2 6 2 1", "6 -2 1 -2", "6 2 1 2",
"-2 5 -2 -1", "2 5 2 -1", "5 -2 -1 -2", "5 2 -1 2",
"-2 1 -2 -5", "2 1 2 -5", "1 -2 -5 -2", "1 2 -5 2",
"-2 -1 -2 -6","2 -1 2 -6","-1 -2 -6 -2","-1 2 -6 2"} | 5 |
| Returns: 3 | This is an example of overlap. Once all the segments are drawn, the picture looks like this:
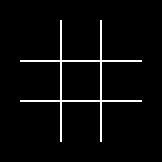
You would need to lift your pen 3 times to trace every segment in this drawing exactly 5 times. |
|
| 5) | | | {"-252927 -1000000 -252927 549481","628981 580961 -971965 580961",
"159038 -171934 159038 -420875","159038 923907 159038 418077",
"1000000 1000000 -909294 1000000","1000000 -420875 1000000 66849",
"1000000 -171934 628981 -171934","411096 66849 411096 -420875",
"-1000000 -420875 -396104 -420875","1000000 1000000 159038 1000000",
"411096 66849 411096 521448","-971965 580961 -909294 580961",
"159038 66849 159038 -1000000","-971965 1000000 725240 1000000",
"-396104 -420875 -396104 -171934","-909294 521448 628981 521448",
"-909294 1000000 -909294 -1000000","628981 1000000 -909294 1000000",
"628981 418077 -396104 418077","-971965 -420875 159038 -420875",
"1000000 -1000000 -396104 -1000000","-971965 66849 159038 66849",
"-909294 418077 1000000 418077","-909294 418077 411096 418077",
"725240 521448 725240 418077","-252927 -1000000 -1000000 -1000000",
"411096 549481 -1000000 549481","628981 -171934 628981 923907",
"-1000000 66849 -1000000 521448","-396104 66849 -396104 1000000",
"628981 -1000000 628981 521448","-971965 521448 -396104 521448",
"-1000000 418077 1000000 418077","-1000000 521448 -252927 521448",
"725240 -420875 725240 -1000000","-1000000 549481 -1000000 -420875",
"159038 521448 -396104 521448","-1000000 521448 -252927 521448",
"628981 580961 628981 549481","628981 -1000000 628981 521448",
"1000000 66849 1000000 -171934","-396104 66849 159038 66849",
"1000000 66849 -396104 66849","628981 1000000 628981 521448",
"-252927 923907 -252927 580961","1000000 549481 -971965 549481",
"-909294 66849 628981 66849","-252927 418077 628981 418077",
"159038 -171934 -909294 -171934","-252927 549481 159038 549481"} | 824759 |
| Returns: 19 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A bunch of people in a large park area are setting up a telephone network using receivers directly connected by wires. There is a lot of spare wire, so the connections need not be straight. The only restriction on the medium is that there be no crossings, since they could cause interference. In other words, if observed from a helicopter above the park, none of the wires can appear to be crossing over one another. Initially, the group of people set up a base network where person 0 is connected to person 1, person 1 is connected to person 2, ..., person i is connected to person i+1, ..., and the last person is connected back to person 0 forming a cycle. Afterwards many other connections were built. The problem is, some of the new connections may have forced crossings to occur.
Given the additional connections that were formed, your method will determine the minimum number of connections that need be removed such that there will not be any crossings. Note that, given a particular set of connections, if it is possible to lay the wires such that they will not cross, the people will do so. This means the people may change their locations and how much wire they use in order to remove crossings. They will not succeed if a crossing is inevitable. Also, since the base network must not be damaged, the removed connections cannot include any of the base network connections.
You will be given 2 int[]s connect1 and connect2 representing the additional connections formed. If the kth elements of connect1 and connect2 are a and b respectively, person a and person b have formed a connection. Note, connections are symmetric, so if a and b are connected then b and a are connected. You will also be given an int numPeople representing the number of people forming the network. Each element of connect1 and connect2 will be integers between 0 and numPeople-1 inclusive. | | Definition | | Class: | TelephoneGame | Method: | howMany | Parameters: | int[], int[], int | Returns: | int | Method signature: | int howMany(int[] connect1, int[] connect2, int numPeople) | (be sure your method is public) |
| | | | Notes | - | Without loss of generality, you can assume that the people are arranged in a circular pattern. Continuing this image, the base network would be seen from a helicopter as a circle. | | Constraints | - | connect1 must contain between 1 and 18 elements inclusive | - | connect1 must contain the same number of elements as connect2 | - | numPeople must be between 4 and 10000 inclusive | - | Element i of connect1 will not be equal to element i of connect2 | - | Each element of connect1 and connect2 will be between 0 and numPeople-1 inclusive | - | There will be no repeated connections. In other words, if a connection from a to b exists, there will be no other connections of the form a to b or b to a allowed as input. This includes the original connections, so there will be no connections from i to i+1, or from 0 to the last person. | | Examples | 0) | | | | Returns: 0 | The given connections can easily be formed without crossings as depicted below:
_______
/ |
0---1 /
| /| /
| / | /
|/ |/
3---2
|
|
| 1) | | | {4,4,4,5,5,6} | {6,7,8,7,8,8} | 10 |
| Returns: 1 | There is no way for all of these connections to be added without a crossing. |
|
| 2) | | | | 3) | | | {0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,18} | {2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,18,19,20} | 100 |
| Returns: 0 | |
| 4) | | | {0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17} | {30,31,32,33,34,35,36,37,38,39,40,41,42,43,44,45,46,47} | 1000 |
| Returns: 16 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | When studying set theory, the line between elements and sets is often blurred. Using the following definitions, you are going to write a function that will help students in a set theory class make such distinctions.
An atom is something that is not a set. In this problem atoms will be strings of lowercase letters.
A set is a collection of elements. The elements can be other sets, or atoms. In this problem, sets will be represented as comma-delimited lists of elements, the whole of which is enclosed in curly brackets ( '{' and '}' ). A set may be empty, and is thus denoted as "{}". This notation convention will be used throughout the rest of the problem statement.
x is a member of a set B if B contains x. For example, a, {b}, and {c,{a}} are all members of {a,{b},{c,{a}}}. b and {} are not members of this set.
Two sets are equivalent if and only if they contain exactly the same elements. Worded differently, two sets are NOT equivalent if there is an element contained by one that isn't contained by the other. For example, {a,{b},c}, {a,c,{b}}, and {a,a,{b},c} are all equivalent since they contain the same elements. The set {a,{b},c,b} is not equivalent to the previous three since none of them contain the element b. Note that duplication of elements does not affect a set.
A set A is a subset of a set B if and only if B contains every element that A contains and possibly others as well. For example, {}, {a,a,b}, {a,{c},b}, and {{c},a,b,c} are all subsets of {{c},a,c,b}. Note that {a,a,b} contains elements a and b, which are both contained in {{c},a,c,b}.
A set A is a proper subset of a set B if and only if A is a subset of B and they are not equivalent.
A set A is the powerset of a set B if and only if A contains every possible subset of B and nothing else. For example, {{a},{b},{a,b},{}} is the powerset of {a,b}. {a,{a},{}} is not the powerset of {a}.
A set A is equipotent to a set B if and only if A and B contain the same number of distinct elements. The word distinct here is only used for added emphasis. For example, {a,b,c}, {aa,bb,cc}, and {d,{e},f,f} are all equipotent to each other because they each contain 3 distinct elements. {a,a,a}, {a,b,c,{}} and {a,a,b} are not equipotent to the previous three.
Given 2 Strings a and b representing sets, your function will return a String[] containing each of the following that holds true for a and b:
"MEMBER" : if a is a member of b
"EQUIVALENT" : if a and b are equivalent
"SUBSET" : if a is a subset of b
"PROPER SUBSET" : if a is a proper subset of b
"POWERSET" : if a is the powerset of b
"EQUIPOTENT" : if a and b are equipotent
The returned String[] should be sorted in lexicographic ('A'-'Z') order.
| | Definition | | Class: | SetComparison | Method: | relation | Parameters: | String, String | Returns: | String[] | Method signature: | String[] relation(String a, String b) | (be sure your method is public) |
| | | | Constraints | - | a must contain between 2 and 50 characters inclusive | - | b must contain between 2 and 50 characters inclusive | - | Both a and b must adhere to the following grammar: VALID = "{" LIST "}" | "{}" LIST = ITEM | ITEM "," LIST ITEM = VALID | ATOM ATOM = positive length lowercase string | - | Both a and b will only contain lowercase letters ('a'-'z'), brackets ('{' and '}'), and commas (',') | | Examples | 0) | | | | Returns: { "PROPER SUBSET", "SUBSET" } | The empty set is always a subset of any set. Since a and b do not represent equivalent sets, it is a proper subset as well. |
|
| 1) | | | "{{a,b},{a},{b},{}}" | "{a,b}" |
| Returns: { "POWERSET" } | Here the set represented by a contains every possible subset of the set represented by b. |
|
| 2) | | | "{a,b,c,d,e}" | "{a,a,b,b,c,c,d,d,e,e}" |
| Returns: { "EQUIPOTENT", "EQUIVALENT", "SUBSET" } | Only difference is repeated elements. |
|
| 3) | | | | Returns: { "MEMBER", "PROPER SUBSET", "SUBSET" } | |
| 4) | | | "{a,a,{a},{a},{a,a,a},{},{},a,aa}" | "{a,{a},{},aa}" |
| Returns: { "EQUIPOTENT", "EQUIVALENT", "SUBSET" } | |
| 5) | | | "{frank,bob}" | "{{},{frank,frank},{bob},{frank,bob,bob},{}}" |
| Returns: { "MEMBER" } | |
| 6) | | | "{{a,b},{a},{a,b},{},{b},{b,a}}" | "{a,b}" |
| Returns: { "POWERSET" } | |
| 7) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | An essential part of circuit design and general system optimization is critical path analysis. On a chip, the critical path represents the longest path any signal would have to travel during execution. In this problem we will be analyzing chip designs to determine their critical path length. The chips in this problem will not contain any cycles, i.e. there exists no path from one component of a chip back to itself.
Given a String[] connects representing the wiring scheme, and a String[] costs representing the cost of each connection, your method will return the size of the most costly path between any 2 components on the chip. In other words, you are to find the longest path in a directed, acyclic graph. Element j of connects will list the components of the chip that can be reached directly from the jth component (0-based). Element j of costs will list the costs of each connection mentioned in the jth element of connects. As mentioned above, the chip will not contain any cyclic paths. For example:
connects = {"1 2",
"2",
""}
costs = {"5 3",
"7",
""}
In this example, component 0 connects to components 1 and 2 with costs 5 and 3 respectively. Component 1 connects to component 2 with a cost of 7. All connections mentioned are directed. This means a connection from component i to component j does not imply a connection from component j to component i. Since we are looking for the longest path between any 2 components, your method would return 12.
| | Definition | | Class: | Circuits | Method: | howLong | Parameters: | String[], String[] | Returns: | int | Method signature: | int howLong(String[] connects, String[] costs) | (be sure your method is public) |
| | | | Constraints | - | connects must contain between 2 and 50 elements inclusive | - | connects must contain the same number of elements as costs | - | Each element of connects must contain between 0 and 50 characters inclusive | - | Each element of costs must contain between 0 and 50 characters inclusive | - | Element i of connects must contain the same number of integers as element i of costs | - | Each integer in each element of connects must be between 0 and the size of connects-1 inclusive | - | Each integer in each element of costs must be between 1 and 1000 inclusive | - | Each element of connects may not contain repeated integers | - | Each element of connects must be a single space delimited list of integers, each of which has no extra leading zeros. There will be no leading or trailing whitespace. | - | Each element of costs must be a single space delimited list of integers, each of which has no extra leading zeros. There will be no leading or trailing whitespace. | - | The circuit may not contain any cycles | | Examples | 0) | | | {"1 2",
"2",
""} | {"5 3",
"7",
""} |
| Returns: 12 | |
| 1) | | | {"1 2 3 4 5","2 3 4 5","3 4 5","4 5","5",""} | {"2 2 2 2 2","2 2 2 2","2 2 2","2 2","2",""} |
| Returns: 10 | The longest path goes from 0-1-2-3-4-5 for a cost of 10. |
|
| 2) | | | {"1","2","3","","5","6","7",""} | {"2","2","2","","3","3","3",""} |
| Returns: 9 | The 0-1-2-3 path costs 6 whereas the 4-5-6-7 path costs 9 |
|
| 3) | | | {"","2 3 5","4 5","5 6","7","7 8","8 9","10",
"10 11 12","11","12","12",""} | {"","3 2 9","2 4","6 9","3","1 2","1 2","5",
"5 6 9","2","5","3",""} |
| Returns: 22 | |
| 4) | | | {"","2 3","3 4 5","4 6","5 6","7","5 7",""} | {"","30 50","19 6 40","12 10","35 23","8","11 20",""} |
| Returns: 105 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Suppose you had an n by n chess board and a super piece called a kingknight. Using only one move the kingknight denoted 'K' below can reach any of the spaces denoted 'X' or 'L' below:
.......
..L.L..
.LXXXL.
..XKX..
.LXXXL.
..L.L..
.......
In other words, the kingknight can move either one space in any direction (vertical, horizontal or diagonally) or can make an 'L' shaped move. An 'L' shaped move involves moving 2 spaces horizontally then 1 space vertically or 2 spaces vertically then 1 space horizontally. In the drawing above, the 'L' shaped moves are marked with 'L's whereas the one space moves are marked with 'X's. In addition, a kingknight may never jump off the board.
Given the size of the board, the start position of the kingknight and the end position of the kingknight, your method will return how many possible ways there are of getting from start to end in exactly numMoves moves. start and finish are int[]s each containing 2 elements. The first element will be the (0-based) row position and the second will be the (0-based) column position. Rows and columns will increment down and to the right respectively. The board itself will have rows and columns ranging from 0 to size-1 inclusive.
Note, two ways of getting from start to end are distinct if their respective move sequences differ in any way. In addition, you are allowed to use spaces on the board (including start and finish) repeatedly during a particular path from start to finish. We will ensure that the total number of paths is less than or equal to 2^63-1 (the upper bound for a long). | | Definition | | Class: | ChessMetric | Method: | howMany | Parameters: | int, int[], int[], int | Returns: | long | Method signature: | long howMany(int size, int[] start, int[] end, int numMoves) | (be sure your method is public) |
| | | | Notes | - | For C++ users: long long is a 64 bit datatype and is specific to the GCC compiler. | | Constraints | - | size will be between 3 and 100 inclusive | - | start will contain exactly 2 elements | - | finish will contain exactly 2 elements | - | Each element of start and finish will be between 1 and size-1 inclusive | - | numMoves will be between 1 and 50 inclusive | - | The total number of paths will be at most 2^63-1. | | Examples | 0) | | | | Returns: 1 | Only 1 way to get to an adjacent square in 1 move |
|
| 1) | | | | Returns: 1 | A single L-shaped move is the only way |
|
| 2) | | | | Returns: 0 | Too far for a single move |
|
| 3) | | | | Returns: 5 | Must count all the ways of leaving and then returning to the corner |
|
| 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
A primary limitation of fighter plane performance is the ability of the
pilot to remain conscious. We have determined that pilots can withstand large
accelerations for short time periods, but that they cannot handle an
extended time period during which the average acceleration is high.
Create a class GForce that contains a method avgAccel that will be given the
period as an int, and int[]'s accel and time
giving a piecewise-linear
approximation to the acceleration experienced over time during a flight.
The method will return the greatest average acceleration experienced over any
interval of length equal to
period. The return value should be the average acceleration as a double.
The piecewise-linear acceleration function is given in order of increasing
times, starting with the beginning of the flight and ending at the end of the
flight. The graph of acceleration versus time is the sequence of straight-line
segments joining adjacent points (timei, acceli).
The average acceleration over an interval is the area under the graph between
the beginning and ending times, divided by the length of the interval.
| | Definition | | Class: | GForce | Method: | avgAccel | Parameters: | int, int[], int[] | Returns: | double | Method signature: | double avgAccel(int period, int[] accel, int[] time) | (be sure your method is public) |
| | | | Notes | - | The returned result must only be relatively close to the correct answer. Specifically, if the returned value either has a relative error or an absolute error less than 10^-9 it is accepted as correct. | | Constraints | - | accel contains between 2 and 50 elements inclusive | - | time contains the same number of elements as accel | - | the elements of time are strictly increasing | - | the elements of time and of accel are between 0 and 10,000 inclusive | - | period is greater than 0 and less than the difference between the first and last elements of time | | Examples | 0) | | | 100 | {1500,1500,500,2000} | {0,100,150,225} |
| Returns: 1500.0 |
accel
2000 + X
1500 + X---X /
1000 + \ /
500 + X
+-+-+-+-+-+-+-+-+-+---- time
-100 0 100 200 300
The four points determine a piecewise linear function with three segments,
whose graph is shown. The interval from 0 to 100 is the one with the
biggest average accel. In this region the average is obviously 1500.
The highest accel value is at t=225, but if we compute the area under the
curve between 125 and 225 we get 112,500 so the average on that interval is
1125 which is not as high as 1500.
|
|
| 1) | | | | Returns: 26.875 |
By symmetry the interval with the highest average must be 750 to 1250.
The area under the curve in this interval consists of two trapezoids, each
with width 250 and heights 23.75 and 30. The sum of their areas is 26.875.
|
|
| 2) | | | 11 | {0,5,5,0,6} | {0,5,15,25,31} |
| Returns: 4.984848484848485 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | The Desert. Failing water, dying camels, howling wind. The desert wind
has hands -- it throws sand in your face, pushes you down, tries to bury you.
We are stranded in the desert.
But we have a map showing where we are, where all the impassable
terrain is located, and where every oasis is located. The map is divided into
square cells, and our trip will be a sequence
of legs, where each leg goes from one cell to one of the 8 adjacent cells. We
must start at our current location and make it to an oasis, any oasis.
Of course, we cannot use any
impassable cell and cannot leave the mapped area. Our survival depends on
how many days it will take to make the journey.
That evil Desert Wind is our nemesis! Each leg takes one day unless we are
traveling directly against the wind. In that case the leg will take three
days. Fortunately, the wind's direction can be determined just before setting
out on a leg and will not change for at least a day.
Create a class DesertWind that contains the method daysNeeded that
takes a String[] theMap and returns the number of days required, assuming the worst
possible wind conditions will occur. Return -1 if no path to an oasis exists.
Each element of theMap gives the terrain for the cells (in west to east order) at
a particular latitude; the first element is the most northern, the last element
is the most southern on the map. Each cell is indicated by a single character:
- '*' is an oasis
- 'X' is impassable
- '@' is the starting location
- '-' is sand
| | Definition | | Class: | DesertWind | Method: | daysNeeded | Parameters: | String[] | Returns: | int | Method signature: | int daysNeeded(String[] theMap) | (be sure your method is public) |
| | | | Constraints | - | theMap has between 2 and 50 elements inclusive, each having the same length | - | the length of each element of theMap is between 2 and 50 inclusive | - | '@' appears exactly once in exactly one element of theMap | - | each element of theMap contains only the characters '*','-','X','@' | | Examples | 0) | | | | Returns: 2 |
- - *
@ - *
X - -
No matter how the wind is blowing we can make it to one of the two northernmost
cells in the second column in one day. Whichever cell we reach and whichever
way the wind is blowing the second day, we can get to an oasis without heading
into the wind.
|
|
| 1) | | | {"-X-*","-@X-","---X","--**"} |
| Returns: 3 |
- X - *
- @ X -
- - - X
- - * *
Unless the wind is from the southeast, we will go southeast on the first day,
and then will have two different directions that lead to an oasis -- one of
them will not be against the wind so we will reach an oasis in 2 days. But if
the wind is from the southeast on the first day, we will go south. Then, if the
wind is from the southeast on the second day we will go east (otherwise we
can go southeast to an oasis). Now we can reach one of the two oases on the
third day, no matter how the wind blows.
There is no way to reach the northeast oasis in less than 4 days if the wind
is evil. Even if the first day the wind is not from the northeast, it would
be a fatal mistake to go that direction. |
|
| 2) | | | {"*--X-----",
"--XX--@--",
"*-X------"} |
| Returns: -1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You have been working with a macro processor that has rules of the following form:
VarChar : VarOrTextChar VarOrTextChar
where VarChar is an uppercase letter,
and VarOrTextChar is an uppercase or lowercase letter
The macro processor only recognizes 2 kinds of characters, namely Variable Characters (uppercase letters) and Text Characters (lowercase letters). If it finds a Variable Character in the text that matches the left side of one of its rules it can replace it with the characters on the right side of that rule. If more than one rule has a left side that matches, the processor can arbitrarily choose which rule to use. This process continues until the processor gets rid of all the Variable Characters. For example, let's say the processor had the following rules:
rules = {"S:aA",
"A:Sb",
"S:ab",
"D:aA"}
Then given the string "S" it could do the following actions:
S => replace S with aA => aA => replace A with Sb => aSb => replace S with ab => aabb
or perhaps
S => replace S with ab => ab.
These are just two possible sequences of actions out of an infinite number of possible sequences that the processor could follow with the above rules. Our problem is, we have only been given the output and are clueless as to the initial input. Given the rules of the processor, and the output text, your method will return all of the Variable Characters that could have produced the given output, in lexicographic ('A' to 'Z') order. Using the rules in the above example, if given output "aabb", your method would return {"D","S"}. | | Definition | | Class: | Macros | Method: | whichOnes | Parameters: | String[], String | Returns: | String[] | Method signature: | String[] whichOnes(String[] rules, String output) | (be sure your method is public) |
| | | | Constraints | - | rules will contain between 1 and 50 elements, inclusive. | - | Each element of rules will have 4 characters. | - | The first character of each element of rules will be an uppercase letter ('A'-'Z'). | - | The second character of each element of rules will be a colon (':'). | - | The third and fourth characters of each element of rules will be uppercase or lowercase letters ('A'-'Z','a'-'z'). | - | output will have between 2 and 50 characters, inclusive. | - | output will contain only lowercase letters ('a'-'z'). | | Examples | 0) | | | {"S:aA",
"A:Sb",
"S:ab",
"D:aA"} | "aabb" |
| Returns: { "D", "S" } | This is the example from the problem statement. |
|
| 1) | | | {"S:aA",
"A:Sb",
"S:ab",
"D:aA"} | "ab" |
| Returns: { "S" } | The only way to produce "ab" is to start with "S" and immediately replace "S" with "ab", using the third rule. |
|
| 2) | | | {"S:aA",
"A:Sb",
"S:ab",
"D:aA"} | "aaaaabbbb" |
| Returns: { } | |
| 3) | | | {"A:aB",
"C:dE",
"Z:FG",
"B:dd"} | "qqqq" |
| Returns: { } | |
| 4) | | | {"A:aB",
"C:dE",
"Z:FG",
"B:dd"} | "add" |
| Returns: { "A" } | |
| 5) | | | {"A:BC",
"B:BC",
"C:BC",
"B:aa",
"C:bb"} | "aaaaaaaaaabbbbbbbbbb" |
| Returns: { "A", "B", "C" } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You work for a very large company that markets many different products. In some cases, one product you market competes with another. To help deal with this situation you have split the intended consumers into two groups, namely Adults and Teenagers. If your company markets 2 products that compete with each other, selling one to Adults and the other to Teenagers will help maximize profits. Given a list of the products that compete with each other, you are going to determine whether all can be marketed such that no pair of competing products are both sold to Teenagers or both sold to Adults. If such an arrangement is not feasible your method will return -1. Otherwise, it should return the number of possible ways of marketing all of the products.
The products will be given in a String[] compete whose kth element describes product k. The kth element will be a single-space delimited list of integers. These integers will refer to the products that the kth product competes with. For example:
compete = {"1 4",
"2",
"3",
"0",
""}
The example above shows product 0 competes with 1 and 4, product 1 competes with 2, product 2 competes with 3, and product 3 competes with 0. Note, competition is symmetric so product 1 competing with product 2 means product 2 competes with product 1 as well.
Ways to market:
1) 0 to Teenagers, 1 to Adults, 2 to Teenagers, 3 to Adults, and 4 to Adults
2) 0 to Adults, 1 to Teenagers, 2 to Adults, 3 to Teenagers, and 4 to Teenagers
Your method would return 2.
| | Definition | | Class: | Marketing | Method: | howMany | Parameters: | String[] | Returns: | long | Method signature: | long howMany(String[] compete) | (be sure your method is public) |
| | | | Constraints | - | compete will contain between 1 and 30 elements, inclusive. | - | Each element of compete will have between 0 and 50 characters, inclusive. | - | Each element of compete will be a single space delimited sequence of integers such that: - All of the integers are unique.
- Each integer contains no extra leading zeros.
- Each integer is between 0 and k-1 inclusive where k is the number of elements in compete.
| - | No element of compete contains leading or trailing whitespace. | - | Element i of compete will not contain the value i. | - | If i occurs in the jth element of compete, j will not occur in the ith element of compete. | | Examples | 0) | | | | 1) | | | | Returns: -1 | Product 0 cannot be marketed with product 1 or 2. Product 1 cannot be marketed with product 2. There is no way to achieve a viable marketing scheme. |
|
| 2) | | | {"1","2","3","0","0 5","1"} |
| Returns: 2 | |
| 3) | | | {"","","","","","","","","","",
"","","","","","","","","","",
"","","","","","","","","",""} |
| Returns: 1073741824 | |
| 4) | | | {"1","2","3","0","5","6","4"} |
| Returns: -1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | It is a common practice in cryptography to remove the spaces from a message
before encoding it to help to disguise its structure.
Even after it is then decoded, we are left with the
problem of putting the spaces back in the message.
Create a class MessageMess that contains a method restore that
takes a String[] dictionary of possible words and
a String message as inputs. It returns the message with single spaces inserted to divide the message into words from the dictionary. If there is more than one way to insert spaces, it returns "AMBIGUOUS!" If there is no way to insert spaces, it returns "IMPOSSIBLE!" The return should never
have any leading or trailing spaces.
| | Definition | | Class: | MessageMess | Method: | restore | Parameters: | String[], String | Returns: | String | Method signature: | String restore(String[] dictionary, String message) | (be sure your method is public) |
| | | | Notes | - | Don't forget the '!' at the end of the two special returns | - | A proper message may require 0 spaces to be inserted | | Constraints | - | dictionary will contain between 1 and 50 elements inclusive | - | the elements of dictionary will be distinct | - | each element of dictionary will contain between 1 and 50 characters | - | message will contain between 1 and 50 characters | - | every character in message and in each element of dictionary will be an uppercase letter 'A'-'Z' | | Examples | 0) | | | {"HI", "YOU", "SAY"} | "HIYOUSAYHI" |
| Returns: "HI YOU SAY HI" |
A word from dictionary may appear multiple times in the message.
|
|
| 1) | | | {"ABC", "BCD", "CD", "ABCB"} | "ABCBCD" |
| Returns: "AMBIGUOUS!" | "ABC BCD" and "ABCB CD" are both possible interpretations of message. |
|
| 2) | | | {"IMPOSS", "SIBLE", "S"} | "IMPOSSIBLE" |
| Returns: "IMPOSSIBLE!" | There is no way to concatenate words from this dictionary
to form "IMPOSSIBLE" |
|
| 3) | | | {"IMPOSS", "SIBLE", "S", "IMPOSSIBLE"} | "IMPOSSIBLE" |
| Returns: "IMPOSSIBLE" | This message can be decoded without ambiguity. This requires the insertion of no spaces since the entire message appears as a word in the dictionary. |
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Skew heaps are an excellent implementation of priority queues. A skew heap is a binary tree like a binary search tree, but with a different ordering of its elements. In a skew heap, the elements are heap-ordered, meaning that every child is larger
than its parent. A left sibling is not required to be smaller than its right sibling, and skew heaps are not required to be
balanced. The heap-ordering property implies that the minimum element in a skew heap is always the root.
Inserting a new element X into a skew heap H is done recursively. The base case applies when H is empty
or when X is smaller than the existing root. The new element becomes the new root, with the old root as its left child.
The recursive case applies when X is larger than the existing root. The left and right subtrees of the existing root are swapped, and X is inserted recursively into the left subtree (which was the right subtree before the swap). These two cases are illustrated below.
BASE CASE (H is empty or X < root of H)
---------------------------------------
Insert(X, H) ===> X
/
H
RECURSIVE CASE (X > Y)
---------------------------------------
Insert(X, Y ) ===> Y
/ \ / \
A B Insert(X,B) A
The following snapshots show the results of sequentially inserting the numbers 0,1,2,3,4,5,6
into an initially empty tree (where all children not shown are assumed to be null):
0 0 0 0 0 0 0
/ / \ / \ / \ / \ / \
1 2 1 1 2 2 1 / \ / \
/ / / 1 2 2 1
3 4 3 / \ / / \ / \
5 3 4 6 4 5 3
Notice how, because of the swaps, new elements are distributed evenly between the two sides of the tree. In this case, the odd elements
(1,3,5) are placed on one side of the tree, and the non-zero even elements (2,4,6) are placed on the other side of the tree.
On the other hand, it is also possible to get an unbalanced tree if the elements are not inserted in sorted order.
For example, sequentially inserting the numbers 6,4,5,2,0,1,3 into an initially empty tree yields
6 4 4 2 0 0 0
/ / \ / / / \ / \
6 5 6 4 2 1 2 2 1
/ \ / / / \
5 6 4 4 3 4
/ \ / \ / \
5 6 5 6 5 6
Notice how elements inserted after the overall minimum element are distributed evenly between the left and right
subtrees of the root (e.g., 1 and 3 in the last tree above). In contrast, elements inserted before the overall minimum
element end up in the same subtree of the root (e.g., 6, 4, 5, and 2 above).
Your task is to write a method that will take a skew heap and return the sequence of insertions that might have created that heap.
If more than one sequence of insertions might have created the final skew heap, return the lexicographically earliest. A sequence
A is lexicographically earlier than a sequence B if the element of A at the leftmost position at which the sequences differ is smaller than the element of B at the same position.
The input heap contains the values 0 through n-1, inclusive, where n is the size of the heap. Each value appears exactly
once in the heap. The shape of the input tree will be represented by a int[], parent, of size n-1.
If the node containing i is a left child, then element i-1 of parent is the value of the node's parent.
If the node containing i is a right child, then element i-1 of parent is 100 plus the value of the parent.
Note that parent is 0-indexed.
The node containing 0 is always the root, so its parent is not represented. For example, the skew heap
0
/ \
1 2
/ / \
5 4 3
would be represented by parent = {0,100,102,2,1}, meaning that
- 1 is the left child of 0.
- 2 is the right child of 0.
- 3 is the right child of 2.
- 4 is the left child of 2.
- 5 is the left child of 1.
No skew heap built entirely by inserts will ever contain a node with an empty left subtree and a non-empty right subtree.
Such heaps will not be permitted as input.
| | Definition | | Class: | SkewHeaps | Method: | history | Parameters: | int[] | Returns: | int[] | Method signature: | int[] history(int[] parent) | (be sure your method is public) |
| | | | Constraints | - | parent contains between 1 and 50 elements, inclusive. | - | Element i of parent is either between 0 and i, inclusive, or between 100 and 100+i, inclusive. | - | No value appears more than once in parent. | - | If the value 100+k appears in parent, for some non-negative k, then the value k must also appear in parent. | | Examples | 0) | | | { 100, 0, 101, 102, 1, 2 } |
| Returns: { 0, 1, 2, 3, 4, 5, 6 } | The tree
0
/ \
/ \
2 1
/ \ / \
6 4 5 3
|
|
| 1) | | | { 100, 0, 2, 102, 4, 104 } |
| Returns: { 4, 6, 5, 2, 0, 1, 3 } | The tree
0
/ \
2 1
/ \
3 4
/ \
5 6
Either {4,6,5,2,0,1,3} or {6,4,5,2,0,1,3} could have created this tree, so we return the lexicographically smallest. |
|
| 2) | | | { 0, 100, 1, 102, 2, 3, 5 } |
| Returns: { 2, 5, 0, 3, 4, 6, 7, 1 } | The tree
0
/ \
1 2
/ / \
3 5 4
/ /
6 7
There are eight sequences that could have produced this tree:
{2,5,0,3,4,6,7,1}
{2,5,0,6,4,3,7,1}
{2,7,0,3,4,6,5,1}
{2,7,0,6,4,3,5,1}
{5,2,0,3,4,6,7,1}
{5,2,0,6,4,3,7,1}
{7,2,0,3,4,6,5,1}
{7,2,0,6,4,3,5,1}
We choose the lexicographically smallest. |
|
| 3) | | | | 4) | | | { 100, 1, 101, 103, 3, 4, 6, 107, 7, 0, 10, 11, 110, 12, 112, 111, 8, 108, 2, 16 } |
| Returns:
{ 8, 18, 17, 7, 9, 0, 11, 6, 12, 4, 16, 3, 15, 1, 20, 2, 10, 5, 13, 19, 14 } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are playing a video game that involves escaping from a dangerous area. Within the area there are DEADLY regions you can't enter, HARMFUL regions that take 1 life for every step you make in them, and NORMAL regions that don't affect you in any way. You will start from (0,0) and have to make it to (500,500) using only Up, Left, Right, and Down steps. The map will be given as a String[] deadly listing the DEADLY regions and a String[] harmful listing the HARMFUL regions. The elements in each of these parameters will be formatted as follows:
Input format(quotes for clarity): "X1 Y1 X2 Y2" where
(X1,Y1) is one corner of the region and
(X2,Y2) is the other corner of the region
The corners of the region are inclusive bounds (i.e. (4,1) and (2,2) include x-values between 4 and 2 inclusive and y-values between 1 and 2 inclusive). All unspecified regions are considered NORMAL. If regions overlap for a particular square, then whichever region is worst takes effect (e.g. DEADLY+HARMFUL = DEADLY, HARMFUL+NORMAL = HARMFUL, HARMFUL+HARMFUL = HARMFUL, DEADLY+NORMAL=DEADLY). Damage taken at each step occurs based on the destination square and not on the starting square (e.g. if the square (500,500) is HARMFUL you WILL take a point of damage stepping onto it; if the square (0,0) is HARMFUL you WON'T take a point of damage stepping off of it; this works analogously for DEADLY squares).
Return the least amount of life you will have to lose in order to reach the destination. Return -1 if there is no path to the destination. Your character is not allowed to leave the map (i.e. have X or Y less than 0 or greater than 500).
| | Definition | | Class: | Escape | Method: | lowest | Parameters: | String[], String[] | Returns: | int | Method signature: | int lowest(String[] harmful, String[] deadly) | (be sure your method is public) |
| | | | Notes | - | If two harmful regions overlap, the area where they overlap is exactly the same as non-overlapping harmful regions (i.e. the effect is NOT cumulative, and the overlapping region still takes exactly 1 life) | | Constraints | - | deadly will contain between 0 and 50 elements inclusive | - | harmful will contain between 0 and 50 elements inclusive | - | Each element of deadly and harmful will be of the form (quotes for clarity): "X1 Y1 X2 Y2" where X1,Y1,X2, and Y2 are integers between 0 and 500 inclusive and contain no leading zeros | - | Each element of deadly and harfmul will contain no leading, trailing or extra whitespace | | Examples | 0) | | | | Returns: 0 | There are no DEADLY or HARMFUL regions. |
|
| 1) | | | {"500 0 0 500"} | {"0 0 0 0"} |
| Returns: 1000 | (0,0) is DEADLY but that doesn't affect our path since we never step onto it (only from it). The rest of the map is NORMAL. |
|
| 2) | | | {"0 0 250 250","250 250 500 500"} | {"0 251 249 500","251 0 500 249"} |
| Returns: 1000 | Just enough space to get around the DEADLY regions. |
|
| 3) | | | {"0 0 250 250","250 250 500 500"} | {"0 250 250 500","250 0 500 250"} |
| Returns: -1 | No way around the DEADLY regions. |
|
| 4) | | | {"468 209 456 32",
"71 260 306 427",
"420 90 424 492",
"374 253 54 253",
"319 334 152 431",
"38 93 204 84",
"246 0 434 263",
"12 18 118 461",
"215 462 44 317",
"447 214 28 475",
"3 89 38 125",
"157 108 138 264",
"363 17 333 387",
"457 362 396 324",
"95 27 374 175",
"381 196 265 302",
"105 255 253 134",
"0 308 453 55",
"169 28 313 498",
"103 247 165 376",
"264 287 363 407",
"185 255 110 415",
"475 126 293 112",
"285 200 66 484",
"60 178 461 301",
"347 352 470 479",
"433 130 383 370",
"405 378 117 377",
"403 324 369 133",
"12 63 174 309",
"181 0 356 56",
"473 380 315 378"} | {"250 384 355 234",
"28 155 470 4",
"333 405 12 456",
"329 221 239 215",
"334 20 429 338",
"85 42 188 388",
"219 187 12 111",
"467 453 358 133",
"472 172 257 288",
"412 246 431 86",
"335 22 448 47",
"150 14 149 11",
"224 136 466 328",
"369 209 184 262",
"274 488 425 195",
"55 82 279 253",
"153 201 65 228",
"208 230 132 223",
"369 305 397 267",
"200 145 98 198",
"422 67 252 479",
"231 252 401 190",
"312 20 0 350",
"406 72 207 294",
"488 329 338 326",
"117 264 497 447",
"491 341 139 438",
"40 413 329 290",
"148 245 53 386",
"147 70 186 131",
"300 407 71 183",
"300 186 251 198",
"178 67 487 77",
"98 158 55 433",
"167 231 253 90",
"268 406 81 271",
"312 161 387 153",
"33 442 25 412",
"56 69 177 428",
"5 92 61 247"} |
| Returns: 254 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You will be given a String[] representing a directed graph. Each element will be formatted as follows:
Node1 Node2 Node3 ...
where NodeJ is a number (0-indexed) representing a node. Element K of the String[] graph will list all of the nodes that node K has a directed edge to. For example:
graph = {"1 2 3",
"0 1 2",
"0",
"1 2"}
Represents a directed graph where:
node 0 has a directed edge to nodes 1, 2, and 3,
node 1 has a directed edge to nodes 0, 1 and 2,
node 2 has a directed edge to node 0, and
node 3 has a directed edge to nodes 1 and 2.
Given a String[] formatted as above representing the directed graph, an int representing the starting node, an int representing the destination node, and an int representing the path length, determine how many unique paths exist from the starting node to the destination node of the specified length. Note, the paths may contain cycles. The length of a path is how many edges it traverses, possibly counting an edge more than once if the path contains a cycle.
If the total number of paths is greater than 2^63-1 (the limit of a long), return -1
| | Definition | | Class: | GraphPaths | Method: | howMany | Parameters: | String[], int, int, int | Returns: | long | Method signature: | long howMany(String[] graph, int start, int destination, int length) | (be sure your method is public) |
| | | | Notes | - | Two paths are unique if they differ at any node. For example: A->B->C->B is unique from A->C->B->B which are both unique from A->B->B->C. | | Constraints | - | graph will contain between 1 and 50 elements inclusive | - | Each element of graph will have length between 1 and 50 inclusive | - | Each element of graph will be a single-space delimited string of zero or more integers with no leading zeros | - | Each element of graph will contain no leading or trailing whitespace | - | Each integer in each element of graph will be between 0 and (size of graph - 1) inclusive where size of graph is the number of elements in graph | - | Each element of graph will not contain any repeated integers | - | start will be between 0 and (size of graph - 1) inclusive | - | destination will be between 0 and (size of graph - 1) inclusive | - | length will be between 1 and 1,000,000,000 inclusive | | Examples | 0) | | | {"1 2 3",
"0 1 2",
"0",
"1 2"} | 0 | 1 | 2 |
| Returns: 2 | |
| 1) | | | {
"1",
"2",
"3",
"4",
"5",
"0 3"
} | 0 | 0 | 10000001 |
| Returns: 0 | Note that all paths from 0 to 0 have length divisible by 3. |
|
| 2) | | | | Returns: -1 | The actual answer to this is 2^63, but that is outside of the range for a long, so we return -1. |
|
| 3) | | | {"0 1 2 3", "0 1 2 3", "0 1 2 3", "0 1 2 3"} | 2 | 2 | 63 |
| Returns: -1 | |
| 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A permutation of the letters in an alphabet can be described by a string that contains
each letter exactly once. For example, CABD describes the permutation of ABCD that maps
A to C, B to A, C to B, and D to D. If we repeatedly
apply that permutation, we will get the sequence ABCD,CABD,BCAD,ABCD,CABD,BCAD,ABCD,... This
permutation is cyclic with length 3.
We want to find the permutation of the first n letters of
ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz
that has the longest cycle. Create
a class Permutation that contains the method best that takes the number of characters n
as input and returns the lexicographically first permutation that has the maximum
possible cycle length.
"Lexicographically first" means the String that would sort first among all the permutations of maximum cycle length, using the ASCII sequence (which sorts uppercase letters before lowercase letters).
| | Definition | | Class: | Permutation | Method: | best | Parameters: | int | Returns: | String | Method signature: | String best(int n) | (be sure your method is public) |
| | | | Constraints | - | n is between 1 and 52 inclusive | | Examples | 0) | | | | Returns: "ACBEFD" | This permutation has cycle length 6. So does BDEFCA, but ACBEFD is lexicographically first. The cycle is: ABCDEF -> ACBEFD -> ABCFDE -> ACBDEF -> ABCEFD -> ACBFDE -> ABCDEF |
|
| 1) | | | | Returns: "BCAEFGD" | This is the lexicographically first permutation with cycle length 12 |
|
| 2) | | | | Returns: "BCDEAGHIJKLFNOPQRSTMVWXYZabcU" | |
| 3) | | | | 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
We all know that there are not necessarily three dimensions, and that there are wormholes scattered throughout the universe which will transport you through time and space. However, because it is often difficult for people to conceptualize higher dimensions, we will probably have to have computers do most of our navigation for us. Your task is to write a program that will simulate a pseudo-random asteroid field, and then navigate a ship through the asteroid field as quickly as possible.
The first step will be to generate the asteroid field. You will be given a int[], dim, that will specify how large you should make each dimension in your asteroid field. For example, if dim is {3,4,5}, the generated asteroid field will be a 3 x 4 x 5 box, with corners at (0,0,0) and (2,3,4). If dim is {3,4,5,4}, the generated asteroid field will be a 3 x 4 x 5 x 4 hyper-box (a hyper box is analogous to a regular box, but in more than three dimensions). Then, you must determine which spaces in the asteroid field actually contain asteroids. This will be done pseudo-randomly using something like the following code, where a and p are inputs (remember that there are a variable number of dimensions):
int current = 1;
for(int i0 = 0; i0<dim0; i0++)
for(int i1 = 0; i1<dim1; i1++)
... one loop per dimension
{
current = (current*a)%p;
if((current&1)==1){
//add an asteroid at (i0,i1,i2,...)
}
}
Once the asteroid field is generated, you have to navigate through it. You will be given a int[], start, and a int[], finish. You must find your way through the asteroid field, as quickly as possible without running into an asteroid. You may move from any location (a0,a1,a2...) to any other location, (b0,b1,b2...) so long as for all i, the differences between ai and bi is either 1 or 0, and bi is between 0 and dimi-1, inclusive. Each such movement takes one unit of time. For example, if you were at the S below, you could move to any of the locations marked with T, where '.' represents open space.
.....
.TTT.
.TST.
.TTT.
.....
In addition to this type of movement, you may also (but are not required to) travel through wormholes. You will be given a String[], wormholes, which specifies the origin, length of time travel, and destination of some wormholes. To go through a wormhole, you must first reach the origin of the wormhole. Then, after going through the wormhole, you will be transported to the destination of the wormhole. Additionally, wormholes transport you in time. So, each wormhole has a fixed amount of time travel associated with it, and each time you go through the wormhole, you will go forward or backward in time by that amount. Each element of wormholes will be formatted as "<origin> <time> <destination>", where <origin> and <destination> and coordinates as a space delimited list. For example, "0 0 -1 3 7" would represent a wormhole that starts at (0,0), transports you back in time 1 unit, and leaves you at (3,7) in space. Thus, if you reached (0,0) 5 units of time after you started, it would be possible to reach (3,7) from there 4 units of time after you started.
Sometimes, it will be impossible to reach finish from start. In this case return, 2^31-1 = 2147483647.
| | Definition | | Class: | HigherMaze | Method: | navigate | Parameters: | int, int, int[], int[], int[], String[] | Returns: | int | Method signature: | int navigate(int a, int p, int[] dim, int[] start, int[] finish, String[] wormholes) | (be sure your method is public) |
| | | | Constraints | - | The product of all the elements of dim will be between 2 and 1000, inclusive. | - | Each element of dim will be between 2 and 20, inclusive. | - | dim will contain between 1 and 5 elements, inclusive. | - | a and p will be between 1 and 2,000,000,000, inclusive. | - | a times p will be between 1 and 2,000,000,000, inclusive. | - | start and finish will be within the hyper-box defined by dim. | - | start and finish will be both be empty space (not an asteroid, possibly a wormhole). | - | wormholes will contain between 0 and 50 elements, inclusive. | - | Each element of wormholes will be formatted as "<origin> <time> <destination>". | - | <origin> and <destination> will both be space delimited (no extra, leading or trailing spaces) lists of integers, representing a coordinate in space within the hyper-box defined by dim. | - | <origin> and <destination> will both be empty space (not an asteroid). | - | <time> will be an integer in the range -1,000,000 to 1,000,000. | - | There will be no way to go back in time infinitely. (In other words, there will be no reachable cycles that take negative amounts of time. However, there may be unreachable ones, but they can not affect the return, since they are unreachable.) | | Examples | 0) | | | | Returns: 6 | This input generates the following two dimensional asteroid field, where an 'X' is an asteroid, and a '.' is open space. (Here, (0,0) is the upper left corner, and (4,4) is the lower right, and (4,0) is in the lower left):
...XX
XX.X.
..XXX
....X
.XXX.
Here is one path that takes 6 time units, where 'S' represents the start, 'F' the finish, and '*' the path.
S*.XX
XX*X.
.*XXX
..**X
.XXXF
|
|
| 1) | | | 138 | 193 | {5,5} | {0,0} | {4,4} | {"0 0 2 4 4"} |
| Returns: 2 | While there is still a path from (0,0) to (4,4) which takes 6 time units, there is also a wormhole, which transports you directly from (0,0) to (4,4), and ahead 2 units of time. |
|
| 2) | | | 138 | 193 | {5,5} | {0,0} | {4,4} | {"0 0 2 4 4","0 0 8 1 4","0 2 5 1 4","1 4 -8 4 4"} |
| Returns: -1 | This is the same asteroid configuration as the previous two examples, but now there are more wormholes. The quickest way to get from (0,0) to (4,4) is to move from from (0,0) to (0,2), which takes 2 units of time. From (0,2) we should go through the wormhole to (1,4), which transports us ahead 5 units of time, for a total of 7. Then, we can take the wormhole from (1,4) to (4,4), which takes us back 8 units of time. Thus, the total time for the trip is 2 + 5 - 8 = -1, and we arrive before we left! (note that two wormholes can start at the same location, and could even start and end at the same location) |
|
| 3) | | | 54 | 73 | {20,20} | {1,2} | {12,12} | {} |
| Returns: 23 | |
| 4) | | | 139 | 193 | {20,20} | {0,2} | {18,19} | {"0 2 -100000 0 19","4 18 -100000 7 0","8 0 1000000 19 10","19 6 -800000 13 8"} |
| Returns: 21 | The only way to get all the way is to go through all 4 wormholes, in order. |
|
| 5) | | | 138 | 193 | {5,5} | {0,0} | {4,0} | {"0 0 -6 4 4"} |
| Returns: -2 | |
| 6) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | In a wireless network, the distance between nodes determines connectivity and latency. The further apart two nodes are the slower the connection. If two nodes are further apart than the maximum allowable range they cannot directly communicate. In our network, there will be 2 kinds of nodes: stationary and roaming. When a roaming node wants to communicate with another roaming node it must first communicate with one of the stationary nodes in its range. The stationary node it connects to will then send the transmission through as many stationary nodes as are necessary before finally sending to the destination roaming node. In other words, the transmission always starts at a roaming node, goes through one or more stationary nodes, and finally arrives at the destination roaming node. For example:
R = roaming, S = stationary
.......--R......
....../.........
.....S--........
........\.......
.........S......
.........|......
..R--.../.......
.....\-S........
Above is one possible route between the two depicted roaming nodes.
In this problem you will be given the initial positions of the roaming nodes and their initial velocities. Each roaming node will either be moving upward, leftward, rightward, or downward at the rate of one square per second. For example:
roamNodes Input Format: Direction X Y
roamNodes = {"UP 9 0","DOWN 2 6"}
statNodes Input Format: X Y
statNodes = {"5 2","9 4","7 7"}
Time 0 Time 1 Time 2
0123456789012345 0123456789012345 0123456789012345
0.......--R...... 0................ 0................
1....../......... 1....../--R...... 1................
2.....S--........ 2.....S.......... 2.....S...R......
3........\....... 3..../........... 3.........|......
4.........S...... 4.../.....S...... 4..R------S......
5.........|...... 5..R............. 5................
6..R--.../....... 6................ 6................
7.....\-S........ 7.......S........ 7.......S........
Above are the positions of the nodes, and some possible routes.
We are going to assume that the routing protocol will always choose the shortest possible end-to-end (roaming node-to-roaming node) route when connecting two roaming nodes. You will be given the wireless range of all nodes, the positions and velocities of the roaming nodes, and the positions of the stationary nodes. You will determine the length of the shortest route that will ever occur between the two roaming nodes in the system at any non-negative integral time. The distance between two nodes is always measured using the cartesian distance formula: sqrt((x2-x1)^2 + (y2-y1)^2). The length of a route is the sum of the distances when travelling from node to node along the route. If two nodes share the same location, their distance is 0. Two nodes (Roaming to Static or Static to Static) can communicate if their distance is less than or equal to the given maximum range. Round final answers to the nearest integer. If the two roaming nodes could never communicate return -1. See examples for further clarification.
Create a class Wireless that contains the method bestRoute, which takes an int range, a String[] roamNodes, and a String[] statNodes and returns an int representing the length of the shortest route between the two roaming nodes in the system at any integral time greater than or equal to 0. If the two nodes could never communicate return -1.
| | Definition | | Class: | Wireless | Method: | bestRoute | Parameters: | int, String[], String[] | Returns: | int | Method signature: | int bestRoute(int range, String[] roamNodes, String[] statNodes) | (be sure your method is public) |
| | | | Notes | - | Two nodes (Roaming to Static or Static to Static) can communicate if their distance if less than or equal to the given maximum range. | - | Final answers must be rounded to the nearest integer (i.e. .5 and greater rounds up, below .5 rounds down) | - | All routes must start at a roaming node, pass through 1 or more static nodes, and complete at a roaming node. | - | UP means INCREASING Y coordinate whereas DOWN means DECREASING Y coordinate | - | RIGHT measn INCREASING X coordinate whereas LEFT means DECREASING X coordinate | | Constraints | - | range will be between 1 and 30000 inclusive | - | roamNodes will contain exactly 2 elements | - | Each element of roamNodes will be of the form: Direction X Y where Direction is one of UP, DOWN, LEFT, or RIGHT X is an integer with no leading zeros between -10000 and 10000 inclusive Y is an integer with no leading zeros between -10000 and 10000 inclusive | - | statNodes will contain between 1 and 30 elements inclusive | - | Each element of statNodes will be of the form: X Y where X is an integer with no leading zeros between -10000 and 10000 inclusive Y is an integer with no leading zeros between -10000 and 10000 inclusive | - | The final answer will be within .4999 of the nearest integer. | | Examples | 0) | | | 1 | {"DOWN 100 200","DOWN 100 200"} | {"1000 1000"} |
| Returns: -1 | The roaming nodes will never be close enough to the static node to communicate. |
|
| 1) | | | 30000 | {"DOWN 10000 10000","RIGHT -10000 -10000"} | {"10000 -10000","10000 -10000"} |
| Returns: 0 | They keep getting closer and closer until finally they all meet at the stationary node at (10000,-10000) |
|
| 2) | | | 3000 | {"DOWN 0 10000","LEFT 10000 0"} | {"14 100","25 -10","98 204","99 1000"} |
| Returns: 49 | |
| 3) | | | 30000 | {"DOWN 0 0","DOWN 0 0"} | {"10000 10000","9000 10000","8000 10000","7000 10000"} |
| Returns: 24413 | |
| 4) | | | 20 | {"DOWN -20 0","DOWN 80 1"} | {"0 0","20 0","40 0","60 1"} |
| Returns: -1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Given a board consisting of empty space, walls, and the starting positions of two players A and B, determine the minimum number of turns it will take for players A and B to switch positions on the board.
During a turn, one or both players may take a step. A step is defined as a unit movement up, down, left, right, or in any of the four diagonals. Players may not step into walls or off the board. Players may never share the same square at the end of a turn. Players may not cross paths during a turn. Crossing paths occurs when players A and B switch positions in a single turn. For example, assume player A is in the upper left corner of the board, and player B is in the square immediately to his right. Player A may not move right while player B moves left, since they would be passing each other. Player A can, however, move right if player B moves in any other direction.
You will be given a String[] board, representing the game board. board will contain exactly one 'A' and exactly one 'B'; each other character will be either '.' (empty space), or 'X' (a wall). Your method should return the minimum number of turns necessary for players A and B to switch positions, or -1 if this is impossible. | | Definition | | Class: | PathFinding | Method: | minTurns | Parameters: | String[] | Returns: | int | Method signature: | int minTurns(String[] board) | (be sure your method is public) |
| | | | Notes | - | (In the following notes, assume that the coordinate system is given as (row, col). For example, the upper-left corner of the board is (0,0), and the square immedately below it is (1,0).) | - | If player A is at (0,0) and player B is at (0,1), player A would be allowed to move down-right at the same time that player B moves down-left. This is not considered crossing paths, even though the two players would meet at (0.5, 0.5). | - | However, if player A is at (0,0) and player B is at (1,1), player A would not be allowed to move down-right at the same time that player B moves up-left. This is considered crossing paths because players A and B have switched positions at the end of a single turn. | - | It is permissible to move diagonally through walls. That is, a player may move from (0,0) to (1,1) even if there are walls at (0,1) and (1,0). | | Constraints | - | board will contain between 2 and 20 elements, inclusive. | - | Each element of board will contain between 2 and 20 characters, inclusive. | - | Each element of board will contain the same number of characters. | - | Each element of board will consist of only the characters '.', 'X'. 'A', and 'B'. | - | board will contain exactly one 'A' and exactly one 'B'. | | Examples | 0) | | | {"....",
".A..",
"..B.",
"...."} |
| Returns: 2 | There are many ways to switch positions in two turns. For example, on turn one, player A could move right while player B moves up-right. Then, on turn two, player A could move down while player B stays where he is. It is illegal for the players to switch positions in a single turn. Therefore, the method returns 2. |
|
| 1) | | | {"XXXXXXXXX",
"A...X...B",
"XXXXXXXXX"} |
| Returns: -1 | Since the players cannot reach each other, they obviously cannot switch positions. |
|
| 2) | | | {"XXXXXXXXX",
"A.......B",
"XXXXXXXXX"} |
| Returns: -1 | Even though the players can reach each other, there is still no way for player B to ever get on the left side of player A. |
|
| 3) | | | {"XXXXXXXXX",
"A.......B",
"XXXX.XXXX"} |
| Returns: 8 | Players A and B spend the first three turns moving towards each other. On turn four, player A moves down-right while player B moves left. On turn five, player A moves up-right while player B moves left. It then takes three more turns of the players moving away from each other before they have switched positions, for a total of 8 turns. |
|
| 4) | | | {"...A.XXXXX.....",
".....XXXXX.....",
"...............",
".....XXXXX.B...",
".....XXXXX....."} |
| Returns: 13 | |
| 5) | | | {"AB.................X",
"XXXXXXXXXXXXXXXXXXX.",
"X..................X",
".XXXXXXXXXXXXXXXXXXX",
"X..................X",
"XXXXXXXXXXXXXXXXXXX.",
"X..................X",
".XXXXXXXXXXXXXXXXXXX",
"X..................X",
"XXXXXXXXXXXXXXXXXXX.",
"X..................X",
".XXXXXXXXXXXXXXXXXXX",
"X..................X",
"XXXXXXXXXXXXXXXXXXX.",
"X..................X",
".XXXXXXXXXXXXXXXXXXX",
"X..................X",
"XXXXXXXXXXXXXXXXXXX.",
"...................X",
".XXXXXXXXXXXXXXXXXXX"} |
| Returns: 379 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are trying to find a way around a maze which consists of a number of rooms, each of which may have doors open to other rooms that are only open at specific time periods. You will start in a certain room, at time = 0. At each time step, a set of doors will be open connecting various rooms to each other. However, the doors are all one-way, and allow passage in only one direction (though there may be two one-way doors between a pair of rooms so that you can go in either direction). Additionally, you have decided to move through a door every time step, so unless there is a door which connects a room to itself (which is possible) you must move to a new room at each time step. Only paths which move through a door at each time step are valid (see example 4).
At each time step, there will be a set of open doors which is represented by a single uppercase letter ('A'-'Z').
The maze will be represented by the input rooms, where each element of rooms tells you which doors leading out of a room are associated with which letters. Each element will be formatted as "<letter>:<adjacent room 1>,<adjacent room 2>,... <letter>:<adjacent room 1>,...". In other words, element i of rooms will be a space delimited list of the rooms that are reachable from room i via doors associated with a certain letter. Thus if
rooms = {"A:1 B:0,1","A:0"}
This means that for the letter 'A', you can get from room 1 to room 0, and from room 0 to room 1. For the letter 'B' you can get from room 0 to room 0 or from room 0 to room 1.
The second input, doors, represents which sets of doors are open at which time steps. So, if doors were "AB", then doors associated with the letter 'A' would be open at time step 0, and doors associated with 'B' would be open at time step 1. Thus, for the above example of rooms, if you started at room 1, there would be 2 possible paths with the same length as doors. You could go from room 1 to room 0 and then to room 0 again (signified "00"), or you could take the path "01".
The starting room will be denoted by the input start, which indicates that you should start in the room represented by the element start of rooms (indexed from 0). Your method should return a int[] representing which rooms can possibly be reached (at the final time step) by going through some door at each time step, and not skipping any time steps. The return should be sorted in ascending order. | | Definition | | Class: | Rooms | Method: | finalRooms | Parameters: | String[], String, int | Returns: | int[] | Method signature: | int[] finalRooms(String[] rooms, String doors, int start) | (be sure your method is public) |
| | | | Notes | - | If an element of rooms does not contain some letter, this indicates that there are no doors coming out of this room associated with that letter. See example 4. | | Constraints | - | Each element of rooms will be formatted as "<letter>:<adjacent room 1>,<adjacent room 2>,... <letter>:<adjacent room 1>,...". For each <letter> present in each element, there will always be at least one adjacent room associated with it. | - | No <letter> will be repeated in any element of rooms. | - | Each <letter> will be an uppercase letter ('A'-'Z'). | - | Each <adjacent room i> will be an integer between 0 and the number of elements in rooms-1, inclusive, with no extra leading zeros. And, for each letter, no room will be repeated. | - | rooms will contain between 1 and 50 elements, inclusive. | - | Each element of rooms will contain between 1 and 50 characters, inclusive. | - | doors will contain between 1 and 50 uppercase letters ('A'-'Z'), inclusive. | - | start will be between 0 and the number of elements in rooms-1, inclusive. | | Examples | 0) | | | {"A:0 B:1","A:1 B:0"} | "AB" | 0 |
| Returns: { 1 } | We start in room 0. During the first time step, the 'A' set of doors is open, so we can go from room 0 to room 0, since the first element of rooms has "A:0". That is our only option, so we go through the door to room 0. At time step 1, the 'B' set of doors is open, and the first element of rooms tells us that we can only go from room 0 to room 1 when the 'B' set of doors is open. So, after two time steps, the only option is to be in room 1. |
|
| 1) | | | {"A:1 B:0","A:0 B:1"} | "AABAAB" | 1 |
| Returns: { 1 } | |
| 2) | | | {"B:1 Z:0","B:0 Z:2","B:2 Z:1"} | "BBZZB" | 0 |
| Returns: { 1 } | |
| 3) | | | {"A:0,1,2,3","A:0,1,2,3","A:0,1,2,3","A:0,1,2,3"} | "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA" | 2 |
| Returns: { 0, 1, 2, 3 } | Here we can get from any room to any other room when the 'A' set of doors is open. |
|
| 4) | | | {"D:0","D:0","D:0","D:0"} | "GDDDDD" | 3 |
| Returns: { } | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
At a recent party, a number of people were loitering around talking to one
another, and generally doing partyish things. It was a very social event, and we
would like to come up with some sort of a metric for how social it was. One
possibility is to find the shortest distance from each person to
every other person, and take the average. Your task is to write a class
Socialize, with a method average, that computes the average distance between
all distinct pairs of people, and returns this average, rounded to the nearest integer (.5 rounds up).
However, our metric would not be very accurate if we just took the distance between people. For example, two people could have been only a few feet from each other, but if there was a wall between them, they wouldn't have been able to socialize.
Thus, we will define the distance between two people as the shortest path between
them, which doesn't go through any obstacles, and where the path goes in
discrete steps, with each step being one unit in any of the four cardinal
directions (east, west, north and south).
Because there are various obstacles, there may be cases where two people are
totally cut off from each other. In this case, you should not include the
distance between them in your calculation.
For example, if the layout were ('P' represents a person, '#' represents some
sort of an obstacle, and '.' represents open floor):
P...P
###..
P...#
####P
There are four people in total, one at each of the following locations (where
the first coordinate is the distance along the x axis, and the second is along
the y axis, with (0,0) at the upper left corner): (0,0),(4,0),(0,2),(4,3)
The person at (0,0) and the person at (4,0) are connected by a path of length 4.
The person at (0,0) and the person at (0,2) are connected by a path of length 8 because to get from one to another, they have to walk around the obstacle between them.
The person at (4,0) and the person at (0,2) are connected by a path of length 6.
The person at (4,3) can not reach any of the other people because he is blocked in (no diagonal movement), thus distances from him to other people do not play a role in the average.
Thus, there are three paths between people, whose lengths are 4,6, and 8. The
average length of these paths is 6, so your method should return 6.
| | Definition | | Class: | Socialize | Method: | average | Parameters: | String[] | Returns: | int | Method signature: | int average(String[] layout) | (be sure your method is public) |
| | | | Notes | - | If there are no pathes between people, return 0. | | Constraints | - | layout contains between 1 and 50 elements, inclusive. | - | each element of layout contains between 1 and 50 characters, inclusive. | - | each element of layout will contain the same number of characters. | - | each character in layout is either '#', '.', or 'P'. | | Examples | 0) | | | | 1) | | | | 2) | | | | 3) | | | {"P...P",
"###..",
"P...#",
"####P"} |
| Returns: 6 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
A traveling salesman has recently decided to go international and sell his
wares around the globe. He has done in depth research and has come up with a
list of cities which he thinks will provide the best market for his goods. In
planning his trip, the salesman wants to minimize the total distance he has to
travel because he does not particularly like traveling (which is unfortunate
for him, as he is a traveling salesman) and furthermore, he figures
the less distance he has to travel, the cheaper his trip will be. However,
this salesman is not particularily good at math, and so you must write a
computer program to help him find his way in the least distance.
You will be given a set of cities defined by their longitudes and latitudes.
In addition, you will be given the radius of the planet that this traveling
salesman resides on. Assume that there are direct flights, both ways, between
every pair of cities that the salesman wants to visit, and that the flights
follow the shortest path possible (over the surface of the planet). In
addition, the first element of the input String[] will be the city in which the
salesman lives, and thus his trip must start and end at this city.
Each city is defined by two numbers, a latitude and a longitude. The latitude
is the number of degrees above the equator, with 90 being the north pole, and
-90 being the south pole. The longitude is the number of degrees east or west
of some arbitrary, predefined point. Thus, 90 degrees east is one quarter of
the way around the globe in the eastern direction.
If the result is not an integer, round it to the nearest integer (.5 rounds up)
| | Definition | | Class: | Travel | Method: | shortest | Parameters: | String[], int | Returns: | int | Method signature: | int shortest(String[] cities, int radius) | (be sure your method is public) |
| | | | Notes | - | Assume the planet is a perfect sphere. | - | To find the cartesion coordinates of a city, assuming the center of the planet is at (0,0,0), use the following formulas: x = r*cos(latitude)*cos(longitude) y = r*cos(latitude)*sin(longitude) z = r*sin(latitude) | | Constraints | - | cities contains between 2 and 9 elements, inclusive. | - | Each element of cities represents a unique point on the globe. | - | Each element of cities is formatted as "<latitude> <longitude>" where <latitude> is an integer in the range -90 to 90, inclusive, and <longitude> is an integer in the range -180 to 180, inclusive. | - | radius is an integer between 1 and 1,000, inclusive. | - | to avoid rounding errors, the shortest path, prior to rounding, will not be within 0.001 of <x>+0.5 for any integer <x>. | | Examples | 0) | | | | Returns: 35 | The two cities are located one degree apart at the same latitude |
|
| 1) | | | {"0 0","0 1","0 -1","-1 0","1 0","-1 -1","1 1","1 -1","-1 1"} | 1 |
| Returns: 0 | |
| 2) | | | {"40 -82","-27 -59","-40 48"
,"26 -12","-31 -37","-30 42"
,"-36 -23","-26 71","-19 83","8 63"} | 698 |
| Returns: 4505 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Tim and Tom are playing a game called Super-Doorknobs. From a starting position inside Tim's house, they each have to try to be the first one to touch a given number of doorknobs in any order. Tom, being aware of his disadvantage of not knowing Tim's house, decides to code up a quick algorithm to tell him
which doorknobs to go after.
Given the configuration of the house and the number of doorknobs they need to hit, return the length of the shortest path that includes touching the necessary number of doorknobs.
The house configuration will be a String[] birds-eye view. The game will start in the top-left corner. The following characters will represent the house:
'.' - empty square.
'o' - square with a doorknob (they touch the doorknob the moment they enter this square).
'#' - a wall that neither of them can run through.
For example, if Tim and Tom were racing to touch 3 doorknobs in the following house configuration (quotes added for clarity):
{".....",
"o....",
"o....",
"o....",
"...o."}
The shortest path is straight down, and has a total length of 3. Therefore the method would return 3.
| | Definition | | Class: | Doorknobs | Method: | shortest | Parameters: | String[], int | Returns: | int | Method signature: | int shortest(String[] house, int doorknobs) | (be sure your method is public) |
| | | | Notes | - | If there is no way to reach <doorknobs> doorknobs from the starting location at the top-left of the house, return -1. | - | Tim and Tom can only move up, down, left, or right. Diagonals are not allowed. | | Constraints | - | house will contain between 5 and 50 elements, inclusive. | - | each element of house will be of length 5 to 50, inclusive. | - | each element of house will be the same length as every other element of house. | - | each element of house will contain only the characters '.', '#', and/or 'o'. | - | the first character of the first element of house (the top-left square) will be a '.'. | - | doorknobs will be between 1 and 4, inclusive. | - | the number of 'o' characters in house is between <doorknobs> and 6, inclusive. | | Examples | 0) | | | {"....."
,"o...."
,"o...."
,"o...."
,"...o."} | 3 |
| Returns: 3 | |
| 1) | | | {"....."
,"o...."
,"o...."
,"o...."
,"...o."} | 4 |
| Returns: 7 | |
| 2) | | | {".#..."
,"#...."
,"...oo"
,"...oo"
,"...oo"} | 1 |
| Returns: -1 | Tim and Tom can't move from the starting location (what an odd house).
|
|
| 3) | | | {"...o."
,"o..o."
,"....."
,"..oo."
,"....."} | 4 |
| Returns: 7 | |
| 4) | | | {"....#"
,".##o#"
,".##oo"
,"o##.#"
,"....#"} | 4 |
| Returns: 12 | |
| 5) | | | {"....#"
,"o##o#"
,".##oo"
,".##.#"
,"....#"} | 4 |
| Returns: 8 | |
| 6) | | | {".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,"...................o.............................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,"........................................o........."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,"..........o......................................."
,".................................................."
,".................................................."
,".................................................."
,".....................................o............"
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."
,".................................................."} | 4 |
| Returns: 106 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
I am considering buying a big new riding mower,
but I need to figure out how much hand trimming I will need to do. My lawn is a
square n x n grid of cells. Each cell is either grass, or it is a bush. It
is not possible to drive the mower over a bush.
The new mower occupies two adjacent cells. As it moves, the back end goes over
the same cell that the front end previously occupied, and the front end can be
steered either
to the next cell in the direction the mower is headed, or to one that is
45 degrees to the left or right.The following diagrams give examples showing the possible
cells (1, 2, or 3)
that the front of the mower could be steered to given the location of the front(F)
and back(B).
- 1 2 - - 1
- F 3 B F 2
B - - - - 3
I will build a shed for the
mower which will occupy two cells that are adjacent (either diagonally or orthogonally).
The shed is really just a roof over a concrete floor, so it is open on all sides and
the mower can freely pass through the shed going any direction. I am willing to destroy bushes to
make room for the shed. Each time I mow I must return the mower to the shed facing the same way as
before
I started mowing.
I don't mind going over the same places more than once,
but every cell containing grass that I can't mow will have to be trimmed by hand.
Create a class LawnMower that contains the method trimNeeded that takes the
width of
my lawn, n, and int[]s row and col giving the locations of my bushes, and returns the
number of cells of grass that I will have to trim by hand (with my shed built
in the best location).
An element of row and the corresponding element of col give the location of a bush.
A bush may be listed more than once -- the repetitions can be ignored.
| | Definition | | Class: | LawnMower | Method: | trimNeeded | Parameters: | int, int[], int[] | Returns: | int | Method signature: | int trimNeeded(int n, int[] row, int[] col) | (be sure your method is public) |
| | | | Constraints | - | n is between 2 and 12 inclusive. | - | row contains between 1 and 50 elements inclusive. | - | col contains the same number of elements as does row. | - | Each element of row is between 0 and n-1 inclusive. | - | Each element of col is between 0 and n-1 inclusive. | | Examples | 0) | | | | Returns: 6 |
B S S -
o - B o
o - - o
- o o -
The best place (there are other equally good places) for the shed is row 0,
columns 1 and 2. The mower
can just barely mow in a circle and get back to the shed. Each 'o' shows
a cell that the lawn mower cuts. Note that there are only two bushes (one is
repeated). Each '-' shows a grass cell that cannot be mowed. |
|
| 1) | | | | Returns: 4 | With no bushes in the way (we can't get the mower into the corner anyway) and a
bigger yard, we can build the shed at row 0, columns 1 and 2, and cut everything
except the 4 corners and the middle. Since one of those corners has a bush, that leaves 4 grass cells that must be trimmed by hand. |
|
| 2) | | | | Returns: 5 | There is just one (repeated) bush. We can build the shed on top of the bush
at rows 3 and 4, column 4.
We will then be able to mow everything except the four corners and the middle. |
|
| 3) | | | | Returns: 6 | This yard is just too small for the mower. We can build the shed on two grass
cells and just leave the mower in the shed; that leaves the 9 cells containing 1 bush, 2 shed, and 6 grass, all of which must be trimmed by hand. |
|
| 4) | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | You are working for the FBI and are trying to locate a particular criminal organization.
Within the organization you know which members communicate with each other. The problem is that the members may go by aliases. Given both the information
in the database about the organization, and the field data about a suspicious
organization, you will determine whether they represent the same group. The two sets of
data represent the same group if and only if they only differ by the names of the
participants.
For example:
Database Field Data
FRANK ----- BOB WILLARD ----- GEORGE
| |
| |
| |
GEORGE GREG
The Database and Field Data represent the same organization even though the participants are
using different names.
Renaming Scheme:
FRANK -> WILLARD FRANK -> WILLARD
BOB -> GEORGE or BOB -> GREG
GEORGE -> GREG GEORGE -> GEORGE
If the database data and the field data represent the same organization return how many of
the members are going by aliases, otherwise return -1. If there is more than
one naming scheme possible in mapping the database information to the field
data, use the one that gives the greatest value for the number of aliases. So
in the previous example we would use the first renaming scheme thus giving 3
instead of 2.
The database data will be given in a String[] database. Each element of database will be in the form
"NAME1 NAME2" meaning that NAME1 and NAME2 communicate with each other. The field data will be given in a String[] fieldData that is formatted in the same way as database. In the above example, the input could have been formatted as:
database = {"FRANK BOB","FRANK GEORGE"}
fieldData = {"WILLARD GREG","GEORGE WILLARD"}
In any particular organization, no two people will have the same name or alias. In other words, no two different people in the database will have the same name in database. In addition, no two different people in the field data will have the same name in fieldData. | | Definition | | Class: | Criminal | Method: | numPseudonyms | Parameters: | String[], String[] | Returns: | int | Method signature: | int numPseudonyms(String[] database, String[] fieldData) | (be sure your method is public) |
| | | | Notes | - | Communication is symmetric. In other words, if FRANK and BOB communicate then BOB and FRANK communicate. | | Constraints | - | database will contain between 1 and 28 elements inclusive | - | fieldData will contain between 1 and 28 elements inclusive | - | Each element of database will contain between 3 and 50 characters inclusive | - | Each element of fieldData will contain between 3 and 50 characters inclusive | - | Each element of database and fieldData will be of the form NAME1_NAME2 where '_' is a single space and NAME1 is different than NAME2 NAME1 and NAME2 will have at least 1 character, and will only contain uppercase letters ('A'-'Z') | - | Each element of database and fieldData will have NO leading or trailing whitespace | - | There will be between 2 and 8 unique names inclusive in database | - | There will be between 2 and 8 unique names inclusive in fieldData | - | database cannot contains any repeated elements. In other words, if database contains an element "A B", it cannot contain another element "A B" or "B A". | - | fieldData cannot contain any repeated elements. In other words, if fieldData contains an element "A B", it cannot contain another element "A B" or "B A". | | Examples | 0) | | | {"FRANK BOB","FRANK GEORGE"} | {"WILLARD GREG","GEORGE WILLARD"} |
| Returns: 3 | |
| 1) | | | {"ADAM FRANK","BOB SUZY"} | {"BRETT GEORGE","BRETT TOM"} |
| Returns: -1 | These can't both be describing the same organization. The fieldData contains 3 distinct people where as the database contains 4. |
|
| 2) | | | {"HARRY LLOYD","GEORGE BILL"} | {"FRANK THOMAS","GEORGE WILL","WILL FRANK"} |
| Returns: -1 | These can't both be describing the same organization. The number of pairs of communicating members differs. |
|
| 3) | | | {"A B","A C","AA BB","AA CC","AA DD"} | {"A B","A C","AA BB","AA CC","AA DD"} |
| Returns: 5 | |
| 4) | | | | 5) | | | {"STEVE BRETT", "STEVE LARS", "STEVE JAMES"}
| {"SCHVEIGUY ADMINBRETT", "ADMINBRETT LBACKSTROM", "LBACKSTROM STEVEVAI"} |
| Returns: -1 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
We have a collection of train cars. We want to hook them together to get the
train with the most cars possible. Some cars have a unique front end, and some
can have either end in front. Two cars can be coupled together only if their
coupling mechanisms are compatible.
Each car is described by a String of uppercase letters. The front end is the
end with the letter that comes first in the alphabet (if it starts and ends
with the same letter, either end can be in front). Two words can be hooked
together only if the two adjoining ends have the same letter. Create a class
WordTrain that contains the method hookUp that takes cars, a String[], and
returns a String which is the longest word train that can be made from cars.
If more than one train of the longest length is possible, return the one that
comes first alphabetically. Remember that the length of a train is the number
of cars, not the number of letters.
The returned string should be all the cars in the train, starting at the
front of the train, concatenated with '-' showing the coupling between adjacent
cars. For alphabetic breaking of ties, the '-' is included in its usual order
in the ASCII sequence.
| | Definition | | Class: | WordTrain | Method: | hookUp | Parameters: | String[] | Returns: | String | Method signature: | String hookUp(String[] cars) | (be sure your method is public) |
| | | | Constraints | - | cars contains between 1 and 50 elements inclusive. | - | Each element of cars contains only uppercase letters ('A'-'Z'). | - | Each element of cars contains between 3 and 10 characters inclusive. | | Examples | 0) | | | | Returns: "ABC-CXX" |
This is the only possible train of length 2. Note that CBA needed to be reversed
so that it was facing the right direction.
|
|
| 1) | | | | 2) | | | {"AUTOMATA","COMPUTER","ROBOT"} |
| Returns: "COMPUTER-ROBOT" | |
| 3) | | | {"AAA","AAAA","AAA","AAA"} |
| Returns: "AAA-AAA-AAA-AAAA" | '-' sorts before uppercase letters
|
|
| 4) | | | {"ABA","BBB","COP","COD","BAD"} |
| Returns: "BBB-BAD" | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A city is square and is laid out in nice regular blocks, except that there is one block that
has a "shortcut", a narrow road that goes diagonally through the block. The bank has
just been robbed, and our one on-duty police car starts to chase down the robbers.
The police and robbers always drive at top speed, and they have exactly the same
top speed. Neither the police nor the robbers can leave the city.
It takes 10 seconds to drive one block. It also takes 10 seconds to drive the
shortcut. The police have one advantage -- they have a "bug" in the robbers' car.
One of the robbers tells the driver which way to turn just before they reach each intersection, so
the police can choose their next turn appropriately.
The robbers are apprehended when the police and robbers are at
the same intersection at the same time or when a head-on collision occurs on
the shortcut. (The regular roads are divided roads, so the police cannot apprehend
the robbers if they pass each other going in opposite directions.) U-turns at intersections are
possible, but neither the police nor the robbers may slow down or stop.
Create a class Robbery that contains the method apprehend that takes
the width of the city, n, and a int[] map, giving the locations of the
shortcut, robbers and police as inputs and returns the amount of time until
the robbers are apprehended. If the police can never apprehend the robbers,
the method should return -1. Assume that both the police and robbers behave
optimally (other than robbing the bank in the first place).
n is the number of blocks in both directions (since the city is square). The
intersections are located at x,y where x and y are between 0 and n inclusive.
map contains exactly 8 values: shortcutX1,shortcutY1,shortcutX2,shortcutY2,
policeX,policeY,robbersX,robbersY.
| | Definition | | Class: | Robbery | Method: | apprehend | Parameters: | int, int[] | Returns: | int | Method signature: | int apprehend(int n, int[] map) | (be sure your method is public) |
| | | | Constraints | - | n is between 2 and 30 inclusive. | - | map contains exactly 8 elements. | - | Each element in map is between 0 and n inclusive. | - | The absolute value of (shortcutX1 - shortcutX2) is 1. | - | The absolute value of (shortcutY1 - shortcutY2) is 1. | - | map specifies distinct locations for the police and robbers. | | Examples | 0) | | | | Returns: 30 |
*---*---*---*
| | | |
*---*---*---*
| / | | |
*---P---*---R
| | | |
*---*---*---*
(All example diagrams have (0,0) in the lower left corner.)
If the robbers go west, the police will go east apprehending in 1*10 seconds.
If the robbers go south, the police will go east. Then the robbers will have
to go north or west and will be apprehended after 2*10 = 20 seconds.
If the robbers go north, they will be trapped when they get to the northeast
corner of the city, and will be apprehended after 30 seconds.
|
|
| 1) | | | | Returns: 50 |
*---*---*---*
| | | |
*---*---*---*
| / | | |
*---P---R---*
| | | |
*---*---*---*
The best strategy for the police is to go west, then northeast (using the
shortcut). The robbers can do no better than to head
north, then east, then south and south. Even so, they will then be trapped in
the southeast corner of the city and will be apprehended 10 seconds later.
|
|
| 2) | | | | Returns: 50 |
*---*---*---*
| | | |
*---R---P---*
| | \ | |
*---*---*---*
| | | |
*---*---*---*
|
|
| 3) | | | | Returns: 70 |
*---*---*---*
| | | |
*---*---*---*
| | | |
*---*---*---*
| \ | | |
*---R---*---P
|
|
| 4) | | | | Returns: 60 |
*---*---*---*---*---*
| | | | | |
*---*---*---*---*---*
| | | | | |
*---*---*---*---*---*
| | \ | | | |
R---P---*---*---*---*
| | | | | |
*---*---*---*---*---*
| | | | | |
*---*---*---*---*---*
|
|
| 5) | | | | Returns: 90 |
P---*---*---*---*---*
| | | | | |
R---*---*---*---*---*
| | \ | | | |
*---*---*---*---*---*
| | | | | |
*---*---*---*---*---*
| | | | | |
*---*---*---*---*---*
| | | | | |
*---*---*---*---*---*
|
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | A river can flow either downhill, or at a level elevation for a certain distance. Given a topographical map (a map representing elevations) and a maximum level flow distance, write a method to determine the largest area that a river can cover, if it starts at any location on the map.
The map will be a String[] representing the elevations of each coordinate, with each elevation being a single digit number between 0 (lowest) and 9 (highest).
The maximum level flow distance, maxDist, will be the longest a river can travel on level ground. So if the distance is 0, a river must flow downhill. If the distance is 1, it can flow to 1 adjacent coordinate of the same altitude, in each direction, before it must flow downhill. If the distance is 2, immediately after flowing downhill (or from the starting point), the river can flow to any adjacent coordinate of the same altitude, and then once again to any coordinate adjacent to the new one and of the same altitude, before it must go down hill. For this calculation, the river flows in the 4 primary directions (north, south, east, and west). Notice that since a river will go in any direction it can, it's more of a "flood" than a "river". Thus when determining the area covered by a river starting at a certain point, you must find the area of all the points that can be reached following the above constraints.
For example:
0000000000
0111111110
0122222210
0123333210
0123443210
0123443210
0123333210
0122222210
0111111110
0000000000
Represents a peak with an elevation of 4.
If maxDist = 2 and the river starts flowing at the '*' then the river can cover an area of 16, shown by '~'.
~~~~~~~000
~~*~~11110
~~22222210
~123333210
~123443210
0123443210
0123333210
0122222210
0111111110
0000000000
However this isn't the largest area. If the river starts flowing at the peak, then the river can cover the entire area of 100.
| | Definition | | Class: | RiverHill | Method: | largest | Parameters: | String[], int | Returns: | int | Method signature: | int largest(String[] map, int maxDist) | (be sure your method is public) |
| | | | Notes | - | The river can only flow north, south, east, and west in determining the maximum level flow distance. | - | Each coordinate has an area of 1. | | Constraints | - | map will contain between 2 and 40 elements, inclusive. | - | each element of map will contain between 2 and 40 characters, inclusive. | - | each element of map will contain the same number of characters as every other element of map. | - | each element of map will contain only the characters '0'-'9' inclusive. There will be no spaces. | - | maxDist will be an integer between 0 and 10, inclusive. | | Examples | 0) | | | {"0000000000"
,"0111111110"
,"0122222210"
,"0123333210"
,"0123443210"
,"0123443210"
,"0123333210"
,"0122222210"
,"0111111110"
,"0000000000"} | 2 |
| Returns: 100 | By starting the river at the peak (in either position), the river will be able to flow over the whole mountain.
|
|
| 1) | | | {"0000000000"
,"0111111110"
,"0122222210"
,"0123333210"
,"0123443210"
,"0123443210"
,"0123333210"
,"0122222210"
,"0111111110"
,"0000000000"} | 1 |
| Returns: 95 | If you started at the northwest corner of the peak, the only spaces not reachable would be the 5 spaces diagonally southeast from the start.
|
|
| 2) | | | {"0000000000"
,"0111111110"
,"0122222210"
,"0123333210"
,"0123443210"
,"0123443210"
,"0123333210"
,"0122222210"
,"0111111110"
,"0000000000"} | 0 |
| Returns: 9 | By starting the river at the peak (at any of the four elevation 4 positions), the river will only be able to flow downward in the 4 main directions, thus it will be two straight streams, with an area of 9.
|
|
| 3) | | | {"555505555"
,"555515555"
,"555525555"
,"555535555"
,"555545555"
,"555545555"
,"555555555"} | 1 |
| Returns: 11 |
5555~5555
5555~5555
5555~5555
5555~5555
55~5~5555
5~*~~5555
55~555555
One of the best rivers starts at the asterix (*).
|
|
| 4) | | | {"5565236785012472"
,"3469912625904101"
,"5943545942017700"
,"9109166568720711"
,"6136079994373860"
,"4129136148916755"
,"2975832922074121"
,"4674050103662805"
,"2494729679116165"
,"1611964958059846"
,"2384128690120444"
,"1010697089697114"
,"0089682613597732"
,"4971774663111966"
,"8543141293776080"
,"4298494798935152"
,"5202757354375791"
,"5376161470010537"
,"5696381123743171"
,"1446566116127226"} | 2 |
| Returns: 32 | One of the best rivers (possibly uniquely the best) starts at the space on the 4th row, 6th column (both 0-indexed). The method returns 32.
|
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | At most ski resorts, the runs (a run is defined as an inclined course on which one can ski downhill) are rated by their difficulty. The three classifications commonly used are Green Circle for easy, Blue Square for medium, and Black Diamond for hard. However, there can be a situation where a run is rated easy, but only feeds into hard runs (meaning that all paths from the easy run to the bottom pass through hard runs). Thus, even though the run itself is easy, it should be classified as Black Diamond, since the easiest path to the base of the mountain involves a hard run. Note that one run may feed into many other runs, and that since you are skiing downhill, once you feed into another run, there will be no way to return to any previously skied run (in other words the transitive closure of feeds is acyclic).
For the purposes of this problem, the terms "easy", "medium", and "hard" shall refer to the actual difficulty of the given run, and "Green Circle", "Blue Square", and "Black Diamond" shall refer to the compound difficulty of the
easiest path to the base of the mountain from that run (called the "classification").
Given the run configuration for a ski resort, return the classification of the requested run.
The run configuration will be in the form of a String[], with each element formatted as (quotes added for clarity):
"<name>:<difficulty>,<feed1>,<feed2>,<feed3>,..."
Where <name> is the run's unique name, <difficulty> is a single character representing the difficulty ('E'asy, 'M'edium, or 'H'ard). The <feedX> arguments are all of the runs that <name> feeds into. There can be between 0 and 5 feeds, inclusive. So for example:
"BLACK STALLION:M,BLUE MOUNTAIN,FLYING TOPCODER,DOKS RUN"
is a medium run named BLACK STALLION, which feeds into BLUE MOUNTAIN, FLYING TOPCODER, and DOKS RUN. If all 3 of these were hard, then BLACK STALLION would have to be classified "BLACK DIAMOND", even though it by itself would have been a "BLUE SQUARE".
For the requested run, return either "BLACK DIAMOND" if the easiest path to the base of the mountain must include a hard run, "BLUE SQUARE" if the easiest path to the base of the mountain must include a medium run, but no hard run, and "GREEN CIRCLE" if the easiest path to the base of the mountain is all easy runs.
If, and only if, a run feeds into no other runs, it ends at the base of the mountain and should be treated as the easiest route from itself to the base. Thus if it is rated 'M', it is classified "BLUE SQUARE".
| | Definition | | Class: | Resort | Method: | classify | Parameters: | String[], String | Returns: | String | Method signature: | String classify(String[] runs, String classify) | (be sure your method is public) |
| | | | Notes | - | The classification of a run is not affected by the difficulty of any runs which feed into it. | | Constraints | - | runs will contain between 1 and 50 elements, inclusive | - | each element of runs will consist of only capital letters ('A'-'Z'), spaces, commas (','), and a colon (':'). | - | each element of runs will contain between 3 and 50 characters, inclusive, and will be formatted as (quotes added for clarity): "<name>:<difficulty>,<feed1>,<feed2>,<feed3>..." | - | there may be between 0 and 5 feeds, inclusive, for each run. | - | there will be no commas except for a single comma between feeds and between the difficulty and the first feed (if there is a first feed). | - | <difficulty> will be a single character capital letter, either 'E', 'M', or 'H'. | - | <name> will be between 1 and 48 characters in length, inclusive. | - | <name> will be unique and consist of only capital letters ('A'-'Z') and spaces, but will not begin or end with a space. | - | each feed will reference a run that appears as <name> in some element of runs (this is necessary to determine its difficulty). | - | within each element of runs, the feeds will be unique. | - | runs will contain no loops, i.e. runs will contain no circular or self references. | - | classify will be between 1 and 48 characters in length, inclusive, and will reference a run that appears as <name> in some element of runs. | | Examples | 0) | | | {"BLACK STALLION:M,BLUE MOUNTAIN,TOPCODER,DOKS RUN"
,"BLUE MOUNTAIN:H"
,"TOPCODER:H"
,"DOKS RUN:H,CHOGYS RUN"
,"CHOGYS RUN:M,EASY RUN,MEDIUM RUN,HARD RUN"
,"EASY RUN:E"
,"MEDIUM RUN:M"
,"HARD RUN:H"} | "BLACK STALLION" |
| Returns: "BLACK DIAMOND" | The path to the base must go through either BLUE MOUNTAIN, TOPCODER, or DOKS RUN, all of which are hards.
|
|
| 1) | | | {"BLACK STALLION:M,BLUE MOUNTAIN,TOPCODER,DOKS RUN"
,"BLUE MOUNTAIN:H"
,"TOPCODER:H"
,"DOKS RUN:H,CHOGYS RUN"
,"CHOGYS RUN:M,EASY RUN,MEDIUM RUN,HARD RUN"
,"EASY RUN:E"
,"MEDIUM RUN:M"
,"HARD RUN:H"} | "DOKS RUN" |
| Returns: "BLACK DIAMOND" | Since DOKS RUN itself is hard, it doesn't matter how hard the runs below it are.
|
|
| 2) | | | {"BLACK STALLION:M,BLUE MOUNTAIN,TOPCODER,DOKS RUN"
,"BLUE MOUNTAIN:H"
,"TOPCODER:H"
,"DOKS RUN:H,CHOGYS RUN"
,"CHOGYS RUN:M,EASY RUN,MEDIUM RUN,HARD RUN"
,"EASY RUN:E"
,"MEDIUM RUN:M"
,"HARD RUN:H"} | "CHOGYS RUN" |
| Returns: "BLUE SQUARE" | CHOGYS RUN is a medium difficulty. Since the easiest path from CHOGYS RUN to the base of the mountain is CHOGYS RUN->EASY RUN, and a medium difficulty is the hardest run in the path, CHOGYS RUN is classified as "BLUE SQUARE".
|
|
| 3) | | | {"NOOB CITY:E,GREEN BARON,RISKY RUN,LEAP OF FAITH"
,"GREEN BARON:E"
,"RISKY RUN:M"
,"LEAP OF FAITH:H"} | "NOOB CITY" |
| Returns: "GREEN CIRCLE" | The easiest path is NOOB CITY->GREEN BARON, both of which are easy.
|
|
| 4) | | | {"NOOB CITY:E,RISKY RUN,LEAP OF FAITH"
,"RISKY RUN:M"
,"LEAP OF FAITH:H"} | "NOOB CITY" |
| Returns: "BLUE SQUARE" | The easiest path is NOOB CITY->RISKY RUN, and RISKY RUN is a medium difficulty.
|
|
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
TopPilot airlines is committed to customer service and convenience. Thus, it
is not simply enough to have a flight from San Francisco, CA to Hartford, CT.
There must be a direct flight.
You will be given a String[] representing one-way flight paths. For each
element in the String[], the element index is the departure airport number
(0-indexed, so the first element is airport 0) and the element is a
comma-delimited list of airport numbers to which there is a direct flight from the departure airport.
Write a method that, given these one-way flight paths, adds direct one-way
flights between airports that are currently connected by non-direct one-way
flights. For example, if there is a direct flight from SFO to JFK and a direct flight from
JFK to LAX, and not a direct flight from SFO to LAX, then add a direct flight from SFO to LAX. Do not, however, add a direct flight from an airport to itself, even if a loop exists. Once this is done, the
method should return the total number of flights provided by TopPilot airlines.
| | Definition | | Class: | TopPilot | Method: | addFlights | Parameters: | String[] | Returns: | int | Method signature: | int addFlights(String[] destinations) | (be sure your method is public) |
| | | | Notes | - | If the destination airport does not appear as an element in destinations (which is always the case when it is greater than 19), there are no departing flights from that airport. | - | Leading zeroes in the numbers are allowed. | | Constraints | - | No airport will have a direct flight to itself. | - | destinations will have between 1 and 20 elements, inclusive. | - | each element of destinations will contain only the characters '0' through '9', and commas ','. | - | each element of destinations will be a comma delimited list of integers, with no numbers repeated, and no leading, trailing, or extra commas. | - | each element of destinations will be contain between 1 and 50 characters, inclusive. | - | destination airport numbers will be between 0 and 50, inclusive. | | Examples | 0) | | | | Returns: 6 | There is a flight from airport 0 to airport 1, from 1 to 2, and from 2 to 3.
We add a direct flight from 0 to 2, from 1 to 3, and from 0 to 3 (note that 3
is not an element, so it has no departing flights). |
|
| 1) | | | | Returns: 14 | We add flights from 0 to 2, 3, 4, and 5. We add flights from 1 to 3, 4, and 5.
We add flights from 2 to 4 and 5. Together with the 5 flights we already
have, there are 14 total. |
|
| 2) | | | | Returns: 6 | The only added flight is from 2 to 0. |
|
| 3) | | | {"1,5,7,8,13,15,16,23,26,35,32,41"
,"0,2,5,6,7,15,18,21,25,31,34,41,49,50"
,"5,10,15,20,25,30,35,40,45,50"} |
| Returns: 62 | |
|
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | PROBLEM STATEMENT
After writing a careful and correct data checking method, a problem writer then
has to code a solution. This is what you should now do.
Implement a class Solver with a method largest() that will take a String[]
lovers as an argument. Each element of lovers will be formatted as follows:
"NAME1 LOVES NAME2" (quotes added for clarity).
With the capital word LOVES in between names, and the names containing only
capital letters [A-Z] and/or hyphens '-'.
For each NAME2 in lovers there will be a corresponding NAME1. One person may
love multiple people (repeated NAME1), multiple people may love one person
(repeated NAME2), but no person may love themselves (NAME1 equals NAME2).
Return the number of people involved in the largest love triangle. That is,
the largest chain of people such that:
A LOVES B
B LOVES C
C LOVES D
D LOVES E
E LOVES A
until the last person loved (NAME2--in this example A) appears somewhere else
in the chain as NAME1, at which point the triangle starts and ends with that
name.
The triangle above consists of 5 people (A B C D and E).
A love triangle can consist of 2 or more people.
DEFINITION
Class name: Solver
Method name: largest
Parameters: String[]
Returns: int
The method signature is:
int largest(String[] lovers)
Be sure your method is public.
TopCoder will ensure the following (there is no difference between these and
the 500 point problem psuedo-restrictions):
*lovers will contain between 2 and 20 elements, inclusive.
*Each element of lovers will contain less than or equal to 40 characters and
will be formatted as
"NAME1 LOVES NAME2" (quotes added for clarity again)
with the capital word LOVES and only one space between words.
*NAME1 and NAME2 will be names of non-zero length.
*NAME1 and NAME2 will not be identical (everyone loves themselves anyway).
*NAME1 and NAME2 will contain only capital letters [A-Z] and/or hyphens '-'.
*For each NAME2 there will be a corresponding NAME1 in lovers. That is,
everyone loves someone else in the problem.
*One person may love multiple people (repeated NAME1 in different elements) and
one person may be loved by multiple people (repeated NAME2 in different
elements).
*It is possible for two elements to be identical.
(ex {"A LOVES B","A LOVES B","B LOVES A"} is valid).
EXAMPLES
{"D LOVES M",
"M LOVES D",
"T LOVES G",
"G LOVES D"}
The largest love triangle (between D and M) is 2.
{"ME LOVES YOU",
"ME LOVES YOU",
"YOU LOVES ME"}
The largest triangle is between YOU and ME, so the method returns 2.
{"A LOVES B",
"B LOVES C",
"C LOVES A",
"B LOVES D",
"D LOVES E",
"E LOVES C",
"E LOVES F",
"F LOVES G",
"G LOVES F"}
This looks as follows:
/---<---\
| |
A->-B->-C
| ^
v |
D->-E
|
v
G<->F
A->B->D->E->C->A is the largest triangle, which is of size 5.
{"A LOVES B",
"B LOVES C",
"C LOVES B",
"B LOVES A"}
Either A->B->A or B->C->B are legal, and both of size 2. We see that
A->B->C->B->A is not legal since, by the above definition of triangle, once
person B appears as both NAME2 and NAME1 in the triangle, the triangle can go
no further and must start and end with person B (giving B->C->B). The method
should return 2.
| | Definition | | Class: | Solver | Method: | largest | Parameters: | String[] | Returns: | int | Method signature: | int largest(String[] param0) | (be sure your method is public) |
| | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | PROBLEM STATEMENT
Using blocks of binary logic defined by their truth tables, build a block with
a desired truth table. Each block of binary logic has two inputs and two
outputs. Block inputs are referred to as i0 and i1; block outputs are referred
to as o0 and o1. Logic inside a block implements its truth tables for each of
its two outputs. To build a block with the target truth table, connect blocks
in a chain, wiring both outputs of a block to both inputs of another block.
Your method should return the number of blocks in the shortest chain
implementing the target truth table, or -1 when it is impossible to build a
chain with the target truth table.
DEFINITION
Class name: BinBlocks
Method name: targetChain
Parameters: String[], String
Return type: int
Method signature: int targetChain(String[] blocks, String target) (make sure
your method is public)
Both the target and the elements of blocks are String representations of truth
tables formatted as follows (quotation marks are used for clarity - they are
not part of the strings):
"abcd,efgh"
Each character represents a digit '0' or '1'. Group abcd represents the truth
table of the output o0, while efgh represents the truth table of the output o1.
The characters of "abcd,efgh" correspond to the following states of inputs:
i0 i1 | o0 o1
-------+-------
0 0 | a e
1 0 | b f
0 1 | c g
1 1 | d h
For example, truth table "0001" corresponds to the truth table of the operation
AND: (i0 AND i1).
TopCoder will check that
- blocks will contain 0 to 50 elements, inclusive, and
- all truth tables are properly formatted
NOTES
* Blocks are ordered. Your method may not alter the order of the blocks while
building the target chain. It may, however, skip any number of blocks.
* For certain values of target, the optimal chain would consist of zero logical
blocks. See examples 1 and 2.
* When connecting logical blocks A and B in a chain, you may wire outputs to
inputs in either of the two ways: A o0 to B i1 and A o1 to B i1, or A o0 to B
i1 and A o1 to B i0. This rule applies even in cases when the optimal chain is
empty.
EXAMPLES
1. blocks= {}, target="0101,0011". targetChain should return 0 because wiring
i0 to o0 and i1 to o1 implements the target truth table.
2. blocks= {"0000,1111","0000,0101"}, target="0011,0101". targetChain should
return 0 because wiring i0 to o1 and i1 to o0 implements the target truth
table, without using any of the blocks.
3. blocks= {"0001,0111","1010,1100"}, target="1110,1000". targetChain should
return 2 because you need to wire both blocks in a sequence to get the target
truth table.
4. blocks= {"0001,0111","0101,0011"}, target="0111,0001". targetChain should
return 1 because the target truth table is the "flipped" truth table of the
block 0.
5. blocks= {}, target="1111,0000". targetChain should return -1 because it is
impossible to implement the target truth table.
6. blocks= {"0101,1010","0110,1001","1110,0001","1010,1100","1000,0001"},
target= "1111,0000". targetChain should return 2. For a graphical
representation of this please refer to
http://www.topcoder.com/contest/blocks.html
7 a. blocks={"0001,1101","1010,1011"}, target="0010,0011". targetChain returns 2
b. blocks={"0001,1101","1010,1011"}, target="0011,0010". targetChain returns 2
c. blocks={"0001,1101","1010,1011"}, target="1110,1111". targetChain returns 2
d. blocks={"0001,1101","1010,1011"}, target="1111,1110". targetChain returns 2
e. blocks={"0001,1101","1010,1011"}, target="0100,0101". targetChain returns 2
f. blocks={"0001,1101","1010,1011"}, target="0101,0100". targetChain returns 2
| | Definition | | Class: | BinBlocks | Method: | targetChain | Parameters: | String[], String | Returns: | int | Method signature: | int targetChain(String[] param0, String param1) | (be sure your method is public) |
| | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | THIS PROBLEM WAS TAKEN FROM THE FINALS OF THE TOPCODER INVITATIONAL TOURNAMENT
PROBLEM STATEMENT
A network consists of a system of numNodes nodes, numbered from 0 to
numNodes-1, and connections between those nodes. A connection has a chance of
being up and a chance of being down. Implement a class Networking that contains
a method getProbability which calculates the chance that there will be a path
from node 0 to node numNodes-1 such that each connection in the path is up.
(See the examples to see how probabilities can be calculated)
DEFINITION
Class Name: Networking
Method Name: getProbability
Parameters: int, String[]
Returns: double
Method signature (be sure your method is public): double getProbability(int
numNodes, String[] connections);
numNodes is an int representing the number of nodes.
connections is a String[] representing the connections. The elements will be
of the form "N1 N2 P" where N1 and N2 represent the numbers of the two nodes
the connection connects, and P is the probability the connection will be up.
There will be precisely one space between N1 and N2 and one space between N2
and P. The nodes are numbered from 0 to numNodes-1. Every connection connects
two nodes (or possibly a node to itself).
OUTPUT
A double representing the probability there exists a path from node 0 to node
numNode-1 such that every connection in the path is up. Your result must be
within 10^-6 of our solution's result (tolerance for double inaccuracies).
NOTES
* A "path" is a series of one or more connections such that each successive
connection starts at the node where the previous connection ended.
* The connections are bi-directional.
* There can be multiple (parallel) connections connecting two nodes.
TopCoder will ensure the validity of the inputs. Inputs are valid if all of
the following criteria are met:
* numNodes is an integer between 2 and 10, inclusive.
* Elements of connections are of the form described in the input above.
* connections has between 0 and 20 elements, inclusive.
* P is a decimal value between 0 and 1 inclusive with exactly one digit before
the decimal point and two digits after the decimal point.
* N1 and N2 are single integer digits between 0 and numNodes-1, inclusive.
EXAMPLES
* Nodes are in series. For example, if numNodes = 3 and connections = {"0 1
0.20", "1 2 0.10"}, the network looks as follows (letters are added for
clarity):
___ ___ ___
| | 0.20 | | 0.10 | |
| 0 |-----------| 1 |------------| 2 |
|___| a |___| b |___|
In this case, the probability of a connection from 0 to 2 is the probability
that a AND b are up. The AND relationship corresponds to multiplication.
Therefore, to calculate this all you need to do is mutliply the probability of
0->1 by the probability of 1->2.
(0.20 * 0.10) = 0.02
So the method should return 0.02.
* Nodes are in parallel. If numNodes = 4 and connections = {"0 1 0.10", "1 3
0.20", "0 2 0.30", "2 3 0.40"}, the network looks as follows:
___
| |
0.10 /-----| 1 |-----\ 0.20
/ |___| \
___ / a b \ ___
| | / \| |
| 0 | / | 3 |
|___| \ /|___|
\ ___ /
\ c | | d /
\------| 2 |-----/
0.30 |___| 0.40
In order for this to happen, either a and b have to be up, or c and d have to
be up. The probability a connection exists from 0 to 3 now becomes the
probability that (a AND b are up) OR (c AND d are up).
This equals P(a AND b up) OR P(c AND d up) = P(a up)*P(b up) + P(c up)*P(d up)
- P(a and b and c and d up) = 0.1 * 0.2 + 0.3 * 0.4 - 0.1 * 0.2 * 0.3 * 0.4 =
0.1376.
(The probability that they are all up is subtracted out at the end because it
is added as both P(a AND b up) and P(c AND d up)).
* If numNodes=2 and connections = {"0 1 0.80"}, there is a path from node 0 to
node 1 when the connection is up, so the method should return 0.8.
* If numNodes=3 and connections = {"0 2 0.50", "0 2 0.50", "0 1 0.10", "1 2
0.20","1 1 0.60"}, there is a path from node 0 from node 2 if either of the
first two connections are up or if both the 3rd and 4th connection are up, so
the method should return 0.755. Note the connection from node 1 to node 1 does
not affect the result.
* If numNodes=4 and connections = {"0 2 0.06", "3 1 1.00", "1 3 0.50", "1 2
0.00"}, the method should return 0.0.
* If numNodes=4 and connections = {"0 1 0.10", "1 3 0.20", "0 2 0.30", "2 3
0.40", "1 2 0.60"}, the method should return 0.17048.
This can be calculated by reducing the network as follows:
The original network looks like:
___
_____| |_____
0.10 / | 1 | \ 0.20
/ |___| \
___ / a | b \ ___
| | / |e \| |
| 0 |/ |0.60 | 3 |
|___|\ | /|___|
\ _|_ /
\ c | | d /
\______| 2 |_____/
0.30 |___| 0.40
This solution can be found by reducing the network.
The chance the network is up is P(e up)*P(Network up, given e up) + P(e
down)*P(Network up, given e down).
P(Network up, given e up):
Given e up, the network becomes:
0.10 0.20
______ ________
___ / a \ ___ / b \ ___
| |/ \| |/ \| |
| 0 |\ c /|1,2|\ d /| 3 |
|___| \______/ |___| \________/ |___|
0.30 0.40
The probability this is up is :
P((a OR c up) AND (b OR d up)) =
P(a OR c up) * P(b OR d up) =
(0.1+ 0.3- 0.1 * 0.3) * (0.2 + 0.4-0.2 * 0.4) = 0.1924
Given e down, the network becomes:
___
| |
0.10 /-----| 1 |-----\ 0.20
/ |___| \
___ / a b \ ___
| | / \| |
| 0 | / | 3 |
|___| \ /|___|
\ ___ /
\ c | | d /
\------| 2 |-----/
0.30 |___| 0.40
And low and behold, this is the network from the last example, and the
probability of it being up is: 0.1376
Therefore, the probability of the entire network being up is:
P(e up)*P(Network up, given e up) + P(e down)*P(Network up, given e down) =
0.6 * 0.1924 + 0.4 * 0.1376 = 0.17048
The method should return 0.17048.
| | Definition | | Class: | Networking | Method: | getProbability | Parameters: | int, String[] | Returns: | double | Method signature: | double getProbability(int param0, String[] param1) | (be sure your method is public) |
| | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Class Name: FSM
Method Name: runFSM
Paramaters: String String String[]
Returns String[]
Implement a class FSM, which contains a method runFSM. The method runs an
augmented finite state machine:
The augmented finite state machine consists of a number of states. Each state
has a unique name of type String. There are edges connecting the states.
These edges are directional: they go in only one direction, their source state
to their sink state (source and sink states are described below). Each edge
has a corresponding symbol and function that are both represented as Strings.
Here is the method signature (be sure your method is public):
String[] runFSM(String symbols, String states, String[] edges)
Input:
*symbols - The first parameter to runFSM is a String containing elements
referred to as symbols that will be passed to the FSM. The elements/symbols
will contain letters only (A-Z and a-z, inclusive). Each element of the
symbols input parameter is separated by one or more spaces.
*states - The second parameter to runFSM is a String containing elements that
refer to the names of the states. The names/states will contain letters (A-Z
and a-z, inclusive) only. The first state in this list is the starting state.
Each element of the states input parameter is separated by one or more spaces.
*edges[] - The third parameter is a String[]. Each element in the String[]
describes an edge. The Strings in the array will contain four sets of letters,
where the sets are separated by one or more spaces. Parsing the String from
the left-most character moving right, the first set of letters is the source
state - the state in which the edge starts. The second set of letters is the
sink state - the state in which the edge ends. The third set of letters is the
symbol corresponding to the edge. The fourth set of letters is the function
corresponding with the edge.
Output:
The output is a String[] containing a list of all the functions called with
their associated symbols as parameters, in order. There should be only one
element in the output for each symbol in the first input String.
The augmented finite state machine is passed a sequence of symbols. Each
symbol is processed as follows:
1. Use the first state (the first element of the states String) as the source
state and let that equal the current state.
2. Determine the first symbol (i.e. the first element of the symbols String)
let that equal the current symbol.
3. Find the edge of the current state that corresponds to the current symbol.
4. Add a String to the output array. The String should consist of the
function name of the edge found in step 3 with the current symbol enclosed in
parentheses (i.e. if the function is "open" and the symbol is "hi", the
function call is "open(hi)").
5. Move to the sink state that corresponds with the current symbol.
6. Let current state be equal to the sink state from step 5
7. Repeat steps 3 - 6 for the remaining symbols.
Note:
* If ever there is no edge leaving the current state with the current symbol,
add the String "ERROR" to the output array, remain at the current state, and go
on to the next symbol.
* No two edges may contain the same source state and symbol.
* All String comparisons are case sensitive.
* The output is a list of the function calls created in step four (above) in
order.
* The sink state can be equal to the source state
* There may be additional spaces at the beginning or end of any input String.
TopCoder will ensure:
- All the Strings and elements of arrays will contain letters (A-Z and a-z,
inclusive) and spaces (' ') only.
- The states mentioned in the edges array will be present in the states String.
- symbols will contain at least one element.
- states will contain at least one element.
- symbols and states will have length between 1 and 50, inclusive.
- edges will contain between 1 and 50 elements, inclusive, and each element
will contain between 1 and 50 characters, inclusive.
- No two edges will have the same source state and symbol
- Elements of the edges array will be well formed. That is, each element will
be in the form: "(source state) (sink) (state symbol) (function)" (the
parentheses are for clarity and should not be included in the String). There
will be exactly four elements separated by one or more spaces
Examples:
Example relating to a vending machine that releases candy every time 20 cents
is put in, assume:
states="Zerocents Fivecents Tencents Fifteencents Twentycents"
and
edges={
"Zerocents Fivecents nickel needMoreOne",
"Zerocents Tencents dime needMoreTwo",
"Fivecents Fifteencents dime needMoreThree",
"Fivecents Tencents nickel needMoreFour",
"Tencents Fifteencents nickel needMoreFive",
"Tencents Twentycents dime releasingCandyOne",
"Fifteencents Twentycents nickel releasingCandyTwo",
"Fifteencents Twentycents dime releasingCandyThree",
"Twentycents Fivecents nickel needMoreSix",
"Twentycents Tencents dime needMoreSeven"
}
If symbols="nickel dime dime", the output should be:
{"needMoreOne(nickel)",
"needMoreThree(dime)",
"releasingCandyThree(dime)"}
If symbols="dime oops dime" the output should be
{"needMoreTwo(dime)",
"ERROR",
"releasingCandyOne(dime)"}
If symbols="dime nickel dime dime dime nickel" the output should be
{"needMoreTwo(dime)",
"needMoreFive(nickel)",
"releasingCandyThree(dime)",
"needMoreSeven(dime)",
"releasingCandyOne(dime),
"needMoreSix(nickel)}
More Examples:
If symbols=" a a a b b c" and
states=" stateZero stateOne stateTwo"
and edges=
{"stateZero stateOne a doSomething",
"stateOne stateTwo b doNothing",
"stateTwo stateZero c doEverything"}
The output should be
{"doSomething(a)",
"ERROR",
"ERROR",
"doNothing(b)",
"ERROR",
"doEverything(c)"}
| | Definition | | Class: | FSM | Method: | runFSM | Parameters: | String, String, String[] | Returns: | String[] | Method signature: | String[] runFSM(String param0, String param1, String[] param2) | (be sure your method is public) |
| | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
Class name: WhaleWatcher
Method name: getWhaleSightings
Parameters: String[], int, int , int , String
Returns: int
Implement a class WhaleWatcher, which has a method getWhaleSightings.
TopCoder will enforce the following rules:
*WhalePositions has between 1 and 10 Strings, inclusive.
*Passengers is between 1 and 100, inclusive.
*StartPosition is between 0 and 9, inclusive
*TripDistance is between 0 and 9, inclusive.
*Weather is one of "Cloudy", "Foggy", "Sunny"
*The elements of WhalePositions are of the form "X,Y", where X and Y represent
integers between 0 and 9, inclusive.
*The elements of WhalePositions will be distinct (not repeated)
(In this problem statement, the variable names will sometimes be enclosed in
angle brackets <> for clarity)
The following are true:
*All action takes place on a 10x10 grid whose cells are referenced by Strings
of the form "X,Y" where X and Y are between 0 and 9.
*The point "0,0" through "9,0" are the cells in the water next to the shoreline.
*The good ship Orca takes on up to 100 passengers and leaves port at a point
represented by "StartPosition,0".
*It then travels straight out to sea until it reaches the position
"StartPosition,TripDistance". That is, the ship starts at the point
"StartPosition,0", moves to "StartPosition,1", etc. until it reaches the point
"StartPosition,TripDistance".
Another way to say this is "The boat starts at <StartPosition> and moves up
<TripDistance> number of grid spaces". If you think of the boat moving through
time, then at time 1, the boat would be at "StartPositionX,0". At time 2, the
boat would be at "StartPosition,1", and at time <TripDistance>, the boat would
be at "StartPosition,TripDistance".
Your method should return the total number of times a passenger sees a whale on
this trip.
*The passenger's position on the boat does not matter.
The passengers will see whales in the following way:
*The passengers never see whales that are directly under the boat.
*If the weather is "Foggy", passengers will only see whales that are in the
cells immediately adjacent to the boat. (If boat is at "2,3", passengers will
see whales that are in the cells "1,4", "2,4", "3,4", "1,3", "3,3", "1,2",
"2,2" and "3,2")
*If the weather is "Cloudy", passengers will see whales up to 2 cells away from
the boat.
*If the weather is "Sunny", passengers will see whales up to 3 cells away from
the boat.
*The boat will move on the aforementioned path one graph unit at a time.
Each time the boat moves, check to see which whales can be seen.
*If a whale is seen by the passengers once, never count that whale again.
The method signature is (be sure your method is public):
public int getWhaleSightings(String[] WhalePositions, int Passengers, int
StartPosition, int TripDistance, String Weather)
Example 1:
WhalePositions = { "2,3", "5,6" }
passengers = 50
StartPosition = 5
TripDistance = 4
Weather = "Sunny"
The boat starts at "5,0" and will move through the cells "5,1", "5,2", "5,3",
and "5,4".
Grid:
0 1 2 3 4 5 6 7 8 9
+-+-+-+-+-+-+-+-+-+-+
9| | | | | | | | | | |
+-+-+-+-+-+-+-+-+-+-+
8| | | | | | | | | | |
+-+-+-+-+-+-+-+-+-+-+
7| | | | | | | | | | |
+-+-+-+-+-+-+-+-+-+-+
6| | | | | |W| | | | |
+-+-+-+-+-+-+-+-+-+-+
5| | | | | | | | | | |
+-+-+-+-+-+-+-+-+-+-+
4| | | | | |5| | | | |
+-+-+-+-+-+-+-+-+-+-+
3| | |W| | |4| | | | |
+-+-+-+-+-+-+-+-+-+-+
2| | | | | |3| | | | |
+-+-+-+-+-+-+-+-+-+-+
1| | | | | |2| | | | |
+-+-+-+-+-+-+-+-+-+-+
0| | | | | |1| | | | |
+-+-+-+-+-+-+-+-+-+-+
0 1 2 3 4 5 6 7 8 9
Legend:
W = whale
A number N = the position the boat is in at time N
When the boat is at: Passengers See Whales at:
---------------------------------------------------------
"5,0" "2,3"
"5,1" "2,3" (2nd sighting)
"5,2" "2,3" (3rd sighting)
"5,3" "2,3" (4th sighting), "5,6"
"5,4" "2,3" (5th sighting), "5,6" (2nd sighting)
The passengers saw a total of 2 whales so return 50 * 2 = 100.
Example 1:
WhalePositions = { "2,3", "5,6", "9,0", "9,1", "8,2"}
passengers = 12
StartPosition = 9
TripDistance = 1
Weather = "Foggy"
The boat starts at "9,0" and will move to the cell "9,1".
Grid:
0 1 2 3 4 5 6 7 8 9
+-+-+-+-+-+-+-+-+-+--+
9| | | | | | | | | | |
+-+-+-+-+-+-+-+-+-+--+
8| | | | | | | | | | |
+-+-+-+-+-+-+-+-+-+--+
7| | | | | | | | | | |
+-+-+-+-+-+-+-+-+-+--+
6| | | | | |W| | | | |
+-+-+-+-+-+-+-+-+-+--+
5| | | | | | | | | | |
+-+-+-+-+-+-+-+-+-+--+
4| | | | | | | | | | |
+-+-+-+-+-+-+-+-+-+--+
3| | |W| | | | | | | |
+-+-+-+-+-+-+-+-+-+--+
2| | | | | | | | |W| |
+-+-+-+-+-+-+-+-+-+--+
1| | | | | | | | | |2W|
+-+-+-+-+-+-+-+-+-+--+
0| | | | | | | | | |1W|
+-+-+-+-+-+-+-+-+-+--+
0 1 2 3 4 5 6 7 8 9
Legend:
W = whale
A number N = the position the boat is in at time N
(The ninth column is widened to accomodate the two chars that show
that the boat and a whale are in the same cell at that time)
When the boat is at: Passengers See Whales at:
---------------------------------------------------------
"9,0" "9,1"
"9,1" "9,0", "8,2"
The passengers saw a total of 3 whales so return 12 * 3 = 36.
Test cases:
WhalePositions = "0,9"
passengers = 14
StartPosition = 0
TripDistance = 9
Weather = "Foggy"
returns 14
WhalePositions = "8,1" "8,2" "9,9"
passengers = 10
StartPosition = 9
TripDistance = 4
Weather = "Cloudy"
returns 20
WhalePositions = "5,5" "4,3" "6,1" "5,9"
passengers = 5
StartPosition = 5
TripDistance = 5
Weather = "Sunny"
returns 15
| | Definition | | Class: | WhaleWatcher | Method: | getWhaleSightings | Parameters: | String[], int, int, int, String | Returns: | int | Method signature: | int getWhaleSightings(String[] param0, int param1, int param2, int param3, String param4) | (be sure your method is public) |
| | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | |
***Note: Please keep programs under 7000 characters in length. Thank you
Class Name: Prerequisites
Mathod Name: orderClasses
Parameters: String[]
Returns: String[]
You are a student at a college with the most unbelievably complex prerequisite
structure ever. To help you schedule your classes, you decided to put together
a program that returns the order in which the classes should be taken.
Implement a class Prerequisites which contains a method orderClasses. The
method takes a String[] that contains the classes you must take and returns a
String[] of classes in the order the classes should be taken so that all
prerequisites are met.
String[] elements will be of the form (and TopCoder will ensure this):
"CLASS: PRE1 PRE2 ..." where PRE1 and PRE2 are prerequisites of CLASS. CLASS,
PRE1, PRE2, ... consist of a department name (3 or 4 capital letters, A-Z
inclusive) followed by a class number (an integer between 100 and 999,
inclusive). The department name should be immediately followed by the class
number with no additional characters, numbers or spaces (i.e. MATH217). It is
not necessary for a class to have prerequisites. In such a case, the colon is
the last character in the String.
You can only take one class at a time, therefore, use the following rules to
determine which class to take :
1) Any prerequisite class(es) listed for a class must be taken before the class
can be taken.
2) If multiple classes can be taken at the same time, you take the one with the
lowest number first, regardless of department.
3) If multiple classes with the same number can be taken at the same time, you
take the department name which comes first in alphabetical order.
4) If the inputted course schedule has errors, return a String[] of length 0.
There is an error if it is impossible to return the classes in an order such
that all prerequisites are met, or if a prerequisite is a course that does not
have its own entry in the inputted String[].
Examples of valid input Strings are:
"CSE111: CSE110 MATH101"
"CSE110:"
Examples of invalid input Strings are:
"CS117:" (department name must consist of 3 - 4 capital letters, inclusive)
"cs117:" (department name must consist of 3 - 4 capital letters, inclusive)
"CS9E11:" (department name must be letters only)
"CSE110: " (no trailing spaces allowed)
"CSE110: CSE101 " (no trailing spaces allowed)
"MATH211: MAM2222" (class number to large)
"MATH211: MAM22" (class number to small)
"ENGIN517: MATH211" (department name to large)
Here is the method signature (be sure your method is public):
String[] orderClasses(String[] classSchedule);
TopCoder will make sure classSchedule contains between 1 and 20 Strings,
inclusive, all of the form above. The Strings will have between 1 and 50
characters, inclusive. TopCoder will check that the syntax of the Strings are
correct: The Strings will contain a valid class name, followed by a colon,
possibly followed by a series of unique prerequisite classes separated by
single spaces. Also, TopCoder will ensure that each class has at most one
entry in the String[].
Examples:
If classSchedule={
"CSE121: CSE110",
"CSE110:",
"MATH122:",
}
The method should return: {"CSE110","CSE121","MATH122"}
If classSchedule={
"ENGL111: ENGL110",
"ENGL110: ENGL111"
}
The method should return: {}
If classSchedule=[
"ENGL111: ENGL110"
}
The method should return: {}
If classSchedule={
"CSE258: CSE244 CSE243 INTR100"
"CSE221: CSE254 INTR100"
"CSE254: CSE111 MATH210 INTR100"
"CSE244: CSE243 MATH210 INTR100"
"MATH210: INTR100"
"CSE101: INTR100"
"CSE111: INTR100"
"ECE201: CSE111 INTR100"
"ECE111: INTR100"
"CSE243: CSE254"
"INTR100:"
}
The method should return:
{"INTR100","CSE101","CSE111","ECE111","ECE201","MATH210","CSE254","CSE221","CSE2
43","CSE244","CSE258"}
| | Definition | | Class: | Prerequisites | Method: | orderClasses | Parameters: | String[] | Returns: | String[] | Method signature: | String[] orderClasses(String[] param0) | (be sure your method is public) |
| | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | Class name: Inheritance
Method name: resolve
Parameters: String[], String, String
Returns: String
As Java programmers, you are familiar with the mechanics of inheritance. Each
class (except for Object) has an ancestor (in Java this ancestor is specified
after the "extends" keyword; when no ancestor is specified, the class extends
Object). Each class inherits certain methods of its parent class, as well as
those of its parent, and so on, all the way back to Object. However, a class
can also define its own version of each of these methods, overriding the one
inherited from its ancestors.
When a method is called on a particular object, there must be a mechanism at
work to determine which class's method is used. For instance, if the toString
method is called on an object of a class that does not implement its own
toString method, then that class's parent is checked to see if it implements
that particular method. If it doesn't, then its parent is checked in the same
manner, all the way up to Object, which has no parent. If even Object does not
implement the method, then that method is not defined for the object it was
called on.
Implement a class Inheritance, which contains a method resolve. The method
takes a description of all the available classes as a String[], a class name as
a String, and a method name called on the class as a String, and returns a
String that is the name of the class that defines the method that should be
executed.
The method signature is:
public String resolve(String[] classes, String className, String methodName);
The String[] contains the descriptions of each of the available classes. Each
element of this String[], describe a single class. The description of a class
is in the form of "CLASSNAME|PARENTNAME|METHOD1,METHOD2...". If the class does
not have a parent (like Object in Java), then PARENTNAME will be of zero
length. The description of the methods is a comma-separated list of method
names. Each method is separated only by a comma (no whitespace).
Given className and methodName, the method returns the name of the class that
defines the method which should be called according to the rules outlined
above. If the method specified by methodName does not exist in the given class
nor any of its ancestors, your method should return the empty string ("").
Constraints:
- The name of the parent class in each class's description must be the name of
some other class
- There cannot be cycles in the inheritance graph (in other words, no class can
be allowed to inherit from any class of which it is an ancestor)
- The names of all classes and methods may only contain letters, digits, and
the underscore character ('_'), and must begin with a letter or underscore
- The name of any class or method may be no less than one character and no more
than 50 characters in length
- There may be at most 50 classes
- Each class may define at most 10 methods
- All method names in the method list for each class must be unique
- The class name passed as the second parameter of your method must be the name
of some class defined in the classes String[]
The tester will ensure that all of the above constraints are met before any
input is passed to your method.
Notes:
- Because of the constraints listed above, there must be at least one class
defined with no ancestor (there may be more than one)
- All string comparisons are case sensitive
Examples:
Given the class description:
["A||a,b,c",
"B|A|b,c,d",
"C||e,f",
"D|C|"
"E|D|f,g"
"F|B|b,h,i"]
-If className="A" and methodName="b" the method returns "A".
-If className="F" and methodName="c" the method returns "B".
-If className="E" and methodName="a" the method returns "".
-If className="D" and methodName="e" the method returns "C".
-If className="F" and methodName="a" the method returns "A".
Given the class description:
["Object||toString,getClass"
"String|Object|toString"
"InputStream|Object|read,close,reset"
"FileInputStream|InputStream|read,close"]
-If className is "String" and methodName is "toString", the method returns
"String".
-If className is "String" and methodName is "getClass", the method returns
"Object".
| | Definition | | Class: | Inheritance | Method: | resolve | Parameters: | String[], String, String | Returns: | String | Method signature: | String resolve(String[] param0, String param1, String param2) | (be sure your method is public) |
| | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | | | Definition | | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
Problem Statement | | | | Definition | | | | |
This problem statement is the exclusive and proprietary property of TopCoder, Inc. Any unauthorized use or reproduction of this information without the prior written consent of TopCoder, Inc. is strictly prohibited. (c)2010, TopCoder, Inc. All rights reserved.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 387 388 389 390 391 392 393 394 395 396 397 398 399 400 401 402 403 404 405 406 407 408 409 410 411 412 413 414 415 416 417 418 419 420 421 422 423 424 425 426 427 428 429 430 431 432 433 434 435 436 437 438 439 440 441 442 443 444 445 446 447 448 449 450 451 452 453 454 455 456 457 458 459 460 461 462 463 464 465 466 467 468 469 470 471 472 473 474 475 476 477 478 479 480 481 482 483 484 485 486 487 488 489 490 491 492 493 494 495 496 497 498 499 500 501 502 503 504 505 506 507 508 509 510 511 512 513 514 515 516 517 518 519 520 521 522 523 524 525 526 527 528 529 530 531 532 533 534 535 536 537 538 539 540 541 542 543 544 545 546 547 548 549 550 551 552 553 554 555 556 557 558 559 560 561 562 563 564 565 566 567 568 569 570 571 572 573 574 575 576 577 578 579 580 581 582 583 584 585 586 587 588 589 590 591 592 593 594 595 596 597 598 599 600 601 602 603 604 605 606 607 608 609 610 611 612 613 614 615 616 617 618 619 620 621 622 623 624 625 626 627 628 629 630 631 632 633 634 635 636 637 638 639 640 641 642 643 644 645 646 647 648 649 650 651 652 653 654 655 656 657 658 659 660 661 662 663 664 665 666 667 668 669 670 671 672 673 674 675 676 677 678 679 680 681 682 683 684 685 686 687 688 689 690 691 692 693 694 695 696 697 698 699 700 701 702 703 704 705 706 707 708 709 710 711 712 713 714 715 716 717 718 719 720 721 722 723 724 725 726 727 728 729 730 731 732 733 734 735 736 737 738 739 740 741 742 743 744 745 746 747 748 749 750 751 752 753 754 755 756 757 758 759 760 761 762 763 764 765 766 767 768 769 770 771 772 773 774 775 776 777 778 779 780 781 782 783 784 785 786 787 788 789 790 791 792 793 794 795 796 797 798 799 800 801 802 803 804 805 806 807 808 809 810 811 812 813 814 815 816 817 818 819 820 821 822 823 824 825 826 827 828 829 830 831 832 833 834 835 836 837 838 839 840 841 842 843 844 845 846 847 848 849 850 851 852 853 854 855 856 857 858 859 860 861 862 863 864 865 866 867 868 869 870 871 872 873 874 875 876 877 878 879 880 881 882 883 884 885 886 887 888 889 890 891 892 893 894 895 896 897 898 899 900 901 902 903 904 905 906 907 908 909 910 911 912 913 914 915 916 917 918 919 920 921 922 923 924 925 926 927 928 929 930 931 932 933 934 935 936 937 938 939 940 941 942 943 944 945 946 947 948 949 950 951 952 953 954 955 956 957 958 959 960 961 962 963 964 965 966 967 968 969 970 971 972 973 974 975 976 977 978 979 980 981 982 983 984 985 986 987 988 989 990 991 992 993 994 995 996 997 998 999 1000 1001 1002 1003 1004 1005 1006 1007 1008 1009 1010 1011 1012 1013 1014 1015 1016 1017 1018 1019 1020 1021 1022 1023 1024 1025 1026 1027 1028 1029 1030 1031 1032 1033 1034 1035 1036 1037 1038 1039 1040 1041 1042 1043 1044 1045 1046 1047 1048 1049 1050 1051 1052 1053 1054 1055 1056 1057 1058 1059 1060 1061 1062 1063 1064 1065 1066 1067 1068 1069 1070 1071 1072 1073 1074 1075 1076 1077 1078 1079 1080 1081 1082 1083 1084 1085 1086 1087 1088 1089 1090 1091 1092 1093 1094 1095 1096 1097 1098 1099 1100 1101 1102 1103 1104 1105 1106 1107 1108 1109 1110 1111 1112 1113 1114 1115 1116 1117 1118 1119 1120 1121 1122 1123 1124 1125 1126 1127 1128 1129 1130 1131 1132 1133 1134 1135 1136 1137 1138 1139 1140 1141 1142 1143 1144 1145 1146 1147 1148 1149 1150 1151 1152 1153 1154 1155 1156 1157 1158 1159 1160 1161 1162 1163 1164 1165 1166 1167 1168 1169 1170 1171 1172 1173 1174 1175 1176 1177 1178 1179 1180 1181 1182 1183 1184 1185 1186 1187 1188 1189 1190 1191 1192 1193 1194 1195 1196 1197 1198 1199 1200 1201 1202 1203 1204 1205 1206 1207 1208 1209 1210 1211 1212 1213 1214 1215 1216 1217 1218 1219 1220 1221 1222 1223 1224 1225 1226 1227 1228 1229 1230 1231 1232 1233 1234 1235 1236 1237 1238 1239 1240 1241 1242 1243 1244 1245 1246 1247 1248 1249 1250 1251 1252 1253 1254 1255 1256 1257 1258 1259 1260 1261 1262 1263 1264 1265 1266 1267 1268 1269 1270 1271 1272 1273 1274 1275 1276 1277 1278 1279 1280 1281 1282 1283 1284 1285 1286 1287 1288 1289 1290 1291 1292 1293 1294 1295 1296 1297 1298 1299 1300 1301 1302 1303 1304 1305 1306 1307 1308 1309 1310 1311 1312 1313 1314 1315 1316 1317 1318 1319 1320 1321 1322 1323 1324 1325 1326 1327 1328 1329 1330 1331 1332 1333 1334 1335 1336 1337 1338 1339 1340 1341 1342 1343 1344 1345 1346 1347 1348 1349 1350 1351 1352 1353 1354 1355 1356 1357 1358 1359 1360 1361 1362 1363 1364 1365 1366 1367 1368 1369 1370 1371 1372 1373 1374 1375 1376 1377 1378 1379 1380 1381 1382 1383 1384 1385 1386 1387 1388 1389 1390 1391 1392 1393 1394 1395 1396 1397 1398 1399 1400 1401 1402 1403 1404 1405 1406 1407 1408 1409 1410 1411 1412 1413 1414 1415 1416 1417 1418 1419 1420 1421 1422 1423 1424 1425 1426 1427 1428 1429 1430 1431 1432 1433 1434 1435 1436 1437 1438 1439 1440 1441 1442 1443 1444 1445 1446 1447 1448 1449 1450 1451 1452 1453 1454 1455 1456 1457 1458 1459 1460 1461 1462 1463 1464 1465 1466 1467 1468 1469 1470 1471 1472 1473 1474 1475 1476 1477 1478 1479 1480 1481 1482 1483 1484 1485 1486 1487 1488 1489 1490 1491 1492 1493 1494 1495 1496 1497 1498 1499 1500 1501 1502 1503 1504 1505 1506 1507 1508 1509 1510 1511 1512 1513 1514 1515 1516 1517 1518 1519 1520 1521 1522 1523 1524 1525 1526 1527 1528 1529 1530 1531 1532 1533 1534 1535 1536 1537 1538 1539 1540 1541 1542 1543 1544 1545 1546 1547 1548 1549 1550 1551 1552 1553 1554 1555 1556 1557 1558 1559 1560 1561 1562 1563 1564 1565 1566 1567 1568 1569 1570 1571 1572 1573 1574 1575 1576 1577 1578 1579 1580 1581 1582 1583 1584 1585 1586 1587 1588 1589 1590 1591 1592 1593 1594 1595 1596 1597 1598 1599 1600 1601 1602 1603 1604 1605 1606 1607 1608 1609 1610 1611 1612 1613 1614 1615 1616 1617 1618 1619 1620 1621 1622 1623 1624 1625 1626 1627 1628 1629 1630 1631 1632 1633 1634 1635 1636 1637 1638 1639 1640 1641 1642 1643 1644 1645 1646 1647 1648 1649 1650 1651 1652 1653 1654 1655 1656 1657 1658 1659 1660 1661 1662 1663 1664 1665 1666 1667 1668 1669 1670 1671 1672 1673 1674 1675 1676 1677 1678 1679 1680 1681 1682 1683 1684 1685 1686 1687 1688 1689 1690 1691 1692 1693 1694 1695 1696 1697 1698 1699 1700 1701 1702 1703 1704 1705 1706 1707 1708 1709 1710 1711 1712 1713 1714 1715 1716 1717 1718 1719 1720 1721 1722 1723 1724 1725 1726 1727 1728 1729 1730 1731 1732 1733 1734 1735 1736 1737 1738 1739 1740 1741 1742 1743 1744 1745 1746 1747 1748 1749 1750 1751 1752 1753 1754 1755 1756 1757 1758 1759 1760 1761 1762 1763 1764 1765 1766 1767 1768 1769 1770 1771 1772 1773 1774 1775 1776 1777 1778 1779 1780 1781 1782 1783 1784 1785 1786 1787 1788 1789 1790 1791 1792 1793 1794 1795 1796 1797 1798 1799 1800 1801 1802 1803 1804 1805 1806 1807 1808 1809 1810 1811 1812 1813 1814 1815 1816 1817 1818 1819 1820 1821 1822 1823 1824 1825 1826 1827 1828 1829 1830 1831 1832 1833 1834 1835 1836 1837 1838 1839 1840 1841 1842 1843 1844 1845 1846 1847 1848 1849 1850 1851 1852 1853 1854 1855 1856 1857 1858 1859 1860 1861 1862 1863 1864 1865 1866 1867 1868 1869 1870 1871 1872 1873 1874 1875 1876 1877 1878 1879 1880 1881 1882 1883 1884 1885 1886 1887 1888 1889 1890 1891 1892 1893 1894 1895 1896 1897 1898 1899 1900 1901 1902 1903 1904 1905 1906 1907 1908 1909 1910 1911 1912 1913 1914 1915 1916 1917 1918 1919 1920 1921 1922 1923 1924 1925 1926 1927 1928 1929 1930 1931 1932 1933 1934 1935 1936 1937 1938 1939 1940 1941 1942 1943 1944 1945 1946 1947 1948 1949 1950 1951 1952 1953 1954 1955 1956 1957 1958 1959 1960 1961 1962 1963 1964 1965 1966 1967 1968 1969 1970 1971 1972 1973 1974 1975 1976 1977 1978 1979 1980 1981 1982 1983 1984 1985 1986 1987 1988 1989 1990 1991 1992 1993 1994 1995 1996 1997 1998 1999 2000 2001 2002 2003 2004 2005 2006 2007 2008 2009 2010 2011 2012 2013 2014 2015 2016 2017 2018 2019 2020 2021 2022 2023 2024 2025 2026 2027 2028 2029 2030 2031 2032 2033 2034 2035 2036 2037 2038 2039 2040 2041 2042 2043 2044 2045 2046 2047 2048 2049 2050 2051 2052 2053 2054 2055 2056 2057 2058 2059 2060 2061 2062 2063 2064 2065 2066 2067 2068 2069 2070 2071 2072 2073 2074 2075 2076 2077 2078 2079 2080 2081 2082 2083 2084 2085 2086 2087 2088 2089 2090 2091 2092 2093 2094 2095 2096 2097 2098 2099 2100 2101 2102 2103 2104 2105 2106 2107 2108 2109 2110 2111 2112 2113 2114 2115 2116 2117 2118 2119 2120 2121 2122 2123 2124 2125 2126 2127 2128 2129 2130 2131 2132 2133 2134 2135 2136 2137 2138 2139 2140 2141 2142 2143 2144 2145 2146 2147 2148 2149 2150 2151 2152 2153 2154 2155 2156 2157 2158 2159 2160 2161 2162 2163 2164 2165 2166 2167 2168 2169 2170 2171 2172 2173 2174 2175 2176 2177 2178 2179 2180 2181 2182 2183 2184 2185 2186 2187 2188 2189 2190 2191 2192 2193 2194 2195 2196 2197 2198 2199 2200 2201 2202 2203 2204 2205 2206 2207 2208 2209 2210 2211 2212 2213 2214 2215 2216 2217 2218 2219 2220 2221 2222 2223 2224 2225 2226 2227 2228 2229 2230 2231 2232 2233 2234 2235 2236 2237 2238 2239 2240 2241 2242 2243 2244 2245 2246 2247 2248 2249 2250 2251 2252 2253 2254 2255 2256 2257 2258 2259 2260 2261 2262 2263 2264 2265 2266 2267 2268 2269 2270 2271 2272 2273 2274 2275 2276 2277 2278 2279 2280 2281 2282 2283 2284 2285 2286 2287 2288 2289 2290 2291 2292 2293 2294 2295 2296 2297 2298 2299 2300 2301 2302 2303 2304 2305 2306 2307 2308 2309 2310 2311 2312 2313 2314 2315 2316 2317 2318 2319 2320 2321 2322 2323 2324 2325 2326 2327 2328 2329 2330 2331 2332 2333 2334 2335 2336 2337 2338 2339 2340 2341 2342 2343 2344 2345 2346 2347 2348 2349 2350 2351 2352 2353 2354 2355 2356 2357 2358 2359 2360 2361 2362 2363 2364 2365 2366 2367 2368 2369 2370 2371 2372 2373 2374 2375 2376 2377 2378 2379 2380 2381 2382 2383 2384 2385 2386 2387 2388 2389 2390 2391 2392 2393 2394 2395 2396 2397 2398 2399 2400 2401 2402 2403 2404 2405 2406 2407 2408 2409 2410 2411 2412 2413 2414 2415 2416 2417 2418 2419 2420 2421 2422 2423 2424 2425 2426 2427 2428 2429 2430 2431 2432 2433 2434 2435 2436 2437 2438 2439 2440 2441 2442 2443 2444 2445 2446 2447 2448 2449 2450 2451 2452 2453 2454 2455 2456 2457 2458 2459 2460 2461 2462 2463 2464 2465 2466 2467 2468 2469 2470 2471 2472 2473 2474 2475 2476 2477 2478 2479 2480 2481 2482 2483 2484 2485 2486 2487 2488 2489 2490 2491 2492 2493 2494 2495 2496 2497 2498 2499 2500 2501 2502 2503 2504 2505 2506 2507 2508 2509 2510 2511 2512 2513 2514 2515 2516 2517 2518 2519 2520 2521 2522 2523 2524 2525 2526 2527 2528 2529 2530 2531 2532 2533 2534 2535 2536 2537 2538 2539 2540 2541 2542 2543 2544 2545 2546 2547 2548 2549 2550 2551 2552 2553 2554 2555 2556 2557 2558 2559 2560 2561 2562 2563 2564 2565 2566 2567 2568 2569 2570 2571 2572 2573 2574 2575 2576 2577 2578 2579 2580 2581 2582 2583 2584 2585 2586 2587 2588 2589 2590 2591 2592 2593 2594 2595 2596 2597 2598 2599 2600 2601 2602 2603 2604 2605 2606 2607 2608 2609 2610 2611 2612 2613 2614 2615 2616 2617 2618 2619 2620 2621 2622 2623 2624 2625 2626 2627 2628 2629 2630 2631 2632 2633 2634 2635 2636 2637 2638 2639 2640 2641 2642 2643 2644 2645 2646 2647 2648 2649 2650 2651 2652 2653 2654 2655 2656 2657 2658 2659 2660 2661 2662 2663 2664 2665 2666 2667 2668 2669 2670 2671 2672 2673 2674 2675 2676 2677 2678 2679 2680 2681 2682 2683 2684 2685 2686 2687 2688 2689 2690 2691 2692 2693 2694 2695 2696 2697 2698 2699 2700 2701 2702 2703 2704 2705 2706 2707 2708 2709 2710 2711 2712 2713 2714 2715 2716 2717 2718 2719 2720 2721 2722 2723 2724 2725 2726 2727 2728 2729 2730 2731 2732 2733 2734 2735 2736 2737 2738 2739 2740 2741 2742 2743 2744 2745 2746 2747 2748 2749 2750 2751 2752 2753 2754 2755 2756 2757 2758 2759 2760 2761 2762 2763 2764 2765 2766 2767 2768 2769 2770 2771 2772 2773 2774 2775 2776 2777 2778 2779 2780 2781 2782 2783 2784 2785 2786 2787 2788 2789 2790 2791 2792 2793 2794 2795 2796 2797 2798 2799 2800 2801 2802 2803 2804 2805 2806 2807 2808 2809 2810 2811 2812 2813 2814 2815 2816 Nikolay.IT 2009-2012
|